
Example code for the PT6312 VFD driver. Also supports the PT6312 in ASCII mode as used in the Philips DVP630 DVD player.
main.cpp
00001 /* mbed PT6312 Test program, for Princeton PT6312 VFD controller 00002 * Copyright (c) 2015, v01: WH, Initial version 00003 * 2015, v02: WH, Test DVD462 and C2233 00004 * 00005 * Permission is hereby granted, free of charge, to any person obtaining a copy 00006 * of this software and associated documentation files (the "Software"), to deal 00007 * in the Software without restriction, including without limitation the rights 00008 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00009 * copies of the Software, and to permit persons to whom the Software is 00010 * furnished to do so, subject to the following conditions: 00011 * 00012 * The above copyright notice and this permission notice shall be included in 00013 * all copies or substantial portions of the Software. 00014 * 00015 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00016 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00017 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00018 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00019 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00020 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00021 * THE SOFTWARE. 00022 */ 00023 #include "mbed.h" 00024 #include "PT6312.h" 00025 00026 00027 #if (DVP630_TEST == 1) 00028 // Philips DVP630 Display Test 00029 00030 Serial pc(USBTX, USBRX); 00031 DigitalOut myled(LED1); 00032 00033 // KeyData_t size is 3 bytes 00034 PT6312::KeyData_t keydata; 00035 00036 // Switchdata is 1 byte 00037 char switchdata; 00038 00039 // PT6312_DVP630 declaration. Setting 7 Grids @ 15 Segments) 00040 PT6312_DVP630 DVP630(p5,p6,p7, p8); 00041 00042 int main() { 00043 00044 pc.printf("Hello World\r\n"); // 00045 00046 DVP630.cls(); 00047 // DVP630.putc('A'); 00048 // wait(1); 00049 00050 DVP630.setUDC(0, UDC_SANDHR); //Set UDC 00051 DVP630.putc((char) 0); 00052 wait(1); 00053 00054 DVP630.setBrightness(PT6312_BRT7); 00055 wait(1); 00056 00057 //wheel 00058 for(int cnt=0;cnt<10;cnt++) { 00059 for(int idx=0;idx<8;idx++) { 00060 DVP630.setUDC(0, WHEEL_ANI[idx]); 00061 DVP630.locate(0); 00062 DVP630.printf("%c%c%c%c%c%c%c",(char)0,(char)0,(char)0,(char)0,(char)0,(char)0,(char)0); 00063 00064 wait(0.1); 00065 } 00066 } 00067 00068 00069 //test to show all chars 00070 wait(1); 00071 DVP630.cls(); 00072 00073 for (int i=0x20; i<0x80; i++) { 00074 // DVP630.cls(); 00075 DVP630.locate(0); 00076 DVP630.printf("0x%2X=%c ", i, (char) i); 00077 wait(0.2); 00078 // pc.getc(); 00079 } 00080 00081 while (1) { 00082 00083 // Check and read keydata 00084 if (DVP630.getKeys(&keydata)) { 00085 pc.printf("Keydata 0..2 = 0x%02x 0x%02x 0x%02x\r\n", keydata[0], keydata[1], keydata[2]); 00086 00087 if (keydata[0] == 0x01) { //play 00088 DVP630.cls(); 00089 DVP630.printf("AAAAAAA"); 00090 DVP630.setIcon(PT6312_DVP630::COL3); 00091 DVP630.setIcon(PT6312_DVP630::COL5); 00092 } 00093 00094 if (keydata[0] == 0x02) { //stop 00095 DVP630.cls(); 00096 DVP630.printf("HELLO "); 00097 DVP630.clrIcon(PT6312_DVP630::COL3); 00098 DVP630.clrIcon(PT6312_DVP630::COL5); 00099 } 00100 00101 if (keydata[0] == 0x04) { //open/close 00102 DVP630.cls(); 00103 DVP630.printf("MBED "); 00104 } 00105 00106 } //if Key 00107 00108 00109 // Check and read switch data 00110 switchdata = DVP630.getSwitches(); 00111 00112 if (switchdata != 0) { 00113 pc.printf("Switchdata = 0x%02x\r\n", switchdata); 00114 00115 if (switchdata == PT6312_SW1) { //S1 00116 DVP630.cls(); 00117 DVP630.printf(" HELLO "); 00118 } 00119 00120 if (switchdata == PT6312_SW2) { //S2 00121 DVP630.cls(); 00122 DVP630.printf("BYE "); 00123 } 00124 00125 if (switchdata == PT6312_SW3) { //S3 00126 DVP630.cls(); 00127 DVP630.printf("HI MBED"); 00128 } 00129 00130 DVP630.setLED(switchdata); //write LEDs in same pattern 00131 } //if Switch 00132 00133 myled = !myled; 00134 wait(0.3); 00135 } //while 00136 00137 } 00138 #endif 00139 00140 00141 #if (DVD462_TEST == 1) 00142 // DVD462 PT6312 Test 00143 #include "mbed.h" 00144 #include "PT6312.h" 00145 #include "Font_7Seg.h" 00146 00147 Serial pc(USBTX, USBRX); 00148 DigitalOut myled(LED1); 00149 00150 // DisplayData_t size is 8 bytes (4 digits @ max 16 segments) ... 22 bytes (11 digits @ max 11 segments) 00151 // DisplayData_t size default is 22 bytes (11 digits @ max 11 segments) 00152 PT6312::DisplayData_t all_str = {0xFF,0xFF, 0xFF,0xFF, 0xFF,0xFF, 0xFF,0xFF, 0xFF,0xFF, 0xFF,0xFF}; 00153 PT6312::DisplayData_t bye_str = {0x00,0x00, 0x00,0x00, 0x00,0x00, 0x00,0x00, LO(C7_E),HI(C7_E), LO(C7_Y),HI(C7_Y), LO(C7_B),HI(C7_B)}; 00154 PT6312::DisplayData_t hello_str = {0x00,0x00, 0x00,0x00, LO(C7_O),HI(C7_O), LO(C7_L),HI(C7_L), LO(C7_L),HI(C7_L), LO(C7_E),HI(C7_E), LO(C7_H), HI(C7_H)}; 00155 PT6312::DisplayData_t mbed_str = {LO(C7_D), HI(C7_D), LO(C7_E), HI(C7_E), LO(C7_B), HI(C7_B), LO(C7_M), HI(C7_M)}; 00156 00157 // KeyData_t size is 3 bytes 00158 PT6312::KeyData_t keydata; 00159 00160 // Switchdata is 1 byte 00161 char switchdata; 00162 00163 //PT6312_DVD462 declaration, Setting 6 Grids @ 16 Segments 00164 PT6312_DVD462 DVD462(p5,p6,p7, p8); 00165 00166 char cmd, bits; 00167 int main() { 00168 00169 pc.printf("Hello World\r\n"); // 00170 00171 DVD462.cls(); 00172 DVD462.writeData(all_str); 00173 wait(4); 00174 DVD462.setBrightness(PT6312_BRT3); 00175 wait(1); 00176 DVD462.setBrightness(PT6312_BRT0); 00177 wait(1); 00178 DVD462.setBrightness(PT6312_BRT7); 00179 00180 while (1) { 00181 00182 // Check and read keydata 00183 if (DVD462.getKeys(&keydata)) { 00184 pc.printf("Keydata 0..2 = 0x%02x 0x%02x 0x%02x\r\n", keydata[0], keydata[1], keydata[2]); 00185 00186 if (keydata[0] == 0x01) { //play 00187 DVD462.cls(); 00188 DVD462.writeData(all_str); 00189 } 00190 00191 if (keydata[0] == 0x02) { //pause 00192 DVD462.cls(); 00193 // DVD462.writeData(hello_str); 00194 #if(1) 00195 //test to show all segs 00196 pc.printf("Show all segs\r\n"); 00197 wait(1); 00198 DVD462.cls(); 00199 00200 for (int i=0; i<PT6312_DISPLAY_MEM; i++) { 00201 for (int bit=0; bit<8; bit++) { 00202 DVD462.cls(); 00203 00204 bits = 0x01 << bit; 00205 DVD462.writeData(i, bits); 00206 00207 pc.printf("Idx = %d, Bits = 0x%02x\r\n", i, bits); 00208 // wait(0.5); 00209 cmd = pc.getc(); // wait for key 00210 } 00211 } 00212 pc.printf("Show all segs done\r\n"); 00213 #endif 00214 00215 } 00216 00217 00218 if (keydata[0] == 0x04) { //stop 00219 // DVD462.cls(); 00220 // DVD462.writeData(mbed_str); 00221 00222 #if(0) 00223 //test to show all chars 00224 pc.printf("Show all chars\r\n"); 00225 wait(1); 00226 DVD462.cls(); 00227 00228 for (int i=0; i<26; i++) { 00229 DVD462.writeData(0, FONT_16S[i][0]); 00230 DVD462.writeData(1, FONT_16S[i][1]); 00231 wait(1); 00232 } 00233 pc.printf("Show all chars done\r\n"); 00234 #endif 00235 00236 00237 #if(0) 00238 //test to show all digits (base is 10) 00239 pc.printf("Show all digits\r\n"); 00240 wait(1); 00241 DVD462.cls(); 00242 00243 int val = 1; 00244 for (int i=0; i<DVD462_NR_DIGITS; i++) { 00245 00246 for (int cnt=0; cnt<10; cnt++) { 00247 DVD462.locate(0); 00248 DVD462.printf("%07d", (val * cnt)); 00249 00250 // wait(0.5); 00251 cmd = pc.getc(); // wait for key 00252 } 00253 val = val * 10; 00254 } 00255 pc.printf("Show all digits done\r\n"); 00256 #endif 00257 00258 #if(1) 00259 //test to show all digits (base is 0x10) 00260 pc.printf("Show all digits\r\n"); 00261 wait(1); 00262 DVD462.cls(); 00263 00264 DVD462.printf("%07x", 0x012ABCD); 00265 cmd = pc.getc(); // wait for key 00266 00267 int val = 1; 00268 for (int i=0; i<DVD462_NR_DIGITS; i++) { 00269 00270 for (int cnt=0; cnt<0x10; cnt++) { 00271 DVD462.locate(0); 00272 DVD462.printf("%07x", (val * cnt)); 00273 00274 // wait(0.5); 00275 cmd = pc.getc(); // wait for key 00276 } 00277 val = val * 0x10; 00278 } 00279 pc.printf("Show all digits done\r\n"); 00280 #endif 00281 00282 } 00283 00284 if (keydata[0] == 0x10) { //open/close 00285 // DVD462.cls(); 00286 // DVD462.writeData(mbed_str); 00287 #if(1) 00288 //test to show all icons 00289 pc.printf("Show all icons\r\n"); 00290 wait(1); 00291 DVD462.cls(true); // Also clear all Icons 00292 wait(1); 00293 00294 DVD462.setIcon(PT6312_DVD462::MP3); 00295 DVD462.setIcon(PT6312_DVD462::CD); 00296 DVD462.setIcon(PT6312_DVD462::V); 00297 DVD462.setIcon(PT6312_DVD462::S); 00298 DVD462.setIcon(PT6312_DVD462::DVD); 00299 00300 DVD462.setIcon(PT6312_DVD462::DDD); 00301 DVD462.setIcon(PT6312_DVD462::DTS); 00302 00303 DVD462.setIcon(PT6312_DVD462::COL5); 00304 DVD462.setIcon(PT6312_DVD462::COL3); 00305 00306 DVD462.setIcon(PT6312_DVD462::ARW); 00307 DVD462.setIcon(PT6312_DVD462::ALL); 00308 DVD462.setIcon(PT6312_DVD462::PSE); 00309 DVD462.setIcon(PT6312_DVD462::PLY); 00310 DVD462.setIcon(PT6312_DVD462::PBC); 00311 00312 DVD462.setIcon(PT6312_DVD462::P1); 00313 DVD462.setIcon(PT6312_DVD462::P2); 00314 DVD462.setIcon(PT6312_DVD462::P3); 00315 DVD462.setIcon(PT6312_DVD462::P4); 00316 DVD462.setIcon(PT6312_DVD462::P5); 00317 DVD462.setIcon(PT6312_DVD462::P6); 00318 DVD462.setIcon(PT6312_DVD462::P7); 00319 DVD462.setIcon(PT6312_DVD462::P8); 00320 DVD462.setIcon(PT6312_DVD462::P9); 00321 DVD462.setIcon(PT6312_DVD462::P10); 00322 DVD462.setIcon(PT6312_DVD462::P11); 00323 DVD462.setIcon(PT6312_DVD462::P12); 00324 DVD462.setIcon(PT6312_DVD462::P13); 00325 00326 wait(1); 00327 DVD462.cls(); // clear all, preserve Icons 00328 pc.printf("Show all icons done\r\n"); 00329 #endif 00330 } 00331 00332 if (keydata[0] == 0x20) { //fr 00333 // DVD462.cls(); 00334 // DVD462.writeData(mbed_str); 00335 00336 //write LEDs off 00337 DVD462.setLED(0x01); 00338 } 00339 00340 if (keydata[0] == 0x40) { //ff 00341 // DVD462.cls(); 00342 // DVD462.writeData(mbed_str); 00343 00344 //write LEDs on 00345 DVD462.setLED(0x00); 00346 00347 } 00348 00349 if (keydata[0] == 0x80) { //pwr 00350 DVD462.cls(true); 00351 // DVD462.writeData(mbed_str); 00352 // DVD462.writeData(mbed_str); 00353 00354 float delay=0.1; 00355 // Piechart Icons on 00356 DVD462.setIcon(PT6312_DVD462::P13); wait(delay); 00357 DVD462.setIcon(PT6312_DVD462::P12); wait(delay); 00358 DVD462.setIcon(PT6312_DVD462::P11); wait(delay); 00359 DVD462.setIcon(PT6312_DVD462::P10); wait(delay); 00360 DVD462.setIcon(PT6312_DVD462::P9); wait(delay); 00361 DVD462.setIcon(PT6312_DVD462::P8); wait(delay); 00362 DVD462.setIcon(PT6312_DVD462::P7); wait(delay); 00363 DVD462.setIcon(PT6312_DVD462::P6); wait(delay); 00364 DVD462.setIcon(PT6312_DVD462::P5); wait(delay); 00365 DVD462.setIcon(PT6312_DVD462::P4); wait(delay); 00366 DVD462.setIcon(PT6312_DVD462::P3); wait(delay); 00367 DVD462.setIcon(PT6312_DVD462::P2); wait(delay); 00368 DVD462.setIcon(PT6312_DVD462::P1); wait(delay); 00369 00370 wait(delay); 00371 00372 // Piechart Icons off 00373 DVD462.clrIcon(PT6312_DVD462::P13); wait(delay); 00374 DVD462.clrIcon(PT6312_DVD462::P12); wait(delay); 00375 DVD462.clrIcon(PT6312_DVD462::P11); wait(delay); 00376 DVD462.clrIcon(PT6312_DVD462::P10); wait(delay); 00377 DVD462.clrIcon(PT6312_DVD462::P9); wait(delay); 00378 DVD462.clrIcon(PT6312_DVD462::P8); wait(delay); 00379 DVD462.clrIcon(PT6312_DVD462::P7); wait(delay); 00380 DVD462.clrIcon(PT6312_DVD462::P6); wait(delay); 00381 DVD462.clrIcon(PT6312_DVD462::P5); wait(delay); 00382 DVD462.clrIcon(PT6312_DVD462::P4); wait(delay); 00383 DVD462.clrIcon(PT6312_DVD462::P3); wait(delay); 00384 DVD462.clrIcon(PT6312_DVD462::P2); wait(delay); 00385 DVD462.clrIcon(PT6312_DVD462::P1); wait(delay); 00386 } 00387 00388 } //if Key 00389 00390 00391 // Check and read switch data 00392 switchdata = DVD462.getSwitches(); 00393 00394 if (switchdata != 0) { 00395 pc.printf("Switchdata = 0x%02x\r\n", switchdata); 00396 00397 if (switchdata == PT6312_SW1) { //S1 00398 DVD462.cls(); 00399 DVD462.writeData(hello_str); 00400 } 00401 00402 if (switchdata == PT6312_SW2) { //S2 00403 DVD462.cls(); 00404 DVD462.writeData(bye_str); 00405 } 00406 00407 if (switchdata == PT6312_SW3) { //S3 00408 DVD462.cls(); 00409 DVD462.writeData(mbed_str); 00410 } 00411 00412 DVD462.setLED(switchdata); //write LEDs in same pattern 00413 } //if Switch 00414 00415 00416 myled = !myled; 00417 wait(0.3); 00418 } //while 00419 } 00420 #endif 00421 00422 00423 #if (C2233_TEST == 1) 00424 // C2233 PT6312 Test 00425 #include "mbed.h" 00426 #include "PT6312.h" 00427 #include "Font_7Seg.h" 00428 00429 Serial pc(USBTX, USBRX); 00430 DigitalOut myled(LED1); 00431 00432 // DisplayData_t size is 8 bytes (4 digits @ max 16 segments) ... 22 bytes (11 digits @ max 11 segments) 00433 // DisplayData_t size default is 22 bytes (11 digits @ max 11 segments) 00434 PT6312::DisplayData_t all_str = {0xFF,0xFF, 0xFF,0xFF, 0xFF,0xFF, 0xFF,0xFF, 0xFF,0xFF, 0xFF,0xFF}; 00435 //PT6312::DisplayData_t bye_str = {0x00,0x00, 0x00,0x00, 0x00,0x00, 0x00,0x00, LO(C7_E),HI(C7_E), LO(C7_Y),HI(C7_Y), LO(C7_B),HI(C7_B)}; 00436 PT6312::DisplayData_t hello_str = {0x00,0x00, (C7_O | ((C7_L & 0x01) << 7)),(C7_L >> 1), C7_L,C7_E, C7_H,0x00, 0x00,0x00, 0x00,0x00}; 00437 //PT6312::DisplayData_t mbed_str = {LO(C7_D), HI(C7_D), LO(C7_E), HI(C7_E), LO(C7_B), HI(C7_B), LO(C7_M), HI(C7_M)}; 00438 00439 // KeyData_t size is 3 bytes 00440 PT6312::KeyData_t keydata; 00441 00442 // Switchdata is 1 byte 00443 char switchdata; 00444 00445 //PT6312_C2233 declaration, Setting 6 Grids @ 16 Segments 00446 PT6312_C2233 C2233(p5,p6,p7, p8); 00447 00448 char cmd, bits; 00449 int main() { 00450 00451 pc.printf("Hello World\r\n"); // 00452 00453 C2233.cls(); 00454 C2233.writeData(all_str); 00455 wait(4); 00456 C2233.setBrightness(PT6312_BRT3); 00457 wait(1); 00458 C2233.setBrightness(PT6312_BRT0); 00459 wait(1); 00460 C2233.setBrightness(PT6312_BRT7); 00461 00462 wait(1); 00463 C2233.cls(true); 00464 C2233.writeData(hello_str); 00465 00466 while (1) { 00467 00468 // Check and read keydata 00469 if (C2233.getKeys(&keydata)) { 00470 pc.printf("Keydata 0..2 = 0x%02x 0x%02x 0x%02x\r\n", keydata[0], keydata[1], keydata[2]); 00471 00472 if (keydata[0] == 0x01) { //sw4 00473 C2233.cls(); 00474 C2233.writeData(all_str); 00475 } 00476 00477 if (keydata[0] == 0x02) { //sw7 00478 C2233.cls(); 00479 // C2233.writeData(hello_str); 00480 #if(1) 00481 //test to show all segs 00482 pc.printf("Show all segs\r\n"); 00483 wait(1); 00484 C2233.cls(); 00485 00486 for (int i=0; i<PT6312_DISPLAY_MEM; i++) { 00487 for (int bit=0; bit<8; bit++) { 00488 C2233.cls(); 00489 00490 bits = 0x01 << bit; 00491 C2233.writeData(i, bits); 00492 00493 pc.printf("Idx = %d, Bits = 0x%02x\r\n", i, bits); 00494 // wait(0.5); 00495 cmd = pc.getc(); // wait for key 00496 } 00497 } 00498 pc.printf("Show all segs done\r\n"); 00499 #endif 00500 00501 } 00502 00503 00504 if (keydata[0] == 0x04) { //sw3 00505 // C2233.cls(); 00506 // C2233.writeData(mbed_str); 00507 00508 #if(0) 00509 //test to show all chars 00510 pc.printf("Show all chars\r\n"); 00511 wait(1); 00512 C2233.cls(); 00513 00514 for (int i=0; i<26; i++) { 00515 C2233.writeData(0, FONT_16S[i][0]); 00516 C2233.writeData(1, FONT_16S[i][1]); 00517 wait(1); 00518 } 00519 pc.printf("Show all chars done\r\n"); 00520 #endif 00521 00522 00523 #if(0) 00524 //test to show all digits (base is 10) 00525 pc.printf("Show all digits\r\n"); 00526 wait(1); 00527 C2233.cls(); 00528 00529 int val = 1; 00530 for (int i=0; i<C2233_NR_DIGITS; i++) { 00531 00532 for (int cnt=0; cnt<10; cnt++) { 00533 C2233.locate(0); 00534 C2233.printf("%07d", (val * cnt)); 00535 00536 // wait(0.5); 00537 cmd = pc.getc(); // wait for key 00538 } 00539 val = val * 10; 00540 } 00541 pc.printf("Show all digits done\r\n"); 00542 #endif 00543 00544 #if(1) 00545 //test to show all digits (base is 0x10) 00546 pc.printf("Show all digits\r\n"); 00547 wait(1); 00548 C2233.cls(); 00549 00550 C2233.printf("%07x", 0x012ABCD); 00551 cmd = pc.getc(); // wait for key 00552 00553 int val = 1; 00554 for (int i=0; i<C2233_NR_DIGITS; i++) { 00555 00556 for (int cnt=0; cnt<0x10; cnt++) { 00557 C2233.locate(0); 00558 C2233.printf("%07x", (val * cnt)); 00559 00560 // wait(0.5); 00561 cmd = pc.getc(); // wait for key 00562 } 00563 val = val * 0x10; 00564 } 00565 pc.printf("Show all digits done\r\n"); 00566 #endif 00567 00568 } 00569 00570 if (keydata[0] == 0x10) { //sw5 00571 // C2233.cls(); 00572 // C2233.writeData(mbed_str); 00573 #if(1) 00574 //test to show all icons 00575 pc.printf("Show all icons\r\n"); 00576 wait(1); 00577 C2233.cls(true); // Also clear all Icons 00578 wait(1); 00579 00580 C2233.setIcon(PT6312_C2233::MP3); 00581 C2233.setIcon(PT6312_C2233::PBC); 00582 00583 C2233.setIcon(PT6312_C2233::COL5); 00584 C2233.setIcon(PT6312_C2233::CAM); 00585 00586 C2233.setIcon(PT6312_C2233::COL3); 00587 C2233.setIcon(PT6312_C2233::DDD); 00588 00589 C2233.setIcon(PT6312_C2233::ARW); 00590 C2233.setIcon(PT6312_C2233::ALL); 00591 C2233.setIcon(PT6312_C2233::PSE); 00592 C2233.setIcon(PT6312_C2233::PLY); 00593 C2233.setIcon(PT6312_C2233::CD); 00594 C2233.setIcon(PT6312_C2233::V); 00595 C2233.setIcon(PT6312_C2233::S); 00596 C2233.setIcon(PT6312_C2233::DTS); 00597 00598 C2233.setIcon(PT6312_C2233::P1); 00599 C2233.setIcon(PT6312_C2233::P2); 00600 C2233.setIcon(PT6312_C2233::P3); 00601 C2233.setIcon(PT6312_C2233::P4); 00602 C2233.setIcon(PT6312_C2233::P5); 00603 C2233.setIcon(PT6312_C2233::P6); 00604 C2233.setIcon(PT6312_C2233::P7); 00605 C2233.setIcon(PT6312_C2233::P8); 00606 C2233.setIcon(PT6312_C2233::P9); 00607 C2233.setIcon(PT6312_C2233::P10); 00608 C2233.setIcon(PT6312_C2233::P11); 00609 C2233.setIcon(PT6312_C2233::P12); 00610 C2233.setIcon(PT6312_C2233::P13); 00611 C2233.setIcon(PT6312_C2233::DVD); 00612 00613 wait(1); 00614 C2233.cls(); // clear all, preserve Icons 00615 pc.printf("Show all icons done\r\n"); 00616 #endif 00617 } 00618 00619 if (keydata[0] == 0x20) { //sw6 00620 // C2233.cls(); 00621 // C2233.writeData(mbed_str); 00622 00623 //write LEDs off 00624 C2233.setLED(0x01); 00625 } 00626 00627 if (keydata[0] == 0x40) { //sw2 00628 // C2233.cls(); 00629 // C2233.writeData(mbed_str); 00630 00631 //write LEDs on 00632 C2233.setLED(0x00); 00633 00634 } 00635 00636 if (keydata[0] == 0x80) { //sw1 00637 C2233.cls(true); 00638 // C2233.writeData(mbed_str); 00639 // C2233.writeData(mbed_str); 00640 00641 float delay=0.1; 00642 // Piechart Icons on 00643 C2233.setIcon(PT6312_C2233::P13); wait(delay); 00644 C2233.setIcon(PT6312_C2233::P12); wait(delay); 00645 C2233.setIcon(PT6312_C2233::P11); wait(delay); 00646 C2233.setIcon(PT6312_C2233::P10); wait(delay); 00647 C2233.setIcon(PT6312_C2233::P9); wait(delay); 00648 C2233.setIcon(PT6312_C2233::P8); wait(delay); 00649 C2233.setIcon(PT6312_C2233::P7); wait(delay); 00650 C2233.setIcon(PT6312_C2233::P6); wait(delay); 00651 C2233.setIcon(PT6312_C2233::P5); wait(delay); 00652 C2233.setIcon(PT6312_C2233::P4); wait(delay); 00653 C2233.setIcon(PT6312_C2233::P3); wait(delay); 00654 C2233.setIcon(PT6312_C2233::P2); wait(delay); 00655 C2233.setIcon(PT6312_C2233::P1); wait(delay); 00656 00657 wait(delay); 00658 00659 // Piechart Icons off 00660 C2233.clrIcon(PT6312_C2233::P13); wait(delay); 00661 C2233.clrIcon(PT6312_C2233::P12); wait(delay); 00662 C2233.clrIcon(PT6312_C2233::P11); wait(delay); 00663 C2233.clrIcon(PT6312_C2233::P10); wait(delay); 00664 C2233.clrIcon(PT6312_C2233::P9); wait(delay); 00665 C2233.clrIcon(PT6312_C2233::P8); wait(delay); 00666 C2233.clrIcon(PT6312_C2233::P7); wait(delay); 00667 C2233.clrIcon(PT6312_C2233::P6); wait(delay); 00668 C2233.clrIcon(PT6312_C2233::P5); wait(delay); 00669 C2233.clrIcon(PT6312_C2233::P4); wait(delay); 00670 C2233.clrIcon(PT6312_C2233::P3); wait(delay); 00671 C2233.clrIcon(PT6312_C2233::P2); wait(delay); 00672 C2233.clrIcon(PT6312_C2233::P1); wait(delay); 00673 } 00674 00675 } //if Key 00676 00677 00678 // Check and read switch data (not available on C2233) 00679 switchdata = C2233.getSwitches(); 00680 00681 if (switchdata != 0) { 00682 pc.printf("Switchdata = 0x%02x\r\n", switchdata); 00683 00684 if (switchdata == PT6312_SW1) { //S1 00685 C2233.cls(); 00686 C2233.writeData(hello_str); 00687 } 00688 00689 if (switchdata == PT6312_SW2) { //S2 00690 C2233.cls(); 00691 // C2233.writeData(bye_str); 00692 } 00693 00694 if (switchdata == PT6312_SW3) { //S3 00695 C2233.cls(); 00696 // C2233.writeData(mbed_str); 00697 } 00698 00699 C2233.setLED(switchdata); //write LEDs in same pattern (not available on C2233) 00700 } //if Switch 00701 00702 00703 myled = !myled; 00704 wait(0.3); 00705 } //while 00706 } 00707 #endif 00708 00709 00710 00711 #if(PT6312_TEST == 1) 00712 // Direct PT6312 Test 00713 #include "mbed.h" 00714 #include "PT6312.h" 00715 00716 Serial pc(USBTX, USBRX); 00717 DigitalOut myled(LED1); 00718 00719 // DisplayData_t size is 8 bytes (4 digits @ max 16 segments) ... 22 bytes (11 digits @ max 11 segments) 00720 // DisplayData_t size default is 14 bytes (7 Grids @ 15 Segments) 00721 PT6312::DisplayData_t all_str = {0xFF,0x7F, 0xFF,0x7F, 0xFF,0x7F, 0xFF,0x7F, 0xFF,0x7F, 0xFF,0x7F, 0xFF,0x7F}; 00722 PT6312::DisplayData_t cls_str = {0x00,0x00, 0x00,0x00, 0x00,0x00, 0x00,0x00, 0x00,0x00, 0x00,0x00, 0x00,0x00}; 00723 00724 // KeyData_t size is 3 bytes 00725 PT6312::KeyData_t keydata; 00726 00727 // Switchdata is 1 byte 00728 char switchdata; 00729 00730 PT6312 PT6312(p5,p6,p7, p8); 00731 //PT6312 PT6312(p5,p6,p7, p8, PT6312::Grid4_Seg16); 00732 00733 char cmd, bits; 00734 int main() { 00735 00736 pc.printf("Hello World\r\n"); // 00737 00738 PT6312.cls(); 00739 PT6312.writeData(all_str); 00740 wait(4); 00741 PT6312.setBrightness(PT6312_BRT3); 00742 wait(1); 00743 PT6312.setBrightness(PT6312_BRT0); 00744 wait(1); 00745 PT6312.setBrightness(PT6312_BRT7); 00746 00747 while (1) { 00748 00749 // Check and read keydata 00750 if (PT6312.getKeys(&keydata)) { 00751 pc.printf("Keydata 0..2 = 0x%02x 0x%02x 0x%02x\r\n", keydata[0], keydata[1], keydata[2]); 00752 00753 if (keydata[0] == 0x01) { //play 00754 PT6312.cls(); 00755 PT6312.writeData(all_str); 00756 } 00757 00758 if (keydata[0] == 0x02) { //stop 00759 PT6312.cls(); 00760 PT6312.writeData(cls_str); 00761 } 00762 00763 if (keydata[0] == 0x04) { //open/close 00764 PT6312.cls(); 00765 PT6312.writeData(all_str); 00766 00767 #if(1) 00768 //test to show all segs 00769 pc.printf("Show all segs\r\n"); 00770 wait(1); 00771 PT6312.cls(); 00772 00773 for (int i=0; i<PT6312_DISPLAY_MEM; i++) { 00774 for (int bit=0; bit<8; bit++) { 00775 PT6312.cls(); 00776 00777 bits = 0x01 << bit; 00778 PT6312.writeData(i, bits); 00779 00780 pc.printf("Idx = %d, Bits = 0x%02x\r\n", i, bits); 00781 // wait(0.5); 00782 cmd = pc.getc(); // wait for key 00783 } 00784 } 00785 pc.printf("Show all segs done\r\n"); 00786 #endif 00787 00788 } 00789 00790 } //if Key 00791 00792 00793 // Check and read switch data 00794 switchdata = PT6312.getSwitches(); 00795 00796 if (switchdata != 0) { 00797 pc.printf("Switchdata = 0x%02x\r\n", switchdata); 00798 00799 if (switchdata == PT6312_SW1) { //S1 00800 PT6312.cls(); 00801 PT6312.writeData(all_str); 00802 } 00803 00804 if (switchdata == PT6312_SW2) { //S2 00805 PT6312.cls(); 00806 PT6312.writeData(cls_str); 00807 } 00808 00809 if (switchdata == PT6312_SW3) { //S3 00810 PT6312.cls(); 00811 PT6312.writeData(all_str); 00812 } 00813 00814 PT6312.setLED(switchdata); //write LEDs in same pattern 00815 } //if Switch 00816 00817 00818 myled = !myled; 00819 wait(0.3); 00820 } //while 00821 } 00822 #endif
Generated on Tue Jul 12 2022 21:02:34 by
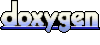