Added methods and features
Fork of SPI_TFT_ILI9341 by
Embed:
(wiki syntax)
Show/hide line numbers
SPI_TFT_ILI9341.h
00001 /* mbed library for 240*320 pixel display TFT based on ILI9341 LCD Controller 00002 * Copyright (c) 2013 Peter Drescher - DC2PD 00003 * 00004 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00005 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00006 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00007 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00008 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00009 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00010 * THE SOFTWARE. 00011 */ 00012 00013 // PD Change the char position handling, use pixel (x,y) instead of (colum, row) 00014 // 30.03.14 WH Added some methods & defines, Fixed typos & warnings, General define for SPI_16 selection 00015 // 00016 00017 #ifndef MBED_SPI_TFT_ILI9341_H 00018 #define MBED_SPI_TFT_ILI9341_H 00019 00020 #include "mbed.h" 00021 #include "GraphicsDisplay.h" 00022 00023 /* Enable or disable 16 bit SPI communication */ 00024 #if defined TARGET_KL25Z 00025 //Always disable for KL25Z since it does not support 16 bit SPI. 00026 #define SPI_16 0 00027 #else 00028 //Disable anyhow 00029 //#define SPI_16 0 00030 00031 //Enable 16 bit SPI. Does improve performance for fill operations. 00032 #define SPI_16 1 00033 #endif 00034 00035 /*Enable characters with transparant background color */ 00036 #define TRANSPARANCY 1 00037 00038 /* Default Display Dimensions */ 00039 #define TFT_WIDTH 240 00040 #define TFT_HEIGHT 320 00041 /* Default Bits per pixel */ 00042 #define TFT_BPP 16 00043 00044 /** @def Compute RGB color in 565 format */ 00045 #define RGB(r,g,b) (((r&0xF8)<<8)|((g&0xFC)<<3)|((b&0xF8)>>3)) //5 red | 6 green | 5 blue 00046 00047 /** @def swap(type, a, b) 00048 * @brief Convenience macro to swap two values. 00049 */ 00050 #define swap(type, a, b) { type tmp = ( a ); ( a ) = ( b ); ( b ) = tmp; } 00051 00052 //#define POLY_Y(Z) ((int32_t)((Points + Z)->X)) 00053 //#define POLY_X(Z) ((int32_t)((Points + Z)->Y)) 00054 // 00055 //#define max(a,b) (((a)>(b))?(a):(b)) 00056 //#define min(a,b) (((a)<(b))?(a):(b)) 00057 //#define ABS(X) ((X) > 0 ? (X) : -(X)) 00058 00059 /* Some RGB color definitions in 565 format and equivalent 888 format */ 00060 #define Black 0x0000 /* 0, 0, 0 */ 00061 #define Navy 0x000F /* 0, 0, 128 */ 00062 #define DarkGreen 0x03E0 /* 0, 128, 0 */ 00063 #define DarkCyan 0x03EF /* 0, 128, 128 */ 00064 #define Maroon 0x7800 /* 128, 0, 0 */ 00065 #define Purple 0x780F /* 128, 0, 128 */ 00066 #define Olive 0x7BE0 /* 128, 128, 0 */ 00067 #define LightGrey 0xC618 /* 192, 192, 192 */ 00068 #define Grey 0xF7DE 00069 #define DarkGrey 0x7BEF /* 128, 128, 128 */ 00070 #define Blue 0x001F /* 0, 0, 255 */ 00071 #define Blue2 0x051F 00072 #define Green 0x07E0 /* 0, 255, 0 */ 00073 #define Cyan 0x07FF /* 0, 255, 255 */ 00074 #define Cyan2 0x7FFF 00075 #define Red 0xF800 /* 255, 0, 0 */ 00076 #define Magenta 0xF81F /* 255, 0, 255 */ 00077 #define Yellow 0xFFE0 /* 255, 255, 0 */ 00078 #define White 0xFFFF /* 255, 255, 255 */ 00079 #define Orange 0xFD20 /* 255, 165, 0 */ 00080 #define Orange2 0x051F 00081 #define GreenYellow 0xAFE5 /* 173, 255, 47 */ 00082 00083 00084 /** 00085 * @brief ILI9341 Registers 00086 */ 00087 #define ILI9341_DISPLAY_RST 0x01 /* SW reset */ 00088 #define READ_DISPLAY_PIXEL_FORMAT 0x0C 00089 00090 #define ILI9341_SLEEP_OUT 0x11 /* Sleep out register */ 00091 #define ILI9341_PARTIAL_MODE 0x12 /* Partial Mode register */ 00092 #define ILI9341_NORMAL_MODE 0x13 /* Normal Mode register */ 00093 #define ILI9341_DISPLAY_INVERT_OFF 0x21 /* Display inversion off register */ 00094 #define ILI9341_DISPLAY_INVERT_ON 0x22 /* Display inversion on register */ 00095 #define ILI9341_GAMMA 0x26 /* Gamma register */ 00096 #define ILI9341_DISPLAY_OFF 0x28 /* Display off register */ 00097 #define ILI9341_DISPLAY_ON 0x29 /* Display on register */ 00098 #define ILI9341_COLUMN_ADDR 0x2A /* Colomn address register */ 00099 #define ILI9341_PAGE_ADDR 0x2B /* Page address register */ 00100 #define ILI9341_GRAM 0x2C /* GRAM register */ 00101 #define READ_MEMORY 0x2E 00102 // 00103 // 00104 #define ILI9341_TEAR_OFF 0x34 /* tearing effect off */ 00105 #define ILI9341_TEAR_ON 0x35 /* tearing effect on */ 00106 #define ILI9341_MAC 0x36 /* Memory Access Control register*/ 00107 #define ILI9341_PIXEL_FORMAT 0x3A /* Pixel Format register */ 00108 #define READ_MEMORY_CONTINUE 0x3E 00109 00110 #define ILI9341_WDB 0x51 /* Write Brightness Display register */ 00111 #define ILI9341_WCD 0x53 /* Write Control Display register*/ 00112 #define ILI9341_RGB_INTERFACE 0xB0 /* RGB Interface Signal Control */ 00113 #define ILI9341_FRC 0xB1 /* Frame Rate Control register */ 00114 #define ILI9341_BPC 0xB5 /* Blanking Porch Control register*/ 00115 #define ILI9341_DFC 0xB6 /* Display Function Control register*/ 00116 #define ILI9341_ENTRY_MODE 0xB7 /* Display Entry mode register*/ 00117 // 00118 #define ILI9341_POWER1 0xC0 /* Power Control 1 register */ 00119 #define ILI9341_POWER2 0xC1 /* Power Control 2 register */ 00120 #define ILI9341_VCOM1 0xC5 /* VCOM Control 1 register */ 00121 #define ILI9341_VCOM2 0xC7 /* VCOM Control 2 register */ 00122 #define ILI9341_POWERA 0xCB /* Power control A register */ 00123 #define ILI9341_POWERB 0xCF /* Power control B register */ 00124 #define ILI9341_PGAMMA 0xE0 /* Positive Gamma Correction register*/ 00125 #define ILI9341_NGAMMA 0xE1 /* Negative Gamma Correction register*/ 00126 #define ILI9341_DTCA 0xE8 /* Driver timing control A */ 00127 #define ILI9341_DTCB 0xEA /* Driver timing control B */ 00128 #define ILI9341_POWER_SEQ 0xED /* Power on sequence register */ 00129 #define UNDOCUMENTED_0xEF 0xEF // !@#$ 00130 #define ILI9341_3GAMMA_EN 0xF2 /* 3 Gamma enable register */ 00131 #define ILI9341_INTERFACE 0xF6 /* Interface control register */ 00132 #define ILI9341_PRC 0xF7 /* Pump ratio control register */ 00133 00134 00135 /** Display control class, based on GraphicsDisplay and TextDisplay 00136 * 00137 * Example: 00138 * @code 00139 * #include "stdio.h" 00140 * #include "mbed.h" 00141 * #include "SPI_TFT_ILI9341.h" 00142 * #include "string" 00143 * #include "Arial12x12.h" 00144 * #include "Arial24x23.h" 00145 * 00146 * 00147 * 00148 * // the TFT is connected to SPI pin 5-7 and IO's 8-10 00149 * SPI_TFT_ILI9341 TFT(p5, p6, p7, p8, p9, p10,"TFT"); // mosi, miso, sclk, cs, reset, dc 00150 * If your display need a signal for switch the backlight use a aditional IO pin in your program 00151 * 00152 * int main() { 00153 * TFT.claim(stdout); // send stdout to the TFT display 00154 * //TFT.claim(stderr); // send stderr to the TFT display 00155 * 00156 * TFT.background(Black); // set background to black 00157 * TFT.foreground(White); // set chars to white 00158 * TFT.cls(); // clear the screen 00159 * TFT.set_font((unsigned char*) Arial12x12); // select the font 00160 * 00161 * TFT.set_orientation(0); 00162 * printf(" Hello Mbed 0"); 00163 * TFT.set_font((unsigned char*) Arial24x23); // select font 2 00164 * TFT.locate(48,115); 00165 * TFT.printf("Bigger Font"); 00166 * } 00167 * @endcode 00168 */ 00169 class SPI_TFT_ILI9341 : public GraphicsDisplay { 00170 public: 00171 00172 /** Display origin */ 00173 enum Origin { 00174 Origin_LeftTop=0, /**< Left Top of panel is origin */ 00175 Origin_RightTop, /**< Right Top of panel is origin */ 00176 Origin_RightBot, /**< Right Bottom of panel is origin */ 00177 Origin_LeftBot /**< Left Bottom panel is origin */ 00178 }; 00179 00180 /** Create a SPI_TFT object connected to SPI and three pins 00181 * 00182 * @param mosi pin connected to SDO of display 00183 * @param miso pin connected to SDI of display 00184 * @param sclk pin connected to RS of display 00185 * @param cs pin connected to CS of display 00186 * @param reset pin connected to RESET of display (may be NC) 00187 * @param dc pin connected to WR of display 00188 * The IM pins have to be set to 1110 (3-0). Note: the M24SR board uses 0110 which also works. 00189 */ 00190 SPI_TFT_ILI9341(PinName mosi, PinName miso, PinName sclk, PinName cs, PinName reset, PinName dc, const char* name ="TFT"); 00191 00192 00193 /** Destructor for SPI_TFT object 00194 * @param none 00195 * @return none 00196 */ 00197 virtual ~SPI_TFT_ILI9341(); 00198 00199 00200 /** Get the width of the screen in pixels 00201 * 00202 * @returns width of screen in pixels 00203 * 00204 */ 00205 virtual int width(); 00206 00207 /** Get the height of the screen in pixels 00208 * 00209 * @returns height of screen in pixels 00210 * 00211 */ 00212 virtual int height(); 00213 00214 /** Draw a pixel at x,y with color 00215 * 00216 * @param x horizontal position 00217 * @param y vertical position 00218 * @param color 16 bit pixel color 00219 */ 00220 virtual void pixel(int x, int y, int colour); 00221 00222 /** draw a circle 00223 * 00224 * @param x0,y0 center 00225 * @param r radius 00226 * @param color 16 bit color * 00227 * 00228 */ 00229 void circle(int x, int y, int r, int colour); 00230 00231 /** draw a filled circle 00232 * 00233 * @param x0,y0 center 00234 * @param r radius 00235 * @param color 16 bit color * 00236 */ 00237 void fillcircle(int x, int y, int r, int colour); 00238 00239 00240 /** draw an oval 00241 * 00242 * @param x,y center 00243 * @param b radius 00244 * @param aspect hor/ver ratio ( hor. oval < 1.0; circle = 1.0; vert. oval > 1.0 ) 00245 * @param color 16 bit color * 00246 * 00247 */ 00248 void oval(int x, int y, int b, int color, float aspect=1.0); 00249 00250 00251 /** draw a filled oval 00252 * 00253 * @param x,y center 00254 * @param b radius 00255 * @param aspect hor/ver ratio ( hor. oval < 1.0; circle = 1.0; vert. oval > 1.0 ) 00256 * @param color 16 bit color * 00257 * 00258 */ 00259 void filloval(int x, int y, int b, int color, float aspect=1.0); 00260 00261 /** draw a 1 pixel line 00262 * 00263 * @param x0,y0 start point 00264 * @param x1,y1 stop point 00265 * @param color 16 bit color 00266 * 00267 */ 00268 void line(int x0, int y0, int x1, int y1, int colour); 00269 00270 /** draw a rect 00271 * 00272 * @param x0,y0 top left corner 00273 * @param x1,y1 down right corner 00274 * @param color 16 bit color 00275 * * 00276 */ 00277 void rect(int x0, int y0, int x1, int y1, int colour); 00278 00279 /** draw a filled rect 00280 * 00281 * @param x0,y0 top left corner 00282 * @param x1,y1 down right corner 00283 * @param color 16 bit color 00284 * 00285 */ 00286 void fillrect(int x0, int y0, int x1, int y1, int colour); 00287 00288 00289 00290 /** draw a rounded rect 00291 * 00292 * @param x0,y0 top left corner 00293 * @param x1,y1 down right corner 00294 * @param color 16 bit color 00295 * * 00296 */ 00297 void roundrect( int x0, int y0, int x1, int y1, int color ); 00298 00299 00300 /** draw a filled rounded rect 00301 * 00302 * @param x0,y0 top left corner 00303 * @param x1,y1 down right corner 00304 * @param color 16 bit color 00305 * 00306 */ 00307 void fillroundrect( int x0, int y0, int x1, int y1, int color ); 00308 00309 /** setup cursor position 00310 * 00311 * @param x x-position (top left) 00312 * @param y y-position 00313 */ 00314 virtual void locate(int x, int y); 00315 00316 /** Fill the screen with _background color 00317 * @param none 00318 * @return none 00319 */ 00320 virtual void cls(); 00321 00322 00323 /** Fill the screen with _background color 00324 * @param none 00325 * @return none 00326 */ 00327 virtual void newcls(); 00328 00329 00330 00331 /** calculate the max number of char in a line 00332 * 00333 * @returns max columns 00334 * depends on actual font size 00335 * 00336 */ 00337 virtual int columns(void); 00338 00339 /** calculate the max number of rows 00340 * 00341 * @returns max row 00342 * depends on actual font size 00343 * 00344 */ 00345 virtual int rows(void); 00346 00347 /** put a char on the screen 00348 * 00349 * @param value char to print 00350 * @returns printed char 00351 * 00352 */ 00353 virtual int _putc(int value); 00354 00355 /** draw a character on given position out of the active font to the TFT 00356 * 00357 * @param x x-position of char (top left) 00358 * @param y y-position 00359 * @param c char to print 00360 * 00361 */ 00362 virtual void character(int x, int y, int c); 00363 00364 /** paint a bitmap on the TFT 00365 * 00366 * @param x,y : upper left corner 00367 * @param w width of bitmap 00368 * @param h height of bitmap 00369 * @param *bitmap pointer to the bitmap data 00370 * 00371 * bitmap format: 16 bit R5 G6 B5 00372 * 00373 * use Gimp to create / load , save as BMP, option 16 bit R5 G6 B5 00374 * use winhex to load this file and mark data stating at offset 0x46 to end 00375 * use edit -> copy block -> C Source to export C array 00376 * paste this array into your program 00377 * 00378 * define the array as static const unsigned char to put it into flash memory 00379 * cast the pointer to (unsigned char *) : 00380 * tft.Bitmap(10,40,309,50,(unsigned char *)scala); 00381 */ 00382 void Bitmap(unsigned int x, unsigned int y, unsigned int w, unsigned int h, unsigned char *bitmap); 00383 00384 00385 /** paint a 16 bit BMP from filesytem on the TFT (slow) 00386 * 00387 * @param x,y : position of upper left corner 00388 * @param *Name_BMP name of the BMP file with drive: "/local/test.bmp" 00389 * 00390 * @returns 1 if bmp file was found and painted 00391 * @returns 0 if bmp file was found not found 00392 * @returns -1 if file is no bmp 00393 * @returns -2 if bmp file is no 16 bit bmp 00394 * @returns -3 if bmp file is to big for screen 00395 * @returns -4 if buffer malloc go wrong 00396 * 00397 * bitmap format: 16 bit R5 G6 B5 00398 * 00399 * use Gimp to create / load , save as BMP, option 16 bit R5 G6 B5 00400 * copy to internal file system or SD card 00401 */ 00402 00403 int BMP_16(unsigned int x, unsigned int y, const char *Name_BMP); 00404 00405 00406 00407 /******************************************************************************* 00408 * Function Name : WriteBMP_FAT 00409 * @brief Displays a bitmap picture loaded in Flash. 00410 * @param Xpos: specifies the X position. 00411 * @param Ypos: specifies the Y position. 00412 * @param BmpAddress: Bmp picture address in Flash. 00413 * @return None 00414 *******************************************************************************/ 00415 void WriteBMP_FAT(uint16_t Xpos, uint16_t Ypos, const char* BmpName); 00416 00417 00418 /** select the font to use 00419 * 00420 * @param f pointer to font array 00421 * 00422 * font array can created with GLCD Font Creator from http://www.mikroe.com 00423 * you have to add 4 parameter at the beginning of the font array to use: 00424 * - the number of byte / char 00425 * - the vertial size in pixel 00426 * - the horizontal size in pixel 00427 * - the number of byte per vertical line 00428 * you also have to change the array to char[] 00429 * 00430 */ 00431 void set_font(unsigned char* f); 00432 00433 /** Set the orientation of the screen 00434 * x,y: 0,0 is always top left 00435 * 00436 * @param o direction to use the screen (0-3) 00437 * 00438 */ 00439 //WH void set_orientation(unsigned int o); 00440 00441 00442 /** Set the origin of the screen 00443 * x,y: 0,0 is always top left 00444 * 00445 * @param origin top left corner of the screen 00446 * @return None 00447 */ 00448 void set_origin(Origin origin); 00449 00450 00451 /** Set background transparancy for characters, meaning that background pixels remain unchanged 00452 * 00453 * @param state transparancy on/off 00454 * @return None 00455 */ 00456 void set_transparancy(bool state); 00457 00458 /** Enable the ILI9341 display 00459 * 00460 * @param on: display On/Off 00461 * @return None 00462 */ 00463 void tft_on(bool on); 00464 00465 /** read out the manufacturer ID of the LCD 00466 * can used for checking the connection to the display 00467 * @returns ID 00468 */ 00469 int Read_ID(void); 00470 00471 00472 protected: 00473 00474 /** draw a horizontal line 00475 * 00476 * @param x0 horizontal start 00477 * @param x1 horizontal stop 00478 * @param y vertical position 00479 * @param color 16 bit color 00480 * @return None 00481 */ 00482 void hline(int x0, int x1, int y, int colour); 00483 00484 /** draw a vertical line 00485 * 00486 * @param x horizontal position 00487 * @param y0 vertical start 00488 * @param y1 vertical stop 00489 * @param color 16 bit color 00490 * @return None 00491 */ 00492 void vline(int x, int y0, int y1, int colour); 00493 00494 /** Set draw window region 00495 * 00496 * @param x horizontal position 00497 * @param y vertical position 00498 * @param w window width in pixel 00499 * @param h window height in pixels 00500 * @return None 00501 */ 00502 virtual void window (unsigned int x,unsigned int y, unsigned int w, unsigned int h); 00503 00504 /** Set draw window region to whole screen 00505 * 00506 * @return None 00507 */ 00508 void window_max (void); 00509 00510 00511 /** Init the ILI9341 controller 00512 * 00513 * @return None 00514 */ 00515 void tft_reset(); 00516 00517 00518 /** HW Reset to ILI9341 controller 00519 * @return None 00520 */ 00521 void _hwreset(); 00522 00523 00524 /** Write data to the LCD controller 00525 * 00526 * @param dat data written to LCD controller 00527 * @return None 00528 */ 00529 //void wr_dat(unsigned int value); 00530 void wr_dat(unsigned char value); 00531 00532 /** Write a command the LCD controller 00533 * 00534 * @param cmd: command to be written 00535 * @return None 00536 */ 00537 void wr_cmd(unsigned char value); 00538 00539 /** Read byte from the LCD controller 00540 * 00541 * @param cmd command to controller 00542 * @returns data from LCD controller 00543 */ 00544 char rd_byte(unsigned char cmd); 00545 00546 00547 /** Read int from the LCD controller 00548 * 00549 * @param cmd command to controller 00550 * @returns data from LCD controller 00551 */ 00552 int rd_32(unsigned char cmd); 00553 00554 00555 /** Write a value to the to a LCD register 00556 * 00557 * @param reg register to be written 00558 * @param val data to be written 00559 * @return None 00560 */ 00561 //void wr_reg (unsigned char reg, unsigned char val); 00562 00563 /** Read a LCD register 00564 * 00565 * @param reg register to be read 00566 * @returns value of the register 00567 */ 00568 //unsigned short rd_reg (unsigned char reg); 00569 00570 00571 SPI _spi; 00572 DigitalOut _cs; 00573 DigitalOut* _reset; 00574 DigitalOut _dc; 00575 00576 unsigned char* _font; 00577 unsigned int _char_x; 00578 unsigned int _char_y; 00579 bool _transparancy; 00580 00581 //WH unsigned int orientation; 00582 Origin _origin; 00583 00584 }; 00585 00586 #endif
Generated on Wed Jul 13 2022 09:36:56 by
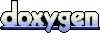