
コネクタ4 : LED コネクタ7 : Buzzer コネクタ13 : Relay コネクタUART:Serial LCD 4つを動かしています
Embed:
(wiki syntax)
Show/hide line numbers
SerialLCD.h
00001 /* 00002 SerialLCD.h - Serial LCD driver Library 00003 2010 Copyright (c) Seeed Technology Inc. All right reserved. 00004 00005 Original Author: Jimbo.We 00006 Contribution: Visweswara R 00007 00008 Modified 15 March,2012 for Arduino 1.0 IDE 00009 by Frankie.Chu 00010 00011 This library is free software; you can redistribute it and/or 00012 modify it under the terms of the GNU Lesser General Public 00013 License as published by the Free Software Foundation; either 00014 version 2.1 of the License, or (at your option) any later version. 00015 00016 This library is distributed in the hope that it will be useful, 00017 but WITHOUT ANY WARRANTY; without even the implied warranty of 00018 MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU 00019 Lesser General Public License for more details. 00020 00021 You should have received a copy of the GNU Lesser General Public 00022 License along with this library; if not, write to the Free Software 00023 Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA 00024 */ 00025 00026 #include "mbed.h" 00027 00028 #ifndef SERIAL_LCD_H 00029 #define SERIAL_LCD_H 00030 00031 00032 //Initialization Commands or Responses 00033 00034 #define SLCD_INIT 0xA3 00035 #define SLCD_INIT_ACK 0xA5 00036 #define SLCD_INIT_DONE 0xAA 00037 00038 //WorkingMode Commands or Responses 00039 #define SLCD_CONTROL_HEADER 0x9F 00040 #define SLCD_CHAR_HEADER 0xFE 00041 #define SLCD_CURSOR_HEADER 0xFF 00042 #define SLCD_CURSOR_ACK 0x5A 00043 00044 #define SLCD_RETURN_HOME 0x61 00045 #define SLCD_DISPLAY_OFF 0x63 00046 #define SLCD_DISPLAY_ON 0x64 00047 #define SLCD_CLEAR_DISPLAY 0x65 00048 #define SLCD_CURSOR_OFF 0x66 00049 #define SLCD_CURSOR_ON 0x67 00050 #define SLCD_BLINK_OFF 0x68 00051 #define SLCD_BLINK_ON 0x69 00052 #define SLCD_SCROLL_LEFT 0x6C 00053 #define SLCD_SCROLL_RIGHT 0x72 00054 #define SLCD_NO_AUTO_SCROLL 0x6A 00055 #define SLCD_AUTO_SCROLL 0x6D 00056 #define SLCD_LEFT_TO_RIGHT 0x70 00057 #define SLCD_RIGHT_TO_LEFT 0x71 00058 #define SLCD_POWER_ON 0x83 00059 #define SLCD_POWER_OFF 0x82 00060 #define SLCD_INVALIDCOMMAND 0x46 00061 #define SLCD_BACKLIGHT_ON 0x81 00062 #define SLCD_BACKLIGHT_OFF 0x80 00063 00064 /* 00065 class SerialLCD : public SoftwareSerial{ 00066 public: 00067 00068 SerialLCD(uint8_t, uint8_t); 00069 void begin(); 00070 void clear(); 00071 void home(); 00072 00073 void noDisplay(); 00074 void display(); 00075 void noBlink(); 00076 void blink(); 00077 void noCursor(); 00078 void cursor(); 00079 void scrollDisplayLeft(); 00080 void scrollDisplayRight(); 00081 void leftToRight(); 00082 void rightToLeft(); 00083 void autoscroll(); 00084 void noAutoscroll(); 00085 00086 void setCursor(uint8_t, uint8_t); 00087 void noPower(void); 00088 void Power(void); 00089 void noBacklight(void); 00090 void backlight(void); 00091 void print(uint8_t b); 00092 void print(const char[]); 00093 void print(unsigned long n, uint8_t base); 00094 00095 }; 00096 */ 00097 #endif
Generated on Sat Jul 23 2022 08:10:37 by
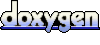