
Test program running on MAX32625MBED. Control through USB Serial commands using a terminal emulator such as teraterm or putty.
Dependencies: MaximTinyTester CmdLine MAX541 USBDevice
Test_Main_MAX11043.cpp
00001 // /******************************************************************************* 00002 // * Copyright (C) 2020 Maxim Integrated Products, Inc., All Rights Reserved. 00003 // * 00004 // * Permission is hereby granted, free of charge, to any person obtaining a 00005 // * copy of this software and associated documentation files (the "Software"), 00006 // * to deal in the Software without restriction, including without limitation 00007 // * the rights to use, copy, modify, merge, publish, distribute, sublicense, 00008 // * and/or sell copies of the Software, and to permit persons to whom the 00009 // * Software is furnished to do so, subject to the following conditions: 00010 // * 00011 // * The above copyright notice and this permission notice shall be included 00012 // * in all copies or substantial portions of the Software. 00013 // * 00014 // * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00015 // * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00016 // * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00017 // * IN NO EVENT SHALL MAXIM INTEGRATED BE LIABLE FOR ANY CLAIM, DAMAGES 00018 // * OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00019 // * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00020 // * OTHER DEALINGS IN THE SOFTWARE. 00021 // * 00022 // * Except as contained in this notice, the name of Maxim Integrated 00023 // * Products, Inc. shall not be used except as stated in the Maxim Integrated 00024 // * Products, Inc. Branding Policy. 00025 // * 00026 // * The mere transfer of this software does not imply any licenses 00027 // * of trade secrets, proprietary technology, copyrights, patents, 00028 // * trademarks, maskwork rights, or any other form of intellectual 00029 // * property whatsoever. Maxim Integrated Products, Inc. retains all 00030 // * ownership rights. 00031 // ******************************************************************************* 00032 // */ 00033 //---------- CODE GENERATOR: testMainCppCodeList 00034 // CODE GENERATOR: example code includes 00035 00036 // example code includes 00037 // standard include for target platform -- Platform_Include_Boilerplate 00038 #include "mbed.h" 00039 // Platforms: 00040 // - MAX32625MBED 00041 // - supports mbed-os-5.11, requires USBDevice library 00042 // - add https://developer.mbed.org/teams/MaximIntegrated/code/USBDevice/ 00043 // - remove max32630fthr library (if present) 00044 // - remove MAX32620FTHR library (if present) 00045 // - MAX32600MBED 00046 // - remove max32630fthr library (if present) 00047 // - remove MAX32620FTHR library (if present) 00048 // - Windows 10 note: Don't connect HDK until you are ready to load new firmware into the board. 00049 // - NUCLEO_F446RE 00050 // - remove USBDevice library 00051 // - remove max32630fthr library (if present) 00052 // - remove MAX32620FTHR library (if present) 00053 // - NUCLEO_F401RE 00054 // - remove USBDevice library 00055 // - remove max32630fthr library (if present) 00056 // - remove MAX32620FTHR library (if present) 00057 // - MAX32630FTHR 00058 // - #include "max32630fthr.h" 00059 // - add http://os.mbed.org/teams/MaximIntegrated/code/max32630fthr/ 00060 // - remove MAX32620FTHR library (if present) 00061 // - MAX32620FTHR 00062 // - #include "MAX32620FTHR.h" 00063 // - remove max32630fthr library (if present) 00064 // - add https://os.mbed.com/teams/MaximIntegrated/code/MAX32620FTHR/ 00065 // - not tested yet 00066 // - MAX32625PICO 00067 // - remove max32630fthr library (if present) 00068 // - remove MAX32620FTHR library (if present) 00069 // - not tested yet 00070 // 00071 // end Platform_Include_Boilerplate 00072 #include "MAX11043.h" 00073 #include "CmdLine.h" 00074 #include "MaximTinyTester.h" 00075 00076 // optional: serial port 00077 // note: some platforms such as Nucleo-F446RE do not support the USBSerial library. 00078 // In those cases, remove the USBDevice lib from the project and rebuild. 00079 #if defined(TARGET_MAX32625MBED) 00080 #include "USBSerial.h" 00081 USBSerial serial; // virtual serial port over USB (DEV connector) 00082 #elif defined(TARGET_MAX32630MBED) 00083 #include "USBSerial.h" 00084 USBSerial serial; // virtual serial port over USB (DEV connector) 00085 #else 00086 //#include "USBSerial.h" 00087 Serial serial(USBTX, USBRX); // tx, rx 00088 #endif 00089 00090 void on_immediate_0x21(); // Unicode (U+0021) ! EXCLAMATION MARK 00091 void on_immediate_0x7b(); // Unicode (U+007B) { LEFT CURLY BRACKET 00092 void on_immediate_0x7d(); // Unicode (U+007D) } RIGHT CURLY BRACKET 00093 00094 #include "CmdLine.h" 00095 00096 # if HAS_DAPLINK_SERIAL 00097 CmdLine cmdLine_DAPLINKserial(DAPLINKserial, "DAPLINK"); 00098 # endif // HAS_DAPLINK_SERIAL 00099 CmdLine cmdLine_serial(serial, "serial"); 00100 00101 00102 //-------------------------------------------------- 00103 00104 00105 #if defined(TARGET) 00106 // TARGET_NAME macros from targets/TARGET_Maxim/TARGET_MAX32625/device/mxc_device.h 00107 // Create a string definition for the TARGET 00108 #define STRING_ARG(arg) #arg 00109 #define STRING_NAME(name) STRING_ARG(name) 00110 #define TARGET_NAME STRING_NAME(TARGET) 00111 #elif defined(TARGET_MAX32600) 00112 #define TARGET_NAME "MAX32600" 00113 #elif defined(TARGET_LPC1768) 00114 #define TARGET_NAME "LPC1768" 00115 #elif defined(TARGET_NUCLEO_F446RE) 00116 #define TARGET_NAME "NUCLEO_F446RE" 00117 #elif defined(TARGET_NUCLEO_F401RE) 00118 #define TARGET_NAME "NUCLEO_F401RE" 00119 #else 00120 #error TARGET NOT DEFINED 00121 #endif 00122 #if defined(TARGET_MAX32630) 00123 //-------------------------------------------------- 00124 // TARGET=MAX32630FTHR ARM Cortex-M4F 96MHz 2048kB Flash 512kB SRAM 00125 // +-------------[microUSB]-------------+ 00126 // | J1 MAX32630FTHR J2 | 00127 // ______ | [ ] RST GND [ ] | 00128 // ______ | [ ] 3V3 BAT+[ ] | 00129 // ______ | [ ] 1V8 reset SW1 | 00130 // ______ | [ ] GND J4 J3 | 00131 // analogIn0/4 | [a] AIN_0 1.2Vfs (bat) SYS [ ] | switched BAT+ 00132 // analogIn1/5 | [a] AIN_1 1.2Vfs PWR [ ] | external pwr btn 00133 // analogIn2 | [a] AIN_2 1.2Vfs +5V VBUS [ ] | USB +5V power 00134 // analogIn3 | [a] AIN_3 1.2Vfs 1-WIRE P4_0 [d] | D0 dig9 00135 // (I2C2.SDA) | [d] P5_7 SDA2 SRN P5_6 [d] | D1 dig8 00136 // (I2C2.SCL) | [d] P6_0 SCL2 SDIO3 P5_5 [d] | D2 dig7 00137 // D13/SCLK | [s] P5_0 SCLK SDIO2 P5_4 [d] | D3 dig6 00138 // D11/MOSI | [s] P5_1 MOSI SSEL P5_3 [d] | D4 dig5 00139 // D12/MISO | [s] P5_2 MISO RTS P3_3 [d] | D5 dig4 00140 // D10/CS | [s] P3_0 RX CTS P3_2 [d] | D6 dig3 00141 // D9 dig0 | [d] P3_1 TX SCL P3_5 [d] | D7 dig2 00142 // ______ | [ ] GND SDA P3_4 [d] | D8 dig1 00143 // | | 00144 // | XIP Flash MAX14690N | 00145 // | XIP_SCLK P1_0 SDA2 P5_7 | 00146 // | XIP_MOSI P1_1 SCL2 P6_0 | 00147 // | XIP_MISO P1_2 PMIC_INIT P3_7 | 00148 // | XIP_SSEL P1_3 MPC P2_7 | 00149 // | XIP_DIO2 P1_4 MON AIN_0 | 00150 // | XIP_DIO3 P1_5 | 00151 // | | 00152 // | PAN1326B MicroSD LED | 00153 // | BT_RX P0_0 SD_SCLK P0_4 r P2_4 | 00154 // | BT_TX P0_1 SD_MOSI P0_5 g P2_5 | 00155 // | BT_CTS P0_2 SD_MISO P0_6 b P2_6 | 00156 // | BT_RTS P0_3 SD_SSEL P0_7 | 00157 // | BT_RST P1_6 DETECT P2_2 | 00158 // | BT_CLK P1_7 SW2 P2_3 | 00159 // +------------------------------------+ 00160 // MAX32630FTHR board has MAX14690 PMIC on I2C bus (P5_7 SDA, P6_0 SCL) at slave address 0101_000r 0x50 (or 0x28 for 7 MSbit address). 00161 // MAX32630FTHR board has BMI160 accelerometer on I2C bus (P5_7 SDA, P6_0 SCL) at slave address 1101_000r 0xD0 (or 0x68 for 7 MSbit address). 00162 // AIN_0 = AIN0 pin fullscale is 1.2V 00163 // AIN_1 = AIN1 pin fullscale is 1.2V 00164 // AIN_2 = AIN2 pin fullscale is 1.2V 00165 // AIN_3 = AIN3 pin fullscale is 1.2V 00166 // AIN_4 = AIN0 / 5.0 fullscale is 6.0V 00167 // AIN_5 = AIN1 / 5.0 fullscale is 6.0V 00168 // AIN_6 = VDDB / 4.0 fullscale is 4.8V 00169 // AIN_7 = VDD18 fullscale is 1.2V 00170 // AIN_8 = VDD12 fullscale is 1.2V 00171 // AIN_9 = VRTC / 2.0 fullscale is 2.4V 00172 // AIN_10 = x undefined? 00173 // AIN_11 = VDDIO / 4.0 fullscale is 4.8V 00174 // AIN_12 = VDDIOH / 4.0 fullscale is 4.8V 00175 // 00176 #include "max32630fthr.h" 00177 MAX32630FTHR pegasus(MAX32630FTHR::VIO_3V3); 00178 #define analogIn4_IS_HIGH_RANGE_OF_analogIn0 1 00179 // MAX32630FTHR board supports only internal VREF = 1.200V at bypass capacitor C15 00180 const float ADC_FULL_SCALE_VOLTAGE = 1.200; 00181 // Arduino connector 00182 #ifndef A0 00183 #define A0 AIN_0 00184 #endif 00185 #ifndef A1 00186 #define A1 AIN_1 00187 #endif 00188 #ifndef A2 00189 #define A2 AIN_2 00190 #endif 00191 #ifndef A3 00192 #define A3 AIN_3 00193 #endif 00194 #ifndef D0 00195 #define D0 P4_0 00196 #endif 00197 #ifndef D1 00198 #define D1 P5_6 00199 #endif 00200 #ifndef D2 00201 #define D2 P5_5 00202 #endif 00203 #ifndef D3 00204 #define D3 P5_4 00205 #endif 00206 #ifndef D4 00207 #define D4 P5_3 00208 #endif 00209 #ifndef D5 00210 #define D5 P3_3 00211 #endif 00212 #ifndef D6 00213 #define D6 P3_2 00214 #endif 00215 #ifndef D7 00216 #define D7 P3_5 00217 #endif 00218 #ifndef D8 00219 #define D8 P3_4 00220 #endif 00221 #ifndef D9 00222 #define D9 P3_1 00223 #endif 00224 #ifndef D10 00225 #define D10 P3_0 00226 #endif 00227 #ifndef D11 00228 #define D11 P5_1 00229 #endif 00230 #ifndef D12 00231 #define D12 P5_2 00232 #endif 00233 #ifndef D13 00234 #define D13 P5_0 00235 #endif 00236 //-------------------------------------------------- 00237 #elif defined(TARGET_MAX32625MBED) 00238 //-------------------------------------------------- 00239 // TARGET=MAX32625MBED ARM Cortex-M4F 96MHz 512kB Flash 160kB SRAM 00240 // +-------------------------------------+ 00241 // | MAX32625MBED Arduino UNO header | 00242 // | | 00243 // | A5/SCL[ ] | P1_7 dig15 00244 // | A4/SDA[ ] | P1_6 dig14 00245 // | AREF=N/C[ ] | 00246 // | GND[ ] | 00247 // | [ ]N/C SCK/13[ ] | P1_0 dig13 00248 // | [ ]IOREF=3V3 MISO/12[ ] | P1_2 dig12 00249 // | [ ]RST MOSI/11[ ]~| P1_1 dig11 00250 // | [ ]3V3 CS/10[ ]~| P1_3 dig10 00251 // | [ ]5V0 9[ ]~| P1_5 dig9 00252 // | [ ]GND 8[ ] | P1_4 dig8 00253 // | [ ]GND | 00254 // | [ ]Vin 7[ ] | P0_7 dig7 00255 // | 6[ ]~| P0_6 dig6 00256 // AIN_0 | [ ]A0 5[ ]~| P0_5 dig5 00257 // AIN_1 | [ ]A1 4[ ] | P0_4 dig4 00258 // AIN_2 | [ ]A2 INT1/3[ ]~| P0_3 dig3 00259 // AIN_3 | [ ]A3 INT0/2[ ] | P0_2 dig2 00260 // dig16 P3_4 | [ ]A4/SDA RST SCK MISO TX>1[ ] | P0_1 dig1 00261 // dig17 P3_5 | [ ]A5/SCL [ ] [ ] [ ] RX<0[ ] | P0_0 dig0 00262 // | [ ] [ ] [ ] | 00263 // | UNO_R3 GND MOSI 5V ____________/ 00264 // \_______________________/ 00265 // 00266 // +------------------------+ 00267 // | | 00268 // | MicroSD LED | 00269 // | SD_SCLK P2_4 r P3_0 | 00270 // | SD_MOSI P2_5 g P3_1 | 00271 // | SD_MISO P2_6 b P3_2 | 00272 // | SD_SSEL P2_7 y P3_3 | 00273 // | | 00274 // | DAPLINK BUTTONS | 00275 // | TX P2_1 SW3 P2_3 | 00276 // | RX P2_0 SW2 P2_2 | 00277 // +------------------------+ 00278 // 00279 // AIN_0 = AIN0 pin fullscale is 1.2V 00280 // AIN_1 = AIN1 pin fullscale is 1.2V 00281 // AIN_2 = AIN2 pin fullscale is 1.2V 00282 // AIN_3 = AIN3 pin fullscale is 1.2V 00283 // AIN_4 = AIN0 / 5.0 fullscale is 6.0V 00284 // AIN_5 = AIN1 / 5.0 fullscale is 6.0V 00285 // AIN_6 = VDDB / 4.0 fullscale is 4.8V 00286 // AIN_7 = VDD18 fullscale is 1.2V 00287 // AIN_8 = VDD12 fullscale is 1.2V 00288 // AIN_9 = VRTC / 2.0 fullscale is 2.4V 00289 // AIN_10 = x undefined? 00290 // AIN_11 = VDDIO / 4.0 fullscale is 4.8V 00291 // AIN_12 = VDDIOH / 4.0 fullscale is 4.8V 00292 // 00293 //#include "max32625mbed.h" // ? 00294 //MAX32625MBED mbed(MAX32625MBED::VIO_3V3); // ? 00295 #define analogIn4_IS_HIGH_RANGE_OF_analogIn0 1 00296 // MAX32630FTHR board supports only internal VREF = 1.200V at bypass capacitor C15 00297 const float ADC_FULL_SCALE_VOLTAGE = 1.200; // TODO: ADC_FULL_SCALE_VOLTAGE Pico? 00298 // Arduino connector 00299 #ifndef A0 00300 #define A0 AIN_0 00301 #endif 00302 #ifndef A1 00303 #define A1 AIN_1 00304 #endif 00305 #ifndef A2 00306 #define A2 AIN_2 00307 #endif 00308 #ifndef A3 00309 #define A3 AIN_3 00310 #endif 00311 #ifndef D0 00312 #define D0 P0_0 00313 #endif 00314 #ifndef D1 00315 #define D1 P0_1 00316 #endif 00317 #ifndef D2 00318 #define D2 P0_2 00319 #endif 00320 #ifndef D3 00321 #define D3 P0_3 00322 #endif 00323 #ifndef D4 00324 #define D4 P0_4 00325 #endif 00326 #ifndef D5 00327 #define D5 P0_5 00328 #endif 00329 #ifndef D6 00330 #define D6 P0_6 00331 #endif 00332 #ifndef D7 00333 #define D7 P0_7 00334 #endif 00335 #ifndef D8 00336 #define D8 P1_4 00337 #endif 00338 #ifndef D9 00339 #define D9 P1_5 00340 #endif 00341 #ifndef D10 00342 #define D10 P1_3 00343 #endif 00344 #ifndef D11 00345 #define D11 P1_1 00346 #endif 00347 #ifndef D12 00348 #define D12 P1_2 00349 #endif 00350 #ifndef D13 00351 #define D13 P1_0 00352 #endif 00353 //-------------------------------------------------- 00354 #elif defined(TARGET_MAX32600) 00355 // target MAX32600 00356 // 00357 #define analogIn4_IS_HIGH_RANGE_OF_analogIn0 0 00358 const float ADC_FULL_SCALE_VOLTAGE = 1.500; 00359 // 00360 //-------------------------------------------------- 00361 #elif defined(TARGET_MAX32620FTHR) 00362 #warning "TARGET_MAX32620FTHR not previously tested; need to define pins..." 00363 #include "MAX32620FTHR.h" 00364 // Initialize I/O voltages on MAX32620FTHR board 00365 MAX32620FTHR fthr(MAX32620FTHR::VIO_3V3); 00366 //#define USE_LEDS 0 ? 00367 #define analogIn4_IS_HIGH_RANGE_OF_analogIn0 1 00368 #warning "TARGET_MAX32620FTHR not previously tested; need to verify ADC_FULL_SCALE_VOLTAGE..." 00369 const float ADC_FULL_SCALE_VOLTAGE = 1.200; 00370 // 00371 //-------------------------------------------------- 00372 #elif defined(TARGET_MAX32625PICO) 00373 #warning "TARGET_MAX32625PICO not previously tested; need to define pins..." 00374 //#define USE_LEDS 0 ? 00375 #define analogIn4_IS_HIGH_RANGE_OF_analogIn0 1 00376 #warning "TARGET_MAX32625PICO not previously tested; need to verify ADC_FULL_SCALE_VOLTAGE..." 00377 const float ADC_FULL_SCALE_VOLTAGE = 1.200; 00378 // 00379 //-------------------------------------------------- 00380 #elif defined(TARGET_NUCLEO_F446RE) || defined(TARGET_NUCLEO_F401RE) 00381 // TODO1: target NUCLEO_F446RE 00382 // 00383 // USER_BUTTON PC13 00384 // LED1 is shared with SPI_SCK on NUCLEO_F446RE PA_5, so don't use LED1. 00385 #define USE_LEDS 0 00386 // SPI spi(SPI_MOSI, SPI_MISO, SPI_SCK); 00387 // Serial serial(SERIAL_TX, SERIAL_RX); 00388 #define analogIn4_IS_HIGH_RANGE_OF_analogIn0 0 00389 const float ADC_FULL_SCALE_VOLTAGE = 3.300; // TODO: ADC_FULL_SCALE_VOLTAGE Pico? 00390 // 00391 //-------------------------------------------------- 00392 #elif defined(TARGET_LPC1768) 00393 //-------------------------------------------------- 00394 // TARGET=LPC1768 ARM Cortex-M3 100 MHz 512kB flash 64kB SRAM 00395 // +-------------[microUSB]-------------+ 00396 // ______ | [ ] GND +3.3V VOUT [ ] | ______ 00397 // ______ | [ ] 4.5V<VIN<9.0V +5.0V VU [ ] | ______ 00398 // ______ | [ ] VB USB.IF- [ ] | ______ 00399 // ______ | [ ] nR USB.IF+ [ ] | ______ 00400 // digitalInOut0 | [ ] p5 MOSI ETHERNET.RD- [ ] | ______ 00401 // digitalInOut1 | [ ] p6 MISO ETHERNET.RD+ [ ] | ______ 00402 // digitalInOut2 | [ ] p7 SCLK ETHERNET.TD- [ ] | ______ 00403 // digitalInOut3 | [ ] p8 ETHERNET.TD+ [ ] | ______ 00404 // digitalInOut4 | [ ] p9 TX SDA USB.D- [ ] | ______ 00405 // digitalInOut5 | [ ] p10 RX SCL USB.D+ [ ] | ______ 00406 // digitalInOut6 | [ ] p11 MOSI CAN-RD p30 [ ] | digitalInOut13 00407 // digitalInOut7 | [ ] p12 MISO CAN-TD p29 [ ] | digitalInOut12 00408 // digitalInOut8 | [ ] p13 TX SCLK SDA TX p28 [ ] | digitalInOut11 00409 // digitalInOut9 | [ ] p14 RX SCL RX p27 [ ] | digitalInOut10 00410 // analogIn0 | [ ] p15 AIN0 3.3Vfs PWM1 p26 [ ] | pwmDriver1 00411 // analogIn1 | [ ] p16 AIN1 3.3Vfs PWM2 p25 [ ] | pwmDriver2 00412 // analogIn2 | [ ] p17 AIN2 3.3Vfs PWM3 p24 [ ] | pwmDriver3 00413 // analogIn3 | [ ] p18 AIN3 AOUT PWM4 p23 [ ] | pwmDriver4 00414 // analogIn4 | [ ] p19 AIN4 3.3Vfs PWM5 p22 [ ] | pwmDriver5 00415 // analogIn5 | [ ] p20 AIN5 3.3Vfs PWM6 p21 [ ] | pwmDriver6 00416 // +------------------------------------+ 00417 // AIN6 = P0.3 = TGT_SBL_RXD? 00418 // AIN7 = P0.2 = TGT_SBL_TXD? 00419 // 00420 //-------------------------------------------------- 00421 // LPC1768 board uses VREF = 3.300V +A3,3V thru L1 to bypass capacitor C14 00422 #define analogIn4_IS_HIGH_RANGE_OF_analogIn0 0 00423 const float ADC_FULL_SCALE_VOLTAGE = 3.300; 00424 #else // not defined(TARGET_LPC1768 etc.) 00425 //-------------------------------------------------- 00426 // unknown target 00427 //-------------------------------------------------- 00428 #endif // target definition 00429 00430 00431 //-------------------------------------------------- 00432 // Option to dedicate SPI port pins 00433 // 00434 // SPI2_MOSI = P5_1 00435 // SPI2_MISO = P5_2 00436 // SPI2_SCK = P5_0 00437 // On this board I'm using P3_0 as spi_cs 00438 // SPI2_SS = P5_3 00439 // SPI2_SDIO2 = P5_4 00440 // SPI2_SDIO3 = P5_5 00441 // SPI2_SRN = P5_6 00442 // 00443 #ifndef HAS_SPI 00444 #define HAS_SPI 1 00445 #endif 00446 #if HAS_SPI 00447 #define SPI_MODE0 0 00448 #define SPI_MODE1 1 00449 #define SPI_MODE2 2 00450 #define SPI_MODE3 3 00451 // 00452 #if defined(TARGET_MAX32630) 00453 // Before setting global variables g_SPI_SCLK_Hz and g_SPI_dataMode, 00454 // workaround for TARGET_MAX32630 SPI_MODE2 SPI_MODE3 problem (issue #30) 00455 #warning "MAX32630 SPI workaround..." 00456 // replace SPI_MODE2 (CPOL=1,CPHA=0) with SPI_MODE1 (CPOL=0,CPHA=1) Falling Edge stable 00457 // replace SPI_MODE3 (CPOL=1,CPHA=1) with SPI_MODE0 (CPOL=0,CPHA=0) Rising Edge stable 00458 # if ((SPI_dataMode) == (SPI_MODE2)) 00459 #warning "MAX32630 SPI_MODE2 workaround, changing SPI_dataMode to SPI_MODE1..." 00460 // SPI_dataMode SPI_MODE2 // CPOL=1,CPHA=0: Falling Edge stable; SCLK idle High 00461 # undef SPI_dataMode 00462 # define SPI_dataMode SPI_MODE1 // CPOL=0,CPHA=1: Falling Edge stable; SCLK idle Low 00463 # elif ((SPI_dataMode) == (SPI_MODE3)) 00464 #warning "MAX32630 SPI_MODE3 workaround, changing SPI_dataMode to SPI_MODE0..." 00465 // SPI_dataMode SPI_MODE3 // CPOL=1,CPHA=1: Rising Edge stable; SCLK idle High 00466 # undef SPI_dataMode 00467 # define SPI_dataMode SPI_MODE0 // CPOL=0,CPHA=0: Rising Edge stable; SCLK idle Low 00468 # endif // workaround for TARGET_MAX32630 SPI_MODE2 SPI_MODE3 problem 00469 // workaround for TARGET_MAX32630 SPI_MODE2 SPI_MODE3 problem (issue #30) 00470 // limit SPI SCLK speed to 6MHz or less 00471 # if ((SPI_SCLK_Hz) > (6000000)) 00472 #warning "MAX32630 SPI speed workaround, changing SPI_SCLK_Hz to 6000000 or 6MHz..." 00473 # undef SPI_SCLK_Hz 00474 # define SPI_SCLK_Hz 6000000 // 6MHz 00475 # endif 00476 #endif 00477 // 00478 uint32_t g_SPI_SCLK_Hz = 24000000; // platform limit 24MHz intSPI_SCLK_Platform_Max_MHz * 1000000 00479 // TODO1: validate g_SPI_SCLK_Hz against system clock frequency SystemCoreClock F_CPU 00480 #if defined(TARGET_NUCLEO_F446RE) || defined(TARGET_NUCLEO_F401RE) 00481 // Nucleo SPI frequency isn't working quite as expected... 00482 // Looks like STMF4 has an spi clock prescaler (2,4,8,16,32,64,128,256) 00483 // so 180MHz->[90.0, 45.0, 22.5, 11.25, 5.625, 2.8125, 1.40625, 0.703125] 00484 // %SC SCLK=1MHz sets spi frequency 703.125kHz 00485 // %SC SCLK=2MHz sets spi frequency 1.40625MHz 00486 // %SC SCLK=3MHz sets spi frequency 2.8125MHz 00487 // %SC SCLK=6MHz sets spi frequency 5.625MHz 00488 // %SC SCLK=12MHz sets spi frequency 11.25MHz 00489 // %SC SCLK=23MHz sets spi frequency 22.5MHz 00490 // %SC SCLK=45MHz sets spi frequency 45.0MHz 00491 // Don't know why I can't reach spi frequency 90.0MHz, but ok whatever. 00492 const uint32_t limit_min_SPI_SCLK_divisor = 2; 00493 const uint32_t limit_max_SPI_SCLK_divisor = 256; 00494 // not really a divisor, just a powers-of-two prescaler with no intermediate divisors. 00495 #else 00496 const uint32_t limit_min_SPI_SCLK_divisor = 2; 00497 const uint32_t limit_max_SPI_SCLK_divisor = 8191; 00498 #endif 00499 const uint32_t limit_max_SPI_SCLK_Hz = (SystemCoreClock / limit_min_SPI_SCLK_divisor); // F_CPU / 2; // 8MHz / 2 = 4MHz 00500 const uint32_t limit_min_SPI_SCLK_Hz = (SystemCoreClock / limit_max_SPI_SCLK_divisor); // F_CPU / 128; // 8MHz / 128 = 62.5kHz 00501 // 00502 uint8_t g_SPI_dataMode = SPI_MODE0; // TODO: missing definition SPI_dataMode; 00503 uint8_t g_SPI_cs_state = 1; 00504 // 00505 #endif 00506 00507 00508 // uncrustify-0.66.1 *INDENT-OFF* 00509 //-------------------------------------------------- 00510 // Declare the DigitalInOut GPIO pins 00511 // Optional digitalInOut support. If there is only one it should be digitalInOut1. 00512 // D) Digital High/Low/Input Pin 00513 #if defined(TARGET_MAX32630) 00514 // +-------------[microUSB]-------------+ 00515 // | J1 MAX32630FTHR J2 | 00516 // | [ ] RST GND [ ] | 00517 // | [ ] 3V3 BAT+[ ] | 00518 // | [ ] 1V8 reset SW1 | 00519 // | [ ] GND J4 J3 | 00520 // | [ ] AIN_0 1.2Vfs (bat) SYS [ ] | 00521 // | [ ] AIN_1 1.2Vfs PWR [ ] | 00522 // | [ ] AIN_2 1.2Vfs +5V VBUS [ ] | 00523 // | [ ] AIN_3 1.2Vfs 1-WIRE P4_0 [ ] | dig9 00524 // dig10 | [x] P5_7 SDA2 SRN P5_6 [ ] | dig8 00525 // dig11 | [x] P6_0 SCL2 SDIO3 P5_5 [ ] | dig7 00526 // dig12 | [x] P5_0 SCLK SDIO2 P5_4 [ ] | dig6 00527 // dig13 | [x] P5_1 MOSI SSEL P5_3 [x] | dig5 00528 // dig14 | [ ] P5_2 MISO RTS P3_3 [ ] | dig4 00529 // dig15 | [ ] P3_0 RX CTS P3_2 [ ] | dig3 00530 // dig0 | [ ] P3_1 TX SCL P3_5 [x] | dig2 00531 // | [ ] GND SDA P3_4 [x] | dig1 00532 // +------------------------------------+ 00533 #define HAS_digitalInOut0 1 // P3_1 TARGET_MAX32630 J1.15 00534 #define HAS_digitalInOut1 1 // P3_4 TARGET_MAX32630 J3.12 00535 #define HAS_digitalInOut2 1 // P3_5 TARGET_MAX32630 J3.11 00536 #define HAS_digitalInOut3 1 // P3_2 TARGET_MAX32630 J3.10 00537 #define HAS_digitalInOut4 1 // P3_3 TARGET_MAX32630 J3.9 00538 #define HAS_digitalInOut5 1 // P5_3 TARGET_MAX32630 J3.8 00539 #define HAS_digitalInOut6 1 // P5_4 TARGET_MAX32630 J3.7 00540 #define HAS_digitalInOut7 1 // P5_5 TARGET_MAX32630 J3.6 00541 #define HAS_digitalInOut8 1 // P5_6 TARGET_MAX32630 J3.5 00542 #define HAS_digitalInOut9 1 // P4_0 TARGET_MAX32630 J3.4 00543 #if HAS_I2C 00544 // avoid resource conflict between P5_7, P6_0 I2C and DigitalInOut 00545 #define HAS_digitalInOut10 0 // P5_7 TARGET_MAX32630 J1.9 00546 #define HAS_digitalInOut11 0 // P6_0 TARGET_MAX32630 J1.10 00547 #else // HAS_I2C 00548 #define HAS_digitalInOut10 1 // P5_7 TARGET_MAX32630 J1.9 00549 #define HAS_digitalInOut11 1 // P6_0 TARGET_MAX32630 J1.10 00550 #endif // HAS_I2C 00551 #if HAS_SPI 00552 // avoid resource conflict between P5_0, P5_1, P5_2 SPI and DigitalInOut 00553 #define HAS_digitalInOut12 0 // P5_0 TARGET_MAX32630 J1.11 00554 #define HAS_digitalInOut13 0 // P5_1 TARGET_MAX32630 J1.12 00555 #define HAS_digitalInOut14 0 // P5_2 TARGET_MAX32630 J1.13 00556 #define HAS_digitalInOut15 0 // P3_0 TARGET_MAX32630 J1.14 00557 #else // HAS_SPI 00558 #define HAS_digitalInOut12 1 // P5_0 TARGET_MAX32630 J1.11 00559 #define HAS_digitalInOut13 1 // P5_1 TARGET_MAX32630 J1.12 00560 #define HAS_digitalInOut14 1 // P5_2 TARGET_MAX32630 J1.13 00561 #define HAS_digitalInOut15 1 // P3_0 TARGET_MAX32630 J1.14 00562 #endif // HAS_SPI 00563 #if HAS_digitalInOut0 00564 DigitalInOut digitalInOut0(P3_1, PIN_INPUT, PullUp, 1); // P3_1 TARGET_MAX32630 J1.15 00565 #endif 00566 #if HAS_digitalInOut1 00567 DigitalInOut digitalInOut1(P3_4, PIN_INPUT, PullUp, 1); // P3_4 TARGET_MAX32630 J3.12 00568 #endif 00569 #if HAS_digitalInOut2 00570 DigitalInOut digitalInOut2(P3_5, PIN_INPUT, PullUp, 1); // P3_5 TARGET_MAX32630 J3.11 00571 #endif 00572 #if HAS_digitalInOut3 00573 DigitalInOut digitalInOut3(P3_2, PIN_INPUT, PullUp, 1); // P3_2 TARGET_MAX32630 J3.10 00574 #endif 00575 #if HAS_digitalInOut4 00576 DigitalInOut digitalInOut4(P3_3, PIN_INPUT, PullUp, 1); // P3_3 TARGET_MAX32630 J3.9 00577 #endif 00578 #if HAS_digitalInOut5 00579 DigitalInOut digitalInOut5(P5_3, PIN_INPUT, PullUp, 1); // P5_3 TARGET_MAX32630 J3.8 00580 #endif 00581 #if HAS_digitalInOut6 00582 DigitalInOut digitalInOut6(P5_4, PIN_INPUT, PullUp, 1); // P5_4 TARGET_MAX32630 J3.7 00583 #endif 00584 #if HAS_digitalInOut7 00585 DigitalInOut digitalInOut7(P5_5, PIN_INPUT, PullUp, 1); // P5_5 TARGET_MAX32630 J3.6 00586 #endif 00587 #if HAS_digitalInOut8 00588 DigitalInOut digitalInOut8(P5_6, PIN_INPUT, PullUp, 1); // P5_6 TARGET_MAX32630 J3.5 00589 #endif 00590 #if HAS_digitalInOut9 00591 DigitalInOut digitalInOut9(P4_0, PIN_INPUT, PullUp, 1); // P4_0 TARGET_MAX32630 J3.4 00592 #endif 00593 #if HAS_digitalInOut10 00594 DigitalInOut digitalInOut10(P5_7, PIN_INPUT, PullUp, 1); // P5_7 TARGET_MAX32630 J1.9 00595 #endif 00596 #if HAS_digitalInOut11 00597 DigitalInOut digitalInOut11(P6_0, PIN_INPUT, PullUp, 1); // P6_0 TARGET_MAX32630 J1.10 00598 #endif 00599 #if HAS_digitalInOut12 00600 DigitalInOut digitalInOut12(P5_0, PIN_INPUT, PullUp, 1); // P5_0 TARGET_MAX32630 J1.11 00601 #endif 00602 #if HAS_digitalInOut13 00603 DigitalInOut digitalInOut13(P5_1, PIN_INPUT, PullUp, 1); // P5_1 TARGET_MAX32630 J1.12 00604 #endif 00605 #if HAS_digitalInOut14 00606 DigitalInOut digitalInOut14(P5_2, PIN_INPUT, PullUp, 1); // P5_2 TARGET_MAX32630 J1.13 00607 #endif 00608 #if HAS_digitalInOut15 00609 DigitalInOut digitalInOut15(P3_0, PIN_INPUT, PullUp, 1); // P3_0 TARGET_MAX32630 J1.14 00610 #endif 00611 //-------------------------------------------------- 00612 #elif defined(TARGET_MAX32625MBED) 00613 // TARGET=MAX32625MBED ARM Cortex-M4F 96MHz 512kB Flash 160kB SRAM 00614 // +-------------------------------------+ 00615 // | MAX32625MBED Arduino UNO header | 00616 // | | 00617 // | A5/SCL[ ] | P1_7 dig15 00618 // | A4/SDA[ ] | P1_6 dig14 00619 // | AREF=N/C[ ] | 00620 // | GND[ ] | 00621 // | [ ]N/C SCK/13[ ] | P1_0 dig13 00622 // | [ ]IOREF=3V3 MISO/12[ ] | P1_2 dig12 00623 // | [ ]RST MOSI/11[ ]~| P1_1 dig11 00624 // | [ ]3V3 CS/10[ ]~| P1_3 dig10 00625 // | [ ]5V0 9[ ]~| P1_5 dig9 00626 // | [ ]GND 8[ ] | P1_4 dig8 00627 // | [ ]GND | 00628 // | [ ]Vin 7[ ] | P0_7 dig7 00629 // | 6[ ]~| P0_6 dig6 00630 // AIN_0 | [ ]A0 5[ ]~| P0_5 dig5 00631 // AIN_1 | [ ]A1 4[ ] | P0_4 dig4 00632 // AIN_2 | [ ]A2 INT1/3[ ]~| P0_3 dig3 00633 // AIN_3 | [ ]A3 INT0/2[ ] | P0_2 dig2 00634 // dig16 P3_4 | [ ]A4/SDA RST SCK MISO TX>1[ ] | P0_1 dig1 00635 // dig17 P3_5 | [ ]A5/SCL [ ] [ ] [ ] RX<0[ ] | P0_0 dig0 00636 // | [ ] [ ] [ ] | 00637 // | UNO_R3 GND MOSI 5V ____________/ 00638 // \_______________________/ 00639 // 00640 #define HAS_digitalInOut0 1 // P0_0 TARGET_MAX32625MBED D0 00641 #define HAS_digitalInOut1 1 // P0_1 TARGET_MAX32625MBED D1 00642 #if APPLICATION_MAX11131 00643 #define HAS_digitalInOut2 0 // P0_2 TARGET_MAX32625MBED D2 -- MAX11131 EOC DigitalIn 00644 #else 00645 #define HAS_digitalInOut2 1 // P0_2 TARGET_MAX32625MBED D2 00646 #endif 00647 #define HAS_digitalInOut3 1 // P0_3 TARGET_MAX32625MBED D3 00648 #define HAS_digitalInOut4 1 // P0_4 TARGET_MAX32625MBED D4 00649 #define HAS_digitalInOut5 1 // P0_5 TARGET_MAX32625MBED D5 00650 #define HAS_digitalInOut6 1 // P0_6 TARGET_MAX32625MBED D6 00651 #define HAS_digitalInOut7 1 // P0_7 TARGET_MAX32625MBED D7 00652 #define HAS_digitalInOut8 1 // P1_4 TARGET_MAX32625MBED D8 00653 #if APPLICATION_MAX11131 00654 #define HAS_digitalInOut9 0 // P1_5 TARGET_MAX32625MBED D9 -- MAX11131 CNVST DigitalOut 00655 #else 00656 #define HAS_digitalInOut9 1 // P1_5 TARGET_MAX32625MBED D9 00657 #endif 00658 #if HAS_SPI 00659 // avoid resource conflict between P5_0, P5_1, P5_2 SPI and DigitalInOut 00660 #define HAS_digitalInOut10 0 // P1_3 TARGET_MAX32635MBED CS/10 00661 #define HAS_digitalInOut11 0 // P1_1 TARGET_MAX32635MBED MOSI/11 00662 #define HAS_digitalInOut12 0 // P1_2 TARGET_MAX32635MBED MISO/12 00663 #define HAS_digitalInOut13 0 // P1_0 TARGET_MAX32635MBED SCK/13 00664 #else // HAS_SPI 00665 #define HAS_digitalInOut10 1 // P1_3 TARGET_MAX32635MBED CS/10 00666 #define HAS_digitalInOut11 1 // P1_1 TARGET_MAX32635MBED MOSI/11 00667 #define HAS_digitalInOut12 1 // P1_2 TARGET_MAX32635MBED MISO/12 00668 #define HAS_digitalInOut13 1 // P1_0 TARGET_MAX32635MBED SCK/13 00669 #endif // HAS_SPI 00670 #if HAS_I2C 00671 // avoid resource conflict between P5_7, P6_0 I2C and DigitalInOut 00672 #define HAS_digitalInOut14 0 // P1_6 TARGET_MAX32635MBED A4/SDA (10pin digital connector) 00673 #define HAS_digitalInOut15 0 // P1_7 TARGET_MAX32635MBED A5/SCL (10pin digital connector) 00674 #define HAS_digitalInOut16 0 // P3_4 TARGET_MAX32635MBED A4/SDA (6pin analog connector) 00675 #define HAS_digitalInOut17 0 // P3_5 TARGET_MAX32635MBED A5/SCL (6pin analog connector) 00676 #else // HAS_I2C 00677 #define HAS_digitalInOut14 1 // P1_6 TARGET_MAX32635MBED A4/SDA (10pin digital connector) 00678 #define HAS_digitalInOut15 1 // P1_7 TARGET_MAX32635MBED A5/SCL (10pin digital connector) 00679 #define HAS_digitalInOut16 1 // P3_4 TARGET_MAX32635MBED A4/SDA (6pin analog connector) 00680 #define HAS_digitalInOut17 1 // P3_5 TARGET_MAX32635MBED A5/SCL (6pin analog connector) 00681 #endif // HAS_I2C 00682 #if HAS_digitalInOut0 00683 DigitalInOut digitalInOut0(P0_0, PIN_INPUT, PullUp, 1); // P0_0 TARGET_MAX32625MBED D0 00684 #endif 00685 #if HAS_digitalInOut1 00686 DigitalInOut digitalInOut1(P0_1, PIN_INPUT, PullUp, 1); // P0_1 TARGET_MAX32625MBED D1 00687 #endif 00688 #if HAS_digitalInOut2 00689 DigitalInOut digitalInOut2(P0_2, PIN_INPUT, PullUp, 1); // P0_2 TARGET_MAX32625MBED D2 00690 #endif 00691 #if HAS_digitalInOut3 00692 DigitalInOut digitalInOut3(P0_3, PIN_INPUT, PullUp, 1); // P0_3 TARGET_MAX32625MBED D3 00693 #endif 00694 #if HAS_digitalInOut4 00695 DigitalInOut digitalInOut4(P0_4, PIN_INPUT, PullUp, 1); // P0_4 TARGET_MAX32625MBED D4 00696 #endif 00697 #if HAS_digitalInOut5 00698 DigitalInOut digitalInOut5(P0_5, PIN_INPUT, PullUp, 1); // P0_5 TARGET_MAX32625MBED D5 00699 #endif 00700 #if HAS_digitalInOut6 00701 DigitalInOut digitalInOut6(P0_6, PIN_INPUT, PullUp, 1); // P0_6 TARGET_MAX32625MBED D6 00702 #endif 00703 #if HAS_digitalInOut7 00704 DigitalInOut digitalInOut7(P0_7, PIN_INPUT, PullUp, 1); // P0_7 TARGET_MAX32625MBED D7 00705 #endif 00706 #if HAS_digitalInOut8 00707 DigitalInOut digitalInOut8(P1_4, PIN_INPUT, PullUp, 1); // P1_4 TARGET_MAX32625MBED D8 00708 #endif 00709 #if HAS_digitalInOut9 00710 DigitalInOut digitalInOut9(P1_5, PIN_INPUT, PullUp, 1); // P1_5 TARGET_MAX32625MBED D9 00711 #endif 00712 #if HAS_digitalInOut10 00713 DigitalInOut digitalInOut10(P1_3, PIN_INPUT, PullUp, 1); // P1_3 TARGET_MAX32635MBED CS/10 00714 #endif 00715 #if HAS_digitalInOut11 00716 DigitalInOut digitalInOut11(P1_1, PIN_INPUT, PullUp, 1); // P1_1 TARGET_MAX32635MBED MOSI/11 00717 #endif 00718 #if HAS_digitalInOut12 00719 DigitalInOut digitalInOut12(P1_2, PIN_INPUT, PullUp, 1); // P1_2 TARGET_MAX32635MBED MISO/12 00720 #endif 00721 #if HAS_digitalInOut13 00722 DigitalInOut digitalInOut13(P1_0, PIN_INPUT, PullUp, 1); // P1_0 TARGET_MAX32635MBED SCK/13 00723 #endif 00724 #if HAS_digitalInOut14 00725 // Ensure that the unused I2C pins do not interfere with analog inputs A4 and A5 00726 // DigitalInOut mode can be one of PullUp, PullDown, PullNone, OpenDrain 00727 DigitalInOut digitalInOut14(P1_6, PIN_INPUT, OpenDrain, 1); // P1_6 TARGET_MAX32635MBED A4/SDA (10pin digital connector) 00728 #endif 00729 #if HAS_digitalInOut15 00730 // Ensure that the unused I2C pins do not interfere with analog inputs A4 and A5 00731 DigitalInOut digitalInOut15(P1_7, PIN_INPUT, OpenDrain, 1); // P1_7 TARGET_MAX32635MBED A5/SCL (10pin digital connector) 00732 #endif 00733 #if HAS_digitalInOut16 00734 // Ensure that the unused I2C pins do not interfere with analog inputs A4 and A5 00735 // DigitalInOut mode can be one of PullUp, PullDown, PullNone, OpenDrain 00736 // PullUp-->3.4V, PullDown-->1.7V, PullNone-->3.5V, OpenDrain-->0.00V 00737 DigitalInOut digitalInOut16(P3_4, PIN_INPUT, OpenDrain, 0); // P3_4 TARGET_MAX32635MBED A4/SDA (6pin analog connector) 00738 #endif 00739 #if HAS_digitalInOut17 00740 // Ensure that the unused I2C pins do not interfere with analog inputs A4 and A5 00741 DigitalInOut digitalInOut17(P3_5, PIN_INPUT, OpenDrain, 0); // P3_5 TARGET_MAX32635MBED A5/SCL (6pin analog connector) 00742 #endif 00743 //-------------------------------------------------- 00744 #elif defined(TARGET_NUCLEO_F446RE) || defined(TARGET_NUCLEO_F401RE) 00745 #define HAS_digitalInOut0 0 // P0_0 TARGET_MAX32625MBED D0 00746 #define HAS_digitalInOut1 0 // P0_1 TARGET_MAX32625MBED D1 00747 #if APPLICATION_MAX11131 00748 #define HAS_digitalInOut2 0 // P0_2 TARGET_MAX32625MBED D2 -- MAX11131 EOC DigitalIn 00749 #else 00750 #define HAS_digitalInOut2 1 // P0_2 TARGET_MAX32625MBED D2 00751 #endif 00752 #define HAS_digitalInOut3 1 // P0_3 TARGET_MAX32625MBED D3 00753 #define HAS_digitalInOut4 1 // P0_4 TARGET_MAX32625MBED D4 00754 #define HAS_digitalInOut5 1 // P0_5 TARGET_MAX32625MBED D5 00755 #define HAS_digitalInOut6 1 // P0_6 TARGET_MAX32625MBED D6 00756 #define HAS_digitalInOut7 1 // P0_7 TARGET_MAX32625MBED D7 00757 #if APPLICATION_MAX5715 00758 #define HAS_digitalInOut8 0 // P1_4 TARGET_MAX32625MBED D8 -- MAX5715 CLRb DigitalOut 00759 #else 00760 #define HAS_digitalInOut8 1 // P1_4 TARGET_MAX32625MBED D8 00761 #endif 00762 #if APPLICATION_MAX5715 00763 #define HAS_digitalInOut9 0 // P1_5 TARGET_MAX32625MBED D9 -- MAX5715 LDACb DigitalOut 00764 #elif APPLICATION_MAX11131 00765 #define HAS_digitalInOut9 0 // P1_5 TARGET_MAX32625MBED D9 -- MAX11131 CNVST DigitalOut 00766 #else 00767 #define HAS_digitalInOut9 1 // P1_5 TARGET_MAX32625MBED D9 00768 #endif 00769 #if HAS_SPI 00770 // avoid resource conflict between P5_0, P5_1, P5_2 SPI and DigitalInOut 00771 // Arduino digital pin D10 SPI function is CS/10 00772 // Arduino digital pin D11 SPI function is MOSI/11 00773 // Arduino digital pin D12 SPI function is MISO/12 00774 // Arduino digital pin D13 SPI function is SCK/13 00775 #define HAS_digitalInOut10 0 00776 #define HAS_digitalInOut11 0 00777 #define HAS_digitalInOut12 0 00778 #define HAS_digitalInOut13 0 00779 #else // HAS_SPI 00780 #define HAS_digitalInOut10 1 00781 #define HAS_digitalInOut11 1 00782 #define HAS_digitalInOut12 1 00783 #define HAS_digitalInOut13 1 00784 #endif // HAS_SPI 00785 #if HAS_I2C 00786 // avoid resource conflict between P5_7, P6_0 I2C and DigitalInOut 00787 // Arduino digital pin D14 I2C function is A4/SDA (10pin digital connector) 00788 // Arduino digital pin D15 I2C function is A5/SCL (10pin digital connector) 00789 // Arduino digital pin D16 I2C function is A4/SDA (6pin analog connector) 00790 // Arduino digital pin D17 I2C function is A5/SCL (6pin analog connector) 00791 #define HAS_digitalInOut14 0 00792 #define HAS_digitalInOut15 0 00793 #define HAS_digitalInOut16 0 00794 #define HAS_digitalInOut17 0 00795 #else // HAS_I2C 00796 #define HAS_digitalInOut14 1 00797 #define HAS_digitalInOut15 1 00798 #define HAS_digitalInOut16 0 00799 #define HAS_digitalInOut17 0 00800 #endif // HAS_I2C 00801 #if HAS_digitalInOut0 00802 DigitalInOut digitalInOut0(D0, PIN_INPUT, PullUp, 1); 00803 #endif 00804 #if HAS_digitalInOut1 00805 DigitalInOut digitalInOut1(D1, PIN_INPUT, PullUp, 1); 00806 #endif 00807 #if HAS_digitalInOut2 00808 DigitalInOut digitalInOut2(D2, PIN_INPUT, PullUp, 1); 00809 #endif 00810 #if HAS_digitalInOut3 00811 DigitalInOut digitalInOut3(D3, PIN_INPUT, PullUp, 1); 00812 #endif 00813 #if HAS_digitalInOut4 00814 DigitalInOut digitalInOut4(D4, PIN_INPUT, PullUp, 1); 00815 #endif 00816 #if HAS_digitalInOut5 00817 DigitalInOut digitalInOut5(D5, PIN_INPUT, PullUp, 1); 00818 #endif 00819 #if HAS_digitalInOut6 00820 DigitalInOut digitalInOut6(D6, PIN_INPUT, PullUp, 1); 00821 #endif 00822 #if HAS_digitalInOut7 00823 DigitalInOut digitalInOut7(D7, PIN_INPUT, PullUp, 1); 00824 #endif 00825 #if HAS_digitalInOut8 00826 DigitalInOut digitalInOut8(D8, PIN_INPUT, PullUp, 1); 00827 #endif 00828 #if HAS_digitalInOut9 00829 DigitalInOut digitalInOut9(D9, PIN_INPUT, PullUp, 1); 00830 #endif 00831 #if HAS_digitalInOut10 00832 // Arduino digital pin D10 SPI function is CS/10 00833 DigitalInOut digitalInOut10(D10, PIN_INPUT, PullUp, 1); 00834 #endif 00835 #if HAS_digitalInOut11 00836 // Arduino digital pin D11 SPI function is MOSI/11 00837 DigitalInOut digitalInOut11(D11, PIN_INPUT, PullUp, 1); 00838 #endif 00839 #if HAS_digitalInOut12 00840 // Arduino digital pin D12 SPI function is MISO/12 00841 DigitalInOut digitalInOut12(D12, PIN_INPUT, PullUp, 1); 00842 #endif 00843 #if HAS_digitalInOut13 00844 // Arduino digital pin D13 SPI function is SCK/13 00845 DigitalInOut digitalInOut13(D13, PIN_INPUT, PullUp, 1); 00846 #endif 00847 #if HAS_digitalInOut14 00848 // Arduino digital pin D14 I2C function is A4/SDA (10pin digital connector) 00849 DigitalInOut digitalInOut14(D14, PIN_INPUT, PullUp, 1); 00850 #endif 00851 #if HAS_digitalInOut15 00852 // Arduino digital pin D15 I2C function is A5/SCL (10pin digital connector) 00853 DigitalInOut digitalInOut15(D15, PIN_INPUT, PullUp, 1); 00854 #endif 00855 #if HAS_digitalInOut16 00856 // Arduino digital pin D16 I2C function is A4/SDA (6pin analog connector) 00857 DigitalInOut digitalInOut16(D16, PIN_INPUT, PullUp, 1); 00858 #endif 00859 #if HAS_digitalInOut17 00860 // Arduino digital pin D17 I2C function is A5/SCL (6pin analog connector) 00861 DigitalInOut digitalInOut17(D17, PIN_INPUT, PullUp, 1); 00862 #endif 00863 //-------------------------------------------------- 00864 #elif defined(TARGET_LPC1768) 00865 #define HAS_digitalInOut0 1 00866 #define HAS_digitalInOut1 1 00867 #define HAS_digitalInOut2 1 00868 #define HAS_digitalInOut3 1 00869 #define HAS_digitalInOut4 1 00870 #define HAS_digitalInOut5 1 00871 #define HAS_digitalInOut6 1 00872 #define HAS_digitalInOut7 1 00873 #define HAS_digitalInOut8 1 00874 #define HAS_digitalInOut9 1 00875 // #define HAS_digitalInOut10 1 00876 // #define HAS_digitalInOut11 1 00877 // #define HAS_digitalInOut12 1 00878 // #define HAS_digitalInOut13 1 00879 // #define HAS_digitalInOut14 1 00880 // #define HAS_digitalInOut15 1 00881 #if HAS_digitalInOut0 00882 DigitalInOut digitalInOut0(p5, PIN_INPUT, PullUp, 1); // TARGET_LPC1768 P0.9/I2STX_SDA/MOSI1/MAT2.3 00883 #endif 00884 #if HAS_digitalInOut1 00885 DigitalInOut digitalInOut1(p6, PIN_INPUT, PullUp, 1); // TARGET_LPC1768 P0.8/I2STX_WS/MISO1/MAT2.2 00886 #endif 00887 #if HAS_digitalInOut2 00888 DigitalInOut digitalInOut2(p7, PIN_INPUT, PullUp, 1); // TARGET_LPC1768 P0.7/I2STX_CLK/SCK1/MAT2.1 00889 #endif 00890 #if HAS_digitalInOut3 00891 DigitalInOut digitalInOut3(p8, PIN_INPUT, PullUp, 1); // TARGET_LPC1768 P0.6/I2SRX_SDA/SSEL1/MAT2.0 00892 #endif 00893 #if HAS_digitalInOut4 00894 DigitalInOut digitalInOut4(p9, PIN_INPUT, PullUp, 1); // TARGET_LPC1768 P0.0/CAN_RX1/TXD3/SDA1 00895 #endif 00896 #if HAS_digitalInOut5 00897 DigitalInOut digitalInOut5(p10, PIN_INPUT, PullUp, 1); // TARGET_LPC1768 P0.1/CAN_TX1/RXD3/SCL1 00898 #endif 00899 #if HAS_digitalInOut6 00900 DigitalInOut digitalInOut6(p11, PIN_INPUT, PullUp, 1); // TARGET_LPC1768 P0.18/DCD1/MOSI0/MOSI1 00901 #endif 00902 #if HAS_digitalInOut7 00903 DigitalInOut digitalInOut7(p12, PIN_INPUT, PullUp, 1); // TARGET_LPC1768 P0.17/CTS1/MISO0/MISO 00904 #endif 00905 #if HAS_digitalInOut8 00906 DigitalInOut digitalInOut8(p13, PIN_INPUT, PullUp, 1); // TARGET_LPC1768 P0.15/TXD1/SCK0/SCK 00907 #endif 00908 #if HAS_digitalInOut9 00909 DigitalInOut digitalInOut9(p14, PIN_INPUT, PullUp, 1); // TARGET_LPC1768 P0.16/RXD1/SSEL0/SSEL 00910 #endif 00911 // 00912 // these pins support analog input analogIn0 .. analogIn5 00913 //DigitalInOut digitalInOut_(p15, PIN_INPUT, PullUp, 1); // TARGET_LPC1768 P0.23/AD0.0/I2SRX_CLK/CAP3.0 00914 //DigitalInOut digitalInOut_(p16, PIN_INPUT, PullUp, 1); // TARGET_LPC1768 P0.24/AD0.1/I2SRX_WS/CAP3.1 00915 //DigitalInOut digitalInOut_(p17, PIN_INPUT, PullUp, 1); // TARGET_LPC1768 P0.25/AD0.2/I2SRX_SDA/TXD3 00916 //DigitalInOut digitalInOut_(p18, PIN_INPUT, PullUp, 1); // TARGET_LPC1768 P0.26/AD0.3/AOUT/RXD3 00917 //DigitalInOut digitalInOut_(p19, PIN_INPUT, PullUp, 1); // TARGET_LPC1768 P1.30/VBUS/AD0.4 00918 //DigitalInOut digitalInOut_(p20, PIN_INPUT, PullUp, 1); // TARGET_LPC1768 P1.31/SCK1/AD0.5 00919 // 00920 // these pins support PWM pwmDriver1 .. pwmDriver6 00921 //DigitalInOut digitalInOut_(p21, PIN_INPUT, PullUp, 1); // TARGET_LPC1768 P2.5/PWM1.6/DTR1/TRACEDATA0 00922 //DigitalInOut digitalInOut_(p22, PIN_INPUT, PullUp, 1); // TARGET_LPC1768 P2.4/PWM1.5/DSR1/TRACEDATA1 00923 //DigitalInOut digitalInOut_(p23, PIN_INPUT, PullUp, 1); // TARGET_LPC1768 P2.3/PWM1.4/DCD1/TRACEDATA2 00924 //DigitalInOut digitalInOut_(p24, PIN_INPUT, PullUp, 1); // TARGET_LPC1768 P2.2/PWM1.3/CTS1/TRACEDATA3 00925 //DigitalInOut digitalInOut_(p25, PIN_INPUT, PullUp, 1); // TARGET_LPC1768 P2.1/PWM1.2/RXD1 00926 //DigitalInOut digitalInOut_(p26, PIN_INPUT, PullUp, 1); // TARGET_LPC1768 P2.0/PWM1.1/TXD1/TRACECLK 00927 // 00928 // these could be additional digitalInOut pins 00929 #if HAS_digitalInOut10 00930 DigitalInOut digitalInOut10(p27, PIN_INPUT, PullUp, 1); // TARGET_LPC1768 P0.11/RXD2/SCL2/MAT3.1 00931 #endif 00932 #if HAS_digitalInOut11 00933 DigitalInOut digitalInOut11(p28, PIN_INPUT, PullUp, 1); // TARGET_LPC1768 P0.10/TXD2/SDA2/MAT3.0 00934 #endif 00935 #if HAS_digitalInOut12 00936 DigitalInOut digitalInOut12(p29, PIN_INPUT, PullUp, 1); // TARGET_LPC1768 P0.5/I2SRX_WS/CAN_TX2/CAP2.1 00937 #endif 00938 #if HAS_digitalInOut13 00939 DigitalInOut digitalInOut13(p30, PIN_INPUT, PullUp, 1); // TARGET_LPC1768 P0.4/I2SRX_CLK/CAN_RX2/CAP2.0 00940 #endif 00941 #if HAS_digitalInOut14 00942 DigitalInOut digitalInOut14(___, PIN_INPUT, PullUp, 1); 00943 #endif 00944 #if HAS_digitalInOut15 00945 DigitalInOut digitalInOut15(___, PIN_INPUT, PullUp, 1); 00946 #endif 00947 #else 00948 // unknown target 00949 #endif 00950 // uncrustify-0.66.1 *INDENT-ON* 00951 #if HAS_digitalInOut0 || HAS_digitalInOut1 \ 00952 || HAS_digitalInOut2 || HAS_digitalInOut3 \ 00953 || HAS_digitalInOut4 || HAS_digitalInOut5 \ 00954 || HAS_digitalInOut6 || HAS_digitalInOut7 \ 00955 || HAS_digitalInOut8 || HAS_digitalInOut9 \ 00956 || HAS_digitalInOut10 || HAS_digitalInOut11 \ 00957 || HAS_digitalInOut12 || HAS_digitalInOut13 \ 00958 || HAS_digitalInOut14 || HAS_digitalInOut15 \ 00959 || HAS_digitalInOut16 || HAS_digitalInOut17 00960 #define HAS_digitalInOuts 1 00961 #endif 00962 00963 // uncrustify-0.66.1 *INDENT-OFF* 00964 //-------------------------------------------------- 00965 // Declare the AnalogIn driver 00966 // Optional analogIn support. If there is only one it should be analogIn1. 00967 // A) analog input 00968 #if defined(TARGET_MAX32630) 00969 #define HAS_analogIn0 1 00970 #define HAS_analogIn1 1 00971 #define HAS_analogIn2 1 00972 #define HAS_analogIn3 1 00973 #define HAS_analogIn4 1 00974 #define HAS_analogIn5 1 00975 #define HAS_analogIn6 1 00976 #define HAS_analogIn7 1 00977 #define HAS_analogIn8 1 00978 #define HAS_analogIn9 1 00979 // #define HAS_analogIn10 0 00980 // #define HAS_analogIn11 0 00981 // #define HAS_analogIn12 0 00982 // #define HAS_analogIn13 0 00983 // #define HAS_analogIn14 0 00984 // #define HAS_analogIn15 0 00985 #if HAS_analogIn0 00986 AnalogIn analogIn0(AIN_0); // TARGET_MAX32630 J1.5 AIN_0 = AIN0 pin fullscale is 1.2V 00987 #endif 00988 #if HAS_analogIn1 00989 AnalogIn analogIn1(AIN_1); // TARGET_MAX32630 J1.6 AIN_1 = AIN1 pin fullscale is 1.2V 00990 #endif 00991 #if HAS_analogIn2 00992 AnalogIn analogIn2(AIN_2); // TARGET_MAX32630 J1.7 AIN_2 = AIN2 pin fullscale is 1.2V 00993 #endif 00994 #if HAS_analogIn3 00995 AnalogIn analogIn3(AIN_3); // TARGET_MAX32630 J1.8 AIN_3 = AIN3 pin fullscale is 1.2V 00996 #endif 00997 #if HAS_analogIn4 00998 AnalogIn analogIn4(AIN_4); // TARGET_MAX32630 J1.5 AIN_4 = AIN0 / 5.0 fullscale is 6.0V 00999 #endif 01000 #if HAS_analogIn5 01001 AnalogIn analogIn5(AIN_5); // TARGET_MAX32630 J1.6 AIN_5 = AIN1 / 5.0 fullscale is 6.0V 01002 #endif 01003 #if HAS_analogIn6 01004 AnalogIn analogIn6(AIN_6); // TARGET_MAX32630 AIN_6 = VDDB / 4.0 fullscale is 4.8V 01005 #endif 01006 #if HAS_analogIn7 01007 AnalogIn analogIn7(AIN_7); // TARGET_MAX32630 AIN_7 = VDD18 fullscale is 1.2V 01008 #endif 01009 #if HAS_analogIn8 01010 AnalogIn analogIn8(AIN_8); // TARGET_MAX32630 AIN_8 = VDD12 fullscale is 1.2V 01011 #endif 01012 #if HAS_analogIn9 01013 AnalogIn analogIn9(AIN_9); // TARGET_MAX32630 AIN_9 = VRTC / 2.0 fullscale is 2.4V 01014 #endif 01015 #if HAS_analogIn10 01016 AnalogIn analogIn10(____); // TARGET_MAX32630 AIN_10 = x undefined? 01017 #endif 01018 #if HAS_analogIn11 01019 AnalogIn analogIn11(____); // TARGET_MAX32630 AIN_11 = VDDIO / 4.0 fullscale is 4.8V 01020 #endif 01021 #if HAS_analogIn12 01022 AnalogIn analogIn12(____); // TARGET_MAX32630 AIN_12 = VDDIOH / 4.0 fullscale is 4.8V 01023 #endif 01024 #if HAS_analogIn13 01025 AnalogIn analogIn13(____); 01026 #endif 01027 #if HAS_analogIn14 01028 AnalogIn analogIn14(____); 01029 #endif 01030 #if HAS_analogIn15 01031 AnalogIn analogIn15(____); 01032 #endif 01033 //-------------------------------------------------- 01034 #elif defined(TARGET_MAX32625MBED) 01035 #define HAS_analogIn0 1 01036 #define HAS_analogIn1 1 01037 #define HAS_analogIn2 1 01038 #define HAS_analogIn3 1 01039 #define HAS_analogIn4 1 01040 #define HAS_analogIn5 1 01041 #if HAS_analogIn0 01042 AnalogIn analogIn0(AIN_0); // TARGET_MAX32630 J1.5 AIN_0 = AIN0 pin fullscale is 1.2V 01043 #endif 01044 #if HAS_analogIn1 01045 AnalogIn analogIn1(AIN_1); // TARGET_MAX32630 J1.6 AIN_1 = AIN1 pin fullscale is 1.2V 01046 #endif 01047 #if HAS_analogIn2 01048 AnalogIn analogIn2(AIN_2); // TARGET_MAX32630 J1.7 AIN_2 = AIN2 pin fullscale is 1.2V 01049 #endif 01050 #if HAS_analogIn3 01051 AnalogIn analogIn3(AIN_3); // TARGET_MAX32630 J1.8 AIN_3 = AIN3 pin fullscale is 1.2V 01052 #endif 01053 #if HAS_analogIn4 01054 AnalogIn analogIn4(AIN_4); // TARGET_MAX32630 J1.5 AIN_4 = AIN0 / 5.0 fullscale is 6.0V 01055 #endif 01056 #if HAS_analogIn5 01057 AnalogIn analogIn5(AIN_5); // TARGET_MAX32630 J1.6 AIN_5 = AIN1 / 5.0 fullscale is 6.0V 01058 #endif 01059 //-------------------------------------------------- 01060 #elif defined(TARGET_MAX32620FTHR) 01061 #warning "TARGET_MAX32620FTHR not previously tested; need to verify analogIn0..." 01062 #define HAS_analogIn0 1 01063 #define HAS_analogIn1 1 01064 #define HAS_analogIn2 1 01065 #define HAS_analogIn3 1 01066 #define HAS_analogIn4 1 01067 #define HAS_analogIn5 1 01068 #define HAS_analogIn6 1 01069 #define HAS_analogIn7 1 01070 #define HAS_analogIn8 1 01071 #define HAS_analogIn9 1 01072 // #define HAS_analogIn10 0 01073 // #define HAS_analogIn11 0 01074 // #define HAS_analogIn12 0 01075 // #define HAS_analogIn13 0 01076 // #define HAS_analogIn14 0 01077 // #define HAS_analogIn15 0 01078 #if HAS_analogIn0 01079 AnalogIn analogIn0(AIN_0); // TARGET_MAX32620FTHR J1.5 AIN_0 = AIN0 pin fullscale is 1.2V 01080 #endif 01081 #if HAS_analogIn1 01082 AnalogIn analogIn1(AIN_1); // TARGET_MAX32620FTHR J1.6 AIN_1 = AIN1 pin fullscale is 1.2V 01083 #endif 01084 #if HAS_analogIn2 01085 AnalogIn analogIn2(AIN_2); // TARGET_MAX32620FTHR J1.7 AIN_2 = AIN2 pin fullscale is 1.2V 01086 #endif 01087 #if HAS_analogIn3 01088 AnalogIn analogIn3(AIN_3); // TARGET_MAX32620FTHR J1.8 AIN_3 = AIN3 pin fullscale is 1.2V 01089 #endif 01090 #if HAS_analogIn4 01091 AnalogIn analogIn4(AIN_4); // TARGET_MAX32620FTHR J1.5 AIN_4 = AIN0 / 5.0 fullscale is 6.0V 01092 #endif 01093 #if HAS_analogIn5 01094 AnalogIn analogIn5(AIN_5); // TARGET_MAX32620FTHR J1.6 AIN_5 = AIN1 / 5.0 fullscale is 6.0V 01095 #endif 01096 #if HAS_analogIn6 01097 AnalogIn analogIn6(AIN_6); // TARGET_MAX32620FTHR AIN_6 = VDDB / 4.0 fullscale is 4.8V 01098 #endif 01099 #if HAS_analogIn7 01100 AnalogIn analogIn7(AIN_7); // TARGET_MAX32620FTHR AIN_7 = VDD18 fullscale is 1.2V 01101 #endif 01102 #if HAS_analogIn8 01103 AnalogIn analogIn8(AIN_8); // TARGET_MAX32620FTHR AIN_8 = VDD12 fullscale is 1.2V 01104 #endif 01105 #if HAS_analogIn9 01106 AnalogIn analogIn9(AIN_9); // TARGET_MAX32620FTHR AIN_9 = VRTC / 2.0 fullscale is 2.4V 01107 #endif 01108 #if HAS_analogIn10 01109 AnalogIn analogIn10(____); // TARGET_MAX32620FTHR AIN_10 = x undefined? 01110 #endif 01111 #if HAS_analogIn11 01112 AnalogIn analogIn11(____); // TARGET_MAX32620FTHR AIN_11 = VDDIO / 4.0 fullscale is 4.8V 01113 #endif 01114 #if HAS_analogIn12 01115 AnalogIn analogIn12(____); // TARGET_MAX32620FTHR AIN_12 = VDDIOH / 4.0 fullscale is 4.8V 01116 #endif 01117 #if HAS_analogIn13 01118 AnalogIn analogIn13(____); 01119 #endif 01120 #if HAS_analogIn14 01121 AnalogIn analogIn14(____); 01122 #endif 01123 #if HAS_analogIn15 01124 AnalogIn analogIn15(____); 01125 #endif 01126 //-------------------------------------------------- 01127 #elif defined(TARGET_MAX32625PICO) 01128 #warning "TARGET_MAX32625PICO not previously tested; need to verify analogIn0..." 01129 #define HAS_analogIn0 1 01130 #define HAS_analogIn1 1 01131 #define HAS_analogIn2 1 01132 #define HAS_analogIn3 1 01133 #define HAS_analogIn4 1 01134 #define HAS_analogIn5 1 01135 #if HAS_analogIn0 01136 AnalogIn analogIn0(AIN_0); // TARGET_MAX32630 J1.5 AIN_0 = AIN0 pin fullscale is 1.2V 01137 #endif 01138 #if HAS_analogIn1 01139 AnalogIn analogIn1(AIN_1); // TARGET_MAX32630 J1.6 AIN_1 = AIN1 pin fullscale is 1.2V 01140 #endif 01141 #if HAS_analogIn2 01142 AnalogIn analogIn2(AIN_2); // TARGET_MAX32630 J1.7 AIN_2 = AIN2 pin fullscale is 1.2V 01143 #endif 01144 #if HAS_analogIn3 01145 AnalogIn analogIn3(AIN_3); // TARGET_MAX32630 J1.8 AIN_3 = AIN3 pin fullscale is 1.2V 01146 #endif 01147 #if HAS_analogIn4 01148 AnalogIn analogIn4(AIN_4); // TARGET_MAX32630 J1.5 AIN_4 = AIN0 / 5.0 fullscale is 6.0V 01149 #endif 01150 #if HAS_analogIn5 01151 AnalogIn analogIn5(AIN_5); // TARGET_MAX32630 J1.6 AIN_5 = AIN1 / 5.0 fullscale is 6.0V 01152 #endif 01153 //-------------------------------------------------- 01154 #elif defined(TARGET_MAX32600) 01155 #define HAS_analogIn0 1 01156 #define HAS_analogIn1 1 01157 #define HAS_analogIn2 1 01158 #define HAS_analogIn3 1 01159 #define HAS_analogIn4 1 01160 #define HAS_analogIn5 1 01161 #if HAS_analogIn0 01162 AnalogIn analogIn0(A0); 01163 #endif 01164 #if HAS_analogIn1 01165 AnalogIn analogIn1(A1); 01166 #endif 01167 #if HAS_analogIn2 01168 AnalogIn analogIn2(A2); 01169 #endif 01170 #if HAS_analogIn3 01171 AnalogIn analogIn3(A3); 01172 #endif 01173 #if HAS_analogIn4 01174 AnalogIn analogIn4(A4); 01175 #endif 01176 #if HAS_analogIn5 01177 AnalogIn analogIn5(A5); 01178 #endif 01179 //-------------------------------------------------- 01180 #elif defined(TARGET_NUCLEO_F446RE) 01181 #define HAS_analogIn0 1 01182 #define HAS_analogIn1 1 01183 #define HAS_analogIn2 1 01184 #define HAS_analogIn3 1 01185 #define HAS_analogIn4 1 01186 #define HAS_analogIn5 1 01187 #if HAS_analogIn0 01188 AnalogIn analogIn0(A0); 01189 #endif 01190 #if HAS_analogIn1 01191 AnalogIn analogIn1(A1); 01192 #endif 01193 #if HAS_analogIn2 01194 AnalogIn analogIn2(A2); 01195 #endif 01196 #if HAS_analogIn3 01197 AnalogIn analogIn3(A3); 01198 #endif 01199 #if HAS_analogIn4 01200 AnalogIn analogIn4(A4); 01201 #endif 01202 #if HAS_analogIn5 01203 AnalogIn analogIn5(A5); 01204 #endif 01205 //-------------------------------------------------- 01206 #elif defined(TARGET_NUCLEO_F401RE) 01207 #define HAS_analogIn0 1 01208 #define HAS_analogIn1 1 01209 #define HAS_analogIn2 1 01210 #define HAS_analogIn3 1 01211 #define HAS_analogIn4 1 01212 #define HAS_analogIn5 1 01213 #if HAS_analogIn0 01214 AnalogIn analogIn0(A0); 01215 #endif 01216 #if HAS_analogIn1 01217 AnalogIn analogIn1(A1); 01218 #endif 01219 #if HAS_analogIn2 01220 AnalogIn analogIn2(A2); 01221 #endif 01222 #if HAS_analogIn3 01223 AnalogIn analogIn3(A3); 01224 #endif 01225 #if HAS_analogIn4 01226 AnalogIn analogIn4(A4); 01227 #endif 01228 #if HAS_analogIn5 01229 AnalogIn analogIn5(A5); 01230 #endif 01231 //-------------------------------------------------- 01232 // TODO1: TARGET=MAX32625MBED ARM Cortex-M4F 96MHz 512kB Flash 160kB SRAM 01233 #elif defined(TARGET_LPC1768) 01234 #define HAS_analogIn0 1 01235 #define HAS_analogIn1 1 01236 #define HAS_analogIn2 1 01237 #define HAS_analogIn3 1 01238 #define HAS_analogIn4 1 01239 #define HAS_analogIn5 1 01240 // #define HAS_analogIn6 1 01241 // #define HAS_analogIn7 1 01242 // #define HAS_analogIn8 1 01243 // #define HAS_analogIn9 1 01244 // #define HAS_analogIn10 1 01245 // #define HAS_analogIn11 1 01246 // #define HAS_analogIn12 1 01247 // #define HAS_analogIn13 1 01248 // #define HAS_analogIn14 1 01249 // #define HAS_analogIn15 1 01250 #if HAS_analogIn0 01251 AnalogIn analogIn0(p15); // TARGET_LPC1768 P0.23/AD0.0/I2SRX_CLK/CAP3.0 01252 #endif 01253 #if HAS_analogIn1 01254 AnalogIn analogIn1(p16); // TARGET_LPC1768 P0.24/AD0.1/I2SRX_WS/CAP3.1 01255 #endif 01256 #if HAS_analogIn2 01257 AnalogIn analogIn2(p17); // TARGET_LPC1768 P0.25/AD0.2/I2SRX_SDA/TXD3 01258 #endif 01259 #if HAS_analogIn3 01260 AnalogIn analogIn3(p18); // TARGET_LPC1768 P0.26/AD0.3/AOUT/RXD3 01261 #endif 01262 #if HAS_analogIn4 01263 AnalogIn analogIn4(p19); // TARGET_LPC1768 P1.30/VBUS/AD0.4 01264 #endif 01265 #if HAS_analogIn5 01266 AnalogIn analogIn5(p20); // TARGET_LPC1768 P1.31/SCK1/AD0.5 01267 #endif 01268 #if HAS_analogIn6 01269 AnalogIn analogIn6(____); 01270 #endif 01271 #if HAS_analogIn7 01272 AnalogIn analogIn7(____); 01273 #endif 01274 #if HAS_analogIn8 01275 AnalogIn analogIn8(____); 01276 #endif 01277 #if HAS_analogIn9 01278 AnalogIn analogIn9(____); 01279 #endif 01280 #if HAS_analogIn10 01281 AnalogIn analogIn10(____); 01282 #endif 01283 #if HAS_analogIn11 01284 AnalogIn analogIn11(____); 01285 #endif 01286 #if HAS_analogIn12 01287 AnalogIn analogIn12(____); 01288 #endif 01289 #if HAS_analogIn13 01290 AnalogIn analogIn13(____); 01291 #endif 01292 #if HAS_analogIn14 01293 AnalogIn analogIn14(____); 01294 #endif 01295 #if HAS_analogIn15 01296 AnalogIn analogIn15(____); 01297 #endif 01298 #else 01299 // unknown target 01300 #endif 01301 // uncrustify-0.66.1 *INDENT-ON* 01302 #if HAS_analogIn0 || HAS_analogIn1 \ 01303 || HAS_analogIn2 || HAS_analogIn3 \ 01304 || HAS_analogIn4 || HAS_analogIn5 \ 01305 || HAS_analogIn6 || HAS_analogIn7 \ 01306 || HAS_analogIn8 || HAS_analogIn9 \ 01307 || HAS_analogIn10 || HAS_analogIn11 \ 01308 || HAS_analogIn12 || HAS_analogIn13 \ 01309 || HAS_analogIn14 || HAS_analogIn15 01310 #define HAS_analogIns 1 01311 #endif 01312 01313 // DigitalInOut pin resource: print the pin index names to serial 01314 #if HAS_digitalInOuts 01315 void list_digitalInOutPins(Stream& serialStream) 01316 { 01317 #if HAS_digitalInOut0 01318 serialStream.printf(" 0"); 01319 #endif 01320 #if HAS_digitalInOut1 01321 serialStream.printf(" 1"); 01322 #endif 01323 #if HAS_digitalInOut2 01324 serialStream.printf(" 2"); 01325 #endif 01326 #if HAS_digitalInOut3 01327 serialStream.printf(" 3"); 01328 #endif 01329 #if HAS_digitalInOut4 01330 serialStream.printf(" 4"); 01331 #endif 01332 #if HAS_digitalInOut5 01333 serialStream.printf(" 5"); 01334 #endif 01335 #if HAS_digitalInOut6 01336 serialStream.printf(" 6"); 01337 #endif 01338 #if HAS_digitalInOut7 01339 serialStream.printf(" 7"); 01340 #endif 01341 #if HAS_digitalInOut8 01342 serialStream.printf(" 8"); 01343 #endif 01344 #if HAS_digitalInOut9 01345 serialStream.printf(" 9"); 01346 #endif 01347 #if HAS_digitalInOut10 01348 serialStream.printf(" 10"); 01349 #endif 01350 #if HAS_digitalInOut11 01351 serialStream.printf(" 11"); 01352 #endif 01353 #if HAS_digitalInOut12 01354 serialStream.printf(" 12"); 01355 #endif 01356 #if HAS_digitalInOut13 01357 serialStream.printf(" 13"); 01358 #endif 01359 #if HAS_digitalInOut14 01360 serialStream.printf(" 14"); 01361 #endif 01362 #if HAS_digitalInOut15 01363 serialStream.printf(" 15"); 01364 #endif 01365 #if HAS_digitalInOut16 01366 serialStream.printf(" 16"); 01367 #endif 01368 #if HAS_digitalInOut17 01369 serialStream.printf(" 17"); 01370 #endif 01371 } 01372 #endif 01373 01374 01375 // DigitalInOut pin resource: search index 01376 #if HAS_digitalInOuts 01377 DigitalInOut& find_digitalInOutPin(int cPinIndex) 01378 { 01379 switch (cPinIndex) 01380 { 01381 default: // default to the first defined digitalInOut pin 01382 #if HAS_digitalInOut0 01383 case '0': case 0x00: return digitalInOut0; 01384 #endif 01385 #if HAS_digitalInOut1 01386 case '1': case 0x01: return digitalInOut1; 01387 #endif 01388 #if HAS_digitalInOut2 01389 case '2': case 0x02: return digitalInOut2; 01390 #endif 01391 #if HAS_digitalInOut3 01392 case '3': case 0x03: return digitalInOut3; 01393 #endif 01394 #if HAS_digitalInOut4 01395 case '4': case 0x04: return digitalInOut4; 01396 #endif 01397 #if HAS_digitalInOut5 01398 case '5': case 0x05: return digitalInOut5; 01399 #endif 01400 #if HAS_digitalInOut6 01401 case '6': case 0x06: return digitalInOut6; 01402 #endif 01403 #if HAS_digitalInOut7 01404 case '7': case 0x07: return digitalInOut7; 01405 #endif 01406 #if HAS_digitalInOut8 01407 case '8': case 0x08: return digitalInOut8; 01408 #endif 01409 #if HAS_digitalInOut9 01410 case '9': case 0x09: return digitalInOut9; 01411 #endif 01412 #if HAS_digitalInOut10 01413 case 'a': case 0x0a: return digitalInOut10; 01414 #endif 01415 #if HAS_digitalInOut11 01416 case 'b': case 0x0b: return digitalInOut11; 01417 #endif 01418 #if HAS_digitalInOut12 01419 case 'c': case 0x0c: return digitalInOut12; 01420 #endif 01421 #if HAS_digitalInOut13 01422 case 'd': case 0x0d: return digitalInOut13; 01423 #endif 01424 #if HAS_digitalInOut14 01425 case 'e': case 0x0e: return digitalInOut14; 01426 #endif 01427 #if HAS_digitalInOut15 01428 case 'f': case 0x0f: return digitalInOut15; 01429 #endif 01430 #if HAS_digitalInOut16 01431 case 'g': case 0x10: return digitalInOut16; 01432 #endif 01433 #if HAS_digitalInOut17 01434 case 'h': case 0x11: return digitalInOut17; 01435 #endif 01436 } 01437 } 01438 #endif 01439 01440 01441 // AnalogIn pin resource: search index 01442 #if HAS_analogIns 01443 AnalogIn& find_analogInPin(int cPinIndex) 01444 { 01445 switch (cPinIndex) 01446 { 01447 default: // default to the first defined analogIn pin 01448 #if HAS_analogIn0 01449 case '0': case 0x00: return analogIn0; 01450 #endif 01451 #if HAS_analogIn1 01452 case '1': case 0x01: return analogIn1; 01453 #endif 01454 #if HAS_analogIn2 01455 case '2': case 0x02: return analogIn2; 01456 #endif 01457 #if HAS_analogIn3 01458 case '3': case 0x03: return analogIn3; 01459 #endif 01460 #if HAS_analogIn4 01461 case '4': case 0x04: return analogIn4; 01462 #endif 01463 #if HAS_analogIn5 01464 case '5': case 0x05: return analogIn5; 01465 #endif 01466 #if HAS_analogIn6 01467 case '6': case 0x06: return analogIn6; 01468 #endif 01469 #if HAS_analogIn7 01470 case '7': case 0x07: return analogIn7; 01471 #endif 01472 #if HAS_analogIn8 01473 case '8': case 0x08: return analogIn8; 01474 #endif 01475 #if HAS_analogIn9 01476 case '9': case 0x09: return analogIn9; 01477 #endif 01478 #if HAS_analogIn10 01479 case 'a': case 0x0a: return analogIn10; 01480 #endif 01481 #if HAS_analogIn11 01482 case 'b': case 0x0b: return analogIn11; 01483 #endif 01484 #if HAS_analogIn12 01485 case 'c': case 0x0c: return analogIn12; 01486 #endif 01487 #if HAS_analogIn13 01488 case 'd': case 0x0d: return analogIn13; 01489 #endif 01490 #if HAS_analogIn14 01491 case 'e': case 0x0e: return analogIn14; 01492 #endif 01493 #if HAS_analogIn15 01494 case 'f': case 0x0f: return analogIn15; 01495 #endif 01496 } 01497 } 01498 #endif 01499 01500 #if HAS_analogIns 01501 const float analogInPin_fullScaleVoltage[] = { 01502 # if defined(TARGET_MAX32630) 01503 ADC_FULL_SCALE_VOLTAGE, // analogIn0 01504 ADC_FULL_SCALE_VOLTAGE, // analogIn1 01505 ADC_FULL_SCALE_VOLTAGE, // analogIn2 01506 ADC_FULL_SCALE_VOLTAGE, // analogIn3 01507 ADC_FULL_SCALE_VOLTAGE * 5.0f, // analogIn4 // AIN_4 = AIN0 / 5.0 fullscale is 6.0V 01508 ADC_FULL_SCALE_VOLTAGE * 5.0f, // analogIn4 // AIN_5 = AIN1 / 5.0 fullscale is 6.0V 01509 ADC_FULL_SCALE_VOLTAGE * 4.0f, // analogIn6 // AIN_6 = VDDB / 4.0 fullscale is 4.8V 01510 ADC_FULL_SCALE_VOLTAGE, // analogIn7 // AIN_7 = VDD18 fullscale is 1.2V 01511 ADC_FULL_SCALE_VOLTAGE, // analogIn8 // AIN_8 = VDD12 fullscale is 1.2V 01512 ADC_FULL_SCALE_VOLTAGE * 2.0f, // analogIn9 // AIN_9 = VRTC / 2.0 fullscale is 2.4V 01513 ADC_FULL_SCALE_VOLTAGE, // analogIn10 // AIN_10 = x undefined? 01514 ADC_FULL_SCALE_VOLTAGE * 4.0f, // analogIn11 // AIN_11 = VDDIO / 4.0 fullscale is 4.8V 01515 ADC_FULL_SCALE_VOLTAGE * 4.0f, // analogIn12 // AIN_12 = VDDIOH / 4.0 fullscale is 4.8V 01516 ADC_FULL_SCALE_VOLTAGE, // analogIn13 01517 ADC_FULL_SCALE_VOLTAGE, // analogIn14 01518 ADC_FULL_SCALE_VOLTAGE // analogIn15 01519 # elif defined(TARGET_MAX32620FTHR) 01520 #warning "TARGET_MAX32620FTHR not previously tested; need to verify analogIn0..." 01521 ADC_FULL_SCALE_VOLTAGE, // analogIn0 01522 ADC_FULL_SCALE_VOLTAGE, // analogIn1 01523 ADC_FULL_SCALE_VOLTAGE, // analogIn2 01524 ADC_FULL_SCALE_VOLTAGE, // analogIn3 01525 ADC_FULL_SCALE_VOLTAGE * 5.0f, // analogIn4 // AIN_4 = AIN0 / 5.0 fullscale is 6.0V 01526 ADC_FULL_SCALE_VOLTAGE * 5.0f, // analogIn4 // AIN_5 = AIN1 / 5.0 fullscale is 6.0V 01527 ADC_FULL_SCALE_VOLTAGE * 4.0f, // analogIn6 // AIN_6 = VDDB / 4.0 fullscale is 4.8V 01528 ADC_FULL_SCALE_VOLTAGE, // analogIn7 // AIN_7 = VDD18 fullscale is 1.2V 01529 ADC_FULL_SCALE_VOLTAGE, // analogIn8 // AIN_8 = VDD12 fullscale is 1.2V 01530 ADC_FULL_SCALE_VOLTAGE * 2.0f, // analogIn9 // AIN_9 = VRTC / 2.0 fullscale is 2.4V 01531 ADC_FULL_SCALE_VOLTAGE, // analogIn10 // AIN_10 = x undefined? 01532 ADC_FULL_SCALE_VOLTAGE * 4.0f, // analogIn11 // AIN_11 = VDDIO / 4.0 fullscale is 4.8V 01533 ADC_FULL_SCALE_VOLTAGE * 4.0f, // analogIn12 // AIN_12 = VDDIOH / 4.0 fullscale is 4.8V 01534 ADC_FULL_SCALE_VOLTAGE, // analogIn13 01535 ADC_FULL_SCALE_VOLTAGE, // analogIn14 01536 ADC_FULL_SCALE_VOLTAGE // analogIn15 01537 #elif defined(TARGET_MAX32625MBED) 01538 ADC_FULL_SCALE_VOLTAGE * 1.0f, // analogIn0 // fullscale is 1.2V 01539 ADC_FULL_SCALE_VOLTAGE * 1.0f, // analogIn1 // fullscale is 1.2V 01540 ADC_FULL_SCALE_VOLTAGE * 1.0f, // analogIn2 // fullscale is 1.2V 01541 ADC_FULL_SCALE_VOLTAGE * 1.0f, // analogIn3 // fullscale is 1.2V 01542 ADC_FULL_SCALE_VOLTAGE * 5.0f, // analogIn4 // AIN_4 = AIN0 / 5.0 fullscale is 6.0V 01543 ADC_FULL_SCALE_VOLTAGE * 5.0f, // analogIn4 // AIN_5 = AIN1 / 5.0 fullscale is 6.0V 01544 ADC_FULL_SCALE_VOLTAGE * 4.0f, // analogIn6 // AIN_6 = VDDB / 4.0 fullscale is 4.8V 01545 ADC_FULL_SCALE_VOLTAGE, // analogIn7 // AIN_7 = VDD18 fullscale is 1.2V 01546 ADC_FULL_SCALE_VOLTAGE, // analogIn8 // AIN_8 = VDD12 fullscale is 1.2V 01547 ADC_FULL_SCALE_VOLTAGE * 2.0f, // analogIn9 // AIN_9 = VRTC / 2.0 fullscale is 2.4V 01548 ADC_FULL_SCALE_VOLTAGE, // analogIn10 // AIN_10 = x undefined? 01549 ADC_FULL_SCALE_VOLTAGE * 4.0f, // analogIn11 // AIN_11 = VDDIO / 4.0 fullscale is 4.8V 01550 ADC_FULL_SCALE_VOLTAGE * 4.0f, // analogIn12 // AIN_12 = VDDIOH / 4.0 fullscale is 4.8V 01551 ADC_FULL_SCALE_VOLTAGE, // analogIn13 01552 ADC_FULL_SCALE_VOLTAGE, // analogIn14 01553 ADC_FULL_SCALE_VOLTAGE // analogIn15 01554 #elif defined(TARGET_NUCLEO_F446RE) 01555 ADC_FULL_SCALE_VOLTAGE, // analogIn0 01556 ADC_FULL_SCALE_VOLTAGE, // analogIn1 01557 ADC_FULL_SCALE_VOLTAGE, // analogIn2 01558 ADC_FULL_SCALE_VOLTAGE, // analogIn3 01559 ADC_FULL_SCALE_VOLTAGE, // analogIn4 01560 ADC_FULL_SCALE_VOLTAGE, // analogIn5 01561 ADC_FULL_SCALE_VOLTAGE, // analogIn6 01562 ADC_FULL_SCALE_VOLTAGE, // analogIn7 01563 ADC_FULL_SCALE_VOLTAGE, // analogIn8 01564 ADC_FULL_SCALE_VOLTAGE, // analogIn9 01565 ADC_FULL_SCALE_VOLTAGE, // analogIn10 01566 ADC_FULL_SCALE_VOLTAGE, // analogIn11 01567 ADC_FULL_SCALE_VOLTAGE, // analogIn12 01568 ADC_FULL_SCALE_VOLTAGE, // analogIn13 01569 ADC_FULL_SCALE_VOLTAGE, // analogIn14 01570 ADC_FULL_SCALE_VOLTAGE // analogIn15 01571 #elif defined(TARGET_NUCLEO_F401RE) 01572 ADC_FULL_SCALE_VOLTAGE, // analogIn0 01573 ADC_FULL_SCALE_VOLTAGE, // analogIn1 01574 ADC_FULL_SCALE_VOLTAGE, // analogIn2 01575 ADC_FULL_SCALE_VOLTAGE, // analogIn3 01576 ADC_FULL_SCALE_VOLTAGE, // analogIn4 01577 ADC_FULL_SCALE_VOLTAGE, // analogIn5 01578 ADC_FULL_SCALE_VOLTAGE, // analogIn6 01579 ADC_FULL_SCALE_VOLTAGE, // analogIn7 01580 ADC_FULL_SCALE_VOLTAGE, // analogIn8 01581 ADC_FULL_SCALE_VOLTAGE, // analogIn9 01582 ADC_FULL_SCALE_VOLTAGE, // analogIn10 01583 ADC_FULL_SCALE_VOLTAGE, // analogIn11 01584 ADC_FULL_SCALE_VOLTAGE, // analogIn12 01585 ADC_FULL_SCALE_VOLTAGE, // analogIn13 01586 ADC_FULL_SCALE_VOLTAGE, // analogIn14 01587 ADC_FULL_SCALE_VOLTAGE // analogIn15 01588 //#elif defined(TARGET_LPC1768) 01589 #else 01590 // unknown target 01591 ADC_FULL_SCALE_VOLTAGE, // analogIn0 01592 ADC_FULL_SCALE_VOLTAGE, // analogIn1 01593 ADC_FULL_SCALE_VOLTAGE, // analogIn2 01594 ADC_FULL_SCALE_VOLTAGE, // analogIn3 01595 ADC_FULL_SCALE_VOLTAGE, // analogIn4 01596 ADC_FULL_SCALE_VOLTAGE, // analogIn5 01597 ADC_FULL_SCALE_VOLTAGE, // analogIn6 01598 ADC_FULL_SCALE_VOLTAGE, // analogIn7 01599 ADC_FULL_SCALE_VOLTAGE, // analogIn8 01600 ADC_FULL_SCALE_VOLTAGE, // analogIn9 01601 ADC_FULL_SCALE_VOLTAGE, // analogIn10 01602 ADC_FULL_SCALE_VOLTAGE, // analogIn11 01603 ADC_FULL_SCALE_VOLTAGE, // analogIn12 01604 ADC_FULL_SCALE_VOLTAGE, // analogIn13 01605 ADC_FULL_SCALE_VOLTAGE, // analogIn14 01606 ADC_FULL_SCALE_VOLTAGE // analogIn15 01607 # endif 01608 }; 01609 #endif 01610 01611 01612 01613 01614 //-------------------------------------------------- 01615 // Option to use LEDs to show status 01616 #ifndef USE_LEDS 01617 #define USE_LEDS 1 01618 #endif 01619 #if USE_LEDS 01620 #if defined(TARGET_MAX32630) 01621 # define LED_ON 0 01622 # define LED_OFF 1 01623 //-------------------------------------------------- 01624 #elif defined(TARGET_MAX32625MBED) 01625 # define LED_ON 0 01626 # define LED_OFF 1 01627 //-------------------------------------------------- 01628 // TODO1: TARGET=MAX32625MBED ARM Cortex-M4F 96MHz 512kB Flash 160kB SRAM 01629 #elif defined(TARGET_LPC1768) 01630 # define LED_ON 1 01631 # define LED_OFF 0 01632 #else // not defined(TARGET_LPC1768 etc.) 01633 // USE_LEDS with some platform other than MAX32630, MAX32625MBED, LPC1768 01634 // bugfix for MAX32600MBED LED blink pattern: check if LED_ON/LED_OFF already defined 01635 # ifndef LED_ON 01636 # define LED_ON 0 01637 # endif 01638 # ifndef LED_OFF 01639 # define LED_OFF 1 01640 # endif 01641 //# define LED_ON 1 01642 //# define LED_OFF 0 01643 #endif // target definition 01644 DigitalOut led1(LED1, LED_OFF); // MAX32630FTHR: LED1 = LED_RED 01645 DigitalOut led2(LED2, LED_OFF); // MAX32630FTHR: LED2 = LED_GREEN 01646 DigitalOut led3(LED3, LED_OFF); // MAX32630FTHR: LED3 = LED_BLUE 01647 DigitalOut led4(LED4, LED_OFF); 01648 #else // USE_LEDS=0 01649 // issue #41 support Nucleo_F446RE 01650 // there are no LED indicators on the board, LED1 interferes with SPI; 01651 // but we still need placeholders led1 led2 led3 led4. 01652 // Declare DigitalOut led1 led2 led3 led4 targeting safe pins. 01653 // PinName NC means NOT_CONNECTED; DigitalOut::is_connected() returns false 01654 # define LED_ON 0 01655 # define LED_OFF 1 01656 DigitalOut led1(NC, LED_OFF); 01657 DigitalOut led2(NC, LED_OFF); 01658 DigitalOut led3(NC, LED_OFF); 01659 DigitalOut led4(NC, LED_OFF); 01660 #endif // USE_LEDS 01661 #define led1_RFailLED led1 01662 #define led2_GPassLED led2 01663 #define led3_BBusyLED led3 01664 01665 //-------------------------------------------------- 01666 01667 01668 // example code board support 01669 //MAX32630FTHR pegasus(MAX32630FTHR::VIO_3V3); 01670 //DigitalOut rLED(LED1); 01671 //DigitalOut gLED(LED2); 01672 //DigitalOut bLED(LED3); 01673 // 01674 // Arduino "shield" connector port definitions (MAX32625MBED shown) 01675 #if defined(TARGET_MAX32625MBED) 01676 #define A0 AIN_0 01677 #define A1 AIN_1 01678 #define A2 AIN_2 01679 #define A3 AIN_3 01680 #define D0 P0_0 01681 #define D1 P0_1 01682 #define D2 P0_2 01683 #define D3 P0_3 01684 #define D4 P0_4 01685 #define D5 P0_5 01686 #define D6 P0_6 01687 #define D7 P0_7 01688 #define D8 P1_4 01689 #define D9 P1_5 01690 #define D10 P1_3 01691 #define D11 P1_1 01692 #define D12 P1_2 01693 #define D13 P1_0 01694 #endif 01695 01696 // example code declare SPI interface 01697 #if defined(TARGET_MAX32625MBED) 01698 // SPI spi(SPI1_MOSI, SPI1_MISO, SPI1_SCK); // mosi, miso, sclk spi1 TARGET_MAX32625MBED: P1_1 P1_2 P1_0 Arduino 10-pin header D11 D12 D13 D10 01699 // DigitalOut spi_cs(SPI1_SS); // TARGET_MAX32625MBED: not connected 01700 // Support SPI hardware-controlled CS instead of GPIO CS (mbed) 01701 // 2020-02-19 MAX32625MBED GPIO CS envelope is 23us (11.4us before SCLK and 8.6us after SCLK). 01702 // 2020-02-19 MAX32625MBED SPI controlled CS envelope 4 channel read reduced to 4.2us (24MHz SCLK), 1.2us setup, 0us hold. 01703 // 2020-02-19 MAX11043 slowest EOC rate is 9us. 01704 SPI spi(SPI1_MOSI, SPI1_MISO, SPI1_SCK, SPI1_SS); // mosi, miso, sclk spi1 TARGET_MAX32625MBED: P1_1 P1_2 P1_0 Arduino 10-pin header D11 D12 D13 D10 01705 DigitalOut spi_cs(NC); // TARGET_MAX32625MBED: not connected 01706 // PinName NC means NOT_CONNECTED; DigitalOut::is_connected() returns false 01707 // add m_cs_pin.is_connected() guard before writing m_cs_pin = m_SPI_cs_state 01708 // to avoid runtime error Assertion failed: obj->name != (PinName)NC 01709 #elif defined(TARGET_MAX32600MBED) 01710 SPI spi(SPI2_MOSI, SPI2_MISO, SPI2_SCK); // mosi, miso, sclk spi1 TARGET_MAX32600MBED: Arduino 10-pin header D11 D12 D13 01711 DigitalOut spi_cs(SPI2_SS); // Generic: Arduino 10-pin header D10 01712 #else 01713 SPI spi(D11, D12, D13); // mosi, miso, sclk spi1 TARGET_MAX32600MBED: Arduino 10-pin header D11 D12 D13 01714 DigitalOut spi_cs(D10); // Generic: Arduino 10-pin header D10 01715 #endif 01716 01717 // example code declare GPIO interface pins 01718 DigitalOut CONVRUN_pin(D9); // Digital Configuration Input to MAX11043 device 01719 DigitalOut SHDN_pin(D8); // Digital Configuration Input to MAX11043 device 01720 DigitalOut DACSTEP_pin(D7); // Digital Configuration Input to MAX11043 device 01721 DigitalOut UP_slash_DWNb_pin(D6); // Digital Configuration Input to MAX11043 device 01722 //-------------------------------------------------- 01723 // MAX11043 ADC Read operations must be synchronized to EOC End Of Conversion 01724 // EOC# asserts low when new data is available. 01725 // Initiate a data read prior to the next rising edge of EOC# or the result is overwritten. 01726 #ifndef MAX11043_EOC_INTERRUPT_POLLING 01727 #define MAX11043_EOC_INTERRUPT_POLLING 1 01728 #endif // MAX11043_EOC_INTERRUPT_POLLING 01729 //-------------------------------------------------- 01730 // MAX11043 ADC Read operations must be synchronized to EOC End Of Conversion 01731 // EOC# asserts low when new data is available. 01732 // Initiate a data read prior to the next rising edge of EOC# or the result is overwritten. 01733 #if MAX11043_EOC_INTERRUPT_POLLING 01734 // MAX11043 EOC End Of Conversion input should be InterruptIn(PinName:EOC_pin).fall(onEOCFallingEdge); 01735 // Workaround using DigitalIn(PinName:EOC_pin) polled to sync with EOC falling edge for ADC reads 01736 // 2020-02-20 MAX11043_EOC_INTERRUPT_POLLING works on MAX32625MBED at 9us conversion rate, with 1us timing margin 01737 DigitalIn EOC_pin(D2); // Digital Event Output from MAX11043 device 01738 #else // MAX11043_EOC_INTERRUPT_POLLING 01739 // MAX11043 EOC End Of Conversion input is InterruptIn(PinName:EOC_pin).fall(onEOCFallingEdge); 01740 InterruptIn EOC_pin(D2); // Digital Event Output from MAX11043 device 01741 #endif // MAX11043_EOC_INTERRUPT_POLLING 01742 //-------------------------------------------------- 01743 // example code declare device instance 01744 MAX11043 g_MAX11043_device(spi, spi_cs, CONVRUN_pin, SHDN_pin, DACSTEP_pin, UP_slash_DWNb_pin, EOC_pin, MAX11043::MAX11043_IC); 01745 01746 01747 //---------------------------------------- 01748 // Global SPI options 01749 // 01750 01751 //-------------------------------------------------- 01752 // Optional Diagnostic function to print SPI transactions 01753 #ifndef MAX11043_ONSPIPRINT 01754 #define MAX11043_ONSPIPRINT 1 01755 #endif // MAX11043_ONSPIPRINT 01756 01757 //---------------------------------------- 01758 // Global I2C options 01759 // 01760 01761 #define APPLICATION_ArduinoPinsMonitor 1 01762 01763 //-------------------------------------------------- 01764 // use BUTTON1 trigger some action 01765 #if defined(TARGET_MAX32630) 01766 #define HAS_BUTTON1_DEMO_INTERRUPT 1 01767 #define HAS_BUTTON2_DEMO 0 01768 #define HAS_BUTTON2_DEMO_INTERRUPT 0 01769 #elif defined(TARGET_MAX32625PICO) 01770 #warning "TARGET_MAX32625PICO not previously tested; need to define buttons..." 01771 #define HAS_BUTTON1_DEMO_INTERRUPT 1 01772 #define HAS_BUTTON2_DEMO 0 01773 #define HAS_BUTTON2_DEMO_INTERRUPT 0 01774 #elif defined(TARGET_MAX32625) 01775 #define HAS_BUTTON1_DEMO_INTERRUPT 1 01776 #define HAS_BUTTON2_DEMO_INTERRUPT 1 01777 #elif defined(TARGET_MAX32620FTHR) 01778 #warning "TARGET_MAX32620FTHR not previously tested; need to define buttons..." 01779 #define BUTTON1 SW1 01780 #define HAS_BUTTON1_DEMO_INTERRUPT 1 01781 #define HAS_BUTTON2_DEMO 0 01782 #define HAS_BUTTON2_DEMO_INTERRUPT 0 01783 #elif defined(TARGET_NUCLEO_F446RE) 01784 #define HAS_BUTTON1_DEMO_INTERRUPT 0 01785 #define HAS_BUTTON2_DEMO_INTERRUPT 0 01786 #elif defined(TARGET_NUCLEO_F401RE) 01787 #define HAS_BUTTON1_DEMO_INTERRUPT 0 01788 #define HAS_BUTTON2_DEMO_INTERRUPT 0 01789 #else 01790 #warning "target not previously tested; need to define buttons..." 01791 #endif 01792 // 01793 #ifndef HAS_BUTTON1_DEMO 01794 #define HAS_BUTTON1_DEMO 0 01795 #endif 01796 #ifndef HAS_BUTTON2_DEMO 01797 #define HAS_BUTTON2_DEMO 0 01798 #endif 01799 // 01800 // avoid runtime error on button1 press [mbed-os-5.11] 01801 // instead of using InterruptIn, use DigitalIn and poll in main while(1) 01802 #ifndef HAS_BUTTON1_DEMO_INTERRUPT_POLLING 01803 #define HAS_BUTTON1_DEMO_INTERRUPT_POLLING 1 01804 #endif 01805 // 01806 #ifndef HAS_BUTTON1_DEMO_INTERRUPT 01807 #define HAS_BUTTON1_DEMO_INTERRUPT 1 01808 #endif 01809 #ifndef HAS_BUTTON2_DEMO_INTERRUPT 01810 #define HAS_BUTTON2_DEMO_INTERRUPT 1 01811 #endif 01812 // 01813 #if HAS_BUTTON1_DEMO_INTERRUPT 01814 # if HAS_BUTTON1_DEMO_INTERRUPT_POLLING 01815 // avoid runtime error on button1 press [mbed-os-5.11] 01816 // instead of using InterruptIn, use DigitalIn and poll in main while(1) 01817 DigitalIn button1(BUTTON1); 01818 # else 01819 InterruptIn button1(BUTTON1); 01820 # endif 01821 #elif HAS_BUTTON1_DEMO 01822 DigitalIn button1(BUTTON1); 01823 #endif 01824 #if HAS_BUTTON2_DEMO_INTERRUPT 01825 # if HAS_BUTTON1_DEMO_INTERRUPT_POLLING 01826 // avoid runtime error on button1 press [mbed-os-5.11] 01827 // instead of using InterruptIn, use DigitalIn and poll in main while(1) 01828 DigitalIn button2(BUTTON2); 01829 # else 01830 InterruptIn button2(BUTTON2); 01831 # endif 01832 #elif HAS_BUTTON2_DEMO 01833 DigitalIn button2(BUTTON2); 01834 #endif 01835 01836 //-------------------------------------------------- 01837 // functions tested by SelfTest() 01838 extern double fn_MAX11043_ADCVoltageOfCode_16bit(uint32_t value_u16); 01839 extern double fn_MAX11043_ADCVoltageOfCode_24bit(uint32_t value_u24); 01840 01841 //-------------------------------------------------- 01842 // optional self-test groups for function SelfTest() 01843 // enable by changing the #define value from 0 to 1 01844 #ifndef MAX11043_SELFTEST_BIP2C16 01845 #define MAX11043_SELFTEST_BIP2C16 1 01846 #endif 01847 01848 #ifndef MAX11043_SELFTEST_BIP2C24 01849 #define MAX11043_SELFTEST_BIP2C24 1 01850 #endif 01851 01852 //-------------------------------------------------- 01853 // When user presses button BUTTON1, perform self test 01854 #if HAS_BUTTON1_DEMO_INTERRUPT 01855 void onButton1FallingEdge(void) 01856 { 01857 void SelfTest(CmdLine & cmdLine); 01858 SelfTest(cmdLine_serial); 01859 } 01860 #endif // HAS_BUTTON1_DEMO_INTERRUPT 01861 01862 //-------------------------------------------------- 01863 // When user presses button BUTTON2, perform demo configuration 01864 #if HAS_BUTTON2_DEMO_INTERRUPT 01865 void onButton2FallingEdge(void) 01866 { 01867 // TBD demo configuration 01868 // CODE GENERATOR: example code: member function Configure_Demo 01869 g_MAX11043_device.Configure_Demo(); 01870 01871 // TODO diagnostic LED 01872 led1 = LED_OFF; led2 = LED_OFF; led3 = LED_ON; // diagnostic rbg led BLUE 01873 } 01874 #endif // HAS_BUTTON2_DEMO_INTERRUPT 01875 01876 //-------------------------------------------------- 01877 void SelfTest(CmdLine & cmdLine) 01878 { 01879 //-------------------------------------------------- 01880 #if analogIn4_IS_HIGH_RANGE_OF_analogIn0 01881 // Platform board uses AIN4,AIN5,.. as high range of AIN0,AIN1,.. 01882 MaximTinyTester tinyTester(cmdLine, analogIn4, analogIn5, analogIn2, analogIn3, analogIn0, analogIn4, led1_RFailLED, led2_GPassLED, led3_BBusyLED); 01883 tinyTester.analogInPin_fullScaleVoltage[0] = analogInPin_fullScaleVoltage[4]; // board support 01884 tinyTester.analogInPin_fullScaleVoltage[1] = analogInPin_fullScaleVoltage[5]; // board support 01885 tinyTester.analogInPin_fullScaleVoltage[2] = analogInPin_fullScaleVoltage[2]; // board support 01886 tinyTester.analogInPin_fullScaleVoltage[3] = analogInPin_fullScaleVoltage[3]; // board support 01887 tinyTester.analogInPin_fullScaleVoltage[4] = analogInPin_fullScaleVoltage[0]; // board support 01888 tinyTester.analogInPin_fullScaleVoltage[5] = analogInPin_fullScaleVoltage[1]; // board support 01889 // low range channels AIN0, AIN1, AIN2, AIN3 01890 #else // analogIn4_IS_HIGH_RANGE_OF_analogIn0 01891 // Platform board uses simple analog inputs 01892 MaximTinyTester tinyTester(cmdLine, analogIn0, analogIn1, analogIn2, analogIn3, analogIn4, analogIn5, led1_RFailLED, led2_GPassLED, led3_BBusyLED); 01893 tinyTester.analogInPin_fullScaleVoltage[0] = analogInPin_fullScaleVoltage[0]; // board support 01894 tinyTester.analogInPin_fullScaleVoltage[1] = analogInPin_fullScaleVoltage[1]; // board support 01895 tinyTester.analogInPin_fullScaleVoltage[2] = analogInPin_fullScaleVoltage[2]; // board support 01896 tinyTester.analogInPin_fullScaleVoltage[3] = analogInPin_fullScaleVoltage[3]; // board support 01897 tinyTester.analogInPin_fullScaleVoltage[4] = analogInPin_fullScaleVoltage[4]; // board support 01898 tinyTester.analogInPin_fullScaleVoltage[5] = analogInPin_fullScaleVoltage[5]; // board support 01899 #endif 01900 tinyTester.clear(); 01901 01902 // CODE GENERATOR: generate SelfTest based on function docstrings @test lines 01903 01904 // CODE GENERATOR: SelfTest ADCVoltageOfCode_16bit @test group BIP2C16 ADCVoltageOfCode_16bit(0x7FFF) expect 2.500 within 0.030 Full Scale 01905 #if MAX11043_SELFTEST_BIP2C16 // group BIP2C16 ADCVoltageOfCode_16bit Full Scale 01906 // ASSERT_EQ(g_MAX11043_device.ADCVoltageOfCode_16bit(0x7FFF), 2.500); // Full Scale 01907 extern double fn_MAX11043_ADCVoltageOfCode_16bit(uint32_t value_u16); 01908 tinyTester.err_threshold = 0.030; // within 0.030 01909 tinyTester.FunctionCall_Expect("MAX11043.ADCVoltageOfCode_16bit", fn_MAX11043_ADCVoltageOfCode_16bit, 0x7FFF, 2.500); // Full Scale 01910 #endif // MAX11043_SELFTEST_BIP2C16 // group BIP2C16 01911 01912 // CODE GENERATOR: SelfTest ADCVoltageOfCode_16bit @test group BIP2C16 ADCVoltageOfCode_16bit(0x7FFF) expect 2.500 Full Scale 01913 #if MAX11043_SELFTEST_BIP2C16 // group BIP2C16 ADCVoltageOfCode_16bit Full Scale 01914 // ASSERT_EQ(g_MAX11043_device.ADCVoltageOfCode_16bit(0x7FFF), 2.500); // Full Scale 01915 extern double fn_MAX11043_ADCVoltageOfCode_16bit(uint32_t value_u16); 01916 tinyTester.FunctionCall_Expect("MAX11043.ADCVoltageOfCode_16bit", fn_MAX11043_ADCVoltageOfCode_16bit, 0x7FFF, 2.500); // Full Scale 01917 #endif // MAX11043_SELFTEST_BIP2C16 // group BIP2C16 01918 01919 // CODE GENERATOR: SelfTest ADCVoltageOfCode_16bit @test group BIP2C16 ADCVoltageOfCode_16bit(0x6666) expect 2.000 Two Volts 01920 #if MAX11043_SELFTEST_BIP2C16 // group BIP2C16 ADCVoltageOfCode_16bit Two Volts 01921 // ASSERT_EQ(g_MAX11043_device.ADCVoltageOfCode_16bit(0x6666), 2.000); // Two Volts 01922 extern double fn_MAX11043_ADCVoltageOfCode_16bit(uint32_t value_u16); 01923 tinyTester.FunctionCall_Expect("MAX11043.ADCVoltageOfCode_16bit", fn_MAX11043_ADCVoltageOfCode_16bit, 0x6666, 2.000); // Two Volts 01924 #endif // MAX11043_SELFTEST_BIP2C16 // group BIP2C16 01925 01926 // CODE GENERATOR: SelfTest ADCVoltageOfCode_16bit @test group BIP2C16 ADCVoltageOfCode_16bit(0x6000) expect 1.875 75% Scale 01927 #if MAX11043_SELFTEST_BIP2C16 // group BIP2C16 ADCVoltageOfCode_16bit 75% Scale 01928 // ASSERT_EQ(g_MAX11043_device.ADCVoltageOfCode_16bit(0x6000), 1.875); // 75% Scale 01929 extern double fn_MAX11043_ADCVoltageOfCode_16bit(uint32_t value_u16); 01930 tinyTester.FunctionCall_Expect("MAX11043.ADCVoltageOfCode_16bit", fn_MAX11043_ADCVoltageOfCode_16bit, 0x6000, 1.875); // 75% Scale 01931 #endif // MAX11043_SELFTEST_BIP2C16 // group BIP2C16 01932 01933 // CODE GENERATOR: SelfTest ADCVoltageOfCode_16bit @test group BIP2C16 ADCVoltageOfCode_16bit(0x4000) expect 1.250 Mid Scale 01934 #if MAX11043_SELFTEST_BIP2C16 // group BIP2C16 ADCVoltageOfCode_16bit Mid Scale 01935 // ASSERT_EQ(g_MAX11043_device.ADCVoltageOfCode_16bit(0x4000), 1.250); // Mid Scale 01936 extern double fn_MAX11043_ADCVoltageOfCode_16bit(uint32_t value_u16); 01937 tinyTester.FunctionCall_Expect("MAX11043.ADCVoltageOfCode_16bit", fn_MAX11043_ADCVoltageOfCode_16bit, 0x4000, 1.250); // Mid Scale 01938 #endif // MAX11043_SELFTEST_BIP2C16 // group BIP2C16 01939 01940 // CODE GENERATOR: SelfTest ADCVoltageOfCode_16bit @test group BIP2C16 ADCVoltageOfCode_16bit(0x3333) expect 1.000 One Volt 01941 #if MAX11043_SELFTEST_BIP2C16 // group BIP2C16 ADCVoltageOfCode_16bit One Volt 01942 // ASSERT_EQ(g_MAX11043_device.ADCVoltageOfCode_16bit(0x3333), 1.000); // One Volt 01943 extern double fn_MAX11043_ADCVoltageOfCode_16bit(uint32_t value_u16); 01944 tinyTester.FunctionCall_Expect("MAX11043.ADCVoltageOfCode_16bit", fn_MAX11043_ADCVoltageOfCode_16bit, 0x3333, 1.000); // One Volt 01945 #endif // MAX11043_SELFTEST_BIP2C16 // group BIP2C16 01946 01947 // CODE GENERATOR: SelfTest ADCVoltageOfCode_16bit @test group BIP2C16 ADCVoltageOfCode_16bit(0x2000) expect 0.625 25% Scale 01948 #if MAX11043_SELFTEST_BIP2C16 // group BIP2C16 ADCVoltageOfCode_16bit 25% Scale 01949 // ASSERT_EQ(g_MAX11043_device.ADCVoltageOfCode_16bit(0x2000), 0.625); // 25% Scale 01950 extern double fn_MAX11043_ADCVoltageOfCode_16bit(uint32_t value_u16); 01951 tinyTester.FunctionCall_Expect("MAX11043.ADCVoltageOfCode_16bit", fn_MAX11043_ADCVoltageOfCode_16bit, 0x2000, 0.625); // 25% Scale 01952 #endif // MAX11043_SELFTEST_BIP2C16 // group BIP2C16 01953 01954 // CODE GENERATOR: SelfTest ADCVoltageOfCode_16bit @test group BIP2C16 ADCVoltageOfCode_16bit(0x051e) expect 0.100 100mV 01955 #if MAX11043_SELFTEST_BIP2C16 // group BIP2C16 ADCVoltageOfCode_16bit 100mV 01956 // ASSERT_EQ(g_MAX11043_device.ADCVoltageOfCode_16bit(0x051e), 0.100); // 100mV 01957 extern double fn_MAX11043_ADCVoltageOfCode_16bit(uint32_t value_u16); 01958 tinyTester.FunctionCall_Expect("MAX11043.ADCVoltageOfCode_16bit", fn_MAX11043_ADCVoltageOfCode_16bit, 0x051e, 0.100); // 100mV 01959 #endif // MAX11043_SELFTEST_BIP2C16 // group BIP2C16 01960 01961 // CODE GENERATOR: SelfTest ADCVoltageOfCode_16bit @test group BIP2C16 ADCVoltageOfCode_16bit(0x0000) expect 0.00000894069671 Three LSB 01962 #if MAX11043_SELFTEST_BIP2C16 // group BIP2C16 ADCVoltageOfCode_16bit Three LSB 01963 // ASSERT_EQ(g_MAX11043_device.ADCVoltageOfCode_16bit(0x0000), 0.00000894069671); // Three LSB 01964 extern double fn_MAX11043_ADCVoltageOfCode_16bit(uint32_t value_u16); 01965 tinyTester.FunctionCall_Expect("MAX11043.ADCVoltageOfCode_16bit", fn_MAX11043_ADCVoltageOfCode_16bit, 0x0000, 0.00000894069671); // Three LSB 01966 #endif // MAX11043_SELFTEST_BIP2C16 // group BIP2C16 01967 01968 // CODE GENERATOR: SelfTest ADCVoltageOfCode_16bit @test group BIP2C16 ADCVoltageOfCode_16bit(0x0000) expect 0.00000596046447 Two LSB 01969 #if MAX11043_SELFTEST_BIP2C16 // group BIP2C16 ADCVoltageOfCode_16bit Two LSB 01970 // ASSERT_EQ(g_MAX11043_device.ADCVoltageOfCode_16bit(0x0000), 0.00000596046447); // Two LSB 01971 extern double fn_MAX11043_ADCVoltageOfCode_16bit(uint32_t value_u16); 01972 tinyTester.FunctionCall_Expect("MAX11043.ADCVoltageOfCode_16bit", fn_MAX11043_ADCVoltageOfCode_16bit, 0x0000, 0.00000596046447); // Two LSB 01973 #endif // MAX11043_SELFTEST_BIP2C16 // group BIP2C16 01974 01975 // CODE GENERATOR: SelfTest ADCVoltageOfCode_16bit @test group BIP2C16 ADCVoltageOfCode_16bit(0x0000) expect 0.0000029802326 One LSB 01976 #if MAX11043_SELFTEST_BIP2C16 // group BIP2C16 ADCVoltageOfCode_16bit One LSB 01977 // ASSERT_EQ(g_MAX11043_device.ADCVoltageOfCode_16bit(0x0000), 0.0000029802326); // One LSB 01978 extern double fn_MAX11043_ADCVoltageOfCode_16bit(uint32_t value_u16); 01979 tinyTester.FunctionCall_Expect("MAX11043.ADCVoltageOfCode_16bit", fn_MAX11043_ADCVoltageOfCode_16bit, 0x0000, 0.0000029802326); // One LSB 01980 #endif // MAX11043_SELFTEST_BIP2C16 // group BIP2C16 01981 01982 // CODE GENERATOR: SelfTest ADCVoltageOfCode_16bit @test group BIP2C16 ADCVoltageOfCode_16bit(0x0000) expect 0.0 Zero Scale 01983 #if MAX11043_SELFTEST_BIP2C16 // group BIP2C16 ADCVoltageOfCode_16bit Zero Scale 01984 // ASSERT_EQ(g_MAX11043_device.ADCVoltageOfCode_16bit(0x0000), 0.0); // Zero Scale 01985 extern double fn_MAX11043_ADCVoltageOfCode_16bit(uint32_t value_u16); 01986 tinyTester.FunctionCall_Expect("MAX11043.ADCVoltageOfCode_16bit", fn_MAX11043_ADCVoltageOfCode_16bit, 0x0000, 0.0); // Zero Scale 01987 #endif // MAX11043_SELFTEST_BIP2C16 // group BIP2C16 01988 01989 // CODE GENERATOR: SelfTest ADCVoltageOfCode_16bit @test group BIP2C16 ADCVoltageOfCode_16bit(0xFFFF) expect -0.0000029802326 Negative One LSB 01990 #if MAX11043_SELFTEST_BIP2C16 // group BIP2C16 ADCVoltageOfCode_16bit Negative One LSB 01991 // ASSERT_EQ(g_MAX11043_device.ADCVoltageOfCode_16bit(0xFFFF), -0.0000029802326); // Negative One LSB 01992 extern double fn_MAX11043_ADCVoltageOfCode_16bit(uint32_t value_u16); 01993 tinyTester.FunctionCall_Expect("MAX11043.ADCVoltageOfCode_16bit", fn_MAX11043_ADCVoltageOfCode_16bit, 0xFFFF, -0.0000029802326); // Negative One LSB 01994 #endif // MAX11043_SELFTEST_BIP2C16 // group BIP2C16 01995 01996 // CODE GENERATOR: SelfTest ADCVoltageOfCode_16bit @test group BIP2C16 ADCVoltageOfCode_16bit(0xFFFF) expect -0.0000059604644 Negative Two LSB 01997 #if MAX11043_SELFTEST_BIP2C16 // group BIP2C16 ADCVoltageOfCode_16bit Negative Two LSB 01998 // ASSERT_EQ(g_MAX11043_device.ADCVoltageOfCode_16bit(0xFFFF), -0.0000059604644); // Negative Two LSB 01999 extern double fn_MAX11043_ADCVoltageOfCode_16bit(uint32_t value_u16); 02000 tinyTester.FunctionCall_Expect("MAX11043.ADCVoltageOfCode_16bit", fn_MAX11043_ADCVoltageOfCode_16bit, 0xFFFF, -0.0000059604644); // Negative Two LSB 02001 #endif // MAX11043_SELFTEST_BIP2C16 // group BIP2C16 02002 02003 // CODE GENERATOR: SelfTest ADCVoltageOfCode_16bit @test group BIP2C16 ADCVoltageOfCode_16bit(0xFFFF) expect -0.0000089406967 Negative Three LSB 02004 #if MAX11043_SELFTEST_BIP2C16 // group BIP2C16 ADCVoltageOfCode_16bit Negative Three LSB 02005 // ASSERT_EQ(g_MAX11043_device.ADCVoltageOfCode_16bit(0xFFFF), -0.0000089406967); // Negative Three LSB 02006 extern double fn_MAX11043_ADCVoltageOfCode_16bit(uint32_t value_u16); 02007 tinyTester.FunctionCall_Expect("MAX11043.ADCVoltageOfCode_16bit", fn_MAX11043_ADCVoltageOfCode_16bit, 0xFFFF, -0.0000089406967); // Negative Three LSB 02008 #endif // MAX11043_SELFTEST_BIP2C16 // group BIP2C16 02009 02010 // CODE GENERATOR: SelfTest ADCVoltageOfCode_16bit @test group BIP2C16 ADCVoltageOfCode_16bit(0xFAE1) expect -0.100 Negative 100mV 02011 #if MAX11043_SELFTEST_BIP2C16 // group BIP2C16 ADCVoltageOfCode_16bit Negative 100mV 02012 // ASSERT_EQ(g_MAX11043_device.ADCVoltageOfCode_16bit(0xFAE1), -0.100); // Negative 100mV 02013 extern double fn_MAX11043_ADCVoltageOfCode_16bit(uint32_t value_u16); 02014 tinyTester.FunctionCall_Expect("MAX11043.ADCVoltageOfCode_16bit", fn_MAX11043_ADCVoltageOfCode_16bit, 0xFAE1, -0.100); // Negative 100mV 02015 #endif // MAX11043_SELFTEST_BIP2C16 // group BIP2C16 02016 02017 // CODE GENERATOR: SelfTest ADCVoltageOfCode_16bit @test group BIP2C16 ADCVoltageOfCode_16bit(0xE000) expect -0.625 Negative 25% Scale 02018 #if MAX11043_SELFTEST_BIP2C16 // group BIP2C16 ADCVoltageOfCode_16bit Negative 25% Scale 02019 // ASSERT_EQ(g_MAX11043_device.ADCVoltageOfCode_16bit(0xE000), -0.625); // Negative 25% Scale 02020 extern double fn_MAX11043_ADCVoltageOfCode_16bit(uint32_t value_u16); 02021 tinyTester.FunctionCall_Expect("MAX11043.ADCVoltageOfCode_16bit", fn_MAX11043_ADCVoltageOfCode_16bit, 0xE000, -0.625); // Negative 25% Scale 02022 #endif // MAX11043_SELFTEST_BIP2C16 // group BIP2C16 02023 02024 // CODE GENERATOR: SelfTest ADCVoltageOfCode_16bit @test group BIP2C16 ADCVoltageOfCode_16bit(0xCCCC) expect -1.000 Negative One Volt 02025 #if MAX11043_SELFTEST_BIP2C16 // group BIP2C16 ADCVoltageOfCode_16bit Negative One Volt 02026 // ASSERT_EQ(g_MAX11043_device.ADCVoltageOfCode_16bit(0xCCCC), -1.000); // Negative One Volt 02027 extern double fn_MAX11043_ADCVoltageOfCode_16bit(uint32_t value_u16); 02028 tinyTester.FunctionCall_Expect("MAX11043.ADCVoltageOfCode_16bit", fn_MAX11043_ADCVoltageOfCode_16bit, 0xCCCC, -1.000); // Negative One Volt 02029 #endif // MAX11043_SELFTEST_BIP2C16 // group BIP2C16 02030 02031 // CODE GENERATOR: SelfTest ADCVoltageOfCode_16bit @test group BIP2C16 ADCVoltageOfCode_16bit(0xC000) expect -1.250 Negative Mid Scale 02032 #if MAX11043_SELFTEST_BIP2C16 // group BIP2C16 ADCVoltageOfCode_16bit Negative Mid Scale 02033 // ASSERT_EQ(g_MAX11043_device.ADCVoltageOfCode_16bit(0xC000), -1.250); // Negative Mid Scale 02034 extern double fn_MAX11043_ADCVoltageOfCode_16bit(uint32_t value_u16); 02035 tinyTester.FunctionCall_Expect("MAX11043.ADCVoltageOfCode_16bit", fn_MAX11043_ADCVoltageOfCode_16bit, 0xC000, -1.250); // Negative Mid Scale 02036 #endif // MAX11043_SELFTEST_BIP2C16 // group BIP2C16 02037 02038 // CODE GENERATOR: SelfTest ADCVoltageOfCode_16bit @test group BIP2C16 ADCVoltageOfCode_16bit(0xA000) expect -1.875 Negative 75% Scale 02039 #if MAX11043_SELFTEST_BIP2C16 // group BIP2C16 ADCVoltageOfCode_16bit Negative 75% Scale 02040 // ASSERT_EQ(g_MAX11043_device.ADCVoltageOfCode_16bit(0xA000), -1.875); // Negative 75% Scale 02041 extern double fn_MAX11043_ADCVoltageOfCode_16bit(uint32_t value_u16); 02042 tinyTester.FunctionCall_Expect("MAX11043.ADCVoltageOfCode_16bit", fn_MAX11043_ADCVoltageOfCode_16bit, 0xA000, -1.875); // Negative 75% Scale 02043 #endif // MAX11043_SELFTEST_BIP2C16 // group BIP2C16 02044 02045 // CODE GENERATOR: SelfTest ADCVoltageOfCode_16bit @test group BIP2C16 ADCVoltageOfCode_16bit(0x9999) expect -2.000 Negative Two Volts 02046 #if MAX11043_SELFTEST_BIP2C16 // group BIP2C16 ADCVoltageOfCode_16bit Negative Two Volts 02047 // ASSERT_EQ(g_MAX11043_device.ADCVoltageOfCode_16bit(0x9999), -2.000); // Negative Two Volts 02048 extern double fn_MAX11043_ADCVoltageOfCode_16bit(uint32_t value_u16); 02049 tinyTester.FunctionCall_Expect("MAX11043.ADCVoltageOfCode_16bit", fn_MAX11043_ADCVoltageOfCode_16bit, 0x9999, -2.000); // Negative Two Volts 02050 #endif // MAX11043_SELFTEST_BIP2C16 // group BIP2C16 02051 02052 // CODE GENERATOR: SelfTest ADCVoltageOfCode_16bit @test group BIP2C16 ADCVoltageOfCode_16bit(0x8000) expect -2.500 Negative Full Scale 02053 #if MAX11043_SELFTEST_BIP2C16 // group BIP2C16 ADCVoltageOfCode_16bit Negative Full Scale 02054 // ASSERT_EQ(g_MAX11043_device.ADCVoltageOfCode_16bit(0x8000), -2.500); // Negative Full Scale 02055 extern double fn_MAX11043_ADCVoltageOfCode_16bit(uint32_t value_u16); 02056 tinyTester.FunctionCall_Expect("MAX11043.ADCVoltageOfCode_16bit", fn_MAX11043_ADCVoltageOfCode_16bit, 0x8000, -2.500); // Negative Full Scale 02057 #endif // MAX11043_SELFTEST_BIP2C16 // group BIP2C16 02058 02059 // CODE GENERATOR: SelfTest ADCVoltageOfCode_16bit @test group BIP2C16 ADCVoltageOfCode_16bit(0x8000) expect -2.500 Negative Full Scale 02060 #if MAX11043_SELFTEST_BIP2C16 // group BIP2C16 ADCVoltageOfCode_16bit Negative Full Scale 02061 // ASSERT_EQ(g_MAX11043_device.ADCVoltageOfCode_16bit(0x8000), -2.500); // Negative Full Scale 02062 extern double fn_MAX11043_ADCVoltageOfCode_16bit(uint32_t value_u16); 02063 tinyTester.FunctionCall_Expect("MAX11043.ADCVoltageOfCode_16bit", fn_MAX11043_ADCVoltageOfCode_16bit, 0x8000, -2.500); // Negative Full Scale 02064 #endif // MAX11043_SELFTEST_BIP2C16 // group BIP2C16 02065 02066 // CODE GENERATOR: SelfTest ADCVoltageOfCode_24bit @test group BIP2C24 ADCVoltageOfCode_24bit(0x7FFFFF) expect 2.500 within 0.030 Full Scale 02067 #if MAX11043_SELFTEST_BIP2C24 // group BIP2C24 ADCVoltageOfCode_24bit Full Scale 02068 // ASSERT_EQ(g_MAX11043_device.ADCVoltageOfCode_24bit(0x7FFFFF), 2.500); // Full Scale 02069 extern double fn_MAX11043_ADCVoltageOfCode_24bit(uint32_t value_u24); 02070 tinyTester.err_threshold = 0.030; // within 0.030 02071 tinyTester.FunctionCall_Expect("MAX11043.ADCVoltageOfCode_24bit", fn_MAX11043_ADCVoltageOfCode_24bit, 0x7FFFFF, 2.500); // Full Scale 02072 #endif // MAX11043_SELFTEST_BIP2C24 // group BIP2C24 02073 02074 // CODE GENERATOR: SelfTest ADCVoltageOfCode_24bit @test group BIP2C24 ADCVoltageOfCode_24bit(0x7FFFFE) expect 2.500 Full Scale 02075 #if MAX11043_SELFTEST_BIP2C24 // group BIP2C24 ADCVoltageOfCode_24bit Full Scale 02076 // ASSERT_EQ(g_MAX11043_device.ADCVoltageOfCode_24bit(0x7FFFFE), 2.500); // Full Scale 02077 extern double fn_MAX11043_ADCVoltageOfCode_24bit(uint32_t value_u24); 02078 tinyTester.FunctionCall_Expect("MAX11043.ADCVoltageOfCode_24bit", fn_MAX11043_ADCVoltageOfCode_24bit, 0x7FFFFE, 2.500); // Full Scale 02079 #endif // MAX11043_SELFTEST_BIP2C24 // group BIP2C24 02080 02081 // CODE GENERATOR: SelfTest ADCVoltageOfCode_24bit @test group BIP2C24 ADCVoltageOfCode_24bit(0x666666) expect 2.000 Two Volts 02082 #if MAX11043_SELFTEST_BIP2C24 // group BIP2C24 ADCVoltageOfCode_24bit Two Volts 02083 // ASSERT_EQ(g_MAX11043_device.ADCVoltageOfCode_24bit(0x666666), 2.000); // Two Volts 02084 extern double fn_MAX11043_ADCVoltageOfCode_24bit(uint32_t value_u24); 02085 tinyTester.FunctionCall_Expect("MAX11043.ADCVoltageOfCode_24bit", fn_MAX11043_ADCVoltageOfCode_24bit, 0x666666, 2.000); // Two Volts 02086 #endif // MAX11043_SELFTEST_BIP2C24 // group BIP2C24 02087 02088 // CODE GENERATOR: SelfTest ADCVoltageOfCode_24bit @test group BIP2C24 ADCVoltageOfCode_24bit(0x600000) expect 1.875 75% Scale 02089 #if MAX11043_SELFTEST_BIP2C24 // group BIP2C24 ADCVoltageOfCode_24bit 75% Scale 02090 // ASSERT_EQ(g_MAX11043_device.ADCVoltageOfCode_24bit(0x600000), 1.875); // 75% Scale 02091 extern double fn_MAX11043_ADCVoltageOfCode_24bit(uint32_t value_u24); 02092 tinyTester.FunctionCall_Expect("MAX11043.ADCVoltageOfCode_24bit", fn_MAX11043_ADCVoltageOfCode_24bit, 0x600000, 1.875); // 75% Scale 02093 #endif // MAX11043_SELFTEST_BIP2C24 // group BIP2C24 02094 02095 // CODE GENERATOR: SelfTest ADCVoltageOfCode_24bit @test group BIP2C24 ADCVoltageOfCode_24bit(0x400000) expect 1.250 Mid Scale 02096 #if MAX11043_SELFTEST_BIP2C24 // group BIP2C24 ADCVoltageOfCode_24bit Mid Scale 02097 // ASSERT_EQ(g_MAX11043_device.ADCVoltageOfCode_24bit(0x400000), 1.250); // Mid Scale 02098 extern double fn_MAX11043_ADCVoltageOfCode_24bit(uint32_t value_u24); 02099 tinyTester.FunctionCall_Expect("MAX11043.ADCVoltageOfCode_24bit", fn_MAX11043_ADCVoltageOfCode_24bit, 0x400000, 1.250); // Mid Scale 02100 #endif // MAX11043_SELFTEST_BIP2C24 // group BIP2C24 02101 02102 // CODE GENERATOR: SelfTest ADCVoltageOfCode_24bit @test group BIP2C24 ADCVoltageOfCode_24bit(0x333333) expect 1.000 One Volt 02103 #if MAX11043_SELFTEST_BIP2C24 // group BIP2C24 ADCVoltageOfCode_24bit One Volt 02104 // ASSERT_EQ(g_MAX11043_device.ADCVoltageOfCode_24bit(0x333333), 1.000); // One Volt 02105 extern double fn_MAX11043_ADCVoltageOfCode_24bit(uint32_t value_u24); 02106 tinyTester.FunctionCall_Expect("MAX11043.ADCVoltageOfCode_24bit", fn_MAX11043_ADCVoltageOfCode_24bit, 0x333333, 1.000); // One Volt 02107 #endif // MAX11043_SELFTEST_BIP2C24 // group BIP2C24 02108 02109 // CODE GENERATOR: SelfTest ADCVoltageOfCode_24bit @test group BIP2C24 ADCVoltageOfCode_24bit(0x200000) expect 0.625 25% Scale 02110 #if MAX11043_SELFTEST_BIP2C24 // group BIP2C24 ADCVoltageOfCode_24bit 25% Scale 02111 // ASSERT_EQ(g_MAX11043_device.ADCVoltageOfCode_24bit(0x200000), 0.625); // 25% Scale 02112 extern double fn_MAX11043_ADCVoltageOfCode_24bit(uint32_t value_u24); 02113 tinyTester.FunctionCall_Expect("MAX11043.ADCVoltageOfCode_24bit", fn_MAX11043_ADCVoltageOfCode_24bit, 0x200000, 0.625); // 25% Scale 02114 #endif // MAX11043_SELFTEST_BIP2C24 // group BIP2C24 02115 02116 // CODE GENERATOR: SelfTest ADCVoltageOfCode_24bit @test group BIP2C24 ADCVoltageOfCode_24bit(0x051eb8) expect 0.100 100mV 02117 #if MAX11043_SELFTEST_BIP2C24 // group BIP2C24 ADCVoltageOfCode_24bit 100mV 02118 // ASSERT_EQ(g_MAX11043_device.ADCVoltageOfCode_24bit(0x051eb8), 0.100); // 100mV 02119 extern double fn_MAX11043_ADCVoltageOfCode_24bit(uint32_t value_u24); 02120 tinyTester.FunctionCall_Expect("MAX11043.ADCVoltageOfCode_24bit", fn_MAX11043_ADCVoltageOfCode_24bit, 0x051eb8, 0.100); // 100mV 02121 #endif // MAX11043_SELFTEST_BIP2C24 // group BIP2C24 02122 02123 // CODE GENERATOR: SelfTest ADCVoltageOfCode_24bit @test group BIP2C24 ADCVoltageOfCode_24bit(0x000003) expect 0.00000894069671 Three LSB 02124 #if MAX11043_SELFTEST_BIP2C24 // group BIP2C24 ADCVoltageOfCode_24bit Three LSB 02125 // ASSERT_EQ(g_MAX11043_device.ADCVoltageOfCode_24bit(0x000003), 0.00000894069671); // Three LSB 02126 extern double fn_MAX11043_ADCVoltageOfCode_24bit(uint32_t value_u24); 02127 tinyTester.FunctionCall_Expect("MAX11043.ADCVoltageOfCode_24bit", fn_MAX11043_ADCVoltageOfCode_24bit, 0x000003, 0.00000894069671); // Three LSB 02128 #endif // MAX11043_SELFTEST_BIP2C24 // group BIP2C24 02129 02130 // CODE GENERATOR: SelfTest ADCVoltageOfCode_24bit @test group BIP2C24 ADCVoltageOfCode_24bit(0x000002) expect 0.00000596046447 Two LSB 02131 #if MAX11043_SELFTEST_BIP2C24 // group BIP2C24 ADCVoltageOfCode_24bit Two LSB 02132 // ASSERT_EQ(g_MAX11043_device.ADCVoltageOfCode_24bit(0x000002), 0.00000596046447); // Two LSB 02133 extern double fn_MAX11043_ADCVoltageOfCode_24bit(uint32_t value_u24); 02134 tinyTester.FunctionCall_Expect("MAX11043.ADCVoltageOfCode_24bit", fn_MAX11043_ADCVoltageOfCode_24bit, 0x000002, 0.00000596046447); // Two LSB 02135 #endif // MAX11043_SELFTEST_BIP2C24 // group BIP2C24 02136 02137 // CODE GENERATOR: SelfTest ADCVoltageOfCode_24bit @test group BIP2C24 ADCVoltageOfCode_24bit(0x000001) expect 0.0000029802326 One LSB 02138 #if MAX11043_SELFTEST_BIP2C24 // group BIP2C24 ADCVoltageOfCode_24bit One LSB 02139 // ASSERT_EQ(g_MAX11043_device.ADCVoltageOfCode_24bit(0x000001), 0.0000029802326); // One LSB 02140 extern double fn_MAX11043_ADCVoltageOfCode_24bit(uint32_t value_u24); 02141 tinyTester.FunctionCall_Expect("MAX11043.ADCVoltageOfCode_24bit", fn_MAX11043_ADCVoltageOfCode_24bit, 0x000001, 0.0000029802326); // One LSB 02142 #endif // MAX11043_SELFTEST_BIP2C24 // group BIP2C24 02143 02144 // CODE GENERATOR: SelfTest ADCVoltageOfCode_24bit @test group BIP2C24 ADCVoltageOfCode_24bit(0x000000) expect 0.0 Zero Scale 02145 #if MAX11043_SELFTEST_BIP2C24 // group BIP2C24 ADCVoltageOfCode_24bit Zero Scale 02146 // ASSERT_EQ(g_MAX11043_device.ADCVoltageOfCode_24bit(0x000000), 0.0); // Zero Scale 02147 extern double fn_MAX11043_ADCVoltageOfCode_24bit(uint32_t value_u24); 02148 tinyTester.FunctionCall_Expect("MAX11043.ADCVoltageOfCode_24bit", fn_MAX11043_ADCVoltageOfCode_24bit, 0x000000, 0.0); // Zero Scale 02149 #endif // MAX11043_SELFTEST_BIP2C24 // group BIP2C24 02150 02151 // CODE GENERATOR: SelfTest ADCVoltageOfCode_24bit @test group BIP2C24 ADCVoltageOfCode_24bit(0xFFFFFF) expect -0.0000029802326 Negative One LSB 02152 #if MAX11043_SELFTEST_BIP2C24 // group BIP2C24 ADCVoltageOfCode_24bit Negative One LSB 02153 // ASSERT_EQ(g_MAX11043_device.ADCVoltageOfCode_24bit(0xFFFFFF), -0.0000029802326); // Negative One LSB 02154 extern double fn_MAX11043_ADCVoltageOfCode_24bit(uint32_t value_u24); 02155 tinyTester.FunctionCall_Expect("MAX11043.ADCVoltageOfCode_24bit", fn_MAX11043_ADCVoltageOfCode_24bit, 0xFFFFFF, -0.0000029802326); // Negative One LSB 02156 #endif // MAX11043_SELFTEST_BIP2C24 // group BIP2C24 02157 02158 // CODE GENERATOR: SelfTest ADCVoltageOfCode_24bit @test group BIP2C24 ADCVoltageOfCode_24bit(0xFFFFFE) expect -0.0000059604644 Negative Two LSB 02159 #if MAX11043_SELFTEST_BIP2C24 // group BIP2C24 ADCVoltageOfCode_24bit Negative Two LSB 02160 // ASSERT_EQ(g_MAX11043_device.ADCVoltageOfCode_24bit(0xFFFFFE), -0.0000059604644); // Negative Two LSB 02161 extern double fn_MAX11043_ADCVoltageOfCode_24bit(uint32_t value_u24); 02162 tinyTester.FunctionCall_Expect("MAX11043.ADCVoltageOfCode_24bit", fn_MAX11043_ADCVoltageOfCode_24bit, 0xFFFFFE, -0.0000059604644); // Negative Two LSB 02163 #endif // MAX11043_SELFTEST_BIP2C24 // group BIP2C24 02164 02165 // CODE GENERATOR: SelfTest ADCVoltageOfCode_24bit @test group BIP2C24 ADCVoltageOfCode_24bit(0xFFFFFD) expect -0.0000089406967 Negative Three LSB 02166 #if MAX11043_SELFTEST_BIP2C24 // group BIP2C24 ADCVoltageOfCode_24bit Negative Three LSB 02167 // ASSERT_EQ(g_MAX11043_device.ADCVoltageOfCode_24bit(0xFFFFFD), -0.0000089406967); // Negative Three LSB 02168 extern double fn_MAX11043_ADCVoltageOfCode_24bit(uint32_t value_u24); 02169 tinyTester.FunctionCall_Expect("MAX11043.ADCVoltageOfCode_24bit", fn_MAX11043_ADCVoltageOfCode_24bit, 0xFFFFFD, -0.0000089406967); // Negative Three LSB 02170 #endif // MAX11043_SELFTEST_BIP2C24 // group BIP2C24 02171 02172 // CODE GENERATOR: SelfTest ADCVoltageOfCode_24bit @test group BIP2C24 ADCVoltageOfCode_24bit(0xFAE148) expect -0.100 Negative 100mV 02173 #if MAX11043_SELFTEST_BIP2C24 // group BIP2C24 ADCVoltageOfCode_24bit Negative 100mV 02174 // ASSERT_EQ(g_MAX11043_device.ADCVoltageOfCode_24bit(0xFAE148), -0.100); // Negative 100mV 02175 extern double fn_MAX11043_ADCVoltageOfCode_24bit(uint32_t value_u24); 02176 tinyTester.FunctionCall_Expect("MAX11043.ADCVoltageOfCode_24bit", fn_MAX11043_ADCVoltageOfCode_24bit, 0xFAE148, -0.100); // Negative 100mV 02177 #endif // MAX11043_SELFTEST_BIP2C24 // group BIP2C24 02178 02179 // CODE GENERATOR: SelfTest ADCVoltageOfCode_24bit @test group BIP2C24 ADCVoltageOfCode_24bit(0xE00000) expect -0.625 Negative 25% Scale 02180 #if MAX11043_SELFTEST_BIP2C24 // group BIP2C24 ADCVoltageOfCode_24bit Negative 25% Scale 02181 // ASSERT_EQ(g_MAX11043_device.ADCVoltageOfCode_24bit(0xE00000), -0.625); // Negative 25% Scale 02182 extern double fn_MAX11043_ADCVoltageOfCode_24bit(uint32_t value_u24); 02183 tinyTester.FunctionCall_Expect("MAX11043.ADCVoltageOfCode_24bit", fn_MAX11043_ADCVoltageOfCode_24bit, 0xE00000, -0.625); // Negative 25% Scale 02184 #endif // MAX11043_SELFTEST_BIP2C24 // group BIP2C24 02185 02186 // CODE GENERATOR: SelfTest ADCVoltageOfCode_24bit @test group BIP2C24 ADCVoltageOfCode_24bit(0xCCCCCD) expect -1.000 Negative One Volt 02187 #if MAX11043_SELFTEST_BIP2C24 // group BIP2C24 ADCVoltageOfCode_24bit Negative One Volt 02188 // ASSERT_EQ(g_MAX11043_device.ADCVoltageOfCode_24bit(0xCCCCCD), -1.000); // Negative One Volt 02189 extern double fn_MAX11043_ADCVoltageOfCode_24bit(uint32_t value_u24); 02190 tinyTester.FunctionCall_Expect("MAX11043.ADCVoltageOfCode_24bit", fn_MAX11043_ADCVoltageOfCode_24bit, 0xCCCCCD, -1.000); // Negative One Volt 02191 #endif // MAX11043_SELFTEST_BIP2C24 // group BIP2C24 02192 02193 // CODE GENERATOR: SelfTest ADCVoltageOfCode_24bit @test group BIP2C24 ADCVoltageOfCode_24bit(0xC00000) expect -1.250 Negative Mid Scale 02194 #if MAX11043_SELFTEST_BIP2C24 // group BIP2C24 ADCVoltageOfCode_24bit Negative Mid Scale 02195 // ASSERT_EQ(g_MAX11043_device.ADCVoltageOfCode_24bit(0xC00000), -1.250); // Negative Mid Scale 02196 extern double fn_MAX11043_ADCVoltageOfCode_24bit(uint32_t value_u24); 02197 tinyTester.FunctionCall_Expect("MAX11043.ADCVoltageOfCode_24bit", fn_MAX11043_ADCVoltageOfCode_24bit, 0xC00000, -1.250); // Negative Mid Scale 02198 #endif // MAX11043_SELFTEST_BIP2C24 // group BIP2C24 02199 02200 // CODE GENERATOR: SelfTest ADCVoltageOfCode_24bit @test group BIP2C24 ADCVoltageOfCode_24bit(0xA00000) expect -1.875 Negative 75% Scale 02201 #if MAX11043_SELFTEST_BIP2C24 // group BIP2C24 ADCVoltageOfCode_24bit Negative 75% Scale 02202 // ASSERT_EQ(g_MAX11043_device.ADCVoltageOfCode_24bit(0xA00000), -1.875); // Negative 75% Scale 02203 extern double fn_MAX11043_ADCVoltageOfCode_24bit(uint32_t value_u24); 02204 tinyTester.FunctionCall_Expect("MAX11043.ADCVoltageOfCode_24bit", fn_MAX11043_ADCVoltageOfCode_24bit, 0xA00000, -1.875); // Negative 75% Scale 02205 #endif // MAX11043_SELFTEST_BIP2C24 // group BIP2C24 02206 02207 // CODE GENERATOR: SelfTest ADCVoltageOfCode_24bit @test group BIP2C24 ADCVoltageOfCode_24bit(0x99999A) expect -2.000 Negative Two Volts 02208 #if MAX11043_SELFTEST_BIP2C24 // group BIP2C24 ADCVoltageOfCode_24bit Negative Two Volts 02209 // ASSERT_EQ(g_MAX11043_device.ADCVoltageOfCode_24bit(0x99999A), -2.000); // Negative Two Volts 02210 extern double fn_MAX11043_ADCVoltageOfCode_24bit(uint32_t value_u24); 02211 tinyTester.FunctionCall_Expect("MAX11043.ADCVoltageOfCode_24bit", fn_MAX11043_ADCVoltageOfCode_24bit, 0x99999A, -2.000); // Negative Two Volts 02212 #endif // MAX11043_SELFTEST_BIP2C24 // group BIP2C24 02213 02214 // CODE GENERATOR: SelfTest ADCVoltageOfCode_24bit @test group BIP2C24 ADCVoltageOfCode_24bit(0x800001) expect -2.500 Negative Full Scale 02215 #if MAX11043_SELFTEST_BIP2C24 // group BIP2C24 ADCVoltageOfCode_24bit Negative Full Scale 02216 // ASSERT_EQ(g_MAX11043_device.ADCVoltageOfCode_24bit(0x800001), -2.500); // Negative Full Scale 02217 extern double fn_MAX11043_ADCVoltageOfCode_24bit(uint32_t value_u24); 02218 tinyTester.FunctionCall_Expect("MAX11043.ADCVoltageOfCode_24bit", fn_MAX11043_ADCVoltageOfCode_24bit, 0x800001, -2.500); // Negative Full Scale 02219 #endif // MAX11043_SELFTEST_BIP2C24 // group BIP2C24 02220 02221 // CODE GENERATOR: SelfTest ADCVoltageOfCode_24bit @test group BIP2C24 ADCVoltageOfCode_24bit(0x800000) expect -2.500 Negative Full Scale 02222 #if MAX11043_SELFTEST_BIP2C24 // group BIP2C24 ADCVoltageOfCode_24bit Negative Full Scale 02223 // ASSERT_EQ(g_MAX11043_device.ADCVoltageOfCode_24bit(0x800000), -2.500); // Negative Full Scale 02224 extern double fn_MAX11043_ADCVoltageOfCode_24bit(uint32_t value_u24); 02225 tinyTester.FunctionCall_Expect("MAX11043.ADCVoltageOfCode_24bit", fn_MAX11043_ADCVoltageOfCode_24bit, 0x800000, -2.500); // Negative Full Scale 02226 #endif // MAX11043_SELFTEST_BIP2C24 // group BIP2C24 02227 02228 // 02229 #if INJECT_SELFTEST_FAIL 02230 // Test of the pass/fail report mechanism 02231 tinyTester.FAIL(); 02232 cmdLine.serial().print(F("injecting one false failure for test reporting")); 02233 #endif 02234 // 02235 // Report number of pass and number of fail test results 02236 tinyTester.Report_Summary(); 02237 } 02238 02239 //-------------------------------------------------- 02240 // selftest: define function under test 02241 // double MAX11043::ADCVoltageOfCode_16bit(uint32_t value_u16) 02242 double fn_MAX11043_ADCVoltageOfCode_16bit(uint32_t value_u16) 02243 { 02244 return g_MAX11043_device.ADCVoltageOfCode_16bit(value_u16); 02245 } 02246 02247 //-------------------------------------------------- 02248 // selftest: define function under test 02249 // double MAX11043::ADCVoltageOfCode_24bit(uint32_t value_u24) 02250 double fn_MAX11043_ADCVoltageOfCode_24bit(uint32_t value_u24) 02251 { 02252 return g_MAX11043_device.ADCVoltageOfCode_24bit(value_u24); 02253 } 02254 02255 02256 //-------------------------------------------------- 02257 inline void print_command_prompt() 02258 { 02259 cmdLine_serial.serial().printf("\r\n> "); 02260 02261 } 02262 02263 02264 //-------------------------------------------------- 02265 void pinsMonitor_submenu_onEOLcommandParser(CmdLine& cmdLine) 02266 { 02267 // % diagnostic commands submenu 02268 // %Hpin -- digital output high 02269 // %Lpin -- digital output low 02270 // %?pin -- digital input 02271 // %A %Apin -- analog input 02272 // %Ppin df=xx -- pwm output 02273 // %Wpin -- measure high pulsewidth input in usec 02274 // %wpin -- measure low pulsewidth input in usec 02275 // %I... -- I2C diagnostics 02276 // %IP -- I2C probe 02277 // %IC scl=100khz ADDR=? -- I2C configure 02278 // %IW byte byte ... byte RD=? ADDR=0x -- write 02279 // %IR ADDR=? RD=? -- read 02280 // %I^ cmd=? -- i2c_smbus_read_word_data 02281 // %S... -- SPI diagnostics 02282 // %SC sclk=1Mhz -- SPI configure 02283 // %SW -- write (write and read) 02284 // %SR -- read (alias for %SW because SPI always write and read) 02285 // A-Z,a-z,0-9 reserved for application use 02286 // 02287 char strPinIndex[3]; 02288 strPinIndex[0] = cmdLine[2]; 02289 strPinIndex[1] = cmdLine[3]; 02290 strPinIndex[2] = '\0'; 02291 int pinIndex = strtoul(strPinIndex, NULL, 10); // strtol(str, NULL, 10): get decimal value 02292 //cmdLine.serial().printf(" pinIndex=%d ", pinIndex); 02293 // 02294 // get next character 02295 switch (cmdLine[1]) 02296 { 02297 #if HAS_digitalInOuts 02298 case 'H': case 'h': 02299 { 02300 // %Hpin -- digital output high 02301 #if ARDUINO_STYLE 02302 pinMode(pinIndex, OUTPUT); // digital pins 0, 1, 2, .. 13, analog input pins A0, A1, .. A5 02303 digitalWrite(pinIndex, HIGH); // digital pins 0, 1, 2, .. 13, analog input pins A0, A1, .. A5 02304 #else 02305 DigitalInOut& digitalInOutPin = find_digitalInOutPin(pinIndex); 02306 digitalInOutPin.output(); 02307 digitalInOutPin.write(1); 02308 #endif 02309 cmdLine.serial().printf(" digitalInOutPin %d Output High ", pinIndex); 02310 } 02311 break; 02312 case 'L': case 'l': 02313 { 02314 // %Lpin -- digital output low 02315 #if ARDUINO_STYLE 02316 pinMode(pinIndex, OUTPUT); // digital pins 0, 1, 2, .. 13, analog input pins A0, A1, .. A5 02317 digitalWrite(pinIndex, LOW); // digital pins 0, 1, 2, .. 13, analog input pins A0, A1, .. A5 02318 #else 02319 DigitalInOut& digitalInOutPin = find_digitalInOutPin(pinIndex); 02320 digitalInOutPin.output(); 02321 digitalInOutPin.write(0); 02322 #endif 02323 cmdLine.serial().printf(" digitalInOutPin %d Output Low ", pinIndex); 02324 } 02325 break; 02326 case '?': 02327 { 02328 // %?pin -- digital input 02329 #if ARDUINO_STYLE 02330 pinMode(pinIndex, INPUT); // digital pins 0, 1, 2, .. 13, analog input pins A0, A1, .. A5 02331 #else 02332 DigitalInOut& digitalInOutPin = find_digitalInOutPin(pinIndex); 02333 digitalInOutPin.input(); 02334 #endif 02335 serial.printf(" digitalInOutPin %d Input ", pinIndex); 02336 #if ARDUINO_STYLE 02337 int value = digitalRead(pinIndex); 02338 #else 02339 int value = digitalInOutPin.read(); 02340 #endif 02341 cmdLine.serial().printf("%d ", value); 02342 } 02343 break; 02344 #endif 02345 // 02346 #if HAS_analogIns 02347 case 'A': case 'a': 02348 { 02349 // %A %Apin -- analog input 02350 #if analogIn4_IS_HIGH_RANGE_OF_analogIn0 02351 // Platform board uses AIN4,AIN5,.. as high range of AIN0,AIN1,.. 02352 for (int pinIndex = 0; pinIndex < 2; pinIndex++) 02353 { 02354 int cPinIndex = '0' + pinIndex; 02355 AnalogIn& analogInPin = find_analogInPin(cPinIndex); 02356 float adc_full_scale_voltage = analogInPin_fullScaleVoltage[pinIndex]; 02357 float normValue_0_1 = analogInPin.read(); 02358 // 02359 int pinIndexH = pinIndex + 4; 02360 int cPinIndexH = '0' + pinIndexH; 02361 AnalogIn& analogInPinH = find_analogInPin(cPinIndexH); 02362 float adc_full_scale_voltageH = analogInPin_fullScaleVoltage[pinIndexH]; 02363 float normValueH_0_1 = analogInPinH.read(); 02364 // 02365 cmdLine.serial().printf("AIN%c = %7.3f%% = %1.3fV AIN%c = %7.3f%% = %1.3fV \r\n", 02366 cPinIndex, 02367 normValue_0_1 * 100.0, 02368 normValue_0_1 * adc_full_scale_voltage, 02369 cPinIndexH, 02370 normValueH_0_1 * 100.0, 02371 normValueH_0_1 * adc_full_scale_voltageH 02372 ); 02373 } 02374 for (int pinIndex = 2; pinIndex < 4; pinIndex++) 02375 { 02376 int cPinIndex = '0' + pinIndex; 02377 AnalogIn& analogInPin = find_analogInPin(cPinIndex); 02378 float adc_full_scale_voltage = analogInPin_fullScaleVoltage[pinIndex]; 02379 float normValue_0_1 = analogInPin.read(); 02380 // 02381 cmdLine.serial().printf("AIN%c = %7.3f%% = %1.3fV\r\n", 02382 cPinIndex, 02383 normValue_0_1 * 100.0, 02384 normValue_0_1 * adc_full_scale_voltage 02385 ); 02386 } 02387 #else // analogIn4_IS_HIGH_RANGE_OF_analogIn0 02388 // Platform board uses simple analog inputs 02389 // assume standard Arduino analog inputs A0-A5 02390 for (int pinIndex = 0; pinIndex < 6; pinIndex++) 02391 { 02392 int cPinIndex = '0' + pinIndex; 02393 AnalogIn& analogInPin = find_analogInPin(cPinIndex); 02394 float adc_full_scale_voltage = analogInPin_fullScaleVoltage[pinIndex]; 02395 float normValue_0_1 = analogInPin.read(); 02396 // 02397 cmdLine.serial().printf("AIN%c = %7.3f%% = %1.3fV\r\n", 02398 cPinIndex, 02399 normValue_0_1 * 100.0, 02400 normValue_0_1 * adc_full_scale_voltage 02401 ); 02402 } 02403 #endif // analogIn4_IS_HIGH_RANGE_OF_analogIn0 02404 } 02405 break; 02406 #endif 02407 // 02408 #if HAS_SPI2_MAX541 02409 case 'D': case 'd': 02410 { 02411 // %D -- DAC output MAX541 (SPI2) -- need cmdLine.parse_float(voltageV) 02412 // MAX541 max541(spi2_max541, spi2_max541_cs); 02413 float voltageV = max541.Get_Voltage(); 02414 // if (cmdLine[2] == '+') { 02415 // // %D+ 02416 // voltageV = voltageV * 1.25f; 02417 // if (voltageV >= max541.VRef) voltageV = max541.VRef; 02418 // SelfTest_MAX541_Voltage(cmdLine, max541, voltageV); 02419 // } 02420 // else if (cmdLine[2] == '-') { 02421 // // %D- 02422 // voltageV = voltageV * 0.75f; 02423 // if (voltageV < 0.1f) voltageV = 0.1f; 02424 // SelfTest_MAX541_Voltage(cmdLine, max541, voltageV); 02425 // } 02426 if (cmdLine.parse_float("V", voltageV)) 02427 { 02428 // %D V=1.234 -- set voltage 02429 max541.Set_Voltage(voltageV); 02430 } 02431 else if (cmdLine.parse_float("TEST", voltageV)) 02432 { 02433 // %D TEST=1.234 -- set voltage and compare with AIN0 02434 SelfTest_MAX541_Voltage(cmdLine, max541, voltageV); 02435 } 02436 else if (cmdLine.parse_float("CAL", voltageV)) 02437 { 02438 // %D CAL=1.234 -- calibrate VRef and compare with AIN0 02439 02440 max541.Set_Code(0x8000); // we don't know the fullscale voltage yet, so set code to midscale 02441 double max541_midscale_V = analogInPin_fullScaleVoltage[4] * analogIn4.read(); // TARGET_MAX32630 J1.5 AIN_4 = AIN0 / 5.0 fullscale is 6.0V 02442 const int average_count = 100; 02443 const double average_K = 0.25; 02444 for (int count = 0; count < average_count; count++) { 02445 double measurement_V = analogInPin_fullScaleVoltage[4] * analogIn4.read(); // TARGET_MAX32630 J1.5 AIN_4 = AIN0 / 5.0 fullscale is 6.0V 02446 max541_midscale_V = ((1 - average_K) * max541_midscale_V) + (average_K * measurement_V); 02447 } 02448 max541.VRef = 2.0 * max541_midscale_V; 02449 cmdLine.serial().printf( 02450 "\r\n MAX541 midscale = %1.3fV, so fullscale = %1.3fV", 02451 max541_midscale_V, max541.VRef); 02452 // Detect whether MAX541 is really connected to MAX32625MBED.AIN0/AIN4 02453 voltageV = 1.0f; 02454 SelfTest_MAX541_Voltage(cmdLine, max541, voltageV); 02455 } 02456 else { 02457 // %D -- print MAX541 DAC status 02458 cmdLine.serial().printf("MAX541 code=0x%4.4x = %1.3fV VRef=%1.3fV\r\n", 02459 max541.Get_Code(), max541.Get_Voltage(), max541.VRef); 02460 } 02461 } 02462 break; 02463 #endif 02464 02465 // 02466 #if HAS_I2C // SUPPORT_I2C 02467 case 'I': case 'i': 02468 // %I... -- I2C diagnostics 02469 // %IP -- I2C probe 02470 // %IC scl=100khz ADDR=? -- I2C configure 02471 // %IW byte byte ... byte RD=? ADDR=0x -- write 02472 // %IR ADDR=? RD=? -- read 02473 // %I^ cmd=? -- i2c_smbus_read_word_data 02474 // get next character 02475 // TODO: parse cmdLine arg (ADDR=\d+)? --> g_I2C_deviceAddress7 02476 cmdLine.parse_byte_hex("ADDR", g_I2C_deviceAddress7); 02477 // TODO: parse cmdLine arg (RD=\d)? --> g_I2C_read_count 02478 g_I2C_read_count = 0; // read count must be reset every command 02479 cmdLine.parse_byte_dec("RD", g_I2C_read_count); 02480 // TODO: parse cmdLine arg (CMD=\d)? --> g_I2C_command_regAddress 02481 cmdLine.parse_byte_hex("CMD", g_I2C_command_regAddress); 02482 switch (cmdLine[2]) 02483 { 02484 case 'P': case 'p': 02485 { 02486 // %IP -- I2C probe 02487 HuntAttachedI2CDevices(cmdLine, 0x03, 0x77); 02488 } 02489 break; 02490 case 'C': case 'c': 02491 { 02492 bool isUpdatedI2CConfig = false; 02493 // %IC scl=100khz ADDR=? -- I2C configure 02494 // parse cmdLine arg (SCL=\d+(kHZ|MHZ)?)? --> g_I2C_SCL_Hz 02495 if (cmdLine.parse_frequency_Hz("SCL", g_I2C_SCL_Hz)) 02496 { 02497 isUpdatedI2CConfig = true; 02498 // TODO1: validate g_I2C_SCL_Hz against system clock frequency F_CPU 02499 if (g_I2C_SCL_Hz > limit_max_I2C_SCL_Hz) 02500 { 02501 g_I2C_SCL_Hz = limit_max_I2C_SCL_Hz; 02502 } 02503 if (g_I2C_SCL_Hz < limit_min_I2C_SCL_Hz) 02504 { 02505 g_I2C_SCL_Hz = limit_min_I2C_SCL_Hz; 02506 } 02507 } 02508 if (isUpdatedI2CConfig) 02509 { 02510 // declare in narrower scope: MAX32625MBED I2C i2cMaster(...) 02511 I2C i2cMaster(I2C0_SDA, I2C0_SCL); // sda scl TARGET_MAX32635MBED: P1_6, P1_7 Arduino 10-pin header 02512 i2cMaster.frequency(g_I2C_SCL_Hz); 02513 i2cMaster.start(); 02514 i2cMaster.stop(); 02515 i2cMaster.frequency(g_I2C_SCL_Hz); 02516 cmdLine.serial().printf( 02517 "\r\n %%IC ADDR=0x%2.2x=(0x%2.2x>>1) SCL=%d=%1.3fkHz -- I2C config", 02518 g_I2C_deviceAddress7, (g_I2C_deviceAddress7 << 1), g_I2C_SCL_Hz, 02519 (g_I2C_SCL_Hz / 1000.)); 02520 i2cMaster.start(); 02521 i2cMaster.stop(); 02522 } 02523 } 02524 break; 02525 case 'W': case 'w': 02526 { 02527 // declare in narrower scope: MAX32625MBED I2C i2cMaster(...) 02528 I2C i2cMaster(I2C0_SDA, I2C0_SCL); // sda scl TARGET_MAX32635MBED: P1_6, P1_7 Arduino 10-pin header 02529 i2cMaster.frequency(g_I2C_SCL_Hz); 02530 // %IW byte byte ... byte RD=? ADDR=0x -- write 02531 // parse cmdLine byte list --> int byteCount; int mosiData[MAX_SPI_BYTE_COUNT]; 02532 #define MAX_I2C_BYTE_COUNT 32 02533 size_t byteCount = byteCount; 02534 static char mosiData[MAX_I2C_BYTE_COUNT]; 02535 static char misoData[MAX_I2C_BYTE_COUNT]; 02536 if (cmdLine.parse_byteCount_byteList_hex(byteCount, mosiData, 02537 MAX_I2C_BYTE_COUNT)) 02538 { 02539 // hex dump mosiData[0..byteCount-1] 02540 cmdLine.serial().printf( 02541 "\r\nADDR=0x%2.2x=(0x%2.2x>>1) byteCount:%d RD=%d\r\nI2C MOSI->", 02542 g_I2C_deviceAddress7, 02543 (g_I2C_deviceAddress7 << 1), byteCount, g_I2C_read_count); 02544 for (unsigned int byteIndex = 0; byteIndex < byteCount; byteIndex++) 02545 { 02546 cmdLine.serial().printf(" 0x%2.2X", mosiData[byteIndex]); 02547 } 02548 // 02549 // TODO: i2c transfer 02550 //const int addr7bit = 0x48; // 7 bit I2C address 02551 //const int addr8bit = 0x48 << 1; // 8bit I2C address, 0x90 02552 // /* int */ i2cMaster.read (int addr8bit, char *data, int length, bool repeated=false) // Read from an I2C slave. 02553 // /* int */ i2cMaster.read (int ack) // Read a single byte from the I2C bus. 02554 // /* int */ i2cMaster.write (int addr8bit, const char *data, int length, bool repeated=false) // Write to an I2C slave. 02555 // /* int */ i2cMaster.write (int data) // Write single byte out on the I2C bus. 02556 // /* void */ i2cMaster.start (void) // Creates a start condition on the I2C bus. 02557 // /* void */ i2cMaster.stop (void) // Creates a stop condition on the I2C bus. 02558 // /* int */ i2cMaster.transfer (int addr8bit, const char *tx_buffer, int tx_length, char *rx_buffer, int rx_length, const event_callback_t &callback, int event=I2C_EVENT_TRANSFER_COMPLETE, bool repeated=false) // Start nonblocking I2C transfer. More... 02559 // /* void */ i2cMaster.abort_transfer () // Abort the ongoing I2C transfer. More... 02560 const int addr8bit = g_I2C_deviceAddress7 << 1; // 8bit I2C address, 0x90 02561 unsigned int misoLength = 0; 02562 bool repeated = (g_I2C_read_count > 0); 02563 // 02564 int writeStatus = i2cMaster.write (addr8bit, mosiData, byteCount, repeated); 02565 switch (writeStatus) 02566 { 02567 case 0: cmdLine.serial().printf(" ack "); break; 02568 case 1: cmdLine.serial().printf(" nack "); break; 02569 default: cmdLine.serial().printf(" {writeStatus 0x%2.2X} ", 02570 writeStatus); 02571 } 02572 if (repeated) 02573 { 02574 int readStatus = 02575 i2cMaster.read (addr8bit, misoData, g_I2C_read_count, false); 02576 switch (readStatus) 02577 { 02578 case 1: cmdLine.serial().printf(" nack "); break; 02579 case 0: cmdLine.serial().printf(" ack "); break; 02580 default: cmdLine.serial().printf(" {readStatus 0x%2.2X} ", 02581 readStatus); 02582 } 02583 } 02584 // 02585 if (misoLength > 0) 02586 { 02587 // hex dump misoData[0..byteCount-1] 02588 cmdLine.serial().printf(" MISO<-"); 02589 for (unsigned int byteIndex = 0; byteIndex < g_I2C_read_count; 02590 byteIndex++) 02591 { 02592 cmdLine.serial().printf(" 0x%2.2X", misoData[byteIndex]); 02593 } 02594 } 02595 cmdLine.serial().printf(" "); 02596 } 02597 } 02598 break; 02599 case 'R': case 'r': 02600 { 02601 // declare in narrower scope: MAX32625MBED I2C i2cMaster(...) 02602 I2C i2cMaster(I2C0_SDA, I2C0_SCL); // sda scl TARGET_MAX32635MBED: P1_6, P1_7 Arduino 10-pin header 02603 i2cMaster.frequency(g_I2C_SCL_Hz); 02604 // %IR ADDR=? RD=? -- read 02605 // TODO: i2c transfer 02606 //const int addr7bit = 0x48; // 7 bit I2C address 02607 //const int addr8bit = 0x48 << 1; // 8bit I2C address, 0x90 02608 // /* int */ i2cMaster.read (int addr8bit, char *data, int length, bool repeated=false) // Read from an I2C slave. 02609 // /* int */ i2cMaster.read (int ack) // Read a single byte from the I2C bus. 02610 // /* int */ i2cMaster.write (int addr8bit, const char *data, int length, bool repeated=false) // Write to an I2C slave. 02611 // /* int */ i2cMaster.write (int data) // Write single byte out on the I2C bus. 02612 // /* void */ i2cMaster.start (void) // Creates a start condition on the I2C bus. 02613 // /* void */ i2cMaster.stop (void) // Creates a stop condition on the I2C bus. 02614 // /* int */ i2cMaster.transfer (int addr8bit, const char *tx_buffer, int tx_length, char *rx_buffer, int rx_length, const event_callback_t &callback, int event=I2C_EVENT_TRANSFER_COMPLETE, bool repeated=false) // Start nonblocking I2C transfer. More... 02615 // /* void */ i2cMaster.abort_transfer () // Abort the ongoing I2C transfer. More... 02616 } 02617 break; 02618 case '^': 02619 { 02620 // declare in narrower scope: MAX32625MBED I2C i2cMaster(...) 02621 I2C i2cMaster(I2C0_SDA, I2C0_SCL); // sda scl TARGET_MAX32635MBED: P1_6, P1_7 Arduino 10-pin header 02622 i2cMaster.frequency(g_I2C_SCL_Hz); 02623 // %I^ cmd=? -- i2c_smbus_read_word_data 02624 // TODO: i2c transfer 02625 //const int addr7bit = 0x48; // 7 bit I2C address 02626 //const int addr8bit = 0x48 << 1; // 8bit I2C address, 0x90 02627 // /* int */ i2cMaster.read (int addr8bit, char *data, int length, bool repeated=false) // Read from an I2C slave. 02628 // /* int */ i2cMaster.read (int ack) // Read a single byte from the I2C bus. 02629 // /* int */ i2cMaster.write (int addr8bit, const char *data, int length, bool repeated=false) // Write to an I2C slave. 02630 // /* int */ i2cMaster.write (int data) // Write single byte out on the I2C bus. 02631 // /* void */ i2cMaster.start (void) // Creates a start condition on the I2C bus. 02632 // /* void */ i2cMaster.stop (void) // Creates a stop condition on the I2C bus. 02633 // /* int */ i2cMaster.transfer (int addr8bit, const char *tx_buffer, int tx_length, char *rx_buffer, int rx_length, const event_callback_t &callback, int event=I2C_EVENT_TRANSFER_COMPLETE, bool repeated=false) // Start nonblocking I2C transfer. More... 02634 // /* void */ i2cMaster.abort_transfer () // Abort the ongoing I2C transfer. More... 02635 } 02636 break; 02637 } // switch(cmdLine[2]) 02638 break; 02639 #endif 02640 // 02641 #if HAS_SPI // SUPPORT_SPI 02642 case 'S': case 's': 02643 { 02644 // %S... -- SPI diagnostics 02645 // %SC sclk=1Mhz -- SPI configure 02646 // %SW -- write (write and read) 02647 // %SR -- read (alias for %SW because SPI always write and read) 02648 // 02649 // Process arguments SCLK=\d+(kHZ|MHZ) CPOL=\d CPHA=\d 02650 bool isUpdatedSPIConfig = false; 02651 // parse cmdLine arg (CPOL=\d)? --> g_SPI_dataMode | SPI_MODE2 02652 // parse cmdLine arg (CPHA=\d)? --> g_SPI_dataMode | SPI_MODE1 02653 if (cmdLine.parse_flag("CPOL", g_SPI_dataMode, SPI_MODE2)) 02654 { 02655 isUpdatedSPIConfig = true; 02656 } 02657 if (cmdLine.parse_flag("CPHA", g_SPI_dataMode, SPI_MODE1)) 02658 { 02659 isUpdatedSPIConfig = true; 02660 } 02661 if (cmdLine.parse_flag("CS", g_SPI_cs_state, 1)) 02662 { 02663 isUpdatedSPIConfig = true; 02664 } 02665 // parse cmdLine arg (SCLK=\d+(kHZ|MHZ)?)? --> g_SPI_SCLK_Hz 02666 if (cmdLine.parse_frequency_Hz("SCLK", g_SPI_SCLK_Hz)) 02667 { 02668 isUpdatedSPIConfig = true; 02669 // TODO1: validate g_SPI_SCLK_Hz against system clock frequency F_CPU 02670 if (g_SPI_SCLK_Hz > limit_max_SPI_SCLK_Hz) 02671 { 02672 g_SPI_SCLK_Hz = limit_max_SPI_SCLK_Hz; 02673 } 02674 if (g_SPI_SCLK_Hz < limit_min_SPI_SCLK_Hz) 02675 { 02676 g_SPI_SCLK_Hz = limit_min_SPI_SCLK_Hz; 02677 } 02678 } 02679 // Update SPI configuration 02680 if (isUpdatedSPIConfig) 02681 { 02682 // %SC sclk=1Mhz -- SPI configure 02683 spi_cs = g_SPI_cs_state; 02684 spi.format(8,g_SPI_dataMode); // int bits_must_be_8, int mode=0_3 CPOL=0,CPHA=0 02685 #if APPLICATION_MAX5715 02686 g_MAX5715_device.spi_frequency(g_SPI_SCLK_Hz); 02687 #elif APPLICATION_MAX11131 02688 g_MAX11131_device.spi_frequency(g_SPI_SCLK_Hz); 02689 #elif APPLICATION_MAX5171 02690 g_MAX5171_device.spi_frequency(g_SPI_SCLK_Hz); 02691 #elif APPLICATION_MAX11410 02692 g_MAX11410_device.spi_frequency(g_SPI_SCLK_Hz); 02693 #elif APPLICATION_MAX12345 02694 g_MAX12345_device.spi_frequency(g_SPI_SCLK_Hz); 02695 #else 02696 spi.frequency(g_SPI_SCLK_Hz); // int SCLK_Hz=1000000 = 1MHz (initial default) 02697 #endif 02698 // 02699 double ideal_divisor = ((double)SystemCoreClock) / g_SPI_SCLK_Hz; 02700 int actual_divisor = (int)(ideal_divisor + 0.0); // frequency divisor truncate 02701 double actual_SCLK_Hz = SystemCoreClock / actual_divisor; 02702 // 02703 // fixed: mbed-os-5.11: [Warning] format '%d' expects argument of type 'int', but argument 6 has type 'uint32_t {aka long unsigned int}' [-Wformat=] 02704 cmdLine.serial().printf( 02705 "\r\n %%SC CPOL=%d CPHA=%d CS=%d SCLK=%ld=%1.3fMHz (%1.1fMHz/%1.2f = actual %1.3fMHz) -- SPI config", 02706 ((g_SPI_dataMode & SPI_MODE2) ? 1 : 0), 02707 ((g_SPI_dataMode & SPI_MODE1) ? 1 : 0), 02708 g_SPI_cs_state, 02709 g_SPI_SCLK_Hz, 02710 (g_SPI_SCLK_Hz / 1000000.), 02711 ((double)(SystemCoreClock / 1000000.)), 02712 ideal_divisor, 02713 (actual_SCLK_Hz / 1000000.) 02714 ); 02715 } 02716 // get next character 02717 switch (cmdLine[2]) 02718 { 02719 case 'C': case 's': 02720 // %SC sclk=1Mhz -- SPI configure 02721 break; 02722 case 'D': case 'd': 02723 // %SD -- SPI diagnostic messages enable 02724 if (g_MAX11043_device.onSPIprint) { 02725 g_MAX11043_device.onSPIprint = NULL; 02726 } 02727 else { 02728 void onSPIprint_handler(size_t byteCount, uint8_t mosiData[], uint8_t misoData[]); 02729 g_MAX11043_device.onSPIprint = onSPIprint_handler; 02730 } 02731 break; 02732 case 'W': case 'R': case 'w': case 'r': 02733 { 02734 // %SW -- write (write and read) 02735 // %SR -- read (alias for %SW because SPI always write and read) 02736 // parse cmdLine byte list --> int byteCount; int mosiData[MAX_SPI_BYTE_COUNT]; 02737 #define MAX_SPI_BYTE_COUNT 32 02738 size_t byteCount = byteCount; 02739 static char mosiData[MAX_SPI_BYTE_COUNT]; 02740 static char misoData[MAX_SPI_BYTE_COUNT]; 02741 if (cmdLine.parse_byteCount_byteList_hex(byteCount, mosiData, 02742 MAX_SPI_BYTE_COUNT)) 02743 { 02744 // hex dump mosiData[0..byteCount-1] 02745 cmdLine.serial().printf("\r\nSPI"); 02746 if (byteCount > 7) { 02747 cmdLine.serial().printf(" byteCount:%d", byteCount); 02748 } 02749 cmdLine.serial().printf(" MOSI->"); 02750 for (unsigned int byteIndex = 0; byteIndex < byteCount; byteIndex++) 02751 { 02752 cmdLine.serial().printf(" 0x%2.2X", mosiData[byteIndex]); 02753 } 02754 spi_cs = 0; 02755 unsigned int numBytesTransferred = 02756 spi.write(mosiData, byteCount, misoData, byteCount); 02757 spi_cs = 1; 02758 // hex dump misoData[0..byteCount-1] 02759 cmdLine.serial().printf(" MISO<-"); 02760 for (unsigned int byteIndex = 0; byteIndex < numBytesTransferred; 02761 byteIndex++) 02762 { 02763 cmdLine.serial().printf(" 0x%2.2X", misoData[byteIndex]); 02764 } 02765 cmdLine.serial().printf(" "); 02766 } 02767 } 02768 break; 02769 } // switch(cmdLine[2]) 02770 } // case 'S': // %S... -- SPI diagnostics 02771 break; 02772 #endif 02773 // 02774 // A-Z,a-z,0-9 reserved for application use 02775 } // switch(cmdLine[1]) 02776 } // end void pinsMonitor_submenu_onEOLcommandParser(CmdLine & cmdLine) 02777 02778 02779 //-------------------------------------------------- 02780 void main_menu_status(CmdLine & cmdLine) 02781 { 02782 cmdLine.serial().printf("\r\nMain menu"); 02783 02784 cmdLine.serial().printf(" MAX11043 24-bit 200ksps Delta-Sigma ADC"); 02785 02786 //cmdLine.serial().print(" %s", TARGET_NAME); 02787 if (cmdLine.nameStr()) 02788 { 02789 cmdLine.serial().printf(" [%s]", cmdLine.nameStr()); 02790 02791 } 02792 cmdLine.serial().printf("\r\n ? -- help"); 02793 02794 } 02795 02796 02797 //-------------------------------------------------- 02798 void main_menu_help(CmdLine & cmdLine) 02799 { 02800 // ? -- help 02801 //~ cmdLine.serial().print(F("\r\nMenu:")); 02802 cmdLine.serial().printf("\r\n # -- lines beginning with # are comments"); 02803 02804 cmdLine.serial().printf("\r\n . -- SelfTest"); 02805 02806 //cmdLine.serial().print(F("\r\n ! -- Initial Configuration")); 02807 // 02808 // % standardize diagnostic commands 02809 // %Hpin -- digital output high 02810 // %Lpin -- digital output low 02811 // %?pin -- digital input 02812 // %A %Apin -- analog input 02813 // %Ppin df=xx -- pwm output 02814 // %Wpin -- measure high pulsewidth input in usec 02815 // %wpin -- measure low pulsewidth input in usec 02816 // %I... -- I2C diagnostics 02817 // %IP -- I2C probe 02818 // %IC scl=100khz ADDR=? -- I2C configure 02819 // %IW ADDR=? cmd=? data,data,data -- write 02820 // %IR ADDR=? RD=? -- read 02821 // %I^ cmd=? -- i2c_smbus_read_word_data 02822 // %S... -- SPI diagnostics 02823 // %SC sclk=1Mhz -- SPI configure 02824 // %SW -- write (write and read) 02825 // %SR -- read (alias for %SW because SPI always write and read) 02826 // A-Z,a-z,0-9 reserved for application use 02827 // 02828 #if HAS_digitalInOuts 02829 // %Hpin -- digital output high 02830 // %Lpin -- digital output low 02831 // %?pin -- digital input 02832 cmdLine.serial().printf("\r\n %%Hn {pin:"); 02833 list_digitalInOutPins(cmdLine.serial()); 02834 cmdLine.serial().printf("} -- High Output"); 02835 cmdLine.serial().printf("\r\n %%Ln {pin:"); 02836 list_digitalInOutPins(cmdLine.serial()); 02837 cmdLine.serial().printf("} -- Low Output"); 02838 cmdLine.serial().printf("\r\n %%?n {pin:"); 02839 list_digitalInOutPins(cmdLine.serial()); 02840 cmdLine.serial().printf("} -- Input"); 02841 #endif 02842 02843 #if HAS_analogIns 02844 // Menu A) analogRead A0..7 02845 // %A %Apin -- analog input 02846 // analogRead(pinIndex) // analog input pins A0, A1, A2, A3, A4, A5; float voltage = analogRead(A0) * (5.0 / 1023.0) 02847 cmdLine.serial().printf("\r\n %%A -- analogRead"); 02848 #endif 02849 02850 #if HAS_SPI2_MAX541 02851 // TODO1: MAX541 max541(spi2_max541, spi2_max541_cs); 02852 cmdLine.serial().printf("\r\n %%D -- DAC output MAX541 (SPI2)"); 02853 #endif 02854 02855 #if HAS_I2C // SUPPORT_I2C 02856 // TODO: support I2C HAS_I2C // SUPPORT_I2C 02857 // VERIFY: I2C utility commands SUPPORT_I2C 02858 // VERIFY: report g_I2C_SCL_Hz = (F_CPU / ((TWBR * 2) + 16)) from last Wire_Sr.setClock(I2C_SCL_Hz); 02859 // %I... -- I2C diagnostics 02860 // %IP -- I2C probe 02861 // %IC scl=100khz ADDR=? -- I2C configure 02862 // %IW byte byte ... byte RD=? ADDR=0x -- write 02863 // %IR ADDR=? RD=? -- read 02864 // %I^ cmd=? -- i2c_smbus_read_word_data 02865 //g_I2C_SCL_Hz = (F_CPU / ((TWBR * 2) + 16)); // 'F_CPU' 'TWBR' not declared in this scope 02866 cmdLine.serial().printf("\r\n %%IC ADDR=0x%2.2x=(0x%2.2x>>1) SCL=%d=%1.3fkHz -- I2C config", 02867 g_I2C_deviceAddress7, (g_I2C_deviceAddress7 << 1), g_I2C_SCL_Hz, 02868 (g_I2C_SCL_Hz / 1000.)); 02869 cmdLine.serial().printf("\r\n %%IW byte byte ... byte RD=? ADDR=0x%2.2x -- I2C write/read", 02870 g_I2C_deviceAddress7); 02871 // 02872 #if SUPPORT_I2C 02873 // Menu ^ cmd=?) i2c_smbus_read_word_data 02874 cmdLine.serial().printf("\r\n %%I^ cmd=? -- i2c_smbus_read_word_data"); 02875 // test low-level I2C i2c_smbus_read_word_data 02876 #endif // SUPPORT_I2C 02877 //cmdLine.serial().printf(" H) Hunt for attached I2C devices"); 02878 cmdLine.serial().printf("\r\n %%IP -- I2C Probe for attached devices"); 02879 // cmdLine.serial().printf(" s) search i2c address"); 02880 #endif // SUPPORT_I2C 02881 02882 #if HAS_SPI // SUPPORT_SPI 02883 // TODO: support SPI HAS_SPI // SUPPORT_SPI 02884 // SPI test command S (mosiData)+ 02885 // %S... -- SPI diagnostics 02886 // %SC sclk=1Mhz -- SPI configure 02887 // %SW -- write (write and read) 02888 // %SR -- read (alias for %SW because SPI always write and read) 02889 // spi.format(8,0); // int bits_must_be_8, int mode=0_3 CPOL=0,CPHA=0 rising edge (initial default) 02890 // spi.format(8,1); // int bits_must_be_8, int mode=0_3 CPOL=0,CPHA=1 falling edge (initial default) 02891 // spi.format(8,2); // int bits_must_be_8, int mode=0_3 CPOL=1,CPHA=0 falling edge (initial default) 02892 // spi.format(8,3); // int bits_must_be_8, int mode=0_3 CPOL=1,CPHA=1 rising edge (initial default) 02893 // spi.frequency(1000000); // int SCLK_Hz=1000000 = 1MHz (initial default) 02894 // mode | POL PHA 02895 // -----+-------- 02896 // 0 | 0 0 02897 // 1 | 0 1 02898 // 2 | 1 0 02899 // 3 | 1 1 02900 //cmdLine.serial().printf(" S) SPI mosi,mosi,...mosi hex bytes SCLK=1000000 CPOL=0 CPHA=0"); 02901 // fixed: mbed-os-5.11: [Warning] format '%d' expects argument of type 'int', but argument 3 has type 'uint32_t {aka long unsigned int}' [-Wformat=] 02902 cmdLine.serial().printf("\r\n %%SC SCLK=%ld=%1.3fMHz CPOL=%d CPHA=%d -- SPI config", 02903 g_SPI_SCLK_Hz, (g_SPI_SCLK_Hz / 1000000.), 02904 ((g_SPI_dataMode & SPI_MODE2) ? 1 : 0), 02905 ((g_SPI_dataMode & SPI_MODE1) ? 1 : 0)); 02906 cmdLine.serial().printf("\r\n %%SD -- SPI diagnostic messages "); 02907 if (g_MAX11043_device.onSPIprint) { 02908 cmdLine.serial().printf("hide"); 02909 } 02910 else { 02911 cmdLine.serial().printf("show"); 02912 } 02913 cmdLine.serial().printf("\r\n %%SW mosi,mosi,...mosi -- SPI write hex bytes"); 02914 // VERIFY: parse new SPI settings parse_strCommandArgs() SCLK=1000000 CPOL=0 CPHA=0 02915 #endif // SUPPORT_SPI 02916 // 02917 // Application-specific commands (help text) here 02918 // 02919 #if APPLICATION_ArduinoPinsMonitor 02920 cmdLine.serial().printf("\r\n A-Z,a-z,0-9 -- reserved for application use"); // ArduinoPinsMonitor 02921 #endif // APPLICATION_ArduinoPinsMonitor 02922 // 02923 02924 extern bool MAX11043_menu_help(CmdLine & cmdLine); // defined in Test_Menu_MAX11043.cpp\n 02925 MAX11043_menu_help(cmdLine); 02926 } 02927 02928 02929 02930 //-------------------------------------------------- 02931 // main menu command-line parser 02932 // invoked by CmdLine::append(char ch) or CmdLine::idleAppendIfReadable() 02933 void main_menu_onEOLcommandParser(CmdLine & cmdLine) 02934 { 02935 // DIAGNOSTIC: print line buffer 02936 //~ cmdLine.serial().printf("\r\nmain_menu_onEOLcommandParser: ~%s~\r\n", cmdLine.str()); 02937 // 02938 switch (cmdLine[0]) 02939 { 02940 case '?': 02941 main_menu_status(cmdLine); 02942 main_menu_help(cmdLine); 02943 // print command prompt 02944 //cmdLine.serial().printf("\r\n>"); 02945 break; 02946 case '\r': case '\n': // ignore blank line 02947 case '\0': // ignore empty line 02948 case '#': // ignore comment line 02949 // # -- lines beginning with # are comments 02950 main_menu_status(cmdLine); 02951 //~ main_menu_help(cmdLine); 02952 // print command prompt 02953 //cmdLine.serial().printf("\r\n>"); 02954 break; 02955 #if ECHO_EOF_ON_EOL 02956 case '\x04': // Unicode (U+0004) EOT END OF TRANSMISSION = CTRL+D as EOF end of file 02957 cmdLine.serial().printf("\x04"); // immediately echo EOF for test scripting 02958 diagnostic_led_EOF(); 02959 break; 02960 case '\x1a': // Unicode (U+001A) SUB SUBSTITUTE = CTRL+Z as EOF end of file 02961 cmdLine.serial().printf("\x1a"); // immediately echo EOF for test scripting 02962 diagnostic_led_EOF(); 02963 break; 02964 #endif 02965 #if APPLICATION_ArduinoPinsMonitor 02966 case '.': 02967 { 02968 // . -- SelfTest 02969 cmdLine.serial().printf("SelfTest()"); 02970 SelfTest(cmdLine); 02971 } 02972 break; 02973 case '%': 02974 { 02975 pinsMonitor_submenu_onEOLcommandParser(cmdLine); 02976 } 02977 break; // case '%' 02978 #endif // APPLICATION_ArduinoPinsMonitor 02979 // 02980 // Application-specific commands here 02981 // alphanumeric command codes A-Z,a-z,0-9 reserved for application use 02982 // 02983 #if APPLICATION_ArduinoPinsMonitor 02984 #endif // APPLICATION_ArduinoPinsMonitor 02985 02986 // 02987 // add new commands here 02988 // 02989 default: 02990 extern bool MAX11043_menu_onEOLcommandParser(CmdLine & cmdLine); // defined in Test_Menu_MAX11043.cpp 02991 if (!MAX11043_menu_onEOLcommandParser(cmdLine)) 02992 { // not_handled_by_device_submenu 02993 cmdLine.serial().printf("\r\n unknown command 0x%2.2x \"%s\"\r\n", cmdLine.str()[0], cmdLine.str()); 02994 02995 # if HAS_DAPLINK_SERIAL 02996 cmdLine_DAPLINKserial.serial().printf("\r\n unknown command 0x%2.2x \"%s\"\r\n", cmdLine.str()[0], cmdLine.str()); 02997 02998 # endif // HAS_DAPLINK_SERIAL 02999 } 03000 } // switch (cmdLine[0]) 03001 // 03002 // print command prompt 03003 cmdLine.serial().printf("\r\nMAX11043 > "); 03004 03005 } // end void main_menu_onEOLcommandParser(CmdLine & cmdLine) 03006 03007 //-------------------------------------------------- 03008 #if MAX11043_ONSPIPRINT 03009 // Optional Diagnostic function to print SPI transactions 03010 void onSPIprint_handler(size_t byteCount, uint8_t mosiData[], uint8_t misoData[]) 03011 { 03012 cmdLine_serial.serial().printf("\r\nSPI MOSI->"); 03013 for (uint8_t index = 0; index < byteCount; index++) { 03014 cmdLine_serial.serial().printf(" 0x%2.2X", mosiData[index]); 03015 } 03016 cmdLine_serial.serial().printf(" MISO<-"); 03017 for (uint8_t index = 0; index < byteCount; index++) { 03018 cmdLine_serial.serial().printf(" 0x%2.2X", misoData[index]); 03019 } 03020 cmdLine_serial.serial().printf(" "); 03021 } 03022 #endif // MAX11043_ONSPIPRINT 03023 03024 //-------------------------------------------------- 03025 void InitializeConfiguration() 03026 { 03027 // CODE GENERATOR: example code: member function Init 03028 # if HAS_DAPLINK_SERIAL 03029 cmdLine_DAPLINKserial.serial().printf("\r\nMAX11043_Init()"); 03030 03031 # endif 03032 cmdLine_serial.serial().printf("\r\nMAX11043_Init()"); 03033 03034 // Initialize MAX11043 and verify device ID 03035 if (g_MAX11043_device.Init() == 0) 03036 { // init failed; try "safe mode" SPI at slower SCLK rate 03037 cmdLine_serial.serial().printf("\r\nMAX11043 Init failed; retry at SPI SCLK frequency 2000000 Hz\r\n"); 03038 03039 g_SPI_SCLK_Hz = 2000000; 03040 g_MAX11043_device.spi_frequency(2000000); 03041 g_MAX11043_device.Init(); 03042 } 03043 // CODE GENERATOR: get spi properties from device 03044 if (g_SPI_SCLK_Hz > g_MAX11043_device.get_spi_frequency()) 03045 { // Device limits SPI SCLK frequency 03046 g_SPI_SCLK_Hz = g_MAX11043_device.get_spi_frequency(); 03047 cmdLine_serial.serial().printf("\r\nMAX11043 limits SPI SCLK frequency to %d Hz\r\n", g_SPI_SCLK_Hz); 03048 03049 g_MAX11043_device.Init(); 03050 } 03051 if (g_MAX11043_device.get_spi_frequency() > g_SPI_SCLK_Hz) 03052 { // Platform limits SPI SCLK frequency 03053 g_MAX11043_device.spi_frequency(g_SPI_SCLK_Hz); 03054 cmdLine_serial.serial().printf("\r\nPlatform limits MAX11043 SPI SCLK frequency to %d Hz\r\n", g_SPI_SCLK_Hz); 03055 03056 g_MAX11043_device.Init(); 03057 } 03058 g_SPI_dataMode = g_MAX11043_device.get_spi_dataMode(); 03059 03060 # if MAX11043_ONSPIPRINT 03061 // Optional Diagnostic function to print SPI transactions 03062 g_MAX11043_device.onSPIprint = onSPIprint_handler; 03063 # endif 03064 } 03065 03066 //-------------------------------------------------- 03067 // diagnostic rbg led GREEN 03068 void diagnostic_led_EOF() 03069 { 03070 #if USE_LEDS 03071 led1 = LED_ON; led2 = LED_ON; led3 = LED_OFF; // diagnostic rbg led RED+GREEN=YELLOW 03072 // TODO1: mbed-os-5.11: [Warning] 'static osStatus rtos::Thread::wait(uint32_t)' is deprecated: Static methods only affecting current thread cause confusion. Replaced by ThisThread::sleep_for. [since mbed-os-5.10] [-Wdeprecated-declarations] 03073 ThisThread::sleep_for(250); // [since mbed-os-5.10] vs Thread::wait(250); 03074 led1 = LED_OFF; led2 = LED_ON; led3 = LED_OFF; // diagnostic rbg led GREEN 03075 ThisThread::sleep_for(250); // [since mbed-os-5.10] vs Thread::wait(250); 03076 led1 = LED_ON; led2 = LED_ON; led3 = LED_OFF; // diagnostic rbg led RED+GREEN=YELLOW 03077 ThisThread::sleep_for(250); // [since mbed-os-5.10] vs Thread::wait(250); 03078 led1 = LED_OFF; led2 = LED_ON; led3 = LED_OFF; // diagnostic rbg led GREEN 03079 ThisThread::sleep_for(250); // [since mbed-os-5.10] vs Thread::wait(250); 03080 #endif // USE_LEDS 03081 } 03082 03083 //-------------------------------------------------- 03084 // Support commands that get handled immediately w/o waiting for EOL 03085 // handled as immediate command, do not append to buffer 03086 void on_immediate_0x21() // Unicode (U+0021) ! EXCLAMATION MARK 03087 { 03088 #if USE_LEDS 03089 led1 = LED_OFF; led2 = LED_OFF; led3 = LED_ON; // diagnostic rbg led BLUE 03090 #endif // USE_LEDS 03091 InitializeConfiguration(); 03092 ThisThread::sleep_for(125); // [since mbed-os-5.10] vs Thread::wait(125); 03093 } 03094 03095 //-------------------------------------------------- 03096 // Support commands that get handled immediately w/o waiting for EOL 03097 // handled as immediate command, do not append to buffer 03098 void on_immediate_0x7b() // Unicode (U+007B) { LEFT CURLY BRACKET 03099 { 03100 #if HAS_BUTTON2_DEMO_INTERRUPT 03101 onButton2FallingEdge(); 03102 #endif 03103 } 03104 03105 //-------------------------------------------------- 03106 // Support commands that get handled immediately w/o waiting for EOL 03107 // handled as immediate command, do not append to buffer 03108 void on_immediate_0x7d() // Unicode (U+007D) } RIGHT CURLY BRACKET 03109 { 03110 #if HAS_BUTTON1_DEMO_INTERRUPT 03111 onButton1FallingEdge(); 03112 #endif 03113 } 03114 03115 //---------------------------------------- 03116 // example code main function 03117 int main() 03118 { 03119 // Configure serial ports 03120 cmdLine_serial.clear(); 03121 //~ cmdLine_serial.serial().printf("\r\n cmdLine_serial.serial().printf test\r\n"); 03122 cmdLine_serial.onEOLcommandParser = main_menu_onEOLcommandParser; 03123 cmdLine_serial.diagnostic_led_EOF = diagnostic_led_EOF; 03124 /// CmdLine::set_immediate_handler(char, functionPointer_void_void_on_immediate_0x21); 03125 cmdLine_serial.on_immediate_0x21 = on_immediate_0x21; 03126 cmdLine_serial.on_immediate_0x7b = on_immediate_0x7b; 03127 cmdLine_serial.on_immediate_0x7d = on_immediate_0x7d; 03128 # if HAS_DAPLINK_SERIAL 03129 cmdLine_DAPLINKserial.clear(); 03130 //~ cmdLine_DAPLINKserial.serial().printf("\r\n cmdLine_DAPLINKserial.serial().printf test\r\n"); 03131 cmdLine_DAPLINKserial.onEOLcommandParser = main_menu_onEOLcommandParser; 03132 /// @todo CmdLine::set_immediate_handler(char, functionPointer_void_void_on_immediate_0x21); 03133 cmdLine_DAPLINKserial.on_immediate_0x21 = on_immediate_0x21; 03134 cmdLine_DAPLINKserial.on_immediate_0x7b = on_immediate_0x7b; 03135 cmdLine_DAPLINKserial.on_immediate_0x7d = on_immediate_0x7d; 03136 # endif 03137 03138 03139 //print_banner(); 03140 03141 03142 03143 #if USE_LEDS 03144 #if defined(TARGET_MAX32630) 03145 led1 = LED_ON; led2 = LED_OFF; led3 = LED_OFF; // diagnostic rbg led RED 03146 ThisThread::sleep_for(125); // [since mbed-os-5.10] vs Thread::wait(125); 03147 led1 = LED_OFF; led2 = LED_ON; led3 = LED_OFF; // diagnostic rbg led GREEN 03148 ThisThread::sleep_for(125); // [since mbed-os-5.10] vs Thread::wait(125); 03149 led1 = LED_OFF; led2 = LED_OFF; led3 = LED_ON; // diagnostic rbg led BLUE 03150 ThisThread::sleep_for(125); // [since mbed-os-5.10] vs Thread::wait(125); 03151 led1 = LED_ON; led2 = LED_ON; led3 = LED_ON; // diagnostic rbg led RED+GREEN+BLUE=WHITE 03152 ThisThread::sleep_for(125); // [since mbed-os-5.10] vs Thread::wait(125); 03153 led1 = LED_OFF; led2 = LED_ON; led3 = LED_ON; // diagnostic rbg led GREEN+BLUE=CYAN 03154 ThisThread::sleep_for(125); // [since mbed-os-5.10] vs Thread::wait(125); 03155 led1 = LED_ON; led2 = LED_OFF; led3 = LED_ON; // diagnostic rbg led RED+BLUE=MAGENTA 03156 ThisThread::sleep_for(125); // [since mbed-os-5.10] vs Thread::wait(125); 03157 led1 = LED_ON; led2 = LED_ON; led3 = LED_OFF; // diagnostic rbg led RED+GREEN=YELLOW 03158 ThisThread::sleep_for(125); // [since mbed-os-5.10] vs Thread::wait(125); 03159 led1 = LED_OFF; led2 = LED_OFF; led3 = LED_OFF; // diagnostic rbg led BLACK 03160 ThisThread::sleep_for(125); // [since mbed-os-5.10] vs Thread::wait(125); 03161 #elif defined(TARGET_MAX32625MBED) 03162 led1 = LED_ON; led2 = LED_OFF; led3 = LED_OFF; // diagnostic rbg led RED 03163 ThisThread::sleep_for(125); // [since mbed-os-5.10] vs Thread::wait(125); 03164 led1 = LED_OFF; led2 = LED_ON; led3 = LED_OFF; // diagnostic rbg led GREEN 03165 ThisThread::sleep_for(125); // [since mbed-os-5.10] vs Thread::wait(125); 03166 led1 = LED_OFF; led2 = LED_OFF; led3 = LED_ON; // diagnostic rbg led BLUE 03167 ThisThread::sleep_for(125); // [since mbed-os-5.10] vs Thread::wait(125); 03168 led1 = LED_ON; led2 = LED_ON; led3 = LED_ON; // diagnostic rbg led RED+GREEN+BLUE=WHITE 03169 ThisThread::sleep_for(125); // [since mbed-os-5.10] vs Thread::wait(125); 03170 led1 = LED_OFF; led2 = LED_ON; led3 = LED_ON; // diagnostic rbg led GREEN+BLUE=CYAN 03171 ThisThread::sleep_for(125); // [since mbed-os-5.10] vs Thread::wait(125); 03172 led1 = LED_ON; led2 = LED_OFF; led3 = LED_ON; // diagnostic rbg led RED+BLUE=MAGENTA 03173 ThisThread::sleep_for(125); // [since mbed-os-5.10] vs Thread::wait(125); 03174 led1 = LED_ON; led2 = LED_ON; led3 = LED_OFF; // diagnostic rbg led RED+GREEN=YELLOW 03175 ThisThread::sleep_for(125); // [since mbed-os-5.10] vs Thread::wait(125); 03176 led1 = LED_OFF; led2 = LED_OFF; led3 = LED_OFF; // diagnostic rbg led BLACK 03177 ThisThread::sleep_for(125); // [since mbed-os-5.10] vs Thread::wait(125); 03178 #else // not defined(TARGET_LPC1768 etc.) 03179 led1 = LED_ON; 03180 led2 = LED_OFF; 03181 led3 = LED_OFF; 03182 led4 = LED_OFF; 03183 ThisThread::sleep_for(75); // [since mbed-os-5.10] vs Thread::wait(75); 03184 //led1 = LED_ON; 03185 led2 = LED_ON; 03186 ThisThread::sleep_for(75); // [since mbed-os-5.10] vs Thread::wait(75); 03187 led1 = LED_OFF; 03188 //led2 = LED_ON; 03189 led3 = LED_ON; 03190 ThisThread::sleep_for(75); // [since mbed-os-5.10] vs Thread::wait(75); 03191 led2 = LED_OFF; 03192 //led3 = LED_ON; 03193 led4 = LED_ON; 03194 ThisThread::sleep_for(75); // [since mbed-os-5.10] vs Thread::wait(75); 03195 led3 = LED_OFF; 03196 led4 = LED_ON; 03197 // 03198 #endif // target definition 03199 #endif 03200 03201 // cmd_TE(); 03202 03203 // #if USE_LEDS 03204 // rgb_led.white(); // diagnostic rbg led RED+GREEN+BLUE=WHITE 03205 // #endif // USE_LEDS 03206 led1 = LED_ON; 03207 led2 = LED_ON; 03208 led3 = LED_ON; 03209 03210 InitializeConfiguration(); 03211 // CODE GENERATOR: example code: member function Init 03212 g_MAX11043_device.Init(); 03213 03214 // example code: serial port banner message 03215 #if defined(TARGET_MAX32625MBED) 03216 serial.printf("MAX32625MBED "); 03217 #elif defined(TARGET_MAX32600MBED) 03218 serial.printf("MAX32600MBED "); 03219 #elif defined(TARGET_NUCLEO_F446RE) 03220 serial.printf("NUCLEO_F446RE "); 03221 #endif 03222 serial.printf("MAX11043BOB\r\n"); 03223 03224 03225 while (1) { 03226 #if HAS_BUTTON1_DEMO_INTERRUPT_POLLING 03227 // avoid runtime error on button1 press [mbed-os-5.11] 03228 // instead of using InterruptIn, use DigitalIn and poll in main while(1) 03229 # if HAS_BUTTON1_DEMO_INTERRUPT 03230 static int button1_value_prev = 1; 03231 static int button1_value_now = 1; 03232 button1_value_prev = button1_value_now; 03233 button1_value_now = button1.read(); 03234 if ((button1_value_prev - button1_value_now) == 1) 03235 { 03236 // on button1 falling edge (button1 press) 03237 onButton1FallingEdge(); 03238 } 03239 # endif // HAS_BUTTON1_DEMO_INTERRUPT 03240 # if HAS_BUTTON2_DEMO_INTERRUPT 03241 static int button2_value_prev = 1; 03242 static int button2_value_now = 1; 03243 button2_value_prev = button2_value_now; 03244 button2_value_now = button2.read(); 03245 if ((button2_value_prev - button2_value_now) == 1) 03246 { 03247 // on button2 falling edge (button2 press) 03248 onButton2FallingEdge(); 03249 } 03250 # endif // HAS_BUTTON2_DEMO_INTERRUPT 03251 #endif 03252 # if HAS_DAPLINK_SERIAL 03253 if (DAPLINKserial.readable()) { 03254 cmdLine_DAPLINKserial.append(DAPLINKserial.getc()); 03255 } 03256 # endif // HAS_DAPLINK_SERIAL 03257 if (serial.readable()) { 03258 int c = serial.getc(); 03259 cmdLine_serial.append(c); 03260 #if IGNORE_AT_COMMANDS 03261 # if HAS_DAPLINK_SERIAL 03262 cmdLine_DAPLINKserial.serial().printf("%c", c); 03263 # endif // HAS_DAPLINK_SERIAL 03264 #endif // IGNORE_AT_COMMANDS 03265 // 03266 } 03267 } // while(1) 03268 } 03269 //---------- CODE GENERATOR: end testMainCppCodeList
Generated on Wed Jul 13 2022 22:23:56 by
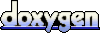