wolfSSL SSL/TLS library, support up to TLS1.3
Dependents: CyaSSL-Twitter-OAuth4Tw Example-client-tls-cert TwitterReader TweetTest ... more
blake2-impl.h
00001 /* 00002 BLAKE2 reference source code package - reference C implementations 00003 00004 Written in 2012 by Samuel Neves <sneves@dei.uc.pt> 00005 00006 To the extent possible under law, the author(s) have dedicated all copyright 00007 and related and neighboring rights to this software to the public domain 00008 worldwide. This software is distributed without any warranty. 00009 00010 You should have received a copy of the CC0 Public Domain Dedication along with 00011 this software. If not, see <http://creativecommons.org/publicdomain/zero/1.0/>. 00012 */ 00013 /* blake2-impl.h 00014 * 00015 * Copyright (C) 2006-2020 wolfSSL Inc. 00016 * 00017 * This file is part of wolfSSL. 00018 * 00019 * wolfSSL is free software; you can redistribute it and/or modify 00020 * it under the terms of the GNU General Public License as published by 00021 * the Free Software Foundation; either version 2 of the License, or 00022 * (at your option) any later version. 00023 * 00024 * wolfSSL is distributed in the hope that it will be useful, 00025 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00026 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00027 * GNU General Public License for more details. 00028 * 00029 * You should have received a copy of the GNU General Public License 00030 * along with this program; if not, write to the Free Software 00031 * Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1335, USA 00032 */ 00033 00034 00035 00036 #ifndef WOLFCRYPT_BLAKE2_IMPL_H 00037 #define WOLFCRYPT_BLAKE2_IMPL_H 00038 00039 #include <wolfssl/wolfcrypt/types.h > 00040 00041 static WC_INLINE word32 load32( const void *src ) 00042 { 00043 #if defined(LITTLE_ENDIAN_ORDER) 00044 return *( word32 * )( src ); 00045 #else 00046 const byte *p = ( byte * )src; 00047 word32 w = *p++; 00048 w |= ( word32 )( *p++ ) << 8; 00049 w |= ( word32 )( *p++ ) << 16; 00050 w |= ( word32 )( *p++ ) << 24; 00051 return w; 00052 #endif 00053 } 00054 00055 static WC_INLINE word64 load64( const void *src ) 00056 { 00057 #if defined(LITTLE_ENDIAN_ORDER) 00058 return *( word64 * )( src ); 00059 #else 00060 const byte *p = ( byte * )src; 00061 word64 w = *p++; 00062 w |= ( word64 )( *p++ ) << 8; 00063 w |= ( word64 )( *p++ ) << 16; 00064 w |= ( word64 )( *p++ ) << 24; 00065 w |= ( word64 )( *p++ ) << 32; 00066 w |= ( word64 )( *p++ ) << 40; 00067 w |= ( word64 )( *p++ ) << 48; 00068 w |= ( word64 )( *p++ ) << 56; 00069 return w; 00070 #endif 00071 } 00072 00073 static WC_INLINE void store32( void *dst, word32 w ) 00074 { 00075 #if defined(LITTLE_ENDIAN_ORDER) 00076 *( word32 * )( dst ) = w; 00077 #else 00078 byte *p = ( byte * )dst; 00079 *p++ = ( byte )w; w >>= 8; 00080 *p++ = ( byte )w; w >>= 8; 00081 *p++ = ( byte )w; w >>= 8; 00082 *p++ = ( byte )w; 00083 #endif 00084 } 00085 00086 static WC_INLINE void store64( void *dst, word64 w ) 00087 { 00088 #if defined(LITTLE_ENDIAN_ORDER) 00089 *( word64 * )( dst ) = w; 00090 #else 00091 byte *p = ( byte * )dst; 00092 *p++ = ( byte )w; w >>= 8; 00093 *p++ = ( byte )w; w >>= 8; 00094 *p++ = ( byte )w; w >>= 8; 00095 *p++ = ( byte )w; w >>= 8; 00096 *p++ = ( byte )w; w >>= 8; 00097 *p++ = ( byte )w; w >>= 8; 00098 *p++ = ( byte )w; w >>= 8; 00099 *p++ = ( byte )w; 00100 #endif 00101 } 00102 00103 static WC_INLINE word64 load48( const void *src ) 00104 { 00105 const byte *p = ( const byte * )src; 00106 word64 w = *p++; 00107 w |= ( word64 )( *p++ ) << 8; 00108 w |= ( word64 )( *p++ ) << 16; 00109 w |= ( word64 )( *p++ ) << 24; 00110 w |= ( word64 )( *p++ ) << 32; 00111 w |= ( word64 )( *p++ ) << 40; 00112 return w; 00113 } 00114 00115 static WC_INLINE void store48( void *dst, word64 w ) 00116 { 00117 byte *p = ( byte * )dst; 00118 *p++ = ( byte )w; w >>= 8; 00119 *p++ = ( byte )w; w >>= 8; 00120 *p++ = ( byte )w; w >>= 8; 00121 *p++ = ( byte )w; w >>= 8; 00122 *p++ = ( byte )w; w >>= 8; 00123 *p++ = ( byte )w; 00124 } 00125 00126 static WC_INLINE word32 rotl32( const word32 w, const unsigned c ) 00127 { 00128 return ( w << c ) | ( w >> ( 32 - c ) ); 00129 } 00130 00131 static WC_INLINE word64 rotl64( const word64 w, const unsigned c ) 00132 { 00133 return ( w << c ) | ( w >> ( 64 - c ) ); 00134 } 00135 00136 static WC_INLINE word32 rotr32( const word32 w, const unsigned c ) 00137 { 00138 return ( w >> c ) | ( w << ( 32 - c ) ); 00139 } 00140 00141 static WC_INLINE word64 rotr64( const word64 w, const unsigned c ) 00142 { 00143 return ( w >> c ) | ( w << ( 64 - c ) ); 00144 } 00145 00146 /* prevents compiler optimizing out memset() */ 00147 static WC_INLINE void secure_zero_memory( void *v, word64 n ) 00148 { 00149 volatile byte *p = ( volatile byte * )v; 00150 00151 while( n-- ) *p++ = 0; 00152 } 00153 00154 #endif /* WOLFCRYPT_BLAKE2_IMPL_H */ 00155 00156
Generated on Tue Jul 12 2022 20:58:34 by
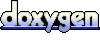