A Command Interpreter with support for used defined commands, subsystems, macros, help and parameter parsing.
cmdb.h
00001 /* mbed Command Interpreter Library 00002 * Copyright (c) 2011 wvd_vegt 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a copy 00005 * of this software and associated documentation files (the "Software"), to deal 00006 * in the Software without restriction, including without limitation the rights 00007 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00008 * copies of the Software, and to permit persons to whom the Software is 00009 * furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included in 00012 * all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00015 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00016 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00017 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00018 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00019 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00020 * THE SOFTWARE. 00021 */ 00022 00023 #ifndef MBED_CMDB_H 00024 #define MBED_CMDB_H 00025 00026 #include "mbed.h" 00027 00028 #include <vector> 00029 #include <limits> 00030 00031 //------------------------------------------------------------------------------ 00032 00033 /** Max size of an Ansi escape code. 00034 */ 00035 #define MAX_ESC_LEN 5 00036 00037 /** Max (strlen) of a Param. 00038 */ 00039 #define MAX_PARM_LEN 32 00040 00041 /** Max eight parms. 00042 */ 00043 #define MAX_ARGS 8 00044 00045 /** Max 132 characters commandline. 00046 */ 00047 #define MAX_CMD_LEN 132 00048 00049 /** 'Show' hidden subsystems and commands. 00050 */ 00051 #define SHOWHIDDEN 00052 00053 /** Enable macro commands. 00054 */ 00055 #define ENABLEMACROS 00056 00057 /** Enable statemachine. 00058 * 00059 * Used to implement a series of commands running at power-up. 00060 * 00061 * @note Not Implemented! 00062 */ 00063 #undef STATEMACHINE 00064 00065 /** Enable subsystem prompts. 00066 * 00067 * When defined, prompts will reflect the SubSystem. 00068 */ 00069 #define SUBSYSTEMPROMPTS 00070 00071 //------------------------------------------------------------------------------ 00072 00073 /** 8 bit limits. 00074 * 00075 * @see http://www.daniweb.com/forums/thread18963.html 00076 */ 00077 #define MIN_BYTE std::numeric_limits<unsigned char>::min() 00078 00079 /** 8 bit limits. 00080 * 00081 * @see http://www.daniweb.com/forums/thread18963.html 00082 */ 00083 #define MAX_BYTE std::numeric_limits<unsigned char>::max() 00084 00085 /** 8 bit limits. 00086 * 00087 * @see http://www.daniweb.com/forums/thread18963.html 00088 */ 00089 #define MIN_CHAR std::numeric_limits<signed char>::min() 00090 00091 /** 8 bit limits. 00092 * 00093 * @see http://www.daniweb.com/forums/thread18963.html 00094 */ 00095 #define MAX_CHAR std::numeric_limits<signed char>::max() 00096 00097 /** 16 bit limits. 00098 * 00099 * @see http://www.daniweb.com/forums/thread18963.html 00100 */ 00101 #define MIN_SHORT std::numeric_limits<short int>::min() 00102 00103 /** 16 bit limits. 00104 * 00105 * @see http://www.daniweb.com/forums/thread18963.html 00106 */ 00107 #define MAX_SHORT std::numeric_limits<short int>::max() 00108 00109 /** 16 bit limits. 00110 * 00111 * @see http://www.daniweb.com/forums/thread18963.html 00112 */ 00113 #define MIN_USHORT std::numeric_limits<unsigned short int>::min() 00114 00115 /** 16 bit limits. 00116 * 00117 * @see http://www.daniweb.com/forums/thread18963.html 00118 */ 00119 #define MAX_USHORT std::numeric_limits<unsigned short int>::max() 00120 00121 /** 32 bit limits. 00122 * 00123 * @see http://www.daniweb.com/forums/thread18963.html 00124 */ 00125 #define MIN_INT std::numeric_limits<int>::min() 00126 #define MAX_INT std::numeric_limits<int>::max() 00127 00128 /** 32 bit limits. 00129 * 00130 * @see http://www.daniweb.com/forums/thread18963.html 00131 */ 00132 #define MIN_UINT std::numeric_limits<unsigned int>::min() 00133 #define MAX_UINT std::numeric_limits<unsigned int>::max() 00134 00135 /** 32 bit limits. 00136 * 00137 * @see http://www.daniweb.com/forums/thread18963.html 00138 */ 00139 #define MIN_LONG std::numeric_limits<long>::min() 00140 #define MAX_LONG std::numeric_limits<long>::max() 00141 00142 //------------------------------------------------------------------------------ 00143 00144 /** Description of a command. 00145 */ 00146 struct cmd { 00147 public: 00148 const char *cmdstr; 00149 int subs; 00150 int cid; 00151 const char *parms; 00152 const char *cmddescr; 00153 const char *parmdescr; 00154 }; 00155 00156 //------------------------------------------------------------------------------ 00157 00158 /** Cr. 00159 */ 00160 static const char cr = '\r'; 00161 00162 /** Lf. 00163 */ 00164 static const char lf = '\n'; 00165 00166 /** Bell. 00167 */ 00168 static const char bell = '\7'; 00169 00170 /** Escape. 00171 */ 00172 static const char esc = '\033'; 00173 00174 /** Space. 00175 */ 00176 static const char sp = ' '; 00177 00178 /** CrLf. 00179 */ 00180 static const char crlf[] = "\r\n"; 00181 00182 /** Backspace that 'tries' to wipe the last character. 00183 */ 00184 static const char bs[] = "\b \b"; 00185 00186 /** VT100 Bold Command. 00187 */ 00188 static const char boldon[] = "\033[1m"; 00189 00190 /** VT100 Normal Command. 00191 */ 00192 static const char boldoff[] = "\033[0m"; 00193 00194 /** VT100 Cls Command. 00195 */ 00196 static const char cls[] = "\033[2J"; 00197 00198 /** VT100 Home Command. 00199 */ 00200 static const char home[] = "\033[H"; 00201 00202 /** The default command prompt. 00203 */ 00204 static const char PROMPT[] = "CMD>"; 00205 00206 //------------------------------------------------------------------------------ 00207 00208 /** Subsystem Id for a Subsystem. 00209 */ 00210 #define SUBSYSTEM -1 00211 00212 /** Subsystem Id for a Global Command (always available). 00213 */ 00214 #define GLOBALCMD -2 00215 00216 /** Subsystem Id for a Hidden Subsystem (ommitted from help). 00217 */ 00218 #define HIDDENSUB -3 00219 00220 /** Predefined Dump Command. 00221 */ 00222 #define CID_COMMANDS 9989 00223 00224 /** Predefined Boot Command. 00225 */ 00226 #define CID_BOOT 9990 00227 00228 /** Predefined Macro Command. 00229 * 00230 * This command will take a string with spaces replace by _ and cr replace by | for later replay with run. 00231 */ 00232 #define CID_MACRO 9991 00233 00234 /** Predefined Macro Command. 00235 * 00236 * This command replay a macro. 00237 */ 00238 #define CID_RUN 9992 00239 00240 /** Predefined Macro Command. 00241 * 00242 * This command print the current macro. 00243 */ 00244 #define CID_MACROS 9993 00245 00246 /** Predefined Echo Command. 00247 * 00248 * This command turn echo on or off. 00249 */ 00250 #define CID_ECHO 9994 00251 00252 /** Predefined VT100 Bold Command. 00253 * 00254 * This command turn VT100 bold usage on or off. 00255 */ 00256 #define CID_BOLD 9995 00257 00258 /** Predefined VT100 Cls Command. 00259 * 00260 * This command will clear the screen. 00261 */ 00262 #define CID_CLS 9996 00263 00264 /** Predefined Idle Command. 00265 * 00266 * This command will return to the global command level, leaving the active subsystem. 00267 */ 00268 #define CID_IDLE 9997 00269 00270 /** Predefined Help Command. 00271 * 00272 * This command will either print all active command (without parameters) or a more detailed 00273 * help for a command passed as parameter. 00274 */ 00275 #define CID_HELP 9998 00276 00277 /** Predefided Semi Command. 00278 * 00279 * CID_LAST only functions as a special Commend Id to signal unknown commands. 00280 */ 00281 #define CID_LAST 9999 00282 00283 //------------------------------------------------------------------------------ 00284 00285 /** The Boot Command. 00286 * 00287 * @note: this command can be used to list all commands in Windows ini file format for host processing. 00288 * 00289 * Optional. 00290 */ 00291 static const cmd COMMANDS = {"Commands",GLOBALCMD,CID_COMMANDS,"","Dump Commands"}; 00292 00293 /** The Boot Command. 00294 * 00295 * Optional. 00296 */ 00297 static const cmd BOOT = {"Boot",GLOBALCMD,CID_BOOT,"","Boot mBed"}; 00298 00299 /** The Macro Command. 00300 * 00301 * Optional. 00302 */ 00303 static const cmd MACRO = {"Macro",GLOBALCMD,CID_MACRO,"%s","Define macro (sp->_, cr->|)","command(s)"}; 00304 00305 /** The Run Command. 00306 * 00307 * Optional. 00308 */ 00309 static const cmd RUN = {"Run",GLOBALCMD,CID_RUN,"","Run a macro"}; 00310 00311 /** The Macros Command. 00312 * 00313 * Optional. 00314 */ 00315 static const cmd MACROS = {"Macros",GLOBALCMD,CID_MACROS,"","List macro(s)"}; 00316 00317 /** The Echo Command. 00318 * 00319 * Optional. 00320 */ 00321 static const cmd ECHO = {"Echo",GLOBALCMD,CID_ECHO,"%bu","Echo On|Off (1|0)","state"}; 00322 00323 /** The Bold Command. 00324 * 00325 * Optional. 00326 */ 00327 static const cmd BOLD = {"Bold",GLOBALCMD,CID_BOLD,"%bu","Bold On|Off (1|0)","state"}; 00328 00329 /** The Cls Command. 00330 * 00331 * Optional. 00332 */ 00333 static const cmd CLS = {"Cls",GLOBALCMD,CID_CLS,"","Clears the terminal screen"}; 00334 00335 /** The Idle Command. 00336 * 00337 * Mandatory if you use subsystems. 00338 */ 00339 static const cmd IDLE = {"Idle",GLOBALCMD,CID_IDLE,"","Deselect Subsystems"}; 00340 00341 /** The Help Command. 00342 * 00343 * Mandatory. 00344 */ 00345 static const cmd HELP = {"Help",GLOBALCMD,CID_HELP,"%s","Help"}; 00346 00347 //------------------------------------------------------------------------------ 00348 00349 /** We'll only define the 4 cursor codes at the moment. 00350 */ 00351 #define ESC_TBL_LEN 4 00352 00353 /** Escape code definition struct. 00354 */ 00355 struct esc { 00356 char *escstr; 00357 int id; 00358 }; 00359 00360 /** The Escape Code Id's. 00361 */ 00362 enum { 00363 EID_CURSOR_UP, 00364 EID_CURSOR_DOWN, 00365 EID_CURSOR_RIGHT, 00366 EID_CURSOR_LEFT, 00367 EID_LAST 00368 }; 00369 00370 /** The Escape Codes Table. 00371 */ 00372 static const struct esc esc_tbl [ESC_TBL_LEN] = { 00373 { "\033[A", EID_CURSOR_UP }, 00374 { "\033[B", EID_CURSOR_DOWN }, 00375 { "\033[C", EID_CURSOR_RIGHT }, 00376 { "\033[D", EID_CURSOR_LEFT }, 00377 }; 00378 00379 //------------------------------------------------------------------------------ 00380 00381 /** The Command Interpreter Version. 00382 */ 00383 #define CMDB_VERSION 0.81 00384 00385 //------------------------------------------------------------------------------ 00386 00387 /** Command Interpreter class. 00388 * 00389 * Steps to take: 00390 * 00391 * 1) Create a std::vector<cmd> and fill it with at least 00392 * the mandatory commands IDLE and HELP. 00393 * 00394 * 2) Create an Cmdb class instance and pass it the vector, 00395 * a Serial port object like Serial serial(USBTX, USBRX); 00396 * and finally a command dispatcher function. 00397 * 00398 * 3) Feed the interpreter with characters received from your serial port. 00399 * Note: Cmdb self does not retrieve input it must be handed to it. 00400 * It implements basic members for checking/reading the serial port. 00401 * 00402 * 4) Handle commands added by the application by the Cid and parameters passed. 00403 * 00404 * Note: Predefined commands and all subsystems transitions are handled by the internal dispatcher. 00405 * So the passed dispatcher only has to handle user/application defined commands'. 00406 * 00407 * @see main.cpp for a demo. 00408 */ 00409 class Cmdb { 00410 public: 00411 /** Create a Command Interpreter. 00412 * 00413 * @see http://www.newty.de/fpt/fpt.html#chapter2 for function pointers. 00414 * @see http://stackoverflow.com/questions/9410/how-do-you-pass-a-function-as-a-parameter-in-c 00415 * @see http://www.daniweb.com/forums/thread293338.html 00416 * 00417 * @param serial a Serial port used for communication. 00418 * @param cmds a vector with the command table. 00419 */ 00420 Cmdb(const RawSerial& _serial, std::vector<cmd>& _cmds, void (*_callback)(Cmdb&,int) ); 00421 00422 /** The version of the Command Interpreter. 00423 * 00424 * returns the version. 00425 */ 00426 static float version() { 00427 return CMDB_VERSION; 00428 } 00429 00430 /** NULL is used as No Comment Value. 00431 */ 00432 static const char* NoComment; 00433 00434 /** Column 72 is used as Default Comment Starting Position. 00435 */ 00436 static int DefComPos; 00437 00438 /** Checks if the macro buffer has any characters left. 00439 * 00440 * @returns true if any characters left. 00441 */ 00442 bool macro_hasnext(); 00443 00444 /** Gets the next character from the macro buffer and 00445 * advances the macro buffer pointer. 00446 * 00447 * @note Do not call if no more characters are left! 00448 * 00449 * @returns the next character. 00450 */ 00451 char macro_next(); 00452 00453 /** Gets the next character from the macro buffer 00454 * but does not advance the macro buffer pointer. 00455 * 00456 * @note Do not call if no more characters are left! 00457 * 00458 * @returns the next character. 00459 */ 00460 char macro_peek(); 00461 00462 /** Resets the macro buffer and macro buffer pointer. 00463 * 00464 */ 00465 void macro_reset(); 00466 00467 /** Checks if the serial port has any characters 00468 * left to read by calling serial.readable(). 00469 * 00470 * @returns true if any characters available. 00471 */ 00472 bool hasnext(); 00473 00474 /** Gets the next character from the serial port by 00475 * calling serial.getc(). 00476 * 00477 * Do not call if no characters are left! 00478 * 00479 * @returns the next character. 00480 */ 00481 char next(); 00482 00483 /** Add a character to the command being processed. 00484 * If a cr is added, the command is parsed and executed if possible 00485 * If supported special keys are encountered (like backspace, delete and cursor up) they are processed. 00486 * 00487 * @param c the character to add. 00488 * 00489 * @returns true if a command was recognized and executed. 00490 */ 00491 bool scan(const char c); 00492 00493 /** printf substitute using the serial parameter passed to the constructor. 00494 * 00495 * @see http://www.cplusplus.com/reference/clibrary/cstdio/printf/ 00496 * 00497 * @parm format the printf format string. 00498 * @parm ... optional paramaters to be merged into the format string. 00499 * 00500 * @returns the printf return value. 00501 */ 00502 int printf(const char *format, ...); 00503 00504 /** print is simply printf without parameters using the serial parameter passed to the constructor. 00505 * 00506 * @parm msg the string to print. 00507 * 00508 * @returns the printf return value. 00509 */ 00510 int print(const char *msg); 00511 00512 /** println is simply printf without parameters using the serial parameter passed to the constructor. 00513 * 00514 * @parm msg the string to print followed by a crlf. 00515 * 00516 * @returns the printf return value. 00517 */ 00518 int println(const char *msg); 00519 00520 /** printch is simply putc subsitute using the serial parameter passed to the constructor. 00521 * 00522 * @parm msg the string to print. 00523 * 00524 * @returns the printf return value. 00525 */ 00526 char printch(const char ch); 00527 00528 /** printsection prints an inifile Section Header 00529 * like: 00530 * 00531 * [Section]\r\n 00532 * 00533 * Usage: cmdb.printsection("GP"); 00534 * 00535 * @parm section the section to print. 00536 * 00537 * @returns the printf return value. 00538 */ 00539 int printsection(const char *section); 00540 00541 /** printmsg prints an inifile Msg Key=Value pair. 00542 * like: 00543 * 00544 * Msg={msg}\r\n 00545 * 00546 * Usage: cmdb.printmsg("Validation successfull"); 00547 * 00548 * @parm msg the msg to print. 00549 * 00550 * @returns the printf return value. 00551 */ 00552 int printmsg(const char *msg); 00553 00554 /** printerror prints an inifile Error Section Header and Error Msg Key=Value pair. 00555 * like: 00556 * 00557 * [Error]\r\nmsg={errormsg}\r\n 00558 * 00559 * Usage: cmdb.printerror("Data Size Incorrect"); 00560 * 00561 * @parm errormsg the error msg to print. 00562 * 00563 * @returns the printf return value. 00564 */ 00565 int printerror(const char *errormsg); 00566 00567 /** printerror prints an inifile Error Section Header and Error Msg Key=Value pair. 00568 * like: 00569 * 00570 * [Error]\r\nmsg={errormsg}\r\n 00571 * 00572 * Usage: cmdb.printerrorf("Data Size Incorrect %d", 15); 00573 * 00574 * @parm format the error msg to print. 00575 * @parm parameter to print. 00576 * 00577 * @returns the printf return value. 00578 */ 00579 int printerrorf(const char *format, ...); 00580 00581 /** printvalue prints an inifile Key/Value Pair 00582 * like: 00583 * 00584 * Key=Value ;comment\r\n 00585 * 00586 * Note: the Comment is (if present) located at position 72. 00587 * 00588 * Usage: cmdb.printvaluef("Value", Cmdb::DefComPos, "Hex", "0x%8.8X", LPC_RTC->GPREG0); 00589 * 00590 * @parm key the key to print. 00591 * @parm comment the comment to print. 00592 * @parm width the location of the comment to print. 00593 * @parm format the value to print. 00594 * @parm parameter to print. 00595 * 00596 * @returns the printf return value. 00597 */ 00598 int printvaluef(const char *key, const int width, const char *comment, const char *format, ...); 00599 00600 /** printvalue prints an inifile Key/Value Pair 00601 * like: 00602 * 00603 * Key=Value\r\n 00604 * 00605 * Usage: cmdb.printvaluef("Value", "0x%8.8X", LPC_RTC->GPREG0); 00606 * 00607 * @parm key the key to print. 00608 * @parm format the value to print. 00609 * @parm parameter to print. 00610 * 00611 * @returns the printf return value. 00612 */ 00613 int printvaluef(const char *key, const char *format, ...); 00614 00615 /** printvalue prints an inifile Key/Value Pair 00616 * like: 00617 * 00618 * Key=Value ;comment\r\n 00619 * 00620 * Note the Comment is (if present) located at position 72. 00621 * 00622 * @parm key the key to print. 00623 * @parm value the value to print. 00624 * @parm comment the comment to print. 00625 * @parm width the location of the comment to print. 00626 * 00627 * @returns the printf return value. 00628 */ 00629 int printvalue(const char *key, const char *value, const char *comment = NoComment, const int width = DefComPos); 00630 00631 int printcomment(const char *comment, const int width = DefComPos); 00632 00633 //------------------------------------------------------------------------------ 00634 00635 /** Initializes the parser (called by the constructor). 00636 * 00637 * @parm full if true the macro buffer is also cleared else only the command interpreter is reset. 00638 */ 00639 void init(const char full); 00640 00641 //------------------------------------------------------------------------------ 00642 //----These helper functions retieve parameters in the correct format. 00643 //------------------------------------------------------------------------------ 00644 00645 /** Typecasts parameter ndx to a bool. 00646 * 00647 * mask: %bu 00648 * 00649 * @parm the parameter index 00650 * 00651 * @return a bool 00652 */ 00653 bool BOOLPARM(int ndx) { 00654 return parms[ndx].val.uc!=0; 00655 } 00656 00657 /** Typecasts parameter ndx to a byte/unsigned char. 00658 * 00659 * mask: %bu 00660 * 00661 * @parm the parameter index 00662 * 00663 * @return a byte/unsigned char 00664 */ 00665 unsigned char BYTEPARM(int ndx) { 00666 return parms[ndx].val.uc; 00667 } 00668 00669 /** Typecasts parameter ndx to a char. 00670 * 00671 * mask: %c 00672 * 00673 * @parm the parameter index 00674 * 00675 * @return a char 00676 */ 00677 char CHARPARM(int ndx) { 00678 return parms[ndx].val.c; 00679 } 00680 00681 /** Typecasts parameter ndx to word/unsigned int. 00682 * 00683 * mask: %hu 00684 * 00685 * @parm the parameter index 00686 * 00687 * @return a word/unsigned int 00688 */ 00689 unsigned int WORDPARM(int ndx) { 00690 return parms[ndx].val.ui; 00691 } 00692 00693 /** Typecasts parameter ndx to a unsigned int. 00694 * 00695 * mask: %u 00696 * 00697 * @parm the parameter index 00698 * 00699 * @return a unsigned int 00700 */ 00701 unsigned int UINTPARM(int ndx) { 00702 return parms[ndx].val.ui; 00703 } 00704 00705 /** Typecasts parameter ndx to a int. 00706 * 00707 * mask: %i 00708 * 00709 * @parm the parameter index 00710 * 00711 * @return a int 00712 */ 00713 int INTPARM(int ndx) { 00714 return parms[ndx].val.i; 00715 } 00716 00717 /** Typecasts parameter ndx to a bool. 00718 * 00719 * mask: %lu 00720 * 00721 * @parm the parameter index 00722 * 00723 * @return a bool 00724 */ 00725 unsigned long DWORDPARM(int ndx) { 00726 return parms[ndx].val.ul; 00727 } 00728 00729 /** Typecasts parameter ndx to a long. 00730 * 00731 * mask: %li 00732 * 00733 * @parm the parameter index 00734 * 00735 * @return a long 00736 */ 00737 long LONGPARM(int ndx) { 00738 return parms[ndx].val.l; 00739 } 00740 00741 /** Typecasts parameter ndx to a float. 00742 * 00743 * mask: %f 00744 * 00745 * @parm the parameter index 00746 * 00747 * @return a float 00748 */ 00749 float FLOATPARM(int ndx) { 00750 return parms[ndx].val.f; 00751 } 00752 00753 /** Typecasts parameter ndx to a string. 00754 * 00755 * @note spaces are not allowed as it makes parsing so much harder. 00756 * 00757 * mask: %s 00758 * 00759 * @parm the parameter index 00760 * 00761 * @return a string 00762 */ 00763 char* STRINGPARM(int ndx) { 00764 return parms[ndx].val.s; 00765 } 00766 00767 bool present(char *cmdstr) { 00768 return cmdid_search(cmdstr)!=CID_LAST; 00769 } 00770 00771 void replace(std::vector<cmd>& newcmds) { 00772 cmds.assign(newcmds.begin(), newcmds.end()); 00773 } 00774 00775 00776 int indexof(int cid) { 00777 return cmdid_index(cid); 00778 } 00779 00780 //FAILS... 00781 /* 00782 void insert(int cid, cmd newcmd) { 00783 //Add Command (update our original and then assign/replace cmdb's copy)... 00784 vector<cmd>::iterator iter; 00785 00786 std::vector<cmd> newcmds = std::vector<cmd>(cmds); 00787 00788 iter = newcmds.begin(); 00789 00790 newcmds.insert(iter+indexof(cid)+1,newcmd); 00791 00792 replace(newcmds); 00793 00794 printf("Index: %d\r\n", ndx); 00795 00796 print("check #1\r\n"); 00797 print("check #2\r\n"); 00798 00799 vector<cmd>::iterator it; 00800 it=newcmds.begin(); 00801 00802 print("check #3\r\n"); 00803 ndx++; 00804 newcmds.insert(it, newcmd); 00805 print("check #4\r\n"); 00806 00807 //cmds.push_back(c1); 00808 cmds.assign(newcmds.begin(), newcmds.end()); 00809 } 00810 */ 00811 00812 private: 00813 00814 /** Internal Serial Port Storage. 00815 */ 00816 RawSerial serial; 00817 00818 /** Internal Command Table Vector Storage. 00819 * 00820 * @see http://www.cplusplus.com/reference/stl/vector/ 00821 */ 00822 std::vector<cmd> cmds; 00823 00824 /** C callback function 00825 * 00826 * @see See http://www.newty.de/fpt/fpt.html#chapter2 for function pointers. 00827 * 00828 * C++ member equivalent would be void (Cmdb::*callback)(Cmdb&,int); 00829 */ 00830 void (*user_callback)(Cmdb&,int); 00831 00832 /** Searches the escape code list for a match. 00833 * 00834 * @param char* escstr the escape code to lookup. 00835 * 00836 * @returns the index of the escape code or -1. 00837 */ 00838 int escid_search(char *escstr); 00839 00840 /** Checks if the command table for a match. 00841 * 00842 * @param char* cmdstr the command to lookup. 00843 * 00844 * @returns the id of the command or -1. 00845 */ 00846 int cmdid_search(char *cmdstr); 00847 00848 /** Converts an command id to an index of the command table. 00849 * 00850 * @param cmdid the command id to lookup. 00851 * 00852 * @returns the index of the command or -1. 00853 */ 00854 int cmdid_index(int cmdid); 00855 00856 /** Writes a prompt to the serial port. 00857 * 00858 */ 00859 void prompt(void); 00860 00861 /** Called by cmd_dispatch it parses the command against the command table. 00862 * 00863 * @param cmd the command and paramaters to parse. 00864 * 00865 * @returns the id of the parsed command. 00866 */ 00867 int parse(char *cmd); 00868 00869 /** Called by scan it processes the arguments and dispatches the command. 00870 * 00871 * Note: This member calls the callback callback function. 00872 * 00873 * @param cmd the command to dispatch. 00874 */ 00875 void cmd_dispatcher(char *cmd); 00876 00877 /** Generates Help from the command table and prints it. 00878 * 00879 * @param pre leading text 00880 * @param ndx the index of the command in the command table. 00881 * @param post trailing text. 00882 */ 00883 void cmd_help(char *pre, int ndx, char *post); 00884 00885 /** Dumps all commands in ini file format. 00886 */ 00887 void cmd_dump(); 00888 00889 /** memset wrapper. 00890 * 00891 * @param p The string to be cleared. 00892 * @param siz The string size. 00893 */ 00894 void zeromemory(char *p,unsigned int siz); 00895 00896 /** Case insensitive compare. 00897 * 00898 * @see strcmp. 00899 * 00900 * @param s1 00901 * @param s2 the second string to compare. 00902 * 00903 * @returns 0 if s1=s2, -1 if s1<s2 or +1 if s1>s2. 00904 */ 00905 int stricmp (char *s1, char *s2); 00906 00907 /** Internal Echo Flag Storage. 00908 */ 00909 bool echo; 00910 00911 /** Internal VT100 Bold Flag Storage. 00912 */ 00913 bool bold; 00914 00915 /** Internal Command Table Length Storage. 00916 */ 00917 //int CMD_TBL_LEN; 00918 00919 //Macro's. 00920 /** Internal Macro Pointer. 00921 */ 00922 int macro_ptr; 00923 00924 /** Internal Macro Buffer. 00925 */ 00926 char macro_buf[1 + MAX_CMD_LEN]; 00927 00928 /** Used for parsing parameters. 00929 */ 00930 enum parmtype { 00931 PARM_UNUSED, //0 00932 00933 PARM_FLOAT, //1 (f) 00934 00935 PARM_LONG, //2 (l/ul) 00936 PARM_INT, //3 (i/ui) 00937 PARM_SHORT, //4 (w/uw) 00938 00939 PARM_CHAR, //5 (c/uc) 00940 PARM_STRING //6 (s) 00941 }; 00942 00943 /** Used for parsing parameters. 00944 */ 00945 union value { 00946 float f; 00947 00948 unsigned long ul; 00949 long l; 00950 00951 int i; 00952 unsigned int ui; 00953 00954 short w; 00955 unsigned short uw; 00956 00957 char c; 00958 unsigned char uc; 00959 00960 char s[MAX_PARM_LEN]; 00961 }; 00962 00963 /** Used for parsing parameters. 00964 */ 00965 struct parm { 00966 enum parmtype type; 00967 union value val; 00968 }; 00969 00970 //------------------------------------------------------------------------------ 00971 //----Buffers & Storage. 00972 //------------------------------------------------------------------------------ 00973 00974 /** Command Buffer. 00975 */ 00976 char cmdbuf [1 + MAX_CMD_LEN]; // command buffer 00977 00978 /** Command Buffer Pointer. 00979 */ 00980 char cmdndx; // command index 00981 00982 /** Last Command Buffer (Used when pressing Cursor Up). 00983 */ 00984 char lstbuf [1 + MAX_CMD_LEN]; // last command buffer 00985 00986 /** Escape Code Buffer. 00987 */ 00988 char escbuf [1 + MAX_ESC_LEN]; 00989 00990 /** Escape Code Buffer Pointer. 00991 */ 00992 unsigned char escndx; 00993 00994 /** Storage for Parsed Parameters 00995 */ 00996 struct parm parms[MAX_ARGS]; 00997 00998 /** Parsed Parameters Pointer. 00999 */ 01000 int noparms; 01001 01002 /** Current Selected Subsystem (-1 for Global). 01003 */ 01004 int subsystem; 01005 01006 /** No of arguments found in command. 01007 */ 01008 int argcnt; 01009 01010 /** No of arguments to find in parameter definition (Command Table). 01011 */ 01012 int argfnd; 01013 01014 /** strtoXX() Error detection. 01015 */ 01016 int error; 01017 }; 01018 01019 extern "C" void mbed_reset(); 01020 01021 #endif
Generated on Wed Jul 13 2022 19:03:15 by
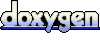