wolfSSL SSL/TLS library, support up to TLS1.3
Dependents: CyaSSL-Twitter-OAuth4Tw Example-client-tls-cert TwitterReader TweetTest ... more
aes.h
00001 /* aes.h 00002 * 00003 * Copyright (C) 2006-2020 wolfSSL Inc. 00004 * 00005 * This file is part of wolfSSL. 00006 * 00007 * wolfSSL is free software; you can redistribute it and/or modify 00008 * it under the terms of the GNU General Public License as published by 00009 * the Free Software Foundation; either version 2 of the License, or 00010 * (at your option) any later version. 00011 * 00012 * wolfSSL is distributed in the hope that it will be useful, 00013 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00014 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00015 * GNU General Public License for more details. 00016 * 00017 * You should have received a copy of the GNU General Public License 00018 * along with this program; if not, write to the Free Software 00019 * Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1335, USA 00020 */ 00021 00022 /*! 00023 \file wolfssl/wolfcrypt/aes.h 00024 */ 00025 00026 00027 #ifndef WOLF_CRYPT_AES_H 00028 #define WOLF_CRYPT_AES_H 00029 00030 #include <wolfssl/wolfcrypt/types.h > 00031 00032 #ifndef NO_AES 00033 00034 #if defined(HAVE_FIPS) && \ 00035 defined(HAVE_FIPS_VERSION) && (HAVE_FIPS_VERSION >= 2) 00036 #include <wolfssl/wolfcrypt/fips.h> 00037 #endif /* HAVE_FIPS_VERSION >= 2 */ 00038 00039 /* included for fips @wc_fips */ 00040 #if defined(HAVE_FIPS) && \ 00041 (!defined(HAVE_FIPS_VERSION) || (HAVE_FIPS_VERSION < 2)) 00042 #include <cyassl/ctaocrypt/aes.h> 00043 #if defined(CYASSL_AES_COUNTER) && !defined(WOLFSSL_AES_COUNTER) 00044 #define WOLFSSL_AES_COUNTER 00045 #endif 00046 #if !defined(WOLFSSL_AES_DIRECT) && defined(CYASSL_AES_DIRECT) 00047 #define WOLFSSL_AES_DIRECT 00048 #endif 00049 #endif 00050 00051 #ifndef WC_NO_RNG 00052 #include <wolfssl/wolfcrypt/random.h > 00053 #endif 00054 #ifdef STM32_CRYPTO 00055 #include <wolfssl/wolfcrypt/port/st/stm32.h> 00056 #endif 00057 00058 #ifdef WOLFSSL_AESNI 00059 00060 #include <wmmintrin.h> 00061 #include <emmintrin.h> 00062 #include <smmintrin.h> 00063 00064 #endif /* WOLFSSL_AESNI */ 00065 00066 00067 #ifdef WOLFSSL_XILINX_CRYPT 00068 #include "xsecure_aes.h" 00069 #endif 00070 00071 #if defined(WOLFSSL_AFALG) || defined(WOLFSSL_AFALG_XILINX_AES) 00072 /* included for struct msghdr */ 00073 #include <wolfssl/wolfcrypt/port/af_alg/wc_afalg.h> 00074 #endif 00075 00076 #if defined(WOLFSSL_DEVCRYPTO_AES) || defined(WOLFSSL_DEVCRYPTO_CBC) 00077 #include <wolfssl/wolfcrypt/port/devcrypto/wc_devcrypto.h> 00078 #endif 00079 00080 #if defined(HAVE_AESGCM) && !defined(WC_NO_RNG) 00081 #include <wolfssl/wolfcrypt/random.h > 00082 #endif 00083 00084 #if defined(WOLFSSL_CRYPTOCELL) 00085 #include <wolfssl/wolfcrypt/port/arm/cryptoCell.h> 00086 #endif 00087 00088 #if defined(WOLFSSL_RENESAS_TSIP_TLS) && \ 00089 defined(WOLFSSL_RENESAS_TSIP_TLS_AES_CRYPT) 00090 #include <wolfssl/wolfcrypt/port/Renesas/renesas-tsip-crypt.h> 00091 #endif 00092 00093 #ifdef __cplusplus 00094 extern "C" { 00095 #endif 00096 00097 #ifndef WOLFSSL_AES_KEY_SIZE_ENUM 00098 #define WOLFSSL_AES_KEY_SIZE_ENUM 00099 /* these are required for FIPS and non-FIPS */ 00100 enum { 00101 AES_128_KEY_SIZE = 16, /* for 128 bit */ 00102 AES_192_KEY_SIZE = 24, /* for 192 bit */ 00103 AES_256_KEY_SIZE = 32, /* for 256 bit */ 00104 00105 AES_IV_SIZE = 16, /* always block size */ 00106 }; 00107 #endif 00108 00109 /* avoid redefinition of structs */ 00110 #if !defined(HAVE_FIPS) || \ 00111 (defined(HAVE_FIPS_VERSION) && (HAVE_FIPS_VERSION >= 2)) 00112 00113 #ifdef WOLFSSL_ASYNC_CRYPT 00114 #include <wolfssl/wolfcrypt/async.h> 00115 #endif 00116 00117 enum { 00118 AES_ENC_TYPE = WC_CIPHER_AES, /* cipher unique type */ 00119 AES_ENCRYPTION = 0, 00120 AES_DECRYPTION = 1, 00121 00122 AES_BLOCK_SIZE = 16, 00123 00124 KEYWRAP_BLOCK_SIZE = 8, 00125 00126 GCM_NONCE_MAX_SZ = 16, /* wolfCrypt's maximum nonce size allowed. */ 00127 GCM_NONCE_MID_SZ = 12, /* The usual default nonce size for AES-GCM. */ 00128 GCM_NONCE_MIN_SZ = 8, /* wolfCrypt's minimum nonce size allowed. */ 00129 CCM_NONCE_MIN_SZ = 7, 00130 CCM_NONCE_MAX_SZ = 13, 00131 CTR_SZ = 4, 00132 AES_IV_FIXED_SZ = 4, 00133 #ifdef WOLFSSL_AES_CFB 00134 AES_CFB_MODE = 1, 00135 #endif 00136 #ifdef WOLFSSL_AES_OFB 00137 AES_OFB_MODE = 2, 00138 #endif 00139 #ifdef WOLFSSL_AES_XTS 00140 AES_XTS_MODE = 3, 00141 #endif 00142 00143 #ifdef HAVE_PKCS11 00144 AES_MAX_ID_LEN = 32, 00145 #endif 00146 }; 00147 00148 00149 struct Aes { 00150 /* AESNI needs key first, rounds 2nd, not sure why yet */ 00151 ALIGN16 word32 key[60]; 00152 word32 rounds; 00153 int keylen; 00154 00155 ALIGN16 word32 reg[AES_BLOCK_SIZE / sizeof(word32)]; /* for CBC mode */ 00156 ALIGN16 word32 tmp[AES_BLOCK_SIZE / sizeof(word32)]; /* same */ 00157 00158 #if defined(HAVE_AESGCM) || defined(HAVE_AESCCM) 00159 word32 invokeCtr[2]; 00160 word32 nonceSz; 00161 #endif 00162 #ifdef HAVE_AESGCM 00163 ALIGN16 byte H[AES_BLOCK_SIZE]; 00164 #ifdef OPENSSL_EXTRA 00165 word32 aadH[4]; /* additional authenticated data GHASH */ 00166 word32 aadLen; /* additional authenticated data len */ 00167 #endif 00168 00169 #ifdef GCM_TABLE 00170 /* key-based fast multiplication table. */ 00171 ALIGN16 byte M0[256][AES_BLOCK_SIZE]; 00172 #endif /* GCM_TABLE */ 00173 #ifdef HAVE_CAVIUM_OCTEON_SYNC 00174 word32 y0; 00175 #endif 00176 #endif /* HAVE_AESGCM */ 00177 #ifdef WOLFSSL_AESNI 00178 byte use_aesni; 00179 #endif /* WOLFSSL_AESNI */ 00180 #ifdef WOLF_CRYPTO_CB 00181 int devId; 00182 void* devCtx; 00183 #endif 00184 #ifdef HAVE_PKCS11 00185 byte id[AES_MAX_ID_LEN]; 00186 int idLen; 00187 #endif 00188 #ifdef WOLFSSL_ASYNC_CRYPT 00189 WC_ASYNC_DEV asyncDev; 00190 #endif /* WOLFSSL_ASYNC_CRYPT */ 00191 #if defined(WOLFSSL_AES_COUNTER) || defined(WOLFSSL_AES_CFB) || \ 00192 defined(WOLFSSL_AES_OFB) || defined(WOLFSSL_AES_XTS) 00193 word32 left; /* unused bytes left from last call */ 00194 #endif 00195 #ifdef WOLFSSL_XILINX_CRYPT 00196 XSecure_Aes xilAes; 00197 XCsuDma dma; 00198 word32 key_init[8]; 00199 word32 kup; 00200 #endif 00201 #if defined(WOLFSSL_AFALG) || defined(WOLFSSL_AFALG_XILINX_AES) 00202 int alFd; /* server socket to bind to */ 00203 int rdFd; /* socket to read from */ 00204 struct msghdr msg; 00205 int dir; /* flag for encrpyt or decrypt */ 00206 #ifdef WOLFSSL_AFALG_XILINX_AES 00207 word32 msgBuf[CMSG_SPACE(4) + CMSG_SPACE(sizeof(struct af_alg_iv) + 00208 GCM_NONCE_MID_SZ)]; 00209 #endif 00210 #endif 00211 #if defined(WOLF_CRYPTO_CB) || (defined(WOLFSSL_DEVCRYPTO) && \ 00212 (defined(WOLFSSL_DEVCRYPTO_AES) || defined(WOLFSSL_DEVCRYPTO_CBC))) || \ 00213 (defined(WOLFSSL_ASYNC_CRYPT) && defined(WC_ASYNC_ENABLE_AES)) 00214 word32 devKey[AES_MAX_KEY_SIZE/WOLFSSL_BIT_SIZE/sizeof(word32)]; /* raw key */ 00215 #ifdef HAVE_CAVIUM_OCTEON_SYNC 00216 int keySet; 00217 #endif 00218 #endif 00219 #if defined(WOLFSSL_DEVCRYPTO) && \ 00220 (defined(WOLFSSL_DEVCRYPTO_AES) || defined(WOLFSSL_DEVCRYPTO_CBC)) 00221 WC_CRYPTODEV ctx; 00222 #endif 00223 #if defined(WOLFSSL_CRYPTOCELL) 00224 aes_context_t ctx; 00225 #endif 00226 #if defined(WOLFSSL_RENESAS_TSIP_TLS) && \ 00227 defined(WOLFSSL_RENESAS_TSIP_TLS_AES_CRYPT) 00228 TSIP_AES_CTX ctx; 00229 #endif 00230 void* heap; /* memory hint to use */ 00231 }; 00232 00233 #ifndef WC_AES_TYPE_DEFINED 00234 typedef struct Aes Aes; 00235 #define WC_AES_TYPE_DEFINED 00236 #endif 00237 00238 #ifdef WOLFSSL_AES_XTS 00239 typedef struct XtsAes { 00240 Aes aes; 00241 Aes tweak; 00242 } XtsAes; 00243 #endif 00244 00245 #ifdef HAVE_AESGCM 00246 typedef struct Gmac { 00247 Aes aes; 00248 } Gmac; 00249 #endif /* HAVE_AESGCM */ 00250 #endif /* HAVE_FIPS */ 00251 00252 00253 /* Authenticate cipher function prototypes */ 00254 typedef int (*wc_AesAuthEncryptFunc)(Aes* aes, byte* out, 00255 const byte* in, word32 sz, 00256 const byte* iv, word32 ivSz, 00257 byte* authTag, word32 authTagSz, 00258 const byte* authIn, word32 authInSz); 00259 typedef int (*wc_AesAuthDecryptFunc)(Aes* aes, byte* out, 00260 const byte* in, word32 sz, 00261 const byte* iv, word32 ivSz, 00262 const byte* authTag, word32 authTagSz, 00263 const byte* authIn, word32 authInSz); 00264 00265 /* AES-CBC */ 00266 WOLFSSL_API int wc_AesSetKey(Aes* aes, const byte* key, word32 len, 00267 const byte* iv, int dir); 00268 WOLFSSL_API int wc_AesSetIV(Aes* aes, const byte* iv); 00269 00270 #ifdef HAVE_AES_CBC 00271 WOLFSSL_API int wc_AesCbcEncrypt(Aes* aes, byte* out, 00272 const byte* in, word32 sz); 00273 WOLFSSL_API int wc_AesCbcDecrypt(Aes* aes, byte* out, 00274 const byte* in, word32 sz); 00275 #endif 00276 00277 #ifdef WOLFSSL_AES_CFB 00278 WOLFSSL_API int wc_AesCfbEncrypt(Aes* aes, byte* out, 00279 const byte* in, word32 sz); 00280 WOLFSSL_API int wc_AesCfb1Encrypt(Aes* aes, byte* out, 00281 const byte* in, word32 sz); 00282 WOLFSSL_API int wc_AesCfb8Encrypt(Aes* aes, byte* out, 00283 const byte* in, word32 sz); 00284 #ifdef HAVE_AES_DECRYPT 00285 WOLFSSL_API int wc_AesCfbDecrypt(Aes* aes, byte* out, 00286 const byte* in, word32 sz); 00287 WOLFSSL_API int wc_AesCfb1Decrypt(Aes* aes, byte* out, 00288 const byte* in, word32 sz); 00289 WOLFSSL_API int wc_AesCfb8Decrypt(Aes* aes, byte* out, 00290 const byte* in, word32 sz); 00291 #endif /* HAVE_AES_DECRYPT */ 00292 #endif /* WOLFSSL_AES_CFB */ 00293 00294 #ifdef WOLFSSL_AES_OFB 00295 WOLFSSL_API int wc_AesOfbEncrypt(Aes* aes, byte* out, 00296 const byte* in, word32 sz); 00297 #ifdef HAVE_AES_DECRYPT 00298 WOLFSSL_API int wc_AesOfbDecrypt(Aes* aes, byte* out, 00299 const byte* in, word32 sz); 00300 #endif /* HAVE_AES_DECRYPT */ 00301 #endif /* WOLFSSL_AES_OFB */ 00302 00303 #ifdef HAVE_AES_ECB 00304 WOLFSSL_API int wc_AesEcbEncrypt(Aes* aes, byte* out, 00305 const byte* in, word32 sz); 00306 WOLFSSL_API int wc_AesEcbDecrypt(Aes* aes, byte* out, 00307 const byte* in, word32 sz); 00308 #endif 00309 00310 /* AES-CTR */ 00311 #ifdef WOLFSSL_AES_COUNTER 00312 WOLFSSL_API int wc_AesCtrEncrypt(Aes* aes, byte* out, 00313 const byte* in, word32 sz); 00314 #endif 00315 /* AES-DIRECT */ 00316 #if defined(WOLFSSL_AES_DIRECT) 00317 WOLFSSL_API void wc_AesEncryptDirect(Aes* aes, byte* out, const byte* in); 00318 WOLFSSL_API void wc_AesDecryptDirect(Aes* aes, byte* out, const byte* in); 00319 WOLFSSL_API int wc_AesSetKeyDirect(Aes* aes, const byte* key, word32 len, 00320 const byte* iv, int dir); 00321 #endif 00322 00323 #ifdef HAVE_AESGCM 00324 #ifdef WOLFSSL_XILINX_CRYPT 00325 WOLFSSL_API int wc_AesGcmSetKey_ex(Aes* aes, const byte* key, word32 len, 00326 word32 kup); 00327 #elif defined(WOLFSSL_AFALG_XILINX_AES) 00328 WOLFSSL_LOCAL int wc_AesGcmSetKey_ex(Aes* aes, const byte* key, word32 len, 00329 word32 kup); 00330 #endif 00331 WOLFSSL_API int wc_AesGcmSetKey(Aes* aes, const byte* key, word32 len); 00332 WOLFSSL_API int wc_AesGcmEncrypt(Aes* aes, byte* out, 00333 const byte* in, word32 sz, 00334 const byte* iv, word32 ivSz, 00335 byte* authTag, word32 authTagSz, 00336 const byte* authIn, word32 authInSz); 00337 WOLFSSL_API int wc_AesGcmDecrypt(Aes* aes, byte* out, 00338 const byte* in, word32 sz, 00339 const byte* iv, word32 ivSz, 00340 const byte* authTag, word32 authTagSz, 00341 const byte* authIn, word32 authInSz); 00342 00343 #ifndef WC_NO_RNG 00344 WOLFSSL_API int wc_AesGcmSetExtIV(Aes* aes, const byte* iv, word32 ivSz); 00345 WOLFSSL_API int wc_AesGcmSetIV(Aes* aes, word32 ivSz, 00346 const byte* ivFixed, word32 ivFixedSz, 00347 WC_RNG* rng); 00348 WOLFSSL_API int wc_AesGcmEncrypt_ex(Aes* aes, byte* out, 00349 const byte* in, word32 sz, 00350 byte* ivOut, word32 ivOutSz, 00351 byte* authTag, word32 authTagSz, 00352 const byte* authIn, word32 authInSz); 00353 #endif /* WC_NO_RNG */ 00354 00355 WOLFSSL_API int wc_GmacSetKey(Gmac* gmac, const byte* key, word32 len); 00356 WOLFSSL_API int wc_GmacUpdate(Gmac* gmac, const byte* iv, word32 ivSz, 00357 const byte* authIn, word32 authInSz, 00358 byte* authTag, word32 authTagSz); 00359 #ifndef WC_NO_RNG 00360 WOLFSSL_API int wc_Gmac(const byte* key, word32 keySz, byte* iv, word32 ivSz, 00361 const byte* authIn, word32 authInSz, 00362 byte* authTag, word32 authTagSz, WC_RNG* rng); 00363 WOLFSSL_API int wc_GmacVerify(const byte* key, word32 keySz, 00364 const byte* iv, word32 ivSz, 00365 const byte* authIn, word32 authInSz, 00366 const byte* authTag, word32 authTagSz); 00367 #endif /* WC_NO_RNG */ 00368 WOLFSSL_LOCAL void GHASH(Aes* aes, const byte* a, word32 aSz, const byte* c, 00369 word32 cSz, byte* s, word32 sSz); 00370 #endif /* HAVE_AESGCM */ 00371 #ifdef HAVE_AESCCM 00372 WOLFSSL_API int wc_AesCcmSetKey(Aes* aes, const byte* key, word32 keySz); 00373 WOLFSSL_API int wc_AesCcmEncrypt(Aes* aes, byte* out, 00374 const byte* in, word32 inSz, 00375 const byte* nonce, word32 nonceSz, 00376 byte* authTag, word32 authTagSz, 00377 const byte* authIn, word32 authInSz); 00378 WOLFSSL_API int wc_AesCcmDecrypt(Aes* aes, byte* out, 00379 const byte* in, word32 inSz, 00380 const byte* nonce, word32 nonceSz, 00381 const byte* authTag, word32 authTagSz, 00382 const byte* authIn, word32 authInSz); 00383 WOLFSSL_API int wc_AesCcmSetNonce(Aes* aes, 00384 const byte* nonce, word32 nonceSz); 00385 WOLFSSL_API int wc_AesCcmEncrypt_ex(Aes* aes, byte* out, 00386 const byte* in, word32 sz, 00387 byte* ivOut, word32 ivOutSz, 00388 byte* authTag, word32 authTagSz, 00389 const byte* authIn, word32 authInSz); 00390 #endif /* HAVE_AESCCM */ 00391 #ifdef HAVE_AES_KEYWRAP 00392 WOLFSSL_API int wc_AesKeyWrap(const byte* key, word32 keySz, 00393 const byte* in, word32 inSz, 00394 byte* out, word32 outSz, 00395 const byte* iv); 00396 WOLFSSL_API int wc_AesKeyUnWrap(const byte* key, word32 keySz, 00397 const byte* in, word32 inSz, 00398 byte* out, word32 outSz, 00399 const byte* iv); 00400 #endif /* HAVE_AES_KEYWRAP */ 00401 00402 #ifdef WOLFSSL_AES_XTS 00403 00404 WOLFSSL_API int wc_AesXtsSetKey(XtsAes* aes, const byte* key, 00405 word32 len, int dir, void* heap, int devId); 00406 00407 WOLFSSL_API int wc_AesXtsEncryptSector(XtsAes* aes, byte* out, 00408 const byte* in, word32 sz, word64 sector); 00409 00410 WOLFSSL_API int wc_AesXtsDecryptSector(XtsAes* aes, byte* out, 00411 const byte* in, word32 sz, word64 sector); 00412 00413 WOLFSSL_API int wc_AesXtsEncrypt(XtsAes* aes, byte* out, 00414 const byte* in, word32 sz, const byte* i, word32 iSz); 00415 00416 WOLFSSL_API int wc_AesXtsDecrypt(XtsAes* aes, byte* out, 00417 const byte* in, word32 sz, const byte* i, word32 iSz); 00418 00419 WOLFSSL_API int wc_AesXtsFree(XtsAes* aes); 00420 #endif 00421 00422 WOLFSSL_API int wc_AesGetKeySize(Aes* aes, word32* keySize); 00423 00424 WOLFSSL_API int wc_AesInit(Aes* aes, void* heap, int devId); 00425 #ifdef HAVE_PKCS11 00426 WOLFSSL_API int wc_AesInit_Id(Aes* aes, unsigned char* id, int len, void* heap, 00427 int devId); 00428 #endif 00429 WOLFSSL_API void wc_AesFree(Aes* aes); 00430 00431 #ifdef __cplusplus 00432 } /* extern "C" */ 00433 #endif 00434 00435 00436 #endif /* NO_AES */ 00437 #endif /* WOLF_CRYPT_AES_H */ 00438
Generated on Tue Jul 12 2022 20:58:32 by
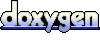