PS2 Library
Embed:
(wiki syntax)
Show/hide line numbers
PS2KB.h
00001 /** 00002 * PS/2 keyboard interface control class (Version 0.0.1) 00003 * 00004 * Copyright (C) 2010 Shinichiro Nakamura (CuBeatSystems) 00005 * http://shinta.main.jp/ 00006 */ 00007 00008 #ifndef _PS2KB_H_ 00009 #define _PS2KB_H_ 00010 00011 #include "mbed.h" 00012 00013 /** 00014 * PS/2 keyboard interface control class. 00015 */ 00016 class PS2KB { 00017 public: 00018 /** 00019 * Create. 00020 * 00021 * @param clk_pin Clock pin. 00022 * @param dat_pin Data pin. 00023 */ 00024 PS2KB(PinName clk_pin, PinName dat_pin); 00025 00026 /** 00027 * Destory. 00028 */ 00029 virtual ~PS2KB(); 00030 00031 /** 00032 * Get a data from a PS/2 device. 00033 * 00034 * @return A data from a PS/2 device. 00035 */ 00036 virtual int getc(void); 00037 00038 /** 00039 * Set timeout. 00040 * 00041 * @param ms Timeout ms. 00042 */ 00043 virtual void setTimeout(int ms); 00044 00045 private: 00046 static const int RINGBUFSIZ = 256; 00047 InterruptIn clk; /**< Interrupt input for CLK. */ 00048 DigitalIn dat; /**< Digital input for DAT. */ 00049 Timeout wdt; /**< Watch dog timer. */ 00050 Timer tot; /**< Timeout timer. */ 00051 int timeout; /**< Timeout[ms] for getc(). */ 00052 00053 typedef struct { 00054 int bitcnt; 00055 int cStart; 00056 int cEnd; 00057 uint8_t buffer[RINGBUFSIZ]; 00058 } work_t; 00059 work_t work; 00060 00061 void func_timeout(void); 00062 void func_fall(void); 00063 00064 void init_work(void); 00065 }; 00066 00067 #endif
Generated on Thu Jul 14 2022 10:47:38 by
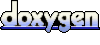