wolfSSL SSL/TLS library, support up to TLS1.3
Dependents: CyaSSL-Twitter-OAuth4Tw Example-client-tls-cert TwitterReader TweetTest ... more
tls13.c
00001 /* tls13.c 00002 * 00003 * Copyright (C) 2006-2020 wolfSSL Inc. 00004 * 00005 * This file is part of wolfSSL. 00006 * 00007 * wolfSSL is free software; you can redistribute it and/or modify 00008 * it under the terms of the GNU General Public License as published by 00009 * the Free Software Foundation; either version 2 of the License, or 00010 * (at your option) any later version. 00011 * 00012 * wolfSSL is distributed in the hope that it will be useful, 00013 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00014 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00015 * GNU General Public License for more details. 00016 * 00017 * You should have received a copy of the GNU General Public License 00018 * along with this program; if not, write to the Free Software 00019 * Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1335, USA 00020 */ 00021 00022 00023 /* 00024 * BUILD_GCM 00025 * Enables AES-GCM ciphersuites. 00026 * HAVE_AESCCM 00027 * Enables AES-CCM ciphersuites. 00028 * HAVE_SESSION_TICKET 00029 * Enables session tickets - required for TLS 1.3 resumption. 00030 * NO_PSK 00031 * Do not enable Pre-Shared Keys. 00032 * TLS13_SUPPORTS_EXPORTERS 00033 * Guard to compile out any code for exporter keys. 00034 * Feature not supported yet. 00035 * WOLFSSL_ASYNC_CRYPT 00036 * Enables the use of asynchronous cryptographic operations. 00037 * This is available for ciphers and certificates. 00038 * HAVE_CHACHA && HAVE_POLY1305 00039 * Enables use of CHACHA20-POLY1305 ciphersuites. 00040 * WOLFSSL_DEBUG_TLS 00041 * Writes out details of TLS 1.3 protocol including handshake message buffers 00042 * and key generation input and output. 00043 * WOLFSSL_EARLY_DATA 00044 * Allow 0-RTT Handshake using Early Data extensions and handshake message 00045 * WOLFSSL_EARLY_DATA_GROUP 00046 * Group EarlyData message with ClientHello when sending 00047 * WOLFSSL_NO_SERVER_GROUPS_EXT 00048 * Do not send the server's groups in an extension when the server's top 00049 * preference is not in client's list. 00050 * WOLFSSL_POST_HANDSHAKE_AUTH 00051 * Allow TLS v1.3 code to perform post-handshake authentication of the 00052 * client. 00053 * WOLFSSL_SEND_HRR_COOKIE 00054 * Send a cookie in hello_retry_request message to enable stateless tracking 00055 * of ClientHello replies. 00056 * WOLFSSL_TLS13 00057 * Enable TLS 1.3 protocol implementation. 00058 * WOLFSSL_TLS13_DRAFT_18 00059 * Conform with Draft 18 of the TLS v1.3 specification. 00060 * WOLFSSL_TLS13_DRAFT_22 00061 * Conform with Draft 22 of the TLS v1.3 specification. 00062 * WOLFSSL_TLS13_DRAFT_23 00063 * Conform with Draft 23 of the TLS v1.3 specification. 00064 * WOLFSSL_TLS13_MIDDLEBOX_COMPAT 00065 * Enable middlebox compatibility in the TLS 1.3 handshake. 00066 * This includes sending ChangeCipherSpec before encrypted messages and 00067 * including a session id. 00068 * WOLFSSL_TLS13_SHA512 00069 * Allow generation of SHA-512 digests in handshake - no ciphersuite 00070 * requires SHA-512 at this time. 00071 * WOLFSSL_TLS13_TICKET_BEFORE_FINISHED 00072 * Allow a NewSessionTicket message to be sent by server before Client's 00073 * Finished message. 00074 * See TLS v1.3 specification, Section 4.6.1, Paragraph 4 (Note). 00075 */ 00076 00077 #ifdef HAVE_CONFIG_H 00078 #include <config.h> 00079 #endif 00080 00081 #include <wolfssl/wolfcrypt/settings.h> 00082 00083 #ifdef WOLFSSL_TLS13 00084 #ifdef HAVE_SESSION_TICKET 00085 #include <wolfssl/wolfcrypt/wc_port.h > 00086 #endif 00087 00088 #ifndef WOLFCRYPT_ONLY 00089 00090 #ifdef HAVE_ERRNO_H 00091 #include <errno.h> 00092 #endif 00093 00094 #include <wolfssl/internal.h> 00095 #include <wolfssl/error-ssl.h> 00096 #include <wolfssl/wolfcrypt/asn.h > 00097 #include <wolfssl/wolfcrypt/dh.h > 00098 #ifdef NO_INLINE 00099 #include <wolfssl/wolfcrypt/misc.h> 00100 #else 00101 #define WOLFSSL_MISC_INCLUDED 00102 #include <wolfcrypt/src/misc.c> 00103 #endif 00104 00105 #ifdef HAVE_NTRU 00106 #include "libntruencrypt/ntru_crypto.h" 00107 #endif 00108 00109 #ifdef __sun 00110 #include <sys/filio.h> 00111 #endif 00112 00113 #ifndef TRUE 00114 #define TRUE 1 00115 #endif 00116 #ifndef FALSE 00117 #define FALSE 0 00118 #endif 00119 00120 #ifndef HAVE_HKDF 00121 #error The build option HAVE_HKDF is required for TLS 1.3 00122 #endif 00123 00124 #ifndef HAVE_TLS_EXTENSIONS 00125 #ifndef _MSC_VER 00126 #error "The build option HAVE_TLS_EXTENSIONS is required for TLS 1.3" 00127 #else 00128 #pragma message("error: The build option HAVE_TLS_EXTENSIONS is required for TLS 1.3") 00129 #endif 00130 #endif 00131 00132 00133 /* Set ret to error value and jump to label. 00134 * 00135 * err The error value to set. 00136 * eLabel The label to jump to. 00137 */ 00138 #define ERROR_OUT(err, eLabel) { ret = (err); goto eLabel; } 00139 00140 00141 /* Extract data using HMAC, salt and input. 00142 * RFC 5869 - HMAC-based Extract-and-Expand Key Derivation Function (HKDF) 00143 * 00144 * prk The generated pseudorandom key. 00145 * salt The salt. 00146 * saltLen The length of the salt. 00147 * ikm The input keying material. 00148 * ikmLen The length of the input keying material. 00149 * mac The type of digest to use. 00150 * returns 0 on success, otherwise failure. 00151 */ 00152 static int Tls13_HKDF_Extract(byte* prk, const byte* salt, int saltLen, 00153 byte* ikm, int ikmLen, int mac) 00154 { 00155 int ret; 00156 int hash = 0; 00157 int len = 0; 00158 00159 switch (mac) { 00160 #ifndef NO_SHA256 00161 case sha256_mac: 00162 hash = WC_SHA256; 00163 len = WC_SHA256_DIGEST_SIZE; 00164 break; 00165 #endif 00166 00167 #ifdef WOLFSSL_SHA384 00168 case sha384_mac: 00169 hash = WC_SHA384; 00170 len = WC_SHA384_DIGEST_SIZE; 00171 break; 00172 #endif 00173 00174 #ifdef WOLFSSL_TLS13_SHA512 00175 case sha512_mac: 00176 hash = WC_SHA512; 00177 len = WC_SHA512_DIGEST_SIZE; 00178 break; 00179 #endif 00180 } 00181 00182 /* When length is 0 then use zeroed data of digest length. */ 00183 if (ikmLen == 0) { 00184 ikmLen = len; 00185 XMEMSET(ikm, 0, len); 00186 } 00187 00188 #ifdef WOLFSSL_DEBUG_TLS 00189 WOLFSSL_MSG(" Salt"); 00190 WOLFSSL_BUFFER(salt, saltLen); 00191 WOLFSSL_MSG(" IKM"); 00192 WOLFSSL_BUFFER(ikm, ikmLen); 00193 #endif 00194 00195 ret = wc_HKDF_Extract(hash, salt, saltLen, ikm, ikmLen, prk); 00196 00197 #ifdef WOLFSSL_DEBUG_TLS 00198 WOLFSSL_MSG(" PRK"); 00199 WOLFSSL_BUFFER(prk, len); 00200 #endif 00201 00202 return ret; 00203 } 00204 00205 /* Expand data using HMAC, salt and label and info. 00206 * TLS v1.3 defines this function. 00207 * 00208 * okm The generated pseudorandom key - output key material. 00209 * okmLen The length of generated pseudorandom key - output key material. 00210 * prk The salt - pseudo-random key. 00211 * prkLen The length of the salt - pseudo-random key. 00212 * protocol The TLS protocol label. 00213 * protocolLen The length of the TLS protocol label. 00214 * info The information to expand. 00215 * infoLen The length of the information. 00216 * digest The type of digest to use. 00217 * returns 0 on success, otherwise failure. 00218 */ 00219 static int HKDF_Expand_Label(byte* okm, word32 okmLen, 00220 const byte* prk, word32 prkLen, 00221 const byte* protocol, word32 protocolLen, 00222 const byte* label, word32 labelLen, 00223 const byte* info, word32 infoLen, 00224 int digest) 00225 { 00226 int ret = 0; 00227 int idx = 0; 00228 byte data[MAX_HKDF_LABEL_SZ]; 00229 00230 /* Output length. */ 00231 data[idx++] = (byte)(okmLen >> 8); 00232 data[idx++] = (byte)okmLen; 00233 /* Length of protocol | label. */ 00234 data[idx++] = (byte)(protocolLen + labelLen); 00235 /* Protocol */ 00236 XMEMCPY(&data[idx], protocol, protocolLen); 00237 idx += protocolLen; 00238 /* Label */ 00239 XMEMCPY(&data[idx], label, labelLen); 00240 idx += labelLen; 00241 /* Length of hash of messages */ 00242 data[idx++] = (byte)infoLen; 00243 /* Hash of messages */ 00244 XMEMCPY(&data[idx], info, infoLen); 00245 idx += infoLen; 00246 00247 #ifdef WOLFSSL_DEBUG_TLS 00248 WOLFSSL_MSG(" PRK"); 00249 WOLFSSL_BUFFER(prk, prkLen); 00250 WOLFSSL_MSG(" Info"); 00251 WOLFSSL_BUFFER(data, idx); 00252 #endif 00253 00254 ret = wc_HKDF_Expand(digest, prk, prkLen, data, idx, okm, okmLen); 00255 00256 #ifdef WOLFSSL_DEBUG_TLS 00257 WOLFSSL_MSG(" OKM"); 00258 WOLFSSL_BUFFER(okm, okmLen); 00259 #endif 00260 00261 ForceZero(data, idx); 00262 00263 return ret; 00264 } 00265 00266 #ifdef WOLFSSL_TLS13_DRAFT_18 00267 /* Size of the TLS v1.3 label use when deriving keys. */ 00268 #define TLS13_PROTOCOL_LABEL_SZ 9 00269 /* The protocol label for TLS v1.3. */ 00270 static const byte tls13ProtocolLabel[TLS13_PROTOCOL_LABEL_SZ + 1] = "TLS 1.3, "; 00271 #else 00272 /* Size of the TLS v1.3 label use when deriving keys. */ 00273 #define TLS13_PROTOCOL_LABEL_SZ 6 00274 /* The protocol label for TLS v1.3. */ 00275 static const byte tls13ProtocolLabel[TLS13_PROTOCOL_LABEL_SZ + 1] = "tls13 "; 00276 #endif 00277 00278 #if !defined(WOLFSSL_TLS13_DRAFT_18) || defined(HAVE_SESSION_TICKET) || \ 00279 !defined(NO_PSK) 00280 /* Derive a key from a message. 00281 * 00282 * ssl The SSL/TLS object. 00283 * output The buffer to hold the derived key. 00284 * outputLen The length of the derived key. 00285 * secret The secret used to derive the key (HMAC secret). 00286 * label The label used to distinguish the context. 00287 * labelLen The length of the label. 00288 * msg The message data to derive key from. 00289 * msgLen The length of the message data to derive key from. 00290 * hashAlgo The hash algorithm to use in the HMAC. 00291 * returns 0 on success, otherwise failure. 00292 */ 00293 static int DeriveKeyMsg(WOLFSSL* ssl, byte* output, int outputLen, 00294 const byte* secret, const byte* label, word32 labelLen, 00295 byte* msg, int msgLen, int hashAlgo) 00296 { 00297 byte hash[WC_MAX_DIGEST_SIZE]; 00298 Digest digest; 00299 word32 hashSz = 0; 00300 const byte* protocol; 00301 word32 protocolLen; 00302 int digestAlg = -1; 00303 int ret = BAD_FUNC_ARG; 00304 00305 switch (hashAlgo) { 00306 #ifndef NO_WOLFSSL_SHA256 00307 case sha256_mac: 00308 ret = wc_InitSha256_ex(&digest.sha256, ssl->heap, INVALID_DEVID); 00309 if (ret == 0) { 00310 ret = wc_Sha256Update(&digest.sha256, msg, msgLen); 00311 if (ret == 0) 00312 ret = wc_Sha256Final(&digest.sha256, hash); 00313 wc_Sha256Free(&digest.sha256); 00314 } 00315 hashSz = WC_SHA256_DIGEST_SIZE; 00316 digestAlg = WC_SHA256; 00317 break; 00318 #endif 00319 #ifdef WOLFSSL_SHA384 00320 case sha384_mac: 00321 ret = wc_InitSha384_ex(&digest.sha384, ssl->heap, INVALID_DEVID); 00322 if (ret == 0) { 00323 ret = wc_Sha384Update(&digest.sha384, msg, msgLen); 00324 if (ret == 0) 00325 ret = wc_Sha384Final(&digest.sha384, hash); 00326 wc_Sha384Free(&digest.sha384); 00327 } 00328 hashSz = WC_SHA384_DIGEST_SIZE; 00329 digestAlg = WC_SHA384; 00330 break; 00331 #endif 00332 #ifdef WOLFSSL_TLS13_SHA512 00333 case sha512_mac: 00334 ret = wc_InitSha512_ex(&digest.sha512, ssl->heap, INVALID_DEVID); 00335 if (ret == 0) { 00336 ret = wc_Sha512Update(&digest.sha512, msg, msgLen); 00337 if (ret == 0) 00338 ret = wc_Sha512Final(&digest.sha512, hash); 00339 wc_Sha512Free(&digest.sha512); 00340 } 00341 hashSz = WC_SHA512_DIGEST_SIZE; 00342 digestAlg = WC_SHA512; 00343 break; 00344 #endif 00345 default: 00346 digestAlg = -1; 00347 break; 00348 } 00349 00350 if (digestAlg < 0) 00351 return HASH_TYPE_E; 00352 00353 if (ret != 0) 00354 return ret; 00355 00356 switch (ssl->version.minor) { 00357 case TLSv1_3_MINOR: 00358 protocol = tls13ProtocolLabel; 00359 protocolLen = TLS13_PROTOCOL_LABEL_SZ; 00360 break; 00361 00362 default: 00363 return VERSION_ERROR; 00364 } 00365 if (outputLen == -1) 00366 outputLen = hashSz; 00367 00368 return HKDF_Expand_Label(output, outputLen, secret, hashSz, 00369 protocol, protocolLen, label, labelLen, 00370 hash, hashSz, digestAlg); 00371 } 00372 #endif 00373 00374 /* Derive a key. 00375 * 00376 * ssl The SSL/TLS object. 00377 * output The buffer to hold the derived key. 00378 * outputLen The length of the derived key. 00379 * secret The secret used to derive the key (HMAC secret). 00380 * label The label used to distinguish the context. 00381 * labelLen The length of the label. 00382 * hashAlgo The hash algorithm to use in the HMAC. 00383 * includeMsgs Whether to include a hash of the handshake messages so far. 00384 * returns 0 on success, otherwise failure. 00385 */ 00386 static int DeriveKey(WOLFSSL* ssl, byte* output, int outputLen, 00387 const byte* secret, const byte* label, word32 labelLen, 00388 int hashAlgo, int includeMsgs) 00389 { 00390 int ret = 0; 00391 byte hash[WC_MAX_DIGEST_SIZE]; 00392 word32 hashSz = 0; 00393 word32 hashOutSz = 0; 00394 const byte* protocol; 00395 word32 protocolLen; 00396 int digestAlg = 0; 00397 00398 switch (hashAlgo) { 00399 #ifndef NO_SHA256 00400 case sha256_mac: 00401 hashSz = WC_SHA256_DIGEST_SIZE; 00402 digestAlg = WC_SHA256; 00403 if (includeMsgs) 00404 ret = wc_Sha256GetHash(&ssl->hsHashes->hashSha256, hash); 00405 break; 00406 #endif 00407 00408 #ifdef WOLFSSL_SHA384 00409 case sha384_mac: 00410 hashSz = WC_SHA384_DIGEST_SIZE; 00411 digestAlg = WC_SHA384; 00412 if (includeMsgs) 00413 ret = wc_Sha384GetHash(&ssl->hsHashes->hashSha384, hash); 00414 break; 00415 #endif 00416 00417 #ifdef WOLFSSL_TLS13_SHA512 00418 case sha512_mac: 00419 hashSz = WC_SHA512_DIGEST_SIZE; 00420 digestAlg = WC_SHA512; 00421 if (includeMsgs) 00422 ret = wc_Sha512GetHash(&ssl->hsHashes->hashSha512, hash); 00423 break; 00424 #endif 00425 } 00426 if (ret != 0) 00427 return ret; 00428 00429 /* Only one protocol version defined at this time. */ 00430 protocol = tls13ProtocolLabel; 00431 protocolLen = TLS13_PROTOCOL_LABEL_SZ; 00432 00433 if (outputLen == -1) 00434 outputLen = hashSz; 00435 if (includeMsgs) 00436 hashOutSz = hashSz; 00437 00438 return HKDF_Expand_Label(output, outputLen, secret, hashSz, 00439 protocol, protocolLen, label, labelLen, 00440 hash, hashOutSz, digestAlg); 00441 } 00442 00443 #ifndef NO_PSK 00444 #ifdef WOLFSSL_TLS13_DRAFT_18 00445 /* The length of the binder key label. */ 00446 #define BINDER_KEY_LABEL_SZ 23 00447 /* The binder key label. */ 00448 static const byte binderKeyLabel[BINDER_KEY_LABEL_SZ + 1] = 00449 "external psk binder key"; 00450 #else 00451 /* The length of the binder key label. */ 00452 #define BINDER_KEY_LABEL_SZ 10 00453 /* The binder key label. */ 00454 static const byte binderKeyLabel[BINDER_KEY_LABEL_SZ + 1] = 00455 "ext binder"; 00456 #endif 00457 /* Derive the binder key. 00458 * 00459 * ssl The SSL/TLS object. 00460 * key The derived key. 00461 * returns 0 on success, otherwise failure. 00462 */ 00463 static int DeriveBinderKey(WOLFSSL* ssl, byte* key) 00464 { 00465 WOLFSSL_MSG("Derive Binder Key"); 00466 return DeriveKeyMsg(ssl, key, -1, ssl->arrays->secret, 00467 binderKeyLabel, BINDER_KEY_LABEL_SZ, 00468 NULL, 0, ssl->specs.mac_algorithm); 00469 } 00470 #endif /* !NO_PSK */ 00471 00472 #ifdef HAVE_SESSION_TICKET 00473 #ifdef WOLFSSL_TLS13_DRAFT_18 00474 /* The length of the binder key resume label. */ 00475 #define BINDER_KEY_RESUME_LABEL_SZ 25 00476 /* The binder key resume label. */ 00477 static const byte binderKeyResumeLabel[BINDER_KEY_RESUME_LABEL_SZ + 1] = 00478 "resumption psk binder key"; 00479 #else 00480 /* The length of the binder key resume label. */ 00481 #define BINDER_KEY_RESUME_LABEL_SZ 10 00482 /* The binder key resume label. */ 00483 static const byte binderKeyResumeLabel[BINDER_KEY_RESUME_LABEL_SZ + 1] = 00484 "res binder"; 00485 #endif 00486 /* Derive the binder resumption key. 00487 * 00488 * ssl The SSL/TLS object. 00489 * key The derived key. 00490 * returns 0 on success, otherwise failure. 00491 */ 00492 static int DeriveBinderKeyResume(WOLFSSL* ssl, byte* key) 00493 { 00494 WOLFSSL_MSG("Derive Binder Key - Resumption"); 00495 return DeriveKeyMsg(ssl, key, -1, ssl->arrays->secret, 00496 binderKeyResumeLabel, BINDER_KEY_RESUME_LABEL_SZ, 00497 NULL, 0, ssl->specs.mac_algorithm); 00498 } 00499 #endif /* HAVE_SESSION_TICKET */ 00500 00501 #ifdef WOLFSSL_EARLY_DATA 00502 #ifdef WOLFSSL_TLS13_DRAFT_18 00503 /* The length of the early traffic label. */ 00504 #define EARLY_TRAFFIC_LABEL_SZ 27 00505 /* The early traffic label. */ 00506 static const byte earlyTrafficLabel[EARLY_TRAFFIC_LABEL_SZ + 1] = 00507 "client early traffic secret"; 00508 #else 00509 /* The length of the early traffic label. */ 00510 #define EARLY_TRAFFIC_LABEL_SZ 11 00511 /* The early traffic label. */ 00512 static const byte earlyTrafficLabel[EARLY_TRAFFIC_LABEL_SZ + 1] = 00513 "c e traffic"; 00514 #endif 00515 /* Derive the early traffic key. 00516 * 00517 * ssl The SSL/TLS object. 00518 * key The derived key. 00519 * returns 0 on success, otherwise failure. 00520 */ 00521 static int DeriveEarlyTrafficSecret(WOLFSSL* ssl, byte* key) 00522 { 00523 int ret; 00524 WOLFSSL_MSG("Derive Early Traffic Secret"); 00525 ret = DeriveKey(ssl, key, -1, ssl->arrays->secret, 00526 earlyTrafficLabel, EARLY_TRAFFIC_LABEL_SZ, 00527 ssl->specs.mac_algorithm, 1); 00528 #ifdef HAVE_SECRET_CALLBACK 00529 if (ret == 0 && ssl->tls13SecretCb != NULL) { 00530 ret = ssl->tls13SecretCb(ssl, CLIENT_EARLY_TRAFFIC_SECRET, key, 00531 ssl->specs.hash_size, ssl->tls13SecretCtx); 00532 if (ret != 0) { 00533 return TLS13_SECRET_CB_E; 00534 } 00535 } 00536 #endif /* HAVE_SECRET_CALLBACK */ 00537 return ret; 00538 } 00539 00540 #ifdef TLS13_SUPPORTS_EXPORTERS 00541 #ifdef WOLFSSL_TLS13_DRAFT_18 00542 /* The length of the early exporter label. */ 00543 #define EARLY_EXPORTER_LABEL_SZ 28 00544 /* The early exporter label. */ 00545 static const byte earlyExporterLabel[EARLY_EXPORTER_LABEL_SZ + 1] = 00546 "early exporter master secret"; 00547 #else 00548 /* The length of the early exporter label. */ 00549 #define EARLY_EXPORTER_LABEL_SZ 12 00550 /* The early exporter label. */ 00551 static const byte earlyExporterLabel[EARLY_EXPORTER_LABEL_SZ + 1] = 00552 "e exp master"; 00553 #endif 00554 /* Derive the early exporter key. 00555 * 00556 * ssl The SSL/TLS object. 00557 * key The derived key. 00558 * returns 0 on success, otherwise failure. 00559 */ 00560 static int DeriveEarlyExporterSecret(WOLFSSL* ssl, byte* key) 00561 { 00562 int ret; 00563 WOLFSSL_MSG("Derive Early Exporter Secret"); 00564 ret = DeriveKey(ssl, key, -1, ssl->arrays->secret, 00565 earlyExporterLabel, EARLY_EXPORTER_LABEL_SZ, 00566 ssl->specs.mac_algorithm, 1); 00567 #ifdef HAVE_SECRET_CALLBACK 00568 if (ret == 0 && ssl->tls13SecretCb != NULL) { 00569 ret = ssl->tls13SecretCb(ssl, EARLY_EXPORTER_SECRET, key 00570 ssl->specs.hash_size, ssl->tls13SecretCtx); 00571 if (ret != 0) { 00572 return TLS13_SECRET_CB_E; 00573 } 00574 } 00575 #endif /* HAVE_SECRET_CALLBACK */ 00576 return ret; 00577 } 00578 #endif 00579 #endif 00580 00581 #ifdef WOLFSSL_TLS13_DRAFT_18 00582 /* The length of the client handshake label. */ 00583 #define CLIENT_HANDSHAKE_LABEL_SZ 31 00584 /* The client handshake label. */ 00585 static const byte clientHandshakeLabel[CLIENT_HANDSHAKE_LABEL_SZ + 1] = 00586 "client handshake traffic secret"; 00587 #else 00588 /* The length of the client handshake label. */ 00589 #define CLIENT_HANDSHAKE_LABEL_SZ 12 00590 /* The client handshake label. */ 00591 static const byte clientHandshakeLabel[CLIENT_HANDSHAKE_LABEL_SZ + 1] = 00592 "c hs traffic"; 00593 #endif 00594 /* Derive the client handshake key. 00595 * 00596 * ssl The SSL/TLS object. 00597 * key The derived key. 00598 * returns 0 on success, otherwise failure. 00599 */ 00600 static int DeriveClientHandshakeSecret(WOLFSSL* ssl, byte* key) 00601 { 00602 int ret; 00603 WOLFSSL_MSG("Derive Client Handshake Secret"); 00604 ret = DeriveKey(ssl, key, -1, ssl->arrays->preMasterSecret, 00605 clientHandshakeLabel, CLIENT_HANDSHAKE_LABEL_SZ, 00606 ssl->specs.mac_algorithm, 1); 00607 #ifdef HAVE_SECRET_CALLBACK 00608 if (ret == 0 && ssl->tls13SecretCb != NULL) { 00609 ret = ssl->tls13SecretCb(ssl, CLIENT_HANDSHAKE_TRAFFIC_SECRET, key, 00610 ssl->specs.hash_size, ssl->tls13SecretCtx); 00611 if (ret != 0) { 00612 return TLS13_SECRET_CB_E; 00613 } 00614 } 00615 #endif /* HAVE_SECRET_CALLBACK */ 00616 return ret; 00617 } 00618 00619 #ifdef WOLFSSL_TLS13_DRAFT_18 00620 /* The length of the server handshake label. */ 00621 #define SERVER_HANDSHAKE_LABEL_SZ 31 00622 /* The server handshake label. */ 00623 static const byte serverHandshakeLabel[SERVER_HANDSHAKE_LABEL_SZ + 1] = 00624 "server handshake traffic secret"; 00625 #else 00626 /* The length of the server handshake label. */ 00627 #define SERVER_HANDSHAKE_LABEL_SZ 12 00628 /* The server handshake label. */ 00629 static const byte serverHandshakeLabel[SERVER_HANDSHAKE_LABEL_SZ + 1] = 00630 "s hs traffic"; 00631 #endif 00632 /* Derive the server handshake key. 00633 * 00634 * ssl The SSL/TLS object. 00635 * key The derived key. 00636 * returns 0 on success, otherwise failure. 00637 */ 00638 static int DeriveServerHandshakeSecret(WOLFSSL* ssl, byte* key) 00639 { 00640 int ret; 00641 WOLFSSL_MSG("Derive Server Handshake Secret"); 00642 ret = DeriveKey(ssl, key, -1, ssl->arrays->preMasterSecret, 00643 serverHandshakeLabel, SERVER_HANDSHAKE_LABEL_SZ, 00644 ssl->specs.mac_algorithm, 1); 00645 #ifdef HAVE_SECRET_CALLBACK 00646 if (ret == 0 && ssl->tls13SecretCb != NULL) { 00647 ret = ssl->tls13SecretCb(ssl, SERVER_HANDSHAKE_TRAFFIC_SECRET, key, 00648 ssl->specs.hash_size, ssl->tls13SecretCtx); 00649 if (ret != 0) { 00650 return TLS13_SECRET_CB_E; 00651 } 00652 } 00653 #endif /* HAVE_SECRET_CALLBACK */ 00654 return ret; 00655 } 00656 00657 #ifdef WOLFSSL_TLS13_DRAFT_18 00658 /* The length of the client application traffic label. */ 00659 #define CLIENT_APP_LABEL_SZ 33 00660 /* The client application traffic label. */ 00661 static const byte clientAppLabel[CLIENT_APP_LABEL_SZ + 1] = 00662 "client application traffic secret"; 00663 #else 00664 /* The length of the client application traffic label. */ 00665 #define CLIENT_APP_LABEL_SZ 12 00666 /* The client application traffic label. */ 00667 static const byte clientAppLabel[CLIENT_APP_LABEL_SZ + 1] = 00668 "c ap traffic"; 00669 #endif 00670 /* Derive the client application traffic key. 00671 * 00672 * ssl The SSL/TLS object. 00673 * key The derived key. 00674 * returns 0 on success, otherwise failure. 00675 */ 00676 static int DeriveClientTrafficSecret(WOLFSSL* ssl, byte* key) 00677 { 00678 int ret; 00679 WOLFSSL_MSG("Derive Client Traffic Secret"); 00680 ret = DeriveKey(ssl, key, -1, ssl->arrays->masterSecret, 00681 clientAppLabel, CLIENT_APP_LABEL_SZ, 00682 ssl->specs.mac_algorithm, 1); 00683 #ifdef HAVE_SECRET_CALLBACK 00684 if (ret == 0 && ssl->tls13SecretCb != NULL) { 00685 ret = ssl->tls13SecretCb(ssl, CLIENT_TRAFFIC_SECRET, key, 00686 ssl->specs.hash_size, ssl->tls13SecretCtx); 00687 if (ret != 0) { 00688 return TLS13_SECRET_CB_E; 00689 } 00690 } 00691 #endif /* HAVE_SECRET_CALLBACK */ 00692 return ret; 00693 } 00694 00695 #ifdef WOLFSSL_TLS13_DRAFT_18 00696 /* The length of the server application traffic label. */ 00697 #define SERVER_APP_LABEL_SZ 33 00698 /* The server application traffic label. */ 00699 static const byte serverAppLabel[SERVER_APP_LABEL_SZ + 1] = 00700 "server application traffic secret"; 00701 #else 00702 /* The length of the server application traffic label. */ 00703 #define SERVER_APP_LABEL_SZ 12 00704 /* The server application traffic label. */ 00705 static const byte serverAppLabel[SERVER_APP_LABEL_SZ + 1] = 00706 "s ap traffic"; 00707 #endif 00708 /* Derive the server application traffic key. 00709 * 00710 * ssl The SSL/TLS object. 00711 * key The derived key. 00712 * returns 0 on success, otherwise failure. 00713 */ 00714 static int DeriveServerTrafficSecret(WOLFSSL* ssl, byte* key) 00715 { 00716 int ret; 00717 WOLFSSL_MSG("Derive Server Traffic Secret"); 00718 ret = DeriveKey(ssl, key, -1, ssl->arrays->masterSecret, 00719 serverAppLabel, SERVER_APP_LABEL_SZ, 00720 ssl->specs.mac_algorithm, 1); 00721 #ifdef HAVE_SECRET_CALLBACK 00722 if (ret == 0 && ssl->tls13SecretCb != NULL) { 00723 ret = ssl->tls13SecretCb(ssl, SERVER_TRAFFIC_SECRET, key, 00724 ssl->specs.hash_size, ssl->tls13SecretCtx); 00725 if (ret != 0) { 00726 return TLS13_SECRET_CB_E; 00727 } 00728 } 00729 #endif /* HAVE_SECRET_CALLBACK */ 00730 return ret; 00731 } 00732 00733 #ifdef TLS13_SUPPORTS_EXPORTERS 00734 #ifdef WOLFSSL_TLS13_DRAFT_18 00735 /* The length of the exporter master secret label. */ 00736 #define EXPORTER_MASTER_LABEL_SZ 22 00737 /* The exporter master secret label. */ 00738 static const byte exporterMasterLabel[EXPORTER_MASTER_LABEL_SZ + 1] = 00739 "exporter master secret"; 00740 #else 00741 /* The length of the exporter master secret label. */ 00742 #define EXPORTER_MASTER_LABEL_SZ 10 00743 /* The exporter master secret label. */ 00744 static const byte exporterMasterLabel[EXPORTER_MASTER_LABEL_SZ + 1] = 00745 "exp master"; 00746 #endif 00747 /* Derive the exporter secret. 00748 * 00749 * ssl The SSL/TLS object. 00750 * key The derived key. 00751 * returns 0 on success, otherwise failure. 00752 */ 00753 static int DeriveExporterSecret(WOLFSSL* ssl, byte* key) 00754 { 00755 int ret; 00756 WOLFSSL_MSG("Derive Exporter Secret"); 00757 ret = DeriveKey(ssl, key, -1, ssl->arrays->masterSecret, 00758 exporterMasterLabel, EXPORTER_MASTER_LABEL_SZ, 00759 ssl->specs.mac_algorithm, 1); 00760 #ifdef HAVE_SECRET_CALLBACK 00761 if (ret == 0 && ssl->tls13SecretCb != NULL) { 00762 ret = ssl->tls13SecretCb(ssl, EXPORTER_SECRET, key, 00763 ssl->specs.hash_size, ssl->tls13SecretCtx); 00764 if (ret != 0) { 00765 return TLS13_SECRET_CB_E; 00766 } 00767 } 00768 #endif /* HAVE_SECRET_CALLBACK */ 00769 return ret; 00770 } 00771 #endif 00772 00773 #if defined(HAVE_SESSION_TICKET) || !defined(NO_PSK) 00774 #ifdef WOLFSSL_TLS13_DRAFT_18 00775 /* The length of the resumption master secret label. */ 00776 #define RESUME_MASTER_LABEL_SZ 24 00777 /* The resumption master secret label. */ 00778 static const byte resumeMasterLabel[RESUME_MASTER_LABEL_SZ + 1] = 00779 "resumption master secret"; 00780 #else 00781 /* The length of the resumption master secret label. */ 00782 #define RESUME_MASTER_LABEL_SZ 10 00783 /* The resumption master secret label. */ 00784 static const byte resumeMasterLabel[RESUME_MASTER_LABEL_SZ + 1] = 00785 "res master"; 00786 #endif 00787 /* Derive the resumption secret. 00788 * 00789 * ssl The SSL/TLS object. 00790 * key The derived key. 00791 * returns 0 on success, otherwise failure. 00792 */ 00793 static int DeriveResumptionSecret(WOLFSSL* ssl, byte* key) 00794 { 00795 WOLFSSL_MSG("Derive Resumption Secret"); 00796 return DeriveKey(ssl, key, -1, ssl->arrays->masterSecret, 00797 resumeMasterLabel, RESUME_MASTER_LABEL_SZ, 00798 ssl->specs.mac_algorithm, 1); 00799 } 00800 #endif 00801 00802 /* Length of the finished label. */ 00803 #define FINISHED_LABEL_SZ 8 00804 /* Finished label for generating finished key. */ 00805 static const byte finishedLabel[FINISHED_LABEL_SZ+1] = "finished"; 00806 /* Derive the finished secret. 00807 * 00808 * ssl The SSL/TLS object. 00809 * key The key to use with the HMAC. 00810 * secret The derived secret. 00811 * returns 0 on success, otherwise failure. 00812 */ 00813 static int DeriveFinishedSecret(WOLFSSL* ssl, byte* key, byte* secret) 00814 { 00815 WOLFSSL_MSG("Derive Finished Secret"); 00816 return DeriveKey(ssl, secret, -1, key, finishedLabel, FINISHED_LABEL_SZ, 00817 ssl->specs.mac_algorithm, 0); 00818 } 00819 00820 #ifdef WOLFSSL_TLS13_DRAFT_18 00821 /* The length of the application traffic label. */ 00822 #define APP_TRAFFIC_LABEL_SZ 26 00823 /* The application traffic label. */ 00824 static const byte appTrafficLabel[APP_TRAFFIC_LABEL_SZ + 1] = 00825 "application traffic secret"; 00826 #else 00827 /* The length of the application traffic label. */ 00828 #define APP_TRAFFIC_LABEL_SZ 11 00829 /* The application traffic label. */ 00830 static const byte appTrafficLabel[APP_TRAFFIC_LABEL_SZ + 1] = 00831 "traffic upd"; 00832 #endif 00833 /* Update the traffic secret. 00834 * 00835 * ssl The SSL/TLS object. 00836 * secret The previous secret and derived secret. 00837 * returns 0 on success, otherwise failure. 00838 */ 00839 static int DeriveTrafficSecret(WOLFSSL* ssl, byte* secret) 00840 { 00841 WOLFSSL_MSG("Derive New Application Traffic Secret"); 00842 return DeriveKey(ssl, secret, -1, secret, 00843 appTrafficLabel, APP_TRAFFIC_LABEL_SZ, 00844 ssl->specs.mac_algorithm, 0); 00845 } 00846 00847 /* Derive the early secret using HKDF Extract. 00848 * 00849 * ssl The SSL/TLS object. 00850 */ 00851 static int DeriveEarlySecret(WOLFSSL* ssl) 00852 { 00853 WOLFSSL_MSG("Derive Early Secret"); 00854 #if defined(HAVE_SESSION_TICKET) || !defined(NO_PSK) 00855 return Tls13_HKDF_Extract(ssl->arrays->secret, NULL, 0, 00856 ssl->arrays->psk_key, ssl->arrays->psk_keySz, 00857 ssl->specs.mac_algorithm); 00858 #else 00859 return Tls13_HKDF_Extract(ssl->arrays->secret, NULL, 0, 00860 ssl->arrays->masterSecret, 0, ssl->specs.mac_algorithm); 00861 #endif 00862 } 00863 00864 #ifndef WOLFSSL_TLS13_DRAFT_18 00865 /* The length of the derived label. */ 00866 #define DERIVED_LABEL_SZ 7 00867 /* The derived label. */ 00868 static const byte derivedLabel[DERIVED_LABEL_SZ + 1] = 00869 "derived"; 00870 #endif 00871 /* Derive the handshake secret using HKDF Extract. 00872 * 00873 * ssl The SSL/TLS object. 00874 */ 00875 static int DeriveHandshakeSecret(WOLFSSL* ssl) 00876 { 00877 #ifdef WOLFSSL_TLS13_DRAFT_18 00878 WOLFSSL_MSG("Derive Handshake Secret"); 00879 return Tls13_HKDF_Extract(ssl->arrays->preMasterSecret, 00880 ssl->arrays->secret, ssl->specs.hash_size, 00881 ssl->arrays->preMasterSecret, ssl->arrays->preMasterSz, 00882 ssl->specs.mac_algorithm); 00883 #else 00884 byte key[WC_MAX_DIGEST_SIZE]; 00885 int ret; 00886 00887 WOLFSSL_MSG("Derive Handshake Secret"); 00888 00889 ret = DeriveKeyMsg(ssl, key, -1, ssl->arrays->secret, 00890 derivedLabel, DERIVED_LABEL_SZ, 00891 NULL, 0, ssl->specs.mac_algorithm); 00892 if (ret != 0) 00893 return ret; 00894 00895 return Tls13_HKDF_Extract(ssl->arrays->preMasterSecret, 00896 key, ssl->specs.hash_size, 00897 ssl->arrays->preMasterSecret, ssl->arrays->preMasterSz, 00898 ssl->specs.mac_algorithm); 00899 #endif 00900 } 00901 00902 /* Derive the master secret using HKDF Extract. 00903 * 00904 * ssl The SSL/TLS object. 00905 */ 00906 static int DeriveMasterSecret(WOLFSSL* ssl) 00907 { 00908 #ifdef WOLFSSL_TLS13_DRAFT_18 00909 WOLFSSL_MSG("Derive Master Secret"); 00910 return Tls13_HKDF_Extract(ssl->arrays->masterSecret, 00911 ssl->arrays->preMasterSecret, ssl->specs.hash_size, 00912 ssl->arrays->masterSecret, 0, ssl->specs.mac_algorithm); 00913 #else 00914 byte key[WC_MAX_DIGEST_SIZE]; 00915 int ret; 00916 00917 WOLFSSL_MSG("Derive Master Secret"); 00918 00919 ret = DeriveKeyMsg(ssl, key, -1, ssl->arrays->preMasterSecret, 00920 derivedLabel, DERIVED_LABEL_SZ, 00921 NULL, 0, ssl->specs.mac_algorithm); 00922 if (ret != 0) 00923 return ret; 00924 00925 return Tls13_HKDF_Extract(ssl->arrays->masterSecret, 00926 key, ssl->specs.hash_size, 00927 ssl->arrays->masterSecret, 0, ssl->specs.mac_algorithm); 00928 #endif 00929 } 00930 00931 #ifndef WOLFSSL_TLS13_DRAFT_18 00932 #if defined(HAVE_SESSION_TICKET) 00933 /* Length of the resumption label. */ 00934 #define RESUMPTION_LABEL_SZ 10 00935 /* Resumption label for generating PSK associated with the ticket. */ 00936 static const byte resumptionLabel[RESUMPTION_LABEL_SZ+1] = "resumption"; 00937 /* Derive the PSK associated with the ticket. 00938 * 00939 * ssl The SSL/TLS object. 00940 * nonce The nonce to derive with. 00941 * nonceLen The length of the nonce to derive with. 00942 * secret The derived secret. 00943 * returns 0 on success, otherwise failure. 00944 */ 00945 static int DeriveResumptionPSK(WOLFSSL* ssl, byte* nonce, byte nonceLen, 00946 byte* secret) 00947 { 00948 int digestAlg; 00949 /* Only one protocol version defined at this time. */ 00950 const byte* protocol = tls13ProtocolLabel; 00951 word32 protocolLen = TLS13_PROTOCOL_LABEL_SZ; 00952 00953 WOLFSSL_MSG("Derive Resumption PSK"); 00954 00955 switch (ssl->specs.mac_algorithm) { 00956 #ifndef NO_SHA256 00957 case sha256_mac: 00958 digestAlg = WC_SHA256; 00959 break; 00960 #endif 00961 00962 #ifdef WOLFSSL_SHA384 00963 case sha384_mac: 00964 digestAlg = WC_SHA384; 00965 break; 00966 #endif 00967 00968 #ifdef WOLFSSL_TLS13_SHA512 00969 case sha512_mac: 00970 digestAlg = WC_SHA512; 00971 break; 00972 #endif 00973 00974 default: 00975 return BAD_FUNC_ARG; 00976 } 00977 00978 return HKDF_Expand_Label(secret, ssl->specs.hash_size, 00979 ssl->session.masterSecret, ssl->specs.hash_size, 00980 protocol, protocolLen, resumptionLabel, 00981 RESUMPTION_LABEL_SZ, nonce, nonceLen, digestAlg); 00982 } 00983 #endif /* HAVE_SESSION_TICKET */ 00984 #endif /* WOLFSSL_TLS13_DRAFT_18 */ 00985 00986 00987 /* Calculate the HMAC of message data to this point. 00988 * 00989 * ssl The SSL/TLS object. 00990 * key The HMAC key. 00991 * hash The hash result - verify data. 00992 * returns length of verify data generated. 00993 */ 00994 static int BuildTls13HandshakeHmac(WOLFSSL* ssl, byte* key, byte* hash, 00995 word32* pHashSz) 00996 { 00997 Hmac verifyHmac; 00998 int hashType = WC_SHA256; 00999 int hashSz = WC_SHA256_DIGEST_SIZE; 01000 int ret = BAD_FUNC_ARG; 01001 01002 /* Get the hash of the previous handshake messages. */ 01003 switch (ssl->specs.mac_algorithm) { 01004 #ifndef NO_SHA256 01005 case sha256_mac: 01006 hashType = WC_SHA256; 01007 hashSz = WC_SHA256_DIGEST_SIZE; 01008 ret = wc_Sha256GetHash(&ssl->hsHashes->hashSha256, hash); 01009 break; 01010 #endif /* !NO_SHA256 */ 01011 #ifdef WOLFSSL_SHA384 01012 case sha384_mac: 01013 hashType = WC_SHA384; 01014 hashSz = WC_SHA384_DIGEST_SIZE; 01015 ret = wc_Sha384GetHash(&ssl->hsHashes->hashSha384, hash); 01016 break; 01017 #endif /* WOLFSSL_SHA384 */ 01018 #ifdef WOLFSSL_TLS13_SHA512 01019 case sha512_mac: 01020 hashType = WC_SHA512; 01021 hashSz = WC_SHA512_DIGEST_SIZE; 01022 ret = wc_Sha512GetHash(&ssl->hsHashes->hashSha512, hash); 01023 break; 01024 #endif /* WOLFSSL_TLS13_SHA512 */ 01025 } 01026 if (ret != 0) 01027 return ret; 01028 01029 /* Calculate the verify data. */ 01030 ret = wc_HmacInit(&verifyHmac, ssl->heap, ssl->devId); 01031 if (ret == 0) { 01032 ret = wc_HmacSetKey(&verifyHmac, hashType, key, ssl->specs.hash_size); 01033 if (ret == 0) 01034 ret = wc_HmacUpdate(&verifyHmac, hash, hashSz); 01035 if (ret == 0) 01036 ret = wc_HmacFinal(&verifyHmac, hash); 01037 wc_HmacFree(&verifyHmac); 01038 } 01039 01040 if (pHashSz) 01041 *pHashSz = hashSz; 01042 01043 return ret; 01044 } 01045 01046 /* The length of the label to use when deriving keys. */ 01047 #define WRITE_KEY_LABEL_SZ 3 01048 /* The length of the label to use when deriving IVs. */ 01049 #define WRITE_IV_LABEL_SZ 2 01050 /* The label to use when deriving keys. */ 01051 static const byte writeKeyLabel[WRITE_KEY_LABEL_SZ+1] = "key"; 01052 /* The label to use when deriving IVs. */ 01053 static const byte writeIVLabel[WRITE_IV_LABEL_SZ+1] = "iv"; 01054 01055 /* Derive the keys and IVs for TLS v1.3. 01056 * 01057 * ssl The SSL/TLS object. 01058 * sercret early_data_key when deriving the key and IV for encrypting early 01059 * data application data and end_of_early_data messages. 01060 * handshake_key when deriving keys and IVs for encrypting handshake 01061 * messages. 01062 * traffic_key when deriving first keys and IVs for encrypting 01063 * traffic messages. 01064 * update_traffic_key when deriving next keys and IVs for encrypting 01065 * traffic messages. 01066 * side ENCRYPT_SIDE_ONLY when only encryption secret needs to be derived. 01067 * DECRYPT_SIDE_ONLY when only decryption secret needs to be derived. 01068 * ENCRYPT_AND_DECRYPT_SIDE when both secret needs to be derived. 01069 * store 1 indicates to derive the keys and IVs from derived secret and 01070 * store ready for provisioning. 01071 * returns 0 on success, otherwise failure. 01072 */ 01073 static int DeriveTls13Keys(WOLFSSL* ssl, int secret, int side, int store) 01074 { 01075 int ret = BAD_FUNC_ARG; /* Assume failure */ 01076 int i = 0; 01077 #ifdef WOLFSSL_SMALL_STACK 01078 byte* key_dig; 01079 #else 01080 byte key_dig[MAX_PRF_DIG]; 01081 #endif 01082 int provision; 01083 01084 #ifdef WOLFSSL_SMALL_STACK 01085 key_dig = (byte*)XMALLOC(MAX_PRF_DIG, ssl->heap, DYNAMIC_TYPE_DIGEST); 01086 if (key_dig == NULL) 01087 return MEMORY_E; 01088 #endif 01089 01090 if (side == ENCRYPT_AND_DECRYPT_SIDE) { 01091 provision = PROVISION_CLIENT_SERVER; 01092 } 01093 else { 01094 provision = ((ssl->options.side != WOLFSSL_CLIENT_END) ^ 01095 (side == ENCRYPT_SIDE_ONLY)) ? PROVISION_CLIENT : 01096 PROVISION_SERVER; 01097 } 01098 01099 /* Derive the appropriate secret to use in the HKDF. */ 01100 switch (secret) { 01101 #ifdef WOLFSSL_EARLY_DATA 01102 case early_data_key: 01103 ret = DeriveEarlyTrafficSecret(ssl, ssl->clientSecret); 01104 if (ret != 0) 01105 goto end; 01106 break; 01107 #endif 01108 01109 case handshake_key: 01110 if (provision & PROVISION_CLIENT) { 01111 ret = DeriveClientHandshakeSecret(ssl, 01112 ssl->clientSecret); 01113 if (ret != 0) 01114 goto end; 01115 } 01116 if (provision & PROVISION_SERVER) { 01117 ret = DeriveServerHandshakeSecret(ssl, 01118 ssl->serverSecret); 01119 if (ret != 0) 01120 goto end; 01121 } 01122 break; 01123 01124 case traffic_key: 01125 if (provision & PROVISION_CLIENT) { 01126 ret = DeriveClientTrafficSecret(ssl, ssl->clientSecret); 01127 if (ret != 0) 01128 goto end; 01129 } 01130 if (provision & PROVISION_SERVER) { 01131 ret = DeriveServerTrafficSecret(ssl, ssl->serverSecret); 01132 if (ret != 0) 01133 goto end; 01134 } 01135 break; 01136 01137 case update_traffic_key: 01138 if (provision & PROVISION_CLIENT) { 01139 ret = DeriveTrafficSecret(ssl, ssl->clientSecret); 01140 if (ret != 0) 01141 goto end; 01142 } 01143 if (provision & PROVISION_SERVER) { 01144 ret = DeriveTrafficSecret(ssl, ssl->serverSecret); 01145 if (ret != 0) 01146 goto end; 01147 } 01148 break; 01149 } 01150 01151 if (!store) 01152 goto end; 01153 01154 /* Key data = client key | server key | client IV | server IV */ 01155 01156 if (provision & PROVISION_CLIENT) { 01157 /* Derive the client key. */ 01158 WOLFSSL_MSG("Derive Client Key"); 01159 ret = DeriveKey(ssl, &key_dig[i], ssl->specs.key_size, 01160 ssl->clientSecret, writeKeyLabel, 01161 WRITE_KEY_LABEL_SZ, ssl->specs.mac_algorithm, 0); 01162 if (ret != 0) 01163 goto end; 01164 i += ssl->specs.key_size; 01165 } 01166 01167 if (provision & PROVISION_SERVER) { 01168 /* Derive the server key. */ 01169 WOLFSSL_MSG("Derive Server Key"); 01170 ret = DeriveKey(ssl, &key_dig[i], ssl->specs.key_size, 01171 ssl->serverSecret, writeKeyLabel, 01172 WRITE_KEY_LABEL_SZ, ssl->specs.mac_algorithm, 0); 01173 if (ret != 0) 01174 goto end; 01175 i += ssl->specs.key_size; 01176 } 01177 01178 if (provision & PROVISION_CLIENT) { 01179 /* Derive the client IV. */ 01180 WOLFSSL_MSG("Derive Client IV"); 01181 ret = DeriveKey(ssl, &key_dig[i], ssl->specs.iv_size, 01182 ssl->clientSecret, writeIVLabel, 01183 WRITE_IV_LABEL_SZ, ssl->specs.mac_algorithm, 0); 01184 if (ret != 0) 01185 goto end; 01186 i += ssl->specs.iv_size; 01187 } 01188 01189 if (provision & PROVISION_SERVER) { 01190 /* Derive the server IV. */ 01191 WOLFSSL_MSG("Derive Server IV"); 01192 ret = DeriveKey(ssl, &key_dig[i], ssl->specs.iv_size, 01193 ssl->serverSecret, writeIVLabel, 01194 WRITE_IV_LABEL_SZ, ssl->specs.mac_algorithm, 0); 01195 if (ret != 0) 01196 goto end; 01197 } 01198 01199 /* Store keys and IVs but don't activate them. */ 01200 ret = StoreKeys(ssl, key_dig, provision); 01201 01202 end: 01203 #ifdef WOLFSSL_SMALL_STACK 01204 XFREE(key_dig, ssl->heap, DYNAMIC_TYPE_DIGEST); 01205 #endif 01206 01207 return ret; 01208 } 01209 01210 #ifdef HAVE_SESSION_TICKET 01211 #if defined(USER_TICKS) 01212 #if 0 01213 word32 TimeNowInMilliseconds(void) 01214 { 01215 /* 01216 write your own clock tick function if don't want gettimeofday() 01217 needs millisecond accuracy but doesn't have to correlated to EPOCH 01218 */ 01219 } 01220 #endif 01221 01222 #elif defined(TIME_OVERRIDES) 01223 #ifndef HAVE_TIME_T_TYPE 01224 typedef long time_t; 01225 #endif 01226 extern time_t XTIME(time_t * timer); 01227 01228 /* The time in milliseconds. 01229 * Used for tickets to represent difference between when first seen and when 01230 * sending. 01231 * 01232 * returns the time in milliseconds as a 32-bit value. 01233 */ 01234 word32 TimeNowInMilliseconds(void) 01235 { 01236 return (word32) XTIME(0) * 1000; 01237 } 01238 01239 #elif defined(XTIME_MS) 01240 word32 TimeNowInMilliseconds(void) 01241 { 01242 return (word32)XTIME_MS(0); 01243 } 01244 01245 #elif defined(USE_WINDOWS_API) 01246 /* The time in milliseconds. 01247 * Used for tickets to represent difference between when first seen and when 01248 * sending. 01249 * 01250 * returns the time in milliseconds as a 32-bit value. 01251 */ 01252 word32 TimeNowInMilliseconds(void) 01253 { 01254 static int init = 0; 01255 static LARGE_INTEGER freq; 01256 LARGE_INTEGER count; 01257 01258 if (!init) { 01259 QueryPerformanceFrequency(&freq); 01260 init = 1; 01261 } 01262 01263 QueryPerformanceCounter(&count); 01264 01265 return (word32)(count.QuadPart / (freq.QuadPart / 1000)); 01266 } 01267 01268 #elif defined(HAVE_RTP_SYS) 01269 #include "rtptime.h" 01270 01271 /* The time in milliseconds. 01272 * Used for tickets to represent difference between when first seen and when 01273 * sending. 01274 * 01275 * returns the time in milliseconds as a 32-bit value. 01276 */ 01277 word32 TimeNowInMilliseconds(void) 01278 { 01279 return (word32)rtp_get_system_sec() * 1000; 01280 } 01281 #elif defined(WOLFSSL_DEOS) 01282 word32 TimeNowInMilliseconds(void) 01283 { 01284 const uint32_t systemTickTimeInHz = 1000000 / systemTickInMicroseconds(); 01285 uint32_t *systemTickPtr = systemTickPointer(); 01286 01287 return (word32) (*systemTickPtr/systemTickTimeInHz) * 1000; 01288 } 01289 #elif defined(MICRIUM) 01290 /* The time in milliseconds. 01291 * Used for tickets to represent difference between when first seen and when 01292 * sending. 01293 * 01294 * returns the time in milliseconds as a 32-bit value. 01295 */ 01296 word32 TimeNowInMilliseconds(void) 01297 { 01298 OS_TICK ticks = 0; 01299 OS_ERR err; 01300 01301 ticks = OSTimeGet(&err); 01302 01303 return (word32) (ticks / OSCfg_TickRate_Hz) * 1000; 01304 } 01305 #elif defined(MICROCHIP_TCPIP_V5) 01306 /* The time in milliseconds. 01307 * Used for tickets to represent difference between when first seen and when 01308 * sending. 01309 * 01310 * returns the time in milliseconds as a 32-bit value. 01311 */ 01312 word32 TimeNowInMilliseconds(void) 01313 { 01314 return (word32) (TickGet() / (TICKS_PER_SECOND / 1000)); 01315 } 01316 #elif defined(MICROCHIP_TCPIP) 01317 #if defined(MICROCHIP_MPLAB_HARMONY) 01318 #include <system/tmr/sys_tmr.h> 01319 01320 /* The time in milliseconds. 01321 * Used for tickets to represent difference between when first seen and when 01322 * sending. 01323 * 01324 * returns the time in milliseconds as a 32-bit value. 01325 */ 01326 word32 TimeNowInMilliseconds(void) 01327 { 01328 return (word32)(SYS_TMR_TickCountGet() / 01329 (SYS_TMR_TickCounterFrequencyGet() / 1000)); 01330 } 01331 #else 01332 /* The time in milliseconds. 01333 * Used for tickets to represent difference between when first seen and when 01334 * sending. 01335 * 01336 * returns the time in milliseconds as a 32-bit value. 01337 */ 01338 word32 TimeNowInMilliseconds(void) 01339 { 01340 return (word32)(SYS_TICK_Get() / (SYS_TICK_TicksPerSecondGet() / 1000)); 01341 } 01342 01343 #endif 01344 01345 #elif defined(FREESCALE_MQX) || defined(FREESCALE_KSDK_MQX) 01346 /* The time in milliseconds. 01347 * Used for tickets to represent difference between when first seen and when 01348 * sending. 01349 * 01350 * returns the time in milliseconds as a 32-bit value. 01351 */ 01352 word32 TimeNowInMilliseconds(void) 01353 { 01354 TIME_STRUCT mqxTime; 01355 01356 _time_get_elapsed(&mqxTime); 01357 01358 return (word32) mqxTime.SECONDS * 1000; 01359 } 01360 #elif defined(FREESCALE_FREE_RTOS) || defined(FREESCALE_KSDK_FREERTOS) 01361 #include "include/task.h" 01362 01363 /* The time in milliseconds. 01364 * Used for tickets to represent difference between when first seen and when 01365 * sending. 01366 * 01367 * returns the time in milliseconds as a 32-bit value. 01368 */ 01369 word32 TimeNowInMilliseconds(void) 01370 { 01371 return (unsigned int)(((float)xTaskGetTickCount()) / 01372 (configTICK_RATE_HZ / 1000)); 01373 } 01374 #elif defined(FREESCALE_KSDK_BM) 01375 #include "lwip/sys.h" /* lwIP */ 01376 01377 /* The time in milliseconds. 01378 * Used for tickets to represent difference between when first seen and when 01379 * sending. 01380 * 01381 * returns the time in milliseconds as a 32-bit value. 01382 */ 01383 word32 TimeNowInMilliseconds(void) 01384 { 01385 return sys_now(); 01386 } 01387 #elif defined(WOLFSSL_TIRTOS) 01388 /* The time in milliseconds. 01389 * Used for tickets to represent difference between when first seen and when 01390 * sending. 01391 * 01392 * returns the time in milliseconds as a 32-bit value. 01393 */ 01394 word32 TimeNowInMilliseconds(void) 01395 { 01396 return (word32) Seconds_get() * 1000; 01397 } 01398 #elif defined(WOLFSSL_UTASKER) 01399 /* The time in milliseconds. 01400 * Used for tickets to represent difference between when first seen and when 01401 * sending. 01402 * 01403 * returns the time in milliseconds as a 32-bit value. 01404 */ 01405 word32 TimeNowInMilliseconds(void) 01406 { 01407 return (word32)(uTaskerSystemTick / (TICK_RESOLUTION / 1000)); 01408 } 01409 #else 01410 /* The time in milliseconds. 01411 * Used for tickets to represent difference between when first seen and when 01412 * sending. 01413 * 01414 * returns the time in milliseconds as a 32-bit value. 01415 */ 01416 word32 TimeNowInMilliseconds(void) 01417 { 01418 struct timeval now; 01419 01420 if (gettimeofday(&now, 0) < 0) 01421 return GETTIME_ERROR; 01422 /* Convert to milliseconds number. */ 01423 return (word32)(now.tv_sec * 1000 + now.tv_usec / 1000); 01424 } 01425 #endif 01426 #endif /* HAVE_SESSION_TICKET || !NO_PSK */ 01427 01428 01429 #if !defined(NO_WOLFSSL_SERVER) && (defined(HAVE_SESSION_TICKET) || \ 01430 !defined(NO_PSK)) 01431 /* Add input to all handshake hashes. 01432 * 01433 * ssl The SSL/TLS object. 01434 * input The data to hash. 01435 * sz The size of the data to hash. 01436 * returns 0 on success, otherwise failure. 01437 */ 01438 static int HashInputRaw(WOLFSSL* ssl, const byte* input, int sz) 01439 { 01440 int ret = BAD_FUNC_ARG; 01441 01442 #ifndef NO_SHA256 01443 ret = wc_Sha256Update(&ssl->hsHashes->hashSha256, input, sz); 01444 if (ret != 0) 01445 return ret; 01446 #endif 01447 #ifdef WOLFSSL_SHA384 01448 ret = wc_Sha384Update(&ssl->hsHashes->hashSha384, input, sz); 01449 if (ret != 0) 01450 return ret; 01451 #endif 01452 #ifdef WOLFSSL_TLS13_SHA512 01453 ret = wc_Sha512Update(&ssl->hsHashes->hashSha512, input, sz); 01454 if (ret != 0) 01455 return ret; 01456 #endif 01457 01458 return ret; 01459 } 01460 #endif 01461 01462 /* Extract the handshake header information. 01463 * 01464 * ssl The SSL/TLS object. 01465 * input The buffer holding the message data. 01466 * inOutIdx On entry, the index into the buffer of the handshake data. 01467 * On exit, the start of the handshake data. 01468 * type Type of handshake message. 01469 * size The length of the handshake message data. 01470 * totalSz The total size of data in the buffer. 01471 * returns BUFFER_E if there is not enough input data and 0 on success. 01472 */ 01473 static int GetHandshakeHeader(WOLFSSL* ssl, const byte* input, word32* inOutIdx, 01474 byte* type, word32* size, word32 totalSz) 01475 { 01476 const byte* ptr = input + *inOutIdx; 01477 (void)ssl; 01478 01479 *inOutIdx += HANDSHAKE_HEADER_SZ; 01480 if (*inOutIdx > totalSz) 01481 return BUFFER_E; 01482 01483 *type = ptr[0]; 01484 c24to32(&ptr[1], size); 01485 01486 return 0; 01487 } 01488 01489 /* Add record layer header to message. 01490 * 01491 * output The buffer to write the record layer header into. 01492 * length The length of the record data. 01493 * type The type of record message. 01494 * ssl The SSL/TLS object. 01495 */ 01496 static void AddTls13RecordHeader(byte* output, word32 length, byte type, 01497 WOLFSSL* ssl) 01498 { 01499 RecordLayerHeader* rl; 01500 01501 rl = (RecordLayerHeader*)output; 01502 rl->type = type; 01503 rl->pvMajor = ssl->version.major; 01504 #ifdef WOLFSSL_TLS13_DRAFT_18 01505 rl->pvMinor = TLSv1_MINOR; 01506 #else 01507 /* NOTE: May be TLSv1_MINOR when sending first ClientHello. */ 01508 rl->pvMinor = TLSv1_2_MINOR; 01509 #endif 01510 c16toa((word16)length, rl->length); 01511 } 01512 01513 /* Add handshake header to message. 01514 * 01515 * output The buffer to write the handshake header into. 01516 * length The length of the handshake data. 01517 * fragOffset The offset of the fragment data. (DTLS) 01518 * fragLength The length of the fragment data. (DTLS) 01519 * type The type of handshake message. 01520 * ssl The SSL/TLS object. (DTLS) 01521 */ 01522 static void AddTls13HandShakeHeader(byte* output, word32 length, 01523 word32 fragOffset, word32 fragLength, 01524 byte type, WOLFSSL* ssl) 01525 { 01526 HandShakeHeader* hs; 01527 (void)fragOffset; 01528 (void)fragLength; 01529 (void)ssl; 01530 01531 /* handshake header */ 01532 hs = (HandShakeHeader*)output; 01533 hs->type = type; 01534 c32to24(length, hs->length); 01535 } 01536 01537 01538 /* Add both record layer and handshake header to message. 01539 * 01540 * output The buffer to write the headers into. 01541 * length The length of the handshake data. 01542 * type The type of record layer message. 01543 * ssl The SSL/TLS object. (DTLS) 01544 */ 01545 static void AddTls13Headers(byte* output, word32 length, byte type, 01546 WOLFSSL* ssl) 01547 { 01548 word32 lengthAdj = HANDSHAKE_HEADER_SZ; 01549 word32 outputAdj = RECORD_HEADER_SZ; 01550 01551 AddTls13RecordHeader(output, length + lengthAdj, handshake, ssl); 01552 AddTls13HandShakeHeader(output + outputAdj, length, 0, length, type, ssl); 01553 } 01554 01555 01556 #ifndef NO_CERTS 01557 /* Add both record layer and fragment handshake header to message. 01558 * 01559 * output The buffer to write the headers into. 01560 * fragOffset The offset of the fragment data. (DTLS) 01561 * fragLength The length of the fragment data. (DTLS) 01562 * length The length of the handshake data. 01563 * type The type of record layer message. 01564 * ssl The SSL/TLS object. (DTLS) 01565 */ 01566 static void AddTls13FragHeaders(byte* output, word32 fragSz, word32 fragOffset, 01567 word32 length, byte type, WOLFSSL* ssl) 01568 { 01569 word32 lengthAdj = HANDSHAKE_HEADER_SZ; 01570 word32 outputAdj = RECORD_HEADER_SZ; 01571 (void)fragSz; 01572 01573 AddTls13RecordHeader(output, fragSz + lengthAdj, handshake, ssl); 01574 AddTls13HandShakeHeader(output + outputAdj, length, fragOffset, fragSz, 01575 type, ssl); 01576 } 01577 #endif /* NO_CERTS */ 01578 01579 /* Write the sequence number into the buffer. 01580 * No DTLS v1.3 support. 01581 * 01582 * ssl The SSL/TLS object. 01583 * verifyOrder Which set of sequence numbers to use. 01584 * out The buffer to write into. 01585 */ 01586 static WC_INLINE void WriteSEQ(WOLFSSL* ssl, int verifyOrder, byte* out) 01587 { 01588 word32 seq[2] = {0, 0}; 01589 01590 if (verifyOrder) { 01591 seq[0] = ssl->keys.peer_sequence_number_hi; 01592 seq[1] = ssl->keys.peer_sequence_number_lo++; 01593 /* handle rollover */ 01594 if (seq[1] > ssl->keys.peer_sequence_number_lo) 01595 ssl->keys.peer_sequence_number_hi++; 01596 } 01597 else { 01598 seq[0] = ssl->keys.sequence_number_hi; 01599 seq[1] = ssl->keys.sequence_number_lo++; 01600 /* handle rollover */ 01601 if (seq[1] > ssl->keys.sequence_number_lo) 01602 ssl->keys.sequence_number_hi++; 01603 } 01604 01605 c32toa(seq[0], out); 01606 c32toa(seq[1], out + OPAQUE32_LEN); 01607 } 01608 01609 /* Build the nonce for TLS v1.3 encryption and decryption. 01610 * 01611 * ssl The SSL/TLS object. 01612 * nonce The nonce data to use when encrypting or decrypting. 01613 * iv The derived IV. 01614 * order The side on which the message is to be or was sent. 01615 */ 01616 static WC_INLINE void BuildTls13Nonce(WOLFSSL* ssl, byte* nonce, const byte* iv, 01617 int order) 01618 { 01619 int i; 01620 01621 /* The nonce is the IV with the sequence XORed into the last bytes. */ 01622 WriteSEQ(ssl, order, nonce + AEAD_NONCE_SZ - SEQ_SZ); 01623 for (i = 0; i < AEAD_NONCE_SZ - SEQ_SZ; i++) 01624 nonce[i] = iv[i]; 01625 for (; i < AEAD_NONCE_SZ; i++) 01626 nonce[i] ^= iv[i]; 01627 } 01628 01629 #if defined(HAVE_CHACHA) && defined(HAVE_POLY1305) 01630 /* Encrypt with ChaCha20 and create authenication tag with Poly1305. 01631 * 01632 * ssl The SSL/TLS object. 01633 * output The buffer to write encrypted data and authentication tag into. 01634 * May be the same pointer as input. 01635 * input The data to encrypt. 01636 * sz The number of bytes to encrypt. 01637 * nonce The nonce to use with ChaCha20. 01638 * aad The additional authentication data. 01639 * aadSz The size of the addition authentication data. 01640 * tag The authentication tag buffer. 01641 * returns 0 on success, otherwise failure. 01642 */ 01643 static int ChaCha20Poly1305_Encrypt(WOLFSSL* ssl, byte* output, 01644 const byte* input, word16 sz, byte* nonce, 01645 const byte* aad, word16 aadSz, byte* tag) 01646 { 01647 int ret = 0; 01648 byte poly[CHACHA20_256_KEY_SIZE]; 01649 01650 /* Poly1305 key is 256 bits of zero encrypted with ChaCha20. */ 01651 XMEMSET(poly, 0, sizeof(poly)); 01652 01653 /* Set the nonce for ChaCha and get Poly1305 key. */ 01654 ret = wc_Chacha_SetIV(ssl->encrypt.chacha, nonce, 0); 01655 if (ret != 0) 01656 return ret; 01657 /* Create Poly1305 key using ChaCha20 keystream. */ 01658 ret = wc_Chacha_Process(ssl->encrypt.chacha, poly, poly, sizeof(poly)); 01659 if (ret != 0) 01660 return ret; 01661 ret = wc_Chacha_SetIV(ssl->encrypt.chacha, nonce, 1); 01662 if (ret != 0) 01663 return ret; 01664 /* Encrypt the plain text. */ 01665 ret = wc_Chacha_Process(ssl->encrypt.chacha, output, input, sz); 01666 if (ret != 0) { 01667 ForceZero(poly, sizeof(poly)); 01668 return ret; 01669 } 01670 01671 /* Set key for Poly1305. */ 01672 ret = wc_Poly1305SetKey(ssl->auth.poly1305, poly, sizeof(poly)); 01673 ForceZero(poly, sizeof(poly)); /* done with poly1305 key, clear it */ 01674 if (ret != 0) 01675 return ret; 01676 /* Add authentication code of encrypted data to end. */ 01677 ret = wc_Poly1305_MAC(ssl->auth.poly1305, (byte*)aad, aadSz, output, sz, 01678 tag, POLY1305_AUTH_SZ); 01679 01680 return ret; 01681 } 01682 #endif 01683 01684 #ifdef HAVE_NULL_CIPHER 01685 /* Create authenication tag and copy data over input. 01686 * 01687 * ssl The SSL/TLS object. 01688 * output The buffer to copy data into. 01689 * May be the same pointer as input. 01690 * input The data. 01691 * sz The number of bytes of data. 01692 * nonce The nonce to use with authentication. 01693 * aad The additional authentication data. 01694 * aadSz The size of the addition authentication data. 01695 * tag The authentication tag buffer. 01696 * returns 0 on success, otherwise failure. 01697 */ 01698 static int Tls13IntegrityOnly_Encrypt(WOLFSSL* ssl, byte* output, 01699 const byte* input, word16 sz, 01700 const byte* nonce, 01701 const byte* aad, word16 aadSz, byte* tag) 01702 { 01703 int ret; 01704 01705 /* HMAC: nonce | aad | input */ 01706 ret = wc_HmacUpdate(ssl->encrypt.hmac, nonce, HMAC_NONCE_SZ); 01707 if (ret == 0) 01708 ret = wc_HmacUpdate(ssl->encrypt.hmac, aad, aadSz); 01709 if (ret == 0) 01710 ret = wc_HmacUpdate(ssl->encrypt.hmac, input, sz); 01711 if (ret == 0) 01712 ret = wc_HmacFinal(ssl->encrypt.hmac, tag); 01713 /* Copy the input to output if not the same buffer */ 01714 if (ret == 0 && output != input) 01715 XMEMCPY(output, input, sz); 01716 01717 return ret; 01718 } 01719 #endif 01720 01721 /* Encrypt data for TLS v1.3. 01722 * 01723 * ssl The SSL/TLS object. 01724 * output The buffer to write encrypted data and authentication tag into. 01725 * May be the same pointer as input. 01726 * input The record header and data to encrypt. 01727 * sz The number of bytes to encrypt. 01728 * aad The additional authentication data. 01729 * aadSz The size of the addition authentication data. 01730 * asyncOkay If non-zero can return WC_PENDING_E, otherwise blocks on crypto 01731 * returns 0 on success, otherwise failure. 01732 */ 01733 static int EncryptTls13(WOLFSSL* ssl, byte* output, const byte* input, 01734 word16 sz, const byte* aad, word16 aadSz, int asyncOkay) 01735 { 01736 int ret = 0; 01737 word16 dataSz = sz - ssl->specs.aead_mac_size; 01738 word16 macSz = ssl->specs.aead_mac_size; 01739 word32 nonceSz = 0; 01740 #ifdef WOLFSSL_ASYNC_CRYPT 01741 WC_ASYNC_DEV* asyncDev = NULL; 01742 word32 event_flags = WC_ASYNC_FLAG_CALL_AGAIN; 01743 #endif 01744 01745 WOLFSSL_ENTER("EncryptTls13"); 01746 01747 (void)output; 01748 (void)input; 01749 (void)sz; 01750 (void)dataSz; 01751 (void)macSz; 01752 (void)asyncOkay; 01753 (void)nonceSz; 01754 01755 #ifdef WOLFSSL_ASYNC_CRYPT 01756 if (ssl->error == WC_PENDING_E) { 01757 ssl->error = 0; /* clear async */ 01758 } 01759 #endif 01760 01761 switch (ssl->encrypt.state) { 01762 case CIPHER_STATE_BEGIN: 01763 { 01764 #ifdef WOLFSSL_DEBUG_TLS 01765 WOLFSSL_MSG("Data to encrypt"); 01766 WOLFSSL_BUFFER(input, dataSz); 01767 #if !defined(WOLFSSL_TLS13_DRAFT_18) && !defined(WOLFSSL_TLS13_DRAFT_22) && \ 01768 !defined(WOLFSSL_TLS13_DRAFT_23) 01769 WOLFSSL_MSG("Additional Authentication Data"); 01770 WOLFSSL_BUFFER(aad, aadSz); 01771 #endif 01772 #endif 01773 01774 #ifdef CIPHER_NONCE 01775 if (ssl->encrypt.nonce == NULL) 01776 ssl->encrypt.nonce = (byte*)XMALLOC(AEAD_NONCE_SZ, 01777 ssl->heap, DYNAMIC_TYPE_AES_BUFFER); 01778 if (ssl->encrypt.nonce == NULL) 01779 return MEMORY_E; 01780 01781 BuildTls13Nonce(ssl, ssl->encrypt.nonce, ssl->keys.aead_enc_imp_IV, 01782 CUR_ORDER); 01783 #endif 01784 01785 /* Advance state and proceed */ 01786 ssl->encrypt.state = CIPHER_STATE_DO; 01787 } 01788 FALL_THROUGH; 01789 01790 case CIPHER_STATE_DO: 01791 { 01792 switch (ssl->specs.bulk_cipher_algorithm) { 01793 #ifdef BUILD_AESGCM 01794 case wolfssl_aes_gcm: 01795 #ifdef WOLFSSL_ASYNC_CRYPT 01796 /* initialize event */ 01797 asyncDev = &ssl->encrypt.aes->asyncDev; 01798 ret = wolfSSL_AsyncInit(ssl, asyncDev, event_flags); 01799 if (ret != 0) 01800 break; 01801 #endif 01802 01803 nonceSz = AESGCM_NONCE_SZ; 01804 #if ((defined(HAVE_FIPS) || defined(HAVE_SELFTEST)) && \ 01805 (!defined(HAVE_FIPS_VERSION) || (HAVE_FIPS_VERSION < 2))) 01806 ret = wc_AesGcmEncrypt(ssl->encrypt.aes, output, input, 01807 dataSz, ssl->encrypt.nonce, nonceSz, 01808 output + dataSz, macSz, aad, aadSz); 01809 #else 01810 ret = wc_AesGcmSetExtIV(ssl->encrypt.aes, 01811 ssl->encrypt.nonce, nonceSz); 01812 if (ret == 0) { 01813 ret = wc_AesGcmEncrypt_ex(ssl->encrypt.aes, output, 01814 input, dataSz, ssl->encrypt.nonce, nonceSz, 01815 output + dataSz, macSz, aad, aadSz); 01816 } 01817 #endif 01818 break; 01819 #endif 01820 01821 #ifdef HAVE_AESCCM 01822 case wolfssl_aes_ccm: 01823 #ifdef WOLFSSL_ASYNC_CRYPT 01824 /* initialize event */ 01825 asyncDev = &ssl->encrypt.aes->asyncDev; 01826 ret = wolfSSL_AsyncInit(ssl, asyncDev, event_flags); 01827 if (ret != 0) 01828 break; 01829 #endif 01830 01831 nonceSz = AESCCM_NONCE_SZ; 01832 #if ((defined(HAVE_FIPS) || defined(HAVE_SELFTEST)) && \ 01833 (!defined(HAVE_FIPS_VERSION) || (HAVE_FIPS_VERSION < 2))) 01834 ret = wc_AesCcmEncrypt(ssl->encrypt.aes, output, input, 01835 dataSz, ssl->encrypt.nonce, nonceSz, 01836 output + dataSz, macSz, aad, aadSz); 01837 #else 01838 ret = wc_AesCcmSetNonce(ssl->encrypt.aes, 01839 ssl->encrypt.nonce, nonceSz); 01840 if (ret == 0) { 01841 ret = wc_AesCcmEncrypt_ex(ssl->encrypt.aes, output, 01842 input, dataSz, ssl->encrypt.nonce, nonceSz, 01843 output + dataSz, macSz, aad, aadSz); 01844 } 01845 #endif 01846 break; 01847 #endif 01848 01849 #if defined(HAVE_CHACHA) && defined(HAVE_POLY1305) 01850 case wolfssl_chacha: 01851 ret = ChaCha20Poly1305_Encrypt(ssl, output, input, dataSz, 01852 ssl->encrypt.nonce, aad, aadSz, output + dataSz); 01853 break; 01854 #endif 01855 01856 #ifdef HAVE_NULL_CIPHER 01857 case wolfssl_cipher_null: 01858 ret = Tls13IntegrityOnly_Encrypt(ssl, output, input, dataSz, 01859 ssl->encrypt.nonce, aad, aadSz, output + dataSz); 01860 break; 01861 #endif 01862 01863 default: 01864 WOLFSSL_MSG("wolfSSL Encrypt programming error"); 01865 return ENCRYPT_ERROR; 01866 } 01867 01868 /* Advance state */ 01869 ssl->encrypt.state = CIPHER_STATE_END; 01870 01871 #ifdef WOLFSSL_ASYNC_CRYPT 01872 if (ret == WC_PENDING_E) { 01873 /* if async is not okay, then block */ 01874 if (!asyncOkay) { 01875 ret = wc_AsyncWait(ret, asyncDev, event_flags); 01876 } 01877 else { 01878 /* If pending, then leave and return will resume below */ 01879 return wolfSSL_AsyncPush(ssl, asyncDev); 01880 } 01881 } 01882 #endif 01883 } 01884 FALL_THROUGH; 01885 01886 case CIPHER_STATE_END: 01887 { 01888 #ifdef WOLFSSL_DEBUG_TLS 01889 #ifdef CIPHER_NONCE 01890 WOLFSSL_MSG("Nonce"); 01891 WOLFSSL_BUFFER(ssl->encrypt.nonce, ssl->specs.iv_size); 01892 #endif 01893 WOLFSSL_MSG("Encrypted data"); 01894 WOLFSSL_BUFFER(output, dataSz); 01895 WOLFSSL_MSG("Authentication Tag"); 01896 WOLFSSL_BUFFER(output + dataSz, macSz); 01897 #endif 01898 01899 #ifdef CIPHER_NONCE 01900 ForceZero(ssl->encrypt.nonce, AEAD_NONCE_SZ); 01901 #endif 01902 01903 break; 01904 } 01905 } 01906 01907 /* Reset state */ 01908 ssl->encrypt.state = CIPHER_STATE_BEGIN; 01909 01910 return ret; 01911 } 01912 01913 #if defined(HAVE_CHACHA) && defined(HAVE_POLY1305) 01914 /* Decrypt with ChaCha20 and check authenication tag with Poly1305. 01915 * 01916 * ssl The SSL/TLS object. 01917 * output The buffer to write decrypted data into. 01918 * May be the same pointer as input. 01919 * input The data to decrypt. 01920 * sz The number of bytes to decrypt. 01921 * nonce The nonce to use with ChaCha20. 01922 * aad The additional authentication data. 01923 * aadSz The size of the addition authentication data. 01924 * tagIn The authentication tag data from packet. 01925 * returns 0 on success, otherwise failure. 01926 */ 01927 static int ChaCha20Poly1305_Decrypt(WOLFSSL* ssl, byte* output, 01928 const byte* input, word16 sz, byte* nonce, 01929 const byte* aad, word16 aadSz, 01930 const byte* tagIn) 01931 { 01932 int ret; 01933 byte tag[POLY1305_AUTH_SZ]; 01934 byte poly[CHACHA20_256_KEY_SIZE]; /* generated key for mac */ 01935 01936 /* Poly1305 key is 256 bits of zero encrypted with ChaCha20. */ 01937 XMEMSET(poly, 0, sizeof(poly)); 01938 01939 /* Set nonce and get Poly1305 key. */ 01940 ret = wc_Chacha_SetIV(ssl->decrypt.chacha, nonce, 0); 01941 if (ret != 0) 01942 return ret; 01943 /* Use ChaCha20 keystream to get Poly1305 key for tag. */ 01944 ret = wc_Chacha_Process(ssl->decrypt.chacha, poly, poly, sizeof(poly)); 01945 if (ret != 0) 01946 return ret; 01947 ret = wc_Chacha_SetIV(ssl->decrypt.chacha, nonce, 1); 01948 if (ret != 0) 01949 return ret; 01950 01951 /* Set key for Poly1305. */ 01952 ret = wc_Poly1305SetKey(ssl->auth.poly1305, poly, sizeof(poly)); 01953 ForceZero(poly, sizeof(poly)); /* done with poly1305 key, clear it */ 01954 if (ret != 0) 01955 return ret; 01956 /* Generate authentication tag for encrypted data. */ 01957 if ((ret = wc_Poly1305_MAC(ssl->auth.poly1305, (byte*)aad, aadSz, 01958 (byte*)input, sz, tag, sizeof(tag))) != 0) { 01959 return ret; 01960 } 01961 01962 /* Check tag sent along with packet. */ 01963 if (ConstantCompare(tagIn, tag, POLY1305_AUTH_SZ) != 0) { 01964 WOLFSSL_MSG("MAC did not match"); 01965 return VERIFY_MAC_ERROR; 01966 } 01967 01968 /* If the tag was good decrypt message. */ 01969 ret = wc_Chacha_Process(ssl->decrypt.chacha, output, input, sz); 01970 01971 return ret; 01972 } 01973 #endif 01974 01975 #ifdef HAVE_NULL_CIPHER 01976 /* Check HMAC tag and copy over input. 01977 * 01978 * ssl The SSL/TLS object. 01979 * output The buffer to copy data into. 01980 * May be the same pointer as input. 01981 * input The data. 01982 * sz The number of bytes of data. 01983 * nonce The nonce to use with authentication. 01984 * aad The additional authentication data. 01985 * aadSz The size of the addition authentication data. 01986 * tagIn The authentication tag data from packet. 01987 * returns 0 on success, otherwise failure. 01988 */ 01989 static int Tls13IntegrityOnly_Decrypt(WOLFSSL* ssl, byte* output, 01990 const byte* input, word16 sz, 01991 const byte* nonce, 01992 const byte* aad, word16 aadSz, 01993 const byte* tagIn) 01994 { 01995 int ret; 01996 byte hmac[WC_MAX_DIGEST_SIZE]; 01997 01998 /* HMAC: nonce | aad | input */ 01999 ret = wc_HmacUpdate(ssl->decrypt.hmac, nonce, HMAC_NONCE_SZ); 02000 if (ret == 0) 02001 ret = wc_HmacUpdate(ssl->decrypt.hmac, aad, aadSz); 02002 if (ret == 0) 02003 ret = wc_HmacUpdate(ssl->decrypt.hmac, input, sz); 02004 if (ret == 0) 02005 ret = wc_HmacFinal(ssl->decrypt.hmac, hmac); 02006 /* Check authentication tag matches */ 02007 if (ret == 0 && ConstantCompare(tagIn, hmac, ssl->specs.hash_size) != 0) 02008 ret = DECRYPT_ERROR; 02009 /* Copy the input to output if not the same buffer */ 02010 if (ret == 0 && output != input) 02011 XMEMCPY(output, input, sz); 02012 02013 return ret; 02014 } 02015 #endif 02016 02017 /* Decrypt data for TLS v1.3. 02018 * 02019 * ssl The SSL/TLS object. 02020 * output The buffer to write decrypted data into. 02021 * May be the same pointer as input. 02022 * input The data to decrypt and authentication tag. 02023 * sz The length of the encrypted data plus authentication tag. 02024 * aad The additional authentication data. 02025 * aadSz The size of the addition authentication data. 02026 * returns 0 on success, otherwise failure. 02027 */ 02028 int DecryptTls13(WOLFSSL* ssl, byte* output, const byte* input, word16 sz, 02029 const byte* aad, word16 aadSz) 02030 { 02031 int ret = 0; 02032 word16 dataSz = sz - ssl->specs.aead_mac_size; 02033 word16 macSz = ssl->specs.aead_mac_size; 02034 word32 nonceSz = 0; 02035 02036 WOLFSSL_ENTER("DecryptTls13"); 02037 02038 #ifdef WOLFSSL_ASYNC_CRYPT 02039 ret = wolfSSL_AsyncPop(ssl, &ssl->decrypt.state); 02040 if (ret != WC_NOT_PENDING_E) { 02041 /* check for still pending */ 02042 if (ret == WC_PENDING_E) 02043 return ret; 02044 02045 ssl->error = 0; /* clear async */ 02046 02047 /* let failures through so CIPHER_STATE_END logic is run */ 02048 } 02049 else 02050 #endif 02051 { 02052 /* Reset state */ 02053 ret = 0; 02054 ssl->decrypt.state = CIPHER_STATE_BEGIN; 02055 } 02056 02057 (void)output; 02058 (void)input; 02059 (void)sz; 02060 (void)dataSz; 02061 (void)macSz; 02062 (void)nonceSz; 02063 02064 switch (ssl->decrypt.state) { 02065 case CIPHER_STATE_BEGIN: 02066 { 02067 #ifdef WOLFSSL_DEBUG_TLS 02068 WOLFSSL_MSG("Data to decrypt"); 02069 WOLFSSL_BUFFER(input, dataSz); 02070 #if !defined(WOLFSSL_TLS13_DRAFT_18) && !defined(WOLFSSL_TLS13_DRAFT_22) && \ 02071 !defined(WOLFSSL_TLS13_DRAFT_23) 02072 WOLFSSL_MSG("Additional Authentication Data"); 02073 WOLFSSL_BUFFER(aad, aadSz); 02074 #endif 02075 WOLFSSL_MSG("Authentication tag"); 02076 WOLFSSL_BUFFER(input + dataSz, macSz); 02077 #endif 02078 02079 #ifdef CIPHER_NONCE 02080 if (ssl->decrypt.nonce == NULL) 02081 ssl->decrypt.nonce = (byte*)XMALLOC(AEAD_NONCE_SZ, 02082 ssl->heap, DYNAMIC_TYPE_AES_BUFFER); 02083 if (ssl->decrypt.nonce == NULL) 02084 return MEMORY_E; 02085 02086 BuildTls13Nonce(ssl, ssl->decrypt.nonce, ssl->keys.aead_dec_imp_IV, 02087 PEER_ORDER); 02088 #endif 02089 02090 /* Advance state and proceed */ 02091 ssl->decrypt.state = CIPHER_STATE_DO; 02092 } 02093 FALL_THROUGH; 02094 02095 case CIPHER_STATE_DO: 02096 { 02097 switch (ssl->specs.bulk_cipher_algorithm) { 02098 #ifdef BUILD_AESGCM 02099 case wolfssl_aes_gcm: 02100 #ifdef WOLFSSL_ASYNC_CRYPT 02101 /* initialize event */ 02102 ret = wolfSSL_AsyncInit(ssl, &ssl->decrypt.aes->asyncDev, 02103 WC_ASYNC_FLAG_CALL_AGAIN); 02104 if (ret != 0) 02105 break; 02106 #endif 02107 02108 nonceSz = AESGCM_NONCE_SZ; 02109 ret = wc_AesGcmDecrypt(ssl->decrypt.aes, output, input, 02110 dataSz, ssl->decrypt.nonce, nonceSz, 02111 input + dataSz, macSz, aad, aadSz); 02112 #ifdef WOLFSSL_ASYNC_CRYPT 02113 if (ret == WC_PENDING_E) { 02114 ret = wolfSSL_AsyncPush(ssl, 02115 &ssl->decrypt.aes->asyncDev); 02116 } 02117 #endif 02118 break; 02119 #endif 02120 02121 #ifdef HAVE_AESCCM 02122 case wolfssl_aes_ccm: 02123 #ifdef WOLFSSL_ASYNC_CRYPT 02124 /* initialize event */ 02125 ret = wolfSSL_AsyncInit(ssl, &ssl->decrypt.aes->asyncDev, 02126 WC_ASYNC_FLAG_CALL_AGAIN); 02127 if (ret != 0) 02128 break; 02129 #endif 02130 02131 nonceSz = AESCCM_NONCE_SZ; 02132 ret = wc_AesCcmDecrypt(ssl->decrypt.aes, output, input, 02133 dataSz, ssl->decrypt.nonce, nonceSz, 02134 input + dataSz, macSz, aad, aadSz); 02135 #ifdef WOLFSSL_ASYNC_CRYPT 02136 if (ret == WC_PENDING_E) { 02137 ret = wolfSSL_AsyncPush(ssl, 02138 &ssl->decrypt.aes->asyncDev); 02139 } 02140 #endif 02141 break; 02142 #endif 02143 02144 #if defined(HAVE_CHACHA) && defined(HAVE_POLY1305) 02145 case wolfssl_chacha: 02146 ret = ChaCha20Poly1305_Decrypt(ssl, output, input, dataSz, 02147 ssl->decrypt.nonce, aad, aadSz, input + dataSz); 02148 break; 02149 #endif 02150 02151 #ifdef HAVE_NULL_CIPHER 02152 case wolfssl_cipher_null: 02153 ret = Tls13IntegrityOnly_Decrypt(ssl, output, input, dataSz, 02154 ssl->decrypt.nonce, aad, aadSz, input + dataSz); 02155 break; 02156 #endif 02157 default: 02158 WOLFSSL_MSG("wolfSSL Decrypt programming error"); 02159 return DECRYPT_ERROR; 02160 } 02161 02162 /* Advance state */ 02163 ssl->decrypt.state = CIPHER_STATE_END; 02164 02165 #ifdef WOLFSSL_ASYNC_CRYPT 02166 /* If pending, leave now */ 02167 if (ret == WC_PENDING_E) { 02168 return ret; 02169 } 02170 #endif 02171 } 02172 FALL_THROUGH; 02173 02174 case CIPHER_STATE_END: 02175 { 02176 #ifdef WOLFSSL_DEBUG_TLS 02177 #ifdef CIPHER_NONCE 02178 WOLFSSL_MSG("Nonce"); 02179 WOLFSSL_BUFFER(ssl->decrypt.nonce, ssl->specs.iv_size); 02180 #endif 02181 WOLFSSL_MSG("Decrypted data"); 02182 WOLFSSL_BUFFER(output, dataSz); 02183 #endif 02184 02185 #ifdef CIPHER_NONCE 02186 ForceZero(ssl->decrypt.nonce, AEAD_NONCE_SZ); 02187 #endif 02188 02189 break; 02190 } 02191 } 02192 02193 #ifndef WOLFSSL_EARLY_DATA 02194 if (ret < 0) { 02195 SendAlert(ssl, alert_fatal, bad_record_mac); 02196 ret = VERIFY_MAC_ERROR; 02197 } 02198 #endif 02199 02200 return ret; 02201 } 02202 02203 /* Persistable BuildTls13Message arguments */ 02204 typedef struct BuildMsg13Args { 02205 word32 sz; 02206 word32 idx; 02207 word32 headerSz; 02208 word16 size; 02209 } BuildMsg13Args; 02210 02211 static void FreeBuildMsg13Args(WOLFSSL* ssl, void* pArgs) 02212 { 02213 BuildMsg13Args* args = (BuildMsg13Args*)pArgs; 02214 02215 (void)ssl; 02216 (void)args; 02217 02218 /* no allocations in BuildTls13Message */ 02219 } 02220 02221 /* Build SSL Message, encrypted. 02222 * TLS v1.3 encryption is AEAD only. 02223 * 02224 * ssl The SSL/TLS object. 02225 * output The buffer to write record message to. 02226 * outSz Size of the buffer being written into. 02227 * input The record data to encrypt (excluding record header). 02228 * inSz The size of the record data. 02229 * type The recorder header content type. 02230 * hashOutput Whether to hash the unencrypted record data. 02231 * sizeOnly Only want the size of the record message. 02232 * asyncOkay If non-zero can return WC_PENDING_E, otherwise blocks on crypto 02233 * returns the size of the encrypted record message or negative value on error. 02234 */ 02235 int BuildTls13Message(WOLFSSL* ssl, byte* output, int outSz, const byte* input, 02236 int inSz, int type, int hashOutput, int sizeOnly, int asyncOkay) 02237 { 02238 int ret = 0; 02239 BuildMsg13Args* args; 02240 BuildMsg13Args lcl_args; 02241 #ifdef WOLFSSL_ASYNC_CRYPT 02242 args = (BuildMsg13Args*)ssl->async.args; 02243 typedef char args_test[sizeof(ssl->async.args) >= sizeof(*args) ? 1 : -1]; 02244 (void)sizeof(args_test); 02245 #endif 02246 02247 WOLFSSL_ENTER("BuildTls13Message"); 02248 02249 ret = WC_NOT_PENDING_E; 02250 #ifdef WOLFSSL_ASYNC_CRYPT 02251 if (asyncOkay) { 02252 ret = wolfSSL_AsyncPop(ssl, &ssl->options.buildMsgState); 02253 if (ret != WC_NOT_PENDING_E) { 02254 /* Check for error */ 02255 if (ret < 0) 02256 goto exit_buildmsg; 02257 } 02258 } 02259 else 02260 #endif 02261 { 02262 args = &lcl_args; 02263 } 02264 02265 /* Reset state */ 02266 if (ret == WC_NOT_PENDING_E) { 02267 ret = 0; 02268 ssl->options.buildMsgState = BUILD_MSG_BEGIN; 02269 XMEMSET(args, 0, sizeof(BuildMsg13Args)); 02270 02271 args->sz = RECORD_HEADER_SZ + inSz; 02272 args->idx = RECORD_HEADER_SZ; 02273 args->headerSz = RECORD_HEADER_SZ; 02274 #ifdef WOLFSSL_ASYNC_CRYPT 02275 ssl->async.freeArgs = FreeBuildMsg13Args; 02276 #endif 02277 } 02278 02279 switch (ssl->options.buildMsgState) { 02280 case BUILD_MSG_BEGIN: 02281 { 02282 /* catch mistaken sizeOnly parameter */ 02283 if (sizeOnly) { 02284 if (output || input) { 02285 WOLFSSL_MSG("BuildTls13Message with sizeOnly " 02286 "doesn't need input or output"); 02287 return BAD_FUNC_ARG; 02288 } 02289 } 02290 else if (output == NULL || input == NULL) { 02291 return BAD_FUNC_ARG; 02292 } 02293 02294 /* Record layer content type at the end of record data. */ 02295 args->sz++; 02296 /* Authentication data at the end. */ 02297 args->sz += ssl->specs.aead_mac_size; 02298 02299 if (sizeOnly) 02300 return args->sz; 02301 02302 if (args->sz > (word32)outSz) { 02303 WOLFSSL_MSG("Oops, want to write past output buffer size"); 02304 return BUFFER_E; 02305 } 02306 02307 /* Record data length. */ 02308 args->size = (word16)(args->sz - args->headerSz); 02309 /* Write/update the record header with the new size. 02310 * Always have the content type as application data for encrypted 02311 * messages in TLS v1.3. 02312 */ 02313 AddTls13RecordHeader(output, args->size, application_data, ssl); 02314 02315 /* TLS v1.3 can do in place encryption. */ 02316 if (input != output + args->idx) 02317 XMEMCPY(output + args->idx, input, inSz); 02318 args->idx += inSz; 02319 02320 ssl->options.buildMsgState = BUILD_MSG_HASH; 02321 } 02322 FALL_THROUGH; 02323 02324 case BUILD_MSG_HASH: 02325 { 02326 if (hashOutput) { 02327 ret = HashOutput(ssl, output, args->headerSz + inSz, 0); 02328 if (ret != 0) 02329 goto exit_buildmsg; 02330 } 02331 02332 /* The real record content type goes at the end of the data. */ 02333 output[args->idx++] = (byte)type; 02334 02335 ssl->options.buildMsgState = BUILD_MSG_ENCRYPT; 02336 } 02337 FALL_THROUGH; 02338 02339 case BUILD_MSG_ENCRYPT: 02340 { 02341 #ifdef ATOMIC_USER 02342 if (ssl->ctx->MacEncryptCb) { 02343 /* User Record Layer Callback handling */ 02344 byte* mac = output + args->idx; 02345 output += args->headerSz; 02346 02347 ret = ssl->ctx->MacEncryptCb(ssl, mac, output, inSz, type, 0, 02348 output, output, args->size, ssl->MacEncryptCtx); 02349 } 02350 else 02351 #endif 02352 { 02353 #if defined(WOLFSSL_TLS13_DRAFT_18) || defined(WOLFSSL_TLS13_DRAFT_22) || \ 02354 defined(WOLFSSL_TLS13_DRAFT_23) 02355 output += args->headerSz; 02356 ret = EncryptTls13(ssl, output, output, args->size, NULL, 0, 02357 asyncOkay); 02358 #else 02359 const byte* aad = output; 02360 output += args->headerSz; 02361 ret = EncryptTls13(ssl, output, output, args->size, aad, 02362 RECORD_HEADER_SZ, asyncOkay); 02363 #endif 02364 } 02365 break; 02366 } 02367 } 02368 02369 exit_buildmsg: 02370 02371 WOLFSSL_LEAVE("BuildTls13Message", ret); 02372 02373 #ifdef WOLFSSL_ASYNC_CRYPT 02374 if (ret == WC_PENDING_E) { 02375 return ret; 02376 } 02377 #endif 02378 02379 /* make sure build message state is reset */ 02380 ssl->options.buildMsgState = BUILD_MSG_BEGIN; 02381 02382 /* return sz on success */ 02383 if (ret == 0) 02384 ret = args->sz; 02385 02386 /* Final cleanup */ 02387 FreeBuildMsg13Args(ssl, args); 02388 #ifdef WOLFSSL_ASYNC_CRYPT 02389 ssl->async.freeArgs = NULL; 02390 #endif 02391 02392 return ret; 02393 } 02394 02395 #if defined(HAVE_SESSION_TICKET) || !defined(NO_PSK) 02396 /* Find the cipher suite in the suites set in the SSL. 02397 * 02398 * ssl SSL/TLS object. 02399 * suite Cipher suite to look for. 02400 * returns 1 when suite is found in SSL/TLS object's list and 0 otherwise. 02401 */ 02402 static int FindSuiteSSL(WOLFSSL* ssl, byte* suite) 02403 { 02404 word16 i; 02405 02406 for (i = 0; i < ssl->suites->suiteSz; i += 2) { 02407 if (ssl->suites->suites[i+0] == suite[0] && 02408 ssl->suites->suites[i+1] == suite[1]) { 02409 return 1; 02410 } 02411 } 02412 02413 return 0; 02414 } 02415 #endif 02416 02417 #ifndef WOLFSSL_TLS13_DRAFT_18 02418 #if defined(WOLFSSL_SEND_HRR_COOKIE) && !defined(NO_WOLFSSL_SERVER) 02419 /* Create Cookie extension using the hash of the first ClientHello. 02420 * 02421 * ssl SSL/TLS object. 02422 * hash The hash data. 02423 * hashSz The size of the hash data in bytes. 02424 * returns 0 on success, otherwise failure. 02425 */ 02426 static int CreateCookie(WOLFSSL* ssl, byte* hash, byte hashSz) 02427 { 02428 int ret; 02429 byte mac[WC_MAX_DIGEST_SIZE] = {0}; 02430 Hmac cookieHmac; 02431 byte cookieType = 0; 02432 byte macSz = 0; 02433 02434 #if !defined(NO_SHA) && defined(NO_SHA256) 02435 cookieType = SHA; 02436 macSz = WC_SHA_DIGEST_SIZE; 02437 #endif /* NO_SHA */ 02438 #ifndef NO_SHA256 02439 cookieType = WC_SHA256; 02440 macSz = WC_SHA256_DIGEST_SIZE; 02441 #endif /* NO_SHA256 */ 02442 XMEMSET(&cookieHmac, 0, sizeof(Hmac)); 02443 02444 ret = wc_HmacSetKey(&cookieHmac, cookieType, 02445 ssl->buffers.tls13CookieSecret.buffer, 02446 ssl->buffers.tls13CookieSecret.length); 02447 if (ret != 0) 02448 return ret; 02449 if ((ret = wc_HmacUpdate(&cookieHmac, hash, hashSz)) != 0) 02450 return ret; 02451 if ((ret = wc_HmacFinal(&cookieHmac, mac)) != 0) 02452 return ret; 02453 02454 /* The cookie data is the hash and the integrity check. */ 02455 return TLSX_Cookie_Use(ssl, hash, hashSz, mac, macSz, 1); 02456 } 02457 #endif 02458 02459 /* Restart the handshake hash with a hash of the previous messages. 02460 * 02461 * ssl The SSL/TLS object. 02462 * returns 0 on success, otherwise failure. 02463 */ 02464 static int RestartHandshakeHash(WOLFSSL* ssl) 02465 { 02466 int ret; 02467 Hashes hashes; 02468 byte header[HANDSHAKE_HEADER_SZ] = {0}; 02469 byte* hash = NULL; 02470 byte hashSz = 0; 02471 02472 ret = BuildCertHashes(ssl, &hashes); 02473 if (ret != 0) 02474 return ret; 02475 switch (ssl->specs.mac_algorithm) { 02476 #ifndef NO_SHA256 02477 case sha256_mac: 02478 hash = hashes.sha256; 02479 break; 02480 #endif 02481 #ifdef WOLFSSL_SHA384 02482 case sha384_mac: 02483 hash = hashes.sha384; 02484 break; 02485 #endif 02486 #ifdef WOLFSSL_TLS13_SHA512 02487 case sha512_mac: 02488 hash = hashes.sha512; 02489 break; 02490 #endif 02491 } 02492 hashSz = ssl->specs.hash_size; 02493 02494 /* check hash */ 02495 if (hash == NULL && hashSz > 0) 02496 return BAD_FUNC_ARG; 02497 02498 AddTls13HandShakeHeader(header, hashSz, 0, 0, message_hash, ssl); 02499 02500 WOLFSSL_MSG("Restart Hash"); 02501 WOLFSSL_BUFFER(hash, hashSz); 02502 02503 #if defined(WOLFSSL_SEND_HRR_COOKIE) && !defined(NO_WOLFSSL_SERVER) 02504 if (ssl->options.sendCookie) { 02505 byte cookie[OPAQUE8_LEN + WC_MAX_DIGEST_SIZE + OPAQUE16_LEN * 2]; 02506 TLSX* ext; 02507 word32 idx = 0; 02508 02509 /* Cookie Data = Hash Len | Hash | CS | KeyShare Group */ 02510 cookie[idx++] = hashSz; 02511 if (hash) 02512 XMEMCPY(cookie + idx, hash, hashSz); 02513 idx += hashSz; 02514 cookie[idx++] = ssl->options.cipherSuite0; 02515 cookie[idx++] = ssl->options.cipherSuite; 02516 if ((ext = TLSX_Find(ssl->extensions, TLSX_KEY_SHARE)) != NULL) { 02517 KeyShareEntry* kse = (KeyShareEntry*)ext->data; 02518 c16toa(kse->group, cookie + idx); 02519 idx += OPAQUE16_LEN; 02520 } 02521 return CreateCookie(ssl, cookie, idx); 02522 } 02523 #endif 02524 02525 ret = InitHandshakeHashes(ssl); 02526 if (ret != 0) 02527 return ret; 02528 ret = HashOutputRaw(ssl, header, sizeof(header)); 02529 if (ret != 0) 02530 return ret; 02531 return HashOutputRaw(ssl, hash, hashSz); 02532 } 02533 02534 /* The value in the random field of a ServerHello to indicate 02535 * HelloRetryRequest. 02536 */ 02537 static byte helloRetryRequestRandom[] = { 02538 0xCF, 0x21, 0xAD, 0x74, 0xE5, 0x9A, 0x61, 0x11, 02539 0xBE, 0x1D, 0x8C, 0x02, 0x1E, 0x65, 0xB8, 0x91, 02540 0xC2, 0xA2, 0x11, 0x16, 0x7A, 0xBB, 0x8C, 0x5E, 02541 0x07, 0x9E, 0x09, 0xE2, 0xC8, 0xA8, 0x33, 0x9C 02542 }; 02543 #endif /* WOLFSSL_TLS13_DRAFT_18 */ 02544 02545 #ifndef NO_WOLFSSL_CLIENT 02546 #if defined(HAVE_SESSION_TICKET) || !defined(NO_PSK) 02547 /* Setup pre-shared key based on the details in the extension data. 02548 * 02549 * ssl SSL/TLS object. 02550 * psk Pre-shared key extension data. 02551 * returns 0 on success, PSK_KEY_ERROR when the client PSK callback fails and 02552 * other negative value on failure. 02553 */ 02554 static int SetupPskKey(WOLFSSL* ssl, PreSharedKey* psk) 02555 { 02556 int ret; 02557 byte suite[2]; 02558 02559 if (psk == NULL) 02560 return BAD_FUNC_ARG; 02561 02562 suite[0] = psk->cipherSuite0; 02563 suite[1] = psk->cipherSuite; 02564 if (!FindSuiteSSL(ssl, suite)) 02565 return PSK_KEY_ERROR; 02566 02567 ssl->options.cipherSuite0 = psk->cipherSuite0; 02568 ssl->options.cipherSuite = psk->cipherSuite; 02569 if ((ret = SetCipherSpecs(ssl)) != 0) 02570 return ret; 02571 02572 #ifdef HAVE_SESSION_TICKET 02573 if (psk->resumption) { 02574 #ifdef WOLFSSL_EARLY_DATA 02575 if (ssl->session.maxEarlyDataSz == 0) 02576 ssl->earlyData = no_early_data; 02577 #endif 02578 /* Resumption PSK is master secret. */ 02579 ssl->arrays->psk_keySz = ssl->specs.hash_size; 02580 #ifdef WOLFSSL_TLS13_DRAFT_18 02581 XMEMCPY(ssl->arrays->psk_key, ssl->session.masterSecret, 02582 ssl->arrays->psk_keySz); 02583 #else 02584 if ((ret = DeriveResumptionPSK(ssl, ssl->session.ticketNonce.data, 02585 ssl->session.ticketNonce.len, ssl->arrays->psk_key)) != 0) { 02586 return ret; 02587 } 02588 #endif 02589 } 02590 #endif 02591 #ifndef NO_PSK 02592 if (!psk->resumption) { 02593 #ifndef WOLFSSL_PSK_ONE_ID 02594 const char* cipherName = NULL; 02595 byte cipherSuite0 = TLS13_BYTE, cipherSuite = WOLFSSL_DEF_PSK_CIPHER; 02596 02597 /* Get the pre-shared key. */ 02598 if (ssl->options.client_psk_tls13_cb != NULL) { 02599 ssl->arrays->psk_keySz = ssl->options.client_psk_tls13_cb(ssl, 02600 (char *)psk->identity, ssl->arrays->client_identity, 02601 MAX_PSK_ID_LEN, ssl->arrays->psk_key, MAX_PSK_KEY_LEN, 02602 &cipherName); 02603 if (GetCipherSuiteFromName(cipherName, &cipherSuite0, 02604 &cipherSuite) != 0) { 02605 return PSK_KEY_ERROR; 02606 } 02607 } 02608 else { 02609 ssl->arrays->psk_keySz = ssl->options.client_psk_cb(ssl, 02610 (char *)psk->identity, ssl->arrays->client_identity, 02611 MAX_PSK_ID_LEN, ssl->arrays->psk_key, MAX_PSK_KEY_LEN); 02612 } 02613 if (ssl->arrays->psk_keySz == 0 || 02614 ssl->arrays->psk_keySz > MAX_PSK_KEY_LEN) { 02615 return PSK_KEY_ERROR; 02616 } 02617 02618 if (psk->cipherSuite0 != cipherSuite0 || 02619 psk->cipherSuite != cipherSuite) { 02620 return PSK_KEY_ERROR; 02621 } 02622 #else 02623 /* PSK information loaded during setting of default TLS extensions. */ 02624 #endif 02625 } 02626 #endif 02627 02628 if (ssl->options.noPskDheKe) 02629 ssl->arrays->preMasterSz = 0; 02630 02631 /* Derive the early secret using the PSK. */ 02632 return DeriveEarlySecret(ssl); 02633 } 02634 02635 /* Derive and write the binders into the ClientHello in space left when 02636 * writing the Pre-Shared Key extension. 02637 * 02638 * ssl The SSL/TLS object. 02639 * output The buffer containing the ClientHello. 02640 * idx The index at the end of the completed ClientHello. 02641 * returns 0 on success and otherwise failure. 02642 */ 02643 static int WritePSKBinders(WOLFSSL* ssl, byte* output, word32 idx) 02644 { 02645 int ret; 02646 TLSX* ext; 02647 PreSharedKey* current; 02648 byte binderKey[WC_MAX_DIGEST_SIZE]; 02649 word16 len; 02650 02651 WOLFSSL_ENTER("WritePSKBinders"); 02652 02653 ext = TLSX_Find(ssl->extensions, TLSX_PRE_SHARED_KEY); 02654 if (ext == NULL) 02655 return SANITY_MSG_E; 02656 02657 /* Get the size of the binders to determine where to write binders. */ 02658 ret = TLSX_PreSharedKey_GetSizeBinders((PreSharedKey*)ext->data, 02659 client_hello, &len); 02660 if (ret < 0) 02661 return ret; 02662 idx -= len; 02663 02664 /* Hash truncated ClientHello - up to binders. */ 02665 ret = HashOutput(ssl, output, idx, 0); 02666 if (ret != 0) 02667 return ret; 02668 02669 current = (PreSharedKey*)ext->data; 02670 /* Calculate the binder for each identity based on previous handshake data. 02671 */ 02672 while (current != NULL) { 02673 if ((ret = SetupPskKey(ssl, current)) != 0) 02674 return ret; 02675 02676 #ifdef HAVE_SESSION_TICKET 02677 if (current->resumption) 02678 ret = DeriveBinderKeyResume(ssl, binderKey); 02679 #endif 02680 #ifndef NO_PSK 02681 if (!current->resumption) 02682 ret = DeriveBinderKey(ssl, binderKey); 02683 #endif 02684 if (ret != 0) 02685 return ret; 02686 02687 /* Derive the Finished message secret. */ 02688 ret = DeriveFinishedSecret(ssl, binderKey, 02689 ssl->keys.client_write_MAC_secret); 02690 if (ret != 0) 02691 return ret; 02692 02693 /* Build the HMAC of the handshake message data = binder. */ 02694 ret = BuildTls13HandshakeHmac(ssl, ssl->keys.client_write_MAC_secret, 02695 current->binder, ¤t->binderLen); 02696 if (ret != 0) 02697 return ret; 02698 02699 current = current->next; 02700 } 02701 02702 /* Data entered into extension, now write to message. */ 02703 ret = TLSX_PreSharedKey_WriteBinders((PreSharedKey*)ext->data, output + idx, 02704 client_hello, &len); 02705 if (ret < 0) 02706 return ret; 02707 02708 /* Hash binders to complete the hash of the ClientHello. */ 02709 ret = HashOutputRaw(ssl, output + idx, len); 02710 if (ret < 0) 02711 return ret; 02712 02713 #ifdef WOLFSSL_EARLY_DATA 02714 if (ssl->earlyData != no_early_data) { 02715 if ((ret = SetupPskKey(ssl, (PreSharedKey*)ext->data)) != 0) 02716 return ret; 02717 02718 /* Derive early data encryption key. */ 02719 ret = DeriveTls13Keys(ssl, early_data_key, ENCRYPT_SIDE_ONLY, 1); 02720 if (ret != 0) 02721 return ret; 02722 if ((ret = SetKeysSide(ssl, ENCRYPT_SIDE_ONLY)) != 0) 02723 return ret; 02724 } 02725 #endif 02726 02727 WOLFSSL_LEAVE("WritePSKBinders", ret); 02728 02729 return ret; 02730 } 02731 #endif 02732 02733 /* handle generation of TLS 1.3 client_hello (1) */ 02734 /* Send a ClientHello message to the server. 02735 * Include the information required to start a handshake with servers using 02736 * protocol versions less than TLS v1.3. 02737 * Only a client will send this message. 02738 * 02739 * ssl The SSL/TLS object. 02740 * returns 0 on success and otherwise failure. 02741 */ 02742 int SendTls13ClientHello(WOLFSSL* ssl) 02743 { 02744 byte* output; 02745 word16 length; 02746 word32 idx = RECORD_HEADER_SZ + HANDSHAKE_HEADER_SZ; 02747 int sendSz; 02748 int ret; 02749 02750 WOLFSSL_START(WC_FUNC_CLIENT_HELLO_SEND); 02751 WOLFSSL_ENTER("SendTls13ClientHello"); 02752 02753 #ifdef HAVE_SESSION_TICKET 02754 if (ssl->options.resuming && 02755 (ssl->session.version.major != ssl->version.major || 02756 ssl->session.version.minor != ssl->version.minor)) { 02757 #ifndef WOLFSSL_NO_TLS12 02758 if (ssl->session.version.major == ssl->version.major && 02759 ssl->session.version.minor < ssl->version.minor) { 02760 /* Cannot resume with a different protocol version. */ 02761 ssl->options.resuming = 0; 02762 ssl->version.major = ssl->session.version.major; 02763 ssl->version.minor = ssl->session.version.minor; 02764 return SendClientHello(ssl); 02765 } 02766 else 02767 #endif 02768 return VERSION_ERROR; 02769 } 02770 #endif 02771 02772 if (ssl->suites == NULL) { 02773 WOLFSSL_MSG("Bad suites pointer in SendTls13ClientHello"); 02774 return SUITES_ERROR; 02775 } 02776 02777 /* Version | Random | Session Id | Cipher Suites | Compression */ 02778 length = VERSION_SZ + RAN_LEN + ENUM_LEN + ssl->suites->suiteSz + 02779 SUITE_LEN + COMP_LEN + ENUM_LEN; 02780 #ifndef WOLFSSL_TLS13_DRAFT_18 02781 #if defined(WOLFSSL_TLS13_MIDDLEBOX_COMPAT) 02782 length += ID_LEN; 02783 #else 02784 if (ssl->session.sessionIDSz > 0) 02785 length += ssl->session.sessionIDSz; 02786 #endif 02787 #endif 02788 02789 /* Auto populate extensions supported unless user defined. */ 02790 if ((ret = TLSX_PopulateExtensions(ssl, 0)) != 0) 02791 return ret; 02792 #ifdef WOLFSSL_EARLY_DATA 02793 #ifndef NO_PSK 02794 if (!ssl->options.resuming && 02795 ssl->options.client_psk_tls13_cb == NULL && 02796 ssl->options.client_psk_cb == NULL) 02797 #else 02798 if (!ssl->options.resuming) 02799 #endif 02800 ssl->earlyData = no_early_data; 02801 if (ssl->options.serverState == SERVER_HELLO_RETRY_REQUEST_COMPLETE) 02802 ssl->earlyData = no_early_data; 02803 if (ssl->earlyData == no_early_data) 02804 TLSX_Remove(&ssl->extensions, TLSX_EARLY_DATA, ssl->heap); 02805 if (ssl->earlyData != no_early_data && 02806 (ret = TLSX_EarlyData_Use(ssl, 0)) < 0) { 02807 return ret; 02808 } 02809 #endif 02810 /* Include length of TLS extensions. */ 02811 ret = TLSX_GetRequestSize(ssl, client_hello, &length); 02812 if (ret != 0) 02813 return ret; 02814 02815 /* Total message size. */ 02816 sendSz = length + HANDSHAKE_HEADER_SZ + RECORD_HEADER_SZ; 02817 02818 /* Check buffers are big enough and grow if needed. */ 02819 if ((ret = CheckAvailableSize(ssl, sendSz)) != 0) 02820 return ret; 02821 02822 /* Get position in output buffer to write new message to. */ 02823 output = ssl->buffers.outputBuffer.buffer + 02824 ssl->buffers.outputBuffer.length; 02825 02826 /* Put the record and handshake headers on. */ 02827 AddTls13Headers(output, length, client_hello, ssl); 02828 02829 /* Protocol version - negotiation now in extension: supported_versions. */ 02830 output[idx++] = SSLv3_MAJOR; 02831 output[idx++] = TLSv1_2_MINOR; 02832 /* Keep for downgrade. */ 02833 ssl->chVersion = ssl->version; 02834 02835 /* Client Random */ 02836 if (ssl->options.connectState == CONNECT_BEGIN) { 02837 ret = wc_RNG_GenerateBlock(ssl->rng, output + idx, RAN_LEN); 02838 if (ret != 0) 02839 return ret; 02840 02841 /* Store random for possible second ClientHello. */ 02842 XMEMCPY(ssl->arrays->clientRandom, output + idx, RAN_LEN); 02843 } 02844 else 02845 XMEMCPY(output + idx, ssl->arrays->clientRandom, RAN_LEN); 02846 idx += RAN_LEN; 02847 02848 #ifdef WOLFSSL_TLS13_DRAFT_18 02849 /* TLS v1.3 does not use session id - 0 length. */ 02850 output[idx++] = 0; 02851 #else 02852 if (ssl->session.sessionIDSz > 0) { 02853 /* Session resumption for old versions of protocol. */ 02854 output[idx++] = ID_LEN; 02855 XMEMCPY(output + idx, ssl->session.sessionID, ssl->session.sessionIDSz); 02856 idx += ID_LEN; 02857 } 02858 else { 02859 #ifdef WOLFSSL_TLS13_MIDDLEBOX_COMPAT 02860 output[idx++] = ID_LEN; 02861 XMEMCPY(output + idx, ssl->arrays->clientRandom, ID_LEN); 02862 idx += ID_LEN; 02863 #else 02864 /* TLS v1.3 does not use session id - 0 length. */ 02865 output[idx++] = 0; 02866 #endif /* WOLFSSL_TLS13_MIDDLEBOX_COMPAT */ 02867 } 02868 #endif /* WOLFSSL_TLS13_DRAFT_18 */ 02869 02870 /* Cipher suites */ 02871 c16toa(ssl->suites->suiteSz, output + idx); 02872 idx += OPAQUE16_LEN; 02873 XMEMCPY(output + idx, &ssl->suites->suites, ssl->suites->suiteSz); 02874 idx += ssl->suites->suiteSz; 02875 02876 /* Compression not supported in TLS v1.3. */ 02877 output[idx++] = COMP_LEN; 02878 output[idx++] = NO_COMPRESSION; 02879 02880 /* Write out extensions for a request. */ 02881 length = 0; 02882 ret = TLSX_WriteRequest(ssl, output + idx, client_hello, &length); 02883 if (ret != 0) 02884 return ret; 02885 idx += length; 02886 02887 #if defined(HAVE_SESSION_TICKET) || !defined(NO_PSK) 02888 /* Resumption has a specific set of extensions and binder is calculated 02889 * for each identity. 02890 */ 02891 if (TLSX_Find(ssl->extensions, TLSX_PRE_SHARED_KEY)) 02892 ret = WritePSKBinders(ssl, output, idx); 02893 else 02894 #endif 02895 ret = HashOutput(ssl, output, idx, 0); 02896 if (ret != 0) 02897 return ret; 02898 02899 ssl->options.clientState = CLIENT_HELLO_COMPLETE; 02900 02901 #ifdef WOLFSSL_CALLBACKS 02902 if (ssl->hsInfoOn) AddPacketName(ssl, "ClientHello"); 02903 if (ssl->toInfoOn) { 02904 AddPacketInfo(ssl, "ClientHello", handshake, output, sendSz, 02905 WRITE_PROTO, ssl->heap); 02906 } 02907 #endif 02908 02909 ssl->buffers.outputBuffer.length += sendSz; 02910 02911 #ifdef WOLFSSL_EARLY_DATA_GROUP 02912 if (ssl->earlyData == no_early_data) 02913 #endif 02914 ret = SendBuffered(ssl); 02915 02916 02917 WOLFSSL_LEAVE("SendTls13ClientHello", ret); 02918 WOLFSSL_END(WC_FUNC_CLIENT_HELLO_SEND); 02919 02920 return ret; 02921 } 02922 02923 #ifdef WOLFSSL_TLS13_DRAFT_18 02924 /* handle rocessing of TLS 1.3 hello_retry_request (6) */ 02925 /* Parse and handle a HelloRetryRequest message. 02926 * Only a client will receive this message. 02927 * 02928 * ssl The SSL/TLS object. 02929 * input The message buffer. 02930 * inOutIdx On entry, the index into the message buffer of 02931 * HelloRetryRequest. 02932 * On exit, the index of byte after the HelloRetryRequest message. 02933 * totalSz The length of the current handshake message. 02934 * returns 0 on success and otherwise failure. 02935 */ 02936 static int DoTls13HelloRetryRequest(WOLFSSL* ssl, const byte* input, 02937 word32* inOutIdx, word32 totalSz) 02938 { 02939 int ret; 02940 word32 begin = *inOutIdx; 02941 word32 i = begin; 02942 word16 totalExtSz; 02943 ProtocolVersion pv; 02944 02945 WOLFSSL_ENTER("DoTls13HelloRetryRequest"); 02946 02947 #ifdef WOLFSSL_CALLBACKS 02948 if (ssl->hsInfoOn) AddPacketName(ssl, "HelloRetryRequest"); 02949 if (ssl->toInfoOn) AddLateName("HelloRetryRequest", &ssl->timeoutInfo); 02950 #endif 02951 02952 /* Version info and length field of extension data. */ 02953 if (totalSz < i - begin + OPAQUE16_LEN + OPAQUE16_LEN + OPAQUE16_LEN) 02954 return BUFFER_ERROR; 02955 02956 /* Protocol version. */ 02957 XMEMCPY(&pv, input + i, OPAQUE16_LEN); 02958 i += OPAQUE16_LEN; 02959 ret = CheckVersion(ssl, pv); 02960 if (ret != 0) 02961 return ret; 02962 02963 /* Length of extension data. */ 02964 ato16(&input[i], &totalExtSz); 02965 i += OPAQUE16_LEN; 02966 if (totalExtSz == 0) { 02967 WOLFSSL_MSG("HelloRetryRequest must contain extensions"); 02968 return MISSING_HANDSHAKE_DATA; 02969 } 02970 02971 /* Extension data. */ 02972 if (i - begin + totalExtSz > totalSz) 02973 return BUFFER_ERROR; 02974 if ((ret = TLSX_Parse(ssl, (byte *)(input + i), totalExtSz, 02975 hello_retry_request, NULL)) != 0) 02976 return ret; 02977 /* The KeyShare extension parsing fails when not valid. */ 02978 02979 /* Move index to byte after message. */ 02980 *inOutIdx = i + totalExtSz; 02981 02982 ssl->options.tls1_3 = 1; 02983 ssl->options.serverState = SERVER_HELLO_RETRY_REQUEST_COMPLETE; 02984 02985 WOLFSSL_LEAVE("DoTls13HelloRetryRequest", ret); 02986 02987 return ret; 02988 } 02989 #endif 02990 02991 02992 /* handle processing of TLS 1.3 server_hello (2) and hello_retry_request (6) */ 02993 /* Handle the ServerHello message from the server. 02994 * Only a client will receive this message. 02995 * 02996 * ssl The SSL/TLS object. 02997 * input The message buffer. 02998 * inOutIdx On entry, the index into the message buffer of ServerHello. 02999 * On exit, the index of byte after the ServerHello message. 03000 * helloSz The length of the current handshake message. 03001 * returns 0 on success and otherwise failure. 03002 */ 03003 int DoTls13ServerHello(WOLFSSL* ssl, const byte* input, word32* inOutIdx, 03004 word32 helloSz, byte* extMsgType) 03005 { 03006 ProtocolVersion pv; 03007 word32 i = *inOutIdx; 03008 word32 begin = i; 03009 int ret; 03010 #ifndef WOLFSSL_TLS13_DRAFT_18 03011 byte sessIdSz; 03012 const byte* sessId; 03013 byte b; 03014 int foundVersion; 03015 #endif 03016 word16 totalExtSz; 03017 #if defined(HAVE_SESSION_TICKET) || !defined(NO_PSK) 03018 TLSX* ext; 03019 PreSharedKey* psk = NULL; 03020 #endif 03021 03022 WOLFSSL_START(WC_FUNC_SERVER_HELLO_DO); 03023 WOLFSSL_ENTER("DoTls13ServerHello"); 03024 03025 #ifdef WOLFSSL_CALLBACKS 03026 if (ssl->hsInfoOn) AddPacketName(ssl, "ServerHello"); 03027 if (ssl->toInfoOn) AddLateName("ServerHello", &ssl->timeoutInfo); 03028 #endif 03029 03030 /* Protocol version length check. */ 03031 if (OPAQUE16_LEN > helloSz) 03032 return BUFFER_ERROR; 03033 03034 /* Protocol version */ 03035 XMEMCPY(&pv, input + i, OPAQUE16_LEN); 03036 i += OPAQUE16_LEN; 03037 #ifdef WOLFSSL_TLS13_DRAFT_18 03038 ret = CheckVersion(ssl, pv); 03039 if (ret != 0) 03040 return ret; 03041 if (!IsAtLeastTLSv1_3(pv) && pv.major != TLS_DRAFT_MAJOR) { 03042 #ifndef WOLFSSL_NO_TLS12 03043 if (ssl->options.downgrade) { 03044 ssl->version = pv; 03045 return DoServerHello(ssl, input, inOutIdx, helloSz); 03046 } 03047 #endif 03048 03049 WOLFSSL_MSG("Client using higher version, fatal error"); 03050 return VERSION_ERROR; 03051 } 03052 #else 03053 #ifndef WOLFSSL_NO_TLS12 03054 if (pv.major == ssl->version.major && pv.minor < TLSv1_2_MINOR && 03055 ssl->options.downgrade) { 03056 /* Force client hello version 1.2 to work for static RSA. */ 03057 ssl->chVersion.minor = TLSv1_2_MINOR; 03058 ssl->version.minor = TLSv1_2_MINOR; 03059 return DoServerHello(ssl, input, inOutIdx, helloSz); 03060 } 03061 #endif 03062 if (pv.major != ssl->version.major || pv.minor != TLSv1_2_MINOR) 03063 return VERSION_ERROR; 03064 #endif 03065 03066 #ifdef WOLFSSL_TLS13_DRAFT_18 03067 /* Random length check */ 03068 if ((i - begin) + RAN_LEN > helloSz) 03069 return BUFFER_ERROR; 03070 #else 03071 /* Random and session id length check */ 03072 if ((i - begin) + RAN_LEN + ENUM_LEN > helloSz) 03073 return BUFFER_ERROR; 03074 03075 if (XMEMCMP(input + i, helloRetryRequestRandom, RAN_LEN) == 0) 03076 *extMsgType = hello_retry_request; 03077 #endif 03078 03079 /* Server random - keep for debugging. */ 03080 XMEMCPY(ssl->arrays->serverRandom, input + i, RAN_LEN); 03081 i += RAN_LEN; 03082 03083 #ifndef WOLFSSL_TLS13_DRAFT_18 03084 /* Session id */ 03085 sessIdSz = input[i++]; 03086 if ((i - begin) + sessIdSz > helloSz) 03087 return BUFFER_ERROR; 03088 sessId = input + i; 03089 i += sessIdSz; 03090 #endif /* WOLFSSL_TLS13_DRAFT_18 */ 03091 ssl->options.haveSessionId = 1; 03092 03093 #ifdef WOLFSSL_TLS13_DRAFT_18 03094 /* Ciphersuite check */ 03095 if ((i - begin) + OPAQUE16_LEN + OPAQUE16_LEN > helloSz) 03096 return BUFFER_ERROR; 03097 #else 03098 /* Ciphersuite and compression check */ 03099 if ((i - begin) + OPAQUE16_LEN + OPAQUE8_LEN > helloSz) 03100 return BUFFER_ERROR; 03101 #endif 03102 03103 /* Set the cipher suite from the message. */ 03104 ssl->options.cipherSuite0 = input[i++]; 03105 ssl->options.cipherSuite = input[i++]; 03106 03107 #ifndef WOLFSSL_TLS13_DRAFT_18 03108 /* Compression */ 03109 b = input[i++]; 03110 if (b != 0) { 03111 WOLFSSL_MSG("Must be no compression types in list"); 03112 return INVALID_PARAMETER; 03113 } 03114 #endif 03115 03116 #ifndef WOLFSSL_TLS13_DRAFT_18 03117 if ((i - begin) + OPAQUE16_LEN > helloSz) { 03118 if (!ssl->options.downgrade) 03119 return BUFFER_ERROR; 03120 #ifndef WOLFSSL_NO_TLS12 03121 ssl->version.minor = TLSv1_2_MINOR; 03122 #endif 03123 ssl->options.haveEMS = 0; 03124 } 03125 if ((i - begin) < helloSz) 03126 #endif 03127 { 03128 /* Get extension length and length check. */ 03129 if ((i - begin) + OPAQUE16_LEN > helloSz) 03130 return BUFFER_ERROR; 03131 ato16(&input[i], &totalExtSz); 03132 i += OPAQUE16_LEN; 03133 if ((i - begin) + totalExtSz > helloSz) 03134 return BUFFER_ERROR; 03135 03136 #ifndef WOLFSSL_TLS13_DRAFT_18 03137 /* Need to negotiate version first. */ 03138 if ((ret = TLSX_ParseVersion(ssl, (byte*)input + i, totalExtSz, 03139 *extMsgType, &foundVersion))) { 03140 return ret; 03141 } 03142 if (!foundVersion) { 03143 if (!ssl->options.downgrade) { 03144 WOLFSSL_MSG("Server trying to downgrade to version less than " 03145 "TLS v1.3"); 03146 return VERSION_ERROR; 03147 } 03148 03149 if (pv.minor < ssl->options.minDowngrade) 03150 return VERSION_ERROR; 03151 ssl->version.minor = pv.minor; 03152 } 03153 #endif 03154 03155 /* Parse and handle extensions. */ 03156 ret = TLSX_Parse(ssl, (byte *) input + i, totalExtSz, *extMsgType, 03157 NULL); 03158 if (ret != 0) 03159 return ret; 03160 03161 i += totalExtSz; 03162 } 03163 *inOutIdx = i; 03164 03165 ssl->options.serverState = SERVER_HELLO_COMPLETE; 03166 03167 #ifdef HAVE_SECRET_CALLBACK 03168 if (ssl->sessionSecretCb != NULL) { 03169 int secretSz = SECRET_LEN; 03170 ret = ssl->sessionSecretCb(ssl, ssl->session.masterSecret, 03171 &secretSz, ssl->sessionSecretCtx); 03172 if (ret != 0 || secretSz != SECRET_LEN) { 03173 return SESSION_SECRET_CB_E; 03174 } 03175 } 03176 #endif /* HAVE_SECRET_CALLBACK */ 03177 03178 #ifndef WOLFSSL_TLS13_DRAFT_18 03179 /* Version only negotiated in extensions for TLS v1.3. 03180 * Only now do we know how to deal with session id. 03181 */ 03182 if (!IsAtLeastTLSv1_3(ssl->version)) { 03183 #ifndef WOLFSSL_NO_TLS12 03184 ssl->arrays->sessionIDSz = sessIdSz; 03185 03186 if (ssl->arrays->sessionIDSz > ID_LEN) { 03187 WOLFSSL_MSG("Invalid session ID size"); 03188 ssl->arrays->sessionIDSz = 0; 03189 return BUFFER_ERROR; 03190 } 03191 else if (ssl->arrays->sessionIDSz) { 03192 XMEMCPY(ssl->arrays->sessionID, sessId, ssl->arrays->sessionIDSz); 03193 ssl->options.haveSessionId = 1; 03194 } 03195 03196 /* Force client hello version 1.2 to work for static RSA. */ 03197 ssl->chVersion.minor = TLSv1_2_MINOR; 03198 /* Complete TLS v1.2 processing of ServerHello. */ 03199 ret = CompleteServerHello(ssl); 03200 #else 03201 WOLFSSL_MSG("Client using higher version, fatal error"); 03202 ret = VERSION_ERROR; 03203 #endif 03204 03205 WOLFSSL_LEAVE("DoTls13ServerHello", ret); 03206 03207 return ret; 03208 } 03209 03210 #ifdef WOLFSSL_TLS13_MIDDLEBOX_COMPAT 03211 if (sessIdSz == 0) 03212 return INVALID_PARAMETER; 03213 if (ssl->session.sessionIDSz != 0) { 03214 if (ssl->session.sessionIDSz != sessIdSz || 03215 XMEMCMP(ssl->session.sessionID, sessId, sessIdSz) != 0) { 03216 return INVALID_PARAMETER; 03217 } 03218 } 03219 else if (XMEMCMP(ssl->arrays->clientRandom, sessId, sessIdSz) != 0) 03220 return INVALID_PARAMETER; 03221 #else 03222 if (sessIdSz != ssl->session.sessionIDSz || (sessIdSz > 0 && 03223 XMEMCMP(ssl->session.sessionID, sessId, sessIdSz) != 0)) { 03224 WOLFSSL_MSG("Server sent different session id"); 03225 return INVALID_PARAMETER; 03226 } 03227 #endif /* WOLFSSL_TLS13_MIDDLEBOX_COMPAT */ 03228 #endif 03229 03230 ret = SetCipherSpecs(ssl); 03231 if (ret != 0) 03232 return ret; 03233 #ifdef HAVE_NULL_CIPHER 03234 if (ssl->options.cipherSuite0 == ECC_BYTE && 03235 (ssl->options.cipherSuite == TLS_SHA256_SHA256 || 03236 ssl->options.cipherSuite == TLS_SHA384_SHA384)) { 03237 ; 03238 } 03239 else 03240 #endif 03241 /* Check that the negotiated ciphersuite matches protocol version. */ 03242 if (ssl->options.cipherSuite0 != TLS13_BYTE) { 03243 WOLFSSL_MSG("Server sent non-TLS13 cipher suite in TLS 1.3 packet"); 03244 return INVALID_PARAMETER; 03245 } 03246 03247 #if defined(HAVE_SESSION_TICKET) || !defined(NO_PSK) 03248 #ifndef WOLFSSL_TLS13_DRAFT_18 03249 if (*extMsgType == server_hello) 03250 #endif 03251 { 03252 ext = TLSX_Find(ssl->extensions, TLSX_PRE_SHARED_KEY); 03253 if (ext != NULL) 03254 psk = (PreSharedKey*)ext->data; 03255 while (psk != NULL && !psk->chosen) 03256 psk = psk->next; 03257 if (psk == NULL) { 03258 ssl->options.resuming = 0; 03259 ssl->arrays->psk_keySz = 0; 03260 XMEMSET(ssl->arrays->psk_key, 0, MAX_PSK_KEY_LEN); 03261 } 03262 else if ((ret = SetupPskKey(ssl, psk)) != 0) 03263 return ret; 03264 } 03265 #endif 03266 03267 #ifdef WOLFSSL_TLS13_DRAFT_18 03268 ssl->keys.encryptionOn = 1; 03269 #else 03270 if (*extMsgType == server_hello) { 03271 ssl->keys.encryptionOn = 1; 03272 ssl->options.serverState = SERVER_HELLO_COMPLETE; 03273 } 03274 else { 03275 ssl->options.tls1_3 = 1; 03276 ssl->options.serverState = SERVER_HELLO_RETRY_REQUEST_COMPLETE; 03277 03278 ret = RestartHandshakeHash(ssl); 03279 } 03280 #endif 03281 03282 WOLFSSL_LEAVE("DoTls13ServerHello", ret); 03283 WOLFSSL_END(WC_FUNC_SERVER_HELLO_DO); 03284 03285 return ret; 03286 } 03287 03288 /* handle processing TLS 1.3 encrypted_extensions (8) */ 03289 /* Parse and handle an EncryptedExtensions message. 03290 * Only a client will receive this message. 03291 * 03292 * ssl The SSL/TLS object. 03293 * input The message buffer. 03294 * inOutIdx On entry, the index into the message buffer of 03295 * EncryptedExtensions. 03296 * On exit, the index of byte after the EncryptedExtensions 03297 * message. 03298 * totalSz The length of the current handshake message. 03299 * returns 0 on success and otherwise failure. 03300 */ 03301 static int DoTls13EncryptedExtensions(WOLFSSL* ssl, const byte* input, 03302 word32* inOutIdx, word32 totalSz) 03303 { 03304 int ret; 03305 word32 begin = *inOutIdx; 03306 word32 i = begin; 03307 word16 totalExtSz; 03308 03309 WOLFSSL_START(WC_FUNC_ENCRYPTED_EXTENSIONS_DO); 03310 WOLFSSL_ENTER("DoTls13EncryptedExtensions"); 03311 03312 #ifdef WOLFSSL_CALLBACKS 03313 if (ssl->hsInfoOn) AddPacketName(ssl, "EncryptedExtensions"); 03314 if (ssl->toInfoOn) AddLateName("EncryptedExtensions", &ssl->timeoutInfo); 03315 #endif 03316 03317 /* Length field of extension data. */ 03318 if (totalSz < i - begin + OPAQUE16_LEN) 03319 return BUFFER_ERROR; 03320 ato16(&input[i], &totalExtSz); 03321 i += OPAQUE16_LEN; 03322 03323 /* Extension data. */ 03324 if (i - begin + totalExtSz > totalSz) 03325 return BUFFER_ERROR; 03326 if ((ret = TLSX_Parse(ssl, (byte *)(input + i), totalExtSz, 03327 encrypted_extensions, NULL))) 03328 return ret; 03329 03330 /* Move index to byte after message. */ 03331 *inOutIdx = i + totalExtSz; 03332 03333 /* Always encrypted. */ 03334 *inOutIdx += ssl->keys.padSz; 03335 03336 #ifdef WOLFSSL_EARLY_DATA 03337 if (ssl->earlyData != no_early_data) { 03338 TLSX* ext = TLSX_Find(ssl->extensions, TLSX_EARLY_DATA); 03339 if (ext == NULL || !ext->val) 03340 ssl->earlyData = no_early_data; 03341 } 03342 #endif 03343 03344 #ifdef WOLFSSL_EARLY_DATA 03345 if (ssl->earlyData == no_early_data) { 03346 ret = SetKeysSide(ssl, ENCRYPT_SIDE_ONLY); 03347 if (ret != 0) 03348 return ret; 03349 } 03350 #endif 03351 03352 ssl->options.serverState = SERVER_ENCRYPTED_EXTENSIONS_COMPLETE; 03353 03354 WOLFSSL_LEAVE("DoTls13EncryptedExtensions", ret); 03355 WOLFSSL_END(WC_FUNC_ENCRYPTED_EXTENSIONS_DO); 03356 03357 return ret; 03358 } 03359 03360 /* handle processing TLS v1.3 certificate_request (13) */ 03361 /* Handle a TLS v1.3 CertificateRequest message. 03362 * This message is always encrypted. 03363 * Only a client will receive this message. 03364 * 03365 * ssl The SSL/TLS object. 03366 * input The message buffer. 03367 * inOutIdx On entry, the index into the message buffer of CertificateRequest. 03368 * On exit, the index of byte after the CertificateRequest message. 03369 * size The length of the current handshake message. 03370 * returns 0 on success and otherwise failure. 03371 */ 03372 static int DoTls13CertificateRequest(WOLFSSL* ssl, const byte* input, 03373 word32* inOutIdx, word32 size) 03374 { 03375 word16 len; 03376 word32 begin = *inOutIdx; 03377 int ret = 0; 03378 #ifndef WOLFSSL_TLS13_DRAFT_18 03379 Suites peerSuites; 03380 #endif 03381 #ifdef WOLFSSL_POST_HANDSHAKE_AUTH 03382 CertReqCtx* certReqCtx; 03383 #endif 03384 03385 WOLFSSL_START(WC_FUNC_CERTIFICATE_REQUEST_DO); 03386 WOLFSSL_ENTER("DoTls13CertificateRequest"); 03387 03388 #ifndef WOLFSSL_TLS13_DRAFT_18 03389 XMEMSET(&peerSuites, 0, sizeof(Suites)); 03390 #endif 03391 #ifdef WOLFSSL_CALLBACKS 03392 if (ssl->hsInfoOn) AddPacketName(ssl, "CertificateRequest"); 03393 if (ssl->toInfoOn) AddLateName("CertificateRequest", &ssl->timeoutInfo); 03394 #endif 03395 03396 if ((*inOutIdx - begin) + OPAQUE8_LEN > size) 03397 return BUFFER_ERROR; 03398 03399 /* Length of the request context. */ 03400 len = input[(*inOutIdx)++]; 03401 if ((*inOutIdx - begin) + len > size) 03402 return BUFFER_ERROR; 03403 if (ssl->options.connectState < FINISHED_DONE && len > 0) 03404 return BUFFER_ERROR; 03405 03406 #ifdef WOLFSSL_POST_HANDSHAKE_AUTH 03407 /* CertReqCtx has one byte at end for context value. 03408 * Increase size to handle other implementations sending more than one byte. 03409 * That is, allocate extra space, over one byte, to hold the context value. 03410 */ 03411 certReqCtx = (CertReqCtx*)XMALLOC(sizeof(CertReqCtx) + len - 1, ssl->heap, 03412 DYNAMIC_TYPE_TMP_BUFFER); 03413 if (certReqCtx == NULL) 03414 return MEMORY_E; 03415 certReqCtx->next = ssl->certReqCtx; 03416 certReqCtx->len = len; 03417 XMEMCPY(&certReqCtx->ctx, input + *inOutIdx, len); 03418 ssl->certReqCtx = certReqCtx; 03419 #endif 03420 *inOutIdx += len; 03421 03422 #ifdef WOLFSSL_TLS13_DRAFT_18 03423 /* Signature and hash algorithms. */ 03424 if ((*inOutIdx - begin) + OPAQUE16_LEN > size) 03425 return BUFFER_ERROR; 03426 ato16(input + *inOutIdx, &len); 03427 *inOutIdx += OPAQUE16_LEN; 03428 if ((*inOutIdx - begin) + len > size) 03429 return BUFFER_ERROR; 03430 if (PickHashSigAlgo(ssl, input + *inOutIdx, len) != 0 && 03431 ssl->buffers.certificate && ssl->buffers.certificate->buffer && 03432 ssl->buffers.key && ssl->buffers.key->buffer) { 03433 return INVALID_PARAMETER; 03434 } 03435 *inOutIdx += len; 03436 03437 /* Length of certificate authority data. */ 03438 if ((*inOutIdx - begin) + OPAQUE16_LEN > size) 03439 return BUFFER_ERROR; 03440 ato16(input + *inOutIdx, &len); 03441 *inOutIdx += OPAQUE16_LEN; 03442 if ((*inOutIdx - begin) + len > size) 03443 return BUFFER_ERROR; 03444 03445 /* Certificate authorities. */ 03446 while (len) { 03447 word16 dnSz; 03448 03449 if ((*inOutIdx - begin) + OPAQUE16_LEN > size) 03450 return BUFFER_ERROR; 03451 03452 ato16(input + *inOutIdx, &dnSz); 03453 *inOutIdx += OPAQUE16_LEN; 03454 03455 if ((*inOutIdx - begin) + dnSz > size) 03456 return BUFFER_ERROR; 03457 03458 *inOutIdx += dnSz; 03459 len -= OPAQUE16_LEN + dnSz; 03460 } 03461 03462 /* Certificate extensions */ 03463 if ((*inOutIdx - begin) + OPAQUE16_LEN > size) 03464 return BUFFER_ERROR; 03465 ato16(input + *inOutIdx, &len); 03466 *inOutIdx += OPAQUE16_LEN; 03467 if ((*inOutIdx - begin) + len > size) 03468 return BUFFER_ERROR; 03469 *inOutIdx += len; 03470 #else 03471 /* TODO: Add support for more extensions: 03472 * signed_certificate_timestamp, certificate_authorities, oid_filters. 03473 */ 03474 /* Certificate extensions */ 03475 if ((*inOutIdx - begin) + OPAQUE16_LEN > size) 03476 return BUFFER_ERROR; 03477 ato16(input + *inOutIdx, &len); 03478 *inOutIdx += OPAQUE16_LEN; 03479 if ((*inOutIdx - begin) + len > size) 03480 return BUFFER_ERROR; 03481 if (len == 0) 03482 return INVALID_PARAMETER; 03483 if ((ret = TLSX_Parse(ssl, (byte *)(input + *inOutIdx), len, 03484 certificate_request, &peerSuites))) { 03485 return ret; 03486 } 03487 *inOutIdx += len; 03488 #endif 03489 03490 if (ssl->buffers.certificate && ssl->buffers.certificate->buffer && 03491 ((ssl->buffers.key && ssl->buffers.key->buffer) 03492 #ifdef HAVE_PK_CALLBACKS 03493 || wolfSSL_CTX_IsPrivatePkSet(ssl->ctx) 03494 #endif 03495 )) { 03496 #ifndef WOLFSSL_TLS13_DRAFT_18 03497 if (PickHashSigAlgo(ssl, peerSuites.hashSigAlgo, 03498 peerSuites.hashSigAlgoSz) != 0) { 03499 return INVALID_PARAMETER; 03500 } 03501 #endif 03502 ssl->options.sendVerify = SEND_CERT; 03503 } 03504 else { 03505 ssl->options.sendVerify = SEND_BLANK_CERT; 03506 } 03507 03508 /* This message is always encrypted so add encryption padding. */ 03509 *inOutIdx += ssl->keys.padSz; 03510 03511 WOLFSSL_LEAVE("DoTls13CertificateRequest", ret); 03512 WOLFSSL_END(WC_FUNC_CERTIFICATE_REQUEST_DO); 03513 03514 return ret; 03515 } 03516 03517 #endif /* !NO_WOLFSSL_CLIENT */ 03518 03519 #ifndef NO_WOLFSSL_SERVER 03520 #if defined(HAVE_SESSION_TICKET) || !defined(NO_PSK) 03521 /* Refine list of supported cipher suites to those common to server and client. 03522 * 03523 * ssl SSL/TLS object. 03524 * peerSuites The peer's advertised list of supported cipher suites. 03525 */ 03526 static void RefineSuites(WOLFSSL* ssl, Suites* peerSuites) 03527 { 03528 byte suites[WOLFSSL_MAX_SUITE_SZ]; 03529 int suiteSz = 0; 03530 word16 i, j; 03531 03532 XMEMSET(suites, 0, WOLFSSL_MAX_SUITE_SZ); 03533 03534 for (i = 0; i < ssl->suites->suiteSz; i += 2) { 03535 for (j = 0; j < peerSuites->suiteSz; j += 2) { 03536 if (ssl->suites->suites[i+0] == peerSuites->suites[j+0] && 03537 ssl->suites->suites[i+1] == peerSuites->suites[j+1]) { 03538 suites[suiteSz++] = peerSuites->suites[j+0]; 03539 suites[suiteSz++] = peerSuites->suites[j+1]; 03540 } 03541 } 03542 } 03543 03544 ssl->suites->suiteSz = suiteSz; 03545 XMEMCPY(ssl->suites->suites, &suites, sizeof(suites)); 03546 } 03547 03548 /* Handle any Pre-Shared Key (PSK) extension. 03549 * Must do this in ClientHello as it requires a hash of the truncated message. 03550 * Don't know size of binders until Pre-Shared Key extension has been parsed. 03551 * 03552 * ssl The SSL/TLS object. 03553 * input The ClientHello message. 03554 * helloSz The size of the ClientHello message (including binders if present). 03555 * usingPSK Indicates handshake is using Pre-Shared Keys. 03556 * returns 0 on success and otherwise failure. 03557 */ 03558 static int DoPreSharedKeys(WOLFSSL* ssl, const byte* input, word32 helloSz, 03559 int* usingPSK) 03560 { 03561 int ret; 03562 TLSX* ext; 03563 word16 bindersLen; 03564 PreSharedKey* current; 03565 byte binderKey[WC_MAX_DIGEST_SIZE]; 03566 byte binder[WC_MAX_DIGEST_SIZE]; 03567 word32 binderLen; 03568 word16 modes; 03569 byte suite[2]; 03570 #ifdef WOLFSSL_EARLY_DATA 03571 int pskCnt = 0; 03572 TLSX* extEarlyData; 03573 #endif 03574 #ifndef NO_PSK 03575 const char* cipherName = NULL; 03576 byte cipherSuite0 = TLS13_BYTE; 03577 byte cipherSuite = WOLFSSL_DEF_PSK_CIPHER; 03578 #endif 03579 03580 WOLFSSL_ENTER("DoPreSharedKeys"); 03581 03582 ext = TLSX_Find(ssl->extensions, TLSX_PRE_SHARED_KEY); 03583 if (ext == NULL) { 03584 /* Hash data up to binders for deriving binders in PSK extension. */ 03585 ret = HashInput(ssl, input, helloSz); 03586 return ret; 03587 } 03588 03589 /* Extensions pushed on stack/list and PSK must be last. */ 03590 if (ssl->extensions != ext) 03591 return PSK_KEY_ERROR; 03592 03593 /* Assume we are going to resume with a pre-shared key. */ 03594 ssl->options.resuming = 1; 03595 03596 /* Find the pre-shared key extension and calculate hash of truncated 03597 * ClientHello for binders. 03598 */ 03599 ret = TLSX_PreSharedKey_GetSizeBinders((PreSharedKey*)ext->data, 03600 client_hello, &bindersLen); 03601 if (ret < 0) 03602 return ret; 03603 03604 /* Hash data up to binders for deriving binders in PSK extension. */ 03605 ret = HashInput(ssl, input, helloSz - bindersLen); 03606 if (ret != 0) 03607 return ret; 03608 03609 /* Look through all client's pre-shared keys for a match. */ 03610 current = (PreSharedKey*)ext->data; 03611 while (current != NULL) { 03612 #ifdef WOLFSSL_EARLY_DATA 03613 pskCnt++; 03614 #endif 03615 03616 #ifndef NO_PSK 03617 if (current->identityLen > MAX_PSK_ID_LEN) { 03618 return BUFFER_ERROR; 03619 } 03620 XMEMCPY(ssl->arrays->client_identity, current->identity, 03621 current->identityLen); 03622 ssl->arrays->client_identity[current->identityLen] = '\0'; 03623 #endif 03624 03625 #ifdef HAVE_SESSION_TICKET 03626 /* Decode the identity. */ 03627 if ((ret = DoClientTicket(ssl, current->identity, current->identityLen)) 03628 == WOLFSSL_TICKET_RET_OK) { 03629 word32 now; 03630 int diff; 03631 03632 now = TimeNowInMilliseconds(); 03633 if (now == (word32)GETTIME_ERROR) 03634 return now; 03635 diff = now - ssl->session.ticketSeen; 03636 diff -= current->ticketAge - ssl->session.ticketAdd; 03637 /* Check session and ticket age timeout. 03638 * Allow +/- 1000 milliseconds on ticket age. 03639 */ 03640 if (diff > (int)ssl->timeout * 1000 || diff < -1000 || 03641 diff - MAX_TICKET_AGE_SECS * 1000 > 1000) { 03642 /* Invalid difference, fallback to full handshake. */ 03643 ssl->options.resuming = 0; 03644 break; 03645 } 03646 03647 /* Check whether resumption is possible based on suites in SSL and 03648 * ciphersuite in ticket. 03649 */ 03650 suite[0] = ssl->session.cipherSuite0; 03651 suite[1] = ssl->session.cipherSuite; 03652 if (!FindSuiteSSL(ssl, suite)) { 03653 current = current->next; 03654 continue; 03655 } 03656 03657 #ifdef WOLFSSL_EARLY_DATA 03658 ssl->options.maxEarlyDataSz = ssl->session.maxEarlyDataSz; 03659 #endif 03660 /* Use the same cipher suite as before and set up for use. */ 03661 ssl->options.cipherSuite0 = ssl->session.cipherSuite0; 03662 ssl->options.cipherSuite = ssl->session.cipherSuite; 03663 ret = SetCipherSpecs(ssl); 03664 if (ret != 0) 03665 return ret; 03666 03667 /* Resumption PSK is resumption master secret. */ 03668 ssl->arrays->psk_keySz = ssl->specs.hash_size; 03669 #ifdef WOLFSSL_TLS13_DRAFT_18 03670 XMEMCPY(ssl->arrays->psk_key, ssl->session.masterSecret, 03671 ssl->arrays->psk_keySz); 03672 #else 03673 if ((ret = DeriveResumptionPSK(ssl, ssl->session.ticketNonce.data, 03674 ssl->session.ticketNonce.len, ssl->arrays->psk_key)) != 0) { 03675 return ret; 03676 } 03677 #endif 03678 03679 /* Derive the early secret using the PSK. */ 03680 ret = DeriveEarlySecret(ssl); 03681 if (ret != 0) 03682 return ret; 03683 /* Derive the binder key to use to with HMAC. */ 03684 ret = DeriveBinderKeyResume(ssl, binderKey); 03685 if (ret != 0) 03686 return ret; 03687 } 03688 else 03689 #endif 03690 #ifndef NO_PSK 03691 if ((ssl->options.server_psk_tls13_cb != NULL && 03692 (ssl->arrays->psk_keySz = ssl->options.server_psk_tls13_cb(ssl, 03693 ssl->arrays->client_identity, ssl->arrays->psk_key, 03694 MAX_PSK_KEY_LEN, &cipherName)) != 0 && 03695 GetCipherSuiteFromName(cipherName, &cipherSuite0, 03696 &cipherSuite) == 0) || 03697 (ssl->options.server_psk_cb != NULL && 03698 (ssl->arrays->psk_keySz = ssl->options.server_psk_cb(ssl, 03699 ssl->arrays->client_identity, ssl->arrays->psk_key, 03700 MAX_PSK_KEY_LEN)) != 0)) { 03701 if (ssl->arrays->psk_keySz > MAX_PSK_KEY_LEN) 03702 return PSK_KEY_ERROR; 03703 03704 /* Check whether PSK ciphersuite is in SSL. */ 03705 suite[0] = cipherSuite0; 03706 suite[1] = cipherSuite; 03707 if (!FindSuiteSSL(ssl, suite)) { 03708 current = current->next; 03709 continue; 03710 } 03711 03712 /* Default to ciphersuite if cb doesn't specify. */ 03713 ssl->options.resuming = 0; 03714 03715 /* PSK age is always zero. */ 03716 if (current->ticketAge != ssl->session.ticketAdd) 03717 return PSK_KEY_ERROR; 03718 03719 /* Set PSK ciphersuite into SSL. */ 03720 ssl->options.cipherSuite0 = cipherSuite0; 03721 ssl->options.cipherSuite = cipherSuite; 03722 ret = SetCipherSpecs(ssl); 03723 if (ret != 0) 03724 return ret; 03725 03726 /* Derive the early secret using the PSK. */ 03727 ret = DeriveEarlySecret(ssl); 03728 if (ret != 0) 03729 return ret; 03730 /* Derive the binder key to use to with HMAC. */ 03731 ret = DeriveBinderKey(ssl, binderKey); 03732 if (ret != 0) 03733 return ret; 03734 } 03735 else 03736 #endif 03737 { 03738 current = current->next; 03739 continue; 03740 } 03741 03742 ssl->options.sendVerify = 0; 03743 03744 /* Derive the Finished message secret. */ 03745 ret = DeriveFinishedSecret(ssl, binderKey, 03746 ssl->keys.client_write_MAC_secret); 03747 if (ret != 0) 03748 return ret; 03749 03750 /* Derive the binder and compare with the one in the extension. */ 03751 ret = BuildTls13HandshakeHmac(ssl, 03752 ssl->keys.client_write_MAC_secret, binder, &binderLen); 03753 if (ret != 0) 03754 return ret; 03755 if (binderLen != current->binderLen || 03756 XMEMCMP(binder, current->binder, binderLen) != 0) { 03757 return BAD_BINDER; 03758 } 03759 03760 /* This PSK works, no need to try any more. */ 03761 current->chosen = 1; 03762 ext->resp = 1; 03763 break; 03764 } 03765 03766 /* Hash the rest of the ClientHello. */ 03767 ret = HashInputRaw(ssl, input + helloSz - bindersLen, bindersLen); 03768 if (ret != 0) 03769 return ret; 03770 03771 if (current == NULL) { 03772 #ifdef WOLFSSL_PSK_ID_PROTECTION 03773 #ifndef NO_CERTS 03774 if (ssl->buffers.certChainCnt != 0) 03775 return 0; 03776 #endif 03777 return BAD_BINDER; 03778 #else 03779 return 0; 03780 #endif 03781 } 03782 03783 #ifdef WOLFSSL_EARLY_DATA 03784 extEarlyData = TLSX_Find(ssl->extensions, TLSX_EARLY_DATA); 03785 if (extEarlyData != NULL) { 03786 if (ssl->earlyData != no_early_data && current == ext->data) { 03787 extEarlyData->resp = 1; 03788 03789 /* Derive early data decryption key. */ 03790 ret = DeriveTls13Keys(ssl, early_data_key, DECRYPT_SIDE_ONLY, 1); 03791 if (ret != 0) 03792 return ret; 03793 if ((ret = SetKeysSide(ssl, DECRYPT_SIDE_ONLY)) != 0) 03794 return ret; 03795 03796 ssl->earlyData = process_early_data; 03797 } 03798 else 03799 extEarlyData->resp = 0; 03800 } 03801 #endif 03802 03803 /* Get the PSK key exchange modes the client wants to negotiate. */ 03804 ext = TLSX_Find(ssl->extensions, TLSX_PSK_KEY_EXCHANGE_MODES); 03805 if (ext == NULL) 03806 return MISSING_HANDSHAKE_DATA; 03807 modes = ext->val; 03808 03809 ext = TLSX_Find(ssl->extensions, TLSX_KEY_SHARE); 03810 /* Use (EC)DHE for forward-security if possible. */ 03811 if ((modes & (1 << PSK_DHE_KE)) != 0 && !ssl->options.noPskDheKe && 03812 ext != NULL) { 03813 /* Only use named group used in last session. */ 03814 ssl->namedGroup = ssl->session.namedGroup; 03815 03816 /* Pick key share and Generate a new key if not present. */ 03817 ret = TLSX_KeyShare_Establish(ssl); 03818 if (ret == KEY_SHARE_ERROR) { 03819 ssl->options.serverState = SERVER_HELLO_RETRY_REQUEST_COMPLETE; 03820 ret = 0; 03821 } 03822 else if (ret < 0) 03823 return ret; 03824 03825 /* Send new public key to client. */ 03826 ext->resp = 1; 03827 } 03828 else { 03829 if ((modes & (1 << PSK_KE)) == 0) 03830 return PSK_KEY_ERROR; 03831 ssl->options.noPskDheKe = 1; 03832 ssl->arrays->preMasterSz = 0; 03833 } 03834 03835 *usingPSK = 1; 03836 03837 WOLFSSL_LEAVE("DoPreSharedKeys", ret); 03838 03839 return ret; 03840 } 03841 #endif 03842 03843 #if !defined(WOLFSSL_TLS13_DRAFT_18) && defined(WOLFSSL_SEND_HRR_COOKIE) 03844 /* Check that the Cookie data's integrity. 03845 * 03846 * ssl SSL/TLS object. 03847 * cookie The cookie data - hash and MAC. 03848 * cookieSz The length of the cookie data in bytes. 03849 * returns Length of the hash on success, otherwise failure. 03850 */ 03851 static int CheckCookie(WOLFSSL* ssl, byte* cookie, byte cookieSz) 03852 { 03853 int ret; 03854 byte mac[WC_MAX_DIGEST_SIZE] = {0}; 03855 Hmac cookieHmac; 03856 byte cookieType = 0; 03857 byte macSz = 0; 03858 03859 #if !defined(NO_SHA) && defined(NO_SHA256) 03860 cookieType = SHA; 03861 macSz = WC_SHA_DIGEST_SIZE; 03862 #endif /* NO_SHA */ 03863 #ifndef NO_SHA256 03864 cookieType = WC_SHA256; 03865 macSz = WC_SHA256_DIGEST_SIZE; 03866 #endif /* NO_SHA256 */ 03867 03868 if (cookieSz < ssl->specs.hash_size + macSz) 03869 return HRR_COOKIE_ERROR; 03870 cookieSz -= macSz; 03871 XMEMSET(&cookieHmac, 0, sizeof(Hmac)); 03872 03873 ret = wc_HmacSetKey(&cookieHmac, cookieType, 03874 ssl->buffers.tls13CookieSecret.buffer, 03875 ssl->buffers.tls13CookieSecret.length); 03876 if (ret != 0) 03877 return ret; 03878 if ((ret = wc_HmacUpdate(&cookieHmac, cookie, cookieSz)) != 0) 03879 return ret; 03880 if ((ret = wc_HmacFinal(&cookieHmac, mac)) != 0) 03881 return ret; 03882 03883 if (ConstantCompare(cookie + cookieSz, mac, macSz) != 0) 03884 return HRR_COOKIE_ERROR; 03885 return cookieSz; 03886 } 03887 03888 /* Length of the KeyShare Extension */ 03889 #define HRR_KEY_SHARE_SZ (OPAQUE16_LEN + OPAQUE16_LEN + OPAQUE16_LEN) 03890 /* Length of the Supported Vresions Extension */ 03891 #define HRR_VERSIONS_SZ (OPAQUE16_LEN + OPAQUE16_LEN + OPAQUE16_LEN) 03892 /* Length of the Cookie Extension excluding cookie data */ 03893 #define HRR_COOKIE_HDR_SZ (OPAQUE16_LEN + OPAQUE16_LEN + OPAQUE16_LEN) 03894 #ifdef WOLFSSL_TLS13_DRAFT_18 03895 /* PV | CipherSuite | Ext Len */ 03896 #define HRR_BODY_SZ (OPAQUE16_LEN + OPAQUE16_LEN + OPAQUE16_LEN) 03897 /* HH | PV | CipherSuite | Ext Len | Key Share | Cookie */ 03898 #define MAX_HRR_SZ (HANDSHAKE_HEADER_SZ + \ 03899 HRR_BODY_SZ + \ 03900 HRR_KEY_SHARE_SZ + \ 03901 HRR_COOKIE_HDR_SZ) 03902 #else 03903 /* PV | Random | Session Id | CipherSuite | Compression | Ext Len */ 03904 #define HRR_BODY_SZ (VERSION_SZ + RAN_LEN + ENUM_LEN + ID_LEN + \ 03905 SUITE_LEN + COMP_LEN + OPAQUE16_LEN) 03906 /* HH | PV | CipherSuite | Ext Len | Key Share | Supported Version | Cookie */ 03907 #define MAX_HRR_SZ (HANDSHAKE_HEADER_SZ + \ 03908 HRR_BODY_SZ + \ 03909 HRR_KEY_SHARE_SZ + \ 03910 HRR_VERSIONS_SZ + \ 03911 HRR_COOKIE_HDR_SZ) 03912 #endif 03913 03914 /* Restart the handshake hash from the cookie value. 03915 * 03916 * ssl SSL/TLS object. 03917 * cookie Cookie data from client. 03918 * returns 0 on success, otherwise failure. 03919 */ 03920 static int RestartHandshakeHashWithCookie(WOLFSSL* ssl, Cookie* cookie) 03921 { 03922 byte header[HANDSHAKE_HEADER_SZ] = {0}; 03923 byte hrr[MAX_HRR_SZ] = {0}; 03924 int hrrIdx; 03925 word32 idx; 03926 byte hashSz; 03927 byte* cookieData; 03928 byte cookieDataSz; 03929 word16 length; 03930 int keyShareExt = 0; 03931 int ret; 03932 03933 cookieDataSz = ret = CheckCookie(ssl, &cookie->data, cookie->len); 03934 if (ret < 0) 03935 return ret; 03936 hashSz = cookie->data; 03937 cookieData = &cookie->data; 03938 idx = OPAQUE8_LEN; 03939 03940 /* Restart handshake hash with synthetic message hash. */ 03941 AddTls13HandShakeHeader(header, hashSz, 0, 0, message_hash, ssl); 03942 if ((ret = InitHandshakeHashes(ssl)) != 0) 03943 return ret; 03944 if ((ret = HashOutputRaw(ssl, header, sizeof(header))) != 0) 03945 return ret; 03946 if ((ret = HashOutputRaw(ssl, cookieData + idx, hashSz)) != 0) 03947 return ret; 03948 03949 /* Reconstruct the HelloRetryMessage for handshake hash. */ 03950 #ifdef WOLFSSL_TLS13_DRAFT_18 03951 length = HRR_BODY_SZ + HRR_COOKIE_HDR_SZ + cookie->len; 03952 #else 03953 length = HRR_BODY_SZ - ID_LEN + ssl->session.sessionIDSz + 03954 HRR_COOKIE_HDR_SZ + cookie->len; 03955 length += HRR_VERSIONS_SZ; 03956 #endif 03957 if (cookieDataSz > hashSz + OPAQUE16_LEN) { 03958 keyShareExt = 1; 03959 length += HRR_KEY_SHARE_SZ; 03960 } 03961 #ifdef WOLFSSL_TLS13_DRAFT_18 03962 AddTls13HandShakeHeader(hrr, length, 0, 0, hello_retry_request, ssl); 03963 03964 idx += hashSz; 03965 hrrIdx = HANDSHAKE_HEADER_SZ; 03966 /* The negotiated protocol version. */ 03967 hrr[hrrIdx++] = TLS_DRAFT_MAJOR; 03968 hrr[hrrIdx++] = TLS_DRAFT_MINOR; 03969 /* Cipher Suite */ 03970 hrr[hrrIdx++] = cookieData[idx++]; 03971 hrr[hrrIdx++] = cookieData[idx++]; 03972 03973 /* Extensions' length */ 03974 length -= HRR_BODY_SZ; 03975 c16toa(length, hrr + hrrIdx); 03976 hrrIdx += 2; 03977 #else 03978 AddTls13HandShakeHeader(hrr, length, 0, 0, server_hello, ssl); 03979 03980 idx += hashSz; 03981 hrrIdx = HANDSHAKE_HEADER_SZ; 03982 03983 /* The negotiated protocol version. */ 03984 hrr[hrrIdx++] = ssl->version.major; 03985 hrr[hrrIdx++] = TLSv1_2_MINOR; 03986 03987 /* HelloRetryRequest message has fixed value for random. */ 03988 XMEMCPY(hrr + hrrIdx, helloRetryRequestRandom, RAN_LEN); 03989 hrrIdx += RAN_LEN; 03990 03991 hrr[hrrIdx++] = ssl->session.sessionIDSz; 03992 if (ssl->session.sessionIDSz > 0) { 03993 XMEMCPY(hrr + hrrIdx, ssl->session.sessionID, ssl->session.sessionIDSz); 03994 hrrIdx += ssl->session.sessionIDSz; 03995 } 03996 03997 /* Cipher Suite */ 03998 hrr[hrrIdx++] = cookieData[idx++]; 03999 hrr[hrrIdx++] = cookieData[idx++]; 04000 04001 /* Compression not supported in TLS v1.3. */ 04002 hrr[hrrIdx++] = 0; 04003 04004 /* Extensions' length */ 04005 length -= HRR_BODY_SZ - ID_LEN + ssl->session.sessionIDSz; 04006 c16toa(length, hrr + hrrIdx); 04007 hrrIdx += 2; 04008 04009 #endif 04010 /* Optional KeyShare Extension */ 04011 if (keyShareExt) { 04012 c16toa(TLSX_KEY_SHARE, hrr + hrrIdx); 04013 hrrIdx += 2; 04014 c16toa(OPAQUE16_LEN, hrr + hrrIdx); 04015 hrrIdx += 2; 04016 hrr[hrrIdx++] = cookieData[idx++]; 04017 hrr[hrrIdx++] = cookieData[idx++]; 04018 } 04019 #ifndef WOLFSSL_TLS13_DRAFT_18 04020 c16toa(TLSX_SUPPORTED_VERSIONS, hrr + hrrIdx); 04021 hrrIdx += 2; 04022 c16toa(OPAQUE16_LEN, hrr + hrrIdx); 04023 hrrIdx += 2; 04024 #ifdef WOLFSSL_TLS13_DRAFT 04025 hrr[hrrIdx++] = TLS_DRAFT_MAJOR; 04026 hrr[hrrIdx++] = TLS_DRAFT_MINOR; 04027 #else 04028 hrr[hrrIdx++] = ssl->version.major; 04029 hrr[hrrIdx++] = ssl->version.minor; 04030 #endif 04031 #endif 04032 /* Mandatory Cookie Extension */ 04033 c16toa(TLSX_COOKIE, hrr + hrrIdx); 04034 hrrIdx += 2; 04035 c16toa(cookie->len + OPAQUE16_LEN, hrr + hrrIdx); 04036 hrrIdx += 2; 04037 c16toa(cookie->len, hrr + hrrIdx); 04038 hrrIdx += 2; 04039 04040 #ifdef WOLFSSL_DEBUG_TLS 04041 WOLFSSL_MSG("Reconstucted HelloRetryRequest"); 04042 WOLFSSL_BUFFER(hrr, hrrIdx); 04043 WOLFSSL_MSG("Cookie"); 04044 WOLFSSL_BUFFER(cookieData, cookie->len); 04045 #endif 04046 04047 if ((ret = HashOutputRaw(ssl, hrr, hrrIdx)) != 0) 04048 return ret; 04049 return HashOutputRaw(ssl, cookieData, cookie->len); 04050 } 04051 #endif 04052 04053 /* Do SupportedVersion extension for TLS v1.3+ otherwise it is not. 04054 * 04055 * ssl The SSL/TLS object. 04056 * input The message buffer. 04057 * i The index into the message buffer of ClientHello. 04058 * helloSz The length of the current handshake message. 04059 * returns 0 on success and otherwise failure. 04060 */ 04061 static int DoTls13SupportedVersions(WOLFSSL* ssl, const byte* input, word32 i, 04062 word32 helloSz, int* wantDowngrade) 04063 { 04064 int ret; 04065 byte b; 04066 word16 suiteSz; 04067 word16 totalExtSz; 04068 int foundVersion = 0; 04069 04070 /* Client random */ 04071 i += RAN_LEN; 04072 /* Session id - not used in TLS v1.3 */ 04073 b = input[i++]; 04074 if (i + b > helloSz) { 04075 return BUFFER_ERROR; 04076 } 04077 i += b; 04078 /* Cipher suites */ 04079 if (i + OPAQUE16_LEN > helloSz) 04080 return BUFFER_ERROR; 04081 ato16(input + i, &suiteSz); 04082 i += OPAQUE16_LEN; 04083 if (i + suiteSz + 1 > helloSz) 04084 return BUFFER_ERROR; 04085 i += suiteSz; 04086 /* Compression */ 04087 b = input[i++]; 04088 if (i + b > helloSz) 04089 return BUFFER_ERROR; 04090 i += b; 04091 04092 /* TLS 1.3 must have extensions */ 04093 if (i < helloSz) { 04094 if (i + OPAQUE16_LEN > helloSz) 04095 return BUFFER_ERROR; 04096 ato16(&input[i], &totalExtSz); 04097 i += OPAQUE16_LEN; 04098 if (totalExtSz != helloSz - i) 04099 return BUFFER_ERROR; 04100 04101 /* Need to negotiate version first. */ 04102 if ((ret = TLSX_ParseVersion(ssl, (byte*)input + i, totalExtSz, 04103 client_hello, &foundVersion))) { 04104 return ret; 04105 } 04106 } 04107 *wantDowngrade = !foundVersion || !IsAtLeastTLSv1_3(ssl->version); 04108 04109 return 0; 04110 } 04111 04112 /* Handle a ClientHello handshake message. 04113 * If the protocol version in the message is not TLS v1.3 or higher, use 04114 * DoClientHello() 04115 * Only a server will receive this message. 04116 * 04117 * ssl The SSL/TLS object. 04118 * input The message buffer. 04119 * inOutIdx On entry, the index into the message buffer of ClientHello. 04120 * On exit, the index of byte after the ClientHello message and 04121 * padding. 04122 * helloSz The length of the current handshake message. 04123 * returns 0 on success and otherwise failure. 04124 */ 04125 int DoTls13ClientHello(WOLFSSL* ssl, const byte* input, word32* inOutIdx, 04126 word32 helloSz) 04127 { 04128 int ret = VERSION_ERROR; 04129 byte b = 0; 04130 ProtocolVersion pv; 04131 Suites clSuites; 04132 word32 i = *inOutIdx; 04133 word32 begin = i; 04134 word16 totalExtSz = 0; 04135 int usingPSK = 0; 04136 byte sessIdSz = 0; 04137 int wantDowngrade = 0; 04138 04139 WOLFSSL_START(WC_FUNC_CLIENT_HELLO_DO); 04140 WOLFSSL_ENTER("DoTls13ClientHello"); 04141 04142 XMEMSET(&pv, 0, sizeof(ProtocolVersion)); 04143 XMEMSET(&clSuites, 0, sizeof(Suites)); 04144 04145 #ifdef WOLFSSL_CALLBACKS 04146 if (ssl->hsInfoOn) AddPacketName(ssl, "ClientHello"); 04147 if (ssl->toInfoOn) AddLateName("ClientHello", &ssl->timeoutInfo); 04148 #endif 04149 04150 /* protocol version, random and session id length check */ 04151 if ((i - begin) + OPAQUE16_LEN + RAN_LEN + OPAQUE8_LEN > helloSz) 04152 return BUFFER_ERROR; 04153 04154 /* Protocol version */ 04155 XMEMCPY(&pv, input + i, OPAQUE16_LEN); 04156 ssl->chVersion = pv; /* store */ 04157 i += OPAQUE16_LEN; 04158 if (pv.major < SSLv3_MAJOR) { 04159 WOLFSSL_MSG("Legacy version field contains unsupported value"); 04160 #ifdef WOLFSSL_MYSQL_COMPATIBLE 04161 SendAlert(ssl, alert_fatal, wc_protocol_version); 04162 #else 04163 SendAlert(ssl, alert_fatal, protocol_version); 04164 #endif 04165 return INVALID_PARAMETER; 04166 } 04167 /* Legacy protocol version cannot negotiate TLS 1.3 or higher. */ 04168 if (pv.major > SSLv3_MAJOR || (pv.major == SSLv3_MAJOR && 04169 pv.minor >= TLSv1_3_MINOR)) { 04170 pv.major = SSLv3_MAJOR; 04171 pv.minor = TLSv1_2_MINOR; 04172 wantDowngrade = 1; 04173 ssl->version.minor = pv.minor; 04174 } 04175 /* Legacy version must be [ SSLv3_MAJOR, TLSv1_2_MINOR ] for TLS v1.3 */ 04176 else if (pv.major == SSLv3_MAJOR && pv.minor < TLSv1_2_MINOR) { 04177 wantDowngrade = 1; 04178 ssl->version.minor = pv.minor; 04179 } 04180 else { 04181 ret = DoTls13SupportedVersions(ssl, input + begin, i - begin, helloSz, 04182 &wantDowngrade); 04183 if (ret < 0) 04184 return ret; 04185 } 04186 if (wantDowngrade) { 04187 #ifndef WOLFSSL_NO_TLS12 04188 if (!ssl->options.downgrade) { 04189 WOLFSSL_MSG("Client trying to connect with lesser version than " 04190 "TLS v1.3"); 04191 return VERSION_ERROR; 04192 } 04193 04194 if (pv.minor < ssl->options.minDowngrade) 04195 return VERSION_ERROR; 04196 04197 if ((ret = HashInput(ssl, input + begin, helloSz)) != 0) 04198 return ret; 04199 return DoClientHello(ssl, input, inOutIdx, helloSz); 04200 #else 04201 WOLFSSL_MSG("Client trying to connect with lesser version than " 04202 "TLS v1.3"); 04203 return VERSION_ERROR; 04204 #endif 04205 } 04206 04207 /* Client random */ 04208 XMEMCPY(ssl->arrays->clientRandom, input + i, RAN_LEN); 04209 i += RAN_LEN; 04210 04211 #ifdef WOLFSSL_DEBUG_TLS 04212 WOLFSSL_MSG("client random"); 04213 WOLFSSL_BUFFER(ssl->arrays->clientRandom, RAN_LEN); 04214 #endif 04215 04216 #ifdef WOLFSSL_TLS13_DRAFT_18 04217 /* Session id - empty in TLS v1.3 */ 04218 sessIdSz = input[i++]; 04219 if (sessIdSz > 0 && !ssl->options.downgrade) { 04220 WOLFSSL_MSG("Client sent session id - not supported"); 04221 return BUFFER_ERROR; 04222 } 04223 #else 04224 sessIdSz = input[i++]; 04225 if (sessIdSz != ID_LEN && sessIdSz != 0) 04226 return INVALID_PARAMETER; 04227 #endif 04228 04229 if (sessIdSz + i > helloSz) { 04230 return BUFFER_ERROR; 04231 } 04232 04233 ssl->session.sessionIDSz = sessIdSz; 04234 if (sessIdSz == ID_LEN) { 04235 XMEMCPY(ssl->session.sessionID, input + i, sessIdSz); 04236 i += ID_LEN; 04237 } 04238 04239 /* Cipher suites */ 04240 if ((i - begin) + OPAQUE16_LEN > helloSz) 04241 return BUFFER_ERROR; 04242 ato16(&input[i], &clSuites.suiteSz); 04243 i += OPAQUE16_LEN; 04244 /* suites and compression length check */ 04245 if ((i - begin) + clSuites.suiteSz + OPAQUE8_LEN > helloSz) 04246 return BUFFER_ERROR; 04247 if (clSuites.suiteSz > WOLFSSL_MAX_SUITE_SZ) 04248 return BUFFER_ERROR; 04249 XMEMCPY(clSuites.suites, input + i, clSuites.suiteSz); 04250 i += clSuites.suiteSz; 04251 clSuites.hashSigAlgoSz = 0; 04252 04253 #ifdef HAVE_SERVER_RENEGOTIATION_INFO 04254 ret = FindSuite(&clSuites, 0, TLS_EMPTY_RENEGOTIATION_INFO_SCSV); 04255 if (ret == SUITES_ERROR) 04256 return BUFFER_ERROR; 04257 if (ret >= 0) { 04258 TLSX* extension; 04259 04260 /* check for TLS_EMPTY_RENEGOTIATION_INFO_SCSV suite */ 04261 ret = TLSX_AddEmptyRenegotiationInfo(&ssl->extensions, ssl->heap); 04262 if (ret != WOLFSSL_SUCCESS) 04263 return ret; 04264 04265 extension = TLSX_Find(ssl->extensions, TLSX_RENEGOTIATION_INFO); 04266 if (extension) { 04267 ssl->secure_renegotiation = (SecureRenegotiation*)extension->data; 04268 ssl->secure_renegotiation->enabled = 1; 04269 } 04270 } 04271 #endif /* HAVE_SERVER_RENEGOTIATION_INFO */ 04272 04273 /* Compression */ 04274 b = input[i++]; 04275 if ((i - begin) + b > helloSz) 04276 return BUFFER_ERROR; 04277 if (b != COMP_LEN) { 04278 WOLFSSL_MSG("Must be one compression type in list"); 04279 return INVALID_PARAMETER; 04280 } 04281 b = input[i++]; 04282 if (b != NO_COMPRESSION) { 04283 WOLFSSL_MSG("Must be no compression type in list"); 04284 return INVALID_PARAMETER; 04285 } 04286 04287 /* Extensions */ 04288 if ((i - begin) == helloSz) 04289 return BUFFER_ERROR; 04290 if ((i - begin) + OPAQUE16_LEN > helloSz) 04291 return BUFFER_ERROR; 04292 04293 ato16(&input[i], &totalExtSz); 04294 i += OPAQUE16_LEN; 04295 if ((i - begin) + totalExtSz > helloSz) 04296 return BUFFER_ERROR; 04297 04298 /* Auto populate extensions supported unless user defined. */ 04299 if ((ret = TLSX_PopulateExtensions(ssl, 1)) != 0) 04300 return ret; 04301 04302 /* Parse extensions */ 04303 if ((ret = TLSX_Parse(ssl, (byte*)input + i, totalExtSz, client_hello, 04304 &clSuites))) { 04305 return ret; 04306 } 04307 04308 #if defined(OPENSSL_ALL) || defined(HAVE_STUNNEL) || defined(WOLFSSL_NGINX) || \ 04309 defined(WOLFSSL_HAPROXY) 04310 if ((ret = SNI_Callback(ssl)) != 0) 04311 return ret; 04312 ssl->options.side = WOLFSSL_SERVER_END; 04313 #endif /* OPENSSL_ALL || HAVE_STUNNEL || WOLFSSL_NGINX || WOLFSSL_HAPROXY */ 04314 04315 i += totalExtSz; 04316 *inOutIdx = i; 04317 04318 ssl->options.sendVerify = SEND_CERT; 04319 04320 ssl->options.clientState = CLIENT_HELLO_COMPLETE; 04321 ssl->options.haveSessionId = 1; 04322 04323 #if !defined(WOLFSSL_TLS13_DRAFT_18) && defined(WOLFSSL_SEND_HRR_COOKIE) 04324 if (ssl->options.sendCookie && 04325 ssl->options.serverState == SERVER_HELLO_RETRY_REQUEST_COMPLETE) { 04326 TLSX* ext; 04327 04328 if ((ext = TLSX_Find(ssl->extensions, TLSX_COOKIE)) == NULL) 04329 return HRR_COOKIE_ERROR; 04330 /* Ensure the cookie came from client and isn't the one in the 04331 * response - HelloRetryRequest. 04332 */ 04333 if (ext->resp == 1) 04334 return HRR_COOKIE_ERROR; 04335 ret = RestartHandshakeHashWithCookie(ssl, (Cookie*)ext->data); 04336 if (ret != 0) 04337 return ret; 04338 } 04339 #endif 04340 04341 #if (defined(HAVE_SESSION_TICKET) || !defined(NO_PSK)) && \ 04342 defined(HAVE_TLS_EXTENSIONS) 04343 if (TLSX_Find(ssl->extensions, TLSX_PRE_SHARED_KEY) != NULL) { 04344 /* Refine list for PSK processing. */ 04345 RefineSuites(ssl, &clSuites); 04346 04347 /* Process the Pre-Shared Key extension if present. */ 04348 ret = DoPreSharedKeys(ssl, input + begin, helloSz, &usingPSK); 04349 if (ret != 0) 04350 return ret; 04351 } 04352 else 04353 #endif 04354 { 04355 #ifdef WOLFSSL_EARLY_DATA 04356 ssl->earlyData = no_early_data; 04357 #endif 04358 if ((ret = HashInput(ssl, input + begin, helloSz)) != 0) 04359 return ret; 04360 04361 } 04362 04363 if (!usingPSK) { 04364 if (TLSX_Find(ssl->extensions, TLSX_KEY_SHARE) == NULL) { 04365 WOLFSSL_MSG("Client did not send a KeyShare extension"); 04366 SendAlert(ssl, alert_fatal, missing_extension); 04367 return INCOMPLETE_DATA; 04368 } 04369 if (TLSX_Find(ssl->extensions, TLSX_SIGNATURE_ALGORITHMS) == NULL) { 04370 WOLFSSL_MSG("Client did not send a SignatureAlgorithms extension"); 04371 SendAlert(ssl, alert_fatal, missing_extension); 04372 return INCOMPLETE_DATA; 04373 } 04374 04375 if ((ret = MatchSuite(ssl, &clSuites)) < 0) { 04376 WOLFSSL_MSG("Unsupported cipher suite, ClientHello"); 04377 SendAlert(ssl, alert_fatal, handshake_failure); 04378 return ret; 04379 } 04380 04381 #ifdef HAVE_NULL_CIPHER 04382 if (ssl->options.cipherSuite0 == ECC_BYTE && 04383 (ssl->options.cipherSuite == TLS_SHA256_SHA256 || 04384 ssl->options.cipherSuite == TLS_SHA384_SHA384)) { 04385 ; 04386 } 04387 else 04388 #endif 04389 /* Check that the negotiated ciphersuite matches protocol version. */ 04390 if (ssl->options.cipherSuite0 != TLS13_BYTE) { 04391 WOLFSSL_MSG("Negotiated ciphersuite from lesser version than " 04392 "TLS v1.3"); 04393 SendAlert(ssl, alert_fatal, handshake_failure); 04394 return VERSION_ERROR; 04395 } 04396 04397 #ifdef HAVE_SESSION_TICKET 04398 if (ssl->options.resuming) { 04399 ssl->options.resuming = 0; 04400 XMEMSET(ssl->arrays->psk_key, 0, ssl->specs.hash_size); 04401 } 04402 #endif 04403 04404 /* Derive early secret for handshake secret. */ 04405 if ((ret = DeriveEarlySecret(ssl)) != 0) 04406 return ret; 04407 } 04408 04409 WOLFSSL_LEAVE("DoTls13ClientHello", ret); 04410 WOLFSSL_END(WC_FUNC_CLIENT_HELLO_DO); 04411 04412 return ret; 04413 } 04414 04415 #ifdef WOLFSSL_TLS13_DRAFT_18 04416 /* handle generation of TLS 1.3 hello_retry_request (6) */ 04417 /* Send the HelloRetryRequest message to indicate the negotiated protocol 04418 * version and security parameters the server is willing to use. 04419 * Only a server will send this message. 04420 * 04421 * ssl The SSL/TLS object. 04422 * returns 0 on success, otherwise failure. 04423 */ 04424 int SendTls13HelloRetryRequest(WOLFSSL* ssl) 04425 { 04426 int ret; 04427 byte* output; 04428 word32 length; 04429 word16 len; 04430 word32 idx = RECORD_HEADER_SZ + HANDSHAKE_HEADER_SZ; 04431 int sendSz; 04432 04433 WOLFSSL_ENTER("SendTls13HelloRetryRequest"); 04434 04435 /* Get the length of the extensions that will be written. */ 04436 len = 0; 04437 ret = TLSX_GetResponseSize(ssl, hello_retry_request, &len); 04438 /* There must be extensions sent to indicate what client needs to do. */ 04439 if (ret != 0) 04440 return MISSING_HANDSHAKE_DATA; 04441 04442 /* Protocol version + Extensions */ 04443 length = OPAQUE16_LEN + len; 04444 sendSz = idx + length; 04445 04446 /* Check buffers are big enough and grow if needed. */ 04447 if ((ret = CheckAvailableSize(ssl, sendSz)) != 0) 04448 return ret; 04449 04450 /* Get position in output buffer to write new message to. */ 04451 output = ssl->buffers.outputBuffer.buffer + 04452 ssl->buffers.outputBuffer.length; 04453 /* Add record and handshake headers. */ 04454 AddTls13Headers(output, length, hello_retry_request, ssl); 04455 04456 /* The negotiated protocol version. */ 04457 output[idx++] = TLS_DRAFT_MAJOR; 04458 output[idx++] = TLS_DRAFT_MINOR; 04459 04460 /* Add TLS extensions. */ 04461 ret = TLSX_WriteResponse(ssl, output + idx, hello_retry_request, NULL); 04462 if (ret != 0) 04463 return ret; 04464 idx += len; 04465 04466 #ifdef WOLFSSL_CALLBACKS 04467 if (ssl->hsInfoOn) 04468 AddPacketName(ssl, "HelloRetryRequest"); 04469 if (ssl->toInfoOn) { 04470 AddPacketInfo(ssl, "HelloRetryRequest", handshake, output, sendSz, 04471 WRITE_PROTO, ssl->heap); 04472 } 04473 #endif 04474 if ((ret = HashOutput(ssl, output, idx, 0)) != 0) 04475 return ret; 04476 04477 ssl->buffers.outputBuffer.length += sendSz; 04478 04479 if (!ssl->options.groupMessages) 04480 ret = SendBuffered(ssl); 04481 04482 WOLFSSL_LEAVE("SendTls13HelloRetryRequest", ret); 04483 04484 return ret; 04485 } 04486 #endif /* WOLFSSL_TLS13_DRAFT_18 */ 04487 04488 /* Send TLS v1.3 ServerHello message to client. 04489 * Only a server will send this message. 04490 * 04491 * ssl The SSL/TLS object. 04492 * returns 0 on success, otherwise failure. 04493 */ 04494 #ifdef WOLFSSL_TLS13_DRAFT_18 04495 static 04496 #endif 04497 /* handle generation of TLS 1.3 server_hello (2) */ 04498 int SendTls13ServerHello(WOLFSSL* ssl, byte extMsgType) 04499 { 04500 int ret; 04501 byte* output; 04502 word16 length; 04503 word32 idx = RECORD_HEADER_SZ + HANDSHAKE_HEADER_SZ; 04504 int sendSz; 04505 04506 WOLFSSL_START(WC_FUNC_SERVER_HELLO_SEND); 04507 WOLFSSL_ENTER("SendTls13ServerHello"); 04508 04509 #ifndef WOLFSSL_TLS13_DRAFT_18 04510 if (extMsgType == hello_retry_request) { 04511 WOLFSSL_MSG("wolfSSL Doing HelloRetryRequest"); 04512 if ((ret = RestartHandshakeHash(ssl)) < 0) 04513 return ret; 04514 } 04515 #endif 04516 04517 #ifdef WOLFSSL_TLS13_DRAFT_18 04518 /* Protocol version, server random, cipher suite and extensions. */ 04519 length = VERSION_SZ + RAN_LEN + SUITE_LEN; 04520 ret = TLSX_GetResponseSize(ssl, server_hello, &length); 04521 if (ret != 0) 04522 return ret; 04523 #else 04524 /* Protocol version, server random, session id, cipher suite, compression 04525 * and extensions. 04526 */ 04527 length = VERSION_SZ + RAN_LEN + ENUM_LEN + ssl->session.sessionIDSz + 04528 SUITE_LEN + COMP_LEN; 04529 ret = TLSX_GetResponseSize(ssl, extMsgType, &length); 04530 if (ret != 0) 04531 return ret; 04532 #endif 04533 sendSz = idx + length; 04534 04535 /* Check buffers are big enough and grow if needed. */ 04536 if ((ret = CheckAvailableSize(ssl, sendSz)) != 0) 04537 return ret; 04538 04539 /* Get position in output buffer to write new message to. */ 04540 output = ssl->buffers.outputBuffer.buffer + 04541 ssl->buffers.outputBuffer.length; 04542 04543 /* Put the record and handshake headers on. */ 04544 AddTls13Headers(output, length, server_hello, ssl); 04545 04546 #ifdef WOLFSSL_TLS13_DRAFT_18 04547 /* The negotiated protocol version. */ 04548 output[idx++] = TLS_DRAFT_MAJOR; 04549 output[idx++] = TLS_DRAFT_MINOR; 04550 #else 04551 /* The protocol version must be TLS v1.2 for middleboxes. */ 04552 output[idx++] = ssl->version.major; 04553 output[idx++] = TLSv1_2_MINOR; 04554 #endif 04555 04556 if (extMsgType == server_hello) { 04557 /* Generate server random. */ 04558 if ((ret = wc_RNG_GenerateBlock(ssl->rng, output + idx, RAN_LEN)) != 0) 04559 return ret; 04560 } 04561 #ifndef WOLFSSL_TLS13_DRAFT_18 04562 else { 04563 /* HelloRetryRequest message has fixed value for random. */ 04564 XMEMCPY(output + idx, helloRetryRequestRandom, RAN_LEN); 04565 } 04566 #endif 04567 /* Store in SSL for debugging. */ 04568 XMEMCPY(ssl->arrays->serverRandom, output + idx, RAN_LEN); 04569 idx += RAN_LEN; 04570 04571 #ifdef WOLFSSL_DEBUG_TLS 04572 WOLFSSL_MSG("Server random"); 04573 WOLFSSL_BUFFER(ssl->arrays->serverRandom, RAN_LEN); 04574 #endif 04575 04576 #ifndef WOLFSSL_TLS13_DRAFT_18 04577 output[idx++] = ssl->session.sessionIDSz; 04578 if (ssl->session.sessionIDSz > 0) { 04579 XMEMCPY(output + idx, ssl->session.sessionID, ssl->session.sessionIDSz); 04580 idx += ssl->session.sessionIDSz; 04581 } 04582 #endif 04583 04584 /* Chosen cipher suite */ 04585 output[idx++] = ssl->options.cipherSuite0; 04586 output[idx++] = ssl->options.cipherSuite; 04587 04588 #ifndef WOLFSSL_TLS13_DRAFT_18 04589 /* Compression not supported in TLS v1.3. */ 04590 output[idx++] = 0; 04591 #endif 04592 04593 /* Extensions */ 04594 ret = TLSX_WriteResponse(ssl, output + idx, extMsgType, NULL); 04595 if (ret != 0) 04596 return ret; 04597 04598 ssl->buffers.outputBuffer.length += sendSz; 04599 04600 if ((ret = HashOutput(ssl, output, sendSz, 0)) != 0) 04601 return ret; 04602 04603 #ifdef WOLFSSL_CALLBACKS 04604 if (ssl->hsInfoOn) 04605 AddPacketName(ssl, "ServerHello"); 04606 if (ssl->toInfoOn) { 04607 AddPacketInfo(ssl, "ServerHello", handshake, output, sendSz, 04608 WRITE_PROTO, ssl->heap); 04609 } 04610 #endif 04611 04612 #ifdef WOLFSSL_TLS13_DRAFT_18 04613 ssl->options.serverState = SERVER_HELLO_COMPLETE; 04614 #else 04615 if (extMsgType == server_hello) 04616 ssl->options.serverState = SERVER_HELLO_COMPLETE; 04617 #endif 04618 04619 #ifdef WOLFSSL_TLS13_DRAFT_18 04620 if (!ssl->options.groupMessages) 04621 #else 04622 if (!ssl->options.groupMessages || extMsgType != server_hello) 04623 #endif 04624 ret = SendBuffered(ssl); 04625 04626 WOLFSSL_LEAVE("SendTls13ServerHello", ret); 04627 WOLFSSL_END(WC_FUNC_SERVER_HELLO_SEND); 04628 04629 return ret; 04630 } 04631 04632 /* handle generation of TLS 1.3 encrypted_extensions (8) */ 04633 /* Send the rest of the extensions encrypted under the handshake key. 04634 * This message is always encrypted in TLS v1.3. 04635 * Only a server will send this message. 04636 * 04637 * ssl The SSL/TLS object. 04638 * returns 0 on success, otherwise failure. 04639 */ 04640 static int SendTls13EncryptedExtensions(WOLFSSL* ssl) 04641 { 04642 int ret; 04643 byte* output; 04644 word16 length = 0; 04645 word32 idx = RECORD_HEADER_SZ + HANDSHAKE_HEADER_SZ; 04646 int sendSz; 04647 04648 WOLFSSL_START(WC_FUNC_ENCRYPTED_EXTENSIONS_SEND); 04649 WOLFSSL_ENTER("SendTls13EncryptedExtensions"); 04650 04651 ssl->keys.encryptionOn = 1; 04652 04653 #ifndef WOLFSSL_NO_SERVER_GROUPS_EXT 04654 if ((ret = TLSX_SupportedCurve_CheckPriority(ssl)) != 0) 04655 return ret; 04656 #endif 04657 04658 /* Derive the handshake secret now that we are at first message to be 04659 * encrypted under the keys. 04660 */ 04661 if ((ret = DeriveHandshakeSecret(ssl)) != 0) 04662 return ret; 04663 if ((ret = DeriveTls13Keys(ssl, handshake_key, 04664 ENCRYPT_AND_DECRYPT_SIDE, 1)) != 0) 04665 return ret; 04666 04667 /* Setup encrypt/decrypt keys for following messages. */ 04668 #ifdef WOLFSSL_EARLY_DATA 04669 if ((ret = SetKeysSide(ssl, ENCRYPT_SIDE_ONLY)) != 0) 04670 return ret; 04671 if (ssl->earlyData != process_early_data) { 04672 if ((ret = SetKeysSide(ssl, DECRYPT_SIDE_ONLY)) != 0) 04673 return ret; 04674 } 04675 #else 04676 if ((ret = SetKeysSide(ssl, ENCRYPT_AND_DECRYPT_SIDE)) != 0) 04677 return ret; 04678 #endif 04679 04680 ret = TLSX_GetResponseSize(ssl, encrypted_extensions, &length); 04681 if (ret != 0) 04682 return ret; 04683 04684 sendSz = idx + length; 04685 /* Encryption always on. */ 04686 sendSz += MAX_MSG_EXTRA; 04687 04688 /* Check buffers are big enough and grow if needed. */ 04689 ret = CheckAvailableSize(ssl, sendSz); 04690 if (ret != 0) 04691 return ret; 04692 04693 /* Get position in output buffer to write new message to. */ 04694 output = ssl->buffers.outputBuffer.buffer + 04695 ssl->buffers.outputBuffer.length; 04696 04697 /* Put the record and handshake headers on. */ 04698 AddTls13Headers(output, length, encrypted_extensions, ssl); 04699 04700 ret = TLSX_WriteResponse(ssl, output + idx, encrypted_extensions, NULL); 04701 if (ret != 0) 04702 return ret; 04703 idx += length; 04704 04705 #ifdef WOLFSSL_CALLBACKS 04706 if (ssl->hsInfoOn) 04707 AddPacketName(ssl, "EncryptedExtensions"); 04708 if (ssl->toInfoOn) { 04709 AddPacketInfo(ssl, "EncryptedExtensions", handshake, output, 04710 sendSz, WRITE_PROTO, ssl->heap); 04711 } 04712 #endif 04713 04714 /* This handshake message is always encrypted. */ 04715 sendSz = BuildTls13Message(ssl, output, sendSz, output + RECORD_HEADER_SZ, 04716 idx - RECORD_HEADER_SZ, handshake, 1, 0, 0); 04717 if (sendSz < 0) 04718 return sendSz; 04719 04720 ssl->buffers.outputBuffer.length += sendSz; 04721 04722 ssl->options.serverState = SERVER_ENCRYPTED_EXTENSIONS_COMPLETE; 04723 04724 if (!ssl->options.groupMessages) 04725 ret = SendBuffered(ssl); 04726 04727 WOLFSSL_LEAVE("SendTls13EncryptedExtensions", ret); 04728 WOLFSSL_END(WC_FUNC_ENCRYPTED_EXTENSIONS_SEND); 04729 04730 return ret; 04731 } 04732 04733 #ifndef NO_CERTS 04734 /* handle generation TLS v1.3 certificate_request (13) */ 04735 /* Send the TLS v1.3 CertificateRequest message. 04736 * This message is always encrypted in TLS v1.3. 04737 * Only a server will send this message. 04738 * 04739 * ssl SSL/TLS object. 04740 * reqCtx Request context. 04741 * reqCtxLen Length of context. 0 when sending as part of handshake. 04742 * returns 0 on success, otherwise failure. 04743 */ 04744 static int SendTls13CertificateRequest(WOLFSSL* ssl, byte* reqCtx, 04745 int reqCtxLen) 04746 { 04747 byte* output; 04748 int ret; 04749 int sendSz; 04750 word32 i; 04751 word16 reqSz; 04752 #ifndef WOLFSSL_TLS13_DRAFT_18 04753 TLSX* ext; 04754 #endif 04755 04756 WOLFSSL_START(WC_FUNC_CERTIFICATE_REQUEST_SEND); 04757 WOLFSSL_ENTER("SendTls13CertificateRequest"); 04758 04759 if (ssl->options.side == WOLFSSL_SERVER_END) 04760 InitSuitesHashSigAlgo(ssl->suites, 1, 1, 0, 1, ssl->buffers.keySz); 04761 04762 #ifdef WOLFSSL_TLS13_DRAFT_18 04763 i = RECORD_HEADER_SZ + HANDSHAKE_HEADER_SZ; 04764 reqSz = OPAQUE8_LEN + reqCtxLen + REQ_HEADER_SZ + REQ_HEADER_SZ; 04765 reqSz += LENGTH_SZ + ssl->suites->hashSigAlgoSz; 04766 04767 sendSz = RECORD_HEADER_SZ + HANDSHAKE_HEADER_SZ + reqSz; 04768 /* Always encrypted and make room for padding. */ 04769 sendSz += MAX_MSG_EXTRA; 04770 04771 /* Check buffers are big enough and grow if needed. */ 04772 if ((ret = CheckAvailableSize(ssl, sendSz)) != 0) 04773 return ret; 04774 04775 /* Get position in output buffer to write new message to. */ 04776 output = ssl->buffers.outputBuffer.buffer + 04777 ssl->buffers.outputBuffer.length; 04778 04779 /* Put the record and handshake headers on. */ 04780 AddTls13Headers(output, reqSz, certificate_request, ssl); 04781 04782 /* Certificate request context. */ 04783 output[i++] = reqCtxLen; 04784 if (reqCtxLen != 0) { 04785 XMEMCPY(output + i, reqCtx, reqCtxLen); 04786 i += reqCtxLen; 04787 } 04788 04789 /* supported hash/sig */ 04790 c16toa(ssl->suites->hashSigAlgoSz, &output[i]); 04791 i += LENGTH_SZ; 04792 04793 XMEMCPY(&output[i], ssl->suites->hashSigAlgo, ssl->suites->hashSigAlgoSz); 04794 i += ssl->suites->hashSigAlgoSz; 04795 04796 /* Certificate authorities not supported yet - empty buffer. */ 04797 c16toa(0, &output[i]); 04798 i += REQ_HEADER_SZ; 04799 04800 /* Certificate extensions. */ 04801 c16toa(0, &output[i]); /* auth's */ 04802 i += REQ_HEADER_SZ; 04803 #else 04804 ext = TLSX_Find(ssl->extensions, TLSX_SIGNATURE_ALGORITHMS); 04805 if (ext == NULL) 04806 return EXT_MISSING; 04807 ext->resp = 0; 04808 04809 i = RECORD_HEADER_SZ + HANDSHAKE_HEADER_SZ; 04810 reqSz = (word16)(OPAQUE8_LEN + reqCtxLen); 04811 ret = TLSX_GetRequestSize(ssl, certificate_request, &reqSz); 04812 if (ret != 0) 04813 return ret; 04814 04815 sendSz = i + reqSz; 04816 /* Always encrypted and make room for padding. */ 04817 sendSz += MAX_MSG_EXTRA; 04818 04819 /* Check buffers are big enough and grow if needed. */ 04820 if ((ret = CheckAvailableSize(ssl, sendSz)) != 0) 04821 return ret; 04822 04823 /* Get position in output buffer to write new message to. */ 04824 output = ssl->buffers.outputBuffer.buffer + 04825 ssl->buffers.outputBuffer.length; 04826 04827 /* Put the record and handshake headers on. */ 04828 AddTls13Headers(output, reqSz, certificate_request, ssl); 04829 04830 /* Certificate request context. */ 04831 output[i++] = (byte)reqCtxLen; 04832 if (reqCtxLen != 0) { 04833 XMEMCPY(output + i, reqCtx, reqCtxLen); 04834 i += reqCtxLen; 04835 } 04836 04837 /* Certificate extensions. */ 04838 reqSz = 0; 04839 ret = TLSX_WriteRequest(ssl, output + i, certificate_request, &reqSz); 04840 if (ret != 0) 04841 return ret; 04842 i += reqSz; 04843 #endif 04844 04845 /* Always encrypted. */ 04846 sendSz = BuildTls13Message(ssl, output, sendSz, output + RECORD_HEADER_SZ, 04847 i - RECORD_HEADER_SZ, handshake, 1, 0, 0); 04848 if (sendSz < 0) 04849 return sendSz; 04850 04851 #ifdef WOLFSSL_CALLBACKS 04852 if (ssl->hsInfoOn) 04853 AddPacketName(ssl, "CertificateRequest"); 04854 if (ssl->toInfoOn) { 04855 AddPacketInfo(ssl, "CertificateRequest", handshake, output, 04856 sendSz, WRITE_PROTO, ssl->heap); 04857 } 04858 #endif 04859 04860 ssl->buffers.outputBuffer.length += sendSz; 04861 if (!ssl->options.groupMessages) 04862 ret = SendBuffered(ssl); 04863 04864 WOLFSSL_LEAVE("SendTls13CertificateRequest", ret); 04865 WOLFSSL_END(WC_FUNC_CERTIFICATE_REQUEST_SEND); 04866 04867 return ret; 04868 } 04869 #endif /* NO_CERTS */ 04870 #endif /* NO_WOLFSSL_SERVER */ 04871 04872 #ifndef NO_CERTS 04873 #if !defined(NO_RSA) || defined(HAVE_ECC) || defined(HAVE_ED25519) || \ 04874 defined(HAVE_ED448) 04875 /* Encode the signature algorithm into buffer. 04876 * 04877 * hashalgo The hash algorithm. 04878 * hsType The signature type. 04879 * output The buffer to encode into. 04880 */ 04881 static WC_INLINE void EncodeSigAlg(byte hashAlgo, byte hsType, byte* output) 04882 { 04883 switch (hsType) { 04884 #ifdef HAVE_ECC 04885 case ecc_dsa_sa_algo: 04886 output[0] = hashAlgo; 04887 output[1] = ecc_dsa_sa_algo; 04888 break; 04889 #endif 04890 #ifdef HAVE_ED25519 04891 /* ED25519: 0x0807 */ 04892 case ed25519_sa_algo: 04893 output[0] = ED25519_SA_MAJOR; 04894 output[1] = ED25519_SA_MINOR; 04895 (void)hashAlgo; 04896 break; 04897 #endif 04898 #ifdef HAVE_ED448 04899 /* ED448: 0x0808 */ 04900 case ed448_sa_algo: 04901 output[0] = ED448_SA_MAJOR; 04902 output[1] = ED448_SA_MINOR; 04903 (void)hashAlgo; 04904 break; 04905 #endif 04906 #ifndef NO_RSA 04907 /* PSS signatures: 0x080[4-6] */ 04908 case rsa_pss_sa_algo: 04909 output[0] = rsa_pss_sa_algo; 04910 output[1] = hashAlgo; 04911 break; 04912 #endif 04913 } 04914 } 04915 04916 /* Decode the signature algorithm. 04917 * 04918 * input The encoded signature algorithm. 04919 * hashalgo The hash algorithm. 04920 * hsType The signature type. 04921 * returns INVALID_PARAMETER if not recognized and 0 otherwise. 04922 */ 04923 static WC_INLINE int DecodeTls13SigAlg(byte* input, byte* hashAlgo, 04924 byte* hsType) 04925 { 04926 int ret = 0; 04927 04928 switch (input[0]) { 04929 case NEW_SA_MAJOR: 04930 /* PSS signatures: 0x080[4-6] */ 04931 if (input[1] >= sha256_mac && input[1] <= sha512_mac) { 04932 *hsType = input[0]; 04933 *hashAlgo = input[1]; 04934 } 04935 #ifdef HAVE_ED25519 04936 /* ED25519: 0x0807 */ 04937 else if (input[1] == ED25519_SA_MINOR) { 04938 *hsType = ed25519_sa_algo; 04939 /* Hash performed as part of sign/verify operation. */ 04940 *hashAlgo = sha512_mac; 04941 } 04942 #endif 04943 #ifdef HAVE_ED448 04944 /* ED448: 0x0808 */ 04945 else if (input[1] == ED448_SA_MINOR) { 04946 *hsType = ed448_sa_algo; 04947 /* Hash performed as part of sign/verify operation. */ 04948 *hashAlgo = sha512_mac; 04949 } 04950 #endif 04951 else 04952 ret = INVALID_PARAMETER; 04953 break; 04954 default: 04955 *hashAlgo = input[0]; 04956 *hsType = input[1]; 04957 break; 04958 } 04959 04960 return ret; 04961 } 04962 04963 /* Get the hash of the messages so far. 04964 * 04965 * ssl The SSL/TLS object. 04966 * hash The buffer to write the hash to. 04967 * returns the length of the hash. 04968 */ 04969 static WC_INLINE int GetMsgHash(WOLFSSL* ssl, byte* hash) 04970 { 04971 int ret = 0; 04972 switch (ssl->specs.mac_algorithm) { 04973 #ifndef NO_SHA256 04974 case sha256_mac: 04975 ret = wc_Sha256GetHash(&ssl->hsHashes->hashSha256, hash); 04976 if (ret == 0) 04977 ret = WC_SHA256_DIGEST_SIZE; 04978 break; 04979 #endif /* !NO_SHA256 */ 04980 #ifdef WOLFSSL_SHA384 04981 case sha384_mac: 04982 ret = wc_Sha384GetHash(&ssl->hsHashes->hashSha384, hash); 04983 if (ret == 0) 04984 ret = WC_SHA384_DIGEST_SIZE; 04985 break; 04986 #endif /* WOLFSSL_SHA384 */ 04987 #ifdef WOLFSSL_TLS13_SHA512 04988 case sha512_mac: 04989 ret = wc_Sha512GetHash(&ssl->hsHashes->hashSha512, hash); 04990 if (ret == 0) 04991 ret = WC_SHA512_DIGEST_SIZE; 04992 break; 04993 #endif /* WOLFSSL_TLS13_SHA512 */ 04994 } 04995 return ret; 04996 } 04997 04998 /* The length of the certificate verification label - client and server. */ 04999 #define CERT_VFY_LABEL_SZ 34 05000 /* The server certificate verification label. */ 05001 static const byte serverCertVfyLabel[CERT_VFY_LABEL_SZ] = 05002 "TLS 1.3, server CertificateVerify"; 05003 /* The client certificate verification label. */ 05004 static const byte clientCertVfyLabel[CERT_VFY_LABEL_SZ] = 05005 "TLS 1.3, client CertificateVerify"; 05006 05007 /* The number of prefix bytes for signature data. */ 05008 #define SIGNING_DATA_PREFIX_SZ 64 05009 /* The prefix byte in the signature data. */ 05010 #define SIGNING_DATA_PREFIX_BYTE 0x20 05011 /* Maximum length of the signature data. */ 05012 #define MAX_SIG_DATA_SZ (SIGNING_DATA_PREFIX_SZ + \ 05013 CERT_VFY_LABEL_SZ + \ 05014 WC_MAX_DIGEST_SIZE) 05015 05016 /* Create the signature data for TLS v1.3 certificate verification. 05017 * 05018 * ssl The SSL/TLS object. 05019 * sigData The signature data. 05020 * sigDataSz The length of the signature data. 05021 * check Indicates this is a check not create. 05022 */ 05023 static int CreateSigData(WOLFSSL* ssl, byte* sigData, word16* sigDataSz, 05024 int check) 05025 { 05026 word16 idx; 05027 int side = ssl->options.side; 05028 int ret; 05029 05030 /* Signature Data = Prefix | Label | Handshake Hash */ 05031 XMEMSET(sigData, SIGNING_DATA_PREFIX_BYTE, SIGNING_DATA_PREFIX_SZ); 05032 idx = SIGNING_DATA_PREFIX_SZ; 05033 05034 if ((side == WOLFSSL_SERVER_END && check) || 05035 (side == WOLFSSL_CLIENT_END && !check)) { 05036 XMEMCPY(&sigData[idx], clientCertVfyLabel, CERT_VFY_LABEL_SZ); 05037 } 05038 if ((side == WOLFSSL_CLIENT_END && check) || 05039 (side == WOLFSSL_SERVER_END && !check)) { 05040 XMEMCPY(&sigData[idx], serverCertVfyLabel, CERT_VFY_LABEL_SZ); 05041 } 05042 idx += CERT_VFY_LABEL_SZ; 05043 05044 ret = GetMsgHash(ssl, &sigData[idx]); 05045 if (ret < 0) 05046 return ret; 05047 05048 *sigDataSz = (word16)(idx + ret); 05049 ret = 0; 05050 05051 return ret; 05052 } 05053 05054 #ifndef NO_RSA 05055 /* Encode the PKCS #1.5 RSA signature. 05056 * 05057 * sig The buffer to place the encoded signature into. 05058 * sigData The data to be signed. 05059 * sigDataSz The size of the data to be signed. 05060 * hashAlgo The hash algorithm to use when signing. 05061 * returns the length of the encoded signature or negative on error. 05062 */ 05063 static int CreateRSAEncodedSig(byte* sig, byte* sigData, int sigDataSz, 05064 int sigAlgo, int hashAlgo) 05065 { 05066 Digest digest; 05067 int hashSz = 0; 05068 int ret = BAD_FUNC_ARG; 05069 byte* hash; 05070 05071 (void)sigAlgo; 05072 05073 hash = sig; 05074 05075 /* Digest the signature data. */ 05076 switch (hashAlgo) { 05077 #ifndef NO_WOLFSSL_SHA256 05078 case sha256_mac: 05079 ret = wc_InitSha256(&digest.sha256); 05080 if (ret == 0) { 05081 ret = wc_Sha256Update(&digest.sha256, sigData, sigDataSz); 05082 if (ret == 0) 05083 ret = wc_Sha256Final(&digest.sha256, hash); 05084 wc_Sha256Free(&digest.sha256); 05085 } 05086 hashSz = WC_SHA256_DIGEST_SIZE; 05087 break; 05088 #endif 05089 #ifdef WOLFSSL_SHA384 05090 case sha384_mac: 05091 ret = wc_InitSha384(&digest.sha384); 05092 if (ret == 0) { 05093 ret = wc_Sha384Update(&digest.sha384, sigData, sigDataSz); 05094 if (ret == 0) 05095 ret = wc_Sha384Final(&digest.sha384, hash); 05096 wc_Sha384Free(&digest.sha384); 05097 } 05098 hashSz = WC_SHA384_DIGEST_SIZE; 05099 break; 05100 #endif 05101 #ifdef WOLFSSL_SHA512 05102 case sha512_mac: 05103 ret = wc_InitSha512(&digest.sha512); 05104 if (ret == 0) { 05105 ret = wc_Sha512Update(&digest.sha512, sigData, sigDataSz); 05106 if (ret == 0) 05107 ret = wc_Sha512Final(&digest.sha512, hash); 05108 wc_Sha512Free(&digest.sha512); 05109 } 05110 hashSz = WC_SHA512_DIGEST_SIZE; 05111 break; 05112 #endif 05113 } 05114 05115 if (ret != 0) 05116 return ret; 05117 05118 return hashSz; 05119 } 05120 #endif /* !NO_RSA */ 05121 05122 #ifdef HAVE_ECC 05123 /* Encode the ECC signature. 05124 * 05125 * sigData The data to be signed. 05126 * sigDataSz The size of the data to be signed. 05127 * hashAlgo The hash algorithm to use when signing. 05128 * returns the length of the encoded signature or negative on error. 05129 */ 05130 static int CreateECCEncodedSig(byte* sigData, int sigDataSz, int hashAlgo) 05131 { 05132 Digest digest; 05133 int hashSz = 0; 05134 int ret = BAD_FUNC_ARG; 05135 05136 /* Digest the signature data. */ 05137 switch (hashAlgo) { 05138 #ifndef NO_WOLFSSL_SHA256 05139 case sha256_mac: 05140 ret = wc_InitSha256(&digest.sha256); 05141 if (ret == 0) { 05142 ret = wc_Sha256Update(&digest.sha256, sigData, sigDataSz); 05143 if (ret == 0) 05144 ret = wc_Sha256Final(&digest.sha256, sigData); 05145 wc_Sha256Free(&digest.sha256); 05146 } 05147 hashSz = WC_SHA256_DIGEST_SIZE; 05148 break; 05149 #endif 05150 #ifdef WOLFSSL_SHA384 05151 case sha384_mac: 05152 ret = wc_InitSha384(&digest.sha384); 05153 if (ret == 0) { 05154 ret = wc_Sha384Update(&digest.sha384, sigData, sigDataSz); 05155 if (ret == 0) 05156 ret = wc_Sha384Final(&digest.sha384, sigData); 05157 wc_Sha384Free(&digest.sha384); 05158 } 05159 hashSz = WC_SHA384_DIGEST_SIZE; 05160 break; 05161 #endif 05162 #ifdef WOLFSSL_SHA512 05163 case sha512_mac: 05164 ret = wc_InitSha512(&digest.sha512); 05165 if (ret == 0) { 05166 ret = wc_Sha512Update(&digest.sha512, sigData, sigDataSz); 05167 if (ret == 0) 05168 ret = wc_Sha512Final(&digest.sha512, sigData); 05169 wc_Sha512Free(&digest.sha512); 05170 } 05171 hashSz = WC_SHA512_DIGEST_SIZE; 05172 break; 05173 #endif 05174 } 05175 05176 if (ret != 0) 05177 return ret; 05178 05179 return hashSz; 05180 } 05181 #endif /* HAVE_ECC */ 05182 05183 #ifndef NO_RSA 05184 /* Check that the decrypted signature matches the encoded signature 05185 * based on the digest of the signature data. 05186 * 05187 * ssl The SSL/TLS object. 05188 * sigAlgo The signature algorithm used to generate signature. 05189 * hashAlgo The hash algorithm used to generate signature. 05190 * decSig The decrypted signature. 05191 * decSigSz The size of the decrypted signature. 05192 * returns 0 on success, otherwise failure. 05193 */ 05194 static int CheckRSASignature(WOLFSSL* ssl, int sigAlgo, int hashAlgo, 05195 byte* decSig, word32 decSigSz) 05196 { 05197 int ret = 0; 05198 byte sigData[MAX_SIG_DATA_SZ]; 05199 word16 sigDataSz; 05200 word32 sigSz; 05201 05202 ret = CreateSigData(ssl, sigData, &sigDataSz, 1); 05203 if (ret != 0) 05204 return ret; 05205 05206 if (sigAlgo == rsa_pss_sa_algo) { 05207 enum wc_HashType hashType = WC_HASH_TYPE_NONE; 05208 05209 ret = ConvertHashPss(hashAlgo, &hashType, NULL); 05210 if (ret < 0) 05211 return ret; 05212 05213 /* PSS signature can be done in-place */ 05214 ret = CreateRSAEncodedSig(sigData, sigData, sigDataSz, 05215 sigAlgo, hashAlgo); 05216 if (ret < 0) 05217 return ret; 05218 sigSz = ret; 05219 05220 ret = wc_RsaPSS_CheckPadding(sigData, sigSz, decSig, decSigSz, 05221 hashType); 05222 } 05223 05224 return ret; 05225 } 05226 #endif /* !NO_RSA */ 05227 #endif /* !NO_RSA || HAVE_ECC */ 05228 05229 /* Get the next certificate from the list for writing into the TLS v1.3 05230 * Certificate message. 05231 * 05232 * data The certificate list. 05233 * length The length of the certificate data in the list. 05234 * idx The index of the next certificate. 05235 * returns the length of the certificate data. 0 indicates no more certificates 05236 * in the list. 05237 */ 05238 static word32 NextCert(byte* data, word32 length, word32* idx) 05239 { 05240 word32 len; 05241 05242 /* Is index at end of list. */ 05243 if (*idx == length) 05244 return 0; 05245 05246 /* Length of the current ASN.1 encoded certificate. */ 05247 c24to32(data + *idx, &len); 05248 /* Include the length field. */ 05249 len += 3; 05250 05251 /* Move index to next certificate and return the current certificate's 05252 * length. 05253 */ 05254 *idx += len; 05255 return len; 05256 } 05257 05258 /* Add certificate data and empty extension to output up to the fragment size. 05259 * 05260 * ssl SSL/TLS object. 05261 * cert The certificate data to write out. 05262 * len The length of the certificate data. 05263 * extSz Length of the extension data with the certificate. 05264 * idx The start of the certificate data to write out. 05265 * fragSz The maximum size of this fragment. 05266 * output The buffer to write to. 05267 * returns the number of bytes written. 05268 */ 05269 static word32 AddCertExt(WOLFSSL* ssl, byte* cert, word32 len, word16 extSz, 05270 word32 idx, word32 fragSz, byte* output) 05271 { 05272 word32 i = 0; 05273 word32 copySz = min(len - idx, fragSz); 05274 05275 if (idx < len) { 05276 XMEMCPY(output, cert + idx, copySz); 05277 i = copySz; 05278 if (copySz == fragSz) 05279 return i; 05280 } 05281 copySz = len + extSz - idx - i; 05282 05283 if (extSz == OPAQUE16_LEN) { 05284 if (copySz <= fragSz) { 05285 /* Empty extension */ 05286 output[i++] = 0; 05287 output[i++] = 0; 05288 } 05289 } 05290 else { 05291 byte* certExts = ssl->buffers.certExts->buffer + idx + i - len; 05292 /* Put out as much of the extensions' data as will fit in fragment. */ 05293 if (copySz > fragSz - i) 05294 copySz = fragSz - i; 05295 XMEMCPY(output + i, certExts, copySz); 05296 i += copySz; 05297 } 05298 05299 return i; 05300 } 05301 05302 /* handle generation TLS v1.3 certificate (11) */ 05303 /* Send the certificate for this end and any CAs that help with validation. 05304 * This message is always encrypted in TLS v1.3. 05305 * 05306 * ssl The SSL/TLS object. 05307 * returns 0 on success, otherwise failure. 05308 */ 05309 static int SendTls13Certificate(WOLFSSL* ssl) 05310 { 05311 int ret = 0; 05312 word32 certSz, certChainSz, headerSz, listSz, payloadSz; 05313 word16 extSz = 0; 05314 word32 length, maxFragment; 05315 word32 len = 0; 05316 word32 idx = 0; 05317 word32 offset = OPAQUE16_LEN; 05318 byte* p = NULL; 05319 byte certReqCtxLen = 0; 05320 byte* certReqCtx = NULL; 05321 05322 WOLFSSL_START(WC_FUNC_CERTIFICATE_SEND); 05323 WOLFSSL_ENTER("SendTls13Certificate"); 05324 05325 #ifdef WOLFSSL_POST_HANDSHAKE_AUTH 05326 if (ssl->options.side == WOLFSSL_CLIENT_END && ssl->certReqCtx != NULL) { 05327 certReqCtxLen = ssl->certReqCtx->len; 05328 certReqCtx = &ssl->certReqCtx->ctx; 05329 } 05330 #endif 05331 05332 if (ssl->options.sendVerify == SEND_BLANK_CERT) { 05333 certSz = 0; 05334 certChainSz = 0; 05335 headerSz = OPAQUE8_LEN + certReqCtxLen + CERT_HEADER_SZ; 05336 length = headerSz; 05337 listSz = 0; 05338 } 05339 else { 05340 if (!ssl->buffers.certificate) { 05341 WOLFSSL_MSG("Send Cert missing certificate buffer"); 05342 return BUFFER_ERROR; 05343 } 05344 /* Certificate Data */ 05345 certSz = ssl->buffers.certificate->length; 05346 /* Cert Req Ctx Len | Cert Req Ctx | Cert List Len | Cert Data Len */ 05347 headerSz = OPAQUE8_LEN + certReqCtxLen + CERT_HEADER_SZ + 05348 CERT_HEADER_SZ; 05349 05350 ret = TLSX_GetResponseSize(ssl, certificate, &extSz); 05351 if (ret < 0) 05352 return ret; 05353 05354 /* Create extensions' data if none already present. */ 05355 if (extSz > OPAQUE16_LEN && ssl->buffers.certExts == NULL) { 05356 ret = AllocDer(&ssl->buffers.certExts, extSz, CERT_TYPE, ssl->heap); 05357 if (ret < 0) 05358 return ret; 05359 05360 extSz = 0; 05361 ret = TLSX_WriteResponse(ssl, ssl->buffers.certExts->buffer, 05362 certificate, &extSz); 05363 if (ret < 0) 05364 return ret; 05365 } 05366 05367 /* Length of message data with one certificate and extensions. */ 05368 length = headerSz + certSz + extSz; 05369 /* Length of list data with one certificate and extensions. */ 05370 listSz = CERT_HEADER_SZ + certSz + extSz; 05371 05372 /* Send rest of chain if sending cert (chain has leading size/s). */ 05373 if (certSz > 0 && ssl->buffers.certChainCnt > 0) { 05374 p = ssl->buffers.certChain->buffer; 05375 /* Chain length including extensions. */ 05376 certChainSz = ssl->buffers.certChain->length + 05377 OPAQUE16_LEN * ssl->buffers.certChainCnt; 05378 length += certChainSz; 05379 listSz += certChainSz; 05380 } 05381 else 05382 certChainSz = 0; 05383 } 05384 05385 payloadSz = length; 05386 05387 if (ssl->fragOffset != 0) 05388 length -= (ssl->fragOffset + headerSz); 05389 05390 maxFragment = wolfSSL_GetMaxRecordSize(ssl, MAX_RECORD_SIZE); 05391 05392 while (length > 0 && ret == 0) { 05393 byte* output = NULL; 05394 word32 fragSz = 0; 05395 word32 i = RECORD_HEADER_SZ; 05396 int sendSz = RECORD_HEADER_SZ; 05397 05398 if (ssl->fragOffset == 0) { 05399 if (headerSz + certSz + extSz + certChainSz <= 05400 maxFragment - HANDSHAKE_HEADER_SZ) { 05401 fragSz = headerSz + certSz + extSz + certChainSz; 05402 } 05403 else 05404 fragSz = maxFragment - HANDSHAKE_HEADER_SZ; 05405 05406 sendSz += fragSz + HANDSHAKE_HEADER_SZ; 05407 i += HANDSHAKE_HEADER_SZ; 05408 } 05409 else { 05410 fragSz = min(length, maxFragment); 05411 sendSz += fragSz; 05412 } 05413 05414 sendSz += MAX_MSG_EXTRA; 05415 05416 /* Check buffers are big enough and grow if needed. */ 05417 if ((ret = CheckAvailableSize(ssl, sendSz)) != 0) 05418 return ret; 05419 05420 /* Get position in output buffer to write new message to. */ 05421 output = ssl->buffers.outputBuffer.buffer + 05422 ssl->buffers.outputBuffer.length; 05423 05424 if (ssl->fragOffset == 0) { 05425 AddTls13FragHeaders(output, fragSz, 0, payloadSz, certificate, ssl); 05426 05427 /* Request context. */ 05428 output[i++] = certReqCtxLen; 05429 if (certReqCtxLen > 0) { 05430 XMEMCPY(output + i, certReqCtx, certReqCtxLen); 05431 i += certReqCtxLen; 05432 } 05433 length -= OPAQUE8_LEN + certReqCtxLen; 05434 fragSz -= OPAQUE8_LEN + certReqCtxLen; 05435 /* Certificate list length. */ 05436 c32to24(listSz, output + i); 05437 i += CERT_HEADER_SZ; 05438 length -= CERT_HEADER_SZ; 05439 fragSz -= CERT_HEADER_SZ; 05440 /* Leaf certificate data length. */ 05441 if (certSz > 0) { 05442 c32to24(certSz, output + i); 05443 i += CERT_HEADER_SZ; 05444 length -= CERT_HEADER_SZ; 05445 fragSz -= CERT_HEADER_SZ; 05446 } 05447 } 05448 else 05449 AddTls13RecordHeader(output, fragSz, handshake, ssl); 05450 05451 if (certSz > 0 && ssl->fragOffset < certSz + extSz) { 05452 /* Put in the leaf certificate with extensions. */ 05453 word32 copySz = AddCertExt(ssl, ssl->buffers.certificate->buffer, 05454 certSz, extSz, ssl->fragOffset, fragSz, output + i); 05455 i += copySz; 05456 ssl->fragOffset += copySz; 05457 length -= copySz; 05458 fragSz -= copySz; 05459 if (ssl->fragOffset == certSz + extSz) 05460 FreeDer(&ssl->buffers.certExts); 05461 } 05462 if (certChainSz > 0 && fragSz > 0) { 05463 /* Put in the CA certificates with empty extensions. */ 05464 while (fragSz > 0) { 05465 word32 l; 05466 05467 if (offset == len + OPAQUE16_LEN) { 05468 /* Find next CA certificate to write out. */ 05469 offset = 0; 05470 /* Point to the start of current cert in chain buffer. */ 05471 p = ssl->buffers.certChain->buffer + idx; 05472 len = NextCert(ssl->buffers.certChain->buffer, 05473 ssl->buffers.certChain->length, &idx); 05474 if (len == 0) 05475 break; 05476 } 05477 05478 /* Write out certificate and empty extension. */ 05479 l = AddCertExt(ssl, p, len, OPAQUE16_LEN, offset, fragSz, 05480 output + i); 05481 i += l; 05482 ssl->fragOffset += l; 05483 length -= l; 05484 fragSz -= l; 05485 offset += l; 05486 } 05487 } 05488 05489 if ((int)i - RECORD_HEADER_SZ < 0) { 05490 WOLFSSL_MSG("Send Cert bad inputSz"); 05491 return BUFFER_E; 05492 } 05493 05494 /* This message is always encrypted. */ 05495 sendSz = BuildTls13Message(ssl, output, sendSz, 05496 output + RECORD_HEADER_SZ, 05497 i - RECORD_HEADER_SZ, handshake, 1, 0, 0); 05498 if (sendSz < 0) 05499 return sendSz; 05500 05501 #ifdef WOLFSSL_CALLBACKS 05502 if (ssl->hsInfoOn) 05503 AddPacketName(ssl, "Certificate"); 05504 if (ssl->toInfoOn) { 05505 AddPacketInfo(ssl, "Certificate", handshake, output, 05506 sendSz, WRITE_PROTO, ssl->heap); 05507 } 05508 #endif 05509 05510 ssl->buffers.outputBuffer.length += sendSz; 05511 if (!ssl->options.groupMessages) 05512 ret = SendBuffered(ssl); 05513 } 05514 05515 if (ret != WANT_WRITE) { 05516 /* Clean up the fragment offset. */ 05517 ssl->fragOffset = 0; 05518 if (ssl->options.side == WOLFSSL_SERVER_END) 05519 ssl->options.serverState = SERVER_CERT_COMPLETE; 05520 } 05521 05522 #ifdef WOLFSSL_POST_HANDSHAKE_AUTH 05523 if (ssl->options.side == WOLFSSL_CLIENT_END && ssl->certReqCtx != NULL) { 05524 CertReqCtx* ctx = ssl->certReqCtx; 05525 ssl->certReqCtx = ssl->certReqCtx->next; 05526 XFREE(ctx, ssl->heap, DYNAMIC_TYPE_TMP_BUFFER); 05527 } 05528 #endif 05529 05530 WOLFSSL_LEAVE("SendTls13Certificate", ret); 05531 WOLFSSL_END(WC_FUNC_CERTIFICATE_SEND); 05532 05533 return ret; 05534 } 05535 05536 typedef struct Scv13Args { 05537 byte* output; /* not allocated */ 05538 byte* verify; /* not allocated */ 05539 word32 idx; 05540 word32 sigLen; 05541 int sendSz; 05542 word16 length; 05543 05544 byte sigAlgo; 05545 byte* sigData; 05546 word16 sigDataSz; 05547 } Scv13Args; 05548 05549 static void FreeScv13Args(WOLFSSL* ssl, void* pArgs) 05550 { 05551 Scv13Args* args = (Scv13Args*)pArgs; 05552 05553 (void)ssl; 05554 05555 if (args->sigData) { 05556 XFREE(args->sigData, ssl->heap, DYNAMIC_TYPE_SIGNATURE); 05557 args->sigData = NULL; 05558 } 05559 } 05560 05561 /* handle generation TLS v1.3 certificate_verify (15) */ 05562 /* Send the TLS v1.3 CertificateVerify message. 05563 * A hash of all the message so far is used. 05564 * The signed data is: 05565 * 0x20 * 64 | context string | 0x00 | hash of messages 05566 * This message is always encrypted in TLS v1.3. 05567 * 05568 * ssl The SSL/TLS object. 05569 * returns 0 on success, otherwise failure. 05570 */ 05571 static int SendTls13CertificateVerify(WOLFSSL* ssl) 05572 { 05573 int ret = 0; 05574 buffer* sig = &ssl->buffers.sig; 05575 #ifdef WOLFSSL_ASYNC_CRYPT 05576 Scv13Args* args = (Scv13Args*)ssl->async.args; 05577 typedef char args_test[sizeof(ssl->async.args) >= sizeof(*args) ? 1 : -1]; 05578 (void)sizeof(args_test); 05579 #else 05580 Scv13Args args[1]; 05581 #endif 05582 05583 WOLFSSL_START(WC_FUNC_CERTIFICATE_VERIFY_SEND); 05584 WOLFSSL_ENTER("SendTls13CertificateVerify"); 05585 05586 #ifdef WOLFSSL_ASYNC_CRYPT 05587 ret = wolfSSL_AsyncPop(ssl, &ssl->options.asyncState); 05588 if (ret != WC_NOT_PENDING_E) { 05589 /* Check for error */ 05590 if (ret < 0) 05591 goto exit_scv; 05592 } 05593 else 05594 #endif 05595 { 05596 /* Reset state */ 05597 ret = 0; 05598 ssl->options.asyncState = TLS_ASYNC_BEGIN; 05599 XMEMSET(args, 0, sizeof(Scv13Args)); 05600 #ifdef WOLFSSL_ASYNC_CRYPT 05601 ssl->async.freeArgs = FreeScv13Args; 05602 #endif 05603 } 05604 05605 switch(ssl->options.asyncState) 05606 { 05607 case TLS_ASYNC_BEGIN: 05608 { 05609 if (ssl->options.sendVerify == SEND_BLANK_CERT) { 05610 return 0; /* sent blank cert, can't verify */ 05611 } 05612 05613 args->sendSz = MAX_CERT_VERIFY_SZ + MAX_MSG_EXTRA; 05614 /* Always encrypted. */ 05615 args->sendSz += MAX_MSG_EXTRA; 05616 05617 /* check for available size */ 05618 if ((ret = CheckAvailableSize(ssl, args->sendSz)) != 0) { 05619 goto exit_scv; 05620 } 05621 05622 /* get output buffer */ 05623 args->output = ssl->buffers.outputBuffer.buffer + 05624 ssl->buffers.outputBuffer.length; 05625 05626 /* Advance state and proceed */ 05627 ssl->options.asyncState = TLS_ASYNC_BUILD; 05628 } /* case TLS_ASYNC_BEGIN */ 05629 FALL_THROUGH; 05630 05631 case TLS_ASYNC_BUILD: 05632 { 05633 /* idx is used to track verify pointer offset to output */ 05634 args->idx = RECORD_HEADER_SZ + HANDSHAKE_HEADER_SZ; 05635 args->verify = 05636 &args->output[RECORD_HEADER_SZ + HANDSHAKE_HEADER_SZ]; 05637 05638 if (ssl->buffers.key == NULL) { 05639 #ifdef HAVE_PK_CALLBACKS 05640 if (wolfSSL_CTX_IsPrivatePkSet(ssl->ctx)) 05641 args->length = GetPrivateKeySigSize(ssl); 05642 else 05643 #endif 05644 ERROR_OUT(NO_PRIVATE_KEY, exit_scv); 05645 } 05646 else { 05647 ret = DecodePrivateKey(ssl, &args->length); 05648 if (ret != 0) 05649 goto exit_scv; 05650 } 05651 05652 if (args->length <= 0) { 05653 ERROR_OUT(NO_PRIVATE_KEY, exit_scv); 05654 } 05655 05656 /* Add signature algorithm. */ 05657 if (ssl->hsType == DYNAMIC_TYPE_RSA) 05658 args->sigAlgo = rsa_pss_sa_algo; 05659 else if (ssl->hsType == DYNAMIC_TYPE_ECC) 05660 args->sigAlgo = ecc_dsa_sa_algo; 05661 #ifdef HAVE_ED25519 05662 else if (ssl->hsType == DYNAMIC_TYPE_ED25519) 05663 args->sigAlgo = ed25519_sa_algo; 05664 #endif 05665 #ifdef HAVE_ED448 05666 else if (ssl->hsType == DYNAMIC_TYPE_ED448) 05667 args->sigAlgo = ed448_sa_algo; 05668 #endif 05669 EncodeSigAlg(ssl->suites->hashAlgo, args->sigAlgo, args->verify); 05670 05671 if (ssl->hsType == DYNAMIC_TYPE_RSA) { 05672 int sigLen = MAX_SIG_DATA_SZ; 05673 if (args->length > MAX_SIG_DATA_SZ) 05674 sigLen = args->length; 05675 args->sigData = (byte*)XMALLOC(sigLen, ssl->heap, 05676 DYNAMIC_TYPE_SIGNATURE); 05677 } 05678 else { 05679 args->sigData = (byte*)XMALLOC(MAX_SIG_DATA_SZ, ssl->heap, 05680 DYNAMIC_TYPE_SIGNATURE); 05681 } 05682 if (args->sigData == NULL) { 05683 ERROR_OUT(MEMORY_E, exit_scv); 05684 } 05685 05686 /* Create the data to be signed. */ 05687 ret = CreateSigData(ssl, args->sigData, &args->sigDataSz, 0); 05688 if (ret != 0) 05689 goto exit_scv; 05690 05691 #ifndef NO_RSA 05692 if (ssl->hsType == DYNAMIC_TYPE_RSA) { 05693 /* build encoded signature buffer */ 05694 sig->length = WC_MAX_DIGEST_SIZE; 05695 sig->buffer = (byte*)XMALLOC(sig->length, ssl->heap, 05696 DYNAMIC_TYPE_SIGNATURE); 05697 if (sig->buffer == NULL) { 05698 ERROR_OUT(MEMORY_E, exit_scv); 05699 } 05700 05701 ret = CreateRSAEncodedSig(sig->buffer, args->sigData, 05702 args->sigDataSz, args->sigAlgo, ssl->suites->hashAlgo); 05703 if (ret < 0) 05704 goto exit_scv; 05705 sig->length = ret; 05706 ret = 0; 05707 05708 /* Maximum size of RSA Signature. */ 05709 args->sigLen = args->length; 05710 } 05711 #endif /* !NO_RSA */ 05712 #ifdef HAVE_ECC 05713 if (ssl->hsType == DYNAMIC_TYPE_ECC) { 05714 sig->length = args->sendSz - args->idx - HASH_SIG_SIZE - 05715 VERIFY_HEADER; 05716 ret = CreateECCEncodedSig(args->sigData, 05717 args->sigDataSz, ssl->suites->hashAlgo); 05718 if (ret < 0) 05719 goto exit_scv; 05720 args->sigDataSz = (word16)ret; 05721 ret = 0; 05722 } 05723 #endif /* HAVE_ECC */ 05724 #ifdef HAVE_ED25519 05725 if (ssl->hsType == DYNAMIC_TYPE_ED25519) { 05726 ret = Ed25519CheckPubKey(ssl); 05727 if (ret < 0) { 05728 ERROR_OUT(ret, exit_scv); 05729 } 05730 sig->length = ED25519_SIG_SIZE; 05731 } 05732 #endif /* HAVE_ED25519 */ 05733 #ifdef HAVE_ED448 05734 if (ssl->hsType == DYNAMIC_TYPE_ED448) { 05735 ret = Ed448CheckPubKey(ssl); 05736 if (ret < 0) { 05737 ERROR_OUT(ret, exit_scv); 05738 } 05739 sig->length = ED448_SIG_SIZE; 05740 } 05741 #endif /* HAVE_ED448 */ 05742 05743 /* Advance state and proceed */ 05744 ssl->options.asyncState = TLS_ASYNC_DO; 05745 } /* case TLS_ASYNC_BUILD */ 05746 FALL_THROUGH; 05747 05748 case TLS_ASYNC_DO: 05749 { 05750 #ifdef HAVE_ECC 05751 if (ssl->hsType == DYNAMIC_TYPE_ECC) { 05752 05753 ret = EccSign(ssl, args->sigData, args->sigDataSz, 05754 args->verify + HASH_SIG_SIZE + VERIFY_HEADER, 05755 (word32*)&sig->length, (ecc_key*)ssl->hsKey, 05756 #ifdef HAVE_PK_CALLBACKS 05757 ssl->buffers.key 05758 #else 05759 NULL 05760 #endif 05761 ); 05762 args->length = (word16)sig->length; 05763 } 05764 #endif /* HAVE_ECC */ 05765 #ifdef HAVE_ED25519 05766 if (ssl->hsType == DYNAMIC_TYPE_ED25519) { 05767 ret = Ed25519Sign(ssl, args->sigData, args->sigDataSz, 05768 args->verify + HASH_SIG_SIZE + VERIFY_HEADER, 05769 (word32*)&sig->length, (ed25519_key*)ssl->hsKey, 05770 #ifdef HAVE_PK_CALLBACKS 05771 ssl->buffers.key 05772 #else 05773 NULL 05774 #endif 05775 ); 05776 args->length = (word16)sig->length; 05777 } 05778 #endif 05779 #ifdef HAVE_ED448 05780 if (ssl->hsType == DYNAMIC_TYPE_ED448) { 05781 ret = Ed448Sign(ssl, args->sigData, args->sigDataSz, 05782 args->verify + HASH_SIG_SIZE + VERIFY_HEADER, 05783 (word32*)&sig->length, (ed448_key*)ssl->hsKey, 05784 #ifdef HAVE_PK_CALLBACKS 05785 ssl->buffers.key 05786 #else 05787 NULL 05788 #endif 05789 ); 05790 args->length = (word16)sig->length; 05791 } 05792 #endif 05793 #ifndef NO_RSA 05794 if (ssl->hsType == DYNAMIC_TYPE_RSA) { 05795 ret = RsaSign(ssl, sig->buffer, (word32)sig->length, 05796 args->verify + HASH_SIG_SIZE + VERIFY_HEADER, &args->sigLen, 05797 args->sigAlgo, ssl->suites->hashAlgo, 05798 (RsaKey*)ssl->hsKey, 05799 ssl->buffers.key 05800 ); 05801 if (ret == 0) { 05802 args->length = (word16)args->sigLen; 05803 05804 XMEMCPY(args->sigData, 05805 args->verify + HASH_SIG_SIZE + VERIFY_HEADER, 05806 args->sigLen); 05807 } 05808 } 05809 #endif /* !NO_RSA */ 05810 05811 /* Check for error */ 05812 if (ret != 0) { 05813 goto exit_scv; 05814 } 05815 05816 /* Add signature length. */ 05817 c16toa(args->length, args->verify + HASH_SIG_SIZE); 05818 05819 /* Advance state and proceed */ 05820 ssl->options.asyncState = TLS_ASYNC_VERIFY; 05821 } /* case TLS_ASYNC_DO */ 05822 FALL_THROUGH; 05823 05824 case TLS_ASYNC_VERIFY: 05825 { 05826 #ifndef NO_RSA 05827 if (ssl->hsType == DYNAMIC_TYPE_RSA) { 05828 /* check for signature faults */ 05829 ret = VerifyRsaSign(ssl, args->sigData, args->sigLen, 05830 sig->buffer, (word32)sig->length, args->sigAlgo, 05831 ssl->suites->hashAlgo, (RsaKey*)ssl->hsKey, 05832 ssl->buffers.key 05833 ); 05834 } 05835 #endif /* !NO_RSA */ 05836 05837 /* Check for error */ 05838 if (ret != 0) { 05839 goto exit_scv; 05840 } 05841 05842 /* Advance state and proceed */ 05843 ssl->options.asyncState = TLS_ASYNC_FINALIZE; 05844 } /* case TLS_ASYNC_VERIFY */ 05845 FALL_THROUGH; 05846 05847 case TLS_ASYNC_FINALIZE: 05848 { 05849 /* Put the record and handshake headers on. */ 05850 AddTls13Headers(args->output, args->length + HASH_SIG_SIZE + 05851 VERIFY_HEADER, certificate_verify, ssl); 05852 05853 args->sendSz = RECORD_HEADER_SZ + HANDSHAKE_HEADER_SZ + 05854 args->length + HASH_SIG_SIZE + VERIFY_HEADER; 05855 05856 /* Advance state and proceed */ 05857 ssl->options.asyncState = TLS_ASYNC_END; 05858 } /* case TLS_ASYNC_FINALIZE */ 05859 FALL_THROUGH; 05860 05861 case TLS_ASYNC_END: 05862 { 05863 /* This message is always encrypted. */ 05864 ret = BuildTls13Message(ssl, args->output, 05865 MAX_CERT_VERIFY_SZ + MAX_MSG_EXTRA, 05866 args->output + RECORD_HEADER_SZ, 05867 args->sendSz - RECORD_HEADER_SZ, handshake, 05868 1, 0, 0); 05869 05870 if (ret < 0) { 05871 goto exit_scv; 05872 } 05873 else { 05874 args->sendSz = ret; 05875 ret = 0; 05876 } 05877 05878 #ifdef WOLFSSL_CALLBACKS 05879 if (ssl->hsInfoOn) 05880 AddPacketName(ssl, "CertificateVerify"); 05881 if (ssl->toInfoOn) { 05882 AddPacketInfo(ssl, "CertificateVerify", handshake, 05883 args->output, args->sendSz, WRITE_PROTO, ssl->heap); 05884 } 05885 #endif 05886 05887 ssl->buffers.outputBuffer.length += args->sendSz; 05888 05889 if (!ssl->options.groupMessages) 05890 ret = SendBuffered(ssl); 05891 break; 05892 } 05893 default: 05894 ret = INPUT_CASE_ERROR; 05895 } /* switch(ssl->options.asyncState) */ 05896 05897 exit_scv: 05898 05899 WOLFSSL_LEAVE("SendTls13CertificateVerify", ret); 05900 WOLFSSL_END(WC_FUNC_CERTIFICATE_VERIFY_SEND); 05901 05902 #ifdef WOLFSSL_ASYNC_CRYPT 05903 /* Handle async operation */ 05904 if (ret == WC_PENDING_E) { 05905 return ret; 05906 } 05907 #endif /* WOLFSSL_ASYNC_CRYPT */ 05908 05909 /* Final cleanup */ 05910 FreeScv13Args(ssl, args); 05911 FreeKeyExchange(ssl); 05912 05913 return ret; 05914 } 05915 05916 /* handle processing TLS v1.3 certificate (11) */ 05917 /* Parse and handle a TLS v1.3 Certificate message. 05918 * 05919 * ssl The SSL/TLS object. 05920 * input The message buffer. 05921 * inOutIdx On entry, the index into the message buffer of Certificate. 05922 * On exit, the index of byte after the Certificate message. 05923 * totalSz The length of the current handshake message. 05924 * returns 0 on success and otherwise failure. 05925 */ 05926 static int DoTls13Certificate(WOLFSSL* ssl, byte* input, word32* inOutIdx, 05927 word32 totalSz) 05928 { 05929 int ret; 05930 05931 WOLFSSL_START(WC_FUNC_CERTIFICATE_DO); 05932 WOLFSSL_ENTER("DoTls13Certificate"); 05933 05934 ret = ProcessPeerCerts(ssl, input, inOutIdx, totalSz); 05935 if (ret == 0) { 05936 #if !defined(NO_WOLFSSL_CLIENT) 05937 if (ssl->options.side == WOLFSSL_CLIENT_END) 05938 ssl->options.serverState = SERVER_CERT_COMPLETE; 05939 #endif 05940 #if !defined(NO_WOLFSSL_SERVER) && defined(WOLFSSL_POST_HANDSHAKE_AUTH) 05941 if (ssl->options.side == WOLFSSL_SERVER_END && 05942 ssl->options.handShakeState == HANDSHAKE_DONE) { 05943 /* reset handshake states */ 05944 ssl->options.serverState = SERVER_FINISHED_COMPLETE; 05945 ssl->options.acceptState = TICKET_SENT; 05946 ssl->options.handShakeState = SERVER_FINISHED_COMPLETE; 05947 } 05948 #endif 05949 } 05950 05951 WOLFSSL_LEAVE("DoTls13Certificate", ret); 05952 WOLFSSL_END(WC_FUNC_CERTIFICATE_DO); 05953 05954 return ret; 05955 } 05956 05957 #if !defined(NO_RSA) || defined(HAVE_ECC) || defined(HAVE_ED25519) || \ 05958 defined(HAVE_ED448) 05959 05960 typedef struct Dcv13Args { 05961 byte* output; /* not allocated */ 05962 word32 sendSz; 05963 word16 sz; 05964 word32 sigSz; 05965 word32 idx; 05966 word32 begin; 05967 byte hashAlgo; 05968 byte sigAlgo; 05969 05970 byte* sigData; 05971 word16 sigDataSz; 05972 } Dcv13Args; 05973 05974 static void FreeDcv13Args(WOLFSSL* ssl, void* pArgs) 05975 { 05976 Dcv13Args* args = (Dcv13Args*)pArgs; 05977 05978 if (args->sigData != NULL) { 05979 XFREE(args->sigData, ssl->heap, DYNAMIC_TYPE_SIGNATURE); 05980 args->sigData = NULL; 05981 } 05982 05983 (void)ssl; 05984 } 05985 05986 /* handle processing TLS v1.3 certificate_verify (15) */ 05987 /* Parse and handle a TLS v1.3 CertificateVerify message. 05988 * 05989 * ssl The SSL/TLS object. 05990 * input The message buffer. 05991 * inOutIdx On entry, the index into the message buffer of 05992 * CertificateVerify. 05993 * On exit, the index of byte after the CertificateVerify message. 05994 * totalSz The length of the current handshake message. 05995 * returns 0 on success and otherwise failure. 05996 */ 05997 static int DoTls13CertificateVerify(WOLFSSL* ssl, byte* input, 05998 word32* inOutIdx, word32 totalSz) 05999 { 06000 int ret = 0; 06001 buffer* sig = &ssl->buffers.sig; 06002 #ifdef WOLFSSL_ASYNC_CRYPT 06003 Dcv13Args* args = (Dcv13Args*)ssl->async.args; 06004 typedef char args_test[sizeof(ssl->async.args) >= sizeof(*args) ? 1 : -1]; 06005 (void)sizeof(args_test); 06006 #else 06007 Dcv13Args args[1]; 06008 #endif 06009 06010 WOLFSSL_START(WC_FUNC_CERTIFICATE_VERIFY_DO); 06011 WOLFSSL_ENTER("DoTls13CertificateVerify"); 06012 06013 #ifdef WOLFSSL_ASYNC_CRYPT 06014 ret = wolfSSL_AsyncPop(ssl, &ssl->options.asyncState); 06015 if (ret != WC_NOT_PENDING_E) { 06016 /* Check for error */ 06017 if (ret < 0) 06018 goto exit_dcv; 06019 } 06020 else 06021 #endif 06022 { 06023 /* Reset state */ 06024 ret = 0; 06025 ssl->options.asyncState = TLS_ASYNC_BEGIN; 06026 XMEMSET(args, 0, sizeof(Dcv13Args)); 06027 args->hashAlgo = sha_mac; 06028 args->sigAlgo = anonymous_sa_algo; 06029 args->idx = *inOutIdx; 06030 args->begin = *inOutIdx; 06031 #ifdef WOLFSSL_ASYNC_CRYPT 06032 ssl->async.freeArgs = FreeDcv13Args; 06033 #endif 06034 } 06035 06036 switch(ssl->options.asyncState) 06037 { 06038 case TLS_ASYNC_BEGIN: 06039 { 06040 #ifdef WOLFSSL_CALLBACKS 06041 if (ssl->hsInfoOn) AddPacketName(ssl, "CertificateVerify"); 06042 if (ssl->toInfoOn) AddLateName("CertificateVerify", 06043 &ssl->timeoutInfo); 06044 #endif 06045 06046 /* Advance state and proceed */ 06047 ssl->options.asyncState = TLS_ASYNC_BUILD; 06048 } /* case TLS_ASYNC_BEGIN */ 06049 FALL_THROUGH; 06050 06051 case TLS_ASYNC_BUILD: 06052 { 06053 /* Signature algorithm. */ 06054 if ((args->idx - args->begin) + ENUM_LEN + ENUM_LEN > totalSz) { 06055 ERROR_OUT(BUFFER_ERROR, exit_dcv); 06056 } 06057 ret = DecodeTls13SigAlg(input + args->idx, &args->hashAlgo, 06058 &args->sigAlgo); 06059 if (ret < 0) 06060 goto exit_dcv; 06061 args->idx += OPAQUE16_LEN; 06062 06063 /* Signature length. */ 06064 if ((args->idx - args->begin) + OPAQUE16_LEN > totalSz) { 06065 ERROR_OUT(BUFFER_ERROR, exit_dcv); 06066 } 06067 ato16(input + args->idx, &args->sz); 06068 args->idx += OPAQUE16_LEN; 06069 06070 /* Signature data. */ 06071 if ((args->idx - args->begin) + args->sz > totalSz || 06072 args->sz > ENCRYPT_LEN) { 06073 ERROR_OUT(BUFFER_ERROR, exit_dcv); 06074 } 06075 06076 /* Check for public key of required type. */ 06077 #ifdef HAVE_ED25519 06078 if (args->sigAlgo == ed25519_sa_algo && 06079 !ssl->peerEd25519KeyPresent) { 06080 WOLFSSL_MSG("Oops, peer sent ED25519 key but not in verify"); 06081 } 06082 #endif 06083 #ifdef HAVE_ED448 06084 if (args->sigAlgo == ed448_sa_algo && !ssl->peerEd448KeyPresent) { 06085 WOLFSSL_MSG("Oops, peer sent ED448 key but not in verify"); 06086 } 06087 #endif 06088 #ifdef HAVE_ECC 06089 if (args->sigAlgo == ecc_dsa_sa_algo && 06090 !ssl->peerEccDsaKeyPresent) { 06091 WOLFSSL_MSG("Oops, peer sent ECC key but not in verify"); 06092 } 06093 #endif 06094 #ifndef NO_RSA 06095 if (args->sigAlgo == rsa_sa_algo) { 06096 WOLFSSL_MSG("Oops, peer sent PKCS#1.5 signature"); 06097 ERROR_OUT(INVALID_PARAMETER, exit_dcv); 06098 } 06099 if (args->sigAlgo == rsa_pss_sa_algo && 06100 (ssl->peerRsaKey == NULL || !ssl->peerRsaKeyPresent)) { 06101 WOLFSSL_MSG("Oops, peer sent RSA key but not in verify"); 06102 } 06103 #endif 06104 06105 sig->buffer = (byte*)XMALLOC(args->sz, ssl->heap, 06106 DYNAMIC_TYPE_SIGNATURE); 06107 if (sig->buffer == NULL) { 06108 ERROR_OUT(MEMORY_E, exit_dcv); 06109 } 06110 sig->length = args->sz; 06111 XMEMCPY(sig->buffer, input + args->idx, args->sz); 06112 06113 #ifdef HAVE_ECC 06114 if (ssl->peerEccDsaKeyPresent) { 06115 WOLFSSL_MSG("Doing ECC peer cert verify"); 06116 06117 args->sigData = (byte*)XMALLOC(MAX_SIG_DATA_SZ, ssl->heap, 06118 DYNAMIC_TYPE_SIGNATURE); 06119 if (args->sigData == NULL) { 06120 ERROR_OUT(MEMORY_E, exit_dcv); 06121 } 06122 06123 ret = CreateSigData(ssl, args->sigData, &args->sigDataSz, 1); 06124 if (ret != 0) 06125 goto exit_dcv; 06126 ret = CreateECCEncodedSig(args->sigData, 06127 args->sigDataSz, args->hashAlgo); 06128 if (ret < 0) 06129 goto exit_dcv; 06130 args->sigDataSz = (word16)ret; 06131 ret = 0; 06132 } 06133 #endif 06134 #ifdef HAVE_ED25519 06135 if (ssl->peerEd25519KeyPresent) { 06136 WOLFSSL_MSG("Doing ED25519 peer cert verify"); 06137 06138 args->sigData = (byte*)XMALLOC(MAX_SIG_DATA_SZ, ssl->heap, 06139 DYNAMIC_TYPE_SIGNATURE); 06140 if (args->sigData == NULL) { 06141 ERROR_OUT(MEMORY_E, exit_dcv); 06142 } 06143 06144 CreateSigData(ssl, args->sigData, &args->sigDataSz, 1); 06145 ret = 0; 06146 } 06147 #endif 06148 #ifdef HAVE_ED448 06149 if (ssl->peerEd448KeyPresent) { 06150 WOLFSSL_MSG("Doing ED448 peer cert verify"); 06151 06152 args->sigData = (byte*)XMALLOC(MAX_SIG_DATA_SZ, ssl->heap, 06153 DYNAMIC_TYPE_SIGNATURE); 06154 if (args->sigData == NULL) { 06155 ERROR_OUT(MEMORY_E, exit_dcv); 06156 } 06157 06158 CreateSigData(ssl, args->sigData, &args->sigDataSz, 1); 06159 ret = 0; 06160 } 06161 #endif 06162 06163 /* Advance state and proceed */ 06164 ssl->options.asyncState = TLS_ASYNC_DO; 06165 } /* case TLS_ASYNC_BUILD */ 06166 FALL_THROUGH; 06167 06168 case TLS_ASYNC_DO: 06169 { 06170 #ifndef NO_RSA 06171 if (ssl->peerRsaKey != NULL && ssl->peerRsaKeyPresent != 0) { 06172 WOLFSSL_MSG("Doing RSA peer cert verify"); 06173 06174 ret = RsaVerify(ssl, sig->buffer, (word32)sig->length, &args->output, 06175 args->sigAlgo, args->hashAlgo, ssl->peerRsaKey, 06176 #ifdef HAVE_PK_CALLBACKS 06177 &ssl->buffers.peerRsaKey 06178 #else 06179 NULL 06180 #endif 06181 ); 06182 if (ret >= 0) { 06183 args->sendSz = ret; 06184 ret = 0; 06185 } 06186 } 06187 #endif /* !NO_RSA */ 06188 #ifdef HAVE_ECC 06189 if (ssl->peerEccDsaKeyPresent) { 06190 WOLFSSL_MSG("Doing ECC peer cert verify"); 06191 06192 ret = EccVerify(ssl, input + args->idx, args->sz, 06193 args->sigData, args->sigDataSz, 06194 ssl->peerEccDsaKey, 06195 #ifdef HAVE_PK_CALLBACKS 06196 &ssl->buffers.peerEccDsaKey 06197 #else 06198 NULL 06199 #endif 06200 ); 06201 06202 if (ret >= 0) { 06203 FreeKey(ssl, DYNAMIC_TYPE_ECC, (void**)&ssl->peerEccDsaKey); 06204 ssl->peerEccDsaKeyPresent = 0; 06205 } 06206 } 06207 #endif /* HAVE_ECC */ 06208 #ifdef HAVE_ED25519 06209 if (ssl->peerEd25519KeyPresent) { 06210 WOLFSSL_MSG("Doing ED25519 peer cert verify"); 06211 06212 ret = Ed25519Verify(ssl, input + args->idx, args->sz, 06213 args->sigData, args->sigDataSz, 06214 ssl->peerEd25519Key, 06215 #ifdef HAVE_PK_CALLBACKS 06216 &ssl->buffers.peerEd25519Key 06217 #else 06218 NULL 06219 #endif 06220 ); 06221 06222 if (ret >= 0) { 06223 FreeKey(ssl, DYNAMIC_TYPE_ED25519, 06224 (void**)&ssl->peerEd25519Key); 06225 ssl->peerEd25519KeyPresent = 0; 06226 } 06227 } 06228 #endif 06229 #ifdef HAVE_ED448 06230 if (ssl->peerEd448KeyPresent) { 06231 WOLFSSL_MSG("Doing ED448 peer cert verify"); 06232 06233 ret = Ed448Verify(ssl, input + args->idx, args->sz, 06234 args->sigData, args->sigDataSz, 06235 ssl->peerEd448Key, 06236 #ifdef HAVE_PK_CALLBACKS 06237 &ssl->buffers.peerEd448Key 06238 #else 06239 NULL 06240 #endif 06241 ); 06242 06243 if (ret >= 0) { 06244 FreeKey(ssl, DYNAMIC_TYPE_ED448, 06245 (void**)&ssl->peerEd448Key); 06246 ssl->peerEd448KeyPresent = 0; 06247 } 06248 } 06249 #endif 06250 06251 /* Check for error */ 06252 if (ret != 0) { 06253 goto exit_dcv; 06254 } 06255 06256 /* Advance state and proceed */ 06257 ssl->options.asyncState = TLS_ASYNC_VERIFY; 06258 } /* case TLS_ASYNC_DO */ 06259 FALL_THROUGH; 06260 06261 case TLS_ASYNC_VERIFY: 06262 { 06263 #ifndef NO_RSA 06264 if (ssl->peerRsaKey != NULL && ssl->peerRsaKeyPresent != 0) { 06265 ret = CheckRSASignature(ssl, args->sigAlgo, args->hashAlgo, 06266 args->output, args->sendSz); 06267 if (ret != 0) 06268 goto exit_dcv; 06269 06270 FreeKey(ssl, DYNAMIC_TYPE_RSA, (void**)&ssl->peerRsaKey); 06271 ssl->peerRsaKeyPresent = 0; 06272 } 06273 #endif /* !NO_RSA */ 06274 06275 /* Advance state and proceed */ 06276 ssl->options.asyncState = TLS_ASYNC_FINALIZE; 06277 } /* case TLS_ASYNC_VERIFY */ 06278 FALL_THROUGH; 06279 06280 case TLS_ASYNC_FINALIZE: 06281 { 06282 ssl->options.havePeerVerify = 1; 06283 06284 /* Set final index */ 06285 args->idx += args->sz; 06286 *inOutIdx = args->idx; 06287 06288 /* Encryption is always on: add padding */ 06289 *inOutIdx += ssl->keys.padSz; 06290 06291 /* Advance state and proceed */ 06292 ssl->options.asyncState = TLS_ASYNC_END; 06293 } /* case TLS_ASYNC_FINALIZE */ 06294 06295 case TLS_ASYNC_END: 06296 { 06297 break; 06298 } 06299 default: 06300 ret = INPUT_CASE_ERROR; 06301 } /* switch(ssl->options.asyncState) */ 06302 06303 exit_dcv: 06304 06305 WOLFSSL_LEAVE("DoTls13CertificateVerify", ret); 06306 WOLFSSL_END(WC_FUNC_CERTIFICATE_VERIFY_DO); 06307 06308 #ifdef WOLFSSL_ASYNC_CRYPT 06309 /* Handle async operation */ 06310 if (ret == WC_PENDING_E) { 06311 /* Mark message as not received so it can process again */ 06312 ssl->msgsReceived.got_certificate_verify = 0; 06313 06314 return ret; 06315 } 06316 else 06317 #endif /* WOLFSSL_ASYNC_CRYPT */ 06318 if (ret != 0 && ret != INVALID_PARAMETER) 06319 SendAlert(ssl, alert_fatal, decrypt_error); 06320 06321 /* Final cleanup */ 06322 FreeDcv13Args(ssl, args); 06323 FreeKeyExchange(ssl); 06324 06325 return ret; 06326 } 06327 #endif /* !NO_RSA || HAVE_ECC */ 06328 06329 /* Parse and handle a TLS v1.3 Finished message. 06330 * 06331 * ssl The SSL/TLS object. 06332 * input The message buffer. 06333 * inOutIdx On entry, the index into the message buffer of Finished. 06334 * On exit, the index of byte after the Finished message and padding. 06335 * size Length of message data. 06336 * totalSz Length of remaining data in the message buffer. 06337 * sniff Indicates whether we are sniffing packets. 06338 * returns 0 on success and otherwise failure. 06339 */ 06340 static int DoTls13Finished(WOLFSSL* ssl, const byte* input, word32* inOutIdx, 06341 word32 size, word32 totalSz, int sniff) 06342 { 06343 int ret; 06344 word32 finishedSz = 0; 06345 byte* secret; 06346 byte mac[WC_MAX_DIGEST_SIZE]; 06347 06348 WOLFSSL_START(WC_FUNC_FINISHED_DO); 06349 WOLFSSL_ENTER("DoTls13Finished"); 06350 06351 /* check against totalSz */ 06352 if (*inOutIdx + size + ssl->keys.padSz > totalSz) 06353 return BUFFER_E; 06354 06355 if (ssl->options.handShakeDone) { 06356 ret = DeriveFinishedSecret(ssl, ssl->clientSecret, 06357 ssl->keys.client_write_MAC_secret); 06358 if (ret != 0) 06359 return ret; 06360 06361 secret = ssl->keys.client_write_MAC_secret; 06362 } 06363 else if (ssl->options.side == WOLFSSL_CLIENT_END) { 06364 /* All the handshake messages have been received to calculate 06365 * client and server finished keys. 06366 */ 06367 ret = DeriveFinishedSecret(ssl, ssl->clientSecret, 06368 ssl->keys.client_write_MAC_secret); 06369 if (ret != 0) 06370 return ret; 06371 06372 ret = DeriveFinishedSecret(ssl, ssl->serverSecret, 06373 ssl->keys.server_write_MAC_secret); 06374 if (ret != 0) 06375 return ret; 06376 06377 secret = ssl->keys.server_write_MAC_secret; 06378 } 06379 else 06380 secret = ssl->keys.client_write_MAC_secret; 06381 06382 ret = BuildTls13HandshakeHmac(ssl, secret, mac, &finishedSz); 06383 if (ret != 0) 06384 return ret; 06385 if (size != finishedSz) 06386 return BUFFER_ERROR; 06387 06388 #ifdef WOLFSSL_CALLBACKS 06389 if (ssl->hsInfoOn) AddPacketName(ssl, "Finished"); 06390 if (ssl->toInfoOn) AddLateName("Finished", &ssl->timeoutInfo); 06391 #endif 06392 06393 if (sniff == NO_SNIFF) { 06394 /* Actually check verify data. */ 06395 if (XMEMCMP(input + *inOutIdx, mac, size) != 0){ 06396 WOLFSSL_MSG("Verify finished error on hashes"); 06397 SendAlert(ssl, alert_fatal, decrypt_error); 06398 return VERIFY_FINISHED_ERROR; 06399 } 06400 } 06401 06402 /* Force input exhaustion at ProcessReply by consuming padSz. */ 06403 *inOutIdx += size + ssl->keys.padSz; 06404 06405 if (ssl->options.side == WOLFSSL_SERVER_END && 06406 !ssl->options.handShakeDone) { 06407 #ifdef WOLFSSL_EARLY_DATA 06408 if (ssl->earlyData != no_early_data) { 06409 if ((ret = DeriveTls13Keys(ssl, no_key, DECRYPT_SIDE_ONLY, 1)) != 0) 06410 return ret; 06411 } 06412 #endif 06413 /* Setup keys for application data messages from client. */ 06414 if ((ret = SetKeysSide(ssl, DECRYPT_SIDE_ONLY)) != 0) 06415 return ret; 06416 } 06417 06418 #ifndef NO_WOLFSSL_CLIENT 06419 if (ssl->options.side == WOLFSSL_CLIENT_END) 06420 ssl->options.serverState = SERVER_FINISHED_COMPLETE; 06421 #endif 06422 #ifndef NO_WOLFSSL_SERVER 06423 if (ssl->options.side == WOLFSSL_SERVER_END) { 06424 ssl->options.clientState = CLIENT_FINISHED_COMPLETE; 06425 ssl->options.handShakeState = HANDSHAKE_DONE; 06426 ssl->options.handShakeDone = 1; 06427 } 06428 #endif 06429 06430 WOLFSSL_LEAVE("DoTls13Finished", 0); 06431 WOLFSSL_END(WC_FUNC_FINISHED_DO); 06432 06433 return 0; 06434 } 06435 #endif /* NO_CERTS */ 06436 06437 /* Send the TLS v1.3 Finished message. 06438 * 06439 * ssl The SSL/TLS object. 06440 * returns 0 on success, otherwise failure. 06441 */ 06442 static int SendTls13Finished(WOLFSSL* ssl) 06443 { 06444 int sendSz; 06445 int finishedSz = ssl->specs.hash_size; 06446 byte* input; 06447 byte* output; 06448 int ret; 06449 int headerSz = HANDSHAKE_HEADER_SZ; 06450 int outputSz; 06451 byte* secret; 06452 06453 WOLFSSL_START(WC_FUNC_FINISHED_SEND); 06454 WOLFSSL_ENTER("SendTls13Finished"); 06455 06456 outputSz = WC_MAX_DIGEST_SIZE + DTLS_HANDSHAKE_HEADER_SZ + MAX_MSG_EXTRA; 06457 /* Check buffers are big enough and grow if needed. */ 06458 if ((ret = CheckAvailableSize(ssl, outputSz)) != 0) 06459 return ret; 06460 06461 /* get output buffer */ 06462 output = ssl->buffers.outputBuffer.buffer + 06463 ssl->buffers.outputBuffer.length; 06464 input = output + RECORD_HEADER_SZ; 06465 06466 AddTls13HandShakeHeader(input, finishedSz, 0, finishedSz, finished, ssl); 06467 06468 /* make finished hashes */ 06469 if (ssl->options.handShakeDone) { 06470 ret = DeriveFinishedSecret(ssl, ssl->clientSecret, 06471 ssl->keys.client_write_MAC_secret); 06472 if (ret != 0) 06473 return ret; 06474 06475 secret = ssl->keys.client_write_MAC_secret; 06476 } 06477 else if (ssl->options.side == WOLFSSL_CLIENT_END) 06478 secret = ssl->keys.client_write_MAC_secret; 06479 else { 06480 /* All the handshake messages have been done to calculate client and 06481 * server finished keys. 06482 */ 06483 ret = DeriveFinishedSecret(ssl, ssl->clientSecret, 06484 ssl->keys.client_write_MAC_secret); 06485 if (ret != 0) 06486 return ret; 06487 06488 ret = DeriveFinishedSecret(ssl, ssl->serverSecret, 06489 ssl->keys.server_write_MAC_secret); 06490 if (ret != 0) 06491 return ret; 06492 06493 secret = ssl->keys.server_write_MAC_secret; 06494 } 06495 ret = BuildTls13HandshakeHmac(ssl, secret, &input[headerSz], NULL); 06496 if (ret != 0) 06497 return ret; 06498 06499 /* This message is always encrypted. */ 06500 sendSz = BuildTls13Message(ssl, output, outputSz, input, 06501 headerSz + finishedSz, handshake, 1, 0, 0); 06502 if (sendSz < 0) 06503 return BUILD_MSG_ERROR; 06504 06505 #ifndef NO_SESSION_CACHE 06506 if (!ssl->options.resuming && (ssl->options.side == WOLFSSL_SERVER_END || 06507 (ssl->options.side == WOLFSSL_SERVER_END && ssl->arrays != NULL))) { 06508 AddSession(ssl); /* just try */ 06509 } 06510 #endif 06511 06512 #ifdef WOLFSSL_CALLBACKS 06513 if (ssl->hsInfoOn) AddPacketName(ssl, "Finished"); 06514 if (ssl->toInfoOn) { 06515 AddPacketInfo(ssl, "Finished", handshake, output, sendSz, 06516 WRITE_PROTO, ssl->heap); 06517 } 06518 #endif 06519 06520 ssl->buffers.outputBuffer.length += sendSz; 06521 06522 if (ssl->options.side == WOLFSSL_SERVER_END) { 06523 /* Can send application data now. */ 06524 if ((ret = DeriveMasterSecret(ssl)) != 0) 06525 return ret; 06526 #ifdef WOLFSSL_EARLY_DATA 06527 if ((ret = DeriveTls13Keys(ssl, traffic_key, ENCRYPT_SIDE_ONLY, 1)) 06528 != 0) { 06529 return ret; 06530 } 06531 if ((ret = DeriveTls13Keys(ssl, traffic_key, DECRYPT_SIDE_ONLY, 06532 ssl->earlyData == no_early_data)) != 0) { 06533 return ret; 06534 } 06535 #else 06536 if ((ret = DeriveTls13Keys(ssl, traffic_key, ENCRYPT_AND_DECRYPT_SIDE, 06537 1)) != 0) { 06538 return ret; 06539 } 06540 #endif 06541 if ((ret = SetKeysSide(ssl, ENCRYPT_SIDE_ONLY)) != 0) 06542 return ret; 06543 } 06544 06545 if (ssl->options.side == WOLFSSL_CLIENT_END && 06546 !ssl->options.handShakeDone) { 06547 #ifdef WOLFSSL_EARLY_DATA 06548 if (ssl->earlyData != no_early_data) { 06549 if ((ret = DeriveTls13Keys(ssl, no_key, ENCRYPT_AND_DECRYPT_SIDE, 06550 1)) != 0) { 06551 return ret; 06552 } 06553 } 06554 #endif 06555 /* Setup keys for application data messages. */ 06556 if ((ret = SetKeysSide(ssl, ENCRYPT_AND_DECRYPT_SIDE)) != 0) 06557 return ret; 06558 06559 #if defined(HAVE_SESSION_TICKET) || !defined(NO_PSK) 06560 ret = DeriveResumptionSecret(ssl, ssl->session.masterSecret); 06561 if (ret != 0) 06562 return ret; 06563 #endif 06564 } 06565 06566 #ifndef NO_WOLFSSL_CLIENT 06567 if (ssl->options.side == WOLFSSL_CLIENT_END) { 06568 ssl->options.clientState = CLIENT_FINISHED_COMPLETE; 06569 ssl->options.handShakeState = HANDSHAKE_DONE; 06570 ssl->options.handShakeDone = 1; 06571 } 06572 #endif 06573 #ifndef NO_WOLFSSL_SERVER 06574 if (ssl->options.side == WOLFSSL_SERVER_END) { 06575 ssl->options.serverState = SERVER_FINISHED_COMPLETE; 06576 } 06577 #endif 06578 06579 if ((ret = SendBuffered(ssl)) != 0) 06580 return ret; 06581 06582 WOLFSSL_LEAVE("SendTls13Finished", ret); 06583 WOLFSSL_END(WC_FUNC_FINISHED_SEND); 06584 06585 return ret; 06586 } 06587 06588 /* handle generation TLS v1.3 key_update (24) */ 06589 /* Send the TLS v1.3 KeyUpdate message. 06590 * 06591 * ssl The SSL/TLS object. 06592 * returns 0 on success, otherwise failure. 06593 */ 06594 static int SendTls13KeyUpdate(WOLFSSL* ssl) 06595 { 06596 int sendSz; 06597 byte* input; 06598 byte* output; 06599 int ret; 06600 int headerSz = HANDSHAKE_HEADER_SZ; 06601 int outputSz; 06602 word32 i = RECORD_HEADER_SZ + HANDSHAKE_HEADER_SZ; 06603 06604 WOLFSSL_START(WC_FUNC_KEY_UPDATE_SEND); 06605 WOLFSSL_ENTER("SendTls13KeyUpdate"); 06606 06607 outputSz = OPAQUE8_LEN + MAX_MSG_EXTRA; 06608 /* Check buffers are big enough and grow if needed. */ 06609 if ((ret = CheckAvailableSize(ssl, outputSz)) != 0) 06610 return ret; 06611 06612 /* get output buffer */ 06613 output = ssl->buffers.outputBuffer.buffer + 06614 ssl->buffers.outputBuffer.length; 06615 input = output + RECORD_HEADER_SZ; 06616 06617 AddTls13Headers(output, OPAQUE8_LEN, key_update, ssl); 06618 06619 /* If: 06620 * 1. I haven't sent a KeyUpdate requesting a response and 06621 * 2. This isn't responding to peer KeyUpdate requiring a response then, 06622 * I want a response. 06623 */ 06624 ssl->keys.updateResponseReq = output[i++] = 06625 !ssl->keys.updateResponseReq && !ssl->keys.keyUpdateRespond; 06626 /* Sent response, no longer need to respond. */ 06627 ssl->keys.keyUpdateRespond = 0; 06628 06629 /* This message is always encrypted. */ 06630 sendSz = BuildTls13Message(ssl, output, outputSz, input, 06631 headerSz + OPAQUE8_LEN, handshake, 0, 0, 0); 06632 if (sendSz < 0) 06633 return BUILD_MSG_ERROR; 06634 06635 #ifdef WOLFSSL_CALLBACKS 06636 if (ssl->hsInfoOn) AddPacketName(ssl, "KeyUpdate"); 06637 if (ssl->toInfoOn) { 06638 AddPacketInfo(ssl, "KeyUpdate", handshake, output, sendSz, 06639 WRITE_PROTO, ssl->heap); 06640 } 06641 #endif 06642 06643 ssl->buffers.outputBuffer.length += sendSz; 06644 06645 ret = SendBuffered(ssl); 06646 if (ret != 0 && ret != WANT_WRITE) 06647 return ret; 06648 06649 /* Future traffic uses new encryption keys. */ 06650 if ((ret = DeriveTls13Keys(ssl, update_traffic_key, ENCRYPT_SIDE_ONLY, 1)) 06651 != 0) 06652 return ret; 06653 if ((ret = SetKeysSide(ssl, ENCRYPT_SIDE_ONLY)) != 0) 06654 return ret; 06655 06656 WOLFSSL_LEAVE("SendTls13KeyUpdate", ret); 06657 WOLFSSL_END(WC_FUNC_KEY_UPDATE_SEND); 06658 06659 return ret; 06660 } 06661 06662 /* handle processing TLS v1.3 key_update (24) */ 06663 /* Parse and handle a TLS v1.3 KeyUpdate message. 06664 * 06665 * ssl The SSL/TLS object. 06666 * input The message buffer. 06667 * inOutIdx On entry, the index into the message buffer of Finished. 06668 * On exit, the index of byte after the Finished message and padding. 06669 * totalSz The length of the current handshake message. 06670 * returns 0 on success and otherwise failure. 06671 */ 06672 static int DoTls13KeyUpdate(WOLFSSL* ssl, const byte* input, word32* inOutIdx, 06673 word32 totalSz) 06674 { 06675 int ret; 06676 word32 i = *inOutIdx; 06677 06678 WOLFSSL_START(WC_FUNC_KEY_UPDATE_DO); 06679 WOLFSSL_ENTER("DoTls13KeyUpdate"); 06680 06681 /* check against totalSz */ 06682 if (OPAQUE8_LEN != totalSz) 06683 return BUFFER_E; 06684 06685 switch (input[i]) { 06686 case update_not_requested: 06687 /* This message in response to any outstanding request. */ 06688 ssl->keys.keyUpdateRespond = 0; 06689 ssl->keys.updateResponseReq = 0; 06690 break; 06691 case update_requested: 06692 /* New key update requiring a response. */ 06693 ssl->keys.keyUpdateRespond = 1; 06694 break; 06695 default: 06696 return INVALID_PARAMETER; 06697 } 06698 06699 /* Move index to byte after message. */ 06700 *inOutIdx += totalSz; 06701 /* Always encrypted. */ 06702 *inOutIdx += ssl->keys.padSz; 06703 06704 /* Future traffic uses new decryption keys. */ 06705 if ((ret = DeriveTls13Keys(ssl, update_traffic_key, DECRYPT_SIDE_ONLY, 1)) 06706 != 0) { 06707 return ret; 06708 } 06709 if ((ret = SetKeysSide(ssl, DECRYPT_SIDE_ONLY)) != 0) 06710 return ret; 06711 06712 if (ssl->keys.keyUpdateRespond) 06713 return SendTls13KeyUpdate(ssl); 06714 06715 WOLFSSL_LEAVE("DoTls13KeyUpdate", ret); 06716 WOLFSSL_END(WC_FUNC_KEY_UPDATE_DO); 06717 06718 return 0; 06719 } 06720 06721 #ifdef WOLFSSL_EARLY_DATA 06722 #ifndef NO_WOLFSSL_CLIENT 06723 /* Send the TLS v1.3 EndOfEarlyData message to indicate that there will be no 06724 * more early application data. 06725 * The encryption key now changes to the pre-calculated handshake key. 06726 * 06727 * ssl The SSL/TLS object. 06728 * returns 0 on success and otherwise failure. 06729 */ 06730 static int SendTls13EndOfEarlyData(WOLFSSL* ssl) 06731 { 06732 byte* output; 06733 int ret; 06734 int sendSz; 06735 word32 length; 06736 word32 idx = RECORD_HEADER_SZ + HANDSHAKE_HEADER_SZ; 06737 06738 WOLFSSL_START(WC_FUNC_END_OF_EARLY_DATA_SEND); 06739 WOLFSSL_ENTER("SendTls13EndOfEarlyData"); 06740 06741 length = 0; 06742 sendSz = idx + length + MAX_MSG_EXTRA; 06743 06744 /* Check buffers are big enough and grow if needed. */ 06745 if ((ret = CheckAvailableSize(ssl, sendSz)) != 0) 06746 return ret; 06747 06748 /* Get position in output buffer to write new message to. */ 06749 output = ssl->buffers.outputBuffer.buffer + 06750 ssl->buffers.outputBuffer.length; 06751 06752 /* Put the record and handshake headers on. */ 06753 AddTls13Headers(output, length, end_of_early_data, ssl); 06754 06755 /* This message is always encrypted. */ 06756 sendSz = BuildTls13Message(ssl, output, sendSz, output + RECORD_HEADER_SZ, 06757 idx - RECORD_HEADER_SZ, handshake, 1, 0, 0); 06758 if (sendSz < 0) 06759 return sendSz; 06760 06761 ssl->buffers.outputBuffer.length += sendSz; 06762 06763 if ((ret = SetKeysSide(ssl, ENCRYPT_SIDE_ONLY)) != 0) 06764 return ret; 06765 06766 if (!ssl->options.groupMessages) 06767 ret = SendBuffered(ssl); 06768 06769 WOLFSSL_LEAVE("SendTls13EndOfEarlyData", ret); 06770 WOLFSSL_END(WC_FUNC_END_OF_EARLY_DATA_SEND); 06771 06772 return ret; 06773 } 06774 #endif /* !NO_WOLFSSL_CLIENT */ 06775 06776 #ifndef NO_WOLFSSL_SERVER 06777 /* handle processing of TLS 1.3 end_of_early_data (5) */ 06778 /* Parse the TLS v1.3 EndOfEarlyData message that indicates that there will be 06779 * no more early application data. 06780 * The decryption key now changes to the pre-calculated handshake key. 06781 * 06782 * ssl The SSL/TLS object. 06783 * returns 0 on success and otherwise failure. 06784 */ 06785 static int DoTls13EndOfEarlyData(WOLFSSL* ssl, const byte* input, 06786 word32* inOutIdx, word32 size) 06787 { 06788 int ret; 06789 word32 begin = *inOutIdx; 06790 06791 (void)input; 06792 06793 WOLFSSL_START(WC_FUNC_END_OF_EARLY_DATA_DO); 06794 WOLFSSL_ENTER("DoTls13EndOfEarlyData"); 06795 06796 if ((*inOutIdx - begin) != size) 06797 return BUFFER_ERROR; 06798 06799 if (ssl->earlyData == no_early_data) { 06800 WOLFSSL_MSG("EndOfEarlyData received unexpectedly"); 06801 SendAlert(ssl, alert_fatal, unexpected_message); 06802 return OUT_OF_ORDER_E; 06803 } 06804 06805 ssl->earlyData = done_early_data; 06806 06807 /* Always encrypted. */ 06808 *inOutIdx += ssl->keys.padSz; 06809 06810 ret = SetKeysSide(ssl, DECRYPT_SIDE_ONLY); 06811 06812 WOLFSSL_LEAVE("DoTls13EndOfEarlyData", ret); 06813 WOLFSSL_END(WC_FUNC_END_OF_EARLY_DATA_DO); 06814 06815 return ret; 06816 } 06817 #endif /* !NO_WOLFSSL_SERVER */ 06818 #endif /* WOLFSSL_EARLY_DATA */ 06819 06820 #ifndef NO_WOLFSSL_CLIENT 06821 /* Handle a New Session Ticket handshake message. 06822 * Message contains the information required to perform resumption. 06823 * 06824 * ssl The SSL/TLS object. 06825 * input The message buffer. 06826 * inOutIdx On entry, the index into the message buffer of Finished. 06827 * On exit, the index of byte after the Finished message and padding. 06828 * size The length of the current handshake message. 06829 * returns 0 on success, otherwise failure. 06830 */ 06831 static int DoTls13NewSessionTicket(WOLFSSL* ssl, const byte* input, 06832 word32* inOutIdx, word32 size) 06833 { 06834 #ifdef HAVE_SESSION_TICKET 06835 int ret; 06836 word32 begin = *inOutIdx; 06837 word32 lifetime; 06838 word32 ageAdd; 06839 word16 length; 06840 word32 now; 06841 #ifndef WOLFSSL_TLS13_DRAFT_18 06842 const byte* nonce; 06843 byte nonceLength; 06844 #endif 06845 06846 WOLFSSL_START(WC_FUNC_NEW_SESSION_TICKET_DO); 06847 WOLFSSL_ENTER("DoTls13NewSessionTicket"); 06848 06849 /* Lifetime hint. */ 06850 if ((*inOutIdx - begin) + SESSION_HINT_SZ > size) 06851 return BUFFER_ERROR; 06852 ato32(input + *inOutIdx, &lifetime); 06853 *inOutIdx += SESSION_HINT_SZ; 06854 if (lifetime > MAX_LIFETIME) 06855 return SERVER_HINT_ERROR; 06856 06857 /* Age add. */ 06858 if ((*inOutIdx - begin) + SESSION_ADD_SZ > size) 06859 return BUFFER_ERROR; 06860 ato32(input + *inOutIdx, &ageAdd); 06861 *inOutIdx += SESSION_ADD_SZ; 06862 06863 #ifndef WOLFSSL_TLS13_DRAFT_18 06864 /* Ticket nonce. */ 06865 if ((*inOutIdx - begin) + 1 > size) 06866 return BUFFER_ERROR; 06867 nonceLength = input[*inOutIdx]; 06868 if (nonceLength > MAX_TICKET_NONCE_SZ) { 06869 WOLFSSL_MSG("Nonce length not supported"); 06870 return INVALID_PARAMETER; 06871 } 06872 *inOutIdx += 1; 06873 if ((*inOutIdx - begin) + nonceLength > size) 06874 return BUFFER_ERROR; 06875 nonce = input + *inOutIdx; 06876 *inOutIdx += nonceLength; 06877 #endif 06878 06879 /* Ticket length. */ 06880 if ((*inOutIdx - begin) + LENGTH_SZ > size) 06881 return BUFFER_ERROR; 06882 ato16(input + *inOutIdx, &length); 06883 *inOutIdx += LENGTH_SZ; 06884 if ((*inOutIdx - begin) + length > size) 06885 return BUFFER_ERROR; 06886 06887 if ((ret = SetTicket(ssl, input + *inOutIdx, length)) != 0) 06888 return ret; 06889 *inOutIdx += length; 06890 06891 now = TimeNowInMilliseconds(); 06892 if (now == (word32)GETTIME_ERROR) 06893 return now; 06894 /* Copy in ticket data (server identity). */ 06895 ssl->timeout = lifetime; 06896 ssl->session.timeout = lifetime; 06897 ssl->session.cipherSuite0 = ssl->options.cipherSuite0; 06898 ssl->session.cipherSuite = ssl->options.cipherSuite; 06899 ssl->session.ticketSeen = now; 06900 ssl->session.ticketAdd = ageAdd; 06901 #ifdef WOLFSSL_EARLY_DATA 06902 ssl->session.maxEarlyDataSz = ssl->options.maxEarlyDataSz; 06903 #endif 06904 #ifndef WOLFSSL_TLS13_DRAFT_18 06905 ssl->session.ticketNonce.len = nonceLength; 06906 if (nonceLength > 0) 06907 XMEMCPY(&ssl->session.ticketNonce.data, nonce, nonceLength); 06908 #endif 06909 ssl->session.namedGroup = ssl->namedGroup; 06910 06911 if ((*inOutIdx - begin) + EXTS_SZ > size) 06912 return BUFFER_ERROR; 06913 ato16(input + *inOutIdx, &length); 06914 *inOutIdx += EXTS_SZ; 06915 if ((*inOutIdx - begin) + length != size) 06916 return BUFFER_ERROR; 06917 #ifdef WOLFSSL_EARLY_DATA 06918 ret = TLSX_Parse(ssl, (byte *)input + (*inOutIdx), length, session_ticket, 06919 NULL); 06920 if (ret != 0) 06921 return ret; 06922 #endif 06923 *inOutIdx += length; 06924 06925 #ifndef NO_SESSION_CACHE 06926 AddSession(ssl); 06927 #endif 06928 06929 /* Always encrypted. */ 06930 *inOutIdx += ssl->keys.padSz; 06931 06932 ssl->expect_session_ticket = 0; 06933 #else 06934 (void)ssl; 06935 (void)input; 06936 06937 WOLFSSL_ENTER("DoTls13NewSessionTicket"); 06938 06939 *inOutIdx += size + ssl->keys.padSz; 06940 #endif /* HAVE_SESSION_TICKET */ 06941 06942 WOLFSSL_LEAVE("DoTls13NewSessionTicket", 0); 06943 WOLFSSL_END(WC_FUNC_NEW_SESSION_TICKET_DO); 06944 06945 return 0; 06946 } 06947 #endif /* NO_WOLFSSL_CLIENT */ 06948 06949 #ifndef NO_WOLFSSL_SERVER 06950 #ifdef HAVE_SESSION_TICKET 06951 06952 #ifdef WOLFSSL_TLS13_TICKET_BEFORE_FINISHED 06953 /* Offset of the MAC size in the finished message. */ 06954 #define FINISHED_MSG_SIZE_OFFSET 3 06955 06956 /* Calculate the resumption secret which includes the unseen client finished 06957 * message. 06958 * 06959 * ssl The SSL/TLS object. 06960 * returns 0 on success, otherwise failure. 06961 */ 06962 static int ExpectedResumptionSecret(WOLFSSL* ssl) 06963 { 06964 int ret; 06965 word32 finishedSz = 0; 06966 byte mac[WC_MAX_DIGEST_SIZE]; 06967 Digest digest; 06968 static byte header[] = { 0x14, 0x00, 0x00, 0x00 }; 06969 06970 /* Copy the running hash so we can restore it after. */ 06971 switch (ssl->specs.mac_algorithm) { 06972 #ifndef NO_SHA256 06973 case sha256_mac: 06974 ret = wc_Sha256Copy(&ssl->hsHashes->hashSha256, &digest.sha256); 06975 if (ret != 0) 06976 return ret; 06977 break; 06978 #endif 06979 #ifdef WOLFSSL_SHA384 06980 case sha384_mac: 06981 ret = wc_Sha384Copy(&ssl->hsHashes->hashSha384, &digest.sha384); 06982 if (ret != 0) 06983 return ret; 06984 break; 06985 #endif 06986 #ifdef WOLFSSL_TLS13_SHA512 06987 case sha512_mac: 06988 ret = wc_Sha512Copy(&ssl->hsHashes->hashSha512, &digest.sha512); 06989 if (ret != 0) 06990 return ret; 06991 break; 06992 #endif 06993 } 06994 06995 /* Generate the Client's Finished message and hash it. */ 06996 ret = BuildTls13HandshakeHmac(ssl, ssl->keys.client_write_MAC_secret, mac, 06997 &finishedSz); 06998 if (ret != 0) 06999 return ret; 07000 header[FINISHED_MSG_SIZE_OFFSET] = finishedSz; 07001 #ifdef WOLFSSL_EARLY_DATA 07002 if (ssl->earlyData != no_early_data) { 07003 static byte endOfEarlyData[] = { 0x05, 0x00, 0x00, 0x00 }; 07004 ret = HashInputRaw(ssl, endOfEarlyData, sizeof(endOfEarlyData)); 07005 if (ret != 0) 07006 return ret; 07007 } 07008 #endif 07009 if ((ret = HashInputRaw(ssl, header, sizeof(header))) != 0) 07010 return ret; 07011 if ((ret = HashInputRaw(ssl, mac, finishedSz)) != 0) 07012 return ret; 07013 07014 if ((ret = DeriveResumptionSecret(ssl, ssl->session.masterSecret)) != 0) 07015 return ret; 07016 07017 /* Restore the hash inline with currently seen messages. */ 07018 switch (ssl->specs.mac_algorithm) { 07019 #ifndef NO_SHA256 07020 case sha256_mac: 07021 ret = wc_Sha256Copy(&digest.sha256, &ssl->hsHashes->hashSha256); 07022 if (ret != 0) 07023 return ret; 07024 break; 07025 #endif 07026 #ifdef WOLFSSL_SHA384 07027 case sha384_mac: 07028 ret = wc_Sha384Copy(&digest.sha384, &ssl->hsHashes->hashSha384); 07029 if (ret != 0) 07030 return ret; 07031 break; 07032 #endif 07033 #ifdef WOLFSSL_TLS13_SHA512 07034 case sha512_mac: 07035 ret = wc_Sha512Copy(&digest.sha512, &ssl->hsHashes->hashSha384); 07036 if (ret != 0) 07037 return ret; 07038 break; 07039 #endif 07040 } 07041 07042 return ret; 07043 } 07044 #endif 07045 07046 /* Send New Session Ticket handshake message. 07047 * Message contains the information required to perform resumption. 07048 * 07049 * ssl The SSL/TLS object. 07050 * returns 0 on success, otherwise failure. 07051 */ 07052 static int SendTls13NewSessionTicket(WOLFSSL* ssl) 07053 { 07054 byte* output; 07055 int ret; 07056 int sendSz; 07057 word16 extSz; 07058 word32 length; 07059 word32 idx = RECORD_HEADER_SZ + HANDSHAKE_HEADER_SZ; 07060 07061 WOLFSSL_START(WC_FUNC_NEW_SESSION_TICKET_SEND); 07062 WOLFSSL_ENTER("SendTls13NewSessionTicket"); 07063 07064 #ifdef WOLFSSL_TLS13_TICKET_BEFORE_FINISHED 07065 if (!ssl->msgsReceived.got_finished) { 07066 if ((ret = ExpectedResumptionSecret(ssl)) != 0) 07067 return ret; 07068 } 07069 #endif 07070 07071 #ifndef WOLFSSL_TLS13_DRAFT_18 07072 /* Start ticket nonce at 0 and go up to 255. */ 07073 if (ssl->session.ticketNonce.len == 0) { 07074 ssl->session.ticketNonce.len = DEF_TICKET_NONCE_SZ; 07075 ssl->session.ticketNonce.data[0] = 0; 07076 } 07077 else 07078 ssl->session.ticketNonce.data[0]++; 07079 #endif 07080 07081 if (!ssl->options.noTicketTls13) { 07082 if ((ret = CreateTicket(ssl)) != 0) 07083 return ret; 07084 } 07085 07086 #ifdef WOLFSSL_EARLY_DATA 07087 ssl->session.maxEarlyDataSz = ssl->options.maxEarlyDataSz; 07088 if (ssl->session.maxEarlyDataSz > 0) 07089 TLSX_EarlyData_Use(ssl, ssl->session.maxEarlyDataSz); 07090 extSz = 0; 07091 ret = TLSX_GetResponseSize(ssl, session_ticket, &extSz); 07092 if (ret != 0) 07093 return ret; 07094 #else 07095 extSz = EXTS_SZ; 07096 #endif 07097 07098 /* Lifetime | Age Add | Ticket | Extensions */ 07099 length = SESSION_HINT_SZ + SESSION_ADD_SZ + LENGTH_SZ + 07100 ssl->session.ticketLen + extSz; 07101 #ifndef WOLFSSL_TLS13_DRAFT_18 07102 /* Nonce */ 07103 length += TICKET_NONCE_LEN_SZ + DEF_TICKET_NONCE_SZ; 07104 #endif 07105 sendSz = idx + length + MAX_MSG_EXTRA; 07106 07107 /* Check buffers are big enough and grow if needed. */ 07108 if ((ret = CheckAvailableSize(ssl, sendSz)) != 0) 07109 return ret; 07110 07111 /* Get position in output buffer to write new message to. */ 07112 output = ssl->buffers.outputBuffer.buffer + 07113 ssl->buffers.outputBuffer.length; 07114 07115 /* Put the record and handshake headers on. */ 07116 AddTls13Headers(output, length, session_ticket, ssl); 07117 07118 /* Lifetime hint */ 07119 c32toa(ssl->ctx->ticketHint, output + idx); 07120 idx += SESSION_HINT_SZ; 07121 /* Age add - obfuscator */ 07122 c32toa(ssl->session.ticketAdd, output + idx); 07123 idx += SESSION_ADD_SZ; 07124 07125 #ifndef WOLFSSL_TLS13_DRAFT_18 07126 output[idx++] = ssl->session.ticketNonce.len; 07127 output[idx++] = ssl->session.ticketNonce.data[0]; 07128 #endif 07129 07130 /* length */ 07131 c16toa(ssl->session.ticketLen, output + idx); 07132 idx += LENGTH_SZ; 07133 /* ticket */ 07134 XMEMCPY(output + idx, ssl->session.ticket, ssl->session.ticketLen); 07135 idx += ssl->session.ticketLen; 07136 07137 #ifdef WOLFSSL_EARLY_DATA 07138 extSz = 0; 07139 ret = TLSX_WriteResponse(ssl, output + idx, session_ticket, &extSz); 07140 if (ret != 0) 07141 return ret; 07142 idx += extSz; 07143 #else 07144 /* No extension support - empty extensions. */ 07145 c16toa(0, output + idx); 07146 idx += EXTS_SZ; 07147 #endif 07148 07149 ssl->options.haveSessionId = 1; 07150 07151 #ifndef NO_SESSION_CACHE 07152 AddSession(ssl); 07153 #endif 07154 07155 /* This message is always encrypted. */ 07156 sendSz = BuildTls13Message(ssl, output, sendSz, output + RECORD_HEADER_SZ, 07157 idx - RECORD_HEADER_SZ, handshake, 0, 0, 0); 07158 if (sendSz < 0) 07159 return sendSz; 07160 07161 ssl->buffers.outputBuffer.length += sendSz; 07162 07163 if (!ssl->options.groupMessages) 07164 ret = SendBuffered(ssl); 07165 07166 WOLFSSL_LEAVE("SendTls13NewSessionTicket", 0); 07167 WOLFSSL_END(WC_FUNC_NEW_SESSION_TICKET_SEND); 07168 07169 return ret; 07170 } 07171 #endif /* HAVE_SESSION_TICKET */ 07172 #endif /* NO_WOLFSSL_SERVER */ 07173 07174 /* Make sure no duplicates, no fast forward, or other problems 07175 * 07176 * ssl The SSL/TLS object. 07177 * type Type of handshake message received. 07178 * returns 0 on success, otherwise failure. 07179 */ 07180 static int SanityCheckTls13MsgReceived(WOLFSSL* ssl, byte type) 07181 { 07182 /* verify not a duplicate, mark received, check state */ 07183 switch (type) { 07184 07185 #ifndef NO_WOLFSSL_SERVER 07186 case client_hello: 07187 #ifndef NO_WOLFSSL_CLIENT 07188 if (ssl->options.side == WOLFSSL_CLIENT_END) { 07189 WOLFSSL_MSG("ClientHello received by client"); 07190 return OUT_OF_ORDER_E; 07191 } 07192 #endif 07193 if (ssl->options.clientState >= CLIENT_HELLO_COMPLETE) { 07194 WOLFSSL_MSG("ClientHello received out of order"); 07195 return OUT_OF_ORDER_E; 07196 } 07197 if (ssl->msgsReceived.got_client_hello == 2) { 07198 WOLFSSL_MSG("Too many ClientHello received"); 07199 return DUPLICATE_MSG_E; 07200 } 07201 ssl->msgsReceived.got_client_hello++; 07202 07203 break; 07204 #endif 07205 07206 #ifndef NO_WOLFSSL_CLIENT 07207 case server_hello: 07208 #ifndef NO_WOLFSSL_SERVER 07209 if (ssl->options.side == WOLFSSL_SERVER_END) { 07210 WOLFSSL_MSG("ServerHello received by server"); 07211 return OUT_OF_ORDER_E; 07212 } 07213 #endif 07214 #ifdef WOLFSSL_TLS13_DRAFT_18 07215 if (ssl->msgsReceived.got_server_hello) { 07216 WOLFSSL_MSG("Duplicate ServerHello received"); 07217 return DUPLICATE_MSG_E; 07218 } 07219 ssl->msgsReceived.got_server_hello = 1; 07220 #else 07221 if (ssl->msgsReceived.got_server_hello == 2) { 07222 WOLFSSL_MSG("Duplicate ServerHello received"); 07223 return DUPLICATE_MSG_E; 07224 } 07225 ssl->msgsReceived.got_server_hello++; 07226 #endif 07227 07228 break; 07229 #endif 07230 07231 #ifndef NO_WOLFSSL_CLIENT 07232 case session_ticket: 07233 #ifndef NO_WOLFSSL_SERVER 07234 if (ssl->options.side == WOLFSSL_SERVER_END) { 07235 WOLFSSL_MSG("NewSessionTicket received by server"); 07236 return OUT_OF_ORDER_E; 07237 } 07238 #endif 07239 if (ssl->options.clientState < CLIENT_FINISHED_COMPLETE) { 07240 WOLFSSL_MSG("NewSessionTicket received out of order"); 07241 return OUT_OF_ORDER_E; 07242 } 07243 ssl->msgsReceived.got_session_ticket = 1; 07244 07245 break; 07246 #endif 07247 07248 #ifndef NO_WOLFSSL_SERVER 07249 #ifdef WOLFSSL_EARLY_DATA 07250 case end_of_early_data: 07251 #ifndef NO_WOLFSSL_CLIENT 07252 if (ssl->options.side == WOLFSSL_CLIENT_END) { 07253 WOLFSSL_MSG("EndOfEarlyData received by client"); 07254 return OUT_OF_ORDER_E; 07255 } 07256 #endif 07257 if (ssl->options.serverState < SERVER_FINISHED_COMPLETE) { 07258 WOLFSSL_MSG("EndOfEarlyData received out of order"); 07259 return OUT_OF_ORDER_E; 07260 } 07261 if (ssl->options.clientState >= CLIENT_FINISHED_COMPLETE) { 07262 WOLFSSL_MSG("EndOfEarlyData received out of order"); 07263 return OUT_OF_ORDER_E; 07264 } 07265 if (ssl->msgsReceived.got_end_of_early_data == 1) { 07266 WOLFSSL_MSG("Too many EndOfEarlyData received"); 07267 return DUPLICATE_MSG_E; 07268 } 07269 ssl->msgsReceived.got_end_of_early_data++; 07270 07271 break; 07272 #endif 07273 #endif 07274 07275 #ifdef WOLFSSL_TLS13_DRAFT_18 07276 #ifndef NO_WOLFSSL_CLIENT 07277 case hello_retry_request: 07278 #ifndef NO_WOLFSSL_SERVER 07279 if (ssl->options.side == WOLFSSL_SERVER_END) { 07280 WOLFSSL_MSG("HelloRetryRequest received by server"); 07281 return OUT_OF_ORDER_E; 07282 } 07283 #endif 07284 if (ssl->options.clientState > CLIENT_FINISHED_COMPLETE) { 07285 WOLFSSL_MSG("HelloRetryRequest received out of order"); 07286 return OUT_OF_ORDER_E; 07287 } 07288 if (ssl->msgsReceived.got_hello_retry_request) { 07289 WOLFSSL_MSG("Duplicate HelloRetryRequest received"); 07290 return DUPLICATE_MSG_E; 07291 } 07292 ssl->msgsReceived.got_hello_retry_request = 1; 07293 07294 break; 07295 #endif 07296 #endif 07297 07298 #ifndef NO_WOLFSSL_CLIENT 07299 case encrypted_extensions: 07300 #ifndef NO_WOLFSSL_SERVER 07301 if (ssl->options.side == WOLFSSL_SERVER_END) { 07302 WOLFSSL_MSG("EncryptedExtensions received by server"); 07303 return OUT_OF_ORDER_E; 07304 } 07305 #endif 07306 if (ssl->options.serverState != SERVER_HELLO_COMPLETE) { 07307 WOLFSSL_MSG("EncryptedExtensions received out of order"); 07308 return OUT_OF_ORDER_E; 07309 } 07310 if (ssl->msgsReceived.got_encrypted_extensions) { 07311 WOLFSSL_MSG("Duplicate EncryptedExtensions received"); 07312 return DUPLICATE_MSG_E; 07313 } 07314 ssl->msgsReceived.got_encrypted_extensions = 1; 07315 07316 break; 07317 #endif 07318 07319 case certificate: 07320 #ifndef NO_WOLFSSL_CLIENT 07321 if (ssl->options.side == WOLFSSL_CLIENT_END && 07322 ssl->options.serverState != 07323 SERVER_ENCRYPTED_EXTENSIONS_COMPLETE) { 07324 WOLFSSL_MSG("Certificate received out of order - Client"); 07325 return OUT_OF_ORDER_E; 07326 } 07327 #if defined(HAVE_SESSION_TICKET) || !defined(NO_PSK) 07328 /* Server's authenticating with PSK must not send this. */ 07329 if (ssl->options.side == WOLFSSL_CLIENT_END && 07330 ssl->options.serverState == SERVER_CERT_COMPLETE && 07331 ssl->arrays->psk_keySz != 0) { 07332 WOLFSSL_MSG("Certificate received while using PSK"); 07333 return SANITY_MSG_E; 07334 } 07335 #endif 07336 #endif 07337 #ifndef NO_WOLFSSL_SERVER 07338 if (ssl->options.side == WOLFSSL_SERVER_END && 07339 ssl->options.serverState < SERVER_FINISHED_COMPLETE) { 07340 WOLFSSL_MSG("Certificate received out of order - Server"); 07341 return OUT_OF_ORDER_E; 07342 } 07343 #endif 07344 if (ssl->msgsReceived.got_certificate) { 07345 WOLFSSL_MSG("Duplicate Certificate received"); 07346 return DUPLICATE_MSG_E; 07347 } 07348 ssl->msgsReceived.got_certificate = 1; 07349 07350 break; 07351 07352 #ifndef NO_WOLFSSL_CLIENT 07353 case certificate_request: 07354 #ifndef NO_WOLFSSL_SERVER 07355 if (ssl->options.side == WOLFSSL_SERVER_END) { 07356 WOLFSSL_MSG("CertificateRequest received by server"); 07357 return OUT_OF_ORDER_E; 07358 } 07359 #endif 07360 #ifndef WOLFSSL_POST_HANDSHAKE_AUTH 07361 if (ssl->options.serverState != 07362 SERVER_ENCRYPTED_EXTENSIONS_COMPLETE) { 07363 WOLFSSL_MSG("CertificateRequest received out of order"); 07364 return OUT_OF_ORDER_E; 07365 } 07366 #else 07367 if (ssl->options.serverState != 07368 SERVER_ENCRYPTED_EXTENSIONS_COMPLETE && 07369 (ssl->options.serverState != SERVER_FINISHED_COMPLETE || 07370 ssl->options.clientState != CLIENT_FINISHED_COMPLETE)) { 07371 WOLFSSL_MSG("CertificateRequest received out of order"); 07372 return OUT_OF_ORDER_E; 07373 } 07374 #endif 07375 #if defined(HAVE_SESSION_TICKET) || !defined(NO_PSK) 07376 /* Server's authenticating with PSK must not send this. */ 07377 if (ssl->options.serverState == 07378 SERVER_ENCRYPTED_EXTENSIONS_COMPLETE && 07379 ssl->arrays->psk_keySz != 0) { 07380 WOLFSSL_MSG("CertificateRequset received while using PSK"); 07381 return SANITY_MSG_E; 07382 } 07383 #endif 07384 #ifndef WOLFSSL_POST_HANDSHAKE_AUTH 07385 if (ssl->msgsReceived.got_certificate_request) { 07386 WOLFSSL_MSG("Duplicate CertificateRequest received"); 07387 return DUPLICATE_MSG_E; 07388 } 07389 #endif 07390 ssl->msgsReceived.got_certificate_request = 1; 07391 07392 break; 07393 #endif 07394 07395 case certificate_verify: 07396 #ifndef NO_WOLFSSL_CLIENT 07397 if (ssl->options.side == WOLFSSL_CLIENT_END) { 07398 if (ssl->options.serverState != SERVER_CERT_COMPLETE) { 07399 WOLFSSL_MSG("No Cert before CertVerify"); 07400 return OUT_OF_ORDER_E; 07401 } 07402 #if defined(HAVE_SESSION_TICKET) || !defined(NO_PSK) 07403 /* Server's authenticating with PSK must not send this. */ 07404 if (ssl->options.serverState == SERVER_CERT_COMPLETE && 07405 ssl->arrays->psk_keySz != 0) { 07406 WOLFSSL_MSG("CertificateVerify received while using PSK"); 07407 return SANITY_MSG_E; 07408 } 07409 #endif 07410 } 07411 #endif 07412 #ifndef NO_WOLFSSL_SERVER 07413 if (ssl->options.side == WOLFSSL_SERVER_END) { 07414 if (ssl->options.serverState < SERVER_FINISHED_COMPLETE) { 07415 WOLFSSL_MSG("CertificateVerify received out of order"); 07416 return OUT_OF_ORDER_E; 07417 } 07418 if (ssl->options.clientState < CLIENT_HELLO_COMPLETE) { 07419 WOLFSSL_MSG("CertificateVerify before ClientHello done"); 07420 return OUT_OF_ORDER_E; 07421 } 07422 if (!ssl->msgsReceived.got_certificate) { 07423 WOLFSSL_MSG("No Cert before CertificateVerify"); 07424 return OUT_OF_ORDER_E; 07425 } 07426 } 07427 #endif 07428 if (ssl->msgsReceived.got_certificate_verify) { 07429 WOLFSSL_MSG("Duplicate CertificateVerify received"); 07430 return DUPLICATE_MSG_E; 07431 } 07432 ssl->msgsReceived.got_certificate_verify = 1; 07433 07434 break; 07435 07436 case finished: 07437 #ifndef NO_WOLFSSL_CLIENT 07438 if (ssl->options.side == WOLFSSL_CLIENT_END) { 07439 if (ssl->options.clientState < CLIENT_HELLO_COMPLETE) { 07440 WOLFSSL_MSG("Finished received out of order"); 07441 return OUT_OF_ORDER_E; 07442 } 07443 if (ssl->options.serverState < 07444 SERVER_ENCRYPTED_EXTENSIONS_COMPLETE) { 07445 WOLFSSL_MSG("Finished received out of order"); 07446 return OUT_OF_ORDER_E; 07447 } 07448 } 07449 #endif 07450 #ifndef NO_WOLFSSL_SERVER 07451 if (ssl->options.side == WOLFSSL_SERVER_END) { 07452 if (ssl->options.serverState < SERVER_FINISHED_COMPLETE) { 07453 WOLFSSL_MSG("Finished received out of order"); 07454 return OUT_OF_ORDER_E; 07455 } 07456 if (ssl->options.clientState < CLIENT_HELLO_COMPLETE) { 07457 WOLFSSL_MSG("Finished received out of order"); 07458 return OUT_OF_ORDER_E; 07459 } 07460 #ifdef WOLFSSL_EARLY_DATA 07461 if (ssl->earlyData == process_early_data) { 07462 return OUT_OF_ORDER_E; 07463 } 07464 #endif 07465 } 07466 #endif 07467 if (ssl->msgsReceived.got_finished) { 07468 WOLFSSL_MSG("Duplicate Finished received"); 07469 return DUPLICATE_MSG_E; 07470 } 07471 ssl->msgsReceived.got_finished = 1; 07472 07473 break; 07474 07475 case key_update: 07476 if (!ssl->msgsReceived.got_finished) { 07477 WOLFSSL_MSG("No KeyUpdate before Finished"); 07478 return OUT_OF_ORDER_E; 07479 } 07480 break; 07481 07482 default: 07483 WOLFSSL_MSG("Unknown message type"); 07484 return SANITY_MSG_E; 07485 } 07486 07487 return 0; 07488 } 07489 07490 /* Handle a type of handshake message that has been received. 07491 * 07492 * ssl The SSL/TLS object. 07493 * input The message buffer. 07494 * inOutIdx On entry, the index into the buffer of the current message. 07495 * On exit, the index into the buffer of the next message. 07496 * size The length of the current handshake message. 07497 * totalSz Length of remaining data in the message buffer. 07498 * returns 0 on success and otherwise failure. 07499 */ 07500 int DoTls13HandShakeMsgType(WOLFSSL* ssl, byte* input, word32* inOutIdx, 07501 byte type, word32 size, word32 totalSz) 07502 { 07503 int ret = 0; 07504 word32 inIdx = *inOutIdx; 07505 07506 (void)totalSz; 07507 07508 WOLFSSL_ENTER("DoTls13HandShakeMsgType"); 07509 07510 /* make sure we can read the message */ 07511 if (*inOutIdx + size > totalSz) 07512 return INCOMPLETE_DATA; 07513 07514 /* sanity check msg received */ 07515 if ((ret = SanityCheckTls13MsgReceived(ssl, type)) != 0) { 07516 WOLFSSL_MSG("Sanity Check on handshake message type received failed"); 07517 SendAlert(ssl, alert_fatal, unexpected_message); 07518 return ret; 07519 } 07520 07521 #ifdef WOLFSSL_CALLBACKS 07522 /* add name later, add on record and handshake header part back on */ 07523 if (ssl->toInfoOn) { 07524 int add = RECORD_HEADER_SZ + HANDSHAKE_HEADER_SZ; 07525 AddPacketInfo(ssl, 0, handshake, input + *inOutIdx - add, 07526 size + add, READ_PROTO, ssl->heap); 07527 AddLateRecordHeader(&ssl->curRL, &ssl->timeoutInfo); 07528 } 07529 #endif 07530 07531 if (ssl->options.handShakeState == HANDSHAKE_DONE && 07532 type != session_ticket && type != certificate_request && 07533 type != certificate && type != key_update) { 07534 WOLFSSL_MSG("HandShake message after handshake complete"); 07535 SendAlert(ssl, alert_fatal, unexpected_message); 07536 return OUT_OF_ORDER_E; 07537 } 07538 07539 if (ssl->options.side == WOLFSSL_CLIENT_END && 07540 ssl->options.serverState == NULL_STATE && 07541 type != server_hello && type != hello_retry_request) { 07542 WOLFSSL_MSG("First server message not server hello"); 07543 SendAlert(ssl, alert_fatal, unexpected_message); 07544 return OUT_OF_ORDER_E; 07545 } 07546 07547 if (ssl->options.side == WOLFSSL_SERVER_END && 07548 ssl->options.clientState == NULL_STATE && type != client_hello) { 07549 WOLFSSL_MSG("First client message not client hello"); 07550 SendAlert(ssl, alert_fatal, unexpected_message); 07551 return OUT_OF_ORDER_E; 07552 } 07553 07554 /* above checks handshake state */ 07555 switch (type) { 07556 #ifndef NO_WOLFSSL_CLIENT 07557 /* Messages only received by client. */ 07558 #ifdef WOLFSSL_TLS13_DRAFT_18 07559 case hello_retry_request: 07560 WOLFSSL_MSG("processing hello retry request"); 07561 ret = DoTls13HelloRetryRequest(ssl, input, inOutIdx, size); 07562 break; 07563 #endif 07564 07565 case server_hello: 07566 WOLFSSL_MSG("processing server hello"); 07567 ret = DoTls13ServerHello(ssl, input, inOutIdx, size, &type); 07568 #if !defined(WOLFSSL_NO_CLIENT_AUTH) && \ 07569 ((defined(HAVE_ED25519) && !defined(NO_ED25519_CLIENT_AUTH)) || \ 07570 (defined(HAVE_ED448) && !defined(NO_ED448_CLIENT_AUTH))) 07571 if (ssl->options.resuming || !IsAtLeastTLSv1_2(ssl) || 07572 IsAtLeastTLSv1_3(ssl->version)) { 07573 ssl->options.cacheMessages = 0; 07574 if (ssl->hsHashes->messages != NULL) { 07575 XFREE(ssl->hsHashes->messages, ssl->heap, DYNAMIC_TYPE_HASHES); 07576 ssl->hsHashes->messages = NULL; 07577 } 07578 } 07579 #endif 07580 break; 07581 07582 case encrypted_extensions: 07583 WOLFSSL_MSG("processing encrypted extensions"); 07584 ret = DoTls13EncryptedExtensions(ssl, input, inOutIdx, size); 07585 break; 07586 07587 #ifndef NO_CERTS 07588 case certificate_request: 07589 WOLFSSL_MSG("processing certificate request"); 07590 ret = DoTls13CertificateRequest(ssl, input, inOutIdx, size); 07591 break; 07592 #endif 07593 07594 case session_ticket: 07595 WOLFSSL_MSG("processing new session ticket"); 07596 ret = DoTls13NewSessionTicket(ssl, input, inOutIdx, size); 07597 break; 07598 #endif /* !NO_WOLFSSL_CLIENT */ 07599 07600 #ifndef NO_WOLFSSL_SERVER 07601 /* Messages only received by server. */ 07602 case client_hello: 07603 WOLFSSL_MSG("processing client hello"); 07604 ret = DoTls13ClientHello(ssl, input, inOutIdx, size); 07605 break; 07606 07607 #ifdef WOLFSSL_EARLY_DATA 07608 case end_of_early_data: 07609 WOLFSSL_MSG("processing end of early data"); 07610 ret = DoTls13EndOfEarlyData(ssl, input, inOutIdx, size); 07611 break; 07612 #endif 07613 #endif /* !NO_WOLFSSL_SERVER */ 07614 07615 /* Messages received by both client and server. */ 07616 #ifndef NO_CERTS 07617 case certificate: 07618 WOLFSSL_MSG("processing certificate"); 07619 ret = DoTls13Certificate(ssl, input, inOutIdx, size); 07620 break; 07621 #endif 07622 07623 #if !defined(NO_RSA) || defined(HAVE_ECC) || defined(HAVE_ED25519) || \ 07624 defined(HAVE_ED448) 07625 case certificate_verify: 07626 WOLFSSL_MSG("processing certificate verify"); 07627 ret = DoTls13CertificateVerify(ssl, input, inOutIdx, size); 07628 break; 07629 #endif /* !NO_RSA || HAVE_ECC */ 07630 07631 case finished: 07632 WOLFSSL_MSG("processing finished"); 07633 ret = DoTls13Finished(ssl, input, inOutIdx, size, totalSz, NO_SNIFF); 07634 break; 07635 07636 case key_update: 07637 WOLFSSL_MSG("processing finished"); 07638 ret = DoTls13KeyUpdate(ssl, input, inOutIdx, size); 07639 break; 07640 07641 default: 07642 WOLFSSL_MSG("Unknown handshake message type"); 07643 ret = UNKNOWN_HANDSHAKE_TYPE; 07644 break; 07645 } 07646 07647 /* reset error */ 07648 if (ret == 0 && ssl->error == WC_PENDING_E) 07649 ssl->error = 0; 07650 07651 if (ret == 0 && type != client_hello && type != session_ticket && 07652 type != key_update) { 07653 ret = HashInput(ssl, input + inIdx, size); 07654 } 07655 if (ret == 0 && ssl->buffers.inputBuffer.dynamicFlag) { 07656 ShrinkInputBuffer(ssl, NO_FORCED_FREE); 07657 } 07658 07659 if (ret == BUFFER_ERROR || ret == MISSING_HANDSHAKE_DATA) 07660 SendAlert(ssl, alert_fatal, decode_error); 07661 else if (ret == EXT_NOT_ALLOWED || ret == PEER_KEY_ERROR || 07662 ret == ECC_PEERKEY_ERROR || ret == BAD_KEY_SHARE_DATA || 07663 ret == PSK_KEY_ERROR || ret == INVALID_PARAMETER) { 07664 SendAlert(ssl, alert_fatal, illegal_parameter); 07665 } 07666 07667 if (ret == 0 && ssl->options.tls1_3) { 07668 /* Need to hash input message before deriving secrets. */ 07669 #ifndef NO_WOLFSSL_CLIENT 07670 if (ssl->options.side == WOLFSSL_CLIENT_END) { 07671 if (type == server_hello) { 07672 if ((ret = DeriveEarlySecret(ssl)) != 0) 07673 return ret; 07674 if ((ret = DeriveHandshakeSecret(ssl)) != 0) 07675 return ret; 07676 07677 if ((ret = DeriveTls13Keys(ssl, handshake_key, 07678 ENCRYPT_AND_DECRYPT_SIDE, 1)) != 0) { 07679 return ret; 07680 } 07681 #ifdef WOLFSSL_EARLY_DATA 07682 if ((ret = SetKeysSide(ssl, DECRYPT_SIDE_ONLY)) != 0) 07683 return ret; 07684 #else 07685 if ((ret = SetKeysSide(ssl, ENCRYPT_AND_DECRYPT_SIDE)) != 0) 07686 return ret; 07687 #endif 07688 } 07689 07690 if (type == finished) { 07691 if ((ret = DeriveMasterSecret(ssl)) != 0) 07692 return ret; 07693 #ifdef WOLFSSL_EARLY_DATA 07694 if ((ret = DeriveTls13Keys(ssl, traffic_key, 07695 ENCRYPT_AND_DECRYPT_SIDE, 07696 ssl->earlyData == no_early_data)) != 0) { 07697 return ret; 07698 } 07699 #else 07700 if ((ret = DeriveTls13Keys(ssl, traffic_key, 07701 ENCRYPT_AND_DECRYPT_SIDE, 1)) != 0) { 07702 return ret; 07703 } 07704 #endif 07705 } 07706 #ifdef WOLFSSL_POST_HANDSHAKE_AUTH 07707 if (type == certificate_request && 07708 ssl->options.handShakeState == HANDSHAKE_DONE) { 07709 /* reset handshake states */ 07710 ssl->options.clientState = CLIENT_HELLO_COMPLETE; 07711 ssl->options.connectState = FIRST_REPLY_DONE; 07712 ssl->options.handShakeState = CLIENT_HELLO_COMPLETE; 07713 07714 if (wolfSSL_connect_TLSv13(ssl) != SSL_SUCCESS) 07715 ret = POST_HAND_AUTH_ERROR; 07716 } 07717 #endif 07718 } 07719 #endif /* NO_WOLFSSL_CLIENT */ 07720 07721 #ifndef NO_WOLFSSL_SERVER 07722 #if defined(HAVE_SESSION_TICKET) || !defined(NO_PSK) 07723 if (ssl->options.side == WOLFSSL_SERVER_END && type == finished) { 07724 ret = DeriveResumptionSecret(ssl, ssl->session.masterSecret); 07725 if (ret != 0) 07726 return ret; 07727 } 07728 #endif 07729 #endif /* NO_WOLFSSL_SERVER */ 07730 } 07731 07732 #ifdef WOLFSSL_ASYNC_CRYPT 07733 /* if async, offset index so this msg will be processed again */ 07734 if (ret == WC_PENDING_E && *inOutIdx > 0) { 07735 *inOutIdx -= HANDSHAKE_HEADER_SZ; 07736 } 07737 #endif 07738 07739 WOLFSSL_LEAVE("DoTls13HandShakeMsgType()", ret); 07740 return ret; 07741 } 07742 07743 07744 /* Handle a handshake message that has been received. 07745 * 07746 * ssl The SSL/TLS object. 07747 * input The message buffer. 07748 * inOutIdx On entry, the index into the buffer of the current message. 07749 * On exit, the index into the buffer of the next message. 07750 * totalSz Length of remaining data in the message buffer. 07751 * returns 0 on success and otherwise failure. 07752 */ 07753 int DoTls13HandShakeMsg(WOLFSSL* ssl, byte* input, word32* inOutIdx, 07754 word32 totalSz) 07755 { 07756 int ret = 0; 07757 word32 inputLength; 07758 07759 WOLFSSL_ENTER("DoTls13HandShakeMsg()"); 07760 07761 if (ssl->arrays == NULL) { 07762 byte type; 07763 word32 size; 07764 07765 if (GetHandshakeHeader(ssl, input, inOutIdx, &type, &size, 07766 totalSz) != 0) { 07767 SendAlert(ssl, alert_fatal, unexpected_message); 07768 return PARSE_ERROR; 07769 } 07770 07771 return DoTls13HandShakeMsgType(ssl, input, inOutIdx, type, size, 07772 totalSz); 07773 } 07774 07775 inputLength = ssl->buffers.inputBuffer.length - *inOutIdx - ssl->keys.padSz; 07776 07777 /* If there is a pending fragmented handshake message, 07778 * pending message size will be non-zero. */ 07779 if (ssl->arrays->pendingMsgSz == 0) { 07780 byte type; 07781 word32 size; 07782 07783 if (GetHandshakeHeader(ssl,input, inOutIdx, &type, &size, totalSz) != 0) 07784 return PARSE_ERROR; 07785 07786 /* Cap the maximum size of a handshake message to something reasonable. 07787 * By default is the maximum size of a certificate message assuming 07788 * nine 2048-bit RSA certificates in the chain. */ 07789 if (size > MAX_HANDSHAKE_SZ) { 07790 WOLFSSL_MSG("Handshake message too large"); 07791 return HANDSHAKE_SIZE_ERROR; 07792 } 07793 07794 /* size is the size of the certificate message payload */ 07795 if (inputLength - HANDSHAKE_HEADER_SZ < size) { 07796 ssl->arrays->pendingMsgType = type; 07797 ssl->arrays->pendingMsgSz = size + HANDSHAKE_HEADER_SZ; 07798 ssl->arrays->pendingMsg = (byte*)XMALLOC(size + HANDSHAKE_HEADER_SZ, 07799 ssl->heap, 07800 DYNAMIC_TYPE_ARRAYS); 07801 if (ssl->arrays->pendingMsg == NULL) 07802 return MEMORY_E; 07803 XMEMCPY(ssl->arrays->pendingMsg, 07804 input + *inOutIdx - HANDSHAKE_HEADER_SZ, 07805 inputLength); 07806 ssl->arrays->pendingMsgOffset = inputLength; 07807 *inOutIdx += inputLength + ssl->keys.padSz - HANDSHAKE_HEADER_SZ; 07808 return 0; 07809 } 07810 07811 ret = DoTls13HandShakeMsgType(ssl, input, inOutIdx, type, size, 07812 totalSz); 07813 } 07814 else { 07815 if (inputLength + ssl->arrays->pendingMsgOffset > 07816 ssl->arrays->pendingMsgSz) { 07817 inputLength = ssl->arrays->pendingMsgSz - 07818 ssl->arrays->pendingMsgOffset; 07819 } 07820 XMEMCPY(ssl->arrays->pendingMsg + ssl->arrays->pendingMsgOffset, 07821 input + *inOutIdx, inputLength); 07822 ssl->arrays->pendingMsgOffset += inputLength; 07823 *inOutIdx += inputLength + ssl->keys.padSz; 07824 07825 if (ssl->arrays->pendingMsgOffset == ssl->arrays->pendingMsgSz) 07826 { 07827 word32 idx = 0; 07828 ret = DoTls13HandShakeMsgType(ssl, 07829 ssl->arrays->pendingMsg + HANDSHAKE_HEADER_SZ, 07830 &idx, ssl->arrays->pendingMsgType, 07831 ssl->arrays->pendingMsgSz - HANDSHAKE_HEADER_SZ, 07832 ssl->arrays->pendingMsgSz); 07833 #ifdef WOLFSSL_ASYNC_CRYPT 07834 if (ret == WC_PENDING_E) { 07835 /* setup to process fragment again */ 07836 ssl->arrays->pendingMsgOffset -= inputLength; 07837 *inOutIdx -= inputLength + ssl->keys.padSz; 07838 } 07839 else 07840 #endif 07841 { 07842 XFREE(ssl->arrays->pendingMsg, ssl->heap, DYNAMIC_TYPE_ARRAYS); 07843 ssl->arrays->pendingMsg = NULL; 07844 ssl->arrays->pendingMsgSz = 0; 07845 } 07846 } 07847 } 07848 07849 WOLFSSL_LEAVE("DoTls13HandShakeMsg()", ret); 07850 return ret; 07851 } 07852 07853 #ifndef NO_WOLFSSL_CLIENT 07854 07855 /* The client connecting to the server. 07856 * The protocol version is expecting to be TLS v1.3. 07857 * If the server downgrades, and older versions of the protocol are compiled 07858 * in, the client will fallback to wolfSSL_connect(). 07859 * Please see note at top of README if you get an error from connect. 07860 * 07861 * ssl The SSL/TLS object. 07862 * returns WOLFSSL_SUCCESS on successful handshake, WOLFSSL_FATAL_ERROR when 07863 * unrecoverable error occurs and 0 otherwise. 07864 * For more error information use wolfSSL_get_error(). 07865 */ 07866 int wolfSSL_connect_TLSv13(WOLFSSL* ssl) 07867 { 07868 WOLFSSL_ENTER("wolfSSL_connect_TLSv13()"); 07869 07870 #ifdef HAVE_ERRNO_H 07871 errno = 0; 07872 #endif 07873 07874 if (ssl->options.side != WOLFSSL_CLIENT_END) { 07875 WOLFSSL_ERROR(ssl->error = SIDE_ERROR); 07876 return WOLFSSL_FATAL_ERROR; 07877 } 07878 07879 if (ssl->buffers.outputBuffer.length > 0 07880 #ifdef WOLFSSL_ASYNC_CRYPT 07881 /* do not send buffered or advance state if last error was an 07882 async pending operation */ 07883 && ssl->error != WC_PENDING_E 07884 #endif 07885 ) { 07886 if ((ssl->error = SendBuffered(ssl)) == 0) { 07887 /* fragOffset is non-zero when sending fragments. On the last 07888 * fragment, fragOffset is zero again, and the state can be 07889 * advanced. */ 07890 if (ssl->fragOffset == 0) { 07891 ssl->options.connectState++; 07892 WOLFSSL_MSG("connect state: " 07893 "Advanced from last buffered fragment send"); 07894 } 07895 else { 07896 WOLFSSL_MSG("connect state: " 07897 "Not advanced, more fragments to send"); 07898 } 07899 } 07900 else { 07901 WOLFSSL_ERROR(ssl->error); 07902 return WOLFSSL_FATAL_ERROR; 07903 } 07904 } 07905 07906 switch (ssl->options.connectState) { 07907 07908 case CONNECT_BEGIN: 07909 /* Always send client hello first. */ 07910 if ((ssl->error = SendTls13ClientHello(ssl)) != 0) { 07911 WOLFSSL_ERROR(ssl->error); 07912 return WOLFSSL_FATAL_ERROR; 07913 } 07914 07915 ssl->options.connectState = CLIENT_HELLO_SENT; 07916 WOLFSSL_MSG("connect state: CLIENT_HELLO_SENT"); 07917 #ifdef WOLFSSL_EARLY_DATA 07918 if (ssl->earlyData != no_early_data) { 07919 #if !defined(WOLFSSL_TLS13_DRAFT_18) && \ 07920 defined(WOLFSSL_TLS13_MIDDLEBOX_COMPAT) 07921 if ((ssl->error = SendChangeCipher(ssl)) != 0) { 07922 WOLFSSL_ERROR(ssl->error); 07923 return WOLFSSL_FATAL_ERROR; 07924 } 07925 ssl->options.sentChangeCipher = 1; 07926 #endif 07927 ssl->options.handShakeState = CLIENT_HELLO_COMPLETE; 07928 return WOLFSSL_SUCCESS; 07929 } 07930 #endif 07931 FALL_THROUGH; 07932 07933 case CLIENT_HELLO_SENT: 07934 /* Get the response/s from the server. */ 07935 while (ssl->options.serverState < 07936 SERVER_HELLO_RETRY_REQUEST_COMPLETE) { 07937 if ((ssl->error = ProcessReply(ssl)) < 0) { 07938 WOLFSSL_ERROR(ssl->error); 07939 return WOLFSSL_FATAL_ERROR; 07940 } 07941 } 07942 07943 ssl->options.connectState = HELLO_AGAIN; 07944 WOLFSSL_MSG("connect state: HELLO_AGAIN"); 07945 FALL_THROUGH; 07946 07947 case HELLO_AGAIN: 07948 if (ssl->options.certOnly) 07949 return WOLFSSL_SUCCESS; 07950 07951 if (!ssl->options.tls1_3) { 07952 #ifndef WOLFSSL_NO_TLS12 07953 if (ssl->options.downgrade) 07954 return wolfSSL_connect(ssl); 07955 #endif 07956 07957 WOLFSSL_MSG("Client using higher version, fatal error"); 07958 return VERSION_ERROR; 07959 } 07960 07961 if (ssl->options.serverState == 07962 SERVER_HELLO_RETRY_REQUEST_COMPLETE) { 07963 #if !defined(WOLFSSL_TLS13_DRAFT_18) && \ 07964 defined(WOLFSSL_TLS13_MIDDLEBOX_COMPAT) 07965 if (!ssl->options.sentChangeCipher) { 07966 if ((ssl->error = SendChangeCipher(ssl)) != 0) { 07967 WOLFSSL_ERROR(ssl->error); 07968 return WOLFSSL_FATAL_ERROR; 07969 } 07970 ssl->options.sentChangeCipher = 1; 07971 } 07972 #endif 07973 /* Try again with different security parameters. */ 07974 if ((ssl->error = SendTls13ClientHello(ssl)) != 0) { 07975 WOLFSSL_ERROR(ssl->error); 07976 return WOLFSSL_FATAL_ERROR; 07977 } 07978 } 07979 07980 ssl->options.connectState = HELLO_AGAIN_REPLY; 07981 WOLFSSL_MSG("connect state: HELLO_AGAIN_REPLY"); 07982 FALL_THROUGH; 07983 07984 case HELLO_AGAIN_REPLY: 07985 /* Get the response/s from the server. */ 07986 while (ssl->options.serverState < SERVER_FINISHED_COMPLETE) { 07987 if ((ssl->error = ProcessReply(ssl)) < 0) { 07988 WOLFSSL_ERROR(ssl->error); 07989 return WOLFSSL_FATAL_ERROR; 07990 } 07991 } 07992 07993 ssl->options.connectState = FIRST_REPLY_DONE; 07994 WOLFSSL_MSG("connect state: FIRST_REPLY_DONE"); 07995 FALL_THROUGH; 07996 07997 case FIRST_REPLY_DONE: 07998 #ifdef WOLFSSL_EARLY_DATA 07999 if (ssl->earlyData != no_early_data) { 08000 if ((ssl->error = SendTls13EndOfEarlyData(ssl)) != 0) { 08001 WOLFSSL_ERROR(ssl->error); 08002 return WOLFSSL_FATAL_ERROR; 08003 } 08004 WOLFSSL_MSG("sent: end_of_early_data"); 08005 } 08006 #endif 08007 08008 ssl->options.connectState = FIRST_REPLY_FIRST; 08009 WOLFSSL_MSG("connect state: FIRST_REPLY_FIRST"); 08010 FALL_THROUGH; 08011 08012 case FIRST_REPLY_FIRST: 08013 #if !defined(WOLFSSL_TLS13_DRAFT_18) && \ 08014 defined(WOLFSSL_TLS13_MIDDLEBOX_COMPAT) 08015 if (!ssl->options.sentChangeCipher) { 08016 if ((ssl->error = SendChangeCipher(ssl)) != 0) { 08017 WOLFSSL_ERROR(ssl->error); 08018 return WOLFSSL_FATAL_ERROR; 08019 } 08020 ssl->options.sentChangeCipher = 1; 08021 } 08022 #endif 08023 08024 ssl->options.connectState = FIRST_REPLY_SECOND; 08025 WOLFSSL_MSG("connect state: FIRST_REPLY_SECOND"); 08026 FALL_THROUGH; 08027 08028 case FIRST_REPLY_SECOND: 08029 #ifndef NO_CERTS 08030 if (!ssl->options.resuming && ssl->options.sendVerify) { 08031 ssl->error = SendTls13Certificate(ssl); 08032 if (ssl->error != 0) { 08033 WOLFSSL_ERROR(ssl->error); 08034 return WOLFSSL_FATAL_ERROR; 08035 } 08036 WOLFSSL_MSG("sent: certificate"); 08037 } 08038 #endif 08039 08040 ssl->options.connectState = FIRST_REPLY_THIRD; 08041 WOLFSSL_MSG("connect state: FIRST_REPLY_THIRD"); 08042 FALL_THROUGH; 08043 08044 case FIRST_REPLY_THIRD: 08045 #ifndef NO_CERTS 08046 if (!ssl->options.resuming && ssl->options.sendVerify) { 08047 ssl->error = SendTls13CertificateVerify(ssl); 08048 if (ssl->error != 0) { 08049 WOLFSSL_ERROR(ssl->error); 08050 return WOLFSSL_FATAL_ERROR; 08051 } 08052 WOLFSSL_MSG("sent: certificate verify"); 08053 } 08054 #endif 08055 08056 ssl->options.connectState = FIRST_REPLY_FOURTH; 08057 WOLFSSL_MSG("connect state: FIRST_REPLY_FOURTH"); 08058 FALL_THROUGH; 08059 08060 case FIRST_REPLY_FOURTH: 08061 if ((ssl->error = SendTls13Finished(ssl)) != 0) { 08062 WOLFSSL_ERROR(ssl->error); 08063 return WOLFSSL_FATAL_ERROR; 08064 } 08065 WOLFSSL_MSG("sent: finished"); 08066 08067 ssl->options.connectState = FINISHED_DONE; 08068 WOLFSSL_MSG("connect state: FINISHED_DONE"); 08069 FALL_THROUGH; 08070 08071 case FINISHED_DONE: 08072 #ifndef NO_HANDSHAKE_DONE_CB 08073 if (ssl->hsDoneCb != NULL) { 08074 int cbret = ssl->hsDoneCb(ssl, ssl->hsDoneCtx); 08075 if (cbret < 0) { 08076 ssl->error = cbret; 08077 WOLFSSL_MSG("HandShake Done Cb don't continue error"); 08078 return WOLFSSL_FATAL_ERROR; 08079 } 08080 } 08081 #endif /* NO_HANDSHAKE_DONE_CB */ 08082 08083 if (!ssl->options.keepResources) { 08084 FreeHandshakeResources(ssl); 08085 } 08086 08087 WOLFSSL_LEAVE("wolfSSL_connect_TLSv13()", WOLFSSL_SUCCESS); 08088 return WOLFSSL_SUCCESS; 08089 08090 default: 08091 WOLFSSL_MSG("Unknown connect state ERROR"); 08092 return WOLFSSL_FATAL_ERROR; /* unknown connect state */ 08093 } 08094 } 08095 #endif 08096 08097 #if defined(WOLFSSL_SEND_HRR_COOKIE) 08098 /* Send a cookie with the HelloRetryRequest to avoid storing state. 08099 * 08100 * ssl SSL/TLS object. 08101 * secret Secret to use when generating integrity check for cookie. 08102 * A value of NULL indicates to generate a new random secret. 08103 * secretSz Size of secret data in bytes. 08104 * Use a value of 0 to indicate use of default size. 08105 * returns BAD_FUNC_ARG when ssl is NULL or not using TLS v1.3, SIDE_ERROR when 08106 * called on a client; WOLFSSL_SUCCESS on success and otherwise failure. 08107 */ 08108 int wolfSSL_send_hrr_cookie(WOLFSSL* ssl, const unsigned char* secret, 08109 unsigned int secretSz) 08110 { 08111 int ret; 08112 08113 if (ssl == NULL || !IsAtLeastTLSv1_3(ssl->version)) 08114 return BAD_FUNC_ARG; 08115 #ifndef NO_WOLFSSL_SERVER 08116 if (ssl->options.side == WOLFSSL_CLIENT_END) 08117 return SIDE_ERROR; 08118 08119 if (secretSz == 0) { 08120 #if !defined(NO_SHA) && defined(NO_SHA256) 08121 secretSz = WC_SHA_DIGEST_SIZE; 08122 #endif /* NO_SHA */ 08123 #ifndef NO_SHA256 08124 secretSz = WC_SHA256_DIGEST_SIZE; 08125 #endif /* NO_SHA256 */ 08126 } 08127 08128 if (secretSz != ssl->buffers.tls13CookieSecret.length) { 08129 byte* newSecret; 08130 08131 if (ssl->buffers.tls13CookieSecret.buffer != NULL) { 08132 ForceZero(ssl->buffers.tls13CookieSecret.buffer, 08133 ssl->buffers.tls13CookieSecret.length); 08134 XFREE(ssl->buffers.tls13CookieSecret.buffer, 08135 ssl->heap, DYNAMIC_TYPE_COOKIE_PWD); 08136 } 08137 08138 newSecret = (byte*)XMALLOC(secretSz, ssl->heap, 08139 DYNAMIC_TYPE_COOKIE_PWD); 08140 if (newSecret == NULL) { 08141 ssl->buffers.tls13CookieSecret.buffer = NULL; 08142 ssl->buffers.tls13CookieSecret.length = 0; 08143 WOLFSSL_MSG("couldn't allocate new cookie secret"); 08144 return MEMORY_ERROR; 08145 } 08146 ssl->buffers.tls13CookieSecret.buffer = newSecret; 08147 ssl->buffers.tls13CookieSecret.length = secretSz; 08148 } 08149 08150 /* If the supplied secret is NULL, randomly generate a new secret. */ 08151 if (secret == NULL) { 08152 ret = wc_RNG_GenerateBlock(ssl->rng, 08153 ssl->buffers.tls13CookieSecret.buffer, secretSz); 08154 if (ret < 0) 08155 return ret; 08156 } 08157 else 08158 XMEMCPY(ssl->buffers.tls13CookieSecret.buffer, secret, secretSz); 08159 08160 ssl->options.sendCookie = 1; 08161 08162 ret = WOLFSSL_SUCCESS; 08163 #else 08164 (void)secret; 08165 (void)secretSz; 08166 08167 ret = SIDE_ERROR; 08168 #endif 08169 08170 return ret; 08171 } 08172 #endif 08173 08174 /* Create a key share entry from group. 08175 * Generates a key pair. 08176 * 08177 * ssl The SSL/TLS object. 08178 * group The named group. 08179 * returns 0 on success, otherwise failure. 08180 */ 08181 int wolfSSL_UseKeyShare(WOLFSSL* ssl, word16 group) 08182 { 08183 int ret; 08184 08185 if (ssl == NULL) 08186 return BAD_FUNC_ARG; 08187 08188 ret = TLSX_KeyShare_Use(ssl, group, 0, NULL, NULL); 08189 if (ret != 0) 08190 return ret; 08191 08192 return WOLFSSL_SUCCESS; 08193 } 08194 08195 /* Send no key share entries - use HelloRetryRequest to negotiate shared group. 08196 * 08197 * ssl The SSL/TLS object. 08198 * returns 0 on success, otherwise failure. 08199 */ 08200 int wolfSSL_NoKeyShares(WOLFSSL* ssl) 08201 { 08202 int ret; 08203 08204 if (ssl == NULL) 08205 return BAD_FUNC_ARG; 08206 if (ssl->options.side == WOLFSSL_SERVER_END) 08207 return SIDE_ERROR; 08208 08209 ret = TLSX_KeyShare_Empty(ssl); 08210 if (ret != 0) 08211 return ret; 08212 08213 return WOLFSSL_SUCCESS; 08214 } 08215 08216 /* Do not send a ticket after TLS v1.3 handshake for resumption. 08217 * 08218 * ctx The SSL/TLS CTX object. 08219 * returns BAD_FUNC_ARG when ctx is NULL and 0 on success. 08220 */ 08221 int wolfSSL_CTX_no_ticket_TLSv13(WOLFSSL_CTX* ctx) 08222 { 08223 if (ctx == NULL || !IsAtLeastTLSv1_3(ctx->method->version)) 08224 return BAD_FUNC_ARG; 08225 if (ctx->method->side == WOLFSSL_CLIENT_END) 08226 return SIDE_ERROR; 08227 08228 #ifdef HAVE_SESSION_TICKET 08229 ctx->noTicketTls13 = 1; 08230 #endif 08231 08232 return 0; 08233 } 08234 08235 /* Do not send a ticket after TLS v1.3 handshake for resumption. 08236 * 08237 * ssl The SSL/TLS object. 08238 * returns BAD_FUNC_ARG when ssl is NULL, not using TLS v1.3, or called on 08239 * a client and 0 on success. 08240 */ 08241 int wolfSSL_no_ticket_TLSv13(WOLFSSL* ssl) 08242 { 08243 if (ssl == NULL || !IsAtLeastTLSv1_3(ssl->version)) 08244 return BAD_FUNC_ARG; 08245 if (ssl->options.side == WOLFSSL_CLIENT_END) 08246 return SIDE_ERROR; 08247 08248 #ifdef HAVE_SESSION_TICKET 08249 ssl->options.noTicketTls13 = 1; 08250 #endif 08251 08252 return 0; 08253 } 08254 08255 /* Disallow (EC)DHE key exchange when using pre-shared keys. 08256 * 08257 * ctx The SSL/TLS CTX object. 08258 * returns BAD_FUNC_ARG when ctx is NULL and 0 on success. 08259 */ 08260 int wolfSSL_CTX_no_dhe_psk(WOLFSSL_CTX* ctx) 08261 { 08262 if (ctx == NULL || !IsAtLeastTLSv1_3(ctx->method->version)) 08263 return BAD_FUNC_ARG; 08264 08265 ctx->noPskDheKe = 1; 08266 08267 return 0; 08268 } 08269 08270 /* Disallow (EC)DHE key exchange when using pre-shared keys. 08271 * 08272 * ssl The SSL/TLS object. 08273 * returns BAD_FUNC_ARG when ssl is NULL, or not using TLS v1.3 and 0 on 08274 * success. 08275 */ 08276 int wolfSSL_no_dhe_psk(WOLFSSL* ssl) 08277 { 08278 if (ssl == NULL || !IsAtLeastTLSv1_3(ssl->version)) 08279 return BAD_FUNC_ARG; 08280 08281 ssl->options.noPskDheKe = 1; 08282 08283 return 0; 08284 } 08285 08286 /* Update the keys for encryption and decryption. 08287 * If using non-blocking I/O and WOLFSSL_ERROR_WANT_WRITE is returned then 08288 * calling wolfSSL_write() will have the message sent when ready. 08289 * 08290 * ssl The SSL/TLS object. 08291 * returns BAD_FUNC_ARG when ssl is NULL, or not using TLS v1.3, 08292 * WOLFSSL_ERROR_WANT_WRITE when non-blocking I/O is not ready to write, 08293 * WOLFSSL_SUCCESS on success and otherwise failure. 08294 */ 08295 int wolfSSL_update_keys(WOLFSSL* ssl) 08296 { 08297 int ret; 08298 08299 if (ssl == NULL || !IsAtLeastTLSv1_3(ssl->version)) 08300 return BAD_FUNC_ARG; 08301 08302 ret = SendTls13KeyUpdate(ssl); 08303 if (ret == WANT_WRITE) 08304 ret = WOLFSSL_ERROR_WANT_WRITE; 08305 else if (ret == 0) 08306 ret = WOLFSSL_SUCCESS; 08307 return ret; 08308 } 08309 08310 #if !defined(NO_CERTS) && defined(WOLFSSL_POST_HANDSHAKE_AUTH) 08311 /* Allow post-handshake authentication in TLS v1.3 connections. 08312 * 08313 * ctx The SSL/TLS CTX object. 08314 * returns BAD_FUNC_ARG when ctx is NULL, SIDE_ERROR when not a client and 08315 * 0 on success. 08316 */ 08317 int wolfSSL_CTX_allow_post_handshake_auth(WOLFSSL_CTX* ctx) 08318 { 08319 if (ctx == NULL || !IsAtLeastTLSv1_3(ctx->method->version)) 08320 return BAD_FUNC_ARG; 08321 if (ctx->method->side == WOLFSSL_SERVER_END) 08322 return SIDE_ERROR; 08323 08324 ctx->postHandshakeAuth = 1; 08325 08326 return 0; 08327 } 08328 08329 /* Allow post-handshake authentication in TLS v1.3 connection. 08330 * 08331 * ssl The SSL/TLS object. 08332 * returns BAD_FUNC_ARG when ssl is NULL, or not using TLS v1.3, 08333 * SIDE_ERROR when not a client and 0 on success. 08334 */ 08335 int wolfSSL_allow_post_handshake_auth(WOLFSSL* ssl) 08336 { 08337 if (ssl == NULL || !IsAtLeastTLSv1_3(ssl->version)) 08338 return BAD_FUNC_ARG; 08339 if (ssl->options.side == WOLFSSL_SERVER_END) 08340 return SIDE_ERROR; 08341 08342 ssl->options.postHandshakeAuth = 1; 08343 08344 return 0; 08345 } 08346 08347 /* Request a certificate of the client. 08348 * Can be called any time after handshake completion. 08349 * A maximum of 256 requests can be sent on a connection. 08350 * 08351 * ssl SSL/TLS object. 08352 */ 08353 int wolfSSL_request_certificate(WOLFSSL* ssl) 08354 { 08355 int ret; 08356 #ifndef NO_WOLFSSL_SERVER 08357 CertReqCtx* certReqCtx; 08358 #endif 08359 08360 if (ssl == NULL || !IsAtLeastTLSv1_3(ssl->version)) 08361 return BAD_FUNC_ARG; 08362 #ifndef NO_WOLFSSL_SERVER 08363 if (ssl->options.side == WOLFSSL_CLIENT_END) 08364 return SIDE_ERROR; 08365 if (ssl->options.handShakeState != HANDSHAKE_DONE) 08366 return NOT_READY_ERROR; 08367 if (!ssl->options.postHandshakeAuth) 08368 return POST_HAND_AUTH_ERROR; 08369 08370 certReqCtx = (CertReqCtx*)XMALLOC(sizeof(CertReqCtx), ssl->heap, 08371 DYNAMIC_TYPE_TMP_BUFFER); 08372 if (certReqCtx == NULL) 08373 return MEMORY_E; 08374 XMEMSET(certReqCtx, 0, sizeof(CertReqCtx)); 08375 certReqCtx->next = ssl->certReqCtx; 08376 certReqCtx->len = 1; 08377 if (certReqCtx->next != NULL) 08378 certReqCtx->ctx = certReqCtx->next->ctx + 1; 08379 ssl->certReqCtx = certReqCtx; 08380 08381 ssl->msgsReceived.got_certificate = 0; 08382 ssl->msgsReceived.got_certificate_verify = 0; 08383 ssl->msgsReceived.got_finished = 0; 08384 08385 ret = SendTls13CertificateRequest(ssl, &certReqCtx->ctx, certReqCtx->len); 08386 if (ret == WANT_WRITE) 08387 ret = WOLFSSL_ERROR_WANT_WRITE; 08388 else if (ret == 0) 08389 ret = WOLFSSL_SUCCESS; 08390 #else 08391 ret = SIDE_ERROR; 08392 #endif 08393 08394 return ret; 08395 } 08396 #endif /* !NO_CERTS && WOLFSSL_POST_HANDSHAKE_AUTH */ 08397 08398 #if !defined(WOLFSSL_NO_SERVER_GROUPS_EXT) 08399 /* Get the preferred key exchange group. 08400 * 08401 * ssl The SSL/TLS object. 08402 * returns BAD_FUNC_ARG when ssl is NULL or not using TLS v1.3, 08403 * SIDE_ERROR when not a client, NOT_READY_ERROR when handshake not complete 08404 * and group number on success. 08405 */ 08406 int wolfSSL_preferred_group(WOLFSSL* ssl) 08407 { 08408 if (ssl == NULL || !IsAtLeastTLSv1_3(ssl->version)) 08409 return BAD_FUNC_ARG; 08410 #ifndef NO_WOLFSSL_CLIENT 08411 if (ssl->options.side == WOLFSSL_SERVER_END) 08412 return SIDE_ERROR; 08413 if (ssl->options.handShakeState != HANDSHAKE_DONE) 08414 return NOT_READY_ERROR; 08415 08416 /* Return supported groups only. */ 08417 return TLSX_SupportedCurve_Preferred(ssl, 1); 08418 #else 08419 return SIDE_ERROR; 08420 #endif 08421 } 08422 #endif 08423 08424 /* Sets the key exchange groups in rank order on a context. 08425 * 08426 * ctx SSL/TLS context object. 08427 * groups Array of groups. 08428 * count Number of groups in array. 08429 * returns BAD_FUNC_ARG when ctx or groups is NULL, not using TLS v1.3 or 08430 * count is greater than WOLFSSL_MAX_GROUP_COUNT and WOLFSSL_SUCCESS on success. 08431 */ 08432 int wolfSSL_CTX_set_groups(WOLFSSL_CTX* ctx, int* groups, int count) 08433 { 08434 int i; 08435 08436 if (ctx == NULL || groups == NULL || count > WOLFSSL_MAX_GROUP_COUNT) 08437 return BAD_FUNC_ARG; 08438 if (!IsAtLeastTLSv1_3(ctx->method->version)) 08439 return BAD_FUNC_ARG; 08440 08441 for (i = 0; i < count; i++) 08442 ctx->group[i] = (word16)groups[i]; 08443 ctx->numGroups = (byte)count; 08444 08445 return WOLFSSL_SUCCESS; 08446 } 08447 08448 /* Sets the key exchange groups in rank order. 08449 * 08450 * ssl SSL/TLS object. 08451 * groups Array of groups. 08452 * count Number of groups in array. 08453 * returns BAD_FUNC_ARG when ssl or groups is NULL, not using TLS v1.3 or 08454 * count is greater than WOLFSSL_MAX_GROUP_COUNT and WOLFSSL_SUCCESS on success. 08455 */ 08456 int wolfSSL_set_groups(WOLFSSL* ssl, int* groups, int count) 08457 { 08458 int i; 08459 08460 if (ssl == NULL || groups == NULL || count > WOLFSSL_MAX_GROUP_COUNT) 08461 return BAD_FUNC_ARG; 08462 if (!IsAtLeastTLSv1_3(ssl->version)) 08463 return BAD_FUNC_ARG; 08464 08465 for (i = 0; i < count; i++) 08466 ssl->group[i] = (word16)groups[i]; 08467 ssl->numGroups = (byte)count; 08468 08469 return WOLFSSL_SUCCESS; 08470 } 08471 08472 #ifndef NO_PSK 08473 void wolfSSL_CTX_set_psk_client_tls13_callback(WOLFSSL_CTX* ctx, 08474 wc_psk_client_tls13_callback cb) 08475 { 08476 WOLFSSL_ENTER("SSL_CTX_set_psk_client_tls13_callback"); 08477 08478 if (ctx == NULL) 08479 return; 08480 08481 ctx->havePSK = 1; 08482 ctx->client_psk_tls13_cb = cb; 08483 } 08484 08485 08486 void wolfSSL_set_psk_client_tls13_callback(WOLFSSL* ssl, 08487 wc_psk_client_tls13_callback cb) 08488 { 08489 byte haveRSA = 1; 08490 int keySz = 0; 08491 08492 WOLFSSL_ENTER("SSL_set_psk_client_tls13_callback"); 08493 08494 if (ssl == NULL) 08495 return; 08496 08497 ssl->options.havePSK = 1; 08498 ssl->options.client_psk_tls13_cb = cb; 08499 08500 #ifdef NO_RSA 08501 haveRSA = 0; 08502 #endif 08503 #ifndef NO_CERTS 08504 keySz = ssl->buffers.keySz; 08505 #endif 08506 InitSuites(ssl->suites, ssl->version, keySz, haveRSA, TRUE, 08507 ssl->options.haveDH, ssl->options.haveNTRU, 08508 ssl->options.haveECDSAsig, ssl->options.haveECC, 08509 ssl->options.haveStaticECC, ssl->options.side); 08510 } 08511 08512 08513 void wolfSSL_CTX_set_psk_server_tls13_callback(WOLFSSL_CTX* ctx, 08514 wc_psk_server_tls13_callback cb) 08515 { 08516 WOLFSSL_ENTER("SSL_CTX_set_psk_server_tls13_callback"); 08517 if (ctx == NULL) 08518 return; 08519 ctx->havePSK = 1; 08520 ctx->server_psk_tls13_cb = cb; 08521 } 08522 08523 08524 void wolfSSL_set_psk_server_tls13_callback(WOLFSSL* ssl, 08525 wc_psk_server_tls13_callback cb) 08526 { 08527 byte haveRSA = 1; 08528 int keySz = 0; 08529 08530 WOLFSSL_ENTER("SSL_set_psk_server_tls13_callback"); 08531 if (ssl == NULL) 08532 return; 08533 08534 ssl->options.havePSK = 1; 08535 ssl->options.server_psk_tls13_cb = cb; 08536 08537 #ifdef NO_RSA 08538 haveRSA = 0; 08539 #endif 08540 #ifndef NO_CERTS 08541 keySz = ssl->buffers.keySz; 08542 #endif 08543 InitSuites(ssl->suites, ssl->version, keySz, haveRSA, TRUE, 08544 ssl->options.haveDH, ssl->options.haveNTRU, 08545 ssl->options.haveECDSAsig, ssl->options.haveECC, 08546 ssl->options.haveStaticECC, ssl->options.side); 08547 } 08548 #endif 08549 08550 08551 #ifndef NO_WOLFSSL_SERVER 08552 /* The server accepting a connection from a client. 08553 * The protocol version is expecting to be TLS v1.3. 08554 * If the client downgrades, and older versions of the protocol are compiled 08555 * in, the server will fallback to wolfSSL_accept(). 08556 * Please see note at top of README if you get an error from accept. 08557 * 08558 * ssl The SSL/TLS object. 08559 * returns WOLFSSL_SUCCESS on successful handshake, WOLFSSL_FATAL_ERROR when 08560 * unrecoverable error occurs and 0 otherwise. 08561 * For more error information use wolfSSL_get_error(). 08562 */ 08563 int wolfSSL_accept_TLSv13(WOLFSSL* ssl) 08564 { 08565 word16 havePSK = 0; 08566 WOLFSSL_ENTER("SSL_accept_TLSv13()"); 08567 08568 #ifdef HAVE_ERRNO_H 08569 errno = 0; 08570 #endif 08571 08572 #if defined(HAVE_SESSION_TICKET) || !defined(NO_PSK) 08573 havePSK = ssl->options.havePSK; 08574 #endif 08575 (void)havePSK; 08576 08577 if (ssl->options.side != WOLFSSL_SERVER_END) { 08578 WOLFSSL_ERROR(ssl->error = SIDE_ERROR); 08579 return WOLFSSL_FATAL_ERROR; 08580 } 08581 08582 #ifndef NO_CERTS 08583 /* allow no private key if using PK callbacks and CB is set */ 08584 if (!havePSK) { 08585 if (!ssl->buffers.certificate || 08586 !ssl->buffers.certificate->buffer) { 08587 08588 WOLFSSL_MSG("accept error: server cert required"); 08589 WOLFSSL_ERROR(ssl->error = NO_PRIVATE_KEY); 08590 return WOLFSSL_FATAL_ERROR; 08591 } 08592 08593 #ifdef HAVE_PK_CALLBACKS 08594 if (wolfSSL_CTX_IsPrivatePkSet(ssl->ctx)) { 08595 WOLFSSL_MSG("Using PK for server private key"); 08596 } 08597 else 08598 #endif 08599 if (!ssl->buffers.key || !ssl->buffers.key->buffer) { 08600 WOLFSSL_MSG("accept error: server key required"); 08601 WOLFSSL_ERROR(ssl->error = NO_PRIVATE_KEY); 08602 return WOLFSSL_FATAL_ERROR; 08603 } 08604 } 08605 #endif 08606 08607 if (ssl->buffers.outputBuffer.length > 0 08608 #ifdef WOLFSSL_ASYNC_CRYPT 08609 /* do not send buffered or advance state if last error was an 08610 async pending operation */ 08611 && ssl->error != WC_PENDING_E 08612 #endif 08613 ) { 08614 if ((ssl->error = SendBuffered(ssl)) == 0) { 08615 /* fragOffset is non-zero when sending fragments. On the last 08616 * fragment, fragOffset is zero again, and the state can be 08617 * advanced. */ 08618 if (ssl->fragOffset == 0) { 08619 ssl->options.acceptState++; 08620 WOLFSSL_MSG("accept state: " 08621 "Advanced from last buffered fragment send"); 08622 } 08623 else { 08624 WOLFSSL_MSG("accept state: " 08625 "Not advanced, more fragments to send"); 08626 } 08627 } 08628 else { 08629 WOLFSSL_ERROR(ssl->error); 08630 return WOLFSSL_FATAL_ERROR; 08631 } 08632 } 08633 08634 switch (ssl->options.acceptState) { 08635 08636 #ifdef HAVE_SECURE_RENEGOTIATION 08637 case TLS13_ACCEPT_BEGIN_RENEG: 08638 #endif 08639 case TLS13_ACCEPT_BEGIN : 08640 /* get client_hello */ 08641 while (ssl->options.clientState < CLIENT_HELLO_COMPLETE) { 08642 if ((ssl->error = ProcessReply(ssl)) < 0) { 08643 WOLFSSL_ERROR(ssl->error); 08644 return WOLFSSL_FATAL_ERROR; 08645 } 08646 } 08647 08648 ssl->options.acceptState = TLS13_ACCEPT_CLIENT_HELLO_DONE; 08649 WOLFSSL_MSG("accept state ACCEPT_CLIENT_HELLO_DONE"); 08650 if (!IsAtLeastTLSv1_3(ssl->version)) 08651 return wolfSSL_accept(ssl); 08652 FALL_THROUGH; 08653 08654 case TLS13_ACCEPT_CLIENT_HELLO_DONE : 08655 #ifdef WOLFSSL_TLS13_DRAFT_18 08656 if (ssl->options.serverState == 08657 SERVER_HELLO_RETRY_REQUEST_COMPLETE) { 08658 if ((ssl->error = SendTls13HelloRetryRequest(ssl)) != 0) { 08659 WOLFSSL_ERROR(ssl->error); 08660 return WOLFSSL_FATAL_ERROR; 08661 } 08662 } 08663 08664 ssl->options.acceptState = TLS13_ACCEPT_FIRST_REPLY_DONE; 08665 WOLFSSL_MSG("accept state ACCEPT_FIRST_REPLY_DONE"); 08666 FALL_THROUGH; 08667 08668 case TLS13_ACCEPT_HELLO_RETRY_REQUEST_DONE : 08669 #else 08670 if (ssl->options.serverState == 08671 SERVER_HELLO_RETRY_REQUEST_COMPLETE) { 08672 if ((ssl->error = SendTls13ServerHello(ssl, 08673 hello_retry_request)) != 0) { 08674 WOLFSSL_ERROR(ssl->error); 08675 return WOLFSSL_FATAL_ERROR; 08676 } 08677 } 08678 08679 ssl->options.acceptState = TLS13_ACCEPT_HELLO_RETRY_REQUEST_DONE; 08680 WOLFSSL_MSG("accept state ACCEPT_HELLO_RETRY_REQUEST_DONE"); 08681 FALL_THROUGH; 08682 08683 case TLS13_ACCEPT_HELLO_RETRY_REQUEST_DONE : 08684 #ifdef WOLFSSL_TLS13_MIDDLEBOX_COMPAT 08685 if (ssl->options.serverState == 08686 SERVER_HELLO_RETRY_REQUEST_COMPLETE) { 08687 if ((ssl->error = SendChangeCipher(ssl)) != 0) { 08688 WOLFSSL_ERROR(ssl->error); 08689 return WOLFSSL_FATAL_ERROR; 08690 } 08691 ssl->options.sentChangeCipher = 1; 08692 ssl->options.serverState = SERVER_HELLO_RETRY_REQUEST_COMPLETE; 08693 } 08694 #endif 08695 ssl->options.acceptState = TLS13_ACCEPT_FIRST_REPLY_DONE; 08696 WOLFSSL_MSG("accept state ACCEPT_FIRST_REPLY_DONE"); 08697 FALL_THROUGH; 08698 #endif 08699 08700 case TLS13_ACCEPT_FIRST_REPLY_DONE : 08701 if (ssl->options.serverState == 08702 SERVER_HELLO_RETRY_REQUEST_COMPLETE) { 08703 ssl->options.clientState = CLIENT_HELLO_RETRY; 08704 while (ssl->options.clientState < CLIENT_HELLO_COMPLETE) { 08705 if ((ssl->error = ProcessReply(ssl)) < 0) { 08706 WOLFSSL_ERROR(ssl->error); 08707 return WOLFSSL_FATAL_ERROR; 08708 } 08709 } 08710 } 08711 08712 ssl->options.acceptState = TLS13_ACCEPT_SECOND_REPLY_DONE; 08713 WOLFSSL_MSG("accept state ACCEPT_SECOND_REPLY_DONE"); 08714 FALL_THROUGH; 08715 08716 case TLS13_ACCEPT_SECOND_REPLY_DONE : 08717 if ((ssl->error = SendTls13ServerHello(ssl, server_hello)) != 0) { 08718 WOLFSSL_ERROR(ssl->error); 08719 return WOLFSSL_FATAL_ERROR; 08720 } 08721 ssl->options.acceptState = TLS13_SERVER_HELLO_SENT; 08722 WOLFSSL_MSG("accept state SERVER_HELLO_SENT"); 08723 FALL_THROUGH; 08724 08725 case TLS13_SERVER_HELLO_SENT : 08726 #if !defined(WOLFSSL_TLS13_DRAFT_18) && \ 08727 defined(WOLFSSL_TLS13_MIDDLEBOX_COMPAT) 08728 if (!ssl->options.sentChangeCipher) { 08729 if ((ssl->error = SendChangeCipher(ssl)) != 0) { 08730 WOLFSSL_ERROR(ssl->error); 08731 return WOLFSSL_FATAL_ERROR; 08732 } 08733 ssl->options.sentChangeCipher = 1; 08734 } 08735 #endif 08736 08737 ssl->options.acceptState = TLS13_ACCEPT_THIRD_REPLY_DONE; 08738 WOLFSSL_MSG("accept state ACCEPT_THIRD_REPLY_DONE"); 08739 FALL_THROUGH; 08740 08741 case TLS13_ACCEPT_THIRD_REPLY_DONE : 08742 if (!ssl->options.noPskDheKe) { 08743 ssl->error = TLSX_KeyShare_DeriveSecret(ssl); 08744 if (ssl->error != 0) 08745 return WOLFSSL_FATAL_ERROR; 08746 } 08747 08748 if ((ssl->error = SendTls13EncryptedExtensions(ssl)) != 0) { 08749 WOLFSSL_ERROR(ssl->error); 08750 return WOLFSSL_FATAL_ERROR; 08751 } 08752 ssl->options.acceptState = TLS13_SERVER_EXTENSIONS_SENT; 08753 WOLFSSL_MSG("accept state SERVER_EXTENSIONS_SENT"); 08754 FALL_THROUGH; 08755 08756 case TLS13_SERVER_EXTENSIONS_SENT : 08757 #ifndef NO_CERTS 08758 if (!ssl->options.resuming) { 08759 if (ssl->options.verifyPeer) { 08760 ssl->error = SendTls13CertificateRequest(ssl, NULL, 0); 08761 if (ssl->error != 0) { 08762 WOLFSSL_ERROR(ssl->error); 08763 return WOLFSSL_FATAL_ERROR; 08764 } 08765 } 08766 } 08767 #endif 08768 ssl->options.acceptState = TLS13_CERT_REQ_SENT; 08769 WOLFSSL_MSG("accept state CERT_REQ_SENT"); 08770 FALL_THROUGH; 08771 08772 case TLS13_CERT_REQ_SENT : 08773 #ifndef NO_CERTS 08774 if (!ssl->options.resuming && ssl->options.sendVerify) { 08775 if ((ssl->error = SendTls13Certificate(ssl)) != 0) { 08776 WOLFSSL_ERROR(ssl->error); 08777 return WOLFSSL_FATAL_ERROR; 08778 } 08779 } 08780 #endif 08781 ssl->options.acceptState = TLS13_CERT_SENT; 08782 WOLFSSL_MSG("accept state CERT_SENT"); 08783 FALL_THROUGH; 08784 08785 case TLS13_CERT_SENT : 08786 #ifndef NO_CERTS 08787 if (!ssl->options.resuming && ssl->options.sendVerify) { 08788 if ((ssl->error = SendTls13CertificateVerify(ssl)) != 0) { 08789 WOLFSSL_ERROR(ssl->error); 08790 return WOLFSSL_FATAL_ERROR; 08791 } 08792 } 08793 #endif 08794 ssl->options.acceptState = TLS13_CERT_VERIFY_SENT; 08795 WOLFSSL_MSG("accept state CERT_VERIFY_SENT"); 08796 FALL_THROUGH; 08797 08798 case TLS13_CERT_VERIFY_SENT : 08799 if ((ssl->error = SendTls13Finished(ssl)) != 0) { 08800 WOLFSSL_ERROR(ssl->error); 08801 return WOLFSSL_FATAL_ERROR; 08802 } 08803 08804 ssl->options.acceptState = TLS13_ACCEPT_FINISHED_SENT; 08805 WOLFSSL_MSG("accept state ACCEPT_FINISHED_SENT"); 08806 #ifdef WOLFSSL_EARLY_DATA 08807 if (ssl->earlyData != no_early_data) { 08808 ssl->options.handShakeState = SERVER_FINISHED_COMPLETE; 08809 return WOLFSSL_SUCCESS; 08810 } 08811 #endif 08812 FALL_THROUGH; 08813 08814 case TLS13_ACCEPT_FINISHED_SENT : 08815 #ifdef HAVE_SESSION_TICKET 08816 #ifdef WOLFSSL_TLS13_TICKET_BEFORE_FINISHED 08817 if (!ssl->options.verifyPeer && !ssl->options.noTicketTls13 && 08818 ssl->ctx->ticketEncCb != NULL) { 08819 if ((ssl->error = SendTls13NewSessionTicket(ssl)) != 0) { 08820 WOLFSSL_ERROR(ssl->error); 08821 return WOLFSSL_FATAL_ERROR; 08822 } 08823 } 08824 #endif 08825 #endif /* HAVE_SESSION_TICKET */ 08826 ssl->options.acceptState = TLS13_PRE_TICKET_SENT; 08827 WOLFSSL_MSG("accept state TICKET_SENT"); 08828 FALL_THROUGH; 08829 08830 case TLS13_PRE_TICKET_SENT : 08831 while (ssl->options.clientState < CLIENT_FINISHED_COMPLETE) 08832 if ( (ssl->error = ProcessReply(ssl)) < 0) { 08833 WOLFSSL_ERROR(ssl->error); 08834 return WOLFSSL_FATAL_ERROR; 08835 } 08836 08837 ssl->options.acceptState = TLS13_ACCEPT_FINISHED_DONE; 08838 WOLFSSL_MSG("accept state ACCEPT_FINISHED_DONE"); 08839 FALL_THROUGH; 08840 08841 case TLS13_ACCEPT_FINISHED_DONE : 08842 #ifdef HAVE_SESSION_TICKET 08843 #ifdef WOLFSSL_TLS13_TICKET_BEFORE_FINISHED 08844 if (!ssl->options.verifyPeer) { 08845 } 08846 else 08847 #endif 08848 if (!ssl->options.noTicketTls13 && ssl->ctx->ticketEncCb != NULL) { 08849 if ((ssl->error = SendTls13NewSessionTicket(ssl)) != 0) { 08850 WOLFSSL_ERROR(ssl->error); 08851 return WOLFSSL_FATAL_ERROR; 08852 } 08853 } 08854 #endif /* HAVE_SESSION_TICKET */ 08855 ssl->options.acceptState = TLS13_TICKET_SENT; 08856 WOLFSSL_MSG("accept state TICKET_SENT"); 08857 FALL_THROUGH; 08858 08859 case TLS13_TICKET_SENT : 08860 #ifndef NO_HANDSHAKE_DONE_CB 08861 if (ssl->hsDoneCb) { 08862 int cbret = ssl->hsDoneCb(ssl, ssl->hsDoneCtx); 08863 if (cbret < 0) { 08864 ssl->error = cbret; 08865 WOLFSSL_MSG("HandShake Done Cb don't continue error"); 08866 return WOLFSSL_FATAL_ERROR; 08867 } 08868 } 08869 #endif /* NO_HANDSHAKE_DONE_CB */ 08870 08871 if (!ssl->options.keepResources) { 08872 FreeHandshakeResources(ssl); 08873 } 08874 08875 WOLFSSL_LEAVE("SSL_accept()", WOLFSSL_SUCCESS); 08876 return WOLFSSL_SUCCESS; 08877 08878 default : 08879 WOLFSSL_MSG("Unknown accept state ERROR"); 08880 return WOLFSSL_FATAL_ERROR; 08881 } 08882 } 08883 #endif 08884 08885 #ifdef WOLFSSL_EARLY_DATA 08886 /* Sets the maximum amount of early data that can be seen by server when using 08887 * session tickets for resumption. 08888 * A value of zero indicates no early data is to be sent by client using session 08889 * tickets. 08890 * 08891 * ctx The SSL/TLS CTX object. 08892 * sz Maximum size of the early data. 08893 * returns BAD_FUNC_ARG when ctx is NULL, SIDE_ERROR when not a server and 08894 * 0 on success. 08895 */ 08896 int wolfSSL_CTX_set_max_early_data(WOLFSSL_CTX* ctx, unsigned int sz) 08897 { 08898 if (ctx == NULL || !IsAtLeastTLSv1_3(ctx->method->version)) 08899 return BAD_FUNC_ARG; 08900 if (ctx->method->side == WOLFSSL_CLIENT_END) 08901 return SIDE_ERROR; 08902 08903 ctx->maxEarlyDataSz = sz; 08904 08905 return 0; 08906 } 08907 08908 /* Sets the maximum amount of early data that can be seen by server when using 08909 * session tickets for resumption. 08910 * A value of zero indicates no early data is to be sent by client using session 08911 * tickets. 08912 * 08913 * ssl The SSL/TLS object. 08914 * sz Maximum size of the early data. 08915 * returns BAD_FUNC_ARG when ssl is NULL, or not using TLS v1.3, 08916 * SIDE_ERROR when not a server and 0 on success. 08917 */ 08918 int wolfSSL_set_max_early_data(WOLFSSL* ssl, unsigned int sz) 08919 { 08920 if (ssl == NULL || !IsAtLeastTLSv1_3(ssl->version)) 08921 return BAD_FUNC_ARG; 08922 if (ssl->options.side == WOLFSSL_CLIENT_END) 08923 return SIDE_ERROR; 08924 08925 ssl->options.maxEarlyDataSz = sz; 08926 08927 return 0; 08928 } 08929 08930 /* Write early data to the server. 08931 * 08932 * ssl The SSL/TLS object. 08933 * data Early data to write 08934 * sz The size of the eary data in bytes. 08935 * outSz The number of early data bytes written. 08936 * returns BAD_FUNC_ARG when: ssl, data or outSz is NULL; sz is negative; 08937 * or not using TLS v1.3. SIDE ERROR when not a server. Otherwise the number of 08938 * early data bytes written. 08939 */ 08940 int wolfSSL_write_early_data(WOLFSSL* ssl, const void* data, int sz, int* outSz) 08941 { 08942 int ret = 0; 08943 08944 WOLFSSL_ENTER("SSL_write_early_data()"); 08945 08946 if (ssl == NULL || data == NULL || sz < 0 || outSz == NULL) 08947 return BAD_FUNC_ARG; 08948 if (!IsAtLeastTLSv1_3(ssl->version)) 08949 return BAD_FUNC_ARG; 08950 08951 #ifndef NO_WOLFSSL_CLIENT 08952 if (ssl->options.side == WOLFSSL_SERVER_END) 08953 return SIDE_ERROR; 08954 08955 if (ssl->options.handShakeState == NULL_STATE) { 08956 ssl->earlyData = expecting_early_data; 08957 ret = wolfSSL_connect_TLSv13(ssl); 08958 if (ret != WOLFSSL_SUCCESS) 08959 return WOLFSSL_FATAL_ERROR; 08960 } 08961 if (ssl->options.handShakeState == CLIENT_HELLO_COMPLETE) { 08962 ret = SendData(ssl, data, sz); 08963 if (ret > 0) 08964 *outSz = ret; 08965 } 08966 #else 08967 return SIDE_ERROR; 08968 #endif 08969 08970 WOLFSSL_LEAVE("SSL_write_early_data()", ret); 08971 08972 if (ret < 0) 08973 ret = WOLFSSL_FATAL_ERROR; 08974 return ret; 08975 } 08976 08977 /* Read the any early data from the client. 08978 * 08979 * ssl The SSL/TLS object. 08980 * data Buffer to put the early data into. 08981 * sz The size of the buffer in bytes. 08982 * outSz The number of early data bytes read. 08983 * returns BAD_FUNC_ARG when: ssl, data or outSz is NULL; sz is negative; 08984 * or not using TLS v1.3. SIDE ERROR when not a server. Otherwise the number of 08985 * early data bytes read. 08986 */ 08987 int wolfSSL_read_early_data(WOLFSSL* ssl, void* data, int sz, int* outSz) 08988 { 08989 int ret = 0; 08990 08991 WOLFSSL_ENTER("wolfSSL_read_early_data()"); 08992 08993 08994 if (ssl == NULL || data == NULL || sz < 0 || outSz == NULL) 08995 return BAD_FUNC_ARG; 08996 if (!IsAtLeastTLSv1_3(ssl->version)) 08997 return BAD_FUNC_ARG; 08998 08999 #ifndef NO_WOLFSSL_SERVER 09000 if (ssl->options.side == WOLFSSL_CLIENT_END) 09001 return SIDE_ERROR; 09002 09003 if (ssl->options.handShakeState == NULL_STATE) { 09004 ssl->earlyData = expecting_early_data; 09005 ret = wolfSSL_accept_TLSv13(ssl); 09006 if (ret <= 0) 09007 return WOLFSSL_FATAL_ERROR; 09008 } 09009 if (ssl->options.handShakeState == SERVER_FINISHED_COMPLETE) { 09010 ret = ReceiveData(ssl, (byte*)data, sz, FALSE); 09011 if (ret > 0) 09012 *outSz = ret; 09013 if (ssl->error == ZERO_RETURN) 09014 ssl->error = WOLFSSL_ERROR_NONE; 09015 } 09016 else 09017 ret = 0; 09018 #else 09019 return SIDE_ERROR; 09020 #endif 09021 09022 WOLFSSL_LEAVE("wolfSSL_read_early_data()", ret); 09023 09024 if (ret < 0) 09025 ret = WOLFSSL_FATAL_ERROR; 09026 return ret; 09027 } 09028 #endif 09029 09030 #ifdef HAVE_SECRET_CALLBACK 09031 int wolfSSL_set_tls13_secret_cb(WOLFSSL* ssl, Tls13SecretCb cb, void* ctx) 09032 { 09033 WOLFSSL_ENTER("wolfSSL_set_tls13_secret_cb"); 09034 if (ssl == NULL) 09035 return WOLFSSL_FATAL_ERROR; 09036 09037 ssl->tls13SecretCb = cb; 09038 ssl->tls13SecretCtx = ctx; 09039 09040 return WOLFSSL_SUCCESS; 09041 } 09042 #endif 09043 09044 #undef ERROR_OUT 09045 09046 #endif /* !WOLFCRYPT_ONLY */ 09047 09048 #endif /* WOLFSSL_TLS13 */ 09049
Generated on Tue Jul 12 2022 20:59:07 by
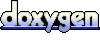