Fork of Edoardo De Marchi's ov7670 library. Works very similar, only few modification were made to support other boards.
Dependents: OV7670 application over USB with KL25Z
Fork of ov7670 by
ov7670.h
00001 // 00002 // OV7670 library 00003 // 00004 00005 #pragma once 00006 #include "mbed.h" 00007 #include "ov7670reg.h" 00008 00009 #define OV7670_WRITE (0x42) 00010 #define OV7670_READ (0x43) 00011 #define OV7670_WRITEWAIT (20) 00012 #define OV7670_NOACK (0) 00013 #define OV7670_REGMAX (201) 00014 #define OV7670_I2CFREQ (100000) 00015 00016 00017 class OV7670 00018 { 00019 public: 00020 00021 OV7670( 00022 PinName sda, // Camera I2C port 00023 PinName scl, // Camera I2C port 00024 PinName vs, // VSYNC 00025 PinName hr, // HREF 00026 PinName we, // WEN 00027 00028 PortName port, // 8bit bus port 00029 int mask, // 0b0000_0M65_4000_0321_L000_0000_0000_0000 = 0x07878000 00030 00031 PinName rt, // /RRST 00032 PinName o, // /OE 00033 PinName rc // RCLK 00034 ); 00035 00036 ~OV7670(); 00037 00038 void CaptureNext(void); // capture request 00039 bool CaptureDone(void); // capture done? (with clear) 00040 void WriteReg(int addr,int data); // write to camera 00041 int ReadReg(int addr); // read from camera 00042 void Reset(void); // reset reg camera 00043 int Init(char c, int n); // Old init reg 00044 int Init(char *format, int n); // init reg 00045 void VsyncHandler(void); // New vsync handler 00046 void HrefHandler(void); // href handler 00047 int ReadOnebyte(int mask1, int offset1, int mask2, int offset2); // Data Read 00048 void ReadStart(void); // Data Start 00049 void ReadStop(void); // Data Stop 00050 00051 00052 private: 00053 I2C _i2c; 00054 InterruptIn vsync,href; 00055 00056 DigitalOut wen; 00057 PortIn data; 00058 DigitalOut rrst,oe,rclk; 00059 volatile int LineCounter; 00060 volatile int LastLines; 00061 volatile bool CaptureReq; 00062 volatile bool Busy; 00063 volatile bool Done; 00064 char *format_temp; 00065 };
Generated on Thu Jul 21 2022 13:36:02 by
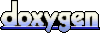