Library for httpserver
Fork of httpServer_with_Ethernt by
Embed:
(wiki syntax)
Show/hide line numbers
FsHandler.cpp
00001 /* FsHandler.cpp */ 00002 #include "mbed.h" 00003 #include "FsHandler.h" 00004 //#define DEBUG 00005 #include "hl_debug.h" 00006 00007 #define Do3 131 //C octave3 00008 #define Do3s 139 //C# 00009 #define Re3 147 //D 00010 #define Re3s 156//D# 00011 #define Mi3 165 //E 00012 #define Fa3 175 //F 00013 #define Fa3s 185 //F# 00014 #define So3 196 //G 00015 #define So3s 208 //G# 00016 #define La3 220 //A 00017 #define La3s 233 //A# 00018 #define Ti3 247 //B 00019 #define Do4 262 //C octave4 00020 #define Do4s 277 //C# 00021 #define Re4 294 //D 00022 #define Re4s 311//D# 00023 #define Mi4 330 //E 00024 #define Fa4 349 //F 00025 #define Fa4s 370 //F# 00026 #define So4 392 //G 00027 #define So4s 415 //G# 00028 #define La4 440 //A 00029 #define La4s 466 //A# 00030 #define Ti4 494 //B 00031 #define Do5 523 //C octave5 00032 #define Do5s 554 //C# 00033 #define Re5 587 //D 00034 #define Re5s 622//D# 00035 #define Mi5 659 //E 00036 #define Fa5 699 //F 00037 #define Fa5s 740 //F# 00038 #define So5 784 //G 00039 #define So5s 831 //G# 00040 #define La5 880 //A 00041 #define La5s 932 //A# 00042 #define Ti5 988 //B 00043 00044 PwmOut Buzzer(D3); 00045 00046 float C_3 = 1000000/Do3, 00047 Cs_3 = 1000000/Do3s, 00048 D_3 = 1000000/Re3, 00049 Ds_3 = 1000000/Re3s, 00050 E_3 = 1000000/Mi3, 00051 F_3 = 1000000/Fa3, 00052 Fs_3 = 1000000/Fa3s, 00053 G_3 = 1000000/So3, 00054 Gs_3 = 1000000/So3s, 00055 A_3 = 1000000/La3, 00056 As_3 = 1000000/La3s, 00057 B_3 = 1000000/Ti3, 00058 C_4 = 1000000/Do4, 00059 Cs_4 = 1000000/Do4s, 00060 D_4 = 1000000/Re4, 00061 Ds_4 = 1000000/Re4s, 00062 E_4 = 1000000/Mi4, 00063 F_4 = 1000000/Fa4, 00064 Fs_4 = 1000000/Fa4s, 00065 G_4 = 1000000/So4, 00066 Gs_4 = 1000000/So4s, 00067 A_4 = 1000000/La4, 00068 As_4 = 1000000/La4s, 00069 B_4 = 1000000/Ti4, 00070 C_5 = 1000000/Do5, 00071 Cs_5 = 1000000/Do5s, 00072 D_5 = 1000000/Re5, 00073 Ds_5 = 1000000/Re5s, 00074 E_5 = 1000000/Mi5, 00075 F_5 = 1000000/Fa5, 00076 Fs_5 = 1000000/Fa5s, 00077 G_5 = 1000000/So5, 00078 Gs_5 = 1000000/So5s, 00079 A_5 = 1000000/La5, 00080 As_5 = 1000000/La5s, 00081 B_5 = 1000000/Ti5; 00082 00083 int tones[] = {E_4, D_4, C_4, D_4, E_4, E_4, E_4, 0, D_4, D_4, D_4, 0, E_4, G_4, G_4, 0, 00084 E_4, D_4, C_4, D_4, E_4, E_4, E_4, 0, D_4, D_4, E_4, D_4, C_4, 0, 0, 0}; 00085 int tones_num = 32; 00086 00087 void Tune(PwmOut name, int period); 00088 void Auto_tunes(PwmOut name, int period); 00089 void Stop_tunes(PwmOut name); 00090 00091 DigitalOut led_red(LED1); 00092 DigitalOut led_green(LED2); 00093 DigitalOut led_blue(LED3); 00094 00095 DigitalIn din(PC_14); 00096 00097 static int matchstrings(const char* one, const char* two) 00098 { 00099 int m = 0; 00100 00101 for (m = 0; m < min(strlen(one), strlen(two)) ; m++) { 00102 if (one[m] != two[m]) 00103 return m; 00104 } 00105 return m; 00106 } 00107 00108 std::map<const char*, const char*> HTTPFsRequestHandler::m_fsMap; 00109 00110 HTTPFsRequestHandler::HTTPFsRequestHandler(const char* rootPath, const char* localPath, HTTPConnection::HTTPMessage& Msg, TCPSocketConnection& Tcp) 00111 : HTTPRequestHandler(Msg, Tcp) 00112 { 00113 m_rootPath = rootPath; 00114 m_localPath = localPath; 00115 00116 string myPath = m_rootPath + m_localPath; 00117 00118 // Now replace the virtual root path with a mounted device path 00119 std::map<const char*, const char*>::iterator it; 00120 const char *bestMatch = NULL; 00121 const char *bestMatchExchange = NULL; 00122 int match_ind = -1; 00123 for (it = m_fsMap.begin() ; it != m_fsMap.end() ; it++) { 00124 // find best match (if the given logical path is containted in the root 00125 int s = matchstrings(myPath.c_str(), it->second); 00126 INFO("Matching Root %s with handler %s results in %d identical characters\n", myPath.c_str(), it->second, s); 00127 if ((s == strlen(it->second)) && (s > match_ind)) { 00128 match_ind = s; 00129 bestMatch = it->first; 00130 bestMatchExchange = it->second; 00131 } 00132 } 00133 00134 if (bestMatch != NULL) { 00135 m_rootPath = bestMatch; 00136 m_localPath = string(myPath).substr(strlen(bestMatchExchange)); 00137 } 00138 00139 handleRequest(); 00140 } 00141 00142 HTTPFsRequestHandler::~HTTPFsRequestHandler() 00143 { 00144 } 00145 00146 int HTTPFsRequestHandler::handleGetRequest() 00147 { 00148 HTTPHeaders headers; 00149 int retval = 0; //success 00150 uint8_t pin_state; 00151 00152 if( std::string::npos != msg.uri.find("get_dio14.cgi") ) 00153 { 00154 if(din) 00155 pin_state = 1; 00156 else 00157 pin_state = 0; 00158 00159 /* 00160 *len = sprintf((char *)buf, "DioCallback({\"dio_p\":\"14\",\ 00161 \"dio_s\":\"%d\"\ 00162 });", 00163 pin_state // Digital io status 00164 ); 00165 00166 00167 Tcp. 00168 */ 00169 } 00170 else //read html pages 00171 { 00172 if (m_localPath.length() > 4) 00173 getStandardHeaders(headers, m_localPath.substr(m_localPath.length()-4).c_str()); 00174 else 00175 getStandardHeaders(headers); 00176 00177 INFO("Handling Get Request (root = %s, local = %s).", m_rootPath.c_str(), m_localPath.c_str()); 00178 00179 std::string reqPath; 00180 00181 // Check if we received a directory with the local bath 00182 if ((m_localPath.length() == 0) || (m_localPath.substr( m_localPath.length()-1, 1) == "/")) { 00183 // yes, we shall append the default page name 00184 m_localPath += "index.html"; 00185 } 00186 00187 reqPath = m_rootPath + m_localPath; 00188 00189 INFO("Mapping \"%s\" to \"%s\"", msg.uri.c_str(), reqPath.c_str()); 00190 00191 FILE *fp = fopen(reqPath.c_str(), "r"); 00192 if (fp != NULL) { 00193 char * pBuffer = NULL; 00194 int sz = 8192; 00195 while( pBuffer == NULL) { 00196 sz /= 2; 00197 pBuffer = (char*)malloc(sz); 00198 if (sz < 128) 00199 error ("OutOfMemory"); 00200 } 00201 00202 // File was found and can be returned 00203 00204 // first determine the size 00205 fseek(fp, 0, SEEK_END); 00206 long size = ftell(fp); 00207 fseek(fp, 0, SEEK_SET); 00208 00209 startResponse(200, size); 00210 while(!feof(fp) && !ferror(fp)) { 00211 int cnt = fread(pBuffer, 1, sz , fp); 00212 if (cnt < 0) 00213 cnt = 0; 00214 processResponse(cnt, pBuffer); 00215 } 00216 delete pBuffer; 00217 endResponse(); 00218 fclose(fp); 00219 } 00220 else { 00221 retval = 404; 00222 ERR("Requested file was not found !"); 00223 } 00224 } 00225 00226 return retval; 00227 } 00228 00229 int HTTPFsRequestHandler::handlePostRequest() 00230 { 00231 int i = 0; 00232 int pin = 0; 00233 00234 if( std::string::npos != msg.uri.find("set_LED.cgi") ) 00235 { 00236 pin = get_http_param_value("pin"); 00237 if(pin==0) Tune(Buzzer, C_4); 00238 else if(pin==1) Tune(Buzzer, Cs_4); 00239 else if(pin==2) Tune(Buzzer, D_4); 00240 else if(pin==3) Tune(Buzzer, Ds_4); 00241 else if(pin==4) Tune(Buzzer, E_4); 00242 else if(pin==5) Tune(Buzzer, F_4); 00243 else if(pin==6) Tune(Buzzer, Fs_4); 00244 else if(pin==7) Tune(Buzzer, G_4); 00245 else if(pin==8) Tune(Buzzer, Gs_4); 00246 else if(pin==9) Tune(Buzzer, A_4); 00247 else if(pin==10) Tune(Buzzer, As_4); 00248 else if(pin==11) Tune(Buzzer, B_4); 00249 else if(pin==12) Tune(Buzzer, C_5); 00250 else if(pin==13) Tune(Buzzer, Cs_5); 00251 else if(pin==14) Tune(Buzzer, D_5); 00252 else if(pin==15) Tune(Buzzer, Ds_5); 00253 else if(pin==16) Tune(Buzzer, E_5); 00254 else if(pin==17) Tune(Buzzer, F_5); 00255 else if(pin==18) Tune(Buzzer, Fs_5); 00256 else if(pin==19) Tune(Buzzer, G_5); 00257 else if(pin==20) Tune(Buzzer, Gs_5); 00258 else if(pin==21) Tune(Buzzer, A_5); 00259 else if(pin==22) Tune(Buzzer, As_5); 00260 else if(pin==23) Tune(Buzzer, B_5); 00261 else 00262 { 00263 WARN("Wrong pin number"); 00264 } 00265 00266 return 0; 00267 } 00268 else if( std::string::npos != msg.uri.find("set_AUTO.cgi") ) 00269 { 00270 pin = get_http_param_value("pin"); 00271 if(pin==0) 00272 { 00273 for(i=0; i<tones_num; i++) 00274 { 00275 Auto_tunes(Buzzer, tones[i]); 00276 wait_ms(124); 00277 } 00278 Stop_tunes(Buzzer); 00279 } 00280 else if(pin == 99) 00281 { 00282 Stop_tunes(Buzzer); 00283 } 00284 else 00285 { 00286 WARN("Wrong pin number"); 00287 } 00288 00289 return 0; 00290 } 00291 else 00292 { 00293 return 404; 00294 } 00295 } 00296 00297 int HTTPFsRequestHandler::handlePutRequest() 00298 { 00299 return 404; 00300 } 00301 00302 uint32_t HTTPFsRequestHandler::get_http_param_value(char* param_name) 00303 { 00304 uint8_t * name = 0; 00305 uint8_t * pos2; 00306 uint16_t len = 0; 00307 char value[10]; 00308 uint32_t ret = 0; 00309 00310 //msg.attri 00311 if((name = (uint8_t *)strstr(msg.attri, param_name))) 00312 { 00313 name += strlen(param_name) + 1; 00314 pos2 = (uint8_t*)strstr((char*)name, "&"); 00315 if(!pos2) 00316 { 00317 pos2 = name + strlen((char*)name); 00318 } 00319 len = pos2 - name; 00320 00321 if(len) 00322 { 00323 strncpy(value, (char*)name, len); 00324 ret = atoi(value); 00325 } 00326 } 00327 return ret; 00328 } 00329 00330 /** 00331 * @brief Tune Function 00332 * @param name : Choose the PwmOut 00333 period : this param is tune value. (C_3...B_5) 00334 * @retval None 00335 */ 00336 void Tune(PwmOut name, int period) 00337 { 00338 name.period_us(period); 00339 name.write(0.50f); // 50% duty cycle 00340 wait_ms(250); // 1/4 beat 00341 name.period_us(0); // Sound off 00342 } 00343 00344 /** 00345 * @brief Auto tunes Function 00346 * @param name : Choose the PwmOut 00347 period : this param is tune value. (C_3...B_5) 00348 * @retval None 00349 */ 00350 void Auto_tunes(PwmOut name, int period) 00351 { 00352 name.period_us(period); 00353 name.write(0.50f); // 50% duty cycle 00354 wait_ms(250); // 1/4 beat 00355 } 00356 00357 void Stop_tunes(PwmOut name) 00358 { 00359 name.period_us(0); 00360 }
Generated on Wed Jul 13 2022 04:13:19 by
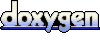