
test
Dependencies: 4DGL-uLCD-SE mbed
main.cpp
00001 // Demo for the uLCD-144-G2 based on the work by Jim Hamblen 00002 00003 #include "mbed.h" 00004 #include "uLCD_4DGL.h" 00005 00006 // TX, RX, and RES pins 00007 uLCD_4DGL uLCD(p9,p10,p11); 00008 00009 int main() { 00010 00011 int x; 00012 int y; 00013 int radius; 00014 int vx; 00015 00016 // Test comment 00017 // Set our UART baudrate to something reasonable 00018 uLCD.baudrate(115200); 00019 00020 // Change background color (must be called before cls) 00021 uLCD.background_color(WHITE); 00022 00023 // Clear screen with background color 00024 uLCD.cls(); 00025 00026 // Change background color of text 00027 uLCD.textbackground_color(WHITE); 00028 00029 // Make some colorful text 00030 uLCD.locate(4, 1); // Move cursor 00031 uLCD.color(BLUE); 00032 uLCD.printf("This is a\n"); 00033 uLCD.locate(5, 3); // Move cursor 00034 uLCD.text_width(2); // 2x normal size 00035 uLCD.text_height(2); // 2x normal size 00036 uLCD.color(RED); // Change text color 00037 uLCD.printf("TEST"); 00038 uLCD.text_width(1); // Normal size 00039 uLCD.text_height(1); // Normal size 00040 uLCD.locate(3, 6); // Move cursor 00041 uLCD.color(BLACK); // Change text color 00042 uLCD.printf("of my new LCD"); 00043 00044 // Initial parameters for the circle 00045 x = 50; 00046 y = 100; 00047 radius = 4; 00048 vx = 1; 00049 00050 // Make a ball bounce back and forth 00051 while (1) { 00052 00053 // Draw a dark green 00054 uLCD.filled_circle(x, y, radius, 0x008000); 00055 00056 // Bounce off the edges 00057 if ((x <= radius + 1) || (x >= 126 - radius)) { 00058 vx = -1 * vx; 00059 } 00060 00061 // Wait before erasing old circle 00062 wait(0.02); // In seconds 00063 00064 // Erase old circle 00065 uLCD.filled_circle(x, y, radius, WHITE); 00066 00067 // Move circle 00068 x = x + vx; 00069 } 00070 }
Generated on Tue Jul 19 2022 12:29:49 by
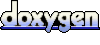