-
Embed:
(wiki syntax)
Show/hide line numbers
PCA9555.cpp
00001 #include <PCA9555.h> 00002 00003 //#include <mbed.h> 00004 00005 //******************************************************************************// 00006 // uiAdr: user address [A2...A0] 00007 //******************************************************************************// 00008 PCA9555::PCA9555(I2C *_i2c, uint8_t uiAdr) // 00009 : PCA9555_W( HARD_ADR | (uiAdr & USER_ADR_MASK) << 1),// Initialisation of const WRITE 00010 PCA9555_R( PCA9555_W | 0x01 ){ // Initialisation of const READ 00011 00012 i2c = _i2c; // 00013 bAck = false; // 00014 } 00015 00016 00017 00018 //******************************************************************************// 00019 // If a bit in this register is set (written with ‘1’), the corresponding port 00020 // pin is enabled as an input with high-impedance output driver. If a bit in 00021 // this register is cleared (written with ‘0’), the corresponding port pin is 00022 // enabled as an output. 00023 //******************************************************************************// 00024 bool PCA9555::confDirGPIO(_GPIO gpio, uint8_t uiData0, uint8_t uiData1){ 00025 00026 if (gpio == BOTH){ // both GPIO ports 00027 cCmd[0] = PORT0_CONFIG; // start with GPIO Port0 00028 cCmd[1] = uiData0; // uiData0 for GPIO Port 0 00029 cCmd[2] = uiData1; // uiData1 for GPIO Port 1 00030 00031 bAck = i2c->write(PCA9555_W, cCmd, 3); // send via I2C 00032 00033 } else { // only 00034 if (gpio == PORT0) cCmd[0] = PORT0_CONFIG; // Port0 00035 else cCmd[0] = PORT1_CONFIG; // Port1 00036 00037 cCmd[1] = uiData0; // uiData 00038 bAck = i2c->write(PCA9555_W, cCmd, 2); // send via I2C 00039 } 00040 00041 return bAck; // return (N)ACK 00042 } 00043 00044 00045 //******************************************************************************// 00046 // 00047 //******************************************************************************// 00048 bool PCA9555::getGPIO(_GPIO gpio){ 00049 00050 if (gpio == BOTH){ // both GPIO ports 00051 cCmd[0] = PORT0_INPUT; // start with GPIO Port0 00052 00053 bAck = i2c->write(PCA9555_W, cCmd, 1); // send via I2C 00054 bAck = i2c->read(PCA9555_R, cCmd, 2); // send via I2C 00055 GPIO0.uiPort = cCmd[0]; 00056 GPIO1.uiPort = cCmd[1]; 00057 00058 } else { // only 00059 if (gpio == PORT0) cCmd[0] = PORT0_INPUT; // Port0 00060 else cCmd[0] = PORT1_INPUT; // Port1 00061 00062 bAck = i2c->write(PCA9555_W, cCmd, 1); // send via I2C 00063 bAck = i2c->read(PCA9555_W, cCmd, 1); // send via I2C 00064 00065 if (gpio == PORT0) GPIO0.uiPort = cCmd[0]; // Port0 00066 else GPIO1.uiPort = cCmd[0]; // Port0 00067 } 00068 00069 return bAck; // return (N)ACK 00070 } 00071 00072 00073 00074 //******************************************************************************// 00075 // 00076 //******************************************************************************// 00077 uint8_t PCA9555::getGPIO0(bool bUpdate){ 00078 00079 if(bUpdate) getGPIO(BOTH); 00080 00081 return GPIO0.uiPort; 00082 } 00083 00084 00085 00086 //******************************************************************************// 00087 // 00088 //******************************************************************************// 00089 bool PCA9555::getGPIO0_B0(bool bUpdate){ 00090 00091 if(bUpdate) getGPIO(BOTH); 00092 00093 return (bool) GPIO0.Pin.B0; 00094 } 00095 00096 00097 00098 //******************************************************************************// 00099 // 00100 //******************************************************************************// 00101 bool PCA9555::getGPIO0_B1(bool bUpdate){ 00102 00103 if(bUpdate) getGPIO(BOTH); 00104 00105 return GPIO0.Pin.B1; 00106 00107 } 00108 00109 00110 00111 //******************************************************************************// 00112 // 00113 //******************************************************************************// 00114 bool PCA9555::getGPIO0_B2(bool bUpdate){ 00115 00116 if(bUpdate) getGPIO(BOTH); 00117 00118 return GPIO0.Pin.B2; 00119 } 00120 00121 00122 00123 //******************************************************************************// 00124 // 00125 //******************************************************************************// 00126 bool PCA9555::getGPIO0_B3(bool bUpdate){ 00127 00128 if(bUpdate) getGPIO(BOTH); 00129 00130 return GPIO0.Pin.B3; 00131 00132 } 00133 00134 00135 00136 //******************************************************************************// 00137 // 00138 //******************************************************************************// 00139 bool PCA9555::getGPIO0_B4(bool bUpdate){ 00140 00141 if(bUpdate) getGPIO(BOTH); 00142 00143 return GPIO0.Pin.B4; 00144 } 00145 00146 00147 00148 //******************************************************************************// 00149 // 00150 //******************************************************************************// 00151 bool PCA9555::getGPIO0_B5(bool bUpdate){ 00152 00153 if(bUpdate) getGPIO(BOTH); 00154 00155 return GPIO0.Pin.B5; 00156 00157 } 00158 00159 00160 00161 //******************************************************************************// 00162 // 00163 //******************************************************************************// 00164 bool PCA9555::getGPIO0_B6(bool bUpdate){ 00165 00166 if(bUpdate) getGPIO(BOTH); 00167 00168 return GPIO0.Pin.B6; 00169 } 00170 00171 00172 00173 //******************************************************************************// 00174 // 00175 //******************************************************************************// 00176 bool PCA9555::getGPIO0_B7(bool bUpdate){ 00177 00178 if(bUpdate) getGPIO(BOTH); 00179 00180 return GPIO0.Pin.B7; 00181 00182 } 00183 00184 00185 //******************************************************************************// 00186 // 00187 //******************************************************************************// 00188 uint8_t PCA9555::getGPIO1(bool bUpdate){ 00189 00190 if(bUpdate) getGPIO(BOTH); 00191 00192 return GPIO1.uiPort; 00193 } 00194 00195 00196 00197 //******************************************************************************// 00198 // 00199 //******************************************************************************// 00200 bool PCA9555::getGPIO1_B0(bool bUpdate){ 00201 00202 if(bUpdate) getGPIO(BOTH); 00203 00204 return GPIO1.Pin.B0; 00205 } 00206 00207 00208 00209 //******************************************************************************// 00210 // 00211 //******************************************************************************// 00212 bool PCA9555::getGPIO1_B1(bool bUpdate){ 00213 00214 if(bUpdate) getGPIO(BOTH); 00215 00216 return GPIO1.Pin.B1; 00217 00218 } 00219 00220 00221 00222 //******************************************************************************// 00223 // 00224 //******************************************************************************// 00225 bool PCA9555::getGPIO1_B2(bool bUpdate){ 00226 00227 if(bUpdate) getGPIO(BOTH); 00228 00229 return GPIO1.Pin.B2; 00230 } 00231 00232 00233 00234 //******************************************************************************// 00235 // 00236 //******************************************************************************// 00237 bool PCA9555::getGPIO1_B3(bool bUpdate){ 00238 00239 if(bUpdate) getGPIO(BOTH); 00240 00241 return GPIO1.Pin.B3; 00242 00243 } 00244 00245 00246 00247 //******************************************************************************// 00248 // 00249 //******************************************************************************// 00250 bool PCA9555::getGPIO1_B4(bool bUpdate){ 00251 00252 if(bUpdate) getGPIO(BOTH); 00253 00254 return GPIO1.Pin.B4; 00255 } 00256 00257 00258 00259 //******************************************************************************// 00260 // 00261 //******************************************************************************// 00262 bool PCA9555::getGPIO1_B5(bool bUpdate){ 00263 00264 if(bUpdate) getGPIO(BOTH); 00265 00266 return GPIO1.Pin.B5; 00267 00268 } 00269 00270 00271 00272 //******************************************************************************// 00273 // 00274 //******************************************************************************// 00275 bool PCA9555::getGPIO1_B6(bool bUpdate){ 00276 00277 if(bUpdate) getGPIO(BOTH); 00278 00279 return GPIO1.Pin.B6; 00280 } 00281 00282 00283 00284 //******************************************************************************// 00285 // 00286 //******************************************************************************// 00287 bool PCA9555::getGPIO1_B7(bool bUpdate){ 00288 00289 if(bUpdate) getGPIO(BOTH); 00290 00291 return GPIO1.Pin.B7; 00292 00293 } 00294 00295 00296 00297 //******************************************************************************// 00298 // 00299 //******************************************************************************// 00300 bool PCA9555::setGPIO(_GPIO gpio, uint8_t uiData0, uint8_t uiData1) 00301 { 00302 if(gpio == BOTH){ // both GPIO ports 00303 cCmd[0] = PORT0_OUTPUT; // start with GPIO Port0 00304 cCmd[1] = uiData0; // uiData0 for GPIO Port 0 00305 cCmd[2] = uiData1; // uiData1 for GPIO Port 1 00306 00307 bAck = i2c->write(PCA9555_W, cCmd, 3); // send via I2C 00308 00309 } else { // only 00310 if (gpio == PORT0) cCmd[0] = PORT0_OUTPUT; // Port0 00311 else cCmd[0] = PORT1_OUTPUT; // Port1 00312 00313 cCmd[1] = uiData0; // uiData 00314 bAck = i2c->write(PCA9555_W, cCmd, 2); // send via I2C 00315 } 00316 00317 return bAck; // return (N)ACK 00318 } 00319 00320 00321 //******************************************************************************// 00322 // 00323 //******************************************************************************// 00324 bool PCA9555::setGPIO0_B0(bool bBit){ 00325 00326 GPIO0.Pin.B0 = bBit; 00327 00328 return setGPIO(PORT0, GPIO0.uiPort); 00329 } 00330 00331 00332 00333 00334 //******************************************************************************// 00335 // 00336 //******************************************************************************// 00337 bool PCA9555::setGPIO0_B1(bool bBit){ 00338 00339 GPIO0.Pin.B1 = bBit; 00340 00341 return setGPIO(PORT0, GPIO0.uiPort); 00342 } 00343 00344 00345 00346 //******************************************************************************// 00347 // 00348 //******************************************************************************// 00349 bool PCA9555::setGPIO0_B2(bool bBit){ 00350 00351 GPIO0.Pin.B2 = bBit; 00352 00353 return setGPIO(PORT0, GPIO0.uiPort); 00354 } 00355 00356 00357 00358 //******************************************************************************// 00359 // 00360 //******************************************************************************// 00361 bool PCA9555::setGPIO0_B4(bool bBit){ 00362 00363 GPIO0.Pin.B4 = bBit; 00364 00365 return setGPIO(PORT0, GPIO0.uiPort); 00366 } 00367 00368 00369 00370 //******************************************************************************// 00371 // 00372 //******************************************************************************// 00373 bool PCA9555::setGPIO0_B5(bool bBit){ 00374 00375 GPIO0.Pin.B5 = bBit; 00376 00377 return setGPIO(PORT0, GPIO0.uiPort); 00378 } 00379 00380 00381 00382 //******************************************************************************// 00383 // 00384 //******************************************************************************// 00385 bool PCA9555::setGPIO0_B6(bool bBit){ 00386 00387 GPIO0.Pin.B6 = bBit; 00388 00389 return setGPIO(PORT0, GPIO0.uiPort); 00390 } 00391 00392 00393 00394 //******************************************************************************// 00395 // 00396 //******************************************************************************// 00397 bool PCA9555::setGPIO0_B7(bool bBit){ 00398 00399 GPIO0.Pin.B7 = bBit; 00400 00401 return setGPIO(PORT0, GPIO0.uiPort); 00402 } 00403 00404 00405 00406 //******************************************************************************// 00407 // 00408 //******************************************************************************// 00409 bool PCA9555::setGPIO1_B0(bool bBit){ 00410 00411 GPIO1.Pin.B0 = bBit; 00412 00413 return setGPIO(PORT1, GPIO1.uiPort); 00414 } 00415 00416 00417 00418 00419 //******************************************************************************// 00420 // 00421 //******************************************************************************// 00422 bool PCA9555::setGPIO1_B1(bool bBit){ 00423 00424 GPIO1.Pin.B1 = bBit; 00425 00426 return setGPIO(PORT1, GPIO1.uiPort); 00427 } 00428 00429 00430 00431 //******************************************************************************// 00432 // 00433 //******************************************************************************// 00434 bool PCA9555::setGPIO1_B2(bool bBit){ 00435 00436 GPIO1.Pin.B2 = bBit; 00437 00438 return setGPIO(PORT1, GPIO1.uiPort); 00439 } 00440 00441 00442 00443 //******************************************************************************// 00444 // 00445 //******************************************************************************// 00446 bool PCA9555::setGPIO1_B4(bool bBit){ 00447 00448 GPIO1.Pin.B4 = bBit; 00449 00450 return setGPIO(PORT1, GPIO1.uiPort); 00451 } 00452 00453 00454 00455 //******************************************************************************// 00456 // 00457 //******************************************************************************// 00458 bool PCA9555::setGPIO1_B5(bool bBit){ 00459 00460 GPIO1.Pin.B5 = bBit; 00461 00462 return setGPIO(PORT1, GPIO1.uiPort); 00463 } 00464 00465 00466 00467 //******************************************************************************// 00468 // 00469 //******************************************************************************// 00470 bool PCA9555::setGPIO1_B6(bool bBit){ 00471 00472 GPIO1.Pin.B6 = bBit; 00473 00474 return setGPIO(PORT1, GPIO1.uiPort); 00475 } 00476 00477 00478 00479 //******************************************************************************// 00480 // 00481 //******************************************************************************// 00482 bool PCA9555::setGPIO1_B7(bool bBit){ 00483 00484 GPIO1.Pin.B7 = bBit; 00485 00486 return setGPIO(PORT1, GPIO1.uiPort); 00487 } 00488
Generated on Sun Jul 17 2022 11:47:04 by
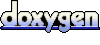