
Poker code for primary Mbed
Embed:
(wiki syntax)
Show/hide line numbers
NokiaLCD.cpp
00001 /* mbed Nokia LCD Library 00002 * Copyright (c) 2007-2010, sford 00003 */ 00004 00005 #include "NokiaLCD.h" 00006 00007 #include "mbed.h" 00008 00009 #define NOKIALCD_ROWS 16 00010 #define NOKIALCD_COLS 16 00011 #define NOKIALCD_WIDTH 130 00012 #define NOKIALCD_HEIGHT 130 00013 #define NOKIALCD_FREQUENCY 5000000 00014 00015 NokiaLCD::NokiaLCD(PinName mosi, PinName sclk, PinName cs, PinName rst, LCDType type) 00016 : _spi(mosi, NC, sclk) 00017 , _rst(rst) 00018 , _cs(cs) 00019 { 00020 00021 _type = type; 00022 00023 _row = 0; 00024 _column = 0; 00025 _foreground = 0x00FFFFFF; 00026 _background = 0x00000000; 00027 00028 reset(); 00029 } 00030 00031 void NokiaLCD::reset() 00032 { 00033 00034 // setup the SPI interface and bring display out of reset 00035 _cs = 1; 00036 _rst = 0; 00037 _spi.format(9); 00038 _spi.frequency(NOKIALCD_FREQUENCY); 00039 wait_ms(1); 00040 _rst = 1; 00041 wait_ms(1); 00042 00043 _cs = 0; 00044 00045 switch (_type) { 00046 case LCD6100: 00047 command(0xCA); // display control 00048 data(0); 00049 data(32); 00050 data(0); 00051 command(0xBB); 00052 data(1); 00053 command(0xD1); // oscillator on 00054 command(0x94); // sleep out 00055 command(0x20); // power control 00056 data(0x0F); 00057 command(0xA7); // invert display 00058 command(0x81); // Voltage control 00059 data(39); // contrast setting: 0..63 00060 data(3); // resistance ratio 00061 wait_ms(1); 00062 command(0xBC); 00063 data(0); 00064 data(1); 00065 data(4); 00066 command(0xAF); // turn on the display 00067 break; 00068 00069 case LCD6610: 00070 command(0xCA); // display control 00071 data(0); 00072 data(31); 00073 data(0); 00074 command(0xBB); 00075 data(1); 00076 command(0xD1); // oscillator on 00077 command(0x94); // sleep out 00078 command(0x20); // power control 00079 data(0x0F); 00080 command(0xA7); // invert display 00081 command(0x81); // Voltage control 00082 data(39); // contrast setting: 0..63 00083 data(3); // resistance ratio 00084 wait_ms(1); 00085 command(0xBC); 00086 data(0); 00087 data(0); 00088 data(2); 00089 command(0xAF); // turn on the display 00090 break; 00091 00092 case PCF8833: 00093 command(0x11); // sleep out 00094 command(0x3A); // column mode 00095 data(0x05); 00096 command(0x36); // madctl 00097 data(0x60); // vertical RAM, flip x 00098 command(0x25); // setcon 00099 data(0x30);// contrast 0x30 00100 wait_ms(2); 00101 command(0x29);//DISPON 00102 command(0x03);//BSTRON 00103 break; 00104 } 00105 00106 _cs = 1; 00107 00108 cls(); 00109 } 00110 00111 void NokiaLCD::command(int value) 00112 { 00113 _spi.write(value & 0xFF); 00114 } 00115 00116 void NokiaLCD::data(int value) 00117 { 00118 _spi.write(value | 0x100); 00119 } 00120 00121 void NokiaLCD::_window(int x, int y, int width, int height) 00122 { 00123 int x1 = x + 0; 00124 int y1 = y + 0; 00125 int x2 = x1 + width - 1; 00126 int y2 = y1 + height - 1; 00127 00128 switch (_type) { 00129 case LCD6100: 00130 case LCD6610: 00131 command(0x15); // column 00132 data(x1); 00133 data(x2); 00134 command(0x75); // row 00135 data(y1); 00136 data(y2); 00137 command(0x5C); // start write to ram 00138 break; 00139 case PCF8833: 00140 command(0x2A); // column 00141 data(x1); 00142 data(x2); 00143 command(0x2B); // row 00144 data(y1); 00145 data(y2); 00146 command(0x2C); // start write to ram 00147 break; 00148 } 00149 } 00150 00151 void NokiaLCD::_putp(int colour) 00152 { 00153 int gr = ((colour >> 20) & 0x0F) 00154 | ((colour >> 8 ) & 0xF0); 00155 int nb = ((colour >> 4 ) & 0x0F); 00156 data(nb); 00157 data(gr); 00158 } 00159 00160 const unsigned char FONT8x8[97][8] = { 00161 0x08,0x08,0x08,0x00,0x00,0x00,0x00,0x00, // columns, rows, num_bytes_per_char 00162 0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00, // space 0x20 00163 0x30,0x78,0x78,0x30,0x30,0x00,0x30,0x00, // ! 00164 0x6C,0x6C,0x6C,0x00,0x00,0x00,0x00,0x00, // " 00165 0x6C,0x6C,0xFE,0x6C,0xFE,0x6C,0x6C,0x00, // # 00166 0x18,0x3E,0x60,0x3C,0x06,0x7C,0x18,0x00, // $ 00167 0x00,0x63,0x66,0x0C,0x18,0x33,0x63,0x00, // % 00168 0x1C,0x36,0x1C,0x3B,0x6E,0x66,0x3B,0x00, // & 00169 0x30,0x30,0x60,0x00,0x00,0x00,0x00,0x00, // ' 00170 0x0C,0x18,0x30,0x30,0x30,0x18,0x0C,0x00, // ( 00171 0x30,0x18,0x0C,0x0C,0x0C,0x18,0x30,0x00, // ) 00172 0x00,0x66,0x3C,0xFF,0x3C,0x66,0x00,0x00, // * 00173 0x00,0x30,0x30,0xFC,0x30,0x30,0x00,0x00, // + 00174 0x00,0x00,0x00,0x00,0x00,0x18,0x18,0x30, // , 00175 0x00,0x00,0x00,0x7E,0x00,0x00,0x00,0x00, // - 00176 0x00,0x00,0x00,0x00,0x00,0x18,0x18,0x00, // . 00177 0x03,0x06,0x0C,0x18,0x30,0x60,0x40,0x00, // / (forward slash) 00178 0x3E,0x63,0x63,0x6B,0x63,0x63,0x3E,0x00, // 0 0x30 00179 0x18,0x38,0x58,0x18,0x18,0x18,0x7E,0x00, // 1 00180 0x3C,0x66,0x06,0x1C,0x30,0x66,0x7E,0x00, // 2 00181 0x3C,0x66,0x06,0x1C,0x06,0x66,0x3C,0x00, // 3 00182 0x0E,0x1E,0x36,0x66,0x7F,0x06,0x0F,0x00, // 4 00183 0x7E,0x60,0x7C,0x06,0x06,0x66,0x3C,0x00, // 5 00184 0x1C,0x30,0x60,0x7C,0x66,0x66,0x3C,0x00, // 6 00185 0x7E,0x66,0x06,0x0C,0x18,0x18,0x18,0x00, // 7 00186 0x3C,0x66,0x66,0x3C,0x66,0x66,0x3C,0x00, // 8 00187 0x3C,0x66,0x66,0x3E,0x06,0x0C,0x38,0x00, // 9 00188 0x00,0x18,0x18,0x00,0x00,0x18,0x18,0x00, // : 00189 0x00,0x18,0x18,0x00,0x00,0x18,0x18,0x30, // ; 00190 0x0C,0x18,0x30,0x60,0x30,0x18,0x0C,0x00, // < 00191 0x00,0x00,0x7E,0x00,0x00,0x7E,0x00,0x00, // = 00192 0x30,0x18,0x0C,0x06,0x0C,0x18,0x30,0x00, // > 00193 0x3C,0x66,0x06,0x0C,0x18,0x00,0x18,0x00, // ? 00194 0x3E,0x63,0x6F,0x69,0x6F,0x60,0x3E,0x00, // @ 0x40 00195 0x18,0x3C,0x66,0x66,0x7E,0x66,0x66,0x00, // A 00196 0x7E,0x33,0x33,0x3E,0x33,0x33,0x7E,0x00, // B 00197 0x1E,0x33,0x60,0x60,0x60,0x33,0x1E,0x00, // C 00198 0x7C,0x36,0x33,0x33,0x33,0x36,0x7C,0x00, // D 00199 0x7F,0x31,0x34,0x3C,0x34,0x31,0x7F,0x00, // E 00200 0x7F,0x31,0x34,0x3C,0x34,0x30,0x78,0x00, // F 00201 0x1E,0x33,0x60,0x60,0x67,0x33,0x1F,0x00, // G 00202 0x66,0x66,0x66,0x7E,0x66,0x66,0x66,0x00, // H 00203 0x3C,0x18,0x18,0x18,0x18,0x18,0x3C,0x00, // I 00204 0x0F,0x06,0x06,0x06,0x66,0x66,0x3C,0x00, // J 00205 0x73,0x33,0x36,0x3C,0x36,0x33,0x73,0x00, // K 00206 0x78,0x30,0x30,0x30,0x31,0x33,0x7F,0x00, // L 00207 0x63,0x77,0x7F,0x7F,0x6B,0x63,0x63,0x00, // M 00208 0x63,0x73,0x7B,0x6F,0x67,0x63,0x63,0x00, // N 00209 0x3E,0x63,0x63,0x63,0x63,0x63,0x3E,0x00, // O 00210 0x7E,0x33,0x33,0x3E,0x30,0x30,0x78,0x00, // P 0x50 00211 0x3C,0x66,0x66,0x66,0x6E,0x3C,0x0E,0x00, // Q 00212 0x7E,0x33,0x33,0x3E,0x36,0x33,0x73,0x00, // R 00213 0x3C,0x66,0x30,0x18,0x0C,0x66,0x3C,0x00, // S 00214 0x7E,0x5A,0x18,0x18,0x18,0x18,0x3C,0x00, // T 00215 0x66,0x66,0x66,0x66,0x66,0x66,0x7E,0x00, // U 00216 0x66,0x66,0x66,0x66,0x66,0x3C,0x18,0x00, // V 00217 0x63,0x63,0x63,0x6B,0x7F,0x77,0x63,0x00, // W 00218 0x63,0x63,0x36,0x1C,0x1C,0x36,0x63,0x00, // X 00219 0x66,0x66,0x66,0x3C,0x18,0x18,0x3C,0x00, // Y 00220 0x7F,0x63,0x46,0x0C,0x19,0x33,0x7F,0x00, // Z 00221 0x3C,0x30,0x30,0x30,0x30,0x30,0x3C,0x00, // [ 00222 0x60,0x30,0x18,0x0C,0x06,0x03,0x01,0x00, // \ (back slash) 00223 0x3C,0x0C,0x0C,0x0C,0x0C,0x0C,0x3C,0x00, // ] 00224 0x08,0x1C,0x36,0x63,0x00,0x00,0x00,0x00, // ^ 00225 0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xFF, // _ 00226 0x18,0x18,0x0C,0x00,0x00,0x00,0x00,0x00, // ` 0x60 00227 0x00,0x00,0x3C,0x06,0x3E,0x66,0x3B,0x00, // a 00228 0x70,0x30,0x3E,0x33,0x33,0x33,0x6E,0x00, // b 00229 0x00,0x00,0x3C,0x66,0x60,0x66,0x3C,0x00, // c 00230 0x0E,0x06,0x3E,0x66,0x66,0x66,0x3B,0x00, // d 00231 0x00,0x00,0x3C,0x66,0x7E,0x60,0x3C,0x00, // e 00232 0x1C,0x36,0x30,0x78,0x30,0x30,0x78,0x00, // f 00233 0x00,0x00,0x3B,0x66,0x66,0x3E,0x06,0x7C, // g 00234 0x70,0x30,0x36,0x3B,0x33,0x33,0x73,0x00, // h 00235 0x18,0x00,0x38,0x18,0x18,0x18,0x3C,0x00, // i 00236 0x06,0x00,0x06,0x06,0x06,0x66,0x66,0x3C, // j 00237 0x70,0x30,0x33,0x36,0x3C,0x36,0x73,0x00, // k 00238 0x38,0x18,0x18,0x18,0x18,0x18,0x3C,0x00, // l 00239 0x00,0x00,0x66,0x7F,0x7F,0x6B,0x63,0x00, // m 00240 0x00,0x00,0x7C,0x66,0x66,0x66,0x66,0x00, // n 00241 0x00,0x00,0x3C,0x66,0x66,0x66,0x3C,0x00, // o 00242 0x00,0x00,0x6E,0x33,0x33,0x3E,0x30,0x78, // p 00243 0x00,0x00,0x3B,0x66,0x66,0x3E,0x06,0x0F, // q 00244 0x00,0x00,0x6E,0x3B,0x33,0x30,0x78,0x00, // r 00245 0x00,0x00,0x3E,0x60,0x3C,0x06,0x7C,0x00, // s 00246 0x08,0x18,0x3E,0x18,0x18,0x1A,0x0C,0x00, // t 00247 0x00,0x00,0x66,0x66,0x66,0x66,0x3B,0x00, // u 00248 0x00,0x00,0x66,0x66,0x66,0x3C,0x18,0x00, // v 00249 0x00,0x00,0x63,0x6B,0x7F,0x7F,0x36,0x00, // w 00250 0x00,0x00,0x63,0x36,0x1C,0x36,0x63,0x00, // x 00251 0x00,0x00,0x66,0x66,0x66,0x3E,0x06,0x7C, // y 00252 0x00,0x00,0x7E,0x4C,0x18,0x32,0x7E,0x00, // z 00253 0x0E,0x18,0x18,0x70,0x18,0x18,0x0E,0x00, // { 00254 0x0C,0x0C,0x0C,0x00,0x0C,0x0C,0x0C,0x00, // | 00255 0x70,0x18,0x18,0x0E,0x18,0x18,0x70,0x00, // } 00256 0x3B,0x6E,0x00,0x00,0x00,0x00,0x00,0x00, // ~ 00257 0x1C,0x36,0x36,0x1C,0x00,0x00,0x00,0x00 00258 }; // DEL 00259 00260 void NokiaLCD::locate(int column, int row) 00261 { 00262 _column = column; 00263 _row = row; 00264 } 00265 00266 void NokiaLCD::newline() 00267 { 00268 _column = 0; 00269 _row++; 00270 if (_row >= _rows) { 00271 _row = 0; 00272 } 00273 } 00274 00275 int NokiaLCD::_putc(int value) 00276 { 00277 int x = _column * 8; // FIXME: Char sizes 00278 int y = _row * 8; 00279 bitblit(x + 1, y + 1, 8, 8, (char*)&(FONT8x8[value - 0x1F][0])); 00280 00281 _column++; 00282 00283 if (_column >= NOKIALCD_COLS) { 00284 _row++; 00285 _row++; 00286 _column = 0; 00287 } 00288 00289 if (_row >= NOKIALCD_ROWS) { 00290 _row = 0; 00291 } 00292 00293 return value; 00294 } 00295 00296 void NokiaLCD::cls() 00297 { 00298 fill(0, 0, NOKIALCD_WIDTH, NOKIALCD_HEIGHT, _background); 00299 _row = 0; 00300 _column = 0; 00301 } 00302 00303 00304 void NokiaLCD::window(int x, int y, int width, int height) 00305 { 00306 _cs = 0; 00307 _window(x, y, width, height); 00308 _cs = 1; 00309 } 00310 00311 void NokiaLCD::putp(int colour) 00312 { 00313 _cs = 0; 00314 _putp(colour); 00315 _cs = 1; 00316 } 00317 00318 void NokiaLCD::pixel(int x, int y, int colour) 00319 { 00320 _cs = 0; 00321 _window(x, y, 1, 1); 00322 switch (_type) { 00323 case LCD6100: 00324 case PCF8833: 00325 00326 _putp(colour); 00327 00328 break; 00329 case LCD6610: 00330 00331 int r4 = (colour >> (16 + 4)) & 0xF; 00332 int g4 = (colour >> (8 + 4)) & 0xF; 00333 int b4 = (colour >> (0 + 4)) & 0xF; 00334 int d1 = (r4 << 4) | g4; 00335 int d2 = (b4 << 4) | r4; 00336 int d3 = (g4 << 4) | b4; 00337 data(d1); 00338 data(d2); 00339 data(d3); 00340 00341 break; 00342 } 00343 _cs = 1; 00344 } 00345 00346 00347 void NokiaLCD::fill(int x, int y, int width, int height, int colour) 00348 { 00349 _cs = 0; 00350 _window(x, y, width, height); 00351 switch (_type) { 00352 case LCD6100: 00353 case PCF8833: 00354 for (int i=0; i<width*height; i++) { 00355 _putp(colour); 00356 } 00357 break; 00358 case LCD6610: 00359 for (int i=0; i<width*height/2; i++) { 00360 int r4 = (colour >> (16 + 4)) & 0xF; 00361 int g4 = (colour >> (8 + 4)) & 0xF; 00362 int b4 = (colour >> (0 + 4)) & 0xF; 00363 int d1 = (r4 << 4) | g4; 00364 int d2 = (b4 << 4) | r4; 00365 int d3 = (g4 << 4) | b4; 00366 data(d1); 00367 data(d2); 00368 data(d3); 00369 } 00370 break; 00371 } 00372 _window(0, 0, NOKIALCD_WIDTH, NOKIALCD_HEIGHT); 00373 _cs = 1; 00374 } 00375 00376 void NokiaLCD::blit(int x, int y, int width, int height, const int* colour) 00377 { 00378 _cs = 0; 00379 _window(x, y, width, height); 00380 00381 switch (_type) { 00382 case LCD6100: 00383 case PCF8833: 00384 for (int i=0; i<width*height; i++) { 00385 _putp(colour[i]); 00386 } 00387 break; 00388 case LCD6610: 00389 for (int i=0; i<width*height/2; i++) { 00390 int r41 = (colour[i*2] >> (16 + 4)) & 0xF; 00391 int g41 = (colour[i*2] >> (8 + 4)) & 0xF; 00392 int b41 = (colour[i*2] >> (0 + 4)) & 0xF; 00393 00394 int r42 = (colour[i*2+1] >> (16 + 4)) & 0xF; 00395 int g42 = (colour[i*2+1] >> (8 + 4)) & 0xF; 00396 int b42 = (colour[i*2+1] >> (0 + 4)) & 0xF; 00397 int d1 = (r41 << 4) | g41; 00398 int d2 = (b41 << 4) | r42; 00399 int d3 = (g42 << 4) | b42; 00400 data(d1); 00401 data(d2); 00402 data(d3); 00403 } 00404 break; 00405 } 00406 _window(0, 0, NOKIALCD_WIDTH, NOKIALCD_HEIGHT); 00407 _cs = 1; 00408 } 00409 00410 void NokiaLCD::bitblit(int x, int y, int width, int height, const char* bitstream) 00411 { 00412 _cs = 0; 00413 _window(x, y, width, height); 00414 00415 switch (_type) { 00416 case LCD6100: 00417 case PCF8833: 00418 for (int i=0; i<height*width; i++) { 00419 int byte = i / 8; 00420 int bit = i % 8; 00421 int colour = ((bitstream[byte] << bit) & 0x80) ? _foreground : _background; 00422 _putp(colour); 00423 } 00424 break; 00425 case LCD6610: 00426 for(int i=0; i<height*width/2; i++) { 00427 int byte1 = (i*2) / 8; 00428 int bit1 = (i*2) % 8; 00429 int colour1 = ((bitstream[byte1] << bit1) & 0x80) ? _foreground : _background; 00430 int byte2 = (i*2+1) / 8; 00431 int bit2 = (i*2+1) % 8; 00432 int colour2 = ((bitstream[byte2] << bit2) & 0x80) ? _foreground : _background; 00433 00434 int r41 = (colour1 >> (16 + 4)) & 0xF; 00435 int g41 = (colour1 >> (8 + 4)) & 0xF; 00436 int b41 = (colour1 >> (0 + 4)) & 0xF; 00437 00438 int r42 = (colour2 >> (16 + 4)) & 0xF; 00439 int g42 = (colour2 >> (8 + 4)) & 0xF; 00440 int b42 = (colour2 >> (0 + 4)) & 0xF; 00441 int d1 = (r41 << 4) | g41; 00442 int d2 = (b41 << 4) | r42; 00443 int d3 = (g42 << 4) | b42; 00444 data(d1); 00445 data(d2); 00446 data(d3); 00447 } 00448 break; 00449 } 00450 _window(0, 0, _width, _height); 00451 _cs = 1; 00452 } 00453 00454 void NokiaLCD::foreground(int c) 00455 { 00456 _foreground = c; 00457 } 00458 00459 void NokiaLCD::background(int c) 00460 { 00461 _background = c; 00462 } 00463 00464 int NokiaLCD::width() 00465 { 00466 return NOKIALCD_WIDTH; 00467 } 00468 00469 int NokiaLCD::height() 00470 { 00471 return NOKIALCD_HEIGHT; 00472 } 00473 00474 int NokiaLCD::columns() 00475 { 00476 return NOKIALCD_COLS; 00477 } 00478 00479 int NokiaLCD::rows() 00480 { 00481 return NOKIALCD_ROWS; 00482 }
Generated on Thu Jul 14 2022 13:33:52 by
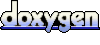