wolfSSL SSL/TLS library, support up to TLS1.3
Dependents: CyaSSL-Twitter-OAuth4Tw Example-client-tls-cert TwitterReader TweetTest ... more
srp.h
00001 /* srp.h 00002 * 00003 * Copyright (C) 2006-2020 wolfSSL Inc. 00004 * 00005 * This file is part of wolfSSL. 00006 * 00007 * wolfSSL is free software; you can redistribute it and/or modify 00008 * it under the terms of the GNU General Public License as published by 00009 * the Free Software Foundation; either version 2 of the License, or 00010 * (at your option) any later version. 00011 * 00012 * wolfSSL is distributed in the hope that it will be useful, 00013 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00014 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00015 * GNU General Public License for more details. 00016 * 00017 * You should have received a copy of the GNU General Public License 00018 * along with this program; if not, write to the Free Software 00019 * Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1335, USA 00020 */ 00021 00022 /*! 00023 \file wolfssl/wolfcrypt/srp.h 00024 */ 00025 00026 #ifdef WOLFCRYPT_HAVE_SRP 00027 00028 #ifndef WOLFCRYPT_SRP_H 00029 #define WOLFCRYPT_SRP_H 00030 00031 #include <wolfssl/wolfcrypt/types.h > 00032 #include <wolfssl/wolfcrypt/sha.h > 00033 #include <wolfssl/wolfcrypt/sha256.h > 00034 #include <wolfssl/wolfcrypt/sha512.h > 00035 #include <wolfssl/wolfcrypt/integer.h> 00036 00037 #ifdef __cplusplus 00038 extern "C" { 00039 #endif 00040 00041 /* Select the largest available hash for the buffer size. */ 00042 #if defined(WOLFSSL_SHA512) 00043 #define SRP_MAX_DIGEST_SIZE WC_SHA512_DIGEST_SIZE 00044 #elif defined(WOLFSSL_SHA384) 00045 #define SRP_MAX_DIGEST_SIZE WC_SHA384_DIGEST_SIZE 00046 #elif !defined(NO_SHA256) 00047 #define SRP_MAX_DIGEST_SIZE WC_SHA256_DIGEST_SIZE 00048 #elif !defined(NO_SHA) 00049 #define SRP_MAX_DIGEST_SIZE WC_SHA_DIGEST_SIZE 00050 #else 00051 #error "You have to have some kind of SHA hash if you want to use SRP." 00052 #endif 00053 00054 /* Set the minimum number of bits acceptable in an SRP modulus */ 00055 #define SRP_MODULUS_MIN_BITS 512 00056 00057 /* Set the minimum number of bits acceptable for private keys (RFC 5054) */ 00058 #define SRP_PRIVATE_KEY_MIN_BITS 256 00059 00060 /* salt size for SRP password */ 00061 #define SRP_SALT_SIZE 16 00062 00063 /** 00064 * SRP side, client or server. 00065 */ 00066 typedef enum { 00067 SRP_CLIENT_SIDE = 0, 00068 SRP_SERVER_SIDE = 1, 00069 } SrpSide; 00070 00071 /** 00072 * SRP hash type, SHA[1|256|384|512]. 00073 */ 00074 typedef enum { 00075 SRP_TYPE_SHA = 1, 00076 SRP_TYPE_SHA256 = 2, 00077 SRP_TYPE_SHA384 = 3, 00078 SRP_TYPE_SHA512 = 4, 00079 } SrpType; 00080 00081 00082 /** 00083 * SRP hash struct. 00084 */ 00085 typedef struct { 00086 byte type; 00087 union { 00088 #ifndef NO_SHA 00089 wc_Sha sha; 00090 #endif 00091 #ifndef NO_SHA256 00092 wc_Sha256 sha256; 00093 #endif 00094 #ifdef WOLFSSL_SHA384 00095 wc_Sha384 sha384; 00096 #endif 00097 #ifdef WOLFSSL_SHA512 00098 wc_Sha512 sha512; 00099 #endif 00100 } data; 00101 } SrpHash; 00102 00103 typedef struct Srp { 00104 SrpSide side; /**< Client or Server, @see SrpSide. */ 00105 SrpType type; /**< Hash type, @see SrpType. */ 00106 byte* user; /**< Username, login. */ 00107 word32 userSz; /**< Username length. */ 00108 byte* salt; /**< Small salt. */ 00109 word32 saltSz; /**< Salt length. */ 00110 mp_int N; /**< Modulus. N = 2q+1, [q, N] are primes.*/ 00111 mp_int g; /**< Generator. A generator modulo N. */ 00112 byte k[SRP_MAX_DIGEST_SIZE]; /**< Multiplier parameter. k = H(N, g) */ 00113 mp_int auth; /**< Client: x = H(salt + H(user:pswd)) */ 00114 /**< Server: v = g ^ x % N */ 00115 mp_int priv; /**< Private ephemeral value. */ 00116 SrpHash client_proof; /**< Client proof. Sent to the Server. */ 00117 SrpHash server_proof; /**< Server proof. Sent to the Client. */ 00118 byte* key; /**< Session key. */ 00119 word32 keySz; /**< Session key length. */ 00120 int (*keyGenFunc_cb) (struct Srp* srp, byte* secret, word32 size); 00121 /**< Function responsible for generating the session key. */ 00122 /**< It MUST use XMALLOC with type DYNAMIC_TYPE_SRP to allocate the */ 00123 /**< key buffer for this structure and set keySz to the buffer size. */ 00124 /**< The default function used by this implementation is a modified */ 00125 /**< version of t_mgf1 that uses the proper hash function according */ 00126 /**< to srp->type. */ 00127 void* heap; /**< heap hint pointer */ 00128 } Srp; 00129 00130 /** 00131 * Initializes the Srp struct for usage. 00132 * 00133 * @param[out] srp the Srp structure to be initialized. 00134 * @param[in] type the hash type to be used. 00135 * @param[in] side the side of the communication. 00136 * 00137 * @return 0 on success, {@literal <} 0 on error. @see error-crypt.h 00138 */ 00139 WOLFSSL_API int wc_SrpInit(Srp* srp, SrpType type, SrpSide side); 00140 00141 /** 00142 * Releases the Srp struct resources after usage. 00143 * 00144 * @param[in,out] srp the Srp structure to be terminated. 00145 */ 00146 WOLFSSL_API void wc_SrpTerm(Srp* srp); 00147 00148 /** 00149 * Sets the username. 00150 * 00151 * This function MUST be called after wc_SrpInit. 00152 * 00153 * @param[in,out] srp the Srp structure. 00154 * @param[in] username the buffer containing the username. 00155 * @param[in] size the username size in bytes 00156 * 00157 * @return 0 on success, {@literal <} 0 on error. @see error-crypt.h 00158 */ 00159 WOLFSSL_API int wc_SrpSetUsername(Srp* srp, const byte* username, word32 size); 00160 00161 00162 /** 00163 * Sets the srp parameters based on the username. 00164 * 00165 * This function MUST be called after wc_SrpSetUsername. 00166 * 00167 * @param[in,out] srp the Srp structure. 00168 * @param[in] N the Modulus. N = 2q+1, [q, N] are primes. 00169 * @param[in] nSz the N size in bytes. 00170 * @param[in] g the Generator modulo N. 00171 * @param[in] gSz the g size in bytes 00172 * @param[in] salt a small random salt. Specific for each username. 00173 * @param[in] saltSz the salt size in bytes 00174 * 00175 * @return 0 on success, {@literal <} 0 on error. @see error-crypt.h 00176 */ 00177 WOLFSSL_API int wc_SrpSetParams(Srp* srp, const byte* N, word32 nSz, 00178 const byte* g, word32 gSz, 00179 const byte* salt, word32 saltSz); 00180 00181 /** 00182 * Sets the password. 00183 * 00184 * Setting the password does not persists the clear password data in the 00185 * srp structure. The client calculates x = H(salt + H(user:pswd)) and stores 00186 * it in the auth field. 00187 * 00188 * This function MUST be called after wc_SrpSetParams and is CLIENT SIDE ONLY. 00189 * 00190 * @param[in,out] srp the Srp structure. 00191 * @param[in] password the buffer containing the password. 00192 * @param[in] size the password size in bytes. 00193 * 00194 * @return 0 on success, {@literal <} 0 on error. @see error-crypt.h 00195 */ 00196 WOLFSSL_API int wc_SrpSetPassword(Srp* srp, const byte* password, word32 size); 00197 00198 /** 00199 * Sets the verifier. 00200 * 00201 * This function MUST be called after wc_SrpSetParams and is SERVER SIDE ONLY. 00202 * 00203 * @param[in,out] srp the Srp structure. 00204 * @param[in] verifier the buffer containing the verifier. 00205 * @param[in] size the verifier size in bytes. 00206 * 00207 * @return 0 on success, {@literal <} 0 on error. @see error-crypt.h 00208 */ 00209 WOLFSSL_API int wc_SrpSetVerifier(Srp* srp, const byte* verifier, word32 size); 00210 00211 /** 00212 * Gets the verifier. 00213 * 00214 * The client calculates the verifier with v = g ^ x % N. 00215 * This function MAY be called after wc_SrpSetPassword and is CLIENT SIDE ONLY. 00216 * 00217 * @param[in,out] srp the Srp structure. 00218 * @param[out] verifier the buffer to write the verifier. 00219 * @param[in,out] size the buffer size in bytes. Will be updated with the 00220 * verifier size. 00221 * 00222 * @return 0 on success, {@literal <} 0 on error. @see error-crypt.h 00223 */ 00224 WOLFSSL_API int wc_SrpGetVerifier(Srp* srp, byte* verifier, word32* size); 00225 00226 /** 00227 * Sets the private ephemeral value. 00228 * 00229 * The private ephemeral value is known as: 00230 * a at the client side. a = random() 00231 * b at the server side. b = random() 00232 * This function is handy for unit test cases or if the developer wants to use 00233 * an external random source to set the ephemeral value. 00234 * This function MAY be called before wc_SrpGetPublic. 00235 * 00236 * @param[in,out] srp the Srp structure. 00237 * @param[in] priv the ephemeral value. 00238 * @param[in] size the private size in bytes. 00239 * 00240 * @return 0 on success, {@literal <} 0 on error. @see error-crypt.h 00241 */ 00242 WOLFSSL_API int wc_SrpSetPrivate(Srp* srp, const byte* priv, word32 size); 00243 00244 /** 00245 * Gets the public ephemeral value. 00246 * 00247 * The public ephemeral value is known as: 00248 * A at the client side. A = g ^ a % N 00249 * B at the server side. B = (k * v + (g ˆ b % N)) % N 00250 * This function MUST be called after wc_SrpSetPassword or wc_SrpSetVerifier. 00251 * 00252 * @param[in,out] srp the Srp structure. 00253 * @param[out] pub the buffer to write the public ephemeral value. 00254 * @param[in,out] size the the buffer size in bytes. Will be updated with 00255 * the ephemeral value size. 00256 * 00257 * @return 0 on success, {@literal <} 0 on error. @see error-crypt.h 00258 */ 00259 WOLFSSL_API int wc_SrpGetPublic(Srp* srp, byte* pub, word32* size); 00260 00261 00262 /** 00263 * Computes the session key. 00264 * 00265 * The key can be accessed at srp->key after success. 00266 * 00267 * @param[in,out] srp the Srp structure. 00268 * @param[in] clientPubKey the client's public ephemeral value. 00269 * @param[in] clientPubKeySz the client's public ephemeral value size. 00270 * @param[in] serverPubKey the server's public ephemeral value. 00271 * @param[in] serverPubKeySz the server's public ephemeral value size. 00272 * 00273 * @return 0 on success, {@literal <} 0 on error. @see error-crypt.h 00274 */ 00275 WOLFSSL_API int wc_SrpComputeKey(Srp* srp, 00276 byte* clientPubKey, word32 clientPubKeySz, 00277 byte* serverPubKey, word32 serverPubKeySz); 00278 00279 /** 00280 * Gets the proof. 00281 * 00282 * This function MUST be called after wc_SrpComputeKey. 00283 * 00284 * @param[in,out] srp the Srp structure. 00285 * @param[out] proof the buffer to write the proof. 00286 * @param[in,out] size the buffer size in bytes. Will be updated with the 00287 * proof size. 00288 * 00289 * @return 0 on success, {@literal <} 0 on error. @see error-crypt.h 00290 */ 00291 WOLFSSL_API int wc_SrpGetProof(Srp* srp, byte* proof, word32* size); 00292 00293 /** 00294 * Verifies the peers proof. 00295 * 00296 * This function MUST be called before wc_SrpGetSessionKey. 00297 * 00298 * @param[in,out] srp the Srp structure. 00299 * @param[in] proof the peers proof. 00300 * @param[in] size the proof size in bytes. 00301 * 00302 * @return 0 on success, {@literal <} 0 on error. @see error-crypt.h 00303 */ 00304 WOLFSSL_API int wc_SrpVerifyPeersProof(Srp* srp, byte* proof, word32 size); 00305 00306 #ifdef __cplusplus 00307 } /* extern "C" */ 00308 #endif 00309 00310 #endif /* WOLFCRYPT_SRP_H */ 00311 #endif /* WOLFCRYPT_HAVE_SRP */ 00312
Generated on Tue Jul 12 2022 20:58:52 by
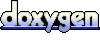