
working version with calibration done
Fork of Eurobot2013 by
Embed:
(wiki syntax)
Show/hide line numbers
system.h
00001 00002 #ifndef SYSTEM_H 00003 #define SYSTEM_H 00004 00005 #include "globals.h" 00006 #include "rtos.h" 00007 00008 //Declaring the onboard LED's for everyone to use 00009 extern DigitalOut OLED1;//(LED1); 00010 extern DigitalOut OLED2;//(LED2); 00011 extern DigitalOut OLED3;//(LED3); 00012 extern DigitalOut OLED4;//(LED4); 00013 00014 //nop style wait function 00015 void nopwait(int ms); 00016 00017 //a type which is a pointer to a rtos thread function 00018 typedef void (*tfuncptr_t)(void const *argument); 00019 00020 //--------------------- 00021 //Signal ticker stuff 00022 #define SIGTICKARGS(thread, signal) \ 00023 (tfuncptr_t) (&Signalsetter::callback), osTimerPeriodic, (void*)(new Signalsetter(thread, signal)) 00024 00025 class Signalsetter { 00026 public: 00027 Signalsetter(Thread& inthread, int insignal) : 00028 thread(inthread) { 00029 signal = insignal; 00030 //pc.printf("ptr saved as %#x \r\n", (int)(&(inthread))); 00031 } 00032 00033 static void callback(void* thisin) { 00034 00035 Signalsetter* fthis = (Signalsetter*)thisin; 00036 //pc.printf("callback will signal thread object at %#x \r\n", (int)(&(fthis->thread))); 00037 fthis->thread.signal_set(fthis->signal); 00038 //delete fthis; //this is useful for single fire tickers! 00039 } 00040 00041 private: 00042 Thread& thread; 00043 int signal; 00044 }; 00045 00046 //--------------------- 00047 //cpu usage measurement function 00048 extern float cpupercent; 00049 void measureCPUidle (void const* arg); 00050 00051 #endif
Generated on Tue Jul 12 2022 18:53:26 by
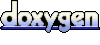