Drive thread library
Embed:
(wiki syntax)
Show/hide line numbers
drive.h
00001 #ifndef __DRIVE_INCLUDED__ 00002 #define __DRIVE_INCLUDED__ 00003 00004 #include "mbed.h" 00005 #include "pid_controller.h" 00006 00007 #define PWMPERIOD 4096 //PWM period in us 00008 00009 #define KP 3 00010 #define KI 0.1 00011 #define KD 0.1 00012 #define PIDSAMPLERATE 0.1 00013 00014 class Driver { 00015 private: 00016 PIDControl pidR; 00017 PIDControl pidL; 00018 00019 InterruptIn l_encoder1; //Left motor encoder signal 1 00020 InterruptIn l_encoder2; //Left motor encoder signal 2 00021 InterruptIn r_encoder1; //Right motor encoder signal 3 00022 InterruptIn r_encoder2; //Right motor encoder signal 4 00023 00024 PwmOut left_motor; // Forward Motor direction 00025 PwmOut left_motor_rev; // Reverse Motor direction 00026 PwmOut right_motor; // Forward Motor direction 00027 PwmOut right_motor_rev; // Reverse Motor direction 00028 00029 long lSpeedGoal;//Goal motor speed 00030 long rSpeedGoal; 00031 00032 long l_RPM;//Measured current speed 00033 long r_RPM; 00034 00035 Mutex lock; 00036 00037 Thread driver_thread; 00038 00039 unsigned int LoutA; //Holds left output A of encoder signal 00040 unsigned int LoutB; //Holds left output B of encoder signal 00041 unsigned int RoutA; //Holds Right output A of encoder signal 00042 unsigned int RoutB; //Holds Right output B of encoder signal 00043 00044 unsigned char l_enc_val; //Sequence that holds current and previous encoder combination values 00045 unsigned char r_enc_val; 00046 char l_enc_direction; 00047 char r_enc_direction; 00048 volatile long l_enc_count; //Holds current direction of left motor 00049 volatile long r_enc_count; //Holds current direction of right motor 00050 00051 //Ticker timer; 00052 volatile long l_old_enc_count; //Hold old encounter value for computation 00053 volatile long r_old_enc_count; 00054 00055 void l_encode_trig();//function for handling encoder trigger 00056 void r_encode_trig(); 00057 00058 void run();//function to run in thread (contains infinite loop) 00059 00060 00061 DigitalOut my_led; 00062 00063 public: 00064 Driver(osPriority, int); 00065 void start(); //starts thread "pid" running run() 00066 //void setVelocity(int speed, char* directoin);//called to set desired speed 00067 void setVelocity(int lSpeed, int rSpeed); 00068 }; 00069 00070 #endif
Generated on Fri Jul 15 2022 22:09:10 by
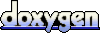