
Poker code for primary Mbed
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include <mbed.h> 00002 #include <string> 00003 #include <list> 00004 #include <mpr121.h> 00005 #include "NokiaLCD.h" 00006 00007 Serial pc(USBTX, USBRX); 00008 00009 Serial device(p13,p14); 00010 00011 // Setup the i2c bus on pins 28 and 27 00012 I2C i2c2(p9, p10); 00013 I2C i2c1(p28, p27); 00014 00015 // Setup the Mpr121: 00016 // constructor(i2c object, i2c address of the mpr121) 00017 Mpr121 mpr2(&i2c1, Mpr121::ADD_VSS); 00018 Mpr121 mpr1(&i2c2, Mpr121::ADD_VSS); 00019 00020 NokiaLCD lcd1(p5, p7, p8, p15, NokiaLCD::LCD6610); // mosi, sclk, cs, rst, type 00021 NokiaLCD lcd3(p5, p7, p16, p17, NokiaLCD::LCD6610); 00022 NokiaLCD lcd2(p5, p7, p18, p19, NokiaLCD::LCD6610); 00023 00024 00025 int z=0, cards[52][2]; 00026 00027 int secondary_players(int player_id, int Total, int face) 00028 { 00029 int temp_int, arr[7],i = 0, Amount = 0, temp_int1; 00030 char temp = '0',operation; 00031 00032 if(player_id == 2) device.putc('3'); 00033 if(player_id == 3) device.putc('4'); 00034 if(player_id == 0) device.putc('6'); 00035 00036 // temp= device.getc(); 00037 wait(0.5); 00038 if((Total > 0) && (face == 0)) { 00039 device.putc('M'); 00040 //temp=device.getc(); 00041 pc.printf("\ninside M"); 00042 temp_int = Total; 00043 //device.putc('0'); 00044 //device.putc('0'); 00045 for(int i=0; i<7; i++) { 00046 // wait(0.5); 00047 temp_int1 = temp_int% ((int)(pow(10, (float)1))); 00048 temp_int = temp_int/10; 00049 if(temp_int1 == 0) device.putc('0'); 00050 if(temp_int1 == 1) device.putc('1'); 00051 if(temp_int1 == 2) device.putc('2'); 00052 if(temp_int1 == 3) device.putc('3'); 00053 if(temp_int1 == 4) device.putc('4'); 00054 if(temp_int1 == 5) device.putc('5'); 00055 if(temp_int1 == 6) device.putc('6'); 00056 if(temp_int1 == 7) device.putc('7'); 00057 if(temp_int1 == 8) device.putc('8'); 00058 if(temp_int1 == 9) device.putc('9'); 00059 pc.printf("\namount:%d", temp_int1); 00060 //temp=device.getc(); 00061 } 00062 device.putc('D'); 00063 operation = device.getc(); 00064 wait(1); 00065 00066 for(int i=0; i<7; i++) arr[i] = 0; 00067 00068 //i = 0; 00069 00070 if(operation == 'C') return -2;//pc.printf("\nCALL"); 00071 if(operation == 'F') return 0;//pc.printf("\nFOLD"); 00072 if(operation == 'R') { 00073 //pc.printf("\nRAISE"); reading the bet amount from secondary mbed 00074 temp = device.getc(); 00075 while(temp != 'R') { 00076 arr[i] = temp; 00077 pc.printf("\narr[i]=%d",arr[i]); 00078 i++; 00079 temp = device.getc(); 00080 } 00081 for(int j=0; j<i; j++) { 00082 Amount = Amount + ((pow (10, (float) (j))) * arr[j]); 00083 } 00084 return Amount; 00085 //pc.printf("\nAmount = %d",Amount); 00086 } 00087 } 00088 00089 if(face != 0) { 00090 device.putc('I'); 00091 00092 if(Total == 1) { 00093 device.putc('S'); 00094 } else if(Total == 2) { 00095 device.putc('C'); 00096 } else if(Total == 3) { 00097 device.putc('H'); 00098 } else device.putc('D'); 00099 if(face==2) device.putc('2'); 00100 else if(face == 3) device.putc('3'); 00101 else if(face == 4) device.putc('4'); 00102 else if(face == 5) device.putc('5'); 00103 else if(face == 6) device.putc('6'); 00104 else if(face == 7) device.putc('7'); 00105 else if(face == 8) device.putc('8'); 00106 else if(face == 9) device.putc('9'); 00107 else if(face == 10) device.putc('0'); 00108 else if(face == 11) device.putc('J'); 00109 else if (face == 12) device.putc('Q'); 00110 else if (face == 13) device.putc('K'); 00111 else if (face == 1) device.putc('A'); 00112 00113 } 00114 00115 if((Total == 0) && (face == 0) && (player_id != 0)) { 00116 device.putc('Z'); 00117 } 00118 00119 } 00120 00121 void print_on_dealerlcd( int n, int card, int suit, int op) 00122 { 00123 00124 //lcd2.reset(); 00125 //lcd3.background(0x73fb76); 00126 //lcd3.cls(); 00127 //lcd3.locate(0,1); 00128 char x, y, w; 00129 int m; 00130 x=0; 00131 if(op == 1) { 00132 lcd3.locate(0,1); 00133 if(n==0)lcd3.printf("Common cards:"); 00134 if(n<3) { 00135 lcd3.locate(2+3*n,4+3*n); 00136 } else { 00137 m=n-3; 00138 lcd3.locate(6+3*m,4+3*m); 00139 } 00140 if((suit==1)||(suit==2))lcd3.foreground(0x000000); 00141 if((suit==3)||(suit==4))lcd3.foreground(0xFF0000); 00142 if(suit == 1) { 00143 y = 'S'; 00144 } else if(suit == 2) { 00145 y = 'C'; 00146 } else if(suit == 3) { 00147 y = 'H'; 00148 } else y = 'D'; 00149 if(card==2) x = '2'; 00150 else if(card == 3) x = '3'; 00151 else if(card == 4) x = '4'; 00152 else if(card == 5) x = '5'; 00153 else if(card == 6) x = '6'; 00154 else if(card == 7) x = '7'; 00155 else if(card == 8) x = '8'; 00156 else if(card == 9) x = '9'; 00157 else if(card == 10) { 00158 x = '1'; 00159 w = '0'; 00160 lcd3.printf(" %c%c",x,w); 00161 lcd3.printf(" %c", y); 00162 } else if(card == 11) x = 'J'; 00163 else if (card == 12) x = 'Q'; 00164 else if (card == 13) x = 'K'; 00165 else if (card == 1) x = 'A'; 00166 if(card!=10) { 00167 lcd3.printf(" %c",x); 00168 lcd3.printf(" %c", y); 00169 } 00170 00171 } else if(op == 2) { 00172 lcd3.locate(0,13); 00173 lcd3.foreground(0x000000); 00174 lcd3.printf("Current player:%d", n+1); 00175 } else if(op == 3) { 00176 lcd3.locate(0,15); 00177 lcd3.foreground(0x000000); 00178 lcd3.printf("Current Pot:%d", n); 00179 } else { 00180 lcd3.reset(); 00181 lcd3.foreground(0x000000); 00182 lcd3.background(0x2b8135); 00183 lcd3.fill(0, 0, 136, 136, 0x2b8135); 00184 lcd3.locate(0,0); 00185 } 00186 } 00187 00188 void print_on_lcd(int n, int suit, int card, int id) 00189 { 00190 char x,y,w; 00191 x = 0; 00192 y = 0; 00193 w = 0; 00194 switch(id) { 00195 case 0: 00196 // lcd1.reset(); 00197 lcd1.background(0x2b8135); 00198 lcd1.foreground(0x000000); 00199 // lcd1.cls(); 00200 lcd1.locate(0,3); 00201 if(n >= 0) { 00202 lcd1.printf("Raise to %d",n); 00203 } 00204 if(n == -1) { 00205 lcd1.printf("Fold"); 00206 } 00207 if(n == -2) { 00208 lcd1.printf("Call"); 00209 } 00210 if(n == -4) { 00211 lcd1.reset(); 00212 lcd1.cls(); 00213 lcd1.printf("Time up!"); 00214 } 00215 if (n==-3) { 00216 lcd1.printf("Exceeds limit, enter new value"); 00217 } 00218 00219 if(n == -6) { 00220 lcd1.locate(5,6); 00221 if(suit == 1) { 00222 y = 'S'; 00223 } else if(suit == 2) { 00224 y = 'C'; 00225 } else if(suit == 3) { 00226 y = 'H'; 00227 } else y = 'D'; 00228 if((y=='S')||(y=='C'))lcd1.foreground(0x000000); 00229 if((y=='H')||(y=='D'))lcd1.foreground(0xFF0000); 00230 if(card==2) x = '2'; 00231 else if(card == 3) x = '3'; 00232 else if(card == 4) x = '4'; 00233 else if(card == 5) x = '5'; 00234 else if(card == 6) x = '6'; 00235 else if(card == 7) x = '7'; 00236 else if(card == 8) x = '8'; 00237 else if(card == 9) x = '9'; 00238 else if(card == 10) { 00239 x = '1'; 00240 w = '0'; 00241 lcd1.printf("%c%c %c", x, w, y); 00242 } else if(card == 11) x = 'J'; 00243 else if (card == 12) x = 'Q'; 00244 else if (card == 13) x = 'K'; 00245 else if (card == 1) x = 'A'; 00246 if(card!=10)lcd1.printf(" %c %c",x, y); 00247 } 00248 if(n == -5) { 00249 lcd1.locate(5,8); 00250 if(suit == 1) { 00251 y = 'S'; 00252 } else if(suit == 2) { 00253 y = 'C'; 00254 } else if(suit == 3) { 00255 y = 'H'; 00256 } else y = 'D'; 00257 if((y=='S')||(y=='C'))lcd1.foreground(0x000000); 00258 if((y=='H')||(y=='D'))lcd1.foreground(0xFF0000); 00259 if(card==2) x = '2'; 00260 else if(card == 3) x = '3'; 00261 else if(card == 4) x = '4'; 00262 else if(card == 5) x = '5'; 00263 else if(card == 6) x = '6'; 00264 else if(card == 7) x = '7'; 00265 else if(card == 8) x = '8'; 00266 else if(card == 9) x = '9'; 00267 else if(card == 10) { 00268 x = '1'; 00269 w = '0'; 00270 lcd1.printf("%c%c %c", x, w, y); 00271 } else if(card == 11) x = 'J'; 00272 else if (card == 12) x = 'Q'; 00273 else if (card == 13) x = 'K'; 00274 else if (card == 1) x = 'A'; 00275 if(card!=10)lcd1.printf(" %c %c", x, y); 00276 } 00277 break; 00278 case -9: 00279 if(suit==0) { 00280 lcd1.foreground(0x000000); 00281 lcd1.locate(0, 1); 00282 lcd1.printf("Current wallet:"); 00283 lcd1.locate(6,3); 00284 lcd1.printf("%d",n); 00285 } else if(suit==1) { 00286 lcd2.foreground(0x000000); 00287 lcd2.locate(0, 1); 00288 lcd2.printf("Current wallet:"); 00289 lcd2.locate(6,3); 00290 lcd2.printf("%d",n); 00291 } 00292 break; 00293 case -8://(bet-Playerbet[ID],ID, 0, -8) 00294 /*if(suit==0) { 00295 lcd1.foreground(0x000000); 00296 lcd1.locate(4, 10); 00297 if(card==0)lcd1.printf("To Call: "); 00298 if(card==1)lcd1.printf("To Raise:"); 00299 lcd1.locate(6,12); 00300 lcd1.printf("%d",n); 00301 } else if(suit==1) { 00302 lcd2.foreground(0x000000); 00303 lcd2.locate(4, 10); 00304 if(card==0)lcd2.printf("To Call:"); 00305 if(card==1)lcd2.printf("To Raise:"); 00306 lcd2.locate(6,12); 00307 lcd2.printf("%d",n); 00308 }*/ 00309 break; 00310 case 1: 00311 //lcd2.reset(); 00312 lcd2.background(0x2b8135); 00313 lcd2.foreground(0x000000); 00314 //lcd2.cls(); 00315 lcd2.locate(0,3); 00316 if(n >= 0) { 00317 lcd2.printf("Raise to %d",n); 00318 } 00319 if(n == -1) { 00320 lcd2.printf("Fold"); 00321 } 00322 if(n == -2) { 00323 lcd2.printf("Call"); 00324 } 00325 if(n == -4) { 00326 lcd2.reset(); 00327 lcd2.cls(); 00328 lcd2.printf("Time up!"); 00329 } 00330 if (n == -3) { 00331 lcd2.printf("Exceeds limit, enter new value"); 00332 } 00333 00334 if(n != -6) { 00335 lcd2.locate(5,6); 00336 if(suit == 1) { 00337 y = 'S'; 00338 } else if(suit == 2) { 00339 y = 'C'; 00340 } else if(suit == 3) { 00341 y = 'H'; 00342 } else y = 'D'; 00343 if((y=='S')||(y=='C'))lcd2.foreground(0x000000); 00344 if((y=='H')||(y=='D'))lcd2.foreground(0xFF0000); 00345 if(card==2) x = '2'; 00346 else if(card == 3) x = '3'; 00347 else if(card == 4) x = '4'; 00348 else if(card == 5) x = '5'; 00349 else if(card == 6) x = '6'; 00350 else if(card == 7) x = '7'; 00351 else if(card == 8) x = '8'; 00352 else if(card == 9) x = '9'; 00353 else if(card == 10) { 00354 x = '1'; 00355 w = '0'; 00356 lcd2.printf("%c%c %c", x, w, y); 00357 } else if(card == 11) x = 'J'; 00358 else if (card == 12) x = 'Q'; 00359 else if (card == 13) x = 'K'; 00360 else if (card == 1) x = 'A'; 00361 if(card!=10)lcd2.printf(" %c %c", x, y); 00362 } 00363 00364 if(n != -5) { 00365 lcd2.locate(5,8); 00366 if(suit == 1) { 00367 y = 'S'; 00368 } else if(suit == 2) { 00369 y = 'C'; 00370 } else if(suit == 3) { 00371 y = 'H'; 00372 } else y = 'D'; 00373 if((y=='S')||(y=='C'))lcd2.foreground(0x000000); 00374 if((y=='H')||(y=='D'))lcd2.foreground(0xFF0000); 00375 if(card==2) x = '2'; 00376 else if(card == 3) x = '3'; 00377 else if(card == 4) x = '4'; 00378 else if(card == 5) x = '5'; 00379 else if(card == 6) x = '6'; 00380 else if(card == 7) x = '7'; 00381 else if(card == 8) x = '8'; 00382 else if(card == 9) x = '9'; 00383 else if(card == 10) { 00384 x = '1'; 00385 w = '0'; 00386 lcd2.printf("%c %c%c", x, w, y); 00387 } else if(card == 11) x = 'J'; 00388 else if (card == 12) x = 'Q'; 00389 else if (card == 13) x = 'K'; 00390 else if (card == 1) x = 'A'; 00391 if(card!=10)lcd2.printf(" %c %c", x, y); 00392 } 00393 break; 00394 case 2: 00395 lcd1.cls(); 00396 break; 00397 case 3: 00398 lcd2.cls(); 00399 break; 00400 default: 00401 lcd2.cls(); 00402 lcd1.cls(); 00403 break; 00404 00405 } 00406 wait(rand()%1); 00407 } 00408 00409 int read_keypad(int n) 00410 { 00411 00412 pc.printf("\nHello from the mbed & mpr121\n\r"); 00413 00414 Timer t; 00415 //float s; 00416 //int turn; 00417 unsigned char dataArray[2]; 00418 unsigned int Amount[6]; 00419 int max = 6; 00420 unsigned int Total = 0; 00421 int key = 0; 00422 int count = 0; 00423 //unsigned int card[2] = {3,12}; 00424 //unsigned char suit[2] = {'S','H'}; 00425 00426 t.reset(); 00427 t.start(); 00428 00429 while(1) { 00430 //pc.printf("\n Entered value is:"); 00431 if(t.read() == 60.0) { 00432 //print_on_lcd(-4, 0, 0, n); 00433 t.stop(); 00434 return 0; 00435 } 00436 if((n == 0)||(n == 1)) { 00437 if(n == 0) { 00438 dataArray[0] = mpr1.read(0x00); 00439 dataArray[1] = mpr1.read(0x01); 00440 } else if(n == 1) { 00441 dataArray[0] = mpr2.read(0x00); 00442 dataArray[1] = mpr2.read(0x01); 00443 } 00444 if(dataArray[0] != 0) { 00445 switch(dataArray[0]) { 00446 case 8: 00447 if(count == max) { 00448 pc.printf("Exceeds limit, enter new value \r\n"); 00449 //print_on_lcd(-3, 0, 0, n); 00450 for(int i=0; i<6; i++) { 00451 Amount[i] = 0; 00452 } 00453 count = 0; 00454 break; 00455 } 00456 pc.printf("MPR value: %d \r\n", 1); 00457 //print_on_lcd(1); 00458 Amount[count] = 1; 00459 count++; 00460 break; 00461 case 128: 00462 if(count == max) { 00463 pc.printf("Exceeds limit, enter new value \r\n"); 00464 //print_on_lcd(-3, 0, 0, n); 00465 for(int i=0; i<6; i++) { 00466 Amount[i] = 0; 00467 } 00468 count = 0; 00469 break; 00470 } 00471 pc.printf("MPR value: %d \r\n", 2); 00472 // print_on_lcd(2); 00473 Amount[count] = 2; 00474 count++; 00475 break; 00476 case 4: 00477 if(count == max) { 00478 pc.printf("Exceeds limit, enter new value \r\n"); 00479 //print_on_lcd(-3, 0, 0, n); 00480 for(int i=0; i<6; i++) { 00481 Amount[i] = 0; 00482 } 00483 count = 0; 00484 break; 00485 } 00486 pc.printf("MPR value: %d \r\n", 4); 00487 // print_on_lcd(4); 00488 Amount[count] = 4; 00489 count++; 00490 break; 00491 case 64: 00492 if(count == max) { 00493 pc.printf("Exceeds limit, enter new value \r\n"); 00494 //print_on_lcd(-3, 0, 0, n); 00495 for(int i=0; i<6; i++) { 00496 Amount[i] = 0; 00497 } 00498 count = 0; 00499 break; 00500 } 00501 pc.printf("MPR value: %d \r\n", 5); 00502 Amount[count] = 5; 00503 // print_on_lcd(5); 00504 count++; 00505 break; 00506 case 2: 00507 if(count == max) { 00508 pc.printf("Exceeds limit, enter new value \r\n"); 00509 //print_on_lcd(-3, 0, 0, n); 00510 for(int i=0; i<6; i++) { 00511 Amount[i] = 0; 00512 } 00513 count = 0; 00514 break; 00515 } 00516 pc.printf("MPR value: %d \r\n", 7); 00517 Amount[count] = 7;//print_on_lcd(7); 00518 count++; 00519 break; 00520 case 32: 00521 if(count == max) { 00522 pc.printf("Exceeds limit, enter new value \r\n"); 00523 //print_on_lcd(-3, 0, 0, n); 00524 for(int i=0; i<6; i++) { 00525 Amount[i] = 0; 00526 } 00527 count = 0; 00528 break; 00529 } 00530 pc.printf("MPR value: %d \r\n", 8);//print_on_lcd(8); 00531 Amount[count] = 8; 00532 count++; 00533 break; 00534 case 1: 00535 00536 if(count != 0) { 00537 while( count != 0) { 00538 Total += Amount[key]*(pow( 10, (float) (count-1) )); 00539 count--; 00540 key++; 00541 } 00542 pc.printf("Total is: %d \r\n",Total); 00543 //print_on_lcd(Total, 0, 0, n); 00544 for(int i=0; i<6; i++) { 00545 Amount[i] = 0; 00546 } 00547 t.stop(); 00548 return Total; 00549 } else { 00550 pc.printf("MPR value: %s \r\n", "Call"); 00551 //print_on_lcd(-2, 0, 0, n); 00552 t.stop(); 00553 return -2; 00554 } 00555 case 16: 00556 if(count == max) { 00557 pc.printf("Exceeds limit, enter new value \r\n"); 00558 //print_on_lcd(-3, 0, 0, n); 00559 for(int i=0; i<6; i++) { 00560 Amount[i] = 0; 00561 } 00562 count = 0; 00563 break; 00564 } 00565 pc.printf("MPR value: %d \r\n", 0); 00566 Amount[count] = 0; 00567 count++; 00568 break; 00569 } 00570 wait(0.5); 00571 dataArray[0] = 0; 00572 } else { 00573 if(dataArray[1] != 0) { 00574 switch(dataArray[1]) { 00575 case 8: 00576 if(count == max) { 00577 pc.printf("Exceeds limit, enter new value \r\n"); 00578 //print_on_lcd(-3, 0, 0, n); 00579 for(int i=0; i<6; i++) { 00580 Amount[i] = 0; 00581 } 00582 count = 0; 00583 break; 00584 } 00585 pc.printf("MPR value: %d \r\n", 3); 00586 Amount[count] = 3; 00587 count++; 00588 break; 00589 case 4: 00590 if(count == max) { 00591 pc.printf("Exceeds limit, enter new value \r\n"); 00592 //print_on_lcd(-3, 0, 0, n); 00593 for(int i=0; i<6; i++) { 00594 Amount[i] = 0; 00595 } 00596 count = 0; 00597 break; 00598 } 00599 pc.printf("MPR value: %d \r\n", 6); 00600 Amount[count] = 6; 00601 count++; 00602 break; 00603 case 2: 00604 if(count == max) { 00605 pc.printf("Exceeds limit, enter new value \r\n"); 00606 //print_on_lcd(-3, 0, 0, n); 00607 for(int i=0; i<6; i++) { 00608 Amount[i] = 0; 00609 } 00610 count = 0; 00611 break; 00612 } 00613 pc.printf("MPR value: %d \r\n", 9); 00614 Amount[count] = 9; 00615 count++; 00616 break; 00617 case 1: 00618 if(count != 0) { 00619 while( count != 0) { 00620 Total += Amount[key]*(pow( 10, (float) (count-1) )); 00621 count--; 00622 key++; 00623 } 00624 pc.printf("Total is: %d \r\n",Total); 00625 //print_on_lcd(Total, 0, 0, n); 00626 for(int i=0; i<6; i++) { 00627 Amount[i] = 0; 00628 } 00629 t.stop(); 00630 return Total; 00631 } else { 00632 pc.printf("MPR value: %s \r\n", "Fold"); 00633 //print_on_lcd(-1, 0, 0, n); 00634 for(int i=0; i<6; i++) { 00635 Amount[i] = 0; 00636 } 00637 count = 0; 00638 t.stop(); 00639 return 0; 00640 } 00641 } 00642 wait(0.5); 00643 dataArray[1] = 0; 00644 } 00645 } 00646 } else { 00647 } 00648 } 00649 } 00650 00651 int checkcard ( int suit, int face, int reset) 00652 { 00653 int i; 00654 00655 switch(z) { 00656 case 0: 00657 cards[0][0]=suit; 00658 cards[0][1]=face; 00659 z++; 00660 break; 00661 default: 00662 for(i=0; i<z; i++) { 00663 if((cards[i][0]==suit)&&(cards[i][1]==face)) { 00664 return 1; 00665 } 00666 } 00667 cards[z][0]=suit; 00668 cards[z][1]=face; 00669 z++; 00670 break; 00671 } 00672 if(reset==1) { 00673 z=0; 00674 } 00675 return 0; 00676 } 00677 00678 //Main Poker Program 00679 //Main Poker Program 00680 int main() 00681 { 00682 //initialize variables 00683 int pot=0, bet, bet2, round, check, temp, hand, suit, ID=0, blind=-1; 00684 int i, k, j, p=0, r, n, t; 00685 00686 //seed random number generator 00687 00688 srand(time(NULL)); 00689 00690 //ask how many players 00691 print_on_dealerlcd( 0, 0, 0, 4); 00692 lcd1.reset(); 00693 lcd1.foreground(0x000000); 00694 lcd1.background(0x2b8135); 00695 lcd1.fill(0, 0, 136, 136, 0x2b8135); 00696 lcd1.locate(0,1); 00697 00698 lcd2.reset(); 00699 lcd2.foreground(0x000000); 00700 lcd2.background(0x2b8135); 00701 lcd2.fill(0, 0, 136, 136, 0x2b8135); 00702 lcd2.locate(0,1); 00703 00704 while(p<2) { 00705 lcd1.printf("How many players?"); 00706 pc.printf("\nAmount of players?"); 00707 p=read_keypad(0); 00708 //p=2; 00709 if(p<2)pc.printf("\nnot enough players..."); 00710 00711 } 00712 int playermoney[p]; 00713 00714 //ask how much each player should start out with 00715 //while((pot<100)||(pot>1000000/p)) { 00716 lcd1.cls(); 00717 lcd1.locate(0,1); 00718 lcd1.printf("Money each player starts with?"); 00719 pc.printf("Money each player starts with?\n"); 00720 pc.printf("\nbetween $100-$%d",1000000/p); 00721 pot=read_keypad(0); 00722 //pot=2000; 00723 //} 00724 for(k=0; k<p; k++) { 00725 playermoney[k]=pot; 00726 pc.printf("player %d\n", k+1); 00727 } 00728 00729 //ask size of blind 00730 while((blind<0)||(blind>pot/16)) { 00731 lcd1.cls(); 00732 lcd1.locate(0,1); 00733 lcd1.printf("Size of large"); 00734 lcd1.locate(0,3); 00735 lcd1.printf("blind?"); 00736 pc.printf("\nSize of large blind?"); 00737 lcd1.locate(0,5); 00738 lcd1.printf("Between $0-$%d",pot/16); 00739 blind=read_keypad(0); 00740 //blind=20; 00741 } 00742 lcd1.cls(); 00743 00744 pot=0; 00745 00746 //determine hands played to see who should be dealer 00747 for(hand=0; p>1; hand++) { 00748 int playerbet[p], playersuit[p][2], playerface[p][2], communitys[5], highcard[p], playerscore[p], AB[p], AP; 00749 int communityf[5], handface[7], handsuit[7], spade, heart, club, diamond, pot=0, count[14], m=0; 00750 i=checkcard(0,0,1); 00751 pc.printf("\n hand %d",hand); 00752 for(k=0; k<p; k++) { 00753 AB[k]=0; 00754 } 00755 AP=p; 00756 t=secondary_players(0, 0, 0); 00757 print_on_lcd(0, 0, 0, -10); 00758 00759 00760 for(round=1; round<5; round++) { 00761 for(k=0; k<p; k++) { 00762 playerbet[k]=0; 00763 } 00764 if(AP>1) { 00765 switch(round) { 00766 //first round lay players cards 00767 case 1 : 00768 print_on_dealerlcd( 0, 0, 0, 4); 00769 for (r=0; r<p; r++) { 00770 for (k=0; k<2; k++) { 00771 playersuit[r][k]= (rand()%4)+1; 00772 playerface[r][k]= (rand()%13)+1; 00773 check = checkcard( playersuit[r][k], playerface[r][k], 0); 00774 pc.printf("\nCheck 1= %d",check); 00775 if(check==1) { 00776 k--; 00777 } else { 00778 if(r<2) { 00779 print_on_lcd(k-6, playersuit[r][k], playerface[r][k], r); 00780 } else { 00781 t=secondary_players(r, playersuit[r][k], playerface[r][k]); 00782 } 00783 pc.printf("\n operation:%d\t suit:%d\t face:%d\t player%d", k-6, playersuit[r][k], playerface[r][k], r+1); 00784 } 00785 } 00786 } 00787 break; 00788 00789 //second round lay 3 cards on table 00790 case 2 : 00791 for(k=0; k<3; k++) { 00792 communitys[k]=(rand()%4)+1; 00793 communityf[k]=(rand()%13)+1; 00794 pc.printf("\n Amount:%d\t card:%d\t face:%d\t suit:%d\t Operation:%d ", 0, k, communityf[k], communitys[k], 1); 00795 //function to make sure no cards are the same 00796 check = checkcard ( communitys[k], communityf[k], 0); 00797 pc.printf("\nCheck 2= %d",check); 00798 if(check==1) { 00799 k--; 00800 } else { 00801 print_on_dealerlcd( k, communityf[k], communitys[k], 1); 00802 pc.printf("\n Amount:%d\t card:%d\t face:%d\t suit:%d\t Operation:%d ", 0, k, communityf[k], communitys[k], 1); 00803 wait(rand()%1); 00804 } 00805 } 00806 break; 00807 00808 //third round lay single card on table 00809 case 3 : 00810 for(k=0; k<1; k++) { 00811 communitys[3]=(rand()%4)+1; 00812 communityf[3]=(rand()%13)+1; 00813 check = checkcard ( communitys[3], communityf[3], 0); 00814 pc.printf("\n Amount:%d\t card:%d\t face:%d\t suit:%d\t Operation:%d ", 0, k, communityf[3], communitys[3], 1); 00815 pc.printf("\nCheck 3= %d",check); 00816 if(check==1) { 00817 k--; 00818 } else { 00819 print_on_dealerlcd( 3, communityf[3], communitys[3], 1); 00820 pc.printf("\n Amount:%d\t card:%d\t face:%d\t suit:%d\t Operation:%d ", 0, 3, communityf[3], communitys[3], 1); 00821 wait(rand()%1); 00822 } 00823 } 00824 break; 00825 00826 //fourth round lay single card on table 00827 case 4 : 00828 for(k=0; k<1; k++) { 00829 communitys[4]=(rand()%4)+1; 00830 communityf[4]=(rand()%13)+1; 00831 check = checkcard ( communitys[4], communityf[4], 0); 00832 if(check==1) { 00833 k--; 00834 pc.printf("\nCheck 4= %d",check); 00835 } else { 00836 print_on_dealerlcd( 4, communityf[4], communitys[4], 1); 00837 wait(rand()%1); 00838 pc.printf("\n Amount:%d\t card:%d\t face:%d \t suit:%d\t Operation:%d ", 0, 4, communityf[4], communitys[4], 1); 00839 } 00840 } 00841 break; 00842 } 00843 00844 //take bets from each player 00845 if(round!=1) bet=0; 00846 if(round==1) bet=blind; 00847 temp = hand%p; 00848 pc.printf("\ntemp:%d",temp); 00849 n=0; 00850 r=0; 00851 bet2=0; 00852 ID = temp; 00853 while(r<p) { 00854 while(ID <= (p-1) ) { 00855 if(AP>1) { 00856 if((m==1)&&(blind!=0)) { 00857 print_on_lcd(playermoney[ID]-blind/2, ID, 0, -9); 00858 } else { 00859 print_on_lcd(playermoney[ID], ID, 0, -9); 00860 } 00861 print_on_dealerlcd( ID, 0, 0, 2); 00862 pc.printf("\nr=%d",r); 00863 pc.printf("\nstart while"); 00864 if(AB[ID]!=1) { 00865 //ID = temp; 00866 //for(ID=hand%p; ID<p; ID++) { 00867 pc.printf("\nin while"); 00868 if((round==1)||(playerbet[ID]!=0)) { 00869 if((round==1)&&(m==0)&&(blind!=0))playerbet[ID]=bet; 00870 if((round==1)&&(m==1)&&(blind!=0))playerbet[ID]=bet/2; 00871 } 00872 if(playerbet[ID]==-2)playerbet[ID]=0; 00873 pc.printf("\npot:%d player%dmoney:%d",pot, ID+1, playermoney[ID]); 00874 pc.printf("\nplayer%d bet?", ID+1); 00875 if(ID>1) { 00876 if((m==0)&&(blind!=0)) { 00877 n=-2; 00878 } else { 00879 n = secondary_players(ID, playermoney[ID],0); 00880 } 00881 } else { 00882 if((m==0)&&(blind!=0)) { 00883 n=-2; 00884 } else { 00885 print_on_lcd(bet-playerbet[ID], ID, 0, -8); 00886 n=read_keypad(ID); 00887 } 00888 } 00889 pc.printf("\nbet:%d player%dbet:%d",bet, ID+1,playerbet[ID]); 00890 switch(n) { 00891 case -2: 00892 if(bet > 0) { 00893 if((blind!=0)&&(m==0)) { 00894 if(playermoney[ID]>playerbet[ID]) { 00895 pot+=playerbet[ID]; 00896 playermoney[ID]-=playerbet[ID]; 00897 print_on_dealerlcd( pot, 0, 0, 3); 00898 r--; 00899 } 00900 } else if((blind!=0)&&(m==1)) { 00901 if(playermoney[ID]>blind) { 00902 pot+=blind; 00903 playermoney[ID]-=blind; 00904 print_on_dealerlcd( pot, 0, 0, 3); 00905 } 00906 } else if(playerbet[ID]==0) { 00907 if(bet<=playermoney[ID]) { 00908 playerbet[ID] = bet; 00909 pot += playerbet[ID]; 00910 playermoney[ID] -= bet; 00911 print_on_dealerlcd( pot, 0, 0, 3); 00912 } else { 00913 playerbet[ID]=0; 00914 AB[ID]=1; 00915 AP--; 00916 if(ID<2) { 00917 print_on_lcd(0, 0, 0, ID+2); 00918 } else { 00919 t=secondary_players(ID, 0, 0); 00920 } 00921 } 00922 } else if(playerbet[ID]!=0) { 00923 //if(m==1)playerbet[ID]=bet; 00924 if(((bet-playerbet[ID])<=playermoney[ID])&&(playerbet[ID]<=bet)) { 00925 bet2 = bet-playerbet[ID]; 00926 pot += bet2 ; 00927 playerbet[ID] = bet; 00928 playermoney[ID] -= bet2; 00929 pc.printf("\ntest"); 00930 print_on_dealerlcd( pot, 0, 0, 3); 00931 } else { 00932 playerbet[ID]=0; 00933 AB[ID]=1; 00934 AP--; 00935 if(ID<2) { 00936 print_on_lcd(0, 0, 0, ID+2); 00937 } else { 00938 t=secondary_players(ID, 0, 0); 00939 } 00940 } 00941 } 00942 } 00943 if(bet ==0)playerbet[ID]=-2; 00944 break; 00945 case 0: 00946 if(bet==0) { 00947 playerbet[ID]=-2; 00948 pc.printf("\n#01 bet:%d player%dbet:%d",bet, ID+1,playerbet[ID]); 00949 break; 00950 } 00951 playerbet[ID]=0; 00952 if((m==1)&&(blind!=0)) { 00953 playermoney[ID] -= bet/2; 00954 pot += bet/2; 00955 print_on_dealerlcd( pot, 0, 0, 3); 00956 } 00957 AB[ID]=1; 00958 AP--; 00959 if(ID<2) { 00960 print_on_lcd(0, 0, 0, ID+2); 00961 } else { 00962 t=secondary_players(ID, 0, 0); 00963 } 00964 pc.printf("\n#02 bet:%d player%dbet:%d",bet, ID+1,playerbet[ID]); 00965 break; 00966 default: 00967 if(playerbet[ID]==0) { 00968 playerbet[ID]=n; 00969 if((playerbet[ID]<=playermoney[ID])&&(playerbet[ID]>=bet)) { 00970 //function to ask for bets and check bet amount 00971 playermoney[ID] -= playerbet[ID]; 00972 pot += playerbet[ID]; 00973 bet = playerbet[ID]; 00974 print_on_dealerlcd( pot, 0, 0, 3); 00975 r=0; 00976 } else { 00977 pc.printf("\nplayer bet Incorrect!"); 00978 ID--; 00979 r--; 00980 } 00981 pc.printf("\ndef bet:%d player%dbet:%d",bet, ID+1,playerbet[ID]); 00982 } else if(playerbet[ID]!=0) { 00983 pc.printf("\ndef1 bet:%d player%dbet:%d pot:%d",bet, ID+1,playerbet[ID], pot); 00984 if((n<=playermoney[ID])&&(n>=bet-playerbet[ID])) { 00985 pot += n; 00986 playerbet[ID] += n; 00987 bet = playerbet[ID]; 00988 playermoney[ID] -= n; 00989 r=0; 00990 print_on_dealerlcd( pot, 0, 0, 3); 00991 } else { 00992 pc.printf("\nplayer bet Incorrect!"); 00993 ID--; 00994 r--; 00995 } 00996 } 00997 } 00998 00999 01000 01001 } 01002 } 01003 print_on_lcd(bet-playerbet[ID], ID, 0, -8); 01004 if(ID<2)print_on_lcd(playermoney[ID], ID, 0, -9); 01005 r++; 01006 ID++; 01007 m++; 01008 pc.printf("\nincrement"); 01009 if(r>=p)break; 01010 } 01011 01012 for(ID=0; ID<temp; ID++) { 01013 if(AP>1) { 01014 if((m=1)&&(blind!=0)) { 01015 print_on_lcd(playermoney[ID]-blind/2, ID, 0, -9); 01016 } else { 01017 print_on_lcd(playermoney[ID], ID, 0, -9); 01018 } 01019 print_on_dealerlcd( ID, 0, 0, 2); 01020 pc.printf("\nr=%d",r); 01021 pc.printf("\nstart for"); 01022 if(AB[ID]!=1) { 01023 //ID = temp; 01024 //for(ID=hand%p; ID<p; ID++) { 01025 pc.printf("\nin for"); 01026 if((round==1)||(playerbet[ID]!=0)) { 01027 if((round==1)&&(m==0)&&(blind!=0))playerbet[ID]=bet; 01028 if((round==1)&&(m==1)&&(blind!=0))playerbet[ID]=bet/2; 01029 } 01030 if(playerbet[ID]==-2)playerbet[ID]=0; 01031 pc.printf("\npot:%d player%dmoney:%d",pot, ID+1, playermoney[ID]); 01032 pc.printf("\nplayer%d bet?", ID+1); 01033 if(ID>1) { 01034 if((m==0)&&(blind!=0)) { 01035 n=-2; 01036 } else { 01037 n = secondary_players(ID, playermoney[ID],0); 01038 } 01039 } else { 01040 if((m==0)&&(blind!=0)) { 01041 n=-2; 01042 } else { 01043 print_on_lcd(bet-playerbet[ID], ID, 0, -8); 01044 n=read_keypad(ID); 01045 } 01046 } 01047 pc.printf("\nbet:%d player%dbet:%d",bet, ID+1,playerbet[ID]); 01048 switch(n) { 01049 case -2: 01050 if(bet > 0) { 01051 if((blind!=0)&&(m==0)) { 01052 if(playermoney[ID]>playerbet[ID]) { 01053 pot+=playerbet[ID]; 01054 playermoney[ID]-=playerbet[ID]; 01055 print_on_dealerlcd( pot, 0, 0, 3); 01056 r--; 01057 } 01058 } else if((blind!=0)&&(m==1)) { 01059 if(playermoney[ID]>blind) { 01060 pot+=blind; 01061 playermoney[ID]-=blind; 01062 print_on_dealerlcd( pot, 0, 0, 3); 01063 } 01064 } else if(playerbet[ID]==0) { 01065 if(bet<=playermoney[ID]) { 01066 playerbet[ID] = bet; 01067 pot += playerbet[ID]; 01068 playermoney[ID] -= bet; 01069 print_on_dealerlcd( pot, 0, 0, 3); 01070 } else { 01071 playerbet[ID]=0; 01072 AB[ID]=1; 01073 AP--; 01074 if(ID<2) { 01075 print_on_lcd(0, 0, 0, ID+2); 01076 } else { 01077 t=secondary_players(ID, 0, 0); 01078 } 01079 } 01080 } else if(playerbet[ID]!=0) { 01081 //if(m==1)playerbet[ID]=bet; 01082 if(((bet-playerbet[ID])<=playermoney[ID])&&(playerbet[ID]<=bet)) { 01083 bet2 = bet-playerbet[ID]; 01084 pot += bet2 ; 01085 playerbet[ID] = bet; 01086 playermoney[ID] -= bet2; 01087 pc.printf("\ntest"); 01088 print_on_dealerlcd( pot, 0, 0, 3); 01089 } else { 01090 playerbet[ID]=0; 01091 AB[ID]=1; 01092 AP--; 01093 if(ID<2) { 01094 print_on_lcd(0, 0, 0, ID+2); 01095 } else { 01096 t=secondary_players(ID, 0, 0); 01097 } 01098 } 01099 } 01100 } 01101 if(bet ==0)playerbet[ID]=-2; 01102 break; 01103 case 0: 01104 if(bet==0) { 01105 playerbet[ID]=-2; 01106 pc.printf("\n#01 bet:%d player%dbet:%d",bet, ID+1,playerbet[ID]); 01107 break; 01108 } 01109 playerbet[ID]=0; 01110 if((m==1)&&(blind!=0)) { 01111 playermoney[ID] -= bet/2; 01112 pot += bet/2; 01113 print_on_dealerlcd( pot, 0, 0, 3); 01114 } 01115 AB[ID]=1; 01116 AP--; 01117 if(ID<2) { 01118 print_on_lcd(0, 0, 0, ID+2); 01119 } else { 01120 t=secondary_players(ID, 0, 0); 01121 } 01122 pc.printf("\n#02 bet:%d player%dbet:%d",bet, ID+1,playerbet[ID]); 01123 break; 01124 default: 01125 if(playerbet[ID]==0) { 01126 playerbet[ID]=n; 01127 if((playerbet[ID]<=playermoney[ID])&&(playerbet[ID]>=bet)) { 01128 //function to ask for bets and check bet amount 01129 playermoney[ID] -= playerbet[ID]; 01130 pot += playerbet[ID]; 01131 bet = playerbet[ID]; 01132 print_on_dealerlcd( pot, 0, 0, 3); 01133 r=0; 01134 } else { 01135 pc.printf("\nplayer bet Incorrect!"); 01136 ID--; 01137 r--; 01138 } 01139 pc.printf("\ndef bet:%d player%dbet:%d",bet, ID+1,playerbet[ID]); 01140 } else if(playerbet[ID]!=0) { 01141 pc.printf("\ndef1 bet:%d player%dbet:%d pot:%d",bet, ID+1,playerbet[ID], pot); 01142 if((n<=playermoney[ID])&&(n>=bet-playerbet[ID])) { 01143 pot += n; 01144 playerbet[ID] += n; 01145 bet = playerbet[ID]; 01146 playermoney[ID] -= n; 01147 r=0; 01148 print_on_dealerlcd( pot, 0, 0, 3); 01149 } else { 01150 pc.printf("\nplayer bet Incorrect!"); 01151 ID--; 01152 r--; 01153 } 01154 } 01155 } 01156 01157 01158 01159 } 01160 } 01161 print_on_lcd(bet-playerbet[ID], ID, 0, -8); 01162 if(ID<2)print_on_lcd(playermoney[ID], ID, 0, -9); 01163 r++; 01164 //ID++; 01165 m++; 01166 pc.printf("\nincrement"); 01167 if(r>=p)break; 01168 } 01169 } 01170 01171 } 01172 } 01173 01174 //determine player's score 01175 if(AP==1) { 01176 for(k=0; k<p; k++) { 01177 if(AB[k]==1)playerscore[k]=0; 01178 if(AB[k]!=1)playerscore[k]=10; 01179 } 01180 } 01181 if(AP>1) { 01182 for (k=0; k<p; k++) { 01183 spade=0; 01184 heart=0; 01185 diamond=0; 01186 club=0; 01187 suit=0; 01188 for(i=0; i<14; i++) { 01189 count[i]=0; 01190 } 01191 playerscore[k]=0; 01192 if(playerbet[k]==0) { 01193 playerscore[k]=0; 01194 } else { 01195 for (j=0; j<5; j++) { 01196 handface[j] = communityf[j]; 01197 handsuit[j] = communitys[j]; 01198 } 01199 handface[5] = playerface[k][0]; 01200 handface[6] = playerface[k][1]; 01201 handsuit[5] = playersuit[k][0]; 01202 handsuit[6] = playersuit[k][1]; 01203 if(playerface[k][0]>=playerface[k][1]) { 01204 highcard[k]=playerface[k][0]; 01205 } else { 01206 highcard[k]=playerface[k][1]; 01207 } 01208 for(j=0; j<7; j++) { 01209 pc.printf("\nplayer%d face:%d", k+1, handface[j]); 01210 } 01211 pc.printf("\nplayer%d highcard:%d",k+1, highcard[k]); 01212 01213 //player score ranking 01214 //Straight Flush = 9 01215 //Four of a kind = 8 01216 //Full House = 7 01217 //Flush = 6 01218 //Straight = 5 01219 //Threee of a kind = 4 01220 //Two pair = 3 01221 //One Pair = 2 01222 //High Card = 1 01223 //determine how many cards of each suit and face value there are 01224 for(i=0; i<7; i++) { 01225 if(handsuit[i] == 1)spade++; 01226 if(handsuit[i] == 2)club++; 01227 if(handsuit[i] == 3)heart++; 01228 if(handsuit[i] == 4)diamond++; 01229 } 01230 for(r=0; r<7; r++) { 01231 for(j=0; j<13; j++) { 01232 if(handface[r]==j+1)count[j]++; 01233 } 01234 } 01235 for(j=0; j<14; j++) { 01236 pc.printf("\nplayer%d r=%d count=%d",k+1,j,count[j]); 01237 } 01238 count[13]=count[0]; 01239 01240 //determine if four of a kind 01241 for(r=0; r<13; r++) { 01242 if(count[r]==4) playerscore[k] = 8; 01243 } 01244 pc.printf("\nfour of a kind:%d", playerscore[k]); 01245 //determine if Full House 01246 if(playerscore[k]==0) { 01247 for(i=0; i<7; i++) { 01248 if(count[i] == 3) { 01249 for(j=0; j<7; j++) { 01250 if(count[j] >= 2) { 01251 playerscore[k]=7; 01252 } 01253 } 01254 } 01255 } 01256 } 01257 //Determine if flush 01258 if((spade >= 5)&&((playersuit[k][1]== 1)||(playersuit[k][0] == 1))) { 01259 playerscore[k] = 6; 01260 suit = 1; 01261 } 01262 if((club >= 5)&&((playersuit[k][1]== 2)||(playersuit[k][0]==2))) { 01263 playerscore[k] = 6; 01264 suit = 2; 01265 } 01266 if((heart >= 5)&&((playersuit[k][1]== 3)||(playersuit[k][0]==3))) { 01267 playerscore[k] = 6; 01268 suit = 3; 01269 } 01270 if((diamond >= 5)&&((playersuit[k][1]== 2)||(playersuit[k][0]==2))) { 01271 playerscore[k] = 6; 01272 suit = 4; 01273 } 01274 pc.printf("\nplayer%d suit%d", k+1, suit); 01275 //Determine if straight or straight flush 01276 m=0; 01277 for(i=0; i<14; i++) { 01278 if((count[i]>=1)&&(count[i+1]>=1)) { 01279 m++; 01280 if(m>=4) { 01281 if(((i-3<playerface[k][1])&&(playerface[k][1]<i+2))||((i-3<playerface[k][0])&&(playerface[k][0]<i+2))) { 01282 if(playerscore[k]==6) { 01283 if(((i-3<playerface[k][1])&&(playerface[k][1]<i+2)&&(playersuit[k][1]==suit))||((i-3<playerface[k][0])&&(playerface[k][0]<i+2)&&(playersuit[k][0]==suit))) { 01284 playerscore[k]=9; 01285 } 01286 } else { 01287 playerscore[k]=5; 01288 } 01289 } 01290 } else { 01291 m=0; 01292 } 01293 } 01294 } 01295 01296 01297 //Determine if three of a kind, two pair, or pair 01298 m=0; 01299 if(playerscore[k]==0) { 01300 for(i=0; i<13; i++) { 01301 if(count[i]==3) { 01302 playerscore[k]=4; 01303 } else { 01304 if(count[i]==2) { 01305 m++; 01306 } 01307 01308 } 01309 } 01310 if(m==2)playerscore[k]=3; 01311 if(m==1)playerscore[k]=2; 01312 } 01313 01314 01315 //determine if only high card 01316 if(playerscore[k] == 0) playerscore[k] = 1; 01317 } 01318 pc.printf("\nprelim player%d score:%d",k+1,playerscore[k]); 01319 } 01320 } 01321 //determine winner based off player score 01322 temp=0; 01323 for(i=0; i<p; i++) { 01324 if(temp<=playerscore[i]) { 01325 temp=playerscore[i]; 01326 } else { 01327 playerscore[i]=0; 01328 } 01329 } 01330 for(i=0; i<p; i++) { 01331 if(playerscore[i]<temp)playerscore[i]=0; 01332 } 01333 //determine amount of winners 01334 m=0; 01335 for(i=0; i<p; i++) { 01336 if(playerscore[i]>0) m++; 01337 //pc.printf("\n amount of winners:%d", m); 01338 } 01339 //Determine high card if multiple winners 01340 temp=0; 01341 if(m>1) { 01342 for(i=0; i<p; i++) { 01343 if(temp<=highcard[i]) { 01344 temp=highcard[i]; 01345 } else { 01346 highcard[i]=0; 01347 } 01348 } 01349 for(i=0; i<p; i++) { 01350 if(highcard[i]<temp)playerscore[i]=0; 01351 } 01352 } 01353 //determine if two players have the same high card 01354 m=0; 01355 for(i=0; i<p; i++) { 01356 if(playerscore[i]>0) m++; 01357 //pc.printf("\n amount of winners:%d", m); 01358 } 01359 //distribute winnings 01360 for(i=0; i<p; i++) { 01361 pc.printf("\n player%dscore=%d",i+1,playerscore[i]); 01362 if(playerscore[i]>0) { 01363 playermoney[i] += pot/m ; 01364 pc.printf("\n player%d won $%d",i+1, pot/m); 01365 } 01366 } 01367 for(i=0; i<p; i++) { 01368 playerscore[i]=0; 01369 } 01370 01371 //determine if player is out of money 01372 for(i=0; i<p; i++) { 01373 if(playermoney[i] == 0) p--; 01374 } 01375 if(p==1) { 01376 pc.printf("Play Again?\nFold=No Call=Yes"); 01377 m=read_keypad(0); 01378 if(m==-2) main(); 01379 if(m==-4) return 0; 01380 } 01381 } 01382 }
Generated on Thu Jul 14 2022 13:33:52 by
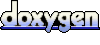