wolfSSL SSL/TLS library, support up to TLS1.3
Dependents: CyaSSL-Twitter-OAuth4Tw Example-client-tls-cert TwitterReader TweetTest ... more
wc_encrypt.c
00001 /* wc_encrypt.c 00002 * 00003 * Copyright (C) 2006-2020 wolfSSL Inc. 00004 * 00005 * This file is part of wolfSSL. 00006 * 00007 * wolfSSL is free software; you can redistribute it and/or modify 00008 * it under the terms of the GNU General Public License as published by 00009 * the Free Software Foundation; either version 2 of the License, or 00010 * (at your option) any later version. 00011 * 00012 * wolfSSL is distributed in the hope that it will be useful, 00013 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00014 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00015 * GNU General Public License for more details. 00016 * 00017 * You should have received a copy of the GNU General Public License 00018 * along with this program; if not, write to the Free Software 00019 * Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1335, USA 00020 */ 00021 00022 00023 #ifdef HAVE_CONFIG_H 00024 #include <config.h> 00025 #endif 00026 00027 #include <wolfssl/wolfcrypt/settings.h> 00028 #include <wolfssl/wolfcrypt/aes.h > 00029 #include <wolfssl/wolfcrypt/des3.h > 00030 #include <wolfssl/wolfcrypt/hash.h > 00031 #include <wolfssl/wolfcrypt/arc4.h > 00032 #include <wolfssl/wolfcrypt/wc_encrypt.h > 00033 #include <wolfssl/wolfcrypt/error-crypt.h > 00034 #include <wolfssl/wolfcrypt/asn.h > 00035 #include <wolfssl/wolfcrypt/coding.h > 00036 #include <wolfssl/wolfcrypt/pwdbased.h > 00037 #include <wolfssl/wolfcrypt/logging.h > 00038 00039 #ifdef NO_INLINE 00040 #include <wolfssl/wolfcrypt/misc.h> 00041 #else 00042 #define WOLFSSL_MISC_INCLUDED 00043 #include <wolfcrypt/src/misc.c> 00044 #endif 00045 00046 #if !defined(NO_AES) && defined(HAVE_AES_CBC) 00047 #ifdef HAVE_AES_DECRYPT 00048 int wc_AesCbcDecryptWithKey(byte* out, const byte* in, word32 inSz, 00049 const byte* key, word32 keySz, const byte* iv) 00050 { 00051 int ret = 0; 00052 #ifdef WOLFSSL_SMALL_STACK 00053 Aes* aes = NULL; 00054 #else 00055 Aes aes[1]; 00056 #endif 00057 00058 if (out == NULL || in == NULL || key == NULL || iv == NULL) { 00059 return BAD_FUNC_ARG; 00060 } 00061 00062 #ifdef WOLFSSL_SMALL_STACK 00063 aes = (Aes*)XMALLOC(sizeof(Aes), NULL, DYNAMIC_TYPE_TMP_BUFFER); 00064 if (aes == NULL) 00065 return MEMORY_E; 00066 #endif 00067 00068 ret = wc_AesInit(aes, NULL, INVALID_DEVID); 00069 if (ret == 0) { 00070 ret = wc_AesSetKey(aes, key, keySz, iv, AES_DECRYPTION); 00071 if (ret == 0) 00072 ret = wc_AesCbcDecrypt(aes, out, in, inSz); 00073 00074 wc_AesFree(aes); 00075 } 00076 00077 #ifdef WOLFSSL_SMALL_STACK 00078 XFREE(aes, NULL, DYNAMIC_TYPE_TMP_BUFFER); 00079 #endif 00080 00081 return ret; 00082 } 00083 #endif /* HAVE_AES_DECRYPT */ 00084 00085 int wc_AesCbcEncryptWithKey(byte* out, const byte* in, word32 inSz, 00086 const byte* key, word32 keySz, const byte* iv) 00087 { 00088 int ret = 0; 00089 #ifdef WOLFSSL_SMALL_STACK 00090 Aes* aes; 00091 #else 00092 Aes aes[1]; 00093 #endif 00094 00095 #ifdef WOLFSSL_SMALL_STACK 00096 aes = (Aes*)XMALLOC(sizeof(Aes), NULL, DYNAMIC_TYPE_TMP_BUFFER); 00097 if (aes == NULL) 00098 return MEMORY_E; 00099 #endif 00100 00101 ret = wc_AesInit(aes, NULL, INVALID_DEVID); 00102 if (ret == 0) { 00103 ret = wc_AesSetKey(aes, key, keySz, iv, AES_ENCRYPTION); 00104 if (ret == 0) 00105 ret = wc_AesCbcEncrypt(aes, out, in, inSz); 00106 00107 wc_AesFree(aes); 00108 } 00109 00110 #ifdef WOLFSSL_SMALL_STACK 00111 XFREE(aes, NULL, DYNAMIC_TYPE_TMP_BUFFER); 00112 #endif 00113 00114 return ret; 00115 } 00116 #endif /* !NO_AES && HAVE_AES_CBC */ 00117 00118 00119 #if !defined(NO_DES3) && !defined(WOLFSSL_TI_CRYPT) 00120 int wc_Des_CbcEncryptWithKey(byte* out, const byte* in, word32 sz, 00121 const byte* key, const byte* iv) 00122 { 00123 int ret = 0; 00124 #ifdef WOLFSSL_SMALL_STACK 00125 Des* des; 00126 #else 00127 Des des[1]; 00128 #endif 00129 00130 #ifdef WOLFSSL_SMALL_STACK 00131 des = (Des*)XMALLOC(sizeof(Des), NULL, DYNAMIC_TYPE_TMP_BUFFER); 00132 if (des == NULL) 00133 return MEMORY_E; 00134 #endif 00135 00136 ret = wc_Des_SetKey(des, key, iv, DES_ENCRYPTION); 00137 if (ret == 0) 00138 ret = wc_Des_CbcEncrypt(des, out, in, sz); 00139 00140 #ifdef WOLFSSL_SMALL_STACK 00141 XFREE(des, NULL, DYNAMIC_TYPE_TMP_BUFFER); 00142 #endif 00143 00144 return ret; 00145 } 00146 00147 int wc_Des_CbcDecryptWithKey(byte* out, const byte* in, word32 sz, 00148 const byte* key, const byte* iv) 00149 { 00150 int ret = 0; 00151 #ifdef WOLFSSL_SMALL_STACK 00152 Des* des; 00153 #else 00154 Des des[1]; 00155 #endif 00156 00157 #ifdef WOLFSSL_SMALL_STACK 00158 des = (Des*)XMALLOC(sizeof(Des), NULL, DYNAMIC_TYPE_TMP_BUFFER); 00159 if (des == NULL) 00160 return MEMORY_E; 00161 #endif 00162 00163 ret = wc_Des_SetKey(des, key, iv, DES_DECRYPTION); 00164 if (ret == 0) 00165 ret = wc_Des_CbcDecrypt(des, out, in, sz); 00166 00167 #ifdef WOLFSSL_SMALL_STACK 00168 XFREE(des, NULL, DYNAMIC_TYPE_TMP_BUFFER); 00169 #endif 00170 00171 return ret; 00172 } 00173 00174 00175 int wc_Des3_CbcEncryptWithKey(byte* out, const byte* in, word32 sz, 00176 const byte* key, const byte* iv) 00177 { 00178 int ret = 0; 00179 #ifdef WOLFSSL_SMALL_STACK 00180 Des3* des3; 00181 #else 00182 Des3 des3[1]; 00183 #endif 00184 00185 #ifdef WOLFSSL_SMALL_STACK 00186 des3 = (Des3*)XMALLOC(sizeof(Des3), NULL, DYNAMIC_TYPE_TMP_BUFFER); 00187 if (des3 == NULL) 00188 return MEMORY_E; 00189 #endif 00190 00191 ret = wc_Des3Init(des3, NULL, INVALID_DEVID); 00192 if (ret == 0) { 00193 ret = wc_Des3_SetKey(des3, key, iv, DES_ENCRYPTION); 00194 if (ret == 0) 00195 ret = wc_Des3_CbcEncrypt(des3, out, in, sz); 00196 wc_Des3Free(des3); 00197 } 00198 00199 #ifdef WOLFSSL_SMALL_STACK 00200 XFREE(des3, NULL, DYNAMIC_TYPE_TMP_BUFFER); 00201 #endif 00202 00203 return ret; 00204 } 00205 00206 00207 int wc_Des3_CbcDecryptWithKey(byte* out, const byte* in, word32 sz, 00208 const byte* key, const byte* iv) 00209 { 00210 int ret = 0; 00211 #ifdef WOLFSSL_SMALL_STACK 00212 Des3* des3; 00213 #else 00214 Des3 des3[1]; 00215 #endif 00216 00217 #ifdef WOLFSSL_SMALL_STACK 00218 des3 = (Des3*)XMALLOC(sizeof(Des3), NULL, DYNAMIC_TYPE_TMP_BUFFER); 00219 if (des3 == NULL) 00220 return MEMORY_E; 00221 #endif 00222 00223 ret = wc_Des3Init(des3, NULL, INVALID_DEVID); 00224 if (ret == 0) { 00225 ret = wc_Des3_SetKey(des3, key, iv, DES_DECRYPTION); 00226 if (ret == 0) 00227 ret = wc_Des3_CbcDecrypt(des3, out, in, sz); 00228 wc_Des3Free(des3); 00229 } 00230 00231 #ifdef WOLFSSL_SMALL_STACK 00232 XFREE(des3, NULL, DYNAMIC_TYPE_TMP_BUFFER); 00233 #endif 00234 00235 return ret; 00236 } 00237 00238 #endif /* !NO_DES3 */ 00239 00240 00241 #ifdef WOLFSSL_ENCRYPTED_KEYS 00242 00243 int wc_BufferKeyDecrypt(EncryptedInfo* info, byte* der, word32 derSz, 00244 const byte* password, int passwordSz, int hashType) 00245 { 00246 int ret = NOT_COMPILED_IN; 00247 #ifdef WOLFSSL_SMALL_STACK 00248 byte* key = NULL; 00249 #else 00250 byte key[WC_MAX_SYM_KEY_SIZE]; 00251 #endif 00252 00253 (void)derSz; 00254 (void)passwordSz; 00255 (void)hashType; 00256 00257 if (der == NULL || password == NULL || info == NULL || info->keySz == 0) { 00258 return BAD_FUNC_ARG; 00259 } 00260 00261 /* use file's salt for key derivation, hex decode first */ 00262 if (Base16_Decode(info->iv, info->ivSz, info->iv, &info->ivSz) != 0) { 00263 return BUFFER_E; 00264 } 00265 if (info->ivSz < PKCS5_SALT_SZ) 00266 return BUFFER_E; 00267 00268 #ifdef WOLFSSL_SMALL_STACK 00269 key = (byte*)XMALLOC(WC_MAX_SYM_KEY_SIZE, NULL, DYNAMIC_TYPE_SYMMETRIC_KEY); 00270 if (key == NULL) { 00271 return MEMORY_E; 00272 } 00273 #endif 00274 00275 (void)XMEMSET(key, 0, WC_MAX_SYM_KEY_SIZE); 00276 00277 #ifndef NO_PWDBASED 00278 if ((ret = wc_PBKDF1(key, password, passwordSz, info->iv, PKCS5_SALT_SZ, 1, 00279 info->keySz, hashType)) != 0) { 00280 #ifdef WOLFSSL_SMALL_STACK 00281 XFREE(key, NULL, DYNAMIC_TYPE_SYMMETRIC_KEY); 00282 #endif 00283 return ret; 00284 } 00285 #endif 00286 00287 #ifndef NO_DES3 00288 if (info->cipherType == WC_CIPHER_DES) 00289 ret = wc_Des_CbcDecryptWithKey(der, der, derSz, key, info->iv); 00290 if (info->cipherType == WC_CIPHER_DES3) 00291 ret = wc_Des3_CbcDecryptWithKey(der, der, derSz, key, info->iv); 00292 #endif /* NO_DES3 */ 00293 #if !defined(NO_AES) && defined(HAVE_AES_CBC) && defined(HAVE_AES_DECRYPT) 00294 if (info->cipherType == WC_CIPHER_AES_CBC) 00295 ret = wc_AesCbcDecryptWithKey(der, der, derSz, key, info->keySz, 00296 info->iv); 00297 #endif /* !NO_AES && HAVE_AES_CBC && HAVE_AES_DECRYPT */ 00298 00299 #ifdef WOLFSSL_SMALL_STACK 00300 XFREE(key, NULL, DYNAMIC_TYPE_SYMMETRIC_KEY); 00301 #endif 00302 00303 return ret; 00304 } 00305 00306 int wc_BufferKeyEncrypt(EncryptedInfo* info, byte* der, word32 derSz, 00307 const byte* password, int passwordSz, int hashType) 00308 { 00309 int ret = NOT_COMPILED_IN; 00310 #ifdef WOLFSSL_SMALL_STACK 00311 byte* key = NULL; 00312 #else 00313 byte key[WC_MAX_SYM_KEY_SIZE]; 00314 #endif 00315 00316 (void)derSz; 00317 (void)passwordSz; 00318 (void)hashType; 00319 00320 if (der == NULL || password == NULL || info == NULL || info->keySz == 0 || 00321 info->ivSz < PKCS5_SALT_SZ) { 00322 return BAD_FUNC_ARG; 00323 } 00324 00325 #ifdef WOLFSSL_SMALL_STACK 00326 key = (byte*)XMALLOC(WC_MAX_SYM_KEY_SIZE, NULL, DYNAMIC_TYPE_SYMMETRIC_KEY); 00327 if (key == NULL) { 00328 return MEMORY_E; 00329 } 00330 #endif /* WOLFSSL_SMALL_STACK */ 00331 00332 (void)XMEMSET(key, 0, WC_MAX_SYM_KEY_SIZE); 00333 00334 #ifndef NO_PWDBASED 00335 if ((ret = wc_PBKDF1(key, password, passwordSz, info->iv, PKCS5_SALT_SZ, 1, 00336 info->keySz, hashType)) != 0) { 00337 #ifdef WOLFSSL_SMALL_STACK 00338 XFREE(key, NULL, DYNAMIC_TYPE_SYMMETRIC_KEY); 00339 #endif 00340 return ret; 00341 } 00342 #endif 00343 00344 #ifndef NO_DES3 00345 if (info->cipherType == WC_CIPHER_DES) 00346 ret = wc_Des_CbcEncryptWithKey(der, der, derSz, key, info->iv); 00347 if (info->cipherType == WC_CIPHER_DES3) 00348 ret = wc_Des3_CbcEncryptWithKey(der, der, derSz, key, info->iv); 00349 #endif /* NO_DES3 */ 00350 #if !defined(NO_AES) && defined(HAVE_AES_CBC) 00351 if (info->cipherType == WC_CIPHER_AES_CBC) 00352 ret = wc_AesCbcEncryptWithKey(der, der, derSz, key, info->keySz, 00353 info->iv); 00354 #endif /* !NO_AES && HAVE_AES_CBC */ 00355 00356 #ifdef WOLFSSL_SMALL_STACK 00357 XFREE(key, NULL, DYNAMIC_TYPE_SYMMETRIC_KEY); 00358 #endif 00359 00360 return ret; 00361 } 00362 00363 #endif /* WOLFSSL_ENCRYPTED_KEYS */ 00364 00365 00366 #if !defined(NO_PWDBASED) && !defined(NO_ASN) 00367 00368 #if defined(HAVE_PKCS8) || defined(HAVE_PKCS12) 00369 /* Decrypt/Encrypt input in place from parameters based on id 00370 * 00371 * returns a negative value on fail case 00372 */ 00373 int wc_CryptKey(const char* password, int passwordSz, byte* salt, 00374 int saltSz, int iterations, int id, byte* input, 00375 int length, int version, byte* cbcIv, int enc, int shaOid) 00376 { 00377 int typeH; 00378 int derivedLen = 0; 00379 int ret = 0; 00380 #ifdef WOLFSSL_SMALL_STACK 00381 byte* key; 00382 #else 00383 byte key[MAX_KEY_SIZE]; 00384 #endif 00385 00386 (void)input; 00387 (void)length; 00388 (void)enc; 00389 00390 WOLFSSL_ENTER("wc_CryptKey"); 00391 00392 switch (id) { 00393 #ifndef NO_DES3 00394 #ifndef NO_MD5 00395 case PBE_MD5_DES: 00396 typeH = WC_MD5; 00397 derivedLen = 16; /* may need iv for v1.5 */ 00398 break; 00399 #endif 00400 #ifndef NO_SHA 00401 case PBE_SHA1_DES: 00402 typeH = WC_SHA; 00403 derivedLen = 16; /* may need iv for v1.5 */ 00404 break; 00405 00406 case PBE_SHA1_DES3: 00407 switch(shaOid) { 00408 case HMAC_SHA256_OID: 00409 typeH = WC_SHA256; 00410 derivedLen = 32; 00411 break; 00412 default: 00413 typeH = WC_SHA; 00414 derivedLen = 32; /* may need iv for v1.5 */ 00415 break; 00416 } 00417 break; 00418 #endif /* !NO_SHA */ 00419 #endif /* !NO_DES3 */ 00420 #if !defined(NO_SHA) && !defined(NO_RC4) 00421 case PBE_SHA1_RC4_128: 00422 typeH = WC_SHA; 00423 derivedLen = 16; 00424 break; 00425 #endif 00426 #if defined(WOLFSSL_AES_256) 00427 case PBE_AES256_CBC: 00428 switch(shaOid) { 00429 case HMAC_SHA256_OID: 00430 typeH = WC_SHA256; 00431 derivedLen = 32; 00432 break; 00433 #ifndef NO_SHA 00434 default: 00435 typeH = WC_SHA; 00436 derivedLen = 32; 00437 break; 00438 #endif 00439 } 00440 break; 00441 #endif /* WOLFSSL_AES_256 && !NO_SHA */ 00442 #if defined(WOLFSSL_AES_128) 00443 case PBE_AES128_CBC: 00444 switch(shaOid) { 00445 case HMAC_SHA256_OID: 00446 typeH = WC_SHA256; 00447 derivedLen = 16; 00448 break; 00449 #ifndef NO_SHA 00450 default: 00451 typeH = WC_SHA; 00452 derivedLen = 16; 00453 break; 00454 #endif 00455 } 00456 break; 00457 #endif /* WOLFSSL_AES_128 && !NO_SHA */ 00458 default: 00459 WOLFSSL_MSG("Unknown/Unsupported encrypt/decrypt id"); 00460 (void)shaOid; 00461 return ALGO_ID_E; 00462 } 00463 00464 #ifdef WOLFSSL_SMALL_STACK 00465 key = (byte*)XMALLOC(MAX_KEY_SIZE, NULL, DYNAMIC_TYPE_TMP_BUFFER); 00466 if (key == NULL) 00467 return MEMORY_E; 00468 #endif 00469 00470 if (version == PKCS5v2) 00471 ret = wc_PBKDF2(key, (byte*)password, passwordSz, 00472 salt, saltSz, iterations, derivedLen, typeH); 00473 #ifndef NO_SHA 00474 else if (version == PKCS5) 00475 ret = wc_PBKDF1(key, (byte*)password, passwordSz, 00476 salt, saltSz, iterations, derivedLen, typeH); 00477 #endif 00478 #ifdef HAVE_PKCS12 00479 else if (version == PKCS12v1) { 00480 int i, idx = 0; 00481 byte unicodePasswd[MAX_UNICODE_SZ]; 00482 00483 if ( (passwordSz * 2 + 2) > (int)sizeof(unicodePasswd)) { 00484 #ifdef WOLFSSL_SMALL_STACK 00485 XFREE(key, NULL, DYNAMIC_TYPE_TMP_BUFFER); 00486 #endif 00487 return UNICODE_SIZE_E; 00488 } 00489 00490 for (i = 0; i < passwordSz; i++) { 00491 unicodePasswd[idx++] = 0x00; 00492 unicodePasswd[idx++] = (byte)password[i]; 00493 } 00494 /* add trailing NULL */ 00495 unicodePasswd[idx++] = 0x00; 00496 unicodePasswd[idx++] = 0x00; 00497 00498 ret = wc_PKCS12_PBKDF(key, unicodePasswd, idx, salt, saltSz, 00499 iterations, derivedLen, typeH, 1); 00500 if (id != PBE_SHA1_RC4_128) 00501 ret += wc_PKCS12_PBKDF(cbcIv, unicodePasswd, idx, salt, saltSz, 00502 iterations, 8, typeH, 2); 00503 } 00504 #endif /* HAVE_PKCS12 */ 00505 else { 00506 #ifdef WOLFSSL_SMALL_STACK 00507 XFREE(key, NULL, DYNAMIC_TYPE_TMP_BUFFER); 00508 #endif 00509 WOLFSSL_MSG("Unknown/Unsupported PKCS version"); 00510 return ALGO_ID_E; 00511 } 00512 00513 if (ret != 0) { 00514 #ifdef WOLFSSL_SMALL_STACK 00515 XFREE(key, NULL, DYNAMIC_TYPE_TMP_BUFFER); 00516 #endif 00517 return ret; 00518 } 00519 00520 switch (id) { 00521 #ifndef NO_DES3 00522 #if !defined(NO_SHA) || !defined(NO_MD5) 00523 case PBE_MD5_DES: 00524 case PBE_SHA1_DES: 00525 { 00526 Des des; 00527 byte* desIv = key + 8; 00528 00529 if (version == PKCS5v2 || version == PKCS12v1) 00530 desIv = cbcIv; 00531 00532 if (enc) { 00533 ret = wc_Des_SetKey(&des, key, desIv, DES_ENCRYPTION); 00534 } 00535 else { 00536 ret = wc_Des_SetKey(&des, key, desIv, DES_DECRYPTION); 00537 } 00538 if (ret != 0) { 00539 #ifdef WOLFSSL_SMALL_STACK 00540 XFREE(key, NULL, DYNAMIC_TYPE_TMP_BUFFER); 00541 #endif 00542 return ret; 00543 } 00544 00545 if (enc) { 00546 wc_Des_CbcEncrypt(&des, input, input, length); 00547 } 00548 else { 00549 wc_Des_CbcDecrypt(&des, input, input, length); 00550 } 00551 break; 00552 } 00553 #endif /* !NO_SHA || !NO_MD5 */ 00554 00555 #ifndef NO_SHA 00556 case PBE_SHA1_DES3: 00557 { 00558 Des3 des; 00559 byte* desIv = key + 24; 00560 00561 if (version == PKCS5v2 || version == PKCS12v1) 00562 desIv = cbcIv; 00563 00564 ret = wc_Des3Init(&des, NULL, INVALID_DEVID); 00565 if (ret != 0) { 00566 #ifdef WOLFSSL_SMALL_STACK 00567 XFREE(key, NULL, DYNAMIC_TYPE_TMP_BUFFER); 00568 #endif 00569 return ret; 00570 } 00571 if (enc) { 00572 ret = wc_Des3_SetKey(&des, key, desIv, DES_ENCRYPTION); 00573 } 00574 else { 00575 ret = wc_Des3_SetKey(&des, key, desIv, DES_DECRYPTION); 00576 } 00577 if (ret != 0) { 00578 #ifdef WOLFSSL_SMALL_STACK 00579 XFREE(key, NULL, DYNAMIC_TYPE_TMP_BUFFER); 00580 #endif 00581 return ret; 00582 } 00583 if (enc) { 00584 ret = wc_Des3_CbcEncrypt(&des, input, input, length); 00585 } 00586 else { 00587 ret = wc_Des3_CbcDecrypt(&des, input, input, length); 00588 } 00589 if (ret != 0) { 00590 #ifdef WOLFSSL_SMALL_STACK 00591 XFREE(key, NULL, DYNAMIC_TYPE_TMP_BUFFER); 00592 #endif 00593 return ret; 00594 } 00595 break; 00596 } 00597 #endif /* !NO_SHA */ 00598 #endif 00599 #if !defined(NO_RC4) && !defined(NO_SHA) 00600 case PBE_SHA1_RC4_128: 00601 { 00602 Arc4 dec; 00603 00604 wc_Arc4SetKey(&dec, key, derivedLen); 00605 wc_Arc4Process(&dec, input, input, length); 00606 break; 00607 } 00608 #endif 00609 #if !defined(NO_AES) && defined(HAVE_AES_CBC) 00610 #ifdef WOLFSSL_AES_256 00611 case PBE_AES256_CBC: 00612 case PBE_AES128_CBC: 00613 { 00614 Aes aes; 00615 ret = wc_AesInit(&aes, NULL, INVALID_DEVID); 00616 if (ret == 0) { 00617 if (enc) { 00618 ret = wc_AesSetKey(&aes, key, derivedLen, cbcIv, 00619 AES_ENCRYPTION); 00620 } 00621 else { 00622 ret = wc_AesSetKey(&aes, key, derivedLen, cbcIv, 00623 AES_DECRYPTION); 00624 } 00625 } 00626 if (ret == 0) { 00627 if (enc) 00628 ret = wc_AesCbcEncrypt(&aes, input, input, length); 00629 else 00630 ret = wc_AesCbcDecrypt(&aes, input, input, length); 00631 } 00632 if (ret != 0) { 00633 #ifdef WOLFSSL_SMALL_STACK 00634 XFREE(key, NULL, DYNAMIC_TYPE_TMP_BUFFER); 00635 #endif 00636 return ret; 00637 } 00638 ForceZero(&aes, sizeof(Aes)); 00639 break; 00640 } 00641 #endif /* WOLFSSL_AES_256 */ 00642 #endif /* !NO_AES && HAVE_AES_CBC */ 00643 00644 default: 00645 #ifdef WOLFSSL_SMALL_STACK 00646 XFREE(key, NULL, DYNAMIC_TYPE_TMP_BUFFER); 00647 #endif 00648 WOLFSSL_MSG("Unknown/Unsupported encrypt/decryption algorithm"); 00649 return ALGO_ID_E; 00650 } 00651 00652 #ifdef WOLFSSL_SMALL_STACK 00653 XFREE(key, NULL, DYNAMIC_TYPE_TMP_BUFFER); 00654 #endif 00655 00656 return ret; 00657 } 00658 00659 #endif /* HAVE_PKCS8 || HAVE_PKCS12 */ 00660 #endif /* !NO_PWDBASED */ 00661
Generated on Tue Jul 12 2022 20:59:07 by
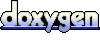