wolfSSL SSL/TLS library, support up to TLS1.3
Dependents: CyaSSL-Twitter-OAuth4Tw Example-client-tls-cert TwitterReader TweetTest ... more
sniffer.h
00001 /* sniffer.h 00002 * 00003 * Copyright (C) 2006-2020 wolfSSL Inc. 00004 * 00005 * This file is part of wolfSSL. 00006 * 00007 * wolfSSL is free software; you can redistribute it and/or modify 00008 * it under the terms of the GNU General Public License as published by 00009 * the Free Software Foundation; either version 2 of the License, or 00010 * (at your option) any later version. 00011 * 00012 * wolfSSL is distributed in the hope that it will be useful, 00013 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00014 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00015 * GNU General Public License for more details. 00016 * 00017 * You should have received a copy of the GNU General Public License 00018 * along with this program; if not, write to the Free Software 00019 * Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1335, USA 00020 */ 00021 00022 00023 00024 #ifndef WOLFSSL_SNIFFER_H 00025 #define WOLFSSL_SNIFFER_H 00026 00027 #include <wolfssl/wolfcrypt/settings.h> 00028 00029 #ifdef _WIN32 00030 #ifdef SSL_SNIFFER_EXPORTS 00031 #define SSL_SNIFFER_API __declspec(dllexport) 00032 #else 00033 #define SSL_SNIFFER_API __declspec(dllimport) 00034 #endif 00035 #else 00036 #define SSL_SNIFFER_API 00037 #endif /* _WIN32 */ 00038 00039 00040 #ifdef __cplusplus 00041 extern "C" { 00042 #endif 00043 00044 /* @param typeK: (formerly keyType) was shadowing a global declaration in 00045 * wolfssl/wolfcrypt/asn.h line 175 00046 */ 00047 WOLFSSL_API 00048 SSL_SNIFFER_API int ssl_SetPrivateKey(const char* address, int port, 00049 const char* keyFile, int typeK, 00050 const char* password, char* error); 00051 00052 WOLFSSL_API 00053 SSL_SNIFFER_API int ssl_SetNamedPrivateKey(const char* name, 00054 const char* address, int port, 00055 const char* keyFile, int typeK, 00056 const char* password, char* error); 00057 00058 WOLFSSL_API 00059 SSL_SNIFFER_API int ssl_DecodePacket(const unsigned char* packet, int length, 00060 unsigned char** data, char* error); 00061 00062 WOLFSSL_API 00063 SSL_SNIFFER_API int ssl_FreeDecodeBuffer(unsigned char** data, char* error); 00064 00065 WOLFSSL_API 00066 SSL_SNIFFER_API int ssl_FreeZeroDecodeBuffer(unsigned char** data, int sz, 00067 char* error); 00068 00069 WOLFSSL_API 00070 SSL_SNIFFER_API int ssl_Trace(const char* traceFile, char* error); 00071 00072 WOLFSSL_API 00073 SSL_SNIFFER_API int ssl_EnableRecovery(int onOff, int maxMemory, char* error); 00074 00075 WOLFSSL_API 00076 SSL_SNIFFER_API int ssl_GetSessionStats(unsigned int* active, 00077 unsigned int* total, 00078 unsigned int* peak, 00079 unsigned int* maxSessions, 00080 unsigned int* missedData, 00081 unsigned int* reassemblyMemory, 00082 char* error); 00083 00084 WOLFSSL_API void ssl_InitSniffer(void); 00085 00086 WOLFSSL_API void ssl_FreeSniffer(void); 00087 00088 00089 /* ssl_SetPrivateKey typeKs */ 00090 enum { 00091 FILETYPE_PEM = 1, 00092 FILETYPE_DER = 2, 00093 }; 00094 00095 00096 /* 00097 * New Sniffer API that provides read-only access to the TLS and cipher 00098 * information associated with the SSL session. 00099 */ 00100 00101 typedef struct SSLInfo 00102 { 00103 unsigned char isValid; 00104 /* indicates if the info in this struct is valid: 0 = no, 1 = yes */ 00105 unsigned char protocolVersionMajor; /* SSL Version: major */ 00106 unsigned char protocolVersionMinor; /* SSL Version: minor */ 00107 unsigned char serverCipherSuite0; /* first byte, normally 0 */ 00108 unsigned char serverCipherSuite; /* second byte, actual suite */ 00109 unsigned char serverCipherSuiteName[256]; 00110 /* cipher name, e.g., "TLS_RSA_..." */ 00111 unsigned char serverNameIndication[128]; 00112 unsigned int keySize; 00113 } SSLInfo; 00114 00115 00116 WOLFSSL_API 00117 SSL_SNIFFER_API int ssl_DecodePacketWithSessionInfo( 00118 const unsigned char* packet, int length, 00119 unsigned char** data, SSLInfo* sslInfo, char* error); 00120 00121 typedef void (*SSLConnCb)(const void* session, SSLInfo* info, void* ctx); 00122 00123 WOLFSSL_API 00124 SSL_SNIFFER_API int ssl_SetConnectionCb(SSLConnCb cb); 00125 00126 WOLFSSL_API 00127 SSL_SNIFFER_API int ssl_SetConnectionCtx(void* ctx); 00128 00129 00130 typedef struct SSLStats 00131 { 00132 unsigned long int sslStandardConns; 00133 unsigned long int sslClientAuthConns; 00134 unsigned long int sslResumedConns; 00135 unsigned long int sslEphemeralMisses; 00136 unsigned long int sslResumeMisses; 00137 unsigned long int sslCiphersUnsupported; 00138 unsigned long int sslKeysUnmatched; 00139 unsigned long int sslKeyFails; 00140 unsigned long int sslDecodeFails; 00141 unsigned long int sslAlerts; 00142 unsigned long int sslDecryptedBytes; 00143 unsigned long int sslEncryptedBytes; 00144 unsigned long int sslEncryptedPackets; 00145 unsigned long int sslDecryptedPackets; 00146 unsigned long int sslKeyMatches; 00147 unsigned long int sslEncryptedConns; 00148 00149 unsigned long int sslResumptionValid; 00150 unsigned long int sslResumptionInserts; 00151 } SSLStats; 00152 00153 00154 WOLFSSL_API 00155 SSL_SNIFFER_API int ssl_ResetStatistics(void); 00156 00157 00158 WOLFSSL_API 00159 SSL_SNIFFER_API int ssl_ReadStatistics(SSLStats* stats); 00160 00161 00162 WOLFSSL_API 00163 SSL_SNIFFER_API int ssl_ReadResetStatistics(SSLStats* stats); 00164 00165 00166 typedef int (*SSLWatchCb)(void* vSniffer, 00167 const unsigned char* certHash, 00168 unsigned int certHashSz, 00169 const unsigned char* certChain, 00170 unsigned int certChainSz, 00171 void* ctx, char* error); 00172 00173 WOLFSSL_API 00174 SSL_SNIFFER_API int ssl_SetWatchKeyCallback(SSLWatchCb cb, char* error); 00175 00176 WOLFSSL_API 00177 SSL_SNIFFER_API int ssl_SetWatchKeyCallback_ex(SSLWatchCb cb, int devId, 00178 char* error); 00179 00180 WOLFSSL_API 00181 SSL_SNIFFER_API int ssl_SetWatchKeyCtx(void* ctx, char* error); 00182 00183 WOLFSSL_API 00184 SSL_SNIFFER_API int ssl_SetWatchKey_buffer(void* vSniffer, 00185 const unsigned char* key, unsigned int keySz, 00186 int keyType, char* error); 00187 00188 WOLFSSL_API 00189 SSL_SNIFFER_API int ssl_SetWatchKey_file(void* vSniffer, 00190 const char* keyFile, int keyType, 00191 const char* password, char* error); 00192 00193 00194 typedef int (*SSLStoreDataCb)(const unsigned char* decryptBuf, 00195 unsigned int decryptBufSz, unsigned int decryptBufOffset, void* ctx); 00196 00197 WOLFSSL_API 00198 SSL_SNIFFER_API int ssl_SetStoreDataCallback(SSLStoreDataCb cb); 00199 00200 WOLFSSL_API 00201 SSL_SNIFFER_API int ssl_DecodePacketWithSessionInfoStoreData( 00202 const unsigned char* packet, int length, void* ctx, 00203 SSLInfo* sslInfo, char* error); 00204 00205 00206 WOLFSSL_API 00207 SSL_SNIFFER_API int ssl_DecodePacketWithChain(void* vChain, 00208 unsigned int chainSz, unsigned char** data, char* error); 00209 00210 00211 WOLFSSL_API 00212 SSL_SNIFFER_API int ssl_DecodePacketWithChainSessionInfoStoreData( 00213 void* vChain, unsigned int chainSz, void* ctx, SSLInfo* sslInfo, 00214 char* error); 00215 00216 #ifdef __cplusplus 00217 } /* extern "C" */ 00218 #endif 00219 00220 #endif /* wolfSSL_SNIFFER_H */ 00221 00222
Generated on Tue Jul 12 2022 20:58:42 by
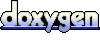