wolfSSL SSL/TLS library, support up to TLS1.3
Dependents: CyaSSL-Twitter-OAuth4Tw Example-client-tls-cert TwitterReader TweetTest ... more
asn_public.h
00001 /* asn_public.h 00002 * 00003 * Copyright (C) 2006-2020 wolfSSL Inc. 00004 * 00005 * This file is part of wolfSSL. 00006 * 00007 * wolfSSL is free software; you can redistribute it and/or modify 00008 * it under the terms of the GNU General Public License as published by 00009 * the Free Software Foundation; either version 2 of the License, or 00010 * (at your option) any later version. 00011 * 00012 * wolfSSL is distributed in the hope that it will be useful, 00013 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00014 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00015 * GNU General Public License for more details. 00016 * 00017 * You should have received a copy of the GNU General Public License 00018 * along with this program; if not, write to the Free Software 00019 * Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1335, USA 00020 */ 00021 00022 /*! 00023 \file wolfssl/wolfcrypt/asn_public.h 00024 */ 00025 00026 #ifndef WOLF_CRYPT_ASN_PUBLIC_H 00027 #define WOLF_CRYPT_ASN_PUBLIC_H 00028 00029 #include <wolfssl/wolfcrypt/types.h > 00030 00031 #ifdef __cplusplus 00032 extern "C" { 00033 #endif 00034 00035 /* guard on redeclaration */ 00036 #ifndef WC_ECCKEY_TYPE_DEFINED 00037 typedef struct ecc_key ecc_key; 00038 #define WC_ECCKEY_TYPE_DEFINED 00039 #endif 00040 #ifndef WC_ED25519KEY_TYPE_DEFINED 00041 typedef struct ed25519_key ed25519_key; 00042 #define WC_ED25519KEY_TYPE_DEFINED 00043 #endif 00044 #ifndef WC_ED448KEY_TYPE_DEFINED 00045 typedef struct ed448_key ed448_key; 00046 #define WC_ED448KEY_TYPE_DEFINED 00047 #endif 00048 #ifndef WC_RSAKEY_TYPE_DEFINED 00049 typedef struct RsaKey RsaKey; 00050 #define WC_RSAKEY_TYPE_DEFINED 00051 #endif 00052 #ifndef WC_RNG_TYPE_DEFINED 00053 typedef struct WC_RNG WC_RNG; 00054 #define WC_RNG_TYPE_DEFINED 00055 #endif 00056 00057 enum Ecc_Sum { 00058 ECC_SECP112R1_OID = 182, 00059 ECC_SECP112R2_OID = 183, 00060 ECC_SECP128R1_OID = 204, 00061 ECC_SECP128R2_OID = 205, 00062 ECC_SECP160R1_OID = 184, 00063 ECC_SECP160R2_OID = 206, 00064 ECC_SECP160K1_OID = 185, 00065 ECC_BRAINPOOLP160R1_OID = 98, 00066 ECC_SECP192R1_OID = 520, 00067 ECC_PRIME192V2_OID = 521, 00068 ECC_PRIME192V3_OID = 522, 00069 ECC_SECP192K1_OID = 207, 00070 ECC_BRAINPOOLP192R1_OID = 100, 00071 ECC_SECP224R1_OID = 209, 00072 ECC_SECP224K1_OID = 208, 00073 ECC_BRAINPOOLP224R1_OID = 102, 00074 ECC_PRIME239V1_OID = 523, 00075 ECC_PRIME239V2_OID = 524, 00076 ECC_PRIME239V3_OID = 525, 00077 ECC_SECP256R1_OID = 526, 00078 ECC_SECP256K1_OID = 186, 00079 ECC_BRAINPOOLP256R1_OID = 104, 00080 ECC_X25519_OID = 365, 00081 ECC_ED25519_OID = 256, 00082 ECC_BRAINPOOLP320R1_OID = 106, 00083 ECC_X448_OID = 362, 00084 ECC_ED448_OID = 257, 00085 ECC_SECP384R1_OID = 210, 00086 ECC_BRAINPOOLP384R1_OID = 108, 00087 ECC_BRAINPOOLP512R1_OID = 110, 00088 ECC_SECP521R1_OID = 211, 00089 }; 00090 00091 00092 /* Certificate file Type */ 00093 enum CertType { 00094 CERT_TYPE = 0, 00095 PRIVATEKEY_TYPE, 00096 DH_PARAM_TYPE, 00097 DSA_PARAM_TYPE, 00098 CRL_TYPE, 00099 CA_TYPE, 00100 ECC_PRIVATEKEY_TYPE, 00101 DSA_PRIVATEKEY_TYPE, 00102 CERTREQ_TYPE, 00103 DSA_TYPE, 00104 ECC_TYPE, 00105 RSA_TYPE, 00106 PUBLICKEY_TYPE, 00107 RSA_PUBLICKEY_TYPE, 00108 ECC_PUBLICKEY_TYPE, 00109 TRUSTED_PEER_TYPE, 00110 EDDSA_PRIVATEKEY_TYPE, 00111 ED25519_TYPE, 00112 ED448_TYPE, 00113 PKCS12_TYPE, 00114 PKCS8_PRIVATEKEY_TYPE, 00115 PKCS8_ENC_PRIVATEKEY_TYPE, 00116 DETECT_CERT_TYPE, 00117 DH_PRIVATEKEY_TYPE, 00118 }; 00119 00120 00121 /* Signature type, by OID sum */ 00122 enum Ctc_SigType { 00123 CTC_SHAwDSA = 517, 00124 CTC_MD2wRSA = 646, 00125 CTC_MD5wRSA = 648, 00126 CTC_SHAwRSA = 649, 00127 CTC_SHAwECDSA = 520, 00128 CTC_SHA224wRSA = 658, 00129 CTC_SHA224wECDSA = 523, 00130 CTC_SHA256wRSA = 655, 00131 CTC_SHA256wECDSA = 524, 00132 CTC_SHA384wRSA = 656, 00133 CTC_SHA384wECDSA = 525, 00134 CTC_SHA512wRSA = 657, 00135 CTC_SHA512wECDSA = 526, 00136 CTC_ED25519 = 256, 00137 CTC_ED448 = 257 00138 }; 00139 00140 enum Ctc_Encoding { 00141 CTC_UTF8 = 0x0c, /* utf8 */ 00142 CTC_PRINTABLE = 0x13 /* printable */ 00143 }; 00144 00145 #ifndef WC_CTC_NAME_SIZE 00146 #define WC_CTC_NAME_SIZE 64 00147 #endif 00148 #ifndef WC_CTC_MAX_ALT_SIZE 00149 #define WC_CTC_MAX_ALT_SIZE 16384 00150 #endif 00151 00152 enum Ctc_Misc { 00153 CTC_COUNTRY_SIZE = 2, 00154 CTC_NAME_SIZE = WC_CTC_NAME_SIZE, 00155 CTC_DATE_SIZE = 32, 00156 CTC_MAX_ALT_SIZE = WC_CTC_MAX_ALT_SIZE, /* may be huge, default: 16384 */ 00157 CTC_SERIAL_SIZE = 20, 00158 CTC_GEN_SERIAL_SZ = 16, 00159 #ifdef WOLFSSL_CERT_EXT 00160 /* AKID could contains: hash + (Option) AuthCertIssuer,AuthCertSerialNum 00161 * We support only hash */ 00162 CTC_MAX_SKID_SIZE = 32, /* SHA256_DIGEST_SIZE */ 00163 CTC_MAX_AKID_SIZE = 32, /* SHA256_DIGEST_SIZE */ 00164 CTC_MAX_CERTPOL_SZ = 64, 00165 CTC_MAX_CERTPOL_NB = 2 /* Max number of Certificate Policy */ 00166 #endif /* WOLFSSL_CERT_EXT */ 00167 }; 00168 00169 /* DER buffer */ 00170 typedef struct DerBuffer { 00171 byte* buffer; 00172 void* heap; 00173 word32 length; 00174 int type; /* enum CertType */ 00175 int dynType; /* DYNAMIC_TYPE_* */ 00176 } DerBuffer; 00177 00178 typedef struct WOLFSSL_ASN1_TIME { 00179 unsigned char data[CTC_DATE_SIZE]; /* date bytes */ 00180 int length; 00181 int type; 00182 } WOLFSSL_ASN1_TIME; 00183 00184 enum { 00185 IV_SZ = 32, /* max iv sz */ 00186 NAME_SZ = 80, /* max one line */ 00187 00188 PEM_PASS_READ = 0, 00189 PEM_PASS_WRITE = 1, 00190 }; 00191 00192 00193 typedef int (pem_password_cb)(char* passwd, int sz, int rw, void* userdata); 00194 00195 typedef struct EncryptedInfo { 00196 pem_password_cb* passwd_cb; 00197 void* passwd_userdata; 00198 00199 long consumed; /* tracks PEM bytes consumed */ 00200 00201 int cipherType; 00202 word32 keySz; 00203 word32 ivSz; /* salt or encrypted IV size */ 00204 00205 char name[NAME_SZ]; /* cipher name, such as "DES-CBC" */ 00206 byte iv[IV_SZ]; /* salt or encrypted IV */ 00207 00208 word16 set:1; /* if encryption set */ 00209 } EncryptedInfo; 00210 00211 00212 #define WOLFSSL_ASN1_INTEGER_MAX 20 00213 typedef struct WOLFSSL_ASN1_INTEGER { 00214 /* size can be increased set at 20 for tag, length then to hold at least 16 00215 * byte type */ 00216 unsigned char intData[WOLFSSL_ASN1_INTEGER_MAX]; 00217 /* ASN_INTEGER | LENGTH | hex of number */ 00218 unsigned char negative; /* negative number flag */ 00219 00220 unsigned char* data; 00221 unsigned int dataMax; /* max size of data buffer */ 00222 unsigned int isDynamic:1; /* flag for if data pointer dynamic (1 is yes 0 is no) */ 00223 00224 int length; 00225 int type; 00226 } WOLFSSL_ASN1_INTEGER; 00227 00228 00229 #if defined(WOLFSSL_CERT_GEN) || defined(WOLFSSL_CERT_EXT) 00230 #ifdef WOLFSSL_EKU_OID 00231 #ifndef CTC_MAX_EKU_NB 00232 #define CTC_MAX_EKU_NB 1 00233 #endif 00234 #ifndef CTC_MAX_EKU_OID_SZ 00235 #define CTC_MAX_EKU_OID_SZ 30 00236 #endif 00237 #else 00238 #undef CTC_MAX_EKU_OID_SZ 00239 #define CTC_MAX_EKU_OID_SZ 0 00240 #endif 00241 #endif /* WOLFSSL_CERT_GEN || WOLFSSL_CERT_EXT */ 00242 00243 #ifdef WOLFSSL_CERT_GEN 00244 00245 #ifdef WOLFSSL_MULTI_ATTRIB 00246 #ifndef CTC_MAX_ATTRIB 00247 #define CTC_MAX_ATTRIB 4 00248 #endif 00249 00250 /* ASN Encoded Name field */ 00251 typedef struct NameAttrib { 00252 int sz; /* actual string value length */ 00253 int id; /* id of name */ 00254 int type; /* enc of name */ 00255 char value[CTC_NAME_SIZE]; /* name */ 00256 } NameAttrib; 00257 #endif /* WOLFSSL_MULTI_ATTRIB */ 00258 00259 00260 typedef struct CertName { 00261 char country[CTC_NAME_SIZE]; 00262 char countryEnc; 00263 char state[CTC_NAME_SIZE]; 00264 char stateEnc; 00265 char locality[CTC_NAME_SIZE]; 00266 char localityEnc; 00267 char sur[CTC_NAME_SIZE]; 00268 char surEnc; 00269 char org[CTC_NAME_SIZE]; 00270 char orgEnc; 00271 char unit[CTC_NAME_SIZE]; 00272 char unitEnc; 00273 char commonName[CTC_NAME_SIZE]; 00274 char commonNameEnc; 00275 char serialDev[CTC_NAME_SIZE]; 00276 char serialDevEnc; 00277 #ifdef WOLFSSL_CERT_EXT 00278 char busCat[CTC_NAME_SIZE]; 00279 char busCatEnc; 00280 char joiC[CTC_NAME_SIZE]; 00281 char joiCEnc; 00282 char joiSt[CTC_NAME_SIZE]; 00283 char joiStEnc; 00284 #endif 00285 char email[CTC_NAME_SIZE]; /* !!!! email has to be last !!!! */ 00286 #ifdef WOLFSSL_MULTI_ATTRIB 00287 NameAttrib name[CTC_MAX_ATTRIB]; 00288 #endif 00289 } CertName; 00290 00291 00292 /* for user to fill for certificate generation */ 00293 typedef struct Cert { 00294 int version; /* x509 version */ 00295 byte serial[CTC_SERIAL_SIZE]; /* serial number */ 00296 int serialSz; /* serial size */ 00297 int sigType; /* signature algo type */ 00298 CertName issuer; /* issuer info */ 00299 int daysValid; /* validity days */ 00300 int selfSigned; /* self signed flag */ 00301 CertName subject; /* subject info */ 00302 int isCA; /* is this going to be a CA */ 00303 /* internal use only */ 00304 int bodySz; /* pre sign total size */ 00305 int keyType; /* public key type of subject */ 00306 #ifdef WOLFSSL_ALT_NAMES 00307 byte altNames[CTC_MAX_ALT_SIZE]; /* altNames copy */ 00308 int altNamesSz; /* altNames size in bytes */ 00309 byte beforeDate[CTC_DATE_SIZE]; /* before date copy */ 00310 int beforeDateSz; /* size of copy */ 00311 byte afterDate[CTC_DATE_SIZE]; /* after date copy */ 00312 int afterDateSz; /* size of copy */ 00313 #endif 00314 #ifdef WOLFSSL_CERT_EXT 00315 byte skid[CTC_MAX_SKID_SIZE]; /* Subject Key Identifier */ 00316 int skidSz; /* SKID size in bytes */ 00317 byte akid[CTC_MAX_AKID_SIZE]; /* Authority Key Identifier */ 00318 int akidSz; /* AKID size in bytes */ 00319 word16 keyUsage; /* Key Usage */ 00320 byte extKeyUsage; /* Extended Key Usage */ 00321 #ifdef WOLFSSL_EKU_OID 00322 /* Extended Key Usage OIDs */ 00323 byte extKeyUsageOID[CTC_MAX_EKU_NB][CTC_MAX_EKU_OID_SZ]; 00324 byte extKeyUsageOIDSz[CTC_MAX_EKU_NB]; 00325 #endif 00326 char certPolicies[CTC_MAX_CERTPOL_NB][CTC_MAX_CERTPOL_SZ]; 00327 word16 certPoliciesNb; /* Number of Cert Policy */ 00328 byte issRaw[sizeof(CertName)]; /* raw issuer info */ 00329 byte sbjRaw[sizeof(CertName)]; /* raw subject info */ 00330 #endif 00331 #ifdef WOLFSSL_CERT_REQ 00332 char challengePw[CTC_NAME_SIZE]; 00333 int challengePwPrintableString; /* encode as PrintableString */ 00334 #endif 00335 void* decodedCert; /* internal DecodedCert allocated from heap */ 00336 byte* der; /* Pointer to buffer of current DecodedCert cache */ 00337 void* heap; /* heap hint */ 00338 } Cert; 00339 00340 00341 /* Initialize and Set Certificate defaults: 00342 version = 3 (0x2) 00343 serial = 0 (Will be randomly generated) 00344 sigType = SHA_WITH_RSA 00345 issuer = blank 00346 daysValid = 500 00347 selfSigned = 1 (true) use subject as issuer 00348 subject = blank 00349 isCA = 0 (false) 00350 keyType = RSA_KEY (default) 00351 */ 00352 WOLFSSL_API int wc_InitCert(Cert*); 00353 WOLFSSL_API int wc_MakeCert_ex(Cert* cert, byte* derBuffer, word32 derSz, 00354 int keyType, void* key, WC_RNG* rng); 00355 WOLFSSL_API int wc_MakeCert(Cert*, byte* derBuffer, word32 derSz, RsaKey*, 00356 ecc_key*, WC_RNG*); 00357 #ifdef WOLFSSL_CERT_REQ 00358 WOLFSSL_API int wc_MakeCertReq_ex(Cert*, byte* derBuffer, word32 derSz, 00359 int, void*); 00360 WOLFSSL_API int wc_MakeCertReq(Cert*, byte* derBuffer, word32 derSz, 00361 RsaKey*, ecc_key*); 00362 #endif 00363 WOLFSSL_API int wc_SignCert_ex(int requestSz, int sType, byte* buffer, 00364 word32 buffSz, int keyType, void* key, 00365 WC_RNG* rng); 00366 WOLFSSL_API int wc_SignCert(int requestSz, int sigType, byte* derBuffer, 00367 word32 derSz, RsaKey*, ecc_key*, WC_RNG*); 00368 WOLFSSL_API int wc_MakeSelfCert(Cert*, byte* derBuffer, word32 derSz, RsaKey*, 00369 WC_RNG*); 00370 WOLFSSL_API int wc_SetIssuer(Cert*, const char*); 00371 WOLFSSL_API int wc_SetSubject(Cert*, const char*); 00372 #ifdef WOLFSSL_ALT_NAMES 00373 WOLFSSL_API int wc_SetAltNames(Cert*, const char*); 00374 #endif 00375 00376 #ifdef WOLFSSL_CERT_GEN_CACHE 00377 WOLFSSL_API void wc_SetCert_Free(Cert* cert); 00378 #endif 00379 00380 WOLFSSL_API int wc_SetIssuerBuffer(Cert*, const byte*, int); 00381 WOLFSSL_API int wc_SetSubjectBuffer(Cert*, const byte*, int); 00382 WOLFSSL_API int wc_SetAltNamesBuffer(Cert*, const byte*, int); 00383 WOLFSSL_API int wc_SetDatesBuffer(Cert*, const byte*, int); 00384 00385 #ifndef NO_ASN_TIME 00386 WOLFSSL_API int wc_GetCertDates(Cert* cert, struct tm* before, 00387 struct tm* after); 00388 #endif 00389 00390 #ifdef WOLFSSL_CERT_EXT 00391 WOLFSSL_API int wc_SetAuthKeyIdFromPublicKey_ex(Cert *cert, int keyType, 00392 void* key); 00393 WOLFSSL_API int wc_SetAuthKeyIdFromPublicKey(Cert *cert, RsaKey *rsakey, 00394 ecc_key *eckey); 00395 WOLFSSL_API int wc_SetAuthKeyIdFromCert(Cert *cert, const byte *der, int derSz); 00396 WOLFSSL_API int wc_SetAuthKeyId(Cert *cert, const char* file); 00397 WOLFSSL_API int wc_SetSubjectKeyIdFromPublicKey_ex(Cert *cert, int keyType, 00398 void* key); 00399 WOLFSSL_API int wc_SetSubjectKeyIdFromPublicKey(Cert *cert, RsaKey *rsakey, 00400 ecc_key *eckey); 00401 WOLFSSL_API int wc_SetSubjectKeyId(Cert *cert, const char* file); 00402 WOLFSSL_API int wc_GetSubjectRaw(byte **subjectRaw, Cert *cert); 00403 WOLFSSL_API int wc_SetSubjectRaw(Cert* cert, const byte* der, int derSz); 00404 WOLFSSL_API int wc_SetIssuerRaw(Cert* cert, const byte* der, int derSz); 00405 00406 #ifdef HAVE_NTRU 00407 WOLFSSL_API int wc_SetSubjectKeyIdFromNtruPublicKey(Cert *cert, byte *ntruKey, 00408 word16 ntruKeySz); 00409 #endif 00410 00411 /* Set the KeyUsage. 00412 * Value is a string separated tokens with ','. Accepted tokens are : 00413 * digitalSignature,nonRepudiation,contentCommitment,keyCertSign,cRLSign, 00414 * dataEncipherment,keyAgreement,keyEncipherment,encipherOnly and decipherOnly. 00415 * 00416 * nonRepudiation and contentCommitment are for the same usage. 00417 */ 00418 WOLFSSL_API int wc_SetKeyUsage(Cert *cert, const char *value); 00419 00420 /* Set ExtendedKeyUsage 00421 * Value is a string separated tokens with ','. Accepted tokens are : 00422 * any,serverAuth,clientAuth,codeSigning,emailProtection,timeStamping,OCSPSigning 00423 */ 00424 WOLFSSL_API int wc_SetExtKeyUsage(Cert *cert, const char *value); 00425 00426 00427 #ifdef WOLFSSL_EKU_OID 00428 /* Set ExtendedKeyUsage with unique OID 00429 * oid is expected to be in byte representation 00430 */ 00431 WOLFSSL_API int wc_SetExtKeyUsageOID(Cert *cert, const char *oid, word32 sz, 00432 byte idx, void* heap); 00433 #endif /* WOLFSSL_EKU_OID */ 00434 #endif /* WOLFSSL_CERT_EXT */ 00435 00436 #ifdef HAVE_NTRU 00437 WOLFSSL_API int wc_MakeNtruCert(Cert*, byte* derBuffer, word32 derSz, 00438 const byte* ntruKey, word16 keySz, 00439 WC_RNG*); 00440 #endif 00441 00442 #endif /* WOLFSSL_CERT_GEN */ 00443 00444 WOLFSSL_API int wc_GetDateInfo(const byte* certDate, int certDateSz, 00445 const byte** date, byte* format, int* length); 00446 #ifndef NO_ASN_TIME 00447 WOLFSSL_API int wc_GetDateAsCalendarTime(const byte* date, int length, 00448 byte format, struct tm* time); 00449 #endif 00450 00451 #if defined(WOLFSSL_PEM_TO_DER) || defined(WOLFSSL_DER_TO_PEM) 00452 00453 WOLFSSL_API int wc_PemGetHeaderFooter(int type, const char** header, 00454 const char** footer); 00455 00456 #endif 00457 00458 WOLFSSL_API int wc_AllocDer(DerBuffer** pDer, word32 length, int type, void* heap); 00459 WOLFSSL_API void wc_FreeDer(DerBuffer** pDer); 00460 00461 #ifdef WOLFSSL_PEM_TO_DER 00462 WOLFSSL_API int wc_PemToDer(const unsigned char* buff, long longSz, int type, 00463 DerBuffer** pDer, void* heap, EncryptedInfo* info, int* eccKey); 00464 00465 WOLFSSL_API int wc_KeyPemToDer(const unsigned char*, int, 00466 unsigned char*, int, const char*); 00467 WOLFSSL_API int wc_CertPemToDer(const unsigned char*, int, 00468 unsigned char*, int, int); 00469 #endif /* WOLFSSL_PEM_TO_DER */ 00470 00471 #if defined(WOLFSSL_CERT_EXT) || defined(WOLFSSL_PUB_PEM_TO_DER) 00472 #ifndef NO_FILESYSTEM 00473 WOLFSSL_API int wc_PemPubKeyToDer(const char* fileName, 00474 unsigned char* derBuf, int derSz); 00475 #endif 00476 00477 WOLFSSL_API int wc_PubKeyPemToDer(const unsigned char*, int, 00478 unsigned char*, int); 00479 #endif /* WOLFSSL_CERT_EXT || WOLFSSL_PUB_PEM_TO_DER */ 00480 00481 #ifdef WOLFSSL_CERT_GEN 00482 #ifndef NO_FILESYSTEM 00483 WOLFSSL_API int wc_PemCertToDer(const char* fileName, 00484 unsigned char* derBuf, int derSz); 00485 #endif 00486 #endif /* WOLFSSL_CERT_GEN */ 00487 00488 #ifdef WOLFSSL_DER_TO_PEM 00489 WOLFSSL_API int wc_DerToPem(const byte* der, word32 derSz, byte* output, 00490 word32 outputSz, int type); 00491 WOLFSSL_API int wc_DerToPemEx(const byte* der, word32 derSz, byte* output, 00492 word32 outputSz, byte *cipherIno, int type); 00493 #endif 00494 00495 #ifndef NO_RSA 00496 #if !defined(HAVE_USER_RSA) 00497 WOLFSSL_API int wc_RsaPublicKeyDecode_ex(const byte* input, word32* inOutIdx, 00498 word32 inSz, const byte** n, word32* nSz, const byte** e, word32* eSz); 00499 #endif 00500 WOLFSSL_API int wc_RsaPublicKeyDerSize(RsaKey* key, int with_header); 00501 #endif 00502 00503 #ifdef HAVE_ECC 00504 /* private key helpers */ 00505 WOLFSSL_API int wc_EccPrivateKeyDecode(const byte*, word32*, 00506 ecc_key*, word32); 00507 WOLFSSL_API int wc_EccKeyToDer(ecc_key*, byte* output, word32 inLen); 00508 WOLFSSL_API int wc_EccPrivateKeyToDer(ecc_key* key, byte* output, 00509 word32 inLen); 00510 WOLFSSL_API int wc_EccPrivateKeyToPKCS8(ecc_key* key, byte* output, 00511 word32* outLen); 00512 00513 /* public key helper */ 00514 WOLFSSL_API int wc_EccPublicKeyDecode(const byte*, word32*, 00515 ecc_key*, word32); 00516 WOLFSSL_API int wc_EccPublicKeyToDer(ecc_key*, byte* output, 00517 word32 inLen, int with_AlgCurve); 00518 WOLFSSL_API int wc_EccPublicKeyDerSize(ecc_key*, int with_AlgCurve); 00519 #endif 00520 00521 #ifdef HAVE_ED25519 00522 /* private key helpers */ 00523 WOLFSSL_API int wc_Ed25519PrivateKeyDecode(const byte*, word32*, 00524 ed25519_key*, word32); 00525 WOLFSSL_API int wc_Ed25519KeyToDer(ed25519_key* key, byte* output, 00526 word32 inLen); 00527 WOLFSSL_API int wc_Ed25519PrivateKeyToDer(ed25519_key* key, byte* output, 00528 word32 inLen); 00529 00530 /* public key helper */ 00531 WOLFSSL_API int wc_Ed25519PublicKeyDecode(const byte*, word32*, 00532 ed25519_key*, word32); 00533 #if (defined(WOLFSSL_CERT_GEN) || defined(WOLFSSL_KEY_GEN)) 00534 WOLFSSL_API int wc_Ed25519PublicKeyToDer(ed25519_key*, byte* output, 00535 word32 inLen, int with_AlgCurve); 00536 #endif 00537 #endif 00538 00539 #ifdef HAVE_ED448 00540 /* private key helpers */ 00541 WOLFSSL_API int wc_Ed448PrivateKeyDecode(const byte*, word32*, 00542 ed448_key*, word32); 00543 WOLFSSL_API int wc_Ed448KeyToDer(ed448_key* key, byte* output, 00544 word32 inLen); 00545 WOLFSSL_API int wc_Ed448PrivateKeyToDer(ed448_key* key, byte* output, 00546 word32 inLen); 00547 00548 /* public key helper */ 00549 WOLFSSL_API int wc_Ed448PublicKeyDecode(const byte*, word32*, 00550 ed448_key*, word32); 00551 #if (defined(WOLFSSL_CERT_GEN) || defined(WOLFSSL_KEY_GEN)) 00552 WOLFSSL_API int wc_Ed448PublicKeyToDer(ed448_key*, byte* output, 00553 word32 inLen, int with_AlgCurve); 00554 #endif 00555 #endif 00556 00557 /* DER encode signature */ 00558 WOLFSSL_API word32 wc_EncodeSignature(byte* out, const byte* digest, 00559 word32 digSz, int hashOID); 00560 WOLFSSL_API int wc_GetCTC_HashOID(int type); 00561 00562 WOLFSSL_API int wc_GetPkcs8TraditionalOffset(byte* input, 00563 word32* inOutIdx, word32 sz); 00564 WOLFSSL_API int wc_CreatePKCS8Key(byte* out, word32* outSz, 00565 byte* key, word32 keySz, int algoID, const byte* curveOID, word32 oidSz); 00566 00567 #ifndef NO_ASN_TIME 00568 /* Time */ 00569 /* Returns seconds (Epoch/UTC) 00570 * timePtr: is "time_t", which is typically "long" 00571 * Example: 00572 long lTime; 00573 rc = wc_GetTime(&lTime, (word32)sizeof(lTime)); 00574 */ 00575 WOLFSSL_API int wc_GetTime(void* timePtr, word32 timeSize); 00576 #endif 00577 00578 #ifdef WOLFSSL_ENCRYPTED_KEYS 00579 WOLFSSL_API int wc_EncryptedInfoGet(EncryptedInfo* info, 00580 const char* cipherInfo); 00581 #endif 00582 00583 00584 #ifdef WOLFSSL_CERT_PIV 00585 00586 typedef struct _wc_CertPIV { 00587 const byte* cert; 00588 word32 certSz; 00589 const byte* certErrDet; 00590 word32 certErrDetSz; 00591 const byte* nonce; /* Identiv Only */ 00592 word32 nonceSz; /* Identiv Only */ 00593 const byte* signedNonce; /* Identiv Only */ 00594 word32 signedNonceSz; /* Identiv Only */ 00595 00596 /* flags */ 00597 word16 compression:2; 00598 word16 isX509:1; 00599 word16 isIdentiv:1; 00600 } wc_CertPIV; 00601 00602 WOLFSSL_API int wc_ParseCertPIV(wc_CertPIV* cert, const byte* buf, word32 totalSz); 00603 #endif /* WOLFSSL_CERT_PIV */ 00604 00605 00606 #ifdef __cplusplus 00607 } /* extern "C" */ 00608 #endif 00609 00610 #endif /* WOLF_CRYPT_ASN_PUBLIC_H */ 00611
Generated on Tue Jul 12 2022 20:58:34 by
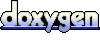