
コネクタ4 : LED コネクタ7 : Buzzer コネクタ13 : Relay コネクタUART:Serial LCD 4つを動かしています
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "SerialLCD.h" 00003 00004 DigitalOut myled(P0_9); 00005 DigitalOut Buzzer(P0_7); 00006 Serial LCD(P0_4, P0_0); //tx, rx 00007 DigitalOut Relay(P0_12); 00008 00009 void ClearDisplay() 00010 { 00011 LCD.putc(SLCD_CONTROL_HEADER); 00012 LCD.putc(SLCD_CLEAR_DISPLAY); 00013 } 00014 00015 void LCDHome() 00016 { 00017 LCD.putc(SLCD_CONTROL_HEADER); 00018 LCD.putc(SLCD_RETURN_HOME); 00019 } 00020 00021 void LCDsetCursor(uint8_t column, uint8_t row) 00022 { 00023 LCD.putc(SLCD_CONTROL_HEADER); 00024 LCD.putc(SLCD_CURSOR_HEADER); 00025 LCD.putc(column); 00026 LCD.putc(row); 00027 } 00028 00029 // Switch the display off without clearing RAM 00030 void LCDnoDisplay() 00031 { 00032 LCD.putc(SLCD_CONTROL_HEADER); 00033 LCD.putc(SLCD_DISPLAY_OFF); 00034 } 00035 00036 // Switch the display on 00037 void LCDdisplay() 00038 { 00039 LCD.putc(SLCD_CONTROL_HEADER); 00040 LCD.putc(SLCD_DISPLAY_ON); 00041 } 00042 00043 // Switch the underline cursor off 00044 void LCDnoCursor() 00045 { 00046 LCD.putc(SLCD_CONTROL_HEADER); 00047 LCD.putc(SLCD_CURSOR_OFF); 00048 } 00049 00050 // Switch the underline cursor on 00051 void LCDcursor() 00052 { 00053 LCD.putc(SLCD_CONTROL_HEADER); 00054 LCD.putc(SLCD_CURSOR_ON); 00055 } 00056 00057 //Turn off the backlight 00058 void LCDnoBacklight(void) 00059 { 00060 LCD.putc(SLCD_CONTROL_HEADER); 00061 LCD.putc(SLCD_BACKLIGHT_OFF); 00062 } 00063 //Turn on the back light 00064 void LCDbacklight(void) 00065 { 00066 LCD.putc(SLCD_CONTROL_HEADER); 00067 LCD.putc(SLCD_BACKLIGHT_ON); 00068 } 00069 00070 void LCDprint(uint8_t b) 00071 { 00072 LCD.putc(SLCD_CHAR_HEADER); 00073 LCD.putc(b); 00074 } 00075 00076 void LCDprintc(const char b[]) 00077 { 00078 LCD.putc(SLCD_CHAR_HEADER); 00079 LCD.printf(b); 00080 } 00081 00082 00083 int main() { 00084 LCD.baud(9600); 00085 //LCD startup 00086 LCD.putc(SLCD_CONTROL_HEADER); 00087 LCD.putc(SLCD_POWER_OFF); 00088 wait_ms(1); 00089 LCD.putc(SLCD_CONTROL_HEADER); 00090 LCD.putc(SLCD_POWER_ON); 00091 wait_ms(1); 00092 LCD.putc(SLCD_INIT_ACK); 00093 while(1) 00094 { 00095 if(LCD.getc()==SLCD_INIT_DONE) 00096 break; 00097 } 00098 wait_ms(2); 00099 00100 //LCD printf 00101 LCDprintc("Hello World"); 00102 00103 //LCD Backlight ON 00104 LCDbacklight(); 00105 00106 //LCD Display ON 00107 LCDdisplay(); 00108 00109 while(1) { 00110 LCDnoBacklight(); 00111 myled = 1; 00112 Buzzer=0; 00113 Relay=1; 00114 wait(0.2); 00115 LCDbacklight(); 00116 myled = 0; 00117 Buzzer=1; 00118 Relay=0; 00119 wait(0.2); 00120 } 00121 }
Generated on Sat Jul 23 2022 08:10:37 by
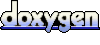