
working version with calibration done
Fork of Eurobot2013 by
Embed:
(wiki syntax)
Show/hide line numbers
IR_Turret.h
00001 #ifndef MBED_IR_TURRET_H 00002 #define MBED_IR_TURRET_H 00003 00004 00005 00006 #include "mbed.h" 00007 #include "PwmIn.h" 00008 #include "globals.h" 00009 #include "geometryfuncs.h" 00010 00011 // Stepper motor defines go here, p30 is reserved for the hardware counter 00012 #define STEPPER_PERIOD 0.0001 //Stepper motor period in seconds, 50% duty cycle 00013 #define STEPPER_DIV 3200 // number of steps per cycle = default * microstep 00014 #define STEP_ANGLE ((float)(2*PI) / STEPPER_DIV) // step angle of stepper 00015 #define IR_TIMEOUT_STEP 45 //about 5 degrees 00016 #define IR_TIMEOUT (STEPPER_PERIOD*IR_TIMEOUT_STEP) 00017 00018 00019 // IR Sensor defines go here 00020 #define PWM_INVERT // inverts the PWM for the IR sensor pwm input 00021 #define PULSEPERIOD_US 1500 00022 #define PULSEPERIOD ((float)1500/1000000) 00023 #define IR0_PULSEWIDTH 1000 00024 #define IR1_PULSEWIDTH 750 00025 #define IR2_PULSEWIDTH 500 00026 #define PULSEWIDTH_TOLERANCE 125 00027 #define STEPS_PER_PULSEPERIOD (PULSEPERIOD/STEPPER_PERIOD) 00028 00029 /* SAMPLE IMPLEMENTATION! 00030 IR_TURRET my_ir_turret(stepper_pin,ir_pin); 00031 00032 00033 void callbinmain(int num, float dist) { 00034 //Here is where you deal with your brand new reading ;D 00035 } 00036 00037 int main() { 00038 pc.printf("Hello World of RobotSonar!\r\n"); 00039 my_srf.callbackfunc = callbinmain; 00040 00041 while (1); 00042 } 00043 00044 */ 00045 00046 class DummyCT; 00047 00048 class IR_TURRET { 00049 public: 00050 00051 IR_TURRET( 00052 PinName step_pin, 00053 PinName ir_pin); 00054 00055 00056 /** A assigns a callback function when a new reading is available **/ 00057 void (*callbackfunc)(int beaconnum, float angle, float variance); 00058 DummyCT* callbackobj; 00059 void (DummyCT::*mcallbackfunc)(int beaconnum, float angle, float variance); 00060 00061 00062 00063 private : 00064 PwmOut _step; 00065 PwmIn _ir; 00066 Timeout _ir_timeout; 00067 void _ir_timeout_isr (void); 00068 void _ir_isr (float pulsewidth); 00069 00070 int step_count_old; 00071 int IR_counter; 00072 int IR_step_counter; 00073 float pulsewidth_max; 00074 float _angle[3]; 00075 float _variance[3]; 00076 00077 00078 00079 }; 00080 00081 #endif
Generated on Tue Jul 12 2022 18:53:25 by
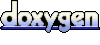