wolfSSL SSL/TLS library, support up to TLS1.3
Dependents: CyaSSL-Twitter-OAuth4Tw Example-client-tls-cert TwitterReader TweetTest ... more
sha3.h
00001 /* sha3.h 00002 * 00003 * Copyright (C) 2006-2020 wolfSSL Inc. 00004 * 00005 * This file is part of wolfSSL. 00006 * 00007 * wolfSSL is free software; you can redistribute it and/or modify 00008 * it under the terms of the GNU General Public License as published by 00009 * the Free Software Foundation; either version 2 of the License, or 00010 * (at your option) any later version. 00011 * 00012 * wolfSSL is distributed in the hope that it will be useful, 00013 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00014 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00015 * GNU General Public License for more details. 00016 * 00017 * You should have received a copy of the GNU General Public License 00018 * along with this program; if not, write to the Free Software 00019 * Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1335, USA 00020 */ 00021 00022 00023 #ifndef WOLF_CRYPT_SHA3_H 00024 #define WOLF_CRYPT_SHA3_H 00025 00026 #include <wolfssl/wolfcrypt/types.h > 00027 00028 #ifdef WOLFSSL_SHA3 00029 00030 #ifdef HAVE_FIPS 00031 /* for fips @wc_fips */ 00032 #include <wolfssl/wolfcrypt/fips.h> 00033 #endif 00034 00035 #ifdef __cplusplus 00036 extern "C" { 00037 #endif 00038 00039 #ifdef WOLFSSL_ASYNC_CRYPT 00040 #include <wolfssl/wolfcrypt/async.h> 00041 #endif 00042 00043 /* in bytes */ 00044 enum { 00045 WC_SHA3_224 = WC_HASH_TYPE_SHA3_224, 00046 WC_SHA3_224_DIGEST_SIZE = 28, 00047 WC_SHA3_224_COUNT = 18, 00048 00049 WC_SHA3_256 = WC_HASH_TYPE_SHA3_256, 00050 WC_SHA3_256_DIGEST_SIZE = 32, 00051 WC_SHA3_256_COUNT = 17, 00052 00053 WC_SHA3_384 = WC_HASH_TYPE_SHA3_384, 00054 WC_SHA3_384_DIGEST_SIZE = 48, 00055 WC_SHA3_384_COUNT = 13, 00056 00057 WC_SHA3_512 = WC_HASH_TYPE_SHA3_512, 00058 WC_SHA3_512_DIGEST_SIZE = 64, 00059 WC_SHA3_512_COUNT = 9, 00060 00061 #ifndef HAVE_SELFTEST 00062 /* These values are used for HMAC, not SHA-3 directly. 00063 * They come from from FIPS PUB 202. */ 00064 WC_SHA3_224_BLOCK_SIZE = 144, 00065 WC_SHA3_256_BLOCK_SIZE = 136, 00066 WC_SHA3_384_BLOCK_SIZE = 104, 00067 WC_SHA3_512_BLOCK_SIZE = 72, 00068 #endif 00069 }; 00070 00071 #ifndef NO_OLD_WC_NAMES 00072 #define SHA3_224 WC_SHA3_224 00073 #define SHA3_224_DIGEST_SIZE WC_SHA3_224_DIGEST_SIZE 00074 #define SHA3_256 WC_SHA3_256 00075 #define SHA3_256_DIGEST_SIZE WC_SHA3_256_DIGEST_SIZE 00076 #define SHA3_384 WC_SHA3_384 00077 #define SHA3_384_DIGEST_SIZE WC_SHA3_384_DIGEST_SIZE 00078 #define SHA3_512 WC_SHA3_512 00079 #define SHA3_512_DIGEST_SIZE WC_SHA3_512_DIGEST_SIZE 00080 #define Sha3 wc_Sha3 00081 #endif 00082 00083 00084 00085 #ifdef WOLFSSL_XILINX_CRYPT 00086 #include "wolfssl/wolfcrypt/port/xilinx/xil-sha3.h" 00087 #elif defined(WOLFSSL_AFALG_XILINX_SHA3) 00088 #include <wolfssl/wolfcrypt/port/af_alg/afalg_hash.h> 00089 #else 00090 00091 /* Sha3 digest */ 00092 struct Sha3 { 00093 /* State data that is processed for each block. */ 00094 word64 s[25]; 00095 /* Unprocessed message data. */ 00096 byte t[200]; 00097 /* Index into unprocessed data to place next message byte. */ 00098 byte i; 00099 00100 void* heap; 00101 00102 #ifdef WOLFSSL_ASYNC_CRYPT 00103 WC_ASYNC_DEV asyncDev; 00104 #endif /* WOLFSSL_ASYNC_CRYPT */ 00105 #if defined(WOLFSSL_HASH_FLAGS) || defined(WOLF_CRYPTO_CB) 00106 word32 flags; /* enum wc_HashFlags in hash.h */ 00107 #endif 00108 }; 00109 00110 #ifndef WC_SHA3_TYPE_DEFINED 00111 typedef struct Sha3 wc_Sha3; 00112 #define WC_SHA3_TYPE_DEFINED 00113 #endif 00114 00115 #endif 00116 00117 typedef wc_Sha3 wc_Shake; 00118 00119 00120 WOLFSSL_API int wc_InitSha3_224(wc_Sha3*, void*, int); 00121 WOLFSSL_API int wc_Sha3_224_Update(wc_Sha3*, const byte*, word32); 00122 WOLFSSL_API int wc_Sha3_224_Final(wc_Sha3*, byte*); 00123 WOLFSSL_API void wc_Sha3_224_Free(wc_Sha3*); 00124 WOLFSSL_API int wc_Sha3_224_GetHash(wc_Sha3*, byte*); 00125 WOLFSSL_API int wc_Sha3_224_Copy(wc_Sha3* src, wc_Sha3* dst); 00126 00127 WOLFSSL_API int wc_InitSha3_256(wc_Sha3*, void*, int); 00128 WOLFSSL_API int wc_Sha3_256_Update(wc_Sha3*, const byte*, word32); 00129 WOLFSSL_API int wc_Sha3_256_Final(wc_Sha3*, byte*); 00130 WOLFSSL_API void wc_Sha3_256_Free(wc_Sha3*); 00131 WOLFSSL_API int wc_Sha3_256_GetHash(wc_Sha3*, byte*); 00132 WOLFSSL_API int wc_Sha3_256_Copy(wc_Sha3* src, wc_Sha3* dst); 00133 00134 WOLFSSL_API int wc_InitSha3_384(wc_Sha3*, void*, int); 00135 WOLFSSL_API int wc_Sha3_384_Update(wc_Sha3*, const byte*, word32); 00136 WOLFSSL_API int wc_Sha3_384_Final(wc_Sha3*, byte*); 00137 WOLFSSL_API void wc_Sha3_384_Free(wc_Sha3*); 00138 WOLFSSL_API int wc_Sha3_384_GetHash(wc_Sha3*, byte*); 00139 WOLFSSL_API int wc_Sha3_384_Copy(wc_Sha3* src, wc_Sha3* dst); 00140 00141 WOLFSSL_API int wc_InitSha3_512(wc_Sha3*, void*, int); 00142 WOLFSSL_API int wc_Sha3_512_Update(wc_Sha3*, const byte*, word32); 00143 WOLFSSL_API int wc_Sha3_512_Final(wc_Sha3*, byte*); 00144 WOLFSSL_API void wc_Sha3_512_Free(wc_Sha3*); 00145 WOLFSSL_API int wc_Sha3_512_GetHash(wc_Sha3*, byte*); 00146 WOLFSSL_API int wc_Sha3_512_Copy(wc_Sha3* src, wc_Sha3* dst); 00147 00148 WOLFSSL_API int wc_InitShake256(wc_Shake*, void*, int); 00149 WOLFSSL_API int wc_Shake256_Update(wc_Shake*, const byte*, word32); 00150 WOLFSSL_API int wc_Shake256_Final(wc_Shake*, byte*, word32); 00151 WOLFSSL_API void wc_Shake256_Free(wc_Shake*); 00152 WOLFSSL_API int wc_Shake256_Copy(wc_Shake* src, wc_Sha3* dst); 00153 00154 #if defined(WOLFSSL_HASH_FLAGS) || defined(WOLF_CRYPTO_CB) 00155 WOLFSSL_API int wc_Sha3_SetFlags(wc_Sha3* sha3, word32 flags); 00156 WOLFSSL_API int wc_Sha3_GetFlags(wc_Sha3* sha3, word32* flags); 00157 #endif 00158 00159 #ifdef __cplusplus 00160 } /* extern "C" */ 00161 #endif 00162 00163 #endif /* WOLFSSL_SHA3 */ 00164 #endif /* WOLF_CRYPT_SHA3_H */ 00165 00166
Generated on Tue Jul 12 2022 20:58:42 by
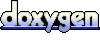