
Testprogram
Dependencies: mbed MPU6050 DS1820
main.cpp
00001 // Diese Software testet die verschiedenen Funktionen des NUClight V3 Boards 00002 // BULME Graz, 00003 // by F. Wolf 20.10.2019 00004 /* 00005 PIN-OUT-NUClight 00006 NUCLEO-L432KC 00007 RGB-rot D1|-------| VIn 00008 RGB-gruen D0| | GND 00009 NRST| | RST 00010 GND| | 5V0 00011 LED1 <-D2| | A7 00012 LED2 <-D3| | A6 -> LED7 00013 SDA (I2C) (MPU6050 gyro) <- D4| | A5 00014 SCL (I2C) (MPU6050 gyro) <- D5| | A4 00015 LED3 <-D6| | A3 -> POTI 00016 nc D7| | A2 -> Taster 00017 nc D8| | A1 -> Taster 00018 LED4 D9| | A0 -> DS18B20 00019 RGB-blau <- D10| | ARF 00020 LED5 <- D11| | 3V0 00021 LED6 <- D12|-------| D13 -> LED8 00022 00023 RGB LED aktiv hight (1) 00024 */ 00025 #include <mbed.h> 00026 #include "MPU6050.h" 00027 #include "DS1820.h" 00028 00029 //****** Definitionen ********************** 00030 Serial pc(SERIAL_TX,SERIAL_RX); //nucleo 00031 00032 // Definition der Taster (Switches) 00033 InterruptIn sw1(A1); 00034 InterruptIn sw2(A2); 00035 AnalogIn ain(A3); 00036 00037 // Definition der 8 LED's 00038 DigitalOut led1(D2); 00039 DigitalOut led2(D3); 00040 DigitalOut led3(D6); 00041 DigitalOut led4(D9); 00042 DigitalOut led5(D11); 00043 DigitalOut led6(D12); 00044 DigitalOut led7(A6); 00045 DigitalOut led8(D13); // on Board LED 00046 00047 // RGB LED 00048 PwmOut RGBg(D0); // gruen LED 00049 PwmOut RGBr(D1); // rote LED 00050 PwmOut RGBb(D10); // blaue LED 00051 00052 // DS1820 Temperatursensor 00053 #define DATA_PIN A0 // DS18B20 00054 DS1820 ds1820(DATA_PIN); // create a ds1820 sensor 00055 00056 // GY-521 Module MPU-6050 3-Achsen-Gyroskop + 3 Achsen Accelerometre 00057 MPU6050 ark(D4,D5); // MPU6050(PinName sda, PinName scl); 00058 00059 // ********** Deklarationen ************** 00060 void RGBtest(); 00061 void LEDtest(); 00062 void LEDonoff(); 00063 00064 // variable 00065 int a; 00066 int num_devices = 0; 00067 00068 // ********** Hauptprogramm ************** 00069 int main() 00070 { 00071 pc.printf("******** TEST-SW *************\r\n"); //HTerm Welcome Message 00072 pc.printf("TEST-SW Welcome to NUClight V3 \r\n"); //HTerm Welcome Message 00073 pc.printf("*******************************\r\n"); //HTerm Welcome Message 00074 while(1) 00075 { 00076 // attach the address of the flip function to the rising edge 00077 sw1.fall(&RGBtest); //RGB-Test Unterprogramm aufrufen 00078 sw2.fall(&LEDonoff); //LED ON OFF - Test Unterprogramm aufrufen 00079 pc.printf("percentage: %3.0f%%\r\n", ain.read()*100.0f); 00080 RGBr.period_ms(20.0f); // 20 mili second period 00081 RGBr.pulsewidth_ms(ain.read()*10.0f); // 5 mili second pulse (on) 00082 00083 wait(1); 00084 // *** MPU6050 (gyro) TEST 00085 // reading Temprature 00086 float temp = ark.getTemp(); 00087 00088 pc.printf("MPU6050-temprature = %0.2f ^C\r\n",temp); 00089 pc.printf("__________________\r\n"); 00090 //reading Gyrometer readings 00091 float gyro[3]; 00092 ark.getGyro(gyro); 00093 pc.printf("Gyroscope\r\n"); 00094 pc.printf("__________________\r\n"); 00095 pc.printf("Gyro0=%0.3f, Gyro1=%0.3f, Gyro2=%0.3f\r\n",gyro[0],gyro[1],gyro[2]); 00096 //reading Acclerometer readings 00097 float acce[3]; 00098 ark.getAccelero(acce); 00099 pc.printf("Accelerometer\r\n"); 00100 pc.printf("__________________\r\n"); 00101 pc.printf("Acce0=%0.3f, Acce1=%0.3f, Acce2=%0.3f\r\n",acce[0],acce[1],acce[2]); 00102 wait(1); //wait 1000ms 00103 00104 //DS18B20 00105 if(ds1820.begin()) 00106 { 00107 ds1820.startConversion(); // start temperature conversion 00108 wait(1.0); // let DS1820 complete the temperature conversion 00109 pc.printf("temprature DS1820 = %3.1f ^C\r\n", ds1820.read(),248); // read temperature 00110 pc.printf("__________________\r\n"); 00111 } 00112 else 00113 { 00114 pc.printf("No DS1820 sensor found!\r\n"); 00115 } 00116 } 00117 } 00118 00119 00120 // ********** Funktionen ************** 00121 // TEST-RGB 00122 //------------------------------------ 00123 void RGBtest() 00124 { 00125 pc.printf("RGBTEST\r\n"); //HTerm Ausgabe 00126 LEDtest(); 00127 for (int t=1; t<15;t++) 00128 { 00129 RGBr.period_ms(20.0f); // 20 mili second period 00130 RGBr.pulsewidth_ms(t); // 5 mili second pulse (on) 00131 wait(0.3); 00132 } 00133 00134 RGBr.pulsewidth_ms(0); // 5 mili second pulse (on) 00135 for (int t=1; t<15;t++) 00136 { 00137 RGBg.period_ms(20.0f); // 20 mili second period 00138 RGBg.pulsewidth_ms(t); // 5 mili second pulse (on) 00139 wait(0.3); 00140 } 00141 00142 RGBr.pulsewidth_ms(0); // 5 mili second pulse (on) 00143 RGBg.pulsewidth_ms(0); // 5 mili second pulse (on) 00144 for (int t=1; t<15;t++) 00145 { 00146 RGBb.period_ms(20.0f); // 20 mili second period 00147 RGBb.pulsewidth_ms(t); // 5 mili second pulse (on) 00148 wait(0.3); 00149 } 00150 wait(1); 00151 RGBr.pulsewidth_ms(0); // RGB-LED off 00152 RGBg.pulsewidth_ms(0); // 00153 RGBb.pulsewidth_ms(0); // 00154 00155 int rr=(rand()%15); 00156 int rg=(rand()%15); 00157 int rb=(rand()%15); 00158 00159 RGBr.period_ms(20.0f); // 20 mili second period 00160 RGBr.pulsewidth_ms(rr); // 5 mili second pulse (on) 00161 00162 RGBg.period_ms(20.0f); // 20 mili second period 00163 RGBg.pulsewidth_ms(rg); // 5 mili second pulse (on) 00164 00165 RGBb.period_ms(20.0f); // 20 mili second period 00166 RGBb.pulsewidth_ms(rb); // 5 mili second pulse (on) 00167 00168 wait(1); 00169 RGBr.pulsewidth_ms(0); // RGB-LED off 00170 RGBg.pulsewidth_ms(0); // 00171 RGBb.pulsewidth_ms(0); // 00172 00173 } 00174 00175 // TEST-LED 00176 //------------------------------------ 00177 void LEDtest() 00178 { 00179 pc.printf("LAUFLICHT UEBER 8 LED (5-mal) \r\n"); //HTerm Ausgabe 00180 a=0; 00181 while (a<5) { 00182 led1=1; //Led1 einschalten 00183 wait(0.2); 00184 led1=0; //Led1 ausschalten 00185 00186 led2=1; //Led2 einschalten 00187 wait(0.2); 00188 led2=0; //Led2 ausschalten 00189 00190 led3=1; //Led3 einschalten 00191 wait(0.2); 00192 led3=0; //Led3 ausschalten 00193 00194 led4=1; //Led1 einschalten 00195 wait(0.2); 00196 led4=0; //Led1 ausschalten 00197 00198 led5=1; //Led2 einschalten 00199 wait(0.2); 00200 led5=0; //Led2 ausschalten 00201 00202 led6=1; //Led3 einschalten 00203 wait(0.2); 00204 led6=0; //Led3 ausschalten 00205 00206 led7=1; //Led2 einschalten 00207 wait(0.2); 00208 led7=0; //Led2 ausschalten 00209 00210 led8=1; //Led3 einschalten 00211 wait(0.2); 00212 led8=0; //Led3 ausschalten 00213 a++; 00214 } 00215 } 00216 00217 // TEST-LED-on-off 00218 //------------------------------------ 00219 void LEDonoff() 00220 { 00221 led1=!led1; //Led1 einschalten 00222 led2=!led2; //Led1 einschalten 00223 led3=!led3; //Led1 einschalten 00224 led4=!led4; //Led1 einschalten 00225 led5=!led5; //Led1 einschalten 00226 led6=!led6; //Led1 einschalten 00227 led7=!led7; //Led1 einschalten 00228 led8=!led8; //Led1 einschalten 00229 } 00230 /******************** ENDE ***********************/
Generated on Fri Jul 15 2022 02:06:15 by
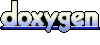