
Simple HTTPS access example with HTTPClient class
Dependencies: EthernetInterface-FRDM HTTPClient mbed-rtos mbed
main.cpp
00001 #include "mbed.h" 00002 #include "EthernetInterface.h" 00003 #include "HTTPClient.h" 00004 00005 EthernetInterface eth; 00006 HTTPClient http; 00007 char recvBuff[1024*20]; 00008 00009 void getline(char *line, int size) { 00010 for(int i=0; i<size; i++) { 00011 if((line[i] = getchar()) == '\r') { 00012 line[i] = '\0' ; 00013 putchar('\n') ; 00014 break ; 00015 } else putchar(line[i]) ; 00016 } 00017 } 00018 00019 void net_main(void const *av) 00020 { 00021 int ret ; 00022 00023 eth.init(); //Use DHCP 00024 printf("HTTP Client, Starting,...\n") ; 00025 while(1) { 00026 if(eth.connect() == 0)break ; 00027 printf("Retry\n") ; 00028 } 00029 00030 /*** HTTP ***/ 00031 printf("\nFetching HTTP\n"); 00032 ret = http.get("http://SERVER_IP/574d76fcb/keys.txt", recvBuff, sizeof(recvBuff)); 00033 if (!ret) { 00034 printf("Result: %s\n", recvBuff); 00035 } else { 00036 printf("Error - ret = %d - HTTP return code = %d\n", ret, http.getHTTPResponseCode()); 00037 } 00038 00039 /*** HTTPS (SSL) ***/ 00040 printf("\nFetching HTTPS\n"); 00041 ret = http.get("https://SERVER_IP/574d76fcb/keys.txt", recvBuff, sizeof(recvBuff)); 00042 if (!ret) { 00043 printf("Result: %s\n", recvBuff); 00044 } else { 00045 printf("Error - ret = %d - HTTP return code = %d\n", ret, http.getHTTPResponseCode()); 00046 } 00047 00048 eth.disconnect(); 00049 while(1) { 00050 } 00051 } 00052 00053 main() 00054 { 00055 00056 #define STACK_SIZE 20000 00057 Thread t(net_main, NULL, osPriorityNormal, STACK_SIZE); 00058 00059 while (true) { 00060 Thread::wait(1000); 00061 } 00062 }
Generated on Sat Jul 16 2022 17:13:54 by
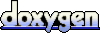