wolfSSL SSL/TLS library, support up to TLS1.3
Dependents: CyaSSL-Twitter-OAuth4Tw Example-client-tls-cert TwitterReader TweetTest ... more
sha.h
00001 /* sha.h 00002 * 00003 * Copyright (C) 2006-2020 wolfSSL Inc. 00004 * 00005 * This file is part of wolfSSL. 00006 * 00007 * wolfSSL is free software; you can redistribute it and/or modify 00008 * it under the terms of the GNU General Public License as published by 00009 * the Free Software Foundation; either version 2 of the License, or 00010 * (at your option) any later version. 00011 * 00012 * wolfSSL is distributed in the hope that it will be useful, 00013 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00014 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00015 * GNU General Public License for more details. 00016 * 00017 * You should have received a copy of the GNU General Public License 00018 * along with this program; if not, write to the Free Software 00019 * Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1335, USA 00020 */ 00021 00022 /*! 00023 \file wolfssl/wolfcrypt/sha.h 00024 */ 00025 00026 00027 #ifndef WOLF_CRYPT_SHA_H 00028 #define WOLF_CRYPT_SHA_H 00029 00030 #include <wolfssl/wolfcrypt/types.h > 00031 00032 #ifndef NO_SHA 00033 00034 #if defined(HAVE_FIPS) && \ 00035 defined(HAVE_FIPS_VERSION) && (HAVE_FIPS_VERSION >= 2) 00036 #include <wolfssl/wolfcrypt/fips.h> 00037 #endif /* HAVE_FIPS_VERSION >= 2 */ 00038 00039 #if defined(HAVE_FIPS) && \ 00040 (!defined(HAVE_FIPS_VERSION) || (HAVE_FIPS_VERSION < 2)) 00041 #define wc_Sha Sha 00042 #define WC_SHA SHA 00043 #define WC_SHA_BLOCK_SIZE SHA_BLOCK_SIZE 00044 #define WC_SHA_DIGEST_SIZE SHA_DIGEST_SIZE 00045 #define WC_SHA_PAD_SIZE SHA_PAD_SIZE 00046 00047 /* for fips @wc_fips */ 00048 #include <cyassl/ctaocrypt/sha.h> 00049 #endif 00050 00051 #ifdef FREESCALE_LTC_SHA 00052 #include "fsl_ltc.h" 00053 #endif 00054 00055 #ifdef __cplusplus 00056 extern "C" { 00057 #endif 00058 00059 /* avoid redefinition of structs */ 00060 #if !defined(HAVE_FIPS) || \ 00061 (defined(HAVE_FIPS_VERSION) && (HAVE_FIPS_VERSION >= 2)) 00062 00063 #ifdef WOLFSSL_MICROCHIP_PIC32MZ 00064 #include <wolfssl/wolfcrypt/port/pic32/pic32mz-crypt.h> 00065 #endif 00066 #ifdef STM32_HASH 00067 #include <wolfssl/wolfcrypt/port/st/stm32.h> 00068 #endif 00069 #ifdef WOLFSSL_ASYNC_CRYPT 00070 #include <wolfssl/wolfcrypt/async.h> 00071 #endif 00072 #ifdef WOLFSSL_ESP32WROOM32_CRYPT 00073 #include <wolfssl/wolfcrypt/port/Espressif/esp32-crypt.h> 00074 #endif 00075 00076 #if !defined(NO_OLD_SHA_NAMES) 00077 #define SHA WC_SHA 00078 #endif 00079 00080 #ifndef NO_OLD_WC_NAMES 00081 #define Sha wc_Sha 00082 #define SHA_BLOCK_SIZE WC_SHA_BLOCK_SIZE 00083 #define SHA_DIGEST_SIZE WC_SHA_DIGEST_SIZE 00084 #define SHA_PAD_SIZE WC_SHA_PAD_SIZE 00085 #endif 00086 00087 /* in bytes */ 00088 enum { 00089 WC_SHA = WC_HASH_TYPE_SHA, 00090 WC_SHA_BLOCK_SIZE = 64, 00091 WC_SHA_DIGEST_SIZE = 20, 00092 WC_SHA_PAD_SIZE = 56 00093 }; 00094 00095 00096 #if defined(WOLFSSL_TI_HASH) 00097 #include "wolfssl/wolfcrypt/port/ti/ti-hash.h" 00098 00099 #elif defined(WOLFSSL_IMX6_CAAM) 00100 #include "wolfssl/wolfcrypt/port/caam/wolfcaam_sha.h" 00101 #elif defined(WOLFSSL_RENESAS_TSIP_CRYPT) && \ 00102 !defined(NO_WOLFSSL_RENESAS_TSIP_CRYPT_HASH) 00103 #include "wolfssl/wolfcrypt/port/Renesas/renesas-tsip-crypt.h" 00104 #else 00105 00106 /* Sha digest */ 00107 struct wc_Sha { 00108 #ifdef FREESCALE_LTC_SHA 00109 ltc_hash_ctx_t ctx; 00110 #elif defined(STM32_HASH) 00111 STM32_HASH_Context stmCtx; 00112 #else 00113 word32 buffLen; /* in bytes */ 00114 word32 loLen; /* length in bytes */ 00115 word32 hiLen; /* length in bytes */ 00116 word32 buffer[WC_SHA_BLOCK_SIZE / sizeof(word32)]; 00117 #ifdef WOLFSSL_PIC32MZ_HASH 00118 word32 digest[PIC32_DIGEST_SIZE / sizeof(word32)]; 00119 #else 00120 word32 digest[WC_SHA_DIGEST_SIZE / sizeof(word32)]; 00121 #endif 00122 void* heap; 00123 #ifdef WOLFSSL_PIC32MZ_HASH 00124 hashUpdCache cache; /* cache for updates */ 00125 #endif 00126 #ifdef WOLFSSL_ASYNC_CRYPT 00127 WC_ASYNC_DEV asyncDev; 00128 #endif /* WOLFSSL_ASYNC_CRYPT */ 00129 #ifdef WOLF_CRYPTO_CB 00130 int devId; 00131 void* devCtx; /* generic crypto callback context */ 00132 #endif 00133 #endif 00134 #if defined(WOLFSSL_ESP32WROOM32_CRYPT) && \ 00135 !defined(NO_WOLFSSL_ESP32WROOM32_CRYPT_HASH) 00136 WC_ESP32SHA ctx; 00137 #endif 00138 #if defined(WOLFSSL_HASH_FLAGS) || defined(WOLF_CRYPTO_CB) 00139 word32 flags; /* enum wc_HashFlags in hash.h */ 00140 #endif 00141 }; 00142 00143 #ifndef WC_SHA_TYPE_DEFINED 00144 typedef struct wc_Sha wc_Sha; 00145 #define WC_SHA_TYPE_DEFINED 00146 #endif 00147 00148 #endif /* WOLFSSL_TI_HASH */ 00149 00150 00151 #endif /* HAVE_FIPS */ 00152 00153 WOLFSSL_API int wc_InitSha(wc_Sha*); 00154 WOLFSSL_API int wc_InitSha_ex(wc_Sha* sha, void* heap, int devId); 00155 WOLFSSL_API int wc_ShaUpdate(wc_Sha*, const byte*, word32); 00156 WOLFSSL_API int wc_ShaFinalRaw(wc_Sha*, byte*); 00157 WOLFSSL_API int wc_ShaFinal(wc_Sha*, byte*); 00158 WOLFSSL_API void wc_ShaFree(wc_Sha*); 00159 00160 WOLFSSL_API int wc_ShaGetHash(wc_Sha*, byte*); 00161 WOLFSSL_API int wc_ShaCopy(wc_Sha*, wc_Sha*); 00162 00163 #ifdef WOLFSSL_PIC32MZ_HASH 00164 WOLFSSL_API void wc_ShaSizeSet(wc_Sha* sha, word32 len); 00165 #endif 00166 00167 #if defined(WOLFSSL_HASH_FLAGS) || defined(WOLF_CRYPTO_CB) 00168 WOLFSSL_API int wc_ShaSetFlags(wc_Sha* sha, word32 flags); 00169 WOLFSSL_API int wc_ShaGetFlags(wc_Sha* sha, word32* flags); 00170 #endif 00171 00172 #ifdef __cplusplus 00173 } /* extern "C" */ 00174 #endif 00175 00176 #endif /* NO_SHA */ 00177 #endif /* WOLF_CRYPT_SHA_H */ 00178 00179
Generated on Tue Jul 12 2022 20:58:42 by
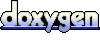