
working version with calibration done
Fork of Eurobot2013 by
Embed:
(wiki syntax)
Show/hide line numbers
RF12B.h
00001 #ifndef _RF12B_H 00002 #define _RF12B_H 00003 00004 #include "mbed.h" 00005 //#include <queue> 00006 00007 enum rfmode_t{RX, TX}; 00008 00009 class DummyCT; 00010 00011 class RF12B { 00012 public: 00013 /* Constructor */ 00014 RF12B(PinName SDI, 00015 PinName SDO, 00016 PinName SCK, 00017 PinName NCS, 00018 PinName NIRQ); 00019 00020 00021 00022 /* Reads a packet of data. Returns false if read failed. Use available() to check how much space to allocate for buffer */ 00023 bool read(unsigned char* data, unsigned int size); 00024 00025 /* Reads a byte of data from the receive buffer 00026 Returns 0xFF if there is no data */ 00027 unsigned char read(); 00028 00029 /* Transmits a packet of data */ 00030 void write(unsigned char* data, unsigned char length); 00031 void write(unsigned char data); /* 1-byte packet */ 00032 // void write(std::queue<char> &data, int length = -1); /* sends a whole queue */ 00033 00034 /* Returns the packet length if data is available in the receive buffer, 0 otherwise*/ 00035 unsigned int available(); 00036 00037 /** A assigns a callback function when a new reading is available **/ 00038 void (*callbackfunc)(unsigned char rx_code); 00039 DummyCT* callbackobj; 00040 void (DummyCT::*mcallbackfunc)(unsigned char rx_code); 00041 00042 protected: 00043 /* Receive FIFO buffer */ 00044 // std::queue<unsigned char> fifo; 00045 // std::queue<unsigned char> temp; //for storing stuff mid-packet 00046 00047 /* SPI module */ 00048 SPI spi; 00049 00050 /* Other digital pins */ 00051 DigitalOut NCS; 00052 InterruptIn NIRQ; 00053 DigitalIn NIRQ_in; 00054 //DigitalOut rfled; 00055 00056 rfmode_t mode; 00057 00058 /* Initialises the RF12B module */ 00059 void init(); 00060 00061 /* Write a command to the RF Module */ 00062 unsigned int writeCmd(unsigned int cmd); 00063 00064 /* Sends a byte of data across RF */ 00065 void send(unsigned char data); 00066 00067 /* Switch module between receive and transmit modes */ 00068 void changeMode(rfmode_t mode); 00069 00070 /* Interrupt routine for data reception */ 00071 void rxISR(); 00072 00073 /* Tell the RF Module this packet is received and wait for the next */ 00074 void resetRX(); 00075 00076 /* Return the RF Module Status word */ 00077 unsigned int status(); 00078 00079 /* Calculate CRC8 */ 00080 unsigned char crc8(unsigned char crc, unsigned char data); 00081 }; 00082 00083 #endif /* _RF12B_H */
Generated on Tue Jul 12 2022 18:53:25 by
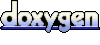