wolfSSL SSL/TLS library, support up to TLS1.3
Dependents: CyaSSL-Twitter-OAuth4Tw Example-client-tls-cert TwitterReader TweetTest ... more
memory.h
00001 /* memory.h 00002 * 00003 * Copyright (C) 2006-2020 wolfSSL Inc. 00004 * 00005 * This file is part of wolfSSL. 00006 * 00007 * wolfSSL is free software; you can redistribute it and/or modify 00008 * it under the terms of the GNU General Public License as published by 00009 * the Free Software Foundation; either version 2 of the License, or 00010 * (at your option) any later version. 00011 * 00012 * wolfSSL is distributed in the hope that it will be useful, 00013 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00014 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00015 * GNU General Public License for more details. 00016 * 00017 * You should have received a copy of the GNU General Public License 00018 * along with this program; if not, write to the Free Software 00019 * Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1335, USA 00020 */ 00021 00022 00023 /* submitted by eof */ 00024 00025 /*! 00026 \file wolfssl/wolfcrypt/memory.h 00027 */ 00028 00029 #ifndef WOLFSSL_MEMORY_H 00030 #define WOLFSSL_MEMORY_H 00031 00032 #ifndef STRING_USER 00033 #include <stdlib.h> 00034 #endif 00035 #include <wolfssl/wolfcrypt/types.h > 00036 00037 #ifdef __cplusplus 00038 extern "C" { 00039 #endif 00040 00041 #ifdef WOLFSSL_FORCE_MALLOC_FAIL_TEST 00042 WOLFSSL_API void wolfSSL_SetMemFailCount(int memFailCount); 00043 #endif 00044 00045 #ifdef WOLFSSL_STATIC_MEMORY 00046 #ifdef WOLFSSL_DEBUG_MEMORY 00047 typedef void *(*wolfSSL_Malloc_cb)(size_t size, void* heap, int type, const char* func, unsigned int line); 00048 typedef void (*wolfSSL_Free_cb)(void *ptr, void* heap, int type, const char* func, unsigned int line); 00049 typedef void *(*wolfSSL_Realloc_cb)(void *ptr, size_t size, void* heap, int type, const char* func, unsigned int line); 00050 WOLFSSL_API void* wolfSSL_Malloc(size_t size, void* heap, int type, const char* func, unsigned int line); 00051 WOLFSSL_API void wolfSSL_Free(void *ptr, void* heap, int type, const char* func, unsigned int line); 00052 WOLFSSL_API void* wolfSSL_Realloc(void *ptr, size_t size, void* heap, int type, const char* func, unsigned int line); 00053 #else 00054 typedef void *(*wolfSSL_Malloc_cb)(size_t size, void* heap, int type); 00055 typedef void (*wolfSSL_Free_cb)(void *ptr, void* heap, int type); 00056 typedef void *(*wolfSSL_Realloc_cb)(void *ptr, size_t size, void* heap, int type); 00057 WOLFSSL_API void* wolfSSL_Malloc(size_t size, void* heap, int type); 00058 WOLFSSL_API void wolfSSL_Free(void *ptr, void* heap, int type); 00059 WOLFSSL_API void* wolfSSL_Realloc(void *ptr, size_t size, void* heap, int type); 00060 #endif /* WOLFSSL_DEBUG_MEMORY */ 00061 #else 00062 #ifdef WOLFSSL_DEBUG_MEMORY 00063 typedef void *(*wolfSSL_Malloc_cb)(size_t size, const char* func, unsigned int line); 00064 typedef void (*wolfSSL_Free_cb)(void *ptr, const char* func, unsigned int line); 00065 typedef void *(*wolfSSL_Realloc_cb)(void *ptr, size_t size, const char* func, unsigned int line); 00066 00067 /* Public in case user app wants to use XMALLOC/XFREE */ 00068 WOLFSSL_API void* wolfSSL_Malloc(size_t size, const char* func, unsigned int line); 00069 WOLFSSL_API void wolfSSL_Free(void *ptr, const char* func, unsigned int line); 00070 WOLFSSL_API void* wolfSSL_Realloc(void *ptr, size_t size, const char* func, unsigned int line); 00071 #else 00072 typedef void *(*wolfSSL_Malloc_cb)(size_t size); 00073 typedef void (*wolfSSL_Free_cb)(void *ptr); 00074 typedef void *(*wolfSSL_Realloc_cb)(void *ptr, size_t size); 00075 /* Public in case user app wants to use XMALLOC/XFREE */ 00076 WOLFSSL_API void* wolfSSL_Malloc(size_t size); 00077 WOLFSSL_API void wolfSSL_Free(void *ptr); 00078 WOLFSSL_API void* wolfSSL_Realloc(void *ptr, size_t size); 00079 #endif /* WOLFSSL_DEBUG_MEMORY */ 00080 #endif /* WOLFSSL_STATIC_MEMORY */ 00081 00082 /* Public get/set functions */ 00083 WOLFSSL_API int wolfSSL_SetAllocators(wolfSSL_Malloc_cb, 00084 wolfSSL_Free_cb, 00085 wolfSSL_Realloc_cb); 00086 WOLFSSL_API int wolfSSL_GetAllocators(wolfSSL_Malloc_cb*, 00087 wolfSSL_Free_cb*, 00088 wolfSSL_Realloc_cb*); 00089 00090 #ifdef WOLFSSL_STATIC_MEMORY 00091 #define WOLFSSL_STATIC_TIMEOUT 1 00092 #ifndef WOLFSSL_STATIC_ALIGN 00093 #define WOLFSSL_STATIC_ALIGN 16 00094 #endif 00095 #ifndef WOLFMEM_MAX_BUCKETS 00096 #define WOLFMEM_MAX_BUCKETS 9 00097 #endif 00098 #define WOLFMEM_DEF_BUCKETS 9 /* number of default memory blocks */ 00099 #ifndef WOLFMEM_IO_SZ 00100 #define WOLFMEM_IO_SZ 16992 /* 16 byte aligned */ 00101 #endif 00102 #ifndef WOLFMEM_BUCKETS 00103 #ifndef SESSION_CERTS 00104 /* default size of chunks of memory to separate into */ 00105 #ifndef LARGEST_MEM_BUCKET 00106 #define LARGEST_MEM_BUCKET 16128 00107 #endif 00108 #define WOLFMEM_BUCKETS 64,128,256,512,1024,2432,3456,4544,\ 00109 LARGEST_MEM_BUCKET 00110 #elif defined (OPENSSL_EXTRA) 00111 /* extra storage in structs for multiple attributes and order */ 00112 #ifndef LARGEST_MEM_BUCKET 00113 #define LARGEST_MEM_BUCKET 25600 00114 #endif 00115 #define WOLFMEM_BUCKETS 64,128,256,512,1024,2432,3360,4480,\ 00116 LARGEST_MEM_BUCKET 00117 #elif defined (WOLFSSL_CERT_EXT) 00118 /* certificate extensions requires 24k for the SSL struct */ 00119 #ifndef LARGEST_MEM_BUCKET 00120 #define LARGEST_MEM_BUCKET 24576 00121 #endif 00122 #define WOLFMEM_BUCKETS 64,128,256,512,1024,2432,3456,4544,\ 00123 LARGEST_MEM_BUCKET 00124 #else 00125 /* increase 23k for object member of WOLFSSL_X509_NAME_ENTRY */ 00126 #ifndef LARGEST_MEM_BUCKET 00127 #define LARGEST_MEM_BUCKET 23440 00128 #endif 00129 #define WOLFMEM_BUCKETS 64,128,256,512,1024,2432,3456,4544,\ 00130 LARGEST_MEM_BUCKET 00131 #endif 00132 #endif 00133 #ifndef WOLFMEM_DIST 00134 #ifndef WOLFSSL_STATIC_MEMORY_SMALL 00135 #define WOLFMEM_DIST 49,10,6,14,5,6,9,1,1 00136 #else 00137 /* Low resource and not RSA */ 00138 #define WOLFMEM_DIST 29, 7,6, 9,4,4,0,0,0 00139 #endif 00140 #endif 00141 00142 /* flags for loading static memory (one hot bit) */ 00143 #define WOLFMEM_GENERAL 0x01 00144 #define WOLFMEM_IO_POOL 0x02 00145 #define WOLFMEM_IO_POOL_FIXED 0x04 00146 #define WOLFMEM_TRACK_STATS 0x08 00147 00148 #ifndef WOLFSSL_MEM_GUARD 00149 #define WOLFSSL_MEM_GUARD 00150 typedef struct WOLFSSL_MEM_STATS WOLFSSL_MEM_STATS; 00151 typedef struct WOLFSSL_MEM_CONN_STATS WOLFSSL_MEM_CONN_STATS; 00152 #endif 00153 00154 struct WOLFSSL_MEM_CONN_STATS { 00155 word32 peakMem; /* peak memory usage */ 00156 word32 curMem; /* current memory usage */ 00157 word32 peakAlloc; /* peak memory allocations */ 00158 word32 curAlloc; /* current memory allocations */ 00159 word32 totalAlloc;/* total memory allocations for lifetime */ 00160 word32 totalFr; /* total frees for lifetime */ 00161 }; 00162 00163 struct WOLFSSL_MEM_STATS { 00164 word32 curAlloc; /* current memory allocations */ 00165 word32 totalAlloc;/* total memory allocations for lifetime */ 00166 word32 totalFr; /* total frees for lifetime */ 00167 word32 totalUse; /* total amount of memory used in blocks */ 00168 word32 avaIO; /* available IO specific pools */ 00169 word32 maxHa; /* max number of concurrent handshakes allowed */ 00170 word32 maxIO; /* max number of concurrent IO connections allowed */ 00171 word32 blockSz[WOLFMEM_MAX_BUCKETS]; /* block sizes in stacks */ 00172 word32 avaBlock[WOLFMEM_MAX_BUCKETS];/* ava block sizes */ 00173 word32 usedBlock[WOLFMEM_MAX_BUCKETS]; 00174 int flag; /* flag used */ 00175 }; 00176 00177 typedef struct wc_Memory wc_Memory; /* internal structure for mem bucket */ 00178 typedef struct WOLFSSL_HEAP { 00179 wc_Memory* ava[WOLFMEM_MAX_BUCKETS]; 00180 wc_Memory* io; /* list of buffers to use for IO */ 00181 word32 maxHa; /* max concurrent handshakes */ 00182 word32 curHa; 00183 word32 maxIO; /* max concurrent IO connections */ 00184 word32 curIO; 00185 word32 sizeList[WOLFMEM_MAX_BUCKETS];/* memory sizes in ava list */ 00186 word32 distList[WOLFMEM_MAX_BUCKETS];/* general distribution */ 00187 word32 inUse; /* amount of memory currently in use */ 00188 word32 ioUse; 00189 word32 alloc; /* total number of allocs */ 00190 word32 frAlc; /* total number of frees */ 00191 int flag; 00192 wolfSSL_Mutex memory_mutex; 00193 } WOLFSSL_HEAP; 00194 00195 /* structure passed into XMALLOC as heap hint 00196 * having this abstraction allows tracking statistics of individual ssl's 00197 */ 00198 typedef struct WOLFSSL_HEAP_HINT { 00199 WOLFSSL_HEAP* memory; 00200 WOLFSSL_MEM_CONN_STATS* stats; /* hold individual connection stats */ 00201 wc_Memory* outBuf; /* set if using fixed io buffers */ 00202 wc_Memory* inBuf; 00203 byte haFlag; /* flag used for checking handshake count */ 00204 } WOLFSSL_HEAP_HINT; 00205 00206 WOLFSSL_API int wc_LoadStaticMemory(WOLFSSL_HEAP_HINT** pHint, 00207 unsigned char* buf, unsigned int sz, int flag, int max); 00208 00209 WOLFSSL_LOCAL int wolfSSL_init_memory_heap(WOLFSSL_HEAP* heap); 00210 WOLFSSL_LOCAL int wolfSSL_load_static_memory(byte* buffer, word32 sz, 00211 int flag, WOLFSSL_HEAP* heap); 00212 WOLFSSL_LOCAL int wolfSSL_GetMemStats(WOLFSSL_HEAP* heap, 00213 WOLFSSL_MEM_STATS* stats); 00214 WOLFSSL_LOCAL int SetFixedIO(WOLFSSL_HEAP* heap, wc_Memory** io); 00215 WOLFSSL_LOCAL int FreeFixedIO(WOLFSSL_HEAP* heap, wc_Memory** io); 00216 00217 WOLFSSL_API int wolfSSL_StaticBufferSz(byte* buffer, word32 sz, int flag); 00218 WOLFSSL_API int wolfSSL_MemoryPaddingSz(void); 00219 #endif /* WOLFSSL_STATIC_MEMORY */ 00220 00221 #ifdef WOLFSSL_STACK_LOG 00222 WOLFSSL_API void __attribute__((no_instrument_function)) 00223 __cyg_profile_func_enter(void *func, void *caller); 00224 WOLFSSL_API void __attribute__((no_instrument_function)) 00225 __cyg_profile_func_exit(void *func, void *caller); 00226 #endif /* WOLFSSL_STACK_LOG */ 00227 00228 #ifdef __cplusplus 00229 } /* extern "C" */ 00230 #endif 00231 00232 #endif /* WOLFSSL_MEMORY_H */ 00233 00234
Generated on Tue Jul 12 2022 20:58:40 by
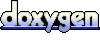