
コネクタ4 : LED コネクタ7 : Buzzer コネクタ13 : Relay コネクタUART:Serial LCD 4つを動かしています
Embed:
(wiki syntax)
Show/hide line numbers
SerialLCD.cpp
00001 /* 00002 SerialLCD.h - Serial LCD driver Library 00003 2010 Copyright (c) Seeed Technology Inc. All right reserved. 00004 00005 Original Author: Jimbo.We 00006 Contribution: Visweswara R 00007 00008 This library is free software; you can redistribute it and/or 00009 modify it under the terms of the GNU Lesser General Public 00010 License as published by the Free Software Foundation; either 00011 version 2.1 of the License, or (at your option) any later version. 00012 00013 This library is distributed in the hope that it will be useful, 00014 but WITHOUT ANY WARRANTY; without even the implied warranty of 00015 MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU 00016 Lesser General Public License for more details. 00017 00018 You should have received a copy of the GNU Lesser General Public 00019 License along with this library; if not, write to the Free Software 00020 Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA 00021 */ 00022 #include "mbed.h" 00023 #include "SerialLCD.h" 00024 /* 00025 SerialLCD::SerialLCD(uint8_t rx, uint8_t tx):SoftwareSerial(rx,tx) 00026 { 00027 00028 } 00029 */ 00030 /********** High level commands, for the user! **********/ 00031 /* 00032 // Initialize the Serial LCD Driver. SerialLCD Module initiates the communication. 00033 void SerialLCD::begin() 00034 { 00035 SoftwareSerial::begin(9600); 00036 delay(2); 00037 SoftwareSerial::write(SLCD_CONTROL_HEADER); 00038 SoftwareSerial::write(SLCD_POWER_OFF); 00039 delay(1); 00040 SoftwareSerial::write(SLCD_CONTROL_HEADER); 00041 SoftwareSerial::write(SLCD_POWER_ON); 00042 delay(1); 00043 SoftwareSerial::write(SLCD_INIT_ACK); 00044 while(1) 00045 { 00046 if (SoftwareSerial::available() > 0 &&SoftwareSerial::read()==SLCD_INIT_DONE) 00047 break; 00048 } 00049 delay(2); 00050 } 00051 00052 // Clear the display 00053 void SerialLCD::clear() 00054 { 00055 SoftwareSerial::write(SLCD_CONTROL_HEADER); 00056 SoftwareSerial::write(SLCD_CLEAR_DISPLAY); 00057 } 00058 00059 // Return to home(top-left corner of LCD) 00060 void SerialLCD::home() 00061 { 00062 SoftwareSerial::write(SLCD_CONTROL_HEADER); 00063 SoftwareSerial::write(SLCD_RETURN_HOME); 00064 delay(2);//this command needs more time; 00065 } 00066 00067 // Set Cursor to (Column,Row) Position 00068 void SerialLCD::setCursor(uint8_t column, uint8_t row) 00069 { 00070 SoftwareSerial::write(SLCD_CONTROL_HEADER); 00071 SoftwareSerial::write(SLCD_CURSOR_HEADER); //cursor header command 00072 SoftwareSerial::write(column); 00073 SoftwareSerial::write(row); 00074 } 00075 00076 // Switch the display off without clearing RAM 00077 void SerialLCD::noDisplay() 00078 { 00079 SoftwareSerial::write(SLCD_CONTROL_HEADER); 00080 SoftwareSerial::write(SLCD_DISPLAY_OFF); 00081 } 00082 00083 // Switch the display on 00084 void SerialLCD::display() 00085 { 00086 SoftwareSerial::write(SLCD_CONTROL_HEADER); 00087 SoftwareSerial::write(SLCD_DISPLAY_ON); 00088 } 00089 00090 // Switch the underline cursor off 00091 void SerialLCD::noCursor() 00092 { 00093 SoftwareSerial::write(SLCD_CONTROL_HEADER); 00094 SoftwareSerial::write(SLCD_CURSOR_OFF); 00095 } 00096 00097 // Switch the underline cursor on 00098 void SerialLCD::cursor() 00099 { 00100 SoftwareSerial::write(SLCD_CONTROL_HEADER); 00101 SoftwareSerial::write(SLCD_CURSOR_ON); 00102 } 00103 00104 // Switch off the blinking cursor 00105 void SerialLCD::noBlink() 00106 { 00107 SoftwareSerial::write(SLCD_CONTROL_HEADER); 00108 SoftwareSerial::write(SLCD_BLINK_OFF); 00109 } 00110 00111 // Switch on the blinking cursor 00112 void SerialLCD::blink() 00113 { 00114 SoftwareSerial::write(SLCD_CONTROL_HEADER); 00115 SoftwareSerial::write(SLCD_BLINK_ON); 00116 } 00117 00118 // Scroll the display left without changing the RAM 00119 void SerialLCD::scrollDisplayLeft(void) 00120 { 00121 SoftwareSerial::write(SLCD_CONTROL_HEADER); 00122 SoftwareSerial::write(SLCD_SCROLL_LEFT); 00123 } 00124 00125 // Scroll the display right without changing the RAM 00126 void SerialLCD::scrollDisplayRight(void) 00127 { 00128 SoftwareSerial::write(SLCD_CONTROL_HEADER); 00129 SoftwareSerial::write(SLCD_SCROLL_RIGHT); 00130 } 00131 00132 // Set the text flow "Left to Right" 00133 void SerialLCD::leftToRight(void) 00134 { 00135 SoftwareSerial::write(SLCD_CONTROL_HEADER); 00136 SoftwareSerial::write(SLCD_LEFT_TO_RIGHT); 00137 } 00138 00139 // Set the text flow "Right to Left" 00140 void SerialLCD::rightToLeft(void) 00141 { 00142 SoftwareSerial::write(SLCD_CONTROL_HEADER); 00143 SoftwareSerial::write(SLCD_RIGHT_TO_LEFT); 00144 } 00145 00146 // This will 'right justify' text from the cursor 00147 void SerialLCD::autoscroll(void) 00148 { 00149 SoftwareSerial::write(SLCD_CONTROL_HEADER); 00150 SoftwareSerial::write(SLCD_AUTO_SCROLL); 00151 } 00152 00153 // This will 'left justify' text from the cursor 00154 void SerialLCD::noAutoscroll(void) 00155 { 00156 SoftwareSerial::write(SLCD_CONTROL_HEADER); 00157 SoftwareSerial::write(SLCD_NO_AUTO_SCROLL); 00158 } 00159 void SerialLCD::Power(void) 00160 { 00161 SoftwareSerial::write(SLCD_CONTROL_HEADER); 00162 SoftwareSerial::write(SLCD_POWER_ON); 00163 } 00164 void SerialLCD::noPower(void) 00165 { 00166 SoftwareSerial::write(SLCD_CONTROL_HEADER); 00167 SoftwareSerial::write(SLCD_POWER_OFF); 00168 } 00169 //Turn off the backlight 00170 void SerialLCD::noBacklight(void) 00171 { 00172 SoftwareSerial::write(SLCD_CONTROL_HEADER); 00173 SoftwareSerial::write(SLCD_BACKLIGHT_OFF); 00174 } 00175 //Turn on the back light 00176 void SerialLCD::backlight(void) 00177 { 00178 SoftwareSerial::write(SLCD_CONTROL_HEADER); 00179 SoftwareSerial::write(SLCD_BACKLIGHT_ON); 00180 } 00181 // Print Commands 00182 00183 void SerialLCD::print(uint8_t b) 00184 { 00185 SoftwareSerial::write(SLCD_CHAR_HEADER); 00186 SoftwareSerial::write(b); 00187 } 00188 void SerialLCD::print(const char b[]) 00189 { 00190 SoftwareSerial::write(SLCD_CHAR_HEADER); 00191 SoftwareSerial::write(b); 00192 } 00193 00194 void SerialLCD::print(unsigned long n, uint8_t base) 00195 { 00196 unsigned char buf[8 * sizeof(long)]; // Assumes 8-bit chars. 00197 unsigned long i = 0; 00198 00199 if (base == 0) print(n); 00200 00201 else if(base!=0) 00202 { 00203 if (n == 0) { 00204 print('0'); 00205 return; 00206 } 00207 00208 while (n > 0) { 00209 buf[i++] = n % base; 00210 n /= base; 00211 } 00212 00213 for (; i > 0; i--) 00214 print((char) (buf[i - 1] < 10 ? 00215 '0' + buf[i - 1] : 00216 'A' + buf[i - 1] - 10)); 00217 } 00218 } 00219 00220 */
Generated on Sat Jul 23 2022 08:10:37 by
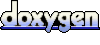