jhgfd
Fork of WizFi310Interface_Legacy_newv34 by
Embed:
(wiki syntax)
Show/hide line numbers
TCPSocketServer.cpp
00001 /* 00002 * Copyright (C) 2013 gsfan, MIT License 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00005 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00006 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00007 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00008 * furnished to do so, subject to the following conditions: 00009 * 00010 * The above copyright notice and this permission notice shall be included in all copies or 00011 * substantial portions of the Software. 00012 * 00013 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00014 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00015 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00016 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00017 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00018 */ 00019 /* Copyright (C) 2014 Wiznet, MIT License 00020 * port to the Wiznet Module WizFi250 00021 */ 00022 /* Copyright (C) 2017 Wiznet, MIT License 00023 * port to the Wiznet Module WizFi310 00024 */ 00025 00026 #include "TCPSocketServer.h" 00027 #include <string> 00028 00029 TCPSocketServer::TCPSocketServer() {} 00030 00031 // Server initialization 00032 int TCPSocketServer::bind(int port) { 00033 00034 _port = port; 00035 return 0; 00036 } 00037 00038 int TCPSocketServer::listen(int backlog) { 00039 _server = true; 00040 if (_cid < 0) { 00041 // Socket open 00042 _server = false; 00043 _cid = _wizfi310->listen(WizFi310::PROTO_TCP, _port); 00044 00045 //WIZ_DBG("TEST CID : %d",_cid); 00046 if (_cid < 0) return -1; 00047 } 00048 00049 if (backlog != 1) 00050 return -1; 00051 return 0; 00052 } 00053 00054 00055 int TCPSocketServer::accept(TCPSocketConnection& connection) { 00056 int acid = -1; 00057 00058 while (1) { 00059 while (acid < 0) { 00060 acid = _wizfi310->accept(_cid); 00061 } 00062 00063 if (acid >= 0) { 00064 connection.acceptCID(acid); 00065 return 0; 00066 } 00067 } 00068 return -1; 00069 }
Generated on Fri Jul 15 2022 14:05:55 by
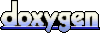