LED Driver, 6 digits @ 8 segm, 8 LEDs, 16 Keys. SPI Interface
Embed:
(wiki syntax)
Show/hide line numbers
Font_7Seg.h
00001 /* mbed LED Font Library, for STM STLED316S controller 00002 * Copyright (c) 2016, v01: WH, Initial version 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a copy 00005 * of this software and associated documentation files (the "Software"), to deal 00006 * in the Software without restriction, including without limitation the rights 00007 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00008 * copies of the Software, and to permit persons to whom the Software is 00009 * furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included in 00012 * all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00015 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00016 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00017 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00018 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00019 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00020 * THE SOFTWARE. 00021 */ 00022 #ifndef MBED_FONT_7SEG_H 00023 #define MBED_FONT_7SEG_H 00024 00025 // Select one of the testboards for STLED316S controller 00026 #include "STLED316S_Config.h" 00027 00028 #if ((STLED316S_TEST == 1) || (ST316BOARD_TEST == 1)) 00029 // Segment bit positions for 7 Segment display using the test display mapping for STLED316S 00030 // Modify this table for different 'bit-to-segment' mappings. The ASCII character defines and the FONT_7S const table below 00031 // will be adapted automatically according to the bit-to-segment mapping. Obviously this will only work when the segment 00032 // mapping is identical for every digit position. This will be the case unless the hardware designer really hates software developers. 00033 // 00034 // A 00035 // ----- 00036 // | | 00037 // F | | B 00038 // | G | 00039 // ----- 00040 // | | 00041 // E | | C 00042 // | | 00043 // ----- * DP 00044 // D 00045 // 00046 #define S7_A 0x01 00047 #define S7_B 0x02 00048 #define S7_C 0x04 00049 #define S7_D 0x08 00050 #define S7_E 0x10 00051 #define S7_F 0x20 00052 #define S7_G 0x40 00053 #define S7_DP 0x80 00054 00055 //Mask for blending out and setting 7 segments digits 00056 #define MASK_7S_ALL = (S7_A | S7_B | S7_C | S7_D | S7_E | S7_F | S7_G} 00057 #endif 00058 00059 00060 // ASCII Font definitions for segments in each character 00061 // 00062 //32 0x20 Symbols 00063 #define C7_SPC (0x0000) 00064 #define C7_EXC (S7_B | S7_C) //! 00065 #define C7_QTE (S7_B | S7_F) //" 00066 #define C7_HSH (S7_C | S7_D | S7_E | S7_G) //# 00067 #define C7_DLR (S7_A | S7_C | S7_D | S7_F | S7_G) //$ 00068 #define C7_PCT (S7_C | S7_F) //% 00069 #define C7_AMP (S7_A | S7_C | S7_D | S7_E | S7_F | S7_G) //& 00070 #define C7_ACC (S7_B) //' 00071 #define C7_LBR (S7_A | S7_D | S7_E | S7_F) //( 00072 #define C7_RBR (S7_A | S7_B | S7_C | S7_D) //) 00073 #define C7_MLT (S7_B | S7_C | S7_E | S7_F | S7_G) //* 00074 #define C7_PLS (S7_B | S7_C | S7_G) //+ 00075 #define C7_CMA (S7_DP) 00076 #define C7_MIN (S7_G) 00077 #define C7_DPT (S7_DP) 00078 #define C7_RS (S7_B | S7_E | S7_G) // / 00079 00080 //48 0x30 Digits 00081 #define C7_0 (S7_A | S7_B | S7_C | S7_D | S7_E | S7_F) 00082 #define C7_1 (S7_B | S7_C) 00083 #define C7_2 (S7_A | S7_B | S7_D | S7_E | S7_G) 00084 #define C7_3 (S7_A | S7_B | S7_C | S7_D | S7_G) 00085 #define C7_4 (S7_B | S7_C | S7_F | S7_G) 00086 #define C7_5 (S7_A | S7_C | S7_D | S7_F | S7_G) 00087 #define C7_6 (S7_A | S7_C | S7_D | S7_E | S7_F | S7_G) 00088 #define C7_7 (S7_A | S7_B | S7_C) 00089 #define C7_8 (S7_A | S7_B | S7_C | S7_D | S7_E | S7_F | S7_G) 00090 #define C7_9 (S7_A | S7_B | S7_C | S7_D | S7_F | S7_G) 00091 00092 //58 0x3A 00093 #define C7_COL (S7_D | S7_G) // : 00094 #define C7_SCL (S7_D | S7_G) // ; 00095 #define C7_LT (S7_D | S7_E | S7_G) // < 00096 #define C7_EQ (S7_D | S7_G) // = 00097 #define C7_GT (S7_C | S7_D | S7_G) // > 00098 #define C7_QM (S7_A | S7_B | S7_E | S7_G) // ? 00099 #define C7_AT (S7_A | S7_B | S7_C | S7_D | S7_E | S7_G) // @ 00100 00101 //65 0x41 Upper case alphabet 00102 #define C7_A (S7_A | S7_B | S7_C | S7_E | S7_F | S7_G ) 00103 #define C7_B (S7_C | S7_D | S7_E | S7_F | S7_G) 00104 #define C7_C (S7_A | S7_D | S7_E | S7_F) 00105 #define C7_D (S7_B | S7_C | S7_D | S7_E | S7_G) 00106 #define C7_E (S7_A | S7_D | S7_E | S7_F | S7_G) 00107 #define C7_F (S7_A | S7_E | S7_F | S7_G) 00108 00109 #define C7_G (S7_A | S7_C | S7_D | S7_E | S7_F) 00110 #define C7_H (S7_B | S7_C | S7_E | S7_F | S7_G) 00111 #define C7_I (S7_B | S7_C) 00112 #define C7_J (S7_B | S7_C | S7_D | S7_E) 00113 #define C7_K (S7_B | S7_C | S7_E | S7_F | S7_G) 00114 #define C7_L (S7_D | S7_E | S7_F) 00115 #define C7_M (S7_A | S7_C | S7_E) 00116 #define C7_N (S7_A | S7_B | S7_C | S7_E | S7_F) 00117 #define C7_O (S7_A | S7_B | S7_C | S7_D | S7_E | S7_F) 00118 #define C7_P (S7_A | S7_B | S7_E | S7_F | S7_G) 00119 #define C7_Q (S7_A | S7_B | S7_C | S7_F | S7_G) 00120 #define C7_R (S7_E | S7_G ) 00121 #define C7_S (S7_A | S7_C | S7_D | S7_F | S7_G) 00122 #define C7_T (S7_D | S7_E | S7_F | S7_G) 00123 #define C7_U (S7_B | S7_C | S7_D | S7_E | S7_F) 00124 #define C7_V (S7_B | S7_C | S7_D | S7_E | S7_F) 00125 #define C7_W (S7_B | S7_D | S7_F) 00126 #define C7_X (S7_B | S7_C | S7_E | S7_F | S7_G) 00127 #define C7_Y (S7_B | S7_C | S7_D | S7_F | S7_G) 00128 #define C7_Z (S7_A | S7_B | S7_D | S7_E | S7_G) 00129 00130 //91 0x5B 00131 #define C7_SBL (S7_A | S7_D | S7_E | S7_F) // [ 00132 #define C7_LS (S7_C | S7_F | S7_G) // left slash 00133 #define C7_SBR (S7_A | S7_B | S7_C | S7_D) // ] 00134 #define C7_PWR (S7_A | S7_B | S7_F) // ^ 00135 #define C7_UDS (S7_D) // _ 00136 #define C7_DSH (S7_F) // ` 00137 00138 //97 0x61 Lower case alphabet 00139 #define C7_a C7_A 00140 #define C7_b C7_B 00141 #define C7_c C7_C 00142 #define C7_d C7_D 00143 #define C7_e C7_E 00144 #define C7_f C7_H 00145 00146 #define C7_g C7_G 00147 #define C7_h C7_H 00148 #define C7_i C7_I 00149 #define C7_j C7_J 00150 #define C7_k C7_K 00151 #define C7_l C7_L 00152 #define C7_m C7_M 00153 //#define C7_n C7_N 00154 #define C7_n (S7_C | S7_E | S7_G) 00155 //#define C7_o C7_O 00156 #define C7_o (S7_C | S7_D | S7_E | S7_G) 00157 #define C7_p C7_P 00158 #define C7_q C7_Q 00159 //#define C7_r C7_R 00160 #define C7_r (S7_E | S7_G) 00161 #define C7_s C7_S 00162 #define C7_t C7_T 00163 #define C7_u C7_U 00164 #define C7_v C7_V 00165 #define C7_w C7_W 00166 #define C7_x C7_X 00167 #define C7_y C7_Y 00168 #define C7_z C7_Z 00169 00170 //123 0x7B 00171 #define C7_CBL (S7_A | S7_D | S7_E | S7_F) // { 00172 #define C7_OR (S7_B | S7_C) // | 00173 #define C7_CBR (S7_A | S7_B | S7_C | S7_D) // } 00174 #define C7_TLD (S7_B | S7_E | S7_G ) // ~ 00175 #define C7_DEL (0x0000) 00176 00177 00178 //User Defined Characters (some examples) 00179 #define C7_DGR (S7_A | S7_B | S7_F | S7_G) //Degrees 00180 00181 // Font data selection for transmission to TM1637 memory 00182 #define LO(x) ( x & 0xFF) 00183 #define HI(x) ((x >> 8) & 0xFF) 00184 00185 00186 // ASCII Font definition table 00187 // 00188 #define FONT_7S_START 0x20 00189 #define FONT_7S_END 0x7F 00190 //#define FONT_7S_NR_CHARS (FONT_7S_END - FONT_7S_START + 1) 00191 extern const char FONT_7S[]; 00192 00193 #endif
Generated on Sat Jul 16 2022 20:44:27 by
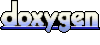