wolfSSL SSL/TLS library, support up to TLS1.3
Dependents: CyaSSL-Twitter-OAuth4Tw Example-client-tls-cert TwitterReader TweetTest ... more
bio.h
00001 /* bio.h 00002 * 00003 * Copyright (C) 2006-2020 wolfSSL Inc. 00004 * 00005 * This file is part of wolfSSL. 00006 * 00007 * wolfSSL is free software; you can redistribute it and/or modify 00008 * it under the terms of the GNU General Public License as published by 00009 * the Free Software Foundation; either version 2 of the License, or 00010 * (at your option) any later version. 00011 * 00012 * wolfSSL is distributed in the hope that it will be useful, 00013 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00014 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00015 * GNU General Public License for more details. 00016 * 00017 * You should have received a copy of the GNU General Public License 00018 * along with this program; if not, write to the Free Software 00019 * Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1335, USA 00020 */ 00021 00022 /* bio.h for openssl */ 00023 00024 00025 #ifndef WOLFSSL_BIO_H_ 00026 #define WOLFSSL_BIO_H_ 00027 00028 #include <wolfssl/openssl/ssl.h> 00029 00030 00031 #ifdef __cplusplus 00032 extern "C" { 00033 #endif 00034 00035 00036 #define BIO_FLAG_BASE64_NO_NL WOLFSSL_BIO_FLAG_BASE64_NO_NL 00037 #define BIO_FLAG_READ WOLFSSL_BIO_FLAG_READ 00038 #define BIO_FLAG_WRITE WOLFSSL_BIO_FLAG_WRITE 00039 #define BIO_FLAG_IO_SPECIAL WOLFSSL_BIO_FLAG_IO_SPECIAL 00040 #define BIO_FLAG_RETRY WOLFSSL_BIO_FLAG_RETRY 00041 00042 #define BIO_new_fp wolfSSL_BIO_new_fp 00043 #define BIO_new_file wolfSSL_BIO_new_file 00044 #define BIO_new_fp wolfSSL_BIO_new_fp 00045 #define BIO_ctrl wolfSSL_BIO_ctrl 00046 #define BIO_ctrl_pending wolfSSL_BIO_ctrl_pending 00047 #define BIO_wpending wolfSSL_BIO_wpending 00048 #define BIO_get_mem_ptr wolfSSL_BIO_get_mem_ptr 00049 #define BIO_int_ctrl wolfSSL_BIO_int_ctrl 00050 #define BIO_reset wolfSSL_BIO_reset 00051 #define BIO_s_file wolfSSL_BIO_s_file 00052 #define BIO_s_bio wolfSSL_BIO_s_bio 00053 #define BIO_s_socket wolfSSL_BIO_s_socket 00054 #define BIO_set_fd wolfSSL_BIO_set_fd 00055 #define BIO_set_close wolfSSL_BIO_set_close 00056 #define BIO_ctrl_reset_read_request wolfSSL_BIO_ctrl_reset_read_request 00057 #define BIO_set_write_buf_size wolfSSL_BIO_set_write_buf_size 00058 #define BIO_make_bio_pair wolfSSL_BIO_make_bio_pair 00059 00060 #define BIO_set_fp wolfSSL_BIO_set_fp 00061 #define BIO_get_fp wolfSSL_BIO_get_fp 00062 #define BIO_seek wolfSSL_BIO_seek 00063 #define BIO_write_filename wolfSSL_BIO_write_filename 00064 #define BIO_set_mem_eof_return wolfSSL_BIO_set_mem_eof_return 00065 00066 #define BIO_find_type wolfSSL_BIO_find_type 00067 #define BIO_next wolfSSL_BIO_next 00068 #define BIO_gets wolfSSL_BIO_gets 00069 #define BIO_puts wolfSSL_BIO_puts 00070 00071 #define BIO_should_retry wolfSSL_BIO_should_retry 00072 00073 #define BIO_TYPE_FILE WOLFSSL_BIO_FILE 00074 #define BIO_TYPE_BIO WOLFSSL_BIO_BIO 00075 #define BIO_TYPE_MEM WOLFSSL_BIO_MEMORY 00076 #define BIO_TYPE_BASE64 WOLFSSL_BIO_BASE64 00077 00078 #define BIO_vprintf wolfSSL_BIO_vprintf 00079 #define BIO_printf wolfSSL_BIO_printf 00080 #define BIO_dump wolfSSL_BIO_dump 00081 00082 /* BIO info callback */ 00083 #define BIO_CB_FREE WOLFSSL_BIO_CB_FREE 00084 #define BIO_CB_READ WOLFSSL_BIO_CB_READ 00085 #define BIO_CB_WRITE WOLFSSL_BIO_CB_WRITE 00086 #define BIO_CB_PUTS WOLFSSL_BIO_CB_PUTS 00087 #define BIO_CB_GETS WOLFSSL_BIO_CB_GETS 00088 #define BIO_CB_CTRL WOLFSSL_BIO_CB_CTRL 00089 #define BIO_CB_RETURN WOLFSSL_BIO_CB_RETURN 00090 00091 #define BIO_set_callback wolfSSL_BIO_set_callback 00092 #define BIO_get_callback wolfSSL_BIO_get_callback 00093 #define BIO_set_callback_arg wolfSSL_BIO_set_callback_arg 00094 #define BIO_get_callback_arg wolfSSL_BIO_get_callback_arg 00095 00096 /* BIO for 1.1.0 or later */ 00097 #define BIO_set_init wolfSSL_BIO_set_init 00098 #define BIO_get_data wolfSSL_BIO_get_data 00099 #define BIO_set_data wolfSSL_BIO_set_data 00100 #define BIO_get_shutdown wolfSSL_BIO_get_shutdown 00101 #define BIO_set_shutdown wolfSSL_BIO_set_shutdown 00102 00103 #define BIO_clear_flags wolfSSL_BIO_clear_flags 00104 #define BIO_set_ex_data wolfSSL_BIO_set_ex_data 00105 #define BIO_get_ex_data wolfSSL_BIO_get_ex_data 00106 00107 /* helper to set specific retry/read flags */ 00108 #define BIO_set_retry_read(bio)\ 00109 wolfSSL_BIO_set_flags((bio), WOLFSSL_BIO_FLAG_RETRY | WOLFSSL_BIO_FLAG_READ) 00110 #define BIO_set_retry_write(bio)\ 00111 wolfSSL_BIO_set_flags((bio), WOLFSSL_BIO_FLAG_RETRY | WOLFSSL_BIO_FLAG_WRITE) 00112 00113 #define BIO_clear_retry_flags wolfSSL_BIO_clear_retry_flags 00114 00115 #define BIO_meth_new wolfSSL_BIO_meth_new 00116 #define BIO_meth_set_write wolfSSL_BIO_meth_set_write 00117 #define BIO_meth_free wolfSSL_BIO_meth_free 00118 #define BIO_meth_set_write wolfSSL_BIO_meth_set_write 00119 #define BIO_meth_set_read wolfSSL_BIO_meth_set_read 00120 #define BIO_meth_set_puts wolfSSL_BIO_meth_set_puts 00121 #define BIO_meth_set_gets wolfSSL_BIO_meth_set_gets 00122 #define BIO_meth_set_ctrl wolfSSL_BIO_meth_set_ctrl 00123 #define BIO_meth_set_create wolfSSL_BIO_meth_set_create 00124 #define BIO_meth_set_destroy wolfSSL_BIO_meth_set_destroy 00125 00126 00127 /* BIO CTRL */ 00128 #define BIO_CTRL_RESET 1 00129 #define BIO_CTRL_EOF 2 00130 #define BIO_CTRL_INFO 3 00131 #define BIO_CTRL_PUSH 6 00132 #define BIO_CTRL_POP 7 00133 #define BIO_CTRL_GET_CLOSE 8 00134 #define BIO_CTRL_SET_CLOSE 9 00135 #define BIO_CTRL_PENDING 10 00136 #define BIO_CTRL_FLUSH 11 00137 #define BIO_CTRL_DUP 12 00138 #define BIO_CTRL_WPENDING 13 00139 00140 #define BIO_C_SET_FILE_PTR 106 00141 #define BIO_C_GET_FILE_PTR 107 00142 #define BIO_C_SET_FILENAME 108 00143 #define BIO_C_SET_BUF_MEM 114 00144 #define BIO_C_GET_BUF_MEM_PTR 115 00145 #define BIO_C_FILE_SEEK 128 00146 #define BIO_C_SET_BUF_MEM_EOF_RETURN 130 00147 #define BIO_C_SET_WRITE_BUF_SIZE 136 00148 #define BIO_C_MAKE_BIO_PAIR 138 00149 00150 #define BIO_CTRL_DGRAM_QUERY_MTU 40 00151 00152 #define BIO_NOCLOSE 0x00 00153 #define BIO_CLOSE 0x01 00154 00155 #define BIO_FP_WRITE 0x04 00156 00157 00158 #ifdef __cplusplus 00159 } /* extern "C" */ 00160 #endif 00161 00162 00163 #endif /* WOLFSSL_BIO_H_ */ 00164 00165
Generated on Tue Jul 12 2022 20:58:34 by
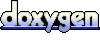