
This program is for OV7670 and TFT-LCD(REL225L01)
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "OV7670.h" 00003 #include "REL225L01.h" 00004 REL225L01 tft; 00005 00006 DigitalOut myled1(LED1); 00007 DigitalOut myled2(LED2); 00008 DigitalOut myled3(LED3); 00009 DigitalOut myled4(LED4); 00010 InterruptIn VSYNC_IN(p5); 00011 DigitalOut CMOS_CS(p20); 00012 DigitalOut EN_EXCLK(p21); 00013 DigitalOut EN_595(p23); 00014 I2C i2c(p9,p10); //sda, scl 00015 00016 00017 void flip1() 00018 { 00019 EN_595 = 0; 00020 tft.lcd_cw_start(); 00021 EN_595 = 1; 00022 EN_EXCLK = 1; 00023 CMOS_CS = 0; 00024 } 00025 00026 void flip2() 00027 { 00028 EN_595 = 0; 00029 tft.lcd_cw_end(); 00030 EN_595 = 1; 00031 EN_EXCLK = 0; 00032 CMOS_CS = 1; 00033 } 00034 00035 00036 int main() { 00037 char cmd[2]; 00038 CMOS_CS = 1; 00039 EN_EXCLK=0; 00040 EN_595=0; 00041 myled1=1; 00042 i2c.start(); 00043 // 00044 //cmd[0] = REG_CLKRC; // 00045 //cmd[1] = 0x01; //Set Camera Active 00046 //i2c.write(OV7670_I2C_ADDR,cmd,2); // send string 00047 //wait(0.07); //could also pull,65ms is typical 00048 00049 regval_list *vals=ov7670_default_regs; 00050 //while(vals->reg_num!=0xff||vals->value!=0xff) 00051 while(vals->reg_num!=0xff||vals->value!=0xff) 00052 { 00053 cmd[0] = vals->reg_num; 00054 cmd[1] = vals->value; 00055 i2c.write(OV7670_I2C_ADDR,cmd,2); 00056 wait(0.01); 00057 vals++; 00058 } 00059 00060 vals=ov7670_fmt_rgb565; 00061 //while(vals->reg_num!=0xff||vals->value!=0xff) 00062 while(vals->reg_num!=0xff||vals->value!=0xff) 00063 { 00064 cmd[0] = vals->reg_num; 00065 cmd[1] = vals->value; 00066 i2c.write(OV7670_I2C_ADDR,cmd,2); 00067 wait(0.01); 00068 vals++; 00069 } 00070 00071 wait(2); 00072 00073 unsigned char v; 00074 // Horizontal setting: 11bits, top 8 live in hstart and hstop. Bottom 3 of 00075 // hstart are in href[2:0], bottom 3 of hstop in href[5:3]. There is 00076 //a mystery "edge offset" value in the top two bit fo href. 00077 00078 cmd[0] = REG_HSTART; 00079 cmd[1] = (hstart>>3)&0xff; 00080 i2c.write(OV7670_I2C_ADDR,cmd,2); 00081 wait(0.01); 00082 cmd[0] = REG_HSTOP; 00083 cmd[1] = (hstop >> 3) & 0xff; 00084 i2c.write(OV7670_I2C_ADDR,cmd,2); 00085 //v = (0xb6 & 0xc0) | ((hstop & 0x07)<<3)| (hstart & 0x07); 00086 v = (0x80 & 0xc0) | ((hstop & 0x07)<<3)| (hstart & 0x07); 00087 wait(0.01); 00088 cmd[0] = REG_HREF; 00089 cmd[1] = v; 00090 i2c.write(OV7670_I2C_ADDR,cmd,2); 00091 00092 /* 00093 * Vertical : simillar arrangement, but only 10 bits 00094 */ 00095 cmd[0] = REG_VSTART; 00096 cmd[1] = (vstart>>2)&0xff; 00097 i2c.write(OV7670_I2C_ADDR,cmd,2); 00098 wait(0.01); 00099 cmd[0] = REG_VSTOP; 00100 cmd[1] = (vstop >>2) & 0xff; 00101 i2c.write(OV7670_I2C_ADDR,cmd,2); 00102 v = (0x0a & 0xf0) | ((vstop & 0x03) <<2) | (vstart & 0x03); 00103 cmd[0] = REG_VREF; 00104 cmd[1] = v; 00105 wait(0.01); 00106 i2c.write(OV7670_I2C_ADDR,cmd,2); 00107 cmd[0] = REG_COM7; 00108 cmd[1] = COM7_FMT_QVGA | COM7_RGB; 00109 wait(0.01); 00110 i2c.write(OV7670_I2C_ADDR,cmd,2); 00111 00112 i2c.stop(); 00113 00114 myled1=0; 00115 myled2=1; 00116 // TFT-LCD setting 00117 tft.lcd_init(); 00118 tft.lcd_clear(BLUE); 00119 //wait(1); 00120 //tft.lcd_clear(RED); 00121 //wait(1); 00122 tft.lcd_cw_start(); 00123 //Changing Internal/External WR, Data by VSYNC signal of CMOS Camera module 00124 //LCD module write period : 2sec 00125 EN_595 = 1; 00126 00127 VSYNC_IN.fall(&flip1); 00128 VSYNC_IN.rise(&flip2); 00129 00130 //wait(0.28); 00131 00132 //Stop Re-Display 00133 //tft.lcd_cw_end(); 00134 //VSYNC_IN.fall(&flip2); 00135 00136 myled2=0; 00137 while(1) { 00138 myled3 = 1; 00139 wait(0.2); 00140 myled3 = 0; 00141 wait(0.2); 00142 } 00143 }
Generated on Wed Jul 20 2022 22:37:56 by
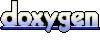