CyaSSL 3.0.0
Dependents: HTTPClient-SSL HTTPClient HTTPClient-SSL http_access ... more
blake2-impl.h
00001 /* 00002 BLAKE2 reference source code package - reference C implementations 00003 00004 Written in 2012 by Samuel Neves <sneves@dei.uc.pt> 00005 00006 To the extent possible under law, the author(s) have dedicated all copyright 00007 and related and neighboring rights to this software to the public domain 00008 worldwide. This software is distributed without any warranty. 00009 00010 You should have received a copy of the CC0 Public Domain Dedication along with 00011 this software. If not, see <http://creativecommons.org/publicdomain/zero/1.0/>. 00012 */ 00013 /* blake2-impl.h 00014 * 00015 * Copyright (C) 2006-2014 wolfSSL Inc. 00016 * 00017 * This file is part of CyaSSL. 00018 * 00019 * CyaSSL is free software; you can redistribute it and/or modify 00020 * it under the terms of the GNU General Public License as published by 00021 * the Free Software Foundation; either version 2 of the License, or 00022 * (at your option) any later version. 00023 * 00024 * CyaSSL is distributed in the hope that it will be useful, 00025 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00026 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00027 * GNU General Public License for more details. 00028 * 00029 * You should have received a copy of the GNU General Public License 00030 * along with this program; if not, write to the Free Software 00031 * Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA 00032 */ 00033 00034 00035 #ifndef CTAOCRYPT_BLAKE2_IMPL_H 00036 #define CTAOCRYPT_BLAKE2_IMPL_H 00037 00038 #include <cyassl/ctaocrypt/types.h> 00039 00040 static inline word32 load32( const void *src ) 00041 { 00042 #if defined(LITTLE_ENDIAN_ORDER) 00043 return *( word32 * )( src ); 00044 #else 00045 const byte *p = ( byte * )src; 00046 word32 w = *p++; 00047 w |= ( word32 )( *p++ ) << 8; 00048 w |= ( word32 )( *p++ ) << 16; 00049 w |= ( word32 )( *p++ ) << 24; 00050 return w; 00051 #endif 00052 } 00053 00054 static inline word64 load64( const void *src ) 00055 { 00056 #if defined(LITTLE_ENDIAN_ORDER) 00057 return *( word64 * )( src ); 00058 #else 00059 const byte *p = ( byte * )src; 00060 word64 w = *p++; 00061 w |= ( word64 )( *p++ ) << 8; 00062 w |= ( word64 )( *p++ ) << 16; 00063 w |= ( word64 )( *p++ ) << 24; 00064 w |= ( word64 )( *p++ ) << 32; 00065 w |= ( word64 )( *p++ ) << 40; 00066 w |= ( word64 )( *p++ ) << 48; 00067 w |= ( word64 )( *p++ ) << 56; 00068 return w; 00069 #endif 00070 } 00071 00072 static inline void store32( void *dst, word32 w ) 00073 { 00074 #if defined(LITTLE_ENDIAN_ORDER) 00075 *( word32 * )( dst ) = w; 00076 #else 00077 byte *p = ( byte * )dst; 00078 *p++ = ( byte )w; w >>= 8; 00079 *p++ = ( byte )w; w >>= 8; 00080 *p++ = ( byte )w; w >>= 8; 00081 *p++ = ( byte )w; 00082 #endif 00083 } 00084 00085 static inline void store64( void *dst, word64 w ) 00086 { 00087 #if defined(LITTLE_ENDIAN_ORDER) 00088 *( word64 * )( dst ) = w; 00089 #else 00090 byte *p = ( byte * )dst; 00091 *p++ = ( byte )w; w >>= 8; 00092 *p++ = ( byte )w; w >>= 8; 00093 *p++ = ( byte )w; w >>= 8; 00094 *p++ = ( byte )w; w >>= 8; 00095 *p++ = ( byte )w; w >>= 8; 00096 *p++ = ( byte )w; w >>= 8; 00097 *p++ = ( byte )w; w >>= 8; 00098 *p++ = ( byte )w; 00099 #endif 00100 } 00101 00102 static inline word64 load48( const void *src ) 00103 { 00104 const byte *p = ( const byte * )src; 00105 word64 w = *p++; 00106 w |= ( word64 )( *p++ ) << 8; 00107 w |= ( word64 )( *p++ ) << 16; 00108 w |= ( word64 )( *p++ ) << 24; 00109 w |= ( word64 )( *p++ ) << 32; 00110 w |= ( word64 )( *p++ ) << 40; 00111 return w; 00112 } 00113 00114 static inline void store48( void *dst, word64 w ) 00115 { 00116 byte *p = ( byte * )dst; 00117 *p++ = ( byte )w; w >>= 8; 00118 *p++ = ( byte )w; w >>= 8; 00119 *p++ = ( byte )w; w >>= 8; 00120 *p++ = ( byte )w; w >>= 8; 00121 *p++ = ( byte )w; w >>= 8; 00122 *p++ = ( byte )w; 00123 } 00124 00125 static inline word32 rotl32( const word32 w, const unsigned c ) 00126 { 00127 return ( w << c ) | ( w >> ( 32 - c ) ); 00128 } 00129 00130 static inline word64 rotl64( const word64 w, const unsigned c ) 00131 { 00132 return ( w << c ) | ( w >> ( 64 - c ) ); 00133 } 00134 00135 static inline word32 rotr32( const word32 w, const unsigned c ) 00136 { 00137 return ( w >> c ) | ( w << ( 32 - c ) ); 00138 } 00139 00140 static inline word64 rotr64( const word64 w, const unsigned c ) 00141 { 00142 return ( w >> c ) | ( w << ( 64 - c ) ); 00143 } 00144 00145 /* prevents compiler optimizing out memset() */ 00146 static inline void secure_zero_memory( void *v, word64 n ) 00147 { 00148 volatile byte *p = ( volatile byte * )v; 00149 00150 while( n-- ) *p++ = 0; 00151 } 00152 00153 #endif /* CTAOCRYPT_BLAKE2_IMPL_H */ 00154 00155
Generated on Wed Jul 13 2022 02:18:39 by
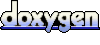