
Testprogram for STLED316S driver lib SPI Interface
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /* mbed STLED316S Test program, for STLED316S LED controller 00002 * Copyright (c) 2016, v01: WH, Initial version 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a copy 00005 * of this software and associated documentation files (the "Software"), to deal 00006 * in the Software without restriction, including without limitation the rights 00007 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00008 * copies of the Software, and to permit persons to whom the Software is 00009 * furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included in 00012 * all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00015 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00016 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00017 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00018 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00019 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00020 * THE SOFTWARE. 00021 */ 00022 #include "mbed.h" 00023 #include "STLED316S.h" 00024 00025 Serial pc(USBTX, USBRX); 00026 DigitalOut myled(LED1); 00027 00028 // Select one of the testboards for STM STLED316S LED controller 00029 00030 #if (STLED316S_TEST == 1) 00031 //High level Control STLED316S 00032 00033 STLED316S::DisplayData_t all_str = {0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF}; 00034 STLED316S::DisplayData_t bye_str = {LO(C7_B), LO(C7_Y), LO(C7_E), 0x00, 0x00, 0x00}; 00035 STLED316S::LedData_t all_led = {0xFF}; 00036 00037 // KeyData_t size is 2 bytes 00038 STLED316S::KeyData_t keydata; 00039 00040 // STLED316S declaration, Default setting 6 Grids @ 8 Segments 00041 STLED316S STLED316S(p5,p6,p7, p8); 00042 00043 char cmd, bits; 00044 int main() { 00045 00046 pc.printf("Hello World\r\n"); // 00047 00048 STLED316S.cls(); 00049 STLED316S.writeData(all_str); 00050 STLED316S.writeLedData(all_led); 00051 wait(2); 00052 00053 #if(1) 00054 pc.printf("Test Global Brightness\r\n"); 00055 STLED316S.setBrightMode(STLED316S::GlobalBright); 00056 00057 STLED316S.setBrightness(STLED316S_BRT3); 00058 wait(1); 00059 STLED316S.setBrightness(STLED316S_BRT0); 00060 wait(1); 00061 STLED316S.setBrightness(STLED316S_BRT3); 00062 00063 STLED316S.clrLed(STLED316S_LED_ALL); 00064 wait(1); 00065 STLED316S.setLed(STLED316S_LED_L1); 00066 wait(1); 00067 STLED316S.clrLed(STLED316S_LED_L1); 00068 00069 STLED316S.setLed(STLED316S_LED_L2); 00070 wait(1); 00071 STLED316S.clrLed(STLED316S_LED_L2); 00072 00073 STLED316S.setLed(STLED316S_LED_L3); 00074 wait(1); 00075 STLED316S.clrLed(STLED316S_LED_L3); 00076 00077 wait(1); 00078 STLED316S.setLed(STLED316S_LED_L1 | STLED316S_LED_L2 | STLED316S_LED_L3); 00079 #endif 00080 00081 while (1) { 00082 // Check and read keydata 00083 if (STLED316S.getKeys(&keydata)) { 00084 pc.printf("Keydata 0..1 = 0x%02X 0x%02X\r\n", keydata[0], keydata[1]); 00085 00086 if (keydata[0] == 0x01) { // Key1&KS1 00087 pc.printf("Test Individual Brightness\r\n"); 00088 STLED316S.setBrightMode(STLED316S::IndivBright); 00089 00090 wait(1); 00091 STLED316S.setLedBrightness(STLED316S_LED_L1, STLED316S_BRT3); 00092 wait(1); 00093 STLED316S.setLedBrightness(STLED316S_LED_L1, STLED316S_BRT0); 00094 00095 wait(1); 00096 STLED316S.setLedBrightness(STLED316S_LED_L2, STLED316S_BRT3); 00097 wait(1); 00098 STLED316S.setLedBrightness(STLED316S_LED_L2, STLED316S_BRT0); 00099 00100 wait(1); 00101 STLED316S.setLedBrightness(STLED316S_LED_L3, STLED316S_BRT3); 00102 wait(1); 00103 STLED316S.setLedBrightness(STLED316S_LED_L3, STLED316S_BRT0); 00104 00105 wait(1); 00106 STLED316S.setDigitBrightness(STLED316S_DIG_D2, STLED316S_BRT3); 00107 wait(1); 00108 STLED316S.setDigitBrightness(STLED316S_DIG_D2, STLED316S_BRT0); 00109 00110 wait(1); 00111 STLED316S.setDigitBrightness(STLED316S_DIG_D3, STLED316S_BRT3); 00112 wait(1); 00113 STLED316S.setDigitBrightness(STLED316S_DIG_D3, STLED316S_BRT0); 00114 00115 wait(1); 00116 STLED316S.setDigitBrightness(STLED316S_DIG_D4, STLED316S_BRT3); 00117 wait(1); 00118 STLED316S.setDigitBrightness(STLED316S_DIG_D4, STLED316S_BRT0); 00119 00120 wait(1); 00121 STLED316S.setDigitBrightness(STLED316S_DIG_D5, STLED316S_BRT3); 00122 wait(1); 00123 STLED316S.setDigitBrightness(STLED316S_DIG_D5, STLED316S_BRT0); 00124 00125 wait(1); 00126 STLED316S.setDigitBrightness(STLED316S_DIG_D6, STLED316S_BRT3); 00127 wait(1); 00128 STLED316S.setDigitBrightness(STLED316S_DIG_D6, STLED316S_BRT0); 00129 00130 wait(1); 00131 STLED316S.setDigitBrightness(STLED316S_DIG_D7, STLED316S_BRT3); 00132 wait(1); 00133 STLED316S.setDigitBrightness(STLED316S_DIG_D7, STLED316S_BRT0); 00134 00135 wait(1); 00136 STLED316S.setBrightMode(STLED316S::GlobalBright); 00137 } 00138 00139 if (keydata[0] == 0x02) { // Key1&KS2 00140 STLED316S.cls(); 00141 } 00142 00143 if (keydata[0] == 0x04) { // Key1&KS3 00144 #if(1) 00145 //test to show all segs 00146 pc.printf("Show all segs\r\n"); 00147 wait(1); 00148 STLED316S.cls(); 00149 00150 for (int i=0; i<STLED316S_DISPLAY_MEM; i++) { 00151 for (int bit=0; bit<8; bit++) { 00152 STLED316S.cls(); 00153 00154 bits = 0x01 << bit; 00155 STLED316S.writeData(i, bits); 00156 00157 pc.printf("Idx = %d, Bits = 0x%02x\r\n", i, bits); 00158 // wait(0.5); 00159 cmd = pc.getc(); // wait for key 00160 } 00161 } 00162 pc.printf("Show all segs done\r\n"); 00163 wait(0.5); 00164 STLED316S.writeData(all_str); 00165 #endif 00166 } 00167 00168 00169 } //if 00170 00171 myled = !myled; 00172 wait(0.3); 00173 } //while 00174 } 00175 #endif 00176 00177 00178 00179 #if (ST316BOARD_TEST == 1) 00180 //High level Control STLED316S test display 00181 00182 STLED316S::DisplayData_t all_str = {0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF}; 00183 STLED316S::DisplayData_t bye_str = {LO(C7_B), LO(C7_Y), LO(C7_E), 0x00, 0x00, 0x00}; 00184 STLED316S::LedData_t all_led = {0xFF}; 00185 00186 // KeyData_t size is 2 bytes 00187 STLED316S::KeyData_t keydata; 00188 00189 // STLED316S test display declaration: 6 Grids @ 8 Segments, 3 LEDs, 3 Keys 00190 STLED316S_BOARD STLED316S(p5,p6,p7, p8); 00191 00192 char cmd, bits; 00193 int main() { 00194 00195 pc.printf("Hello World\r\n"); // 00196 00197 STLED316S.cls(); 00198 STLED316S.writeData(all_str); 00199 STLED316S.writeLedData(all_led); 00200 wait(2); 00201 00202 #if(1) 00203 pc.printf("Test Global Brightness\r\n"); 00204 STLED316S.setBrightMode(STLED316S::GlobalBright); 00205 00206 STLED316S.setBrightness(STLED316S_BRT3); 00207 wait(0.5); 00208 STLED316S.setBrightness(STLED316S_BRT0); 00209 wait(0.5); 00210 STLED316S.setBrightness(STLED316S_BRT3); 00211 00212 STLED316S.clrLed(STLED316S_LED_ALL); 00213 wait(0.5); 00214 STLED316S.setLed(STLED316S_LED_L1); 00215 wait(0.5); 00216 STLED316S.clrLed(STLED316S_LED_L1); 00217 00218 STLED316S.setLed(STLED316S_LED_L2); 00219 wait(0.5); 00220 STLED316S.clrLed(STLED316S_LED_L2); 00221 00222 STLED316S.setLed(STLED316S_LED_L3); 00223 wait(0.5); 00224 STLED316S.clrLed(STLED316S_LED_L3); 00225 00226 wait(0.5); 00227 STLED316S.setLed(STLED316S_LED_L1 | STLED316S_LED_L2 | STLED316S_LED_L3); 00228 #endif 00229 00230 while (1) { 00231 // Check and read keydata 00232 if (STLED316S.getKeys(&keydata)) { 00233 pc.printf("Keydata 0..1 = 0x%02X 0x%02X\r\n", keydata[0], keydata[1]); 00234 00235 if (keydata[0] == 0x01) { // Key1&KS1 00236 pc.printf("Test Individual Brightness\r\n"); 00237 STLED316S.setBrightMode(STLED316S::IndivBright); 00238 00239 wait(1); 00240 STLED316S.setLedBrightness(STLED316S_LED_L1, STLED316S_BRT3); 00241 wait(0.5); 00242 STLED316S.setLedBrightness(STLED316S_LED_L1, STLED316S_BRT0); 00243 00244 wait(0.5); 00245 STLED316S.setLedBrightness(STLED316S_LED_L2, STLED316S_BRT3); 00246 wait(0.5); 00247 STLED316S.setLedBrightness(STLED316S_LED_L2, STLED316S_BRT0); 00248 00249 wait(0.5); 00250 STLED316S.setLedBrightness(STLED316S_LED_L3, STLED316S_BRT3); 00251 wait(0.5); 00252 STLED316S.setLedBrightness(STLED316S_LED_L3, STLED316S_BRT0); 00253 00254 wait(0.5); 00255 STLED316S.setDigitBrightness(STLED316S_DIG_D2, STLED316S_BRT3); 00256 wait(0.5); 00257 STLED316S.setDigitBrightness(STLED316S_DIG_D2, STLED316S_BRT0); 00258 00259 wait(0.5); 00260 STLED316S.setDigitBrightness(STLED316S_DIG_D3, STLED316S_BRT3); 00261 wait(0.5); 00262 STLED316S.setDigitBrightness(STLED316S_DIG_D3, STLED316S_BRT0); 00263 00264 wait(0.5); 00265 STLED316S.setDigitBrightness(STLED316S_DIG_D4, STLED316S_BRT3); 00266 wait(0.5); 00267 STLED316S.setDigitBrightness(STLED316S_DIG_D4, STLED316S_BRT0); 00268 00269 wait(0.5); 00270 STLED316S.setDigitBrightness(STLED316S_DIG_D5, STLED316S_BRT3); 00271 wait(0.5); 00272 STLED316S.setDigitBrightness(STLED316S_DIG_D5, STLED316S_BRT0); 00273 00274 wait(0.5); 00275 STLED316S.setDigitBrightness(STLED316S_DIG_D6, STLED316S_BRT3); 00276 wait(0.5); 00277 STLED316S.setDigitBrightness(STLED316S_DIG_D6, STLED316S_BRT0); 00278 00279 wait(0.5); 00280 STLED316S.setDigitBrightness(STLED316S_DIG_D7, STLED316S_BRT3); 00281 wait(0.5); 00282 STLED316S.setDigitBrightness(STLED316S_DIG_D7, STLED316S_BRT0); 00283 00284 wait(0.5); 00285 STLED316S.setBrightMode(STLED316S::GlobalBright); 00286 } 00287 00288 if (keydata[0] == 0x02) { // Key1&KS2 00289 STLED316S.cls(); 00290 00291 STLED316S.locate(0); 00292 STLED316S.printf("%06x", 0x012345); 00293 wait(1.0); 00294 STLED316S.locate(0); 00295 STLED316S.printf("%06x", 0x789ABC); 00296 wait(1.0); 00297 STLED316S.printf("%01.4f", -0.2345); 00298 00299 wait(2.0); 00300 STLED316S.printf("Hello "); 00301 } 00302 00303 if (keydata[0] == 0x04) { // Key1&KS3 00304 #if(0) 00305 //test to show all segs 00306 pc.printf("Show all segs\r\n"); 00307 wait(1); 00308 STLED316S.cls(); 00309 00310 for (int i=0; i<STLED316S_DISPLAY_MEM; i++) { 00311 for (int bit=0; bit<8; bit++) { 00312 STLED316S.cls(); 00313 00314 bits = 0x01 << bit; 00315 STLED316S.writeData(i, bits); 00316 00317 pc.printf("Idx = %d, Bits = 0x%02x\r\n", i, bits); 00318 // wait(0.5); 00319 cmd = pc.getc(); // wait for key 00320 } 00321 } 00322 pc.printf("Show all segs done\r\n"); 00323 wait(0.5); 00324 STLED316S.writeData(all_str); 00325 #else 00326 //test to show all digits (base is 0x10) 00327 pc.printf("Show all hex digits\r\n"); 00328 wait(1); 00329 STLED316S.cls(); 00330 00331 STLED316S.printf("%06x", 0x01ABCD); 00332 cmd = pc.getc(); // wait for key 00333 00334 for (int i=0; i<ST316BOARD_NR_DIGITS; i++) { 00335 00336 for (int cnt=0; cnt<0x10; cnt++) { 00337 STLED316S.locate(i); 00338 STLED316S.printf("%01x", cnt); 00339 00340 // wait(0.5); 00341 cmd = pc.getc(); // wait for key 00342 } 00343 } 00344 pc.printf("\r\nShow all hex digits done\r\n"); 00345 #endif 00346 } 00347 00348 } //if 00349 00350 myled = !myled; 00351 wait(0.3); 00352 } //while 00353 } 00354 #endif 00355 00356 00357
Generated on Thu Jul 14 2022 02:26:08 by
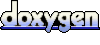