
Connections to Xively working; has 5 channels on Xively (axl_x, axl_y, axl_z, heater_status, temperature)
Dependencies: C12832_lcd EthernetInterface LM75B MMA7660 NTPClient libxively mbed-rtos mbed
main.cpp
00001 #include "mbed.h" 00002 #include "EthernetInterface.h" 00003 #include "NTPClient.h" 00004 #include "C12832_lcd.h" 00005 #include "LM75B.h" 00006 #include "MMA7660.h" 00007 #define XI_FEED_ID 1000476735 // set Xively Feed ID (numerical, no quoutes) 00008 #define XI_API_KEY "ROxvAOZ6RznZjFPGufMNNn1LiMbYseCgEwF0qn1WAmcZPPY2" // set Xively API key (double-quoted string) 00009 00010 #include "app_board_io.h" 00011 00012 #include "xively.h" 00013 #include "xi_err.h" 00014 00015 #define PST_OFFSET 7*60*60 00016 00017 Ticker timer; 00018 C12832_LCD lcd; //Graphics LCD 00019 EthernetInterface eth; //Networking functions 00020 NTPClient ntp; //NTP client 00021 LM75B tmp(p28,p27); 00022 float lowTemp = 74; 00023 float highTemp = 86; 00024 float temp; 00025 int heaterOn = 0; 00026 AnalogIn pot1(p20); 00027 AnalogIn pot2(p19); 00028 #define LOOP_DELAY_MS 100 00029 //update time every 10 minutes 00030 #define UPDATE_TIME 60*1 00031 #define UPDATE_XIVELY 60*1 00032 MMA7660 axl(p28, p27);// accelerometer 00033 int updateTimeFromServer = 1; 00034 00035 //Xively globals 00036 int update_xively = 1; 00037 xi_feed_t feed; 00038 xi_datapoint_t* current_temperature; 00039 xi_datapoint_t* current_x; 00040 xi_datapoint_t* current_y; 00041 xi_datapoint_t* current_z; 00042 xi_datapoint_t* current_heaterstatus; 00043 xi_datastream_t* heaterstatus_datastream; 00044 xi_context_t* xi_context; 00045 00046 00047 void setUpXively() { 00048 memset( &feed, NULL, sizeof( xi_feed_t ) ); 00049 00050 feed.feed_id = XI_FEED_ID; 00051 feed.datastream_count = 5; 00052 00053 feed.datastreams[0].datapoint_count = 1; 00054 xi_datastream_t* temperature_datastream = &feed.datastreams[0]; 00055 strcpy( temperature_datastream->datastream_id, "temperature" ); 00056 current_temperature = &temperature_datastream->datapoints[0]; 00057 00058 feed.datastreams[1].datapoint_count = 1; 00059 xi_datastream_t* axl_x_datastream = &feed.datastreams[1]; 00060 strcpy( axl_x_datastream->datastream_id, "axl_x" ); 00061 current_x = &axl_x_datastream->datapoints[0]; 00062 00063 feed.datastreams[2].datapoint_count = 1; 00064 xi_datastream_t* axl_y_datastream = &feed.datastreams[2]; 00065 strcpy( axl_y_datastream->datastream_id, "axl_y" ); 00066 current_y = &axl_y_datastream->datapoints[0]; 00067 00068 feed.datastreams[3].datapoint_count = 1; 00069 xi_datastream_t* axl_z_datastream = &feed.datastreams[3]; 00070 strcpy( axl_z_datastream->datastream_id, "axl_z" ); 00071 current_z = &axl_z_datastream->datapoints[0]; 00072 00073 feed.datastreams[4].datapoint_count = 1; 00074 heaterstatus_datastream = &feed.datastreams[4]; 00075 strcpy( heaterstatus_datastream->datastream_id, "heater_status" ); 00076 current_heaterstatus = &heaterstatus_datastream->datapoints[0]; 00077 00078 // create the cosm library context 00079 xi_context = xi_create_context( XI_HTTP, XI_API_KEY, feed.feed_id ); 00080 00081 } 00082 00083 void connectToTheInternet() 00084 { 00085 eth.init(); //Init and use DHCP 00086 wait(2); 00087 lcd.cls(); 00088 lcd.printf("Getting IP Address\r\n"); 00089 printf("\n\rGetting IP Address\r\n"); 00090 if(eth.connect(60000)!=0) { 00091 lcd.printf("DHCP error - No IP"); 00092 wait(10); 00093 } else { 00094 lcd.printf("IP is %s\n", eth.getIPAddress()); 00095 printf("IP is %s\n", eth.getIPAddress()); 00096 wait(2); 00097 } 00098 lcd.cls(); 00099 } 00100 00101 void updateTimeRoutine() 00102 { 00103 if (ntp.setTime("0.pool.ntp.org") == 0) { 00104 printf("Time updated!"); 00105 } else { 00106 lcd.locate(0,0); 00107 printf("Time update failed \n\r"); 00108 lcd.printf("Time update failed"); 00109 } 00110 updateTimeFromServer = 0; 00111 } 00112 00113 void updateTime() 00114 { 00115 updateTimeFromServer = 1; 00116 } 00117 00118 void updateXively() { 00119 update_xively = 1; 00120 } 00121 00122 void updateXivelyRoutine() { 00123 xi_set_value_f32( current_temperature, tmp.read() ); 00124 xi_set_value_f32( current_x, axl.x() ); 00125 xi_set_value_f32( current_y, axl.y() ); 00126 xi_set_value_f32( current_z, axl.z() ); 00127 update_xively = 0; 00128 printf( "update...\n" ); 00129 xi_feed_update( xi_context, &feed ); 00130 00131 //xi_response_t myInData; 00132 //myInData = xi_feed_get(xi_context, &feed); 00133 xi_datastream_get(xi_context, feed.feed_id, heaterstatus_datastream->datastream_id, heaterstatus_datastream->datapoints); 00134 printf( "\n\rHEATER STATUS: %d...\n",heaterstatus_datastream->datapoints[0]); 00135 current_heaterstatus = &heaterstatus_datastream->datapoints[0]; 00136 int heatervalue = current_heaterstatus->value.i32_value; 00137 printf("HeaterStatus: %d", heatervalue); 00138 printf( "done...\n" ); 00139 } 00140 00141 //POT values are 0:1, will allow high and low temperatures 00142 //lets you set temp between 50 and 100 degrees 00143 void updateTempsFromPots() 00144 { 00145 float lowTempPot = pot2*50.0+50; 00146 float highTempPot = pot1*50.0+50; 00147 //round to the nearest whole number 00148 lowTempPot = floor(lowTempPot+.5); 00149 highTempPot = floor(highTempPot+.5); 00150 //high temp must be at least 1 degree above low temp 00151 if (highTempPot <= lowTempPot) { 00152 highTempPot = lowTempPot +1; 00153 } 00154 //Refresh the display if the temps have changed by more than a degree 00155 if ((lowTemp != lowTempPot) || (highTemp != highTempPot)) { 00156 lowTemp = lowTempPot; 00157 highTemp = highTempPot; 00158 lcd.locate(0,0); 00159 lcd.printf("\n\r%.1f LOW: %.0f HIGH: %.0f", temp, lowTemp, highTemp); 00160 printf("LOW: %.2f HIGH: %.2f\n\r", lowTemp, highTemp); 00161 } 00162 } 00163 00164 int main() 00165 { 00166 connectToTheInternet(); 00167 setUpXively(); 00168 //Variable to hold the current minute so we only update the display when the minute changes 00169 char currentMinute[2]; 00170 currentMinute[1] = 'a'; 00171 char minute[2]; 00172 00173 float currentTemp = -200; 00174 lcd.printf("Updating time...\r\n"); 00175 printf("Updating time...\r\n"); 00176 if (ntp.setTime("0.pool.ntp.org") == 0) { 00177 printf("Set time successfully\r\n"); 00178 lcd.cls(); 00179 timer.attach(&updateTime, UPDATE_TIME); 00180 timer.attach(&updateXively, UPDATE_XIVELY); 00181 lcd.printf("\n\r\n\rHEATER OFF"); 00182 00183 while(1) { 00184 if(updateTimeFromServer) { 00185 updateTimeRoutine(); 00186 } 00187 if(update_xively){ 00188 updateXivelyRoutine(); 00189 } 00190 //Sets temp from POTs 00191 updateTempsFromPots(); 00192 //Fetch the time 00193 time_t ctTime; 00194 ctTime = time(NULL)- PST_OFFSET; 00195 char timeBuffer[32]; 00196 00197 //See if the minute has changed; set an update display flag if it has 00198 strftime(minute, 8, "%M", localtime(&ctTime)); 00199 if ( (minute[1] != currentMinute[1]) ) { 00200 //Formats the time for display 00201 strftime(timeBuffer, 32, "%a %b %d %I:%M%p\n\r", localtime(&ctTime)); 00202 printf("%s\r", timeBuffer); 00203 lcd.locate(0,0); 00204 lcd.printf("%s\r", timeBuffer); 00205 currentMinute[1] = minute[1]; 00206 } 00207 00208 //Update the temperature display if the temperature, set temp has changed 00209 temp = tmp.read()*9.0/5.0 + 32.0; 00210 00211 //checks if the temperature (rounded to the nearest whole number) has changed 00212 if (floor(temp+.5) != floor(currentTemp+.5)) { 00213 printf("Temp change: %.1f F\n\r", temp); 00214 currentTemp = temp; 00215 lcd.locate(0,0); 00216 //updates the temperature line of the display 00217 lcd.printf("\n\r%.1f LOW: %.0f HIGH: %.0f", temp, lowTemp, highTemp); 00218 } 00219 00220 lcd.locate(0,0); 00221 00222 //Heater logic: turns off if it has gone over the high temp and is on, 00223 //or if under the low temp and is off 00224 if (heaterOn && (temp > highTemp) ) { 00225 printf("Heater turned OFF\n\r"); 00226 heaterOn = 0; 00227 lcd.locate(0,0); 00228 lcd.printf("\n\r\n\rHEATER OFF"); 00229 } else if (!heaterOn && (temp < lowTemp) ) { 00230 printf("Heater turned ON\n\r"); 00231 heaterOn = 1; 00232 lcd.locate(0,0); 00233 lcd.printf("\n\r\n\rHEATER ON"); 00234 } 00235 wait(LOOP_DELAY_MS*.001); 00236 } 00237 } else { 00238 lcd.printf("NTP Error\r\n"); 00239 } 00240 eth.disconnect(); 00241 }
Generated on Mon Jul 25 2022 05:25:32 by
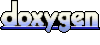