
Test program running on MAX32625MBED. Control through USB Serial commands using a terminal emulator such as teraterm or putty.
Dependencies: MaximTinyTester CmdLine MAX541 USBDevice
MAX11043.h
00001 // /******************************************************************************* 00002 // * Copyright (C) 2020 Maxim Integrated Products, Inc., All Rights Reserved. 00003 // * 00004 // * Permission is hereby granted, free of charge, to any person obtaining a 00005 // * copy of this software and associated documentation files (the "Software"), 00006 // * to deal in the Software without restriction, including without limitation 00007 // * the rights to use, copy, modify, merge, publish, distribute, sublicense, 00008 // * and/or sell copies of the Software, and to permit persons to whom the 00009 // * Software is furnished to do so, subject to the following conditions: 00010 // * 00011 // * The above copyright notice and this permission notice shall be included 00012 // * in all copies or substantial portions of the Software. 00013 // * 00014 // * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00015 // * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00016 // * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00017 // * IN NO EVENT SHALL MAXIM INTEGRATED BE LIABLE FOR ANY CLAIM, DAMAGES 00018 // * OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00019 // * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00020 // * OTHER DEALINGS IN THE SOFTWARE. 00021 // * 00022 // * Except as contained in this notice, the name of Maxim Integrated 00023 // * Products, Inc. shall not be used except as stated in the Maxim Integrated 00024 // * Products, Inc. Branding Policy. 00025 // * 00026 // * The mere transfer of this software does not imply any licenses 00027 // * of trade secrets, proprietary technology, copyrights, patents, 00028 // * trademarks, maskwork rights, or any other form of intellectual 00029 // * property whatsoever. Maxim Integrated Products, Inc. retains all 00030 // * ownership rights. 00031 // ******************************************************************************* 00032 // */ 00033 // ********************************************************************* 00034 // @file MAX11043.h 00035 // ********************************************************************* 00036 // Header file 00037 // DO NOT EDIT; except areas designated "CUSTOMIZE". Automatically generated file. * MANUAL EDITS PRESENT * 00038 // generated by XMLSystemOfDevicesToMBED.py 00039 // System Name = ExampleSystem 00040 // System Description = Device driver example 00041 // Device Name = MAX11043 00042 // Device Description = 200ksps, Low-Power, Serial SPI 24-Bit, 4-Channel, Differential/Single-Ended Input, Simultaneous-Sampling SD ADC 00043 // Device DeviceBriefDescription = 24-bit 200ksps Delta-Sigma ADC 00044 // Device Manufacturer = Maxim Integrated 00045 // Device PartNumber = MAX11043ATL+ 00046 // Device RegValue_Width = DataWidth16bit_HL 00047 // 00048 // ADC MaxOutputDataRate = 200ksps 00049 // ADC NumChannels = 4 00050 // ADC ResolutionBits = 24 00051 // 00052 // SPI CS = ActiveLow 00053 // SPI FrameStart = CS 00054 // SPI CPOL = 0 00055 // SPI CPHA = 0 00056 // SPI MOSI and MISO Data are both stable on Rising edge of SCLK 00057 // SPI SCLK Idle Low 00058 // SPI SCLKMaxMHz = 40 00059 // SPI SCLKMinMHz = 0 00060 // 00061 00062 00063 // Prevent multiple declaration 00064 #ifndef __MAX11043_H__ 00065 #define __MAX11043_H__ 00066 00067 //-------------------------------------------------- 00068 // MAX11043 ADC Read operations must be synchronized to EOC End Of Conversion 00069 // EOC# asserts low when new data is available. 00070 // Initiate a data read prior to the next rising edge of EOC# or the result is overwritten. 00071 #ifndef MAX11043_EOC_INTERRUPT_POLLING 00072 #define MAX11043_EOC_INTERRUPT_POLLING 1 00073 #endif // MAX11043_EOC_INTERRUPT_POLLING 00074 //-------------------------------------------------- 00075 // MAX11043 ADC Read operations must be synchronized to EOC End Of Conversion 00076 // EOC# asserts low when new data is available. 00077 // Initiate a data read prior to the next rising edge of EOC# or the result is overwritten. 00078 #if MAX11043_EOC_INTERRUPT_POLLING 00079 // MAX11043 EOC End Of Conversion input should be InterruptIn(PinName:EOC_pin).fall(onEOCFallingEdge); 00080 // Workaround using DigitalIn(PinName:EOC_pin) polled to sync with EOC falling edge for ADC reads 00081 // 2020-02-20 MAX11043_EOC_INTERRUPT_POLLING works on MAX32625MBED at 9us conversion rate, with 1us timing margin 00082 #else // MAX11043_EOC_INTERRUPT_POLLING 00083 // MAX11043 EOC End Of Conversion input is InterruptIn(PinName:EOC_pin).fall(onEOCFallingEdge); 00084 #endif // MAX11043_EOC_INTERRUPT_POLLING 00085 //-------------------------------------------------- 00086 00087 // standard include for target platform -- Platform_Include_Boilerplate 00088 #include "mbed.h" 00089 // Platforms: 00090 // - MAX32625MBED 00091 // - supports mbed-os-5.11, requires USBDevice library 00092 // - add https://developer.mbed.org/teams/MaximIntegrated/code/USBDevice/ 00093 // - remove max32630fthr library (if present) 00094 // - remove MAX32620FTHR library (if present) 00095 // - MAX32600MBED 00096 // - remove max32630fthr library (if present) 00097 // - remove MAX32620FTHR library (if present) 00098 // - Windows 10 note: Don't connect HDK until you are ready to load new firmware into the board. 00099 // - NUCLEO_F446RE 00100 // - remove USBDevice library 00101 // - remove max32630fthr library (if present) 00102 // - remove MAX32620FTHR library (if present) 00103 // - NUCLEO_F401RE 00104 // - remove USBDevice library 00105 // - remove max32630fthr library (if present) 00106 // - remove MAX32620FTHR library (if present) 00107 // - MAX32630FTHR 00108 // - #include "max32630fthr.h" 00109 // - add http://os.mbed.org/teams/MaximIntegrated/code/max32630fthr/ 00110 // - remove MAX32620FTHR library (if present) 00111 // - MAX32620FTHR 00112 // - #include "MAX32620FTHR.h" 00113 // - remove max32630fthr library (if present) 00114 // - add https://os.mbed.com/teams/MaximIntegrated/code/MAX32620FTHR/ 00115 // - not tested yet 00116 // - MAX32625PICO 00117 // - remove max32630fthr library (if present) 00118 // - remove MAX32620FTHR library (if present) 00119 // - not tested yet 00120 // 00121 // end Platform_Include_Boilerplate 00122 00123 // CODE GENERATOR: conditional defines 00124 // CODE GENERATOR: class declaration and docstrings 00125 /** 00126 * @brief MAX11043 200ksps, Low-Power, Serial SPI 24-Bit, 4-Channel, Differential/Single-Ended Input, Simultaneous-Sampling SD ADC 00127 * 00128 * 00129 * 00130 * Datasheet: https://www.maximintegrated.com/MAX11043 00131 * 00132 * 00133 * 00134 * //---------- CODE GENERATOR: helloCppCodeList 00135 * @code 00136 * // CODE GENERATOR: example code includes 00137 * 00138 * // example code includes 00139 * // standard include for target platform -- Platform_Include_Boilerplate 00140 * #include "mbed.h" 00141 * // Platforms: 00142 * // - MAX32625MBED 00143 * // - supports mbed-os-5.11, requires USBDevice library 00144 * // - add https://developer.mbed.org/teams/MaximIntegrated/code/USBDevice/ 00145 * // - remove max32630fthr library (if present) 00146 * // - remove MAX32620FTHR library (if present) 00147 * // - MAX32600MBED 00148 * // - remove max32630fthr library (if present) 00149 * // - remove MAX32620FTHR library (if present) 00150 * // - Windows 10 note: Don't connect HDK until you are ready to load new firmware into the board. 00151 * // - NUCLEO_F446RE 00152 * // - remove USBDevice library 00153 * // - remove max32630fthr library (if present) 00154 * // - remove MAX32620FTHR library (if present) 00155 * // - NUCLEO_F401RE 00156 * // - remove USBDevice library 00157 * // - remove max32630fthr library (if present) 00158 * // - remove MAX32620FTHR library (if present) 00159 * // - MAX32630FTHR 00160 * // - #include "max32630fthr.h" 00161 * // - add http://os.mbed.org/teams/MaximIntegrated/code/max32630fthr/ 00162 * // - remove MAX32620FTHR library (if present) 00163 * // - MAX32620FTHR 00164 * // - #include "MAX32620FTHR.h" 00165 * // - remove max32630fthr library (if present) 00166 * // - add https://os.mbed.com/teams/MaximIntegrated/code/MAX32620FTHR/ 00167 * // - not tested yet 00168 * // - MAX32625PICO 00169 * // - remove max32630fthr library (if present) 00170 * // - remove MAX32620FTHR library (if present) 00171 * // - not tested yet 00172 * // 00173 * // end Platform_Include_Boilerplate 00174 * #include "MAX11043.h" 00175 * 00176 * // example code board support 00177 * //MAX32630FTHR pegasus(MAX32630FTHR::VIO_3V3); 00178 * //DigitalOut rLED(LED1); 00179 * //DigitalOut gLED(LED2); 00180 * //DigitalOut bLED(LED3); 00181 * // 00182 * // Arduino "shield" connector port definitions (MAX32625MBED shown) 00183 * #if defined(TARGET_MAX32625MBED) 00184 * #define A0 AIN_0 00185 * #define A1 AIN_1 00186 * #define A2 AIN_2 00187 * #define A3 AIN_3 00188 * #define D0 P0_0 00189 * #define D1 P0_1 00190 * #define D2 P0_2 00191 * #define D3 P0_3 00192 * #define D4 P0_4 00193 * #define D5 P0_5 00194 * #define D6 P0_6 00195 * #define D7 P0_7 00196 * #define D8 P1_4 00197 * #define D9 P1_5 00198 * #define D10 P1_3 00199 * #define D11 P1_1 00200 * #define D12 P1_2 00201 * #define D13 P1_0 00202 * #endif 00203 * 00204 * // example code declare SPI interface 00205 * #if defined(TARGET_MAX32625MBED) 00206 * // SPI spi(SPI1_MOSI, SPI1_MISO, SPI1_SCK); // mosi, miso, sclk spi1 TARGET_MAX32625MBED: P1_1 P1_2 P1_0 Arduino 10-pin header D11 D12 D13 D10 00207 * // DigitalOut spi_cs(SPI1_SS); // TARGET_MAX32625MBED: not connected 00208 * // Support SPI hardware-controlled CS instead of GPIO CS (mbed) 00209 * // 2020-02-19 MAX32625MBED GPIO CS envelope is 23us (11.4us before SCLK and 8.6us after SCLK). 00210 * // 2020-02-19 MAX32625MBED SPI controlled CS envelope 4 channel read reduced to 4.2us (24MHz SCLK), 1.2us setup, 0us hold. 00211 * // 2020-02-19 MAX11043 slowest EOC rate is 9us. 00212 * SPI spi(SPI1_MOSI, SPI1_MISO, SPI1_SCK, SPI1_SS); // mosi, miso, sclk spi1 TARGET_MAX32625MBED: P1_1 P1_2 P1_0 Arduino 10-pin header D11 D12 D13 D10 00213 * DigitalOut spi_cs(NC); // TARGET_MAX32625MBED: not connected 00214 * // PinName NC means NOT_CONNECTED; DigitalOut::is_connected() returns false 00215 * // add m_cs_pin.is_connected() guard before writing m_cs_pin = m_SPI_cs_state 00216 * // to avoid runtime error Assertion failed: obj->name != (PinName)NC 00217 * #elif defined(TARGET_MAX32600MBED) 00218 * SPI spi(SPI2_MOSI, SPI2_MISO, SPI2_SCK); // mosi, miso, sclk spi1 TARGET_MAX32600MBED: Arduino 10-pin header D11 D12 D13 00219 * DigitalOut spi_cs(SPI2_SS); // Generic: Arduino 10-pin header D10 00220 * #else 00221 * SPI spi(D11, D12, D13); // mosi, miso, sclk spi1 TARGET_MAX32600MBED: Arduino 10-pin header D11 D12 D13 00222 * DigitalOut spi_cs(D10); // Generic: Arduino 10-pin header D10 00223 * #endif 00224 * 00225 * // example code declare GPIO interface pins 00226 * DigitalOut CONVRUN_pin(D9); // Digital Configuration Input to MAX11043 device 00227 * DigitalOut SHDN_pin(D8); // Digital Configuration Input to MAX11043 device 00228 * DigitalOut DACSTEP_pin(D7); // Digital Configuration Input to MAX11043 device 00229 * DigitalOut UP_slash_DWNb_pin(D6); // Digital Configuration Input to MAX11043 device 00230 * DigitalIn EOC_pin(D2); // Digital Event Output from MAX11043 device 00231 * // example code declare device instance 00232 * MAX11043 g_MAX11043_device(spi, spi_cs, CONVRUN_pin, SHDN_pin, DACSTEP_pin, UP_slash_DWNb_pin, EOC_pin, MAX11043::MAX11043_IC); 00233 * 00234 * // CODE GENERATOR: example code for ADC: serial port declaration 00235 * //-------------------------------------------------- 00236 * // Declare the Serial driver 00237 * // default baud rate settings are 9600 8N1 00238 * // install device driver from http://developer.mbed.org/media/downloads/drivers/mbedWinSerial_16466.exe 00239 * // see docs https://docs.mbed.com/docs/mbed-os-handbook/en/5.5/getting_started/what_need/ 00240 * #if defined(TARGET_MAX32630) 00241 * #include "USBSerial.h" 00242 * // Hardware serial port over DAPLink 00243 * // The default baud rate for the DapLink UART is 9600 00244 * //Serial DAPLINKserial(P2_1, P2_0); // tx, rx 00245 * // #define HAS_DAPLINK_SERIAL 1 00246 * // Virtual serial port over USB 00247 * // The baud rate does not affect the virtual USBSerial UART. 00248 * USBSerial serial; 00249 * //-------------------------------------------------- 00250 * #elif defined(TARGET_MAX32625MBED) 00251 * #include "USBSerial.h" 00252 * // Hardware serial port over DAPLink 00253 * // The default baud rate for the DapLink UART is 9600 00254 * //Serial DAPLINKserial(P2_1, P2_0); // tx, rx 00255 * // #define HAS_DAPLINK_SERIAL 1 00256 * // Virtual serial port over USB 00257 * // The baud rate does not affect the virtual USBSerial UART. 00258 * USBSerial serial; 00259 * //-------------------------------------------------- 00260 * #elif defined(TARGET_MAX32600) 00261 * #include "USBSerial.h" 00262 * // Hardware serial port over DAPLink 00263 * // The default baud rate for the DapLink UART is 9600 00264 * Serial DAPLINKserial(P1_1, P1_0); // tx, rx 00265 * #define HAS_DAPLINK_SERIAL 1 00266 * // Virtual serial port over USB 00267 * // The baud rate does not affect the virtual USBSerial UART. 00268 * USBSerial serial; 00269 * //-------------------------------------------------- 00270 * #elif defined(TARGET_NUCLEO_F446RE) || defined(TARGET_NUCLEO_F401RE) 00271 * Serial serial(SERIAL_TX, SERIAL_RX); // tx, rx 00272 * //-------------------------------------------------- 00273 * #else 00274 * #if defined(SERIAL_TX) 00275 * #warning "target not previously tested; guess serial pins are SERIAL_TX, SERIAL_RX..." 00276 * Serial serial(SERIAL_TX, SERIAL_RX); // tx, rx 00277 * #elif defined(USBTX) 00278 * #warning "target not previously tested; guess serial pins are USBTX, USBRX..." 00279 * Serial serial(USBTX, USBRX); // tx, rx 00280 * #elif defined(UART_TX) 00281 * #warning "target not previously tested; guess serial pins are UART_TX, UART_RX..." 00282 * Serial serial(UART_TX, UART_RX); // tx, rx 00283 * #else 00284 * #warning "target not previously tested; need to define serial pins..." 00285 * #endif 00286 * #endif 00287 * // 00288 * #include "CmdLine.h" 00289 * CmdLine cmdLine(serial, "serial"); 00290 * 00291 * // example code main function 00292 * int main() 00293 * { 00294 * // CODE GENERATOR: example code: member function Init 00295 * g_MAX11043_device.Init(); 00296 * 00297 * while (1) 00298 * { 00299 * // CODE GENERATOR: example code: has no member function REF 00300 * // CODE GENERATOR: example code for ADC: repeat-forever convert and print conversion result, one record per line 00301 * // CODE GENERATOR: ResolutionBits = 24 00302 * // CODE GENERATOR: FScode = None 00303 * // CODE GENERATOR: NumChannels = 4 00304 * while(1) { // this code repeats forever 00305 * // this code repeats forever 00306 * // CODE GENERATOR: example code: has no member function ScanStandardExternalClock 00307 * // CODE GENERATOR: example code: has no member function ReadAINcode 00308 * // wait(3.0); 00309 * // CODE GENERATOR: print conversion result 00310 * // Use Arduino Serial Plotter to view output: Tools | Serial Plotter 00311 * cmdLine.serial().printf("%d", g_MAX11043_device.AINcode[0]); 00312 * for (int index = 1; index <= channelId_0_3; index++) { 00313 * cmdLine.serial().printf(",%d", g_MAX11043_device.AINcode[index]); 00314 * } 00315 * cmdLine.serial().printf("\r\n"); 00316 * 00317 * } // this code repeats forever 00318 * } 00319 * } 00320 * @endcode 00321 * //---------- CODE GENERATOR: end helloCppCodeList 00322 */ 00323 class MAX11043 { 00324 public: 00325 // CODE GENERATOR: TypedefEnum EnumItem declarations 00326 // CODE GENERATOR: TypedefEnum MAX11043_CMDOP_enum_t 00327 //---------------------------------------- 00328 /// Command Operation Format (see function DecodeCommand) 00329 /// 00330 /// Naming convention is CMDOP_bitstream_OPERATION_NAME 00331 /// - 0aaa_aax0 = 5-bit register address field 00332 /// - 0xxx_xxr0 = read/write bit (1=read, 0=write) 00333 /// - xxxx = don't care 00334 typedef enum MAX11043_CMDOP_enum_t { 00335 CMDOP_0aaa_aa00_WriteRegister = 0x00, //!< 8'b00000000 00336 CMDOP_0aaa_aa10_ReadRegister = 0x02, //!< 8'b00000010 00337 CMDOP_1111_1111_NoOperationMOSIidleHigh = 0xff, //!< 8'b11111111 00338 } MAX11043_CMDOP_enum_t; 00339 00340 // CODE GENERATOR: TypedefEnum MAX11043_CMD_enum_t 00341 //---------------------------------------- 00342 /// Register Addresses 00343 /// 00344 /// Naming convention is CMD_bitstream_WrAddr_FunctionName 00345 /// - first byte format is 0aaa_aar0 00346 /// - 0 = bitstream required 0 bit 00347 /// - aaa_aa = bitstream 5-bit register address field 00348 /// - r = bitstream read/write bit (1=read, 0=write) 00349 /// - WrAddr = Write operation to address Addr 00350 /// - RdAddr = Read operation from address Addr 00351 /// - d8 = 8-bit register data field 00352 /// - d16 = 16-bit register data field 00353 /// - d16o8 = 16-bit or 24-bit register data field 00354 /// - d24 = 24-bit register data field 00355 /// - d32 = 32-bit register data field 00356 /// - x = don't care 00357 typedef enum MAX11043_CMD_enum_t { 00358 CMD_0000_0010_d16o8_Rd00_ADCa = 0x02, //!< 8'b00000010 00359 CMD_0000_0110_d16o8_Rd01_ADCb = 0x06, //!< 8'b00000110 00360 CMD_0000_1010_d16o8_Rd02_ADCc = 0x0a, //!< 8'b00001010 00361 CMD_0000_1110_d16o8_Rd03_ADCd = 0x0e, //!< 8'b00001110 00362 CMD_0001_0010_d16o8_d16o8_Rd04_ADCab = 0x12, //!< 8'b00010010 00363 CMD_0001_0110_d16o8_d16o8_Rd05_ADCcd = 0x16, //!< 8'b00010110 00364 CMD_0001_1010_d16o8_d16o8_d16o8_d16o8_Rd06_ADCabcd = 0x1a, //!< 8'b00011010 00365 CMD_0001_1110_d8_Rd07_Status = 0x1e, //!< 8'b00011110 00366 CMD_0010_0000_d16_Wr08_Configuration = 0x20, //!< 8'b00100000 00367 CMD_0010_0010_d16_Rd08_Configuration = 0x22, //!< 8'b00100010 00368 CMD_0010_0100_d16_Wr09_DAC = 0x24, //!< 8'b00100100 00369 CMD_0010_0110_d16_Rd09_DAC = 0x26, //!< 8'b00100110 00370 CMD_0010_1000_d16_Wr0A_DACStep = 0x28, //!< 8'b00101000 00371 CMD_0010_1010_d16_Rd0A_DACStep = 0x2a, //!< 8'b00101010 00372 CMD_0010_1100_d16_Wr0B_DACHDACL = 0x2c, //!< 8'b00101100 00373 CMD_0010_1110_d16_Rd0B_DACHDACL = 0x2e, //!< 8'b00101110 00374 CMD_0011_0000_d16_Wr0C_ConfigA = 0x30, //!< 8'b00110000 00375 CMD_0011_0010_d16_Rd0C_ConfigA = 0x32, //!< 8'b00110010 00376 CMD_0011_0100_d16_Wr0D_ConfigB = 0x34, //!< 8'b00110100 00377 CMD_0011_0110_d16_Rd0D_ConfigB = 0x36, //!< 8'b00110110 00378 CMD_0011_1000_d16_Wr0E_ConfigC = 0x38, //!< 8'b00111000 00379 CMD_0011_1010_d16_Rd0E_ConfigC = 0x3a, //!< 8'b00111010 00380 CMD_0011_1100_d16_Wr0F_ConfigD = 0x3c, //!< 8'b00111100 00381 CMD_0011_1110_d16_Rd0F_ConfigD = 0x3e, //!< 8'b00111110 00382 CMD_0100_0000_d16_Wr10_Reference = 0x40, //!< 8'b01000000 00383 CMD_0100_0010_d16_Rd10_Reference = 0x42, //!< 8'b01000010 00384 CMD_0100_0100_d16_Wr11_AGain = 0x44, //!< 8'b01000100 00385 CMD_0100_0110_d16_Rd11_AGain = 0x46, //!< 8'b01000110 00386 CMD_0100_1000_d16_Wr12_BGain = 0x48, //!< 8'b01001000 00387 CMD_0100_1010_d16_Rd12_BGain = 0x4a, //!< 8'b01001010 00388 CMD_0100_1100_d16_Wr13_CGain = 0x4c, //!< 8'b01001100 00389 CMD_0100_1110_d16_Rd13_CGain = 0x4e, //!< 8'b01001110 00390 CMD_0101_0000_d16_Wr14_DGain = 0x50, //!< 8'b01010000 00391 CMD_0101_0010_d16_Rd14_DGain = 0x52, //!< 8'b01010010 00392 CMD_0101_0100_d8_Wr15_FilterCAddress = 0x54, //!< 8'b01010100 00393 CMD_0101_0110_d8_Rd15_FilterCAddress = 0x56, //!< 8'b01010110 00394 CMD_0101_1000_d32_Wr16_FilterCDataOut = 0x58, //!< 8'b01011000 00395 CMD_0101_1010_d32_Rd16_FilterCDataOut = 0x5a, //!< 8'b01011010 00396 CMD_0101_1100_d32_Wr17_FilterCDataIn = 0x5c, //!< 8'b01011100 00397 CMD_0101_1110_d32_Rd17_FilterCDataIn = 0x5e, //!< 8'b01011110 00398 CMD_0110_0000_d8_Wr18_FlashMode = 0x60, //!< 8'b01100000 00399 CMD_0110_0010_d8_Rd18_FlashMode = 0x62, //!< 8'b01100010 00400 CMD_0110_0100_d16_Wr19_FlashAddr = 0x64, //!< 8'b01100100 00401 CMD_0110_0110_d16_Rd19_FlashAddr = 0x66, //!< 8'b01100110 00402 CMD_0110_1000_d16_Wr1A_FlashDataIn = 0x68, //!< 8'b01101000 00403 CMD_0110_1010_d16_Rd1A_FlashDataIn = 0x6a, //!< 8'b01101010 00404 CMD_0110_1110_d16_Rd1B_FlashDataOut = 0x6e, //!< 8'b01101110 00405 } MAX11043_CMD_enum_t; 00406 00407 // CODE GENERATOR: TypedefEnum MAX11043_STATUS_enum_t 00408 //---------------------------------------- 00409 /// CMD_0001_1110_d8_Rd07_Status regAddr=07h 00410 /// Status: x x FlashBusy BOOT OFLGA OFLGB OFLGC OFLGD 00411 typedef enum MAX11043_STATUS_enum_t { 00412 STATUS_xxxx_xxx1_OverflowFlagOFLGD = 0x01, //!< 8'b00000001 00413 STATUS_xxxx_xx1x_OverflowFlagOFLGC = 0x02, //!< 8'b00000010 00414 STATUS_xxxx_x1xx_OverflowFlagOFLGB = 0x04, //!< 8'b00000100 00415 STATUS_xxxx_1xxx_OverflowFlagOFLGA = 0x08, //!< 8'b00001000 00416 STATUS_xxx1_xxxx_PowerOnResetBOOT = 0x10, //!< 8'b00010000 00417 STATUS_xx1x_xxxx_FlashBusy = 0x20, //!< 8'b00100000 00418 STATUS_x1xx_xxxx_RESERVED = 0x40, //!< 8'b01000000 00419 STATUS_1xxx_xxxx_RESERVED = 0x80, //!< 8'b10000000 00420 } MAX11043_STATUS_enum_t; 00421 00422 // CODE GENERATOR: TypedefEnum MAX11043_CONFIG_enum_t 00423 //---------------------------------------- 00424 /// CMD_0010_0000_d16_Wr08_Configuration regAddr=08h 00425 /// Config: EXTCLK CLKDIV1 CLKDIV0 PD PDA PDB PDC PDD PDDAC PDOSC 24BIT SCHANA SCHANB SCHANC SCHAND DECSEL 00426 typedef enum MAX11043_CONFIG_enum_t { 00427 CONFIG_xxxx_xxxx_xxxx_xxx1_DECSEL12 = 0x0001, //!< 16'b0000000000000001 00428 CONFIG_xxxx_xxxx_xxxx_xx1x_SCHAND = 0x0002, //!< 16'b0000000000000010 00429 CONFIG_xxxx_xxxx_xxxx_x1xx_SCHANC = 0x0004, //!< 16'b0000000000000100 00430 CONFIG_xxxx_xxxx_xxxx_1xxx_SCHANB = 0x0008, //!< 16'b0000000000001000 00431 CONFIG_xxxx_xxxx_xxx1_xxxx_SCHANA = 0x0010, //!< 16'b0000000000010000 00432 CONFIG_xxxx_xxxx_xx1x_xxxx_24BIT = 0x0020, //!< 16'b0000000000100000 00433 CONFIG_xxxx_xxxx_x1xx_xxxx_PDOSC = 0x0040, //!< 16'b0000000001000000 00434 CONFIG_xxxx_xxxx_1xxx_xxxx_PDDAC = 0x0080, //!< 16'b0000000010000000 00435 CONFIG_xxxx_xxx1_xxxx_xxxx_PDD = 0x0100, //!< 16'b0000000100000000 00436 CONFIG_xxxx_xx1x_xxxx_xxxx_PDC = 0x0200, //!< 16'b0000001000000000 00437 CONFIG_xxxx_x1xx_xxxx_xxxx_PDB = 0x0400, //!< 16'b0000010000000000 00438 CONFIG_xxxx_1xxx_xxxx_xxxx_PDA = 0x0800, //!< 16'b0000100000000000 00439 CONFIG_xxx1_xxxx_xxxx_xxxx_PD = 0x1000, //!< 16'b0001000000000000 00440 CONFIG_xx1x_xxxx_xxxx_xxxx_CLKDIV0 = 0x2000, //!< 16'b0010000000000000 00441 CONFIG_x1xx_xxxx_xxxx_xxxx_CLKDIV1 = 0x4000, //!< 16'b0100000000000000 00442 CONFIG_1xxx_xxxx_xxxx_xxxx_EXTCLK = 0x8000, //!< 16'b1000000000000000 00443 } MAX11043_CONFIG_enum_t; 00444 00445 // CODE GENERATOR: TypedefEnum MAX11043_CONFIGABCD_enum_t 00446 //---------------------------------------- 00447 /// CMD_0011_0000_d16_Wr0C_ConfigA regAddr=0Ch 00448 /// CMD_0011_0100_d16_Wr0D_ConfigB regAddr=0Dh 00449 /// CMD_0011_1000_d16_Wr0E_ConfigC regAddr=0Eh 00450 /// CMD_0011_1100_d16_Wr0F_ConfigD regAddr=0Fh 00451 /// ConfigABCD: x x x BDAC[3:0] DIFF EQ MODG[1:0] PDPGA FILT PGAG ENBIASP ENBIASN 00452 typedef enum MAX11043_CONFIGABCD_enum_t { 00453 CONFIGABCD_xxxx_xxxx_xxxx_xxx1_ENBIASN = 0x0001, //!< 16'b0000000000000001 00454 CONFIGABCD_xxxx_xxxx_xxxx_xx1x_ENBIASP = 0x0002, //!< 16'b0000000000000010 00455 CONFIGABCD_xxxx_xxxx_xxxx_x1xx_PGAG16 = 0x0004, //!< 16'b0000000000000100 00456 CONFIGABCD_xxxx_xxxx_xxxx_1xxx_FILTLP = 0x0008, //!< 16'b0000000000001000 00457 CONFIGABCD_xxxx_xxxx_xxx1_xxxx_PDPGA = 0x0010, //!< 16'b0000000000010000 00458 CONFIGABCD_xxxx_xxxx_xx1x_xxxx_MODG0 = 0x0020, //!< 16'b0000000000100000 00459 CONFIGABCD_xxxx_xxxx_x1xx_xxxx_MODG1 = 0x0040, //!< 16'b0000000001000000 00460 CONFIGABCD_xxxx_xxxx_1xxx_xxxx_EQ = 0x0080, //!< 16'b0000000010000000 00461 CONFIGABCD_xxxx_xxx1_xxxx_xxxx_DIFF = 0x0100, //!< 16'b0000000100000000 00462 CONFIGABCD_xxxx_xx1x_xxxx_xxxx_BDAC0 = 0x0200, //!< 16'b0000001000000000 00463 CONFIGABCD_xxxx_x1xx_xxxx_xxxx_BDAC1 = 0x0400, //!< 16'b0000010000000000 00464 CONFIGABCD_xxxx_1xxx_xxxx_xxxx_BDAC2 = 0x0800, //!< 16'b0000100000000000 00465 CONFIGABCD_xxx1_xxxx_xxxx_xxxx_BDAC3 = 0x1000, //!< 16'b0001000000000000 00466 CONFIGABCD_xx1x_xxxx_xxxx_xxxx_RESERVED = 0x2000, //!< 16'b0010000000000000 00467 CONFIGABCD_x1xx_xxxx_xxxx_xxxx_RESERVED = 0x4000, //!< 16'b0100000000000000 00468 CONFIGABCD_1xxx_xxxx_xxxx_xxxx_RESERVED = 0x8000, //!< 16'b1000000000000000 00469 } MAX11043_CONFIGABCD_enum_t; 00470 00471 // CODE GENERATOR: TypedefEnum MAX11043_BDAC_enum_t 00472 //---------------------------------------- 00473 /// CMD_0011_0000_d16_Wr0C_ConfigA regAddr=0Ch 00474 /// CMD_0011_0100_d16_Wr0D_ConfigB regAddr=0Dh 00475 /// CMD_0011_1000_d16_Wr0E_ConfigC regAddr=0Eh 00476 /// CMD_0011_1100_d16_Wr0F_ConfigD regAddr=0Fh 00477 /// 00478 /// CONFIGABCD_xxx1_xxxx_xxxx_xxxx_BDAC3 00479 /// CONFIGABCD_xxxx_1xxx_xxxx_xxxx_BDAC2 00480 /// CONFIGABCD_xxxx_x1xx_xxxx_xxxx_BDAC1 00481 /// CONFIGABCD_xxxx_xx1x_xxxx_xxxx_BDAC0 00482 /// Sets the input bias voltage for AC-coupled signals when ENBIAS_ is set to 1. 00483 /// ConfigABCD: x x x BDAC[3:0] DIFF EQ MODG[1:0] PDPGA FILT PGAG ENBIASP ENBIASN 00484 typedef enum MAX11043_BDAC_enum_t { 00485 BDAC_0000_033pctAVDD = 0x00, //!< 8'b00000000 00486 BDAC_0001_035pctAVDD = 0x01, //!< 8'b00000001 00487 BDAC_0010_038pctAVDD = 0x02, //!< 8'b00000010 00488 BDAC_0011_040pctAVDD = 0x03, //!< 8'b00000011 00489 BDAC_0100_042pctAVDD = 0x04, //!< 8'b00000100 00490 BDAC_0101_044pctAVDD = 0x05, //!< 8'b00000101 00491 BDAC_0110_046pctAVDD = 0x06, //!< 8'b00000110 00492 BDAC_0111_048pctAVDD = 0x07, //!< 8'b00000111 00493 BDAC_1000_050pctAVDD = 0x08, //!< 8'b00001000 00494 BDAC_1001_052pctAVDD = 0x09, //!< 8'b00001001 00495 BDAC_1010_054pctAVDD = 0x0a, //!< 8'b00001010 00496 BDAC_1011_056pctAVDD = 0x0b, //!< 8'b00001011 00497 BDAC_1100_058pctAVDD = 0x0c, //!< 8'b00001100 00498 BDAC_1101_060pctAVDD = 0x0d, //!< 8'b00001101 00499 BDAC_1110_062pctAVDD = 0x0e, //!< 8'b00001110 00500 BDAC_1111_065pctAVDD = 0x0f, //!< 8'b00001111 00501 } MAX11043_BDAC_enum_t; 00502 00503 // CODE GENERATOR: TypedefEnum MAX11043_REFERENCE_enum_t 00504 //---------------------------------------- 00505 /// CMD_0100_0000_d16_Wr10_Reference regAddr=10h 00506 /// ReferenceDelay: 0 0 0 PURGE[4:0] EXTREF EXBUFA EXBUFB EXBUFC EXBUFD EXBUFDAC EXBUFDACH EXBUFDACL 00507 typedef enum MAX11043_REFERENCE_enum_t { 00508 REFERENCE_xxxx_xxxx_xxxx_xxx1_EXBUFDACL = 0x0001, //!< 16'b0000000000000001 00509 REFERENCE_xxxx_xxxx_xxxx_xx1x_EXBUFDACH = 0x0002, //!< 16'b0000000000000010 00510 REFERENCE_xxxx_xxxx_xxxx_x1xx_EXBUFDAC = 0x0004, //!< 16'b0000000000000100 00511 REFERENCE_xxxx_xxxx_xxxx_1xxx_EXBUFD = 0x0008, //!< 16'b0000000000001000 00512 REFERENCE_xxxx_xxxx_xxx1_xxxx_EXBUFC = 0x0010, //!< 16'b0000000000010000 00513 REFERENCE_xxxx_xxxx_xx1x_xxxx_EXBUFB = 0x0020, //!< 16'b0000000000100000 00514 REFERENCE_xxxx_xxxx_x1xx_xxxx_EXBUFA = 0x0040, //!< 16'b0000000001000000 00515 REFERENCE_xxxx_xxxx_1xxx_xxxx_EXTREF = 0x0080, //!< 16'b0000000010000000 00516 REFERENCE_xxxx_xxx1_xxxx_xxxx_PURGE0 = 0x0100, //!< 16'b0000000100000000 00517 REFERENCE_xxxx_xx1x_xxxx_xxxx_PURGE1 = 0x0200, //!< 16'b0000001000000000 00518 REFERENCE_xxxx_x1xx_xxxx_xxxx_PURGE2 = 0x0400, //!< 16'b0000010000000000 00519 REFERENCE_xxxx_1xxx_xxxx_xxxx_PURGE3 = 0x0800, //!< 16'b0000100000000000 00520 REFERENCE_xxx1_xxxx_xxxx_xxxx_PURGE4 = 0x1000, //!< 16'b0001000000000000 00521 REFERENCE_xx1x_xxxx_xxxx_xxxx_RESERVED = 0x2000, //!< 16'b0010000000000000 00522 REFERENCE_x1xx_xxxx_xxxx_xxxx_RESERVED = 0x4000, //!< 16'b0100000000000000 00523 REFERENCE_1xxx_xxxx_xxxx_xxxx_RESERVED = 0x8000, //!< 16'b1000000000000000 00524 } MAX11043_REFERENCE_enum_t; 00525 00526 // TODO1: CODE GENERATOR: ic_variant -- IC's supported with this driver 00527 /** 00528 * @brief IC's supported with this driver 00529 * @details MAX11043 00530 */ 00531 typedef enum 00532 { 00533 MAX11043_IC = 0, 00534 //MAX11043_IC = 1 00535 } MAX11043_ic_t; 00536 00537 // TODO1: CODE GENERATOR: class constructor declaration 00538 /**********************************************************//** 00539 * @brief Constructor for MAX11043 Class. 00540 * 00541 * @details Requires an existing SPI object as well as a DigitalOut object. 00542 * The DigitalOut object is used for a chip enable signal 00543 * 00544 * On Entry: 00545 * @param[in] spi - pointer to existing SPI object 00546 * @param[in] cs_pin - pointer to a DigitalOut pin object 00547 * CODE GENERATOR: class constructor docstrings gpio InputPin pins 00548 * @param[in] CONVRUN_pin - pointer to a DigitalOut pin object 00549 * @param[in] SHDN_pin - pointer to a DigitalOut pin object 00550 * @param[in] DACSTEP_pin - pointer to a DigitalOut pin object 00551 * @param[in] UP_slash_DWNb_pin - pointer to a DigitalOut pin object 00552 * CODE GENERATOR: class constructor docstrings gpio OutputPin pins 00553 * @param[in] EOC_pin - pointer to a DigitalIn pin object 00554 * @param[in] ic_variant - which type of MAX11043 is used 00555 * 00556 * On Exit: 00557 * 00558 * @return None 00559 **************************************************************/ 00560 MAX11043(SPI &spi, DigitalOut &cs_pin, // SPI interface 00561 // CODE GENERATOR: class constructor declaration gpio InputPin pins 00562 DigitalOut &CONVRUN_pin, // Digital Configuration Input to MAX11043 device 00563 DigitalOut &SHDN_pin, // Digital Configuration Input to MAX11043 device 00564 DigitalOut &DACSTEP_pin, // Digital Configuration Input to MAX11043 device 00565 DigitalOut &UP_slash_DWNb_pin, // Digital Configuration Input to MAX11043 device 00566 // CODE GENERATOR: class constructor declaration gpio OutputPin pins 00567 // MAX11043 ADC Read operations must be synchronized to EOC End Of Conversion 00568 #if MAX11043_EOC_INTERRUPT_POLLING 00569 // MAX11043 EOC End Of Conversion input should be InterruptIn(PinName:EOC_pin).fall(onEOCFallingEdge); 00570 // Workaround using DigitalIn(PinName:EOC_pin) polled to sync with EOC falling edge for ADC reads 00571 // TODO: onEOCFallingEdge: replace DigitalIn &EOC_pin with PinName EOC_pin, so that I can create an InterruptIn(PinName:EOC_pin) 00572 DigitalIn &EOC_pin, // Digital Event Output from MAX11043 device 00573 #else // MAX11043_EOC_INTERRUPT_POLLING 00574 // MAX11043 EOC End Of Conversion input is InterruptIn(PinName:EOC_pin).fall(onEOCFallingEdge); 00575 InterruptIn &EOC_pin, // Digital Event Output from MAX11043 device 00576 #endif // MAX11043_EOC_INTERRUPT_POLLING 00577 MAX11043_ic_t ic_variant); 00578 00579 // CODE GENERATOR: class destructor declaration 00580 /************************************************************ 00581 * @brief Default destructor for MAX11043 Class. 00582 * 00583 * @details Destroys SPI object if owner 00584 * 00585 * On Entry: 00586 * 00587 * On Exit: 00588 * 00589 * @return None 00590 **************************************************************/ 00591 ~MAX11043(); 00592 00593 // CODE GENERATOR: Declare SPI diagnostic function pointer void onSPIprint() 00594 /// Function pointer void f(size_t byteCount, uint8_t mosiData[], uint8_t misoData[]) 00595 Callback<void(size_t, uint8_t*, uint8_t*)> onSPIprint; //!< optional @ref onSPIprint SPI diagnostic function 00596 00597 // CODE GENERATOR: spi_frequency setter declaration 00598 /// set SPI SCLK frequency 00599 void spi_frequency(int spi_sclk_Hz); 00600 00601 // CODE GENERATOR: spi_frequency getter declaration and definition 00602 /// get SPI SCLK frequency 00603 int get_spi_frequency() const { return m_SPI_SCLK_Hz; } 00604 00605 // CODE GENERATOR: spi_dataMode getter declaration and definition 00606 /// get SPI mode 00607 int get_spi_dataMode() const { return m_SPI_dataMode; } 00608 00609 //---------------------------------------- 00610 // CODE GENERATOR: omit typedef enum MAX11043_device_t, class members instead of global device object 00611 public: 00612 00613 /// reference voltage, in Volts 00614 double VRef; 00615 00616 /// shadow of register config CMD_0010_0010_d16_Rd08_Configuration 00617 uint16_t config; 00618 00619 /// shadow of register status CMD_0001_1110_d8_Rd07_Status 00620 uint8_t status; 00621 00622 /// shadow of register ADCa CMD_0000_0010_d16o8_Rd00_ADCa 00623 int adca; 00624 00625 /// shadow of register ADCb CMD_0000_0110_d16o8_Rd01_ADCb 00626 int adcb; 00627 00628 /// shadow of register ADCc CMD_0000_1010_d16o8_Rd02_ADCc 00629 int adcc; 00630 00631 /// shadow of register ADCd CMD_0000_1110_d16o8_Rd03_ADCd 00632 int adcd; 00633 00634 // CODE GENERATOR: omit global g_MAX11043_device 00635 00636 // CODE GENERATOR: extern function declarations 00637 // CODE GENERATOR: extern function declaration SPIoutputCS 00638 //---------------------------------------- 00639 // Assert SPI Chip Select 00640 // SPI chip-select for MAX11043 00641 // 00642 void SPIoutputCS(int isLogicHigh); 00643 00644 // CODE GENERATOR: extern function declaration SPIreadWriteWithLowCS 00645 //---------------------------------------- 00646 // SPI read and write arbitrary number of 8-bit bytes 00647 // SPI interface to MAX11043 shift mosiData into MAX11043 DIN 00648 // while simultaneously capturing miso data from MAX11043 DOUT 00649 // 00650 int SPIreadWriteWithLowCS(size_t byteCount, uint8_t mosiData[], uint8_t misoData[]); 00651 00652 // CODE GENERATOR: extern function declaration SHDNoutputValue 00653 //---------------------------------------- 00654 // Assert MAX11043 SHDN pin : High = Shut Down, Low = Normal Operation. 00655 // 00656 void SHDNoutputValue(int isLogicHigh); 00657 00658 // CODE GENERATOR: extern function declaration CONVRUNoutputValue 00659 //---------------------------------------- 00660 // Assert MAX11043 CONVRUN pin : High = start continuous conversions on all 4 channels, Low = Idle. 00661 // 00662 void CONVRUNoutputValue(int isLogicHigh); 00663 00664 // CODE GENERATOR: extern function declaration CONVRUNoutputGetValue 00665 //---------------------------------------- 00666 // Return the state being driven onto the MAX11043 CONVRUN pin. 00667 // 00668 int CONVRUNoutputGetValue(); 00669 00670 // CODE GENERATOR: extern function declaration DACSTEPoutputValue 00671 //---------------------------------------- 00672 // Assert MAX11043 DACSTEP pin : High = Active, Low = Idle. 00673 // 00674 void DACSTEPoutputValue(int isLogicHigh); 00675 00676 // CODE GENERATOR: extern function declaration UP_slash_DWNboutputValue 00677 //---------------------------------------- 00678 // Assert MAX11043 UP_slash_DWNb pin : High = Up, Low = Down. 00679 // 00680 void UP_slash_DWNboutputValue(int isLogicHigh); 00681 00682 // CODE GENERATOR: extern function declaration EOCinputWaitUntilLow 00683 //---------------------------------------- 00684 // Wait for MAX11043 EOC pin low, indicating end of conversion. 00685 // Required when using any of the InternalClock modes. 00686 // 00687 void EOCinputWaitUntilLow(); 00688 00689 // CODE GENERATOR: extern function declaration EOCinputValue 00690 //---------------------------------------- 00691 // Return the status of the MAX11043 EOC pin. 00692 // 00693 int EOCinputValue(); 00694 00695 // CODE GENERATOR: class member data 00696 private: 00697 // CODE GENERATOR: class member data for SPI interface 00698 // SPI object 00699 SPI &m_spi; 00700 int m_SPI_SCLK_Hz; 00701 int m_SPI_dataMode; 00702 int m_SPI_cs_state; 00703 00704 // Selector pin object 00705 DigitalOut &m_cs_pin; 00706 00707 // CODE GENERATOR: class member data for gpio InputPin pins 00708 // InputPin Name = CONVRUN 00709 // InputPin Description = CONVRUN (digital input). Convert Run. Drive high to start continuous conversions on all 4 channels. The device is idle when 00710 // CONVRUN is low. 00711 // InputPin Function = Configuration 00712 DigitalOut &m_CONVRUN_pin; 00713 // 00714 // InputPin Name = SHDN 00715 // InputPin Description = Shutdown (digital input). Active-High Shutdown Input. Drive high to shut down the MAX11043. 00716 // InputPin Function = Configuration 00717 DigitalOut &m_SHDN_pin; 00718 // 00719 // InputPin Name = DACSTEP 00720 // InputPin Description = DACSTEP (digital input). DAC Step Input. Drive high to move the DAC output in the direction of UP/DWN on the next rising 00721 // edge of the system clock. 00722 // InputPin Function = Configuration 00723 DigitalOut &m_DACSTEP_pin; 00724 // 00725 // InputPin Name = UP/DWN# 00726 // InputPin Description = UP/DWN# (digital input). DAC Step Direction Select. Drive high to step up, drive low to step down when DACSTEP is toggled. 00727 // InputPin Function = Configuration 00728 DigitalOut &m_UP_slash_DWNb_pin; 00729 // 00730 // CODE GENERATOR: class member data for gpio OutputPin pins 00731 // OutputPin Name = EOC 00732 // OutputPin Description = End of Conversion Output. Active-Low End-of-Conversion Indicator. EOC asserts low to indicate that new data is ready. 00733 // OutputPin Function = Event 00734 //-------------------------------------------------- 00735 // MAX11043 ADC Read operations must be synchronized to EOC End Of Conversion 00736 // EOC# asserts low when new data is available. 00737 // Initiate a data read prior to the next rising edge of EOC# or the result is overwritten. 00738 #if MAX11043_EOC_INTERRUPT_POLLING 00739 // MAX11043 EOC End Of Conversion input should be InterruptIn(PinName:EOC_pin).fall(onEOCFallingEdge); 00740 // Workaround using DigitalIn(PinName:EOC_pin) polled to sync with EOC falling edge for ADC reads 00741 // 2020-02-20 MAX11043_EOC_INTERRUPT_POLLING works on MAX32625MBED at 9us conversion rate, with 1us timing margin 00742 DigitalIn &m_EOC_pin; 00743 #else // MAX11043_EOC_INTERRUPT_POLLING 00744 // MAX11043 EOC End Of Conversion input is InterruptIn(PinName:EOC_pin).fall(onEOCFallingEdge); 00745 InterruptIn &m_EOC_pin; 00746 #endif // MAX11043_EOC_INTERRUPT_POLLING 00747 //-------------------------------------------------- 00748 // 00749 00750 // Identifies which IC variant is being used 00751 MAX11043_ic_t m_ic_variant; 00752 00753 public: 00754 00755 // CODE GENERATOR: class member function declarations 00756 //---------------------------------------- 00757 /// Menu item '!' 00758 /// Initialize device 00759 /// @return 1 on success; 0 on failure 00760 uint8_t Init(void); 00761 00762 //---------------------------------------- 00763 /// Return the physical voltage corresponding to conversion result 00764 /// (conversion format is Bipolar mode, 2's complement) 00765 /// Does not perform any offset or gain correction. 00766 /// 00767 /// @pre CONFIG_xxxx_xxxx_xx1x_xxxx_24BIT is 0: 16-bit mode is configured 00768 /// @pre g_MAX11043_device.VRef = Voltage of REF input, in Volts 00769 /// @param[in] value_u24: raw 24-bit MAX11043 code (right justified). 00770 /// @return physical voltage corresponding to MAX11043 code. 00771 /// @test group BIP2C16 ADCVoltageOfCode_16bit(0x7FFF) expect 2.500 within 0.030 Full Scale 00772 /// @test group BIP2C16 ADCVoltageOfCode_16bit(0x7FFF) expect 2.500 Full Scale 00773 /// @test group BIP2C16 ADCVoltageOfCode_16bit(0x6666) expect 2.000 Two Volts 00774 /// @test group BIP2C16 ADCVoltageOfCode_16bit(0x6000) expect 1.875 75% Scale 00775 /// @test group BIP2C16 ADCVoltageOfCode_16bit(0x4000) expect 1.250 Mid Scale 00776 /// @test group BIP2C16 ADCVoltageOfCode_16bit(0x3333) expect 1.000 One Volt 00777 /// @test group BIP2C16 ADCVoltageOfCode_16bit(0x2000) expect 0.625 25% Scale 00778 /// @test group BIP2C16 ADCVoltageOfCode_16bit(0x051e) expect 0.100 100mV 00779 /// @test group BIP2C16 ADCVoltageOfCode_16bit(0x0000) expect 0.00000894069671 Three LSB 00780 /// @test group BIP2C16 ADCVoltageOfCode_16bit(0x0000) expect 0.00000596046447 Two LSB 00781 /// @test group BIP2C16 ADCVoltageOfCode_16bit(0x0000) expect 0.0000029802326 One LSB 00782 /// @test group BIP2C16 ADCVoltageOfCode_16bit(0x0000) expect 0.0 Zero Scale 00783 /// @test group BIP2C16 ADCVoltageOfCode_16bit(0xFFFF) expect -0.0000029802326 Negative One LSB 00784 /// @test group BIP2C16 ADCVoltageOfCode_16bit(0xFFFF) expect -0.0000059604644 Negative Two LSB 00785 /// @test group BIP2C16 ADCVoltageOfCode_16bit(0xFFFF) expect -0.0000089406967 Negative Three LSB 00786 /// @test group BIP2C16 ADCVoltageOfCode_16bit(0xFAE1) expect -0.100 Negative 100mV 00787 /// @test group BIP2C16 ADCVoltageOfCode_16bit(0xE000) expect -0.625 Negative 25% Scale 00788 /// @test group BIP2C16 ADCVoltageOfCode_16bit(0xCCCC) expect -1.000 Negative One Volt 00789 /// @test group BIP2C16 ADCVoltageOfCode_16bit(0xC000) expect -1.250 Negative Mid Scale 00790 /// @test group BIP2C16 ADCVoltageOfCode_16bit(0xA000) expect -1.875 Negative 75% Scale 00791 /// @test group BIP2C16 ADCVoltageOfCode_16bit(0x9999) expect -2.000 Negative Two Volts 00792 /// @test group BIP2C16 ADCVoltageOfCode_16bit(0x8000) expect -2.500 Negative Full Scale 00793 /// @test group BIP2C16 ADCVoltageOfCode_16bit(0x8000) expect -2.500 Negative Full Scale 00794 /// 00795 double ADCVoltageOfCode_16bit(uint32_t value_u16); 00796 00797 //---------------------------------------- 00798 /// Return the physical voltage corresponding to conversion result 00799 /// (conversion format is Bipolar mode, 2's complement) 00800 /// Does not perform any offset or gain correction. 00801 /// 00802 /// @pre CONFIG_xxxx_xxxx_xx1x_xxxx_24BIT is 1: 24-bit mode is configured 00803 /// @pre g_MAX11043_device.VRef = Voltage of REF input, in Volts 00804 /// @param[in] value_u24: raw 24-bit MAX11043 code (right justified). 00805 /// @return physical voltage corresponding to MAX11043 code. 00806 /// @test group BIP2C24 ADCVoltageOfCode_24bit(0x7FFFFF) expect 2.500 within 0.030 Full Scale 00807 /// @test group BIP2C24 ADCVoltageOfCode_24bit(0x7FFFFE) expect 2.500 Full Scale 00808 /// @test group BIP2C24 ADCVoltageOfCode_24bit(0x666666) expect 2.000 Two Volts 00809 /// @test group BIP2C24 ADCVoltageOfCode_24bit(0x600000) expect 1.875 75% Scale 00810 /// @test group BIP2C24 ADCVoltageOfCode_24bit(0x400000) expect 1.250 Mid Scale 00811 /// @test group BIP2C24 ADCVoltageOfCode_24bit(0x333333) expect 1.000 One Volt 00812 /// @test group BIP2C24 ADCVoltageOfCode_24bit(0x200000) expect 0.625 25% Scale 00813 /// @test group BIP2C24 ADCVoltageOfCode_24bit(0x051eb8) expect 0.100 100mV 00814 /// @test group BIP2C24 ADCVoltageOfCode_24bit(0x000003) expect 0.00000894069671 Three LSB 00815 /// @test group BIP2C24 ADCVoltageOfCode_24bit(0x000002) expect 0.00000596046447 Two LSB 00816 /// @test group BIP2C24 ADCVoltageOfCode_24bit(0x000001) expect 0.0000029802326 One LSB 00817 /// @test group BIP2C24 ADCVoltageOfCode_24bit(0x000000) expect 0.0 Zero Scale 00818 /// @test group BIP2C24 ADCVoltageOfCode_24bit(0xFFFFFF) expect -0.0000029802326 Negative One LSB 00819 /// @test group BIP2C24 ADCVoltageOfCode_24bit(0xFFFFFE) expect -0.0000059604644 Negative Two LSB 00820 /// @test group BIP2C24 ADCVoltageOfCode_24bit(0xFFFFFD) expect -0.0000089406967 Negative Three LSB 00821 /// @test group BIP2C24 ADCVoltageOfCode_24bit(0xFAE148) expect -0.100 Negative 100mV 00822 /// @test group BIP2C24 ADCVoltageOfCode_24bit(0xE00000) expect -0.625 Negative 25% Scale 00823 /// @test group BIP2C24 ADCVoltageOfCode_24bit(0xCCCCCD) expect -1.000 Negative One Volt 00824 /// @test group BIP2C24 ADCVoltageOfCode_24bit(0xC00000) expect -1.250 Negative Mid Scale 00825 /// @test group BIP2C24 ADCVoltageOfCode_24bit(0xA00000) expect -1.875 Negative 75% Scale 00826 /// @test group BIP2C24 ADCVoltageOfCode_24bit(0x99999A) expect -2.000 Negative Two Volts 00827 /// @test group BIP2C24 ADCVoltageOfCode_24bit(0x800001) expect -2.500 Negative Full Scale 00828 /// @test group BIP2C24 ADCVoltageOfCode_24bit(0x800000) expect -2.500 Negative Full Scale 00829 /// 00830 double ADCVoltageOfCode_24bit(uint32_t value_u24); 00831 00832 // CODE GENERATOR: looks like this is a register access function because 'regAdd' 00833 // CODE GENERATOR: looks like this is a 'write' register access function 00834 //---------------------------------------- 00835 /// Write a MAX11043 register. 00836 /// 00837 /// CMDOP_1aaa_aaaa_ReadRegister bit is cleared 0 indicating a write operation. 00838 /// 00839 /// MAX11043 register length can be determined by function RegSize. 00840 /// 00841 /// For 8-bit register size: 00842 /// 00843 /// SPI 16-bit transfer 00844 /// 00845 /// SPI MOSI = 0aaa_aaaa_dddd_dddd 00846 /// 00847 /// SPI MISO = xxxx_xxxx_xxxx_xxxx 00848 /// 00849 /// For 16-bit register size: 00850 /// 00851 /// SPI 24-bit or 32-bit transfer 00852 /// 00853 /// SPI MOSI = 0aaa_aaaa_dddd_dddd_dddd_dddd 00854 /// 00855 /// SPI MISO = xxxx_xxxx_xxxx_xxxx_xxxx_xxxx 00856 /// 00857 /// For 24-bit register size: 00858 /// 00859 /// SPI 32-bit transfer 00860 /// 00861 /// SPI MOSI = 0aaa_aaaa_dddd_dddd_dddd_dddd_dddd_dddd 00862 /// 00863 /// SPI MISO = xxxx_xxxx_xxxx_xxxx_xxxx_xxxx_xxxx_xxxx 00864 /// 00865 /// @return 1 on success; 0 on failure 00866 uint8_t RegWrite(MAX11043_CMD_enum_t commandByte, uint32_t regData); 00867 00868 // CODE GENERATOR: looks like this is a register access function because 'regAdd' 00869 // CODE GENERATOR: looks like this is a 'read' register access function 00870 //---------------------------------------- 00871 /// Read an 8-bit MAX11043 register 00872 /// 00873 /// CMDOP_1aaa_aaaa_ReadRegister bit is set 1 indicating a read operation. 00874 /// 00875 /// MAX11043 register length can be determined by function RegSize. 00876 /// 00877 /// For 8-bit register size: 00878 /// 00879 /// SPI 16-bit transfer 00880 /// 00881 /// SPI MOSI = 1aaa_aaaa_0000_0000 00882 /// 00883 /// SPI MISO = xxxx_xxxx_dddd_dddd 00884 /// 00885 /// For 16-bit register size: 00886 /// 00887 /// SPI 24-bit or 32-bit transfer 00888 /// 00889 /// SPI MOSI = 1aaa_aaaa_0000_0000_0000_0000 00890 /// 00891 /// SPI MISO = xxxx_xxxx_dddd_dddd_dddd_dddd 00892 /// 00893 /// For 24-bit register size: 00894 /// 00895 /// SPI 32-bit transfer 00896 /// 00897 /// SPI MOSI = 1aaa_aaaa_0000_0000_0000_0000_0000_0000 00898 /// 00899 /// SPI MISO = xxxx_xxxx_dddd_dddd_dddd_dddd_dddd_dddd 00900 /// 00901 /// 00902 /// @return 1 on success; 0 on failure 00903 uint8_t RegRead(MAX11043_CMD_enum_t commandByte, uint32_t* ptrRegData); 00904 00905 // CODE GENERATOR: looks like this is a register access function because 'regAdd' 00906 // CODE GENERATOR: looks like this is a 'size' register access function 00907 //---------------------------------------- 00908 /// Return the size of a MAX11043 register 00909 /// 00910 /// @return 8 for 8-bit, 16 for 16-bit, 24 for 24-bit, else 0 for undefined register size 00911 uint8_t RegSize(MAX11043_CMD_enum_t commandByte); 00912 00913 // CODE GENERATOR: looks like this is a register access function because 'regAdd' 00914 //---------------------------------------- 00915 /// Decode operation from commandByte 00916 /// 00917 /// @return operation such as idle, read register, write register, etc. 00918 MAX11043::MAX11043_CMDOP_enum_t DecodeCommand(MAX11043_CMD_enum_t commandByte); 00919 00920 // CODE GENERATOR: looks like this is a register access function because 'regAdd' 00921 //---------------------------------------- 00922 /// Return the address field of a MAX11043 register 00923 /// 00924 /// @return register address field as given in datasheet 00925 uint8_t RegAddrOfCommand(MAX11043_CMD_enum_t commandByte); 00926 00927 // CODE GENERATOR: looks like this is a register access function because 'regAdd' 00928 // CODE GENERATOR: looks like this is a 'read' register access function 00929 //---------------------------------------- 00930 /// Test whether a command byte is a register read command 00931 /// 00932 /// @return true if command byte is a register read command 00933 uint8_t IsRegReadCommand(MAX11043_CMD_enum_t commandByte); 00934 00935 // CODE GENERATOR: looks like this is a register access function because 'regAdd' 00936 // CODE GENERATOR: looks like this is a 'name' register access function 00937 //---------------------------------------- 00938 /// Return the name of a MAX11043 register 00939 /// 00940 /// @return null-terminated constant C string containing register name or empty string 00941 const char* RegName(MAX11043_CMD_enum_t commandByte); 00942 00943 //---------------------------------------- 00944 /// Menu item '$' -> adca, adcb, adcc, adcd 00945 /// Read ADCabcd 00946 /// 00947 /// @return 1 on success; 0 on failure 00948 uint8_t Read_ADCabcd(void); 00949 00950 //---------------------------------------- 00951 /// Menu item 'GA' 00952 /// Write AGain register 00953 /// 00954 /// @param[in] gain 2's complement, 0x800=0.25V/V, 0x1000=0.5V/V, 0x2000=1V/V, 0x4000=2V/V, default=0x2000 00955 /// 00956 /// @return 1 on success; 0 on failure 00957 uint8_t Write_AGain(uint32_t gain); 00958 00959 //---------------------------------------- 00960 /// Menu item 'XD' 00961 /// Example configuration. 00962 /// Slowest conversion rate 1:6 = 9us, 00963 /// Bypass PGA and filters, Gain=1V/V, 00964 /// AOUT = 2.0V 00965 /// 00966 void Configure_Demo(void); 00967 00968 //---------------------------------------- 00969 /// Menu item 'XX' 00970 /// 00971 /// @return 1 on success; 0 on failure 00972 uint8_t Configure_XXXXX(uint8_t linef, uint8_t rate); 00973 00974 //---------------------------------------- 00975 /// Menu item 'XY' 00976 /// 00977 /// @return 1 on success; 0 on failure 00978 uint8_t Configure_XXXXY(uint8_t linef, uint8_t rate); 00979 00980 }; // end of class MAX11043 00981 00982 #endif // __MAX11043_H__ 00983 00984 // End of file
Generated on Wed Jul 13 2022 22:23:56 by
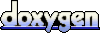