This library is used to make HTTP and HTTPS calls from mbed OS 5 applications.
Fork of mbed-http by
http_parsed_url.h
00001 /* 00002 * PackageLicenseDeclared: Apache-2.0 00003 * Copyright (c) 2017 ARM Limited 00004 * 00005 * Licensed under the Apache License, Version 2.0 (the "License"); 00006 * you may not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * http://www.apache.org/licenses/LICENSE-2.0 00010 * 00011 * Unless required by applicable law or agreed to in writing, software 00012 * distributed under the License is distributed on an "AS IS" BASIS, 00013 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 * See the License for the specific language governing permissions and 00015 * limitations under the License. 00016 */ 00017 00018 #ifndef _MBED_HTTP_PARSED_URL_H_ 00019 #define _MBED_HTTP_PARSED_URL_H_ 00020 00021 #include "http_parser.h" 00022 00023 class ParsedUrl { 00024 public: 00025 ParsedUrl(const char* url) { 00026 struct http_parser_url parsed_url; 00027 http_parser_parse_url(url, strlen(url), false, &parsed_url); 00028 00029 for (size_t ix = 0; ix < UF_MAX; ix++) { 00030 char* value; 00031 if (parsed_url.field_set & (1 << ix)) { 00032 value = (char*)calloc(parsed_url.field_data[ix].len + 1, 1); 00033 memcpy((void*)value, url + parsed_url.field_data[ix].off, 00034 parsed_url.field_data[ix].len); 00035 } 00036 else { 00037 value = (char*)calloc(1, 1); 00038 } 00039 00040 switch ((http_parser_url_fields)ix) { 00041 case UF_SCHEMA: _schema = value; break; 00042 case UF_HOST: _host = value; break; 00043 case UF_PATH: _path = value; break; 00044 case UF_QUERY: _query = value; break; 00045 case UF_USERINFO: _userinfo = value; break; 00046 default: 00047 // PORT is already parsed, FRAGMENT is not relevant for HTTP requests 00048 free((void*)value); 00049 break; 00050 } 00051 } 00052 00053 _port = parsed_url.port; 00054 if (!_port) { 00055 if (strcmp(_schema, "https") == 0) { 00056 _port = 443; 00057 } 00058 else { 00059 _port = 80; 00060 } 00061 } 00062 00063 if (strcmp(_path, "") == 0) { 00064 _path = (char*)calloc(2, 1); 00065 _path[0] = '/'; 00066 } 00067 } 00068 00069 ~ParsedUrl() { 00070 if (_schema) free((void*)_schema); 00071 if (_host) free((void*)_host); 00072 if (_path) free((void*)_path); 00073 if (_query) free((void*)_query); 00074 if (_userinfo) free((void*)_userinfo); 00075 } 00076 00077 uint16_t port() const { return _port; } 00078 char* schema() const { return _schema; } 00079 char* host() const { return _host; } 00080 char* path() const { return _path; } 00081 char* query() const { return _query; } 00082 char* userinfo() const { return _userinfo; } 00083 00084 private: 00085 uint16_t _port; 00086 char* _schema; 00087 char* _host; 00088 char* _path; 00089 char* _query; 00090 char* _userinfo; 00091 }; 00092 00093 #endif // _MBED_HTTP_PARSED_URL_H_
Generated on Fri Jul 15 2022 18:07:13 by
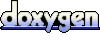