wolfSSL SSL/TLS library, support up to TLS1.3
Dependents: CyaSSL-Twitter-OAuth4Tw Example-client-tls-cert TwitterReader TweetTest ... more
misc.h
00001 /* misc.h 00002 * 00003 * Copyright (C) 2006-2020 wolfSSL Inc. 00004 * 00005 * This file is part of wolfSSL. 00006 * 00007 * wolfSSL is free software; you can redistribute it and/or modify 00008 * it under the terms of the GNU General Public License as published by 00009 * the Free Software Foundation; either version 2 of the License, or 00010 * (at your option) any later version. 00011 * 00012 * wolfSSL is distributed in the hope that it will be useful, 00013 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00014 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00015 * GNU General Public License for more details. 00016 * 00017 * You should have received a copy of the GNU General Public License 00018 * along with this program; if not, write to the Free Software 00019 * Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1335, USA 00020 */ 00021 00022 00023 00024 #ifndef WOLF_CRYPT_MISC_H 00025 #define WOLF_CRYPT_MISC_H 00026 00027 00028 #include <wolfssl/wolfcrypt/types.h > 00029 00030 00031 #ifdef __cplusplus 00032 extern "C" { 00033 #endif 00034 00035 00036 #ifdef NO_INLINE 00037 WOLFSSL_LOCAL 00038 word32 rotlFixed(word32, word32); 00039 WOLFSSL_LOCAL 00040 word32 rotrFixed(word32, word32); 00041 00042 WOLFSSL_LOCAL 00043 word32 ByteReverseWord32(word32); 00044 WOLFSSL_LOCAL 00045 void ByteReverseWords(word32*, const word32*, word32); 00046 00047 WOLFSSL_LOCAL 00048 void XorWords(wolfssl_word*, const wolfssl_word*, word32); 00049 WOLFSSL_LOCAL 00050 void xorbuf(void*, const void*, word32); 00051 00052 WOLFSSL_LOCAL 00053 void ForceZero(const void*, word32); 00054 00055 WOLFSSL_LOCAL 00056 int ConstantCompare(const byte*, const byte*, int); 00057 00058 #ifdef WORD64_AVAILABLE 00059 WOLFSSL_LOCAL 00060 word64 rotlFixed64(word64, word64); 00061 WOLFSSL_LOCAL 00062 word64 rotrFixed64(word64, word64); 00063 00064 WOLFSSL_LOCAL 00065 word64 ByteReverseWord64(word64); 00066 WOLFSSL_LOCAL 00067 void ByteReverseWords64(word64*, const word64*, word32); 00068 #endif /* WORD64_AVAILABLE */ 00069 00070 #ifndef WOLFSSL_HAVE_MIN 00071 #if defined(HAVE_FIPS) && !defined(min) /* so ifdef check passes */ 00072 #define min min 00073 #endif 00074 WOLFSSL_LOCAL word32 min(word32 a, word32 b); 00075 #endif 00076 00077 #ifndef WOLFSSL_HAVE_MAX 00078 #if defined(HAVE_FIPS) && !defined(max) /* so ifdef check passes */ 00079 #define max max 00080 #endif 00081 WOLFSSL_LOCAL word32 max(word32 a, word32 b); 00082 #endif /* WOLFSSL_HAVE_MAX */ 00083 00084 00085 void c32to24(word32 in, word24 out); 00086 void c16toa(word16 u16, byte* c); 00087 void c32toa(word32 u32, byte* c); 00088 void c24to32(const word24 u24, word32* u32); 00089 void ato16(const byte* c, word16* u16); 00090 void ato24(const byte* c, word32* u24); 00091 void ato32(const byte* c, word32* u32); 00092 word32 btoi(byte b); 00093 00094 00095 WOLFSSL_LOCAL byte ctMaskGT(int a, int b); 00096 WOLFSSL_LOCAL byte ctMaskGTE(int a, int b); 00097 WOLFSSL_LOCAL int ctMaskIntGTE(int a, int b); 00098 WOLFSSL_LOCAL byte ctMaskLT(int a, int b); 00099 WOLFSSL_LOCAL byte ctMaskLTE(int a, int b); 00100 WOLFSSL_LOCAL byte ctMaskEq(int a, int b); 00101 WOLFSSL_LOCAL word16 ctMask16GT(int a, int b); 00102 WOLFSSL_LOCAL word16 ctMask16LT(int a, int b); 00103 WOLFSSL_LOCAL word16 ctMask16Eq(int a, int b); 00104 WOLFSSL_LOCAL byte ctMaskNotEq(int a, int b); 00105 WOLFSSL_LOCAL byte ctMaskSel(byte m, byte a, byte b); 00106 WOLFSSL_LOCAL int ctMaskSelInt(byte m, int a, int b); 00107 WOLFSSL_LOCAL byte ctSetLTE(int a, int b); 00108 00109 #endif /* NO_INLINE */ 00110 00111 00112 #ifdef __cplusplus 00113 } /* extern "C" */ 00114 #endif 00115 00116 00117 #endif /* WOLF_CRYPT_MISC_H */ 00118 00119
Generated on Tue Jul 12 2022 20:58:40 by
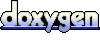