copy 2
Fork of BTW_Eddystone_Solution by
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "MicroBit.h" 00002 00003 MicroBit uBit; 00004 00005 char URL[] = "https://goo.gl/LGZu73"; 00006 00007 // lvl : Pwr@ 1m : Pwr@ 0m 00008 // 0 : -90 : -49 00009 // 1 : -78 : -37 00010 // 2 : -74 : -33 00011 // 3 : -69 : -28 00012 // 4 : -66 : -25 00013 // 5 : -61 : -20 00014 // 6 : -56 : -15 00015 // 7 : -51 : -10 00016 00017 const int8_t CALIBRATED_POWERS[] = {-49, -37, -33, -28, -25, -20, -15, -10}; 00018 00019 uint8_t advertising = 0; 00020 uint8_t tx_power_level = 6; 00021 00022 void startAdvertising() { 00023 uBit.bleManager.advertiseEddystoneUrl(URL, CALIBRATED_POWERS[tx_power_level-1], false); 00024 uBit.bleManager.setTransmitPower(tx_power_level); 00025 uBit.display.scroll("ADV"); 00026 advertising = 1; 00027 } 00028 00029 void stopAdvertising() { 00030 uBit.bleManager.stopAdvertising(); 00031 uBit.display.scroll("OFF"); 00032 advertising = 0; 00033 } 00034 00035 void onButtonA(MicroBitEvent) 00036 { 00037 if (advertising == 1) { 00038 return; 00039 } 00040 startAdvertising(); 00041 } 00042 00043 void onButtonB(MicroBitEvent) 00044 { 00045 if (advertising == 0) { 00046 return; 00047 } 00048 stopAdvertising(); 00049 } 00050 00051 int main() 00052 { 00053 // Initialise the micro:bit runtime. 00054 uBit.init(); 00055 00056 uBit.messageBus.listen(MICROBIT_ID_BUTTON_A, MICROBIT_BUTTON_EVT_CLICK, onButtonA); 00057 uBit.messageBus.listen(MICROBIT_ID_BUTTON_B, MICROBIT_BUTTON_EVT_CLICK, onButtonB); 00058 00059 startAdvertising(); 00060 00061 release_fiber(); 00062 }
Generated on Thu Jul 21 2022 10:49:35 by
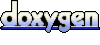