
working version with calibration done
Fork of Eurobot2013 by
Embed:
(wiki syntax)
Show/hide line numbers
RFSRF05.h
00001 #ifndef MBED_RFSRF05_H 00002 #define MBED_RFSRF05_H 00003 00004 00005 00006 #include "mbed.h" 00007 #include "RF12B.h" 00008 #include "globals.h" 00009 00010 00011 #define CODE0 0x22 00012 #define CODE1 0x44 00013 #define CODE2 0x88 00014 00015 /* SAMPLE IMPLEMENTATION! 00016 RFSRF05 my_srf(p13,p21,p22,p23,p24,p25,p26,p5,p6,p7,p8,p9); 00017 00018 00019 void callbinmain(int num, float dist) { 00020 //Here is where you deal with your brand new reading ;D 00021 } 00022 00023 int main() { 00024 pc.printf("Hello World of RobotSonar!\r\n"); 00025 my_srf.callbackfunc = callbinmain; 00026 00027 while (1); 00028 } 00029 00030 */ 00031 00032 class DummyCT; 00033 00034 class RFSRF05 { 00035 public: 00036 00037 RFSRF05( 00038 PinName trigger, 00039 PinName echo0, 00040 PinName echo1, 00041 PinName echo2, 00042 PinName echo3, 00043 PinName echo4, 00044 PinName echo5, 00045 PinName SDI, 00046 PinName SDO, 00047 PinName SCK, 00048 PinName NCS, 00049 PinName NIRQ); 00050 00051 /** A non-blocking function that will return the last measurement 00052 * 00053 * @returns floating point representation of distance in mm 00054 */ 00055 float read0(); 00056 float read1(); 00057 float read2(); 00058 float read(unsigned int beaconnum); 00059 00060 00061 /** A assigns a callback function when a new reading is available **/ 00062 void (*callbackfunc)(int beaconnum, float distance, float variance); 00063 DummyCT* callbackobj; 00064 void (DummyCT::*mcallbackfunc)(int beaconnum, float distance, float variance); 00065 00066 //triggers a read 00067 #ifndef ROBOT_PRIMARY 00068 void startRange(unsigned char rx_code); 00069 #endif 00070 00071 //set codes 00072 void setCode(int code_index, unsigned char code); 00073 unsigned char codes[3]; 00074 00075 /** A short hand way of using the read function */ 00076 //operator float(); 00077 00078 private : 00079 RF12B _rf; 00080 DigitalOut _trigger; 00081 InterruptIn _echo0; 00082 InterruptIn _echo1; 00083 InterruptIn _echo2; 00084 InterruptIn _echo3; 00085 InterruptIn _echo4; 00086 InterruptIn _echo5; 00087 Timer _timer; 00088 Ticker _ticker; 00089 #ifdef ROBOT_PRIMARY 00090 void _startRange(void); 00091 #endif 00092 void _rising (void); 00093 void _falling (void); 00094 float _dist[3]; 00095 int _beacon_counter; 00096 bool ValidPulse; 00097 bool expValidPulse; 00098 00099 }; 00100 00101 #endif
Generated on Tue Jul 12 2022 18:53:26 by
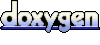