wolfSSL SSL/TLS library, support up to TLS1.3
Dependents: CyaSSL-Twitter-OAuth4Tw Example-client-tls-cert TwitterReader TweetTest ... more
poly1305.h
00001 /* poly1305.h 00002 * 00003 * Copyright (C) 2006-2020 wolfSSL Inc. 00004 * 00005 * This file is part of wolfSSL. 00006 * 00007 * wolfSSL is free software; you can redistribute it and/or modify 00008 * it under the terms of the GNU General Public License as published by 00009 * the Free Software Foundation; either version 2 of the License, or 00010 * (at your option) any later version. 00011 * 00012 * wolfSSL is distributed in the hope that it will be useful, 00013 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00014 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00015 * GNU General Public License for more details. 00016 * 00017 * You should have received a copy of the GNU General Public License 00018 * along with this program; if not, write to the Free Software 00019 * Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1335, USA 00020 */ 00021 00022 /*! 00023 \file wolfssl/wolfcrypt/poly1305.h 00024 */ 00025 00026 #ifndef WOLF_CRYPT_POLY1305_H 00027 #define WOLF_CRYPT_POLY1305_H 00028 00029 #include <wolfssl/wolfcrypt/types.h > 00030 00031 #ifdef HAVE_POLY1305 00032 00033 #ifdef __cplusplus 00034 extern "C" { 00035 #endif 00036 00037 /* auto detect between 32bit / 64bit */ 00038 #if defined(__SIZEOF_INT128__) && defined(__LP64__) 00039 #define WC_HAS_SIZEOF_INT128_64BIT 00040 #endif 00041 00042 #if defined(_MSC_VER) && defined(_M_X64) 00043 #define WC_HAS_MSVC_64BIT 00044 #endif 00045 00046 #if (defined(__GNUC__) && defined(__LP64__) && \ 00047 ((__GNUC__ > 4) || ((__GNUC__ == 4) && (__GNUC_MINOR__ >= 4)))) 00048 #define WC_HAS_GCC_4_4_64BIT 00049 #endif 00050 00051 #ifdef USE_INTEL_SPEEDUP 00052 #elif (defined(WC_HAS_SIZEOF_INT128_64BIT) || defined(WC_HAS_MSVC_64BIT) || \ 00053 defined(WC_HAS_GCC_4_4_64BIT)) 00054 #define POLY130564 00055 #else 00056 #define POLY130532 00057 #endif 00058 00059 enum { 00060 POLY1305 = 7, 00061 POLY1305_BLOCK_SIZE = 16, 00062 POLY1305_DIGEST_SIZE = 16, 00063 }; 00064 00065 #define WC_POLY1305_PAD_SZ 16 00066 #define WC_POLY1305_MAC_SZ 16 00067 00068 /* Poly1305 state */ 00069 typedef struct Poly1305 { 00070 #ifdef USE_INTEL_SPEEDUP 00071 word64 r[3]; 00072 word64 h[3]; 00073 word64 pad[2]; 00074 word64 hh[20]; 00075 word32 r1[8]; 00076 word32 r2[8]; 00077 word32 r3[8]; 00078 word32 r4[8]; 00079 word64 hm[16]; 00080 unsigned char buffer[8*POLY1305_BLOCK_SIZE]; 00081 size_t leftover; 00082 unsigned char finished; 00083 unsigned char started; 00084 #else 00085 #if defined(WOLFSSL_ARMASM) && defined(__aarch64__) 00086 ALIGN128 word32 r[5]; 00087 ALIGN128 word32 r_2[5]; // r^2 00088 ALIGN128 word32 r_4[5]; // r^4 00089 ALIGN128 word32 h[5]; 00090 word32 pad[4]; 00091 word64 leftover; 00092 #else 00093 #if defined(POLY130564) 00094 word64 r[3]; 00095 word64 h[3]; 00096 word64 pad[2]; 00097 #else 00098 word32 r[5]; 00099 word32 h[5]; 00100 word32 pad[4]; 00101 #endif 00102 size_t leftover; 00103 #endif /* WOLFSSL_ARMASM */ 00104 unsigned char buffer[POLY1305_BLOCK_SIZE]; 00105 unsigned char finished; 00106 #endif 00107 } Poly1305; 00108 00109 /* does init */ 00110 00111 WOLFSSL_API int wc_Poly1305SetKey(Poly1305* poly1305, const byte* key, 00112 word32 kySz); 00113 WOLFSSL_API int wc_Poly1305Update(Poly1305* poly1305, const byte*, word32); 00114 WOLFSSL_API int wc_Poly1305Final(Poly1305* poly1305, byte* tag); 00115 00116 /* AEAD Functions */ 00117 WOLFSSL_API int wc_Poly1305_Pad(Poly1305* ctx, word32 lenToPad); 00118 WOLFSSL_API int wc_Poly1305_EncodeSizes(Poly1305* ctx, word32 aadSz, word32 dataSz); 00119 WOLFSSL_API int wc_Poly1305_MAC(Poly1305* ctx, byte* additional, word32 addSz, 00120 byte* input, word32 sz, byte* tag, word32 tagSz); 00121 00122 void poly1305_block(Poly1305* ctx, const unsigned char *m); 00123 void poly1305_blocks(Poly1305* ctx, const unsigned char *m, 00124 size_t bytes); 00125 #ifdef __cplusplus 00126 } /* extern "C" */ 00127 #endif 00128 00129 #endif /* HAVE_POLY1305 */ 00130 #endif /* WOLF_CRYPT_POLY1305_H */ 00131
Generated on Tue Jul 12 2022 20:58:41 by
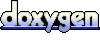