wolfSSL SSL/TLS library, support up to TLS1.3
Dependents: CyaSSL-Twitter-OAuth4Tw Example-client-tls-cert TwitterReader TweetTest ... more
des3.h
00001 /* des3.h 00002 * 00003 * Copyright (C) 2006-2020 wolfSSL Inc. 00004 * 00005 * This file is part of wolfSSL. 00006 * 00007 * wolfSSL is free software; you can redistribute it and/or modify 00008 * it under the terms of the GNU General Public License as published by 00009 * the Free Software Foundation; either version 2 of the License, or 00010 * (at your option) any later version. 00011 * 00012 * wolfSSL is distributed in the hope that it will be useful, 00013 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00014 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00015 * GNU General Public License for more details. 00016 * 00017 * You should have received a copy of the GNU General Public License 00018 * along with this program; if not, write to the Free Software 00019 * Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1335, USA 00020 */ 00021 00022 /*! 00023 \file wolfssl/wolfcrypt/des3.h 00024 */ 00025 00026 #ifndef WOLF_CRYPT_DES3_H 00027 #define WOLF_CRYPT_DES3_H 00028 00029 #include <wolfssl/wolfcrypt/types.h > 00030 00031 #ifndef NO_DES3 00032 00033 #if defined(HAVE_FIPS) && \ 00034 defined(HAVE_FIPS_VERSION) && (HAVE_FIPS_VERSION >= 2) 00035 #include <wolfssl/wolfcrypt/fips.h> 00036 #endif /* HAVE_FIPS_VERSION >= 2 */ 00037 00038 #if defined(HAVE_FIPS) && \ 00039 (!defined(HAVE_FIPS_VERSION) || (HAVE_FIPS_VERSION < 2)) 00040 /* included for fips @wc_fips */ 00041 #include <cyassl/ctaocrypt/des3.h> 00042 #endif 00043 00044 #ifdef __cplusplus 00045 extern "C" { 00046 #endif 00047 00048 /* these are required for FIPS and non-FIPS */ 00049 enum { 00050 DES_KEY_SIZE = 8, /* des */ 00051 DES3_KEY_SIZE = 24, /* 3 des ede */ 00052 DES_IV_SIZE = 8, /* should be the same as DES_BLOCK_SIZE */ 00053 }; 00054 00055 00056 /* avoid redefinition of structs */ 00057 #if !defined(HAVE_FIPS) || \ 00058 (defined(HAVE_FIPS_VERSION) && (HAVE_FIPS_VERSION >= 2)) 00059 00060 #ifdef WOLFSSL_ASYNC_CRYPT 00061 #include <wolfssl/wolfcrypt/async.h> 00062 #endif 00063 00064 enum { 00065 DES_ENC_TYPE = WC_CIPHER_DES, /* cipher unique type */ 00066 DES3_ENC_TYPE = WC_CIPHER_DES3, /* cipher unique type */ 00067 00068 DES_BLOCK_SIZE = 8, 00069 DES_KS_SIZE = 32, /* internal DES key buffer size */ 00070 00071 DES_ENCRYPTION = 0, 00072 DES_DECRYPTION = 1 00073 }; 00074 00075 #define DES_IVLEN 8 00076 #define DES_KEYLEN 8 00077 #define DES3_IVLEN 8 00078 #define DES3_KEYLEN 24 00079 00080 00081 #if defined(STM32_CRYPTO) 00082 enum { 00083 DES_CBC = 0, 00084 DES_ECB = 1 00085 }; 00086 #endif 00087 00088 00089 /* DES encryption and decryption */ 00090 typedef struct Des { 00091 word32 reg[DES_BLOCK_SIZE / sizeof(word32)]; /* for CBC mode */ 00092 word32 tmp[DES_BLOCK_SIZE / sizeof(word32)]; /* same */ 00093 word32 key[DES_KS_SIZE]; 00094 } Des; 00095 00096 00097 /* DES3 encryption and decryption */ 00098 struct Des3 { 00099 word32 key[3][DES_KS_SIZE]; 00100 word32 reg[DES_BLOCK_SIZE / sizeof(word32)]; /* for CBC mode */ 00101 word32 tmp[DES_BLOCK_SIZE / sizeof(word32)]; /* same */ 00102 #ifdef WOLFSSL_ASYNC_CRYPT 00103 WC_ASYNC_DEV asyncDev; 00104 #endif 00105 #if defined(WOLF_CRYPTO_CB) || \ 00106 (defined(WOLFSSL_ASYNC_CRYPT) && defined(WC_ASYNC_ENABLE_3DES)) 00107 word32 devKey[DES3_KEYLEN/sizeof(word32)]; /* raw key */ 00108 #endif 00109 #ifdef WOLF_CRYPTO_CB 00110 int devId; 00111 void* devCtx; 00112 #endif 00113 void* heap; 00114 }; 00115 00116 #ifndef WC_DES3_TYPE_DEFINED 00117 typedef struct Des3 Des3; 00118 #define WC_DES3_TYPE_DEFINED 00119 #endif 00120 #endif /* HAVE_FIPS */ 00121 00122 00123 WOLFSSL_API int wc_Des_SetKey(Des* des, const byte* key, 00124 const byte* iv, int dir); 00125 WOLFSSL_API void wc_Des_SetIV(Des* des, const byte* iv); 00126 WOLFSSL_API int wc_Des_CbcEncrypt(Des* des, byte* out, 00127 const byte* in, word32 sz); 00128 WOLFSSL_API int wc_Des_CbcDecrypt(Des* des, byte* out, 00129 const byte* in, word32 sz); 00130 WOLFSSL_API int wc_Des_EcbEncrypt(Des* des, byte* out, 00131 const byte* in, word32 sz); 00132 WOLFSSL_API int wc_Des3_EcbEncrypt(Des3* des, byte* out, 00133 const byte* in, word32 sz); 00134 00135 /* ECB decrypt same process as encrypt but with decrypt key */ 00136 #define wc_Des_EcbDecrypt wc_Des_EcbEncrypt 00137 #define wc_Des3_EcbDecrypt wc_Des3_EcbEncrypt 00138 00139 WOLFSSL_API int wc_Des3_SetKey(Des3* des, const byte* key, 00140 const byte* iv,int dir); 00141 WOLFSSL_API int wc_Des3_SetIV(Des3* des, const byte* iv); 00142 WOLFSSL_API int wc_Des3_CbcEncrypt(Des3* des, byte* out, 00143 const byte* in,word32 sz); 00144 WOLFSSL_API int wc_Des3_CbcDecrypt(Des3* des, byte* out, 00145 const byte* in,word32 sz); 00146 00147 /* These are only required when using either: 00148 static memory (WOLFSSL_STATIC_MEMORY) or asynchronous (WOLFSSL_ASYNC_CRYPT) */ 00149 WOLFSSL_API int wc_Des3Init(Des3*, void*, int); 00150 WOLFSSL_API void wc_Des3Free(Des3*); 00151 00152 #ifdef __cplusplus 00153 } /* extern "C" */ 00154 #endif 00155 00156 #endif /* NO_DES3 */ 00157 #endif /* WOLF_CRYPT_DES3_H */ 00158 00159
Generated on Tue Jul 12 2022 20:58:34 by
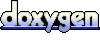