
Simple TCP Client
Dependencies: CyaSSL EthernetInterface mbed-rtos mbed
Fork of SimpleClient-TCP-FRDM by
main.cpp
00001 #include "mbed.h" 00002 #include "EthernetInterface.h" 00003 00004 /*** SSL #include <cyassl/ssl.h> ***/ 00005 00006 #define PORT 80 /*** SSL 443 ***/ 00007 00008 #define err_sys(m) puts(m) 00009 00010 TCPSocketConnection socket; 00011 00012 /*** SSL 00013 static int SocketReceive(CYASSL* ssl, char *buf, int sz, void *ctx) 00014 { 00015 int n ; 00016 int i ; 00017 #define RECV_RETRY 3 00018 for(i=0; i<RECV_RETRY; i++) { 00019 n = socket.receive(buf, sz) ; 00020 if(n >= 0)return n ; 00021 } 00022 printf("SocketReceive:%d/%d\n", n, sz) ; 00023 return n ; 00024 } 00025 00026 static int SocketSend(CYASSL* ssl, char *buf, int sz, void *ctx) 00027 { 00028 int n ; 00029 00030 n = socket.send(buf, sz); 00031 if(n > 0) { 00032 return n ; 00033 } else printf("SocketSend:%d/%d\n", n, sz); 00034 return n ; 00035 } 00036 ***/ 00037 00038 EthernetInterface eth; 00039 00040 void net_main(void const *av) 00041 { 00042 char server_ip[20] ; 00043 00044 eth.init(); //Use DHCP 00045 printf("===== Simple TCP Client ========\n") ; 00046 /*** SSL 00047 printf("===== Simple SSL Client ========\n") ; 00048 ***/ 00049 00050 while(1) { 00051 if(eth.connect()== 0)break ; 00052 wait(0.1); 00053 } 00054 printf("Client IP: %s\n", eth.getIPAddress()); 00055 00056 /*** SSL 00057 CYASSL_CTX* ctx = 0; 00058 CYASSL* ssl = 0; 00059 00060 CYASSL_METHOD* method = CyaTLSv1_2_client_method(); 00061 ***/ 00062 00063 /* Initialize CyaSSL Context */ 00064 /*** SSL 00065 ctx = CyaSSL_CTX_new(method); 00066 if (ctx == NULL) 00067 err_sys("unable to get ctx"); 00068 CyaSSL_CTX_set_verify(ctx, SSL_VERIFY_NONE, 0); 00069 CyaSSL_SetIORecv(ctx, SocketReceive) ; 00070 CyaSSL_SetIOSend(ctx, SocketSend) ; 00071 end SSL ***/ 00072 00073 socket.set_blocking(false, 300) ; 00074 printf("Server IP: ") ; 00075 for(int i=0; i<sizeof(server_ip); i++) { 00076 if((server_ip[i] = getchar()) == '\r') { 00077 server_ip[i] = '\0' ; 00078 putchar('\n') ; 00079 break ; 00080 } else putchar(server_ip[i]) ; 00081 } 00082 00083 while (socket.connect(server_ip, PORT) < 0) { 00084 printf("Unable to connect to (%s) on port (%d)\n", server_ip, PORT); 00085 wait(1); 00086 } 00087 printf("TCP Connected\n") ; 00088 00089 /*** SSL 00090 ssl = CyaSSL_new(ctx); 00091 if (ssl == NULL) 00092 err_sys("unable to get SSL object"); 00093 if (CyaSSL_connect(ssl) != SSL_SUCCESS) { 00094 int err = CyaSSL_get_error(ssl, 0); 00095 printf("err = %d, %s\n", err, 00096 CyaSSL_ERR_error_string(err, "\n")); 00097 err_sys("SSL Connection Error"); 00098 } 00099 printf("SSL Connected\n") ; 00100 ***/ 00101 00102 char msg[] = "GET /congrats.html HTTP/1.0\r\nConnection: Close\r\n\r\n" ; 00103 // const char msg[] = "Hello World\r\n" ; 00104 00105 if ( 00106 /*** SSL 00107 CyaSSL_write(ssl, msg, sizeof(msg)-1) != (sizeof(msg)-1)) ***/ 00108 socket.send(msg, sizeof(msg)-1) != (sizeof(msg)-1)) 00109 err_sys("CyaSSL_write failed"); 00110 00111 char buf[1024]; 00112 int n ; 00113 puts("Server Response:\n") ; 00114 do { 00115 n = /*** SSL CyaSSL_read(ssl, buf, sizeof(buf)-1); ***/ 00116 socket.receive(buf, sizeof(buf)-1); 00117 if (n >= 0) { 00118 buf[n] = 0; 00119 printf("%s", buf); 00120 } else break ; 00121 } while(n > 0) ; 00122 puts("=== === === ===") ; 00123 /*** SSL CyaSSL_free(ssl) ; ***/ 00124 socket.close(); 00125 /*** SSL CyaSSL_CTX_free(ctx) ; ***/ 00126 eth.disconnect(); 00127 } 00128 00129 main() 00130 { 00131 00132 #define STACK_SIZE 20000 00133 Thread t(net_main, NULL, osPriorityNormal, STACK_SIZE); 00134 00135 while (true) { 00136 Thread::wait(1000); 00137 } 00138 }
Generated on Sat Jul 16 2022 04:23:33 by
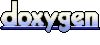