
nRF24L01P Hello World example for mbed 6
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "nRF24L01P.h" 00003 00004 BufferedSerial pc(USBTX, USBRX); // tx, rx 00005 00006 nRF24L01P my_nrf24l01p(p5, p6, p7, p8, p9, p10); // mosi, miso, sck, csn, ce, irq 00007 00008 FileHandle *mbed::mbed_override_console(int fd) 00009 { 00010 return &pc; 00011 } 00012 00013 int main() { 00014 enum { TRANSFER_SIZE = 1 }; 00015 00016 char txData[TRANSFER_SIZE], rxData[TRANSFER_SIZE]; 00017 int txDataCnt = 0; 00018 int rxDataCnt = 0; 00019 00020 my_nrf24l01p.powerUp(); 00021 00022 // Display the (default) setup of the nRF24L01+ chip 00023 printf( "nRF24L01+ Frequency : %d MHz\r\n", my_nrf24l01p.getRfFrequency() ); 00024 printf( "nRF24L01+ Output power : %d dBm\r\n", my_nrf24l01p.getRfOutputPower() ); 00025 printf( "nRF24L01+ Data Rate : %d kbps\r\n", my_nrf24l01p.getAirDataRate() ); 00026 printf( "nRF24L01+ TX Address : 0x%010llX\r\n", my_nrf24l01p.getTxAddress() ); 00027 printf( "nRF24L01+ RX Address : 0x%010llX\r\n", my_nrf24l01p.getRxAddress() ); 00028 00029 printf( "Type keys to test transfers:\r\n"); 00030 00031 my_nrf24l01p.setTransferSize( TRANSFER_SIZE ); 00032 my_nrf24l01p.setRfFrequency(NRF24L01P_MAX_RF_FREQUENCY); 00033 00034 my_nrf24l01p.setReceiveMode(); 00035 my_nrf24l01p.enable(); 00036 00037 while (1) { 00038 00039 // If we've received anything over the host serial link... 00040 if ( pc.readable() ) { 00041 00042 // ...add it to the transmit buffer 00043 pc.read(&txData[txDataCnt], TRANSFER_SIZE); 00044 txDataCnt++; 00045 00046 // If the transmit buffer is full 00047 if ( txDataCnt >= sizeof( txData ) ) { 00048 00049 // Send the transmitbuffer via the nRF24L01+ 00050 my_nrf24l01p.write( NRF24L01P_PIPE_P0, txData, txDataCnt ); 00051 00052 txDataCnt = 0; 00053 } 00054 } 00055 00056 // If we've received anything in the nRF24L01+... 00057 if ( my_nrf24l01p.readable() ) { 00058 00059 // ...read the data into the receive buffer 00060 rxDataCnt = my_nrf24l01p.read( NRF24L01P_PIPE_P0, rxData, sizeof( rxData ) ); 00061 00062 // Display the receive buffer contents via the host serial link 00063 for ( int i = 0; rxDataCnt > 0; rxDataCnt--, i++ ) { 00064 00065 pc.write(&rxData[i], sizeof( rxData )); 00066 } 00067 } 00068 } 00069 }
Generated on Wed Aug 17 2022 13:43:19 by
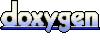