wolfSSL SSL/TLS library, support up to TLS1.3
Dependents: CyaSSL-Twitter-OAuth4Tw Example-client-tls-cert TwitterReader TweetTest ... more
fe_448.h
00001 /* fe448_448.h 00002 * 00003 * Copyright (C) 2006-2020 wolfSSL Inc. 00004 * 00005 * This file is part of wolfSSL. 00006 * 00007 * wolfSSL is free software; you can redistribute it and/or modify 00008 * it under the terms of the GNU General Public License as published by 00009 * the Free Software Foundation; either version 2 of the License, or 00010 * (at your option) any later version. 00011 * 00012 * wolfSSL is distributed in the hope that it will be useful, 00013 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00014 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00015 * GNU General Public License for more details. 00016 * 00017 * You should have received a copy of the GNU General Public License 00018 * along with this program; if not, write to the Free Software 00019 * Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1335, USA 00020 */ 00021 00022 00023 #ifndef WOLF_CRYPT_FE_448_H 00024 #define WOLF_CRYPT_FE_448_H 00025 00026 #include <wolfssl/wolfcrypt/settings.h> 00027 00028 #if defined(HAVE_CURVE448) || defined(HAVE_ED448) 00029 00030 #include <stdint.h> 00031 00032 #include <wolfssl/wolfcrypt/types.h > 00033 00034 #if defined(HAVE___UINT128_T) && !defined(NO_CURVED448_128BIT) 00035 #define CURVED448_128BIT 00036 #endif 00037 00038 #ifdef __cplusplus 00039 extern "C" { 00040 #endif 00041 00042 /* default to be faster but take more memory */ 00043 #if !defined(CURVE448_SMALL) || !defined(ED448_SMALL) 00044 00045 #if defined(CURVED448_128BIT) 00046 typedef int64_t fe448; 00047 #ifdef __SIZEOF_INT128__ 00048 typedef __uint128_t uint128_t; 00049 typedef __int128_t int128_t; 00050 #else 00051 typedef unsigned long uint128_t __attribute__ ((mode(TI))); 00052 typedef long int128_t __attribute__ ((mode(TI))); 00053 #endif 00054 #else 00055 typedef int32_t fe448; 00056 #endif 00057 00058 WOLFSSL_LOCAL void fe448_init(void); 00059 WOLFSSL_LOCAL int curve448(byte* r, const byte* n, const byte* a); 00060 00061 #if !defined(CURVED448_128BIT) 00062 WOLFSSL_LOCAL void fe448_reduce(fe448*); 00063 #else 00064 #define fe448_reduce(a) 00065 #endif 00066 WOLFSSL_LOCAL void fe448_neg(fe448*,const fe448*); 00067 WOLFSSL_LOCAL void fe448_add(fe448*, const fe448*, const fe448*); 00068 WOLFSSL_LOCAL void fe448_sub(fe448*, const fe448*, const fe448*); 00069 WOLFSSL_LOCAL void fe448_mul(fe448*,const fe448*,const fe448*); 00070 WOLFSSL_LOCAL void fe448_sqr(fe448*, const fe448*); 00071 WOLFSSL_LOCAL void fe448_mul39081(fe448*, const fe448*); 00072 WOLFSSL_LOCAL void fe448_invert(fe448*, const fe448*); 00073 00074 WOLFSSL_LOCAL void fe448_0(fe448*); 00075 WOLFSSL_LOCAL void fe448_1(fe448*); 00076 WOLFSSL_LOCAL void fe448_copy(fe448*, const fe448*); 00077 WOLFSSL_LOCAL int fe448_isnonzero(const fe448*); 00078 WOLFSSL_LOCAL int fe448_isnegative(const fe448*); 00079 00080 WOLFSSL_LOCAL void fe448_from_bytes(fe448*,const unsigned char *); 00081 WOLFSSL_LOCAL void fe448_to_bytes(unsigned char *, const fe448*); 00082 00083 WOLFSSL_LOCAL void fe448_cmov(fe448*,const fe448*, int); 00084 WOLFSSL_LOCAL void fe448_pow_2_446_222_1(fe448*,const fe448*); 00085 00086 #else 00087 00088 WOLFSSL_LOCAL void fe448_init(void); 00089 WOLFSSL_LOCAL int curve448(byte* r, const byte* n, const byte* a); 00090 00091 #define fe448_reduce(a) 00092 WOLFSSL_LOCAL void fe448_neg(uint8_t*,const uint8_t*); 00093 WOLFSSL_LOCAL void fe448_add(uint8_t*, const uint8_t*, const uint8_t*); 00094 WOLFSSL_LOCAL void fe448_sub(uint8_t*, const uint8_t*, const uint8_t*); 00095 WOLFSSL_LOCAL void fe448_mul(uint8_t*,const uint8_t*,const uint8_t*); 00096 WOLFSSL_LOCAL void fe448_sqr(uint8_t*, const uint8_t*); 00097 WOLFSSL_LOCAL void fe448_mul39081(uint8_t*, const uint8_t*); 00098 WOLFSSL_LOCAL void fe448_invert(uint8_t*, const uint8_t*); 00099 00100 WOLFSSL_LOCAL void fe448_copy(uint8_t*, const uint8_t*); 00101 WOLFSSL_LOCAL int fe448_isnonzero(const uint8_t*); 00102 00103 WOLFSSL_LOCAL void fe448_norm(byte *a); 00104 00105 WOLFSSL_LOCAL void fe448_cmov(uint8_t*,const uint8_t*, int); 00106 WOLFSSL_LOCAL void fe448_pow_2_446_222_1(uint8_t*,const uint8_t*); 00107 00108 #endif /* !CURVE448_SMALL || !ED448_SMALL */ 00109 00110 #ifdef __cplusplus 00111 } /* extern "C" */ 00112 #endif 00113 00114 #endif /* HAVE_CURVE448 || HAVE_ED448 */ 00115 00116 #endif /* WOLF_CRYPT_FE_448_H */ 00117
Generated on Tue Jul 12 2022 20:58:35 by
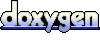