This library is used to make HTTP and HTTPS calls from mbed OS 5 applications.
Fork of mbed-http by
http_request_parser.h
00001 /* 00002 * PackageLicenseDeclared: Apache-2.0 00003 * Copyright (c) 2017 ARM Limited 00004 * 00005 * Licensed under the Apache License, Version 2.0 (the "License"); 00006 * you may not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * http://www.apache.org/licenses/LICENSE-2.0 00010 * 00011 * Unless required by applicable law or agreed to in writing, software 00012 * distributed under the License is distributed on an "AS IS" BASIS, 00013 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 * See the License for the specific language governing permissions and 00015 * limitations under the License. 00016 */ 00017 00018 #ifndef _HTTP_RESPONSE_PARSER_H_ 00019 #define _HTTP_RESPONSE_PARSER_H_ 00020 00021 #include "http_parser.h" 00022 #include "http_response.h" 00023 00024 class HttpParser { 00025 public: 00026 00027 HttpParser(HttpResponse* a_response, http_parser_type parser_type, Callback<void(const char *at, size_t length)> a_body_callback = 0) 00028 : response(a_response), body_callback(a_body_callback) 00029 { 00030 settings = new http_parser_settings(); 00031 00032 settings->on_message_begin = &HttpParser::on_message_begin_callback; 00033 settings->on_url = &HttpParser::on_url_callback; 00034 settings->on_status = &HttpParser::on_status_callback; 00035 settings->on_header_field = &HttpParser::on_header_field_callback; 00036 settings->on_header_value = &HttpParser::on_header_value_callback; 00037 settings->on_headers_complete = &HttpParser::on_headers_complete_callback; 00038 settings->on_chunk_header = &HttpParser::on_chunk_header_callback; 00039 settings->on_chunk_complete = &HttpParser::on_chunk_complete_callback; 00040 settings->on_body = &HttpParser::on_body_callback; 00041 settings->on_message_complete = &HttpParser::on_message_complete_callback; 00042 00043 // Construct the http_parser object 00044 parser = new http_parser(); 00045 http_parser_init(parser, parser_type); 00046 parser->data = (void*)this; 00047 } 00048 00049 ~HttpParser() { 00050 if (parser) { 00051 delete parser; 00052 } 00053 if (settings) { 00054 delete settings; 00055 } 00056 } 00057 00058 size_t execute(const char* buffer, size_t buffer_size) { 00059 return http_parser_execute(parser, settings, buffer, buffer_size); 00060 } 00061 00062 void finish() { 00063 http_parser_execute(parser, settings, NULL, 0); 00064 } 00065 00066 private: 00067 // Member functions 00068 int on_message_begin(http_parser* parser) { 00069 return 0; 00070 } 00071 00072 int on_url(http_parser* parser, const char *at, size_t length) { 00073 string s(at, length); 00074 response->set_url(s); 00075 return 0; 00076 } 00077 00078 int on_status(http_parser* parser, const char *at, size_t length) { 00079 string s(at, length); 00080 response->set_status(parser->status_code, s); 00081 return 0; 00082 } 00083 00084 int on_header_field(http_parser* parser, const char *at, size_t length) { 00085 string s(at, length); 00086 response->set_header_field(s); 00087 return 0; 00088 } 00089 00090 int on_header_value(http_parser* parser, const char *at, size_t length) { 00091 string s(at, length); 00092 response->set_header_value(s); 00093 return 0; 00094 } 00095 00096 int on_headers_complete(http_parser* parser) { 00097 response->set_headers_complete(); 00098 response->set_method((http_method)parser->method); 00099 return 0; 00100 } 00101 00102 int on_body(http_parser* parser, const char *at, size_t length) { 00103 response->increase_body_length(length); 00104 00105 if (body_callback) { 00106 body_callback(at, length); 00107 return 0; 00108 } 00109 00110 response->set_body(at, length); 00111 return 0; 00112 } 00113 00114 int on_message_complete(http_parser* parser) { 00115 response->set_message_complete(); 00116 00117 return 0; 00118 } 00119 00120 int on_chunk_header(http_parser* parser) { 00121 response->set_chunked(); 00122 00123 return 0; 00124 } 00125 00126 int on_chunk_complete(http_parser* parser) { 00127 return 0; 00128 } 00129 00130 // Static http_parser callback functions 00131 static int on_message_begin_callback(http_parser* parser) { 00132 return ((HttpParser*)parser->data)->on_message_begin(parser); 00133 } 00134 00135 static int on_url_callback(http_parser* parser, const char *at, size_t length) { 00136 return ((HttpParser*)parser->data)->on_url(parser, at, length); 00137 } 00138 00139 static int on_status_callback(http_parser* parser, const char *at, size_t length) { 00140 return ((HttpParser*)parser->data)->on_status(parser, at, length); 00141 } 00142 00143 static int on_header_field_callback(http_parser* parser, const char *at, size_t length) { 00144 return ((HttpParser*)parser->data)->on_header_field(parser, at, length); 00145 } 00146 00147 static int on_header_value_callback(http_parser* parser, const char *at, size_t length) { 00148 return ((HttpParser*)parser->data)->on_header_value(parser, at, length); 00149 } 00150 00151 static int on_headers_complete_callback(http_parser* parser) { 00152 return ((HttpParser*)parser->data)->on_headers_complete(parser); 00153 } 00154 00155 static int on_body_callback(http_parser* parser, const char *at, size_t length) { 00156 return ((HttpParser*)parser->data)->on_body(parser, at, length); 00157 } 00158 00159 static int on_message_complete_callback(http_parser* parser) { 00160 return ((HttpParser*)parser->data)->on_message_complete(parser); 00161 } 00162 00163 static int on_chunk_header_callback(http_parser* parser) { 00164 return ((HttpParser*)parser->data)->on_chunk_header(parser); 00165 } 00166 00167 static int on_chunk_complete_callback(http_parser* parser) { 00168 return ((HttpParser*)parser->data)->on_chunk_complete(parser); 00169 } 00170 00171 HttpResponse* response; 00172 Callback<void(const char *at, size_t length)> body_callback; 00173 http_parser* parser; 00174 http_parser_settings* settings; 00175 }; 00176 00177 #endif // _HTTP_RESPONSE_PARSER_H_
Generated on Fri Jul 15 2022 18:07:13 by
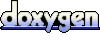