wolfSSL SSL/TLS library, support up to TLS1.3
Dependents: CyaSSL-Twitter-OAuth4Tw Example-client-tls-cert TwitterReader TweetTest ... more
blake2.h
00001 /* blake2.h 00002 * 00003 * Copyright (C) 2006-2020 wolfSSL Inc. 00004 * 00005 * This file is part of wolfSSL. 00006 * 00007 * wolfSSL is free software; you can redistribute it and/or modify 00008 * it under the terms of the GNU General Public License as published by 00009 * the Free Software Foundation; either version 2 of the License, or 00010 * (at your option) any later version. 00011 * 00012 * wolfSSL is distributed in the hope that it will be useful, 00013 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00014 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00015 * GNU General Public License for more details. 00016 * 00017 * You should have received a copy of the GNU General Public License 00018 * along with this program; if not, write to the Free Software 00019 * Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1335, USA 00020 */ 00021 00022 /*! 00023 \file wolfssl/wolfcrypt/blake2.h 00024 */ 00025 00026 #ifndef WOLF_CRYPT_BLAKE2_H 00027 #define WOLF_CRYPT_BLAKE2_H 00028 00029 #include <wolfssl/wolfcrypt/settings.h> 00030 00031 #if defined(HAVE_BLAKE2) || defined(HAVE_BLAKE2S) 00032 00033 #include <wolfssl/wolfcrypt/blake2-int.h> 00034 00035 /* call old functions if using fips for the sake of hmac @wc_fips */ 00036 #ifdef HAVE_FIPS 00037 /* Since hmac can call blake functions provide original calls */ 00038 #define wc_InitBlake2b InitBlake2b 00039 #define wc_Blake2bUpdate Blake2bUpdate 00040 #define wc_Blake2bFinal Blake2bFinal 00041 #endif 00042 00043 #ifdef __cplusplus 00044 extern "C" { 00045 #endif 00046 00047 /* in bytes, variable digest size up to 512 bits (64 bytes) */ 00048 enum { 00049 #ifdef HAVE_BLAKE2B 00050 BLAKE2B_ID = WC_HASH_TYPE_BLAKE2B, 00051 BLAKE2B_256 = 32, /* 256 bit type, SSL default */ 00052 #endif 00053 #ifdef HAVE_BLAKE2S 00054 BLAKE2S_ID = WC_HASH_TYPE_BLAKE2S, 00055 BLAKE2S_256 = 32 /* 256 bit type */ 00056 #endif 00057 }; 00058 00059 00060 #ifdef HAVE_BLAKE2B 00061 /* BLAKE2b digest */ 00062 typedef struct Blake2b { 00063 blake2b_state S[1]; /* our state */ 00064 word32 digestSz; /* digest size used on init */ 00065 } Blake2b; 00066 #endif 00067 00068 #ifdef HAVE_BLAKE2S 00069 /* BLAKE2s digest */ 00070 typedef struct Blake2s { 00071 blake2s_state S[1]; /* our state */ 00072 word32 digestSz; /* digest size used on init */ 00073 } Blake2s; 00074 #endif 00075 00076 00077 #ifdef HAVE_BLAKE2B 00078 WOLFSSL_API int wc_InitBlake2b(Blake2b*, word32); 00079 WOLFSSL_API int wc_Blake2bUpdate(Blake2b*, const byte*, word32); 00080 WOLFSSL_API int wc_Blake2bFinal(Blake2b*, byte*, word32); 00081 #endif 00082 00083 #ifdef HAVE_BLAKE2S 00084 WOLFSSL_API int wc_InitBlake2s(Blake2s*, word32); 00085 WOLFSSL_API int wc_Blake2sUpdate(Blake2s*, const byte*, word32); 00086 WOLFSSL_API int wc_Blake2sFinal(Blake2s*, byte*, word32); 00087 #endif 00088 00089 00090 #ifdef __cplusplus 00091 } 00092 #endif 00093 00094 #endif /* HAVE_BLAKE2 || HAVE_BLAKE2S */ 00095 #endif /* WOLF_CRYPT_BLAKE2_H */ 00096 00097
Generated on Tue Jul 12 2022 20:58:34 by
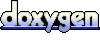