
Modbus-RS485
Dependencies: Modbus nvt_rs485
main.cpp
00001 /* 00002 * The program is a sample code. 00003 * It needs run with some NuMaker-PFM-NUC472 boards. 00004 * 00005 * Please remeber to modify global definition to enable RS485 port on board. 00006 * Modify '//#define DEF_RS485_PORT 1' to '#define DEF_RS485_PORT 1' 00007 */ 00008 00009 /* ----------------------- System includes --------------------------------*/ 00010 #include "mbed.h" 00011 #include "rtos.h" 00012 /*----------------------- Modbus includes ----------------------------------*/ 00013 #include "mb.h" 00014 #include "mbport.h" 00015 00016 /* ----------------------- Defines ------------------------------------------*/ 00017 // Sharing buffer index 00018 enum { 00019 eData_MBInCounter, 00020 eData_MBOutCounter, 00021 eData_MBError, 00022 eData_DI, 00023 eData_DATA, 00024 eData_Cnt 00025 } E_DATA_TYPE; 00026 00027 #define REG_INPUT_START 1 00028 #define REG_INPUT_NREGS eData_Cnt 00029 /* ----------------------- Static variables ---------------------------------*/ 00030 static USHORT usRegInputStart = REG_INPUT_START; 00031 static USHORT usRegInputBuf[REG_INPUT_NREGS]; 00032 00033 DigitalOut led1(LED1); // For temperature worker. 00034 DigitalOut led2(LED2); // For Modbus worker. 00035 DigitalOut led3(LED3); // For Holder CB 00036 00037 #define DEF_PIN_NUM 6 00038 #if defined(TARGET_NUMAKER_PFM_NUC472) // for NUC472 board 00039 DigitalIn DipSwitch[DEF_PIN_NUM] = { PG_1, PG_2, PF_9, PF_10, PC_10, PC_11 } ; 00040 #elif defined(TARGET_NUMAKER_PFM_M453) // for M453 board 00041 DigitalIn DipSwitch[DEF_PIN_NUM] = { PD_6, PD_1, PC_6, PC_7, PC_11, PC_12 } ; 00042 #endif 00043 00044 unsigned short GetValueOnDipSwitch() 00045 { 00046 int i=0; 00047 unsigned short usDipValue = 0x0; 00048 for ( i=0; i<DEF_PIN_NUM ; i++) 00049 usDipValue |= DipSwitch[i].read() << i; 00050 usDipValue = (~usDipValue) & 0x003F; 00051 return usDipValue; 00052 } 00053 00054 void worker_uart(void const *args) 00055 { 00056 int counter=0; 00057 // For UART-SERIAL Tx/Rx Service. 00058 while (true) 00059 { 00060 //xMBPortSerialPolling(); 00061 if ( counter > 10000 ) 00062 { 00063 led2 = !led2; 00064 counter=0; 00065 } 00066 counter++; 00067 } 00068 } 00069 00070 /* ----------------------- Start implementation -----------------------------*/ 00071 int 00072 main( void ) 00073 { 00074 eMBErrorCode eStatus; 00075 //Thread uart_thread(worker_uart); 00076 unsigned short usSlaveID=GetValueOnDipSwitch(); 00077 00078 // Initialise some registers 00079 for (int i=0; i<REG_INPUT_NREGS; i++) 00080 usRegInputBuf[i] = 0x0; 00081 00082 printf("We will set modbus slave ID-%d(0x%x) for the device.\r\n", usSlaveID, usSlaveID ); 00083 00084 /* Enable the Modbus Protocol Stack. */ 00085 if ( (eStatus = eMBInit( MB_RTU, usSlaveID, 0, 115200, MB_PAR_NONE )) != MB_ENOERR ) 00086 goto FAIL_MB; 00087 else if ( (eStatus = eMBEnable( ) ) != MB_ENOERR ) 00088 goto FAIL_MB_1; 00089 else { 00090 for( ;; ) 00091 { 00092 xMBPortSerialPolling(); 00093 if ( eMBPoll( ) != MB_ENOERR ) break; 00094 } 00095 } 00096 00097 FAIL_MB_1: 00098 eMBClose(); 00099 00100 FAIL_MB: 00101 for( ;; ) 00102 { 00103 led2 = !led2; 00104 Thread::wait(200); 00105 } 00106 } 00107 00108 00109 00110 00111 eMBErrorCode 00112 eMBRegInputCB( UCHAR * pucRegBuffer, USHORT usAddress, USHORT usNRegs ) 00113 { 00114 eMBErrorCode eStatus = MB_ENOERR; 00115 int iRegIndex; 00116 00117 if( ( usAddress >= REG_INPUT_START ) 00118 && ( usAddress + usNRegs <= REG_INPUT_START + REG_INPUT_NREGS ) ) 00119 { 00120 iRegIndex = ( int )( usAddress - usRegInputStart ); 00121 while( usNRegs > 0 ) 00122 { 00123 *pucRegBuffer++ = 00124 ( unsigned char )( usRegInputBuf[iRegIndex] >> 8 ); 00125 *pucRegBuffer++ = 00126 ( unsigned char )( usRegInputBuf[iRegIndex] & 0xFF ); 00127 iRegIndex++; 00128 usNRegs--; 00129 } 00130 } 00131 else 00132 { 00133 eStatus = MB_ENOREG; 00134 } 00135 00136 return eStatus; 00137 } 00138 00139 eMBErrorCode 00140 eMBRegHoldingCB( UCHAR * pucRegBuffer, USHORT usAddress, USHORT usNRegs, eMBRegisterMode eMode ) 00141 { 00142 eMBErrorCode eStatus = MB_ENOERR; 00143 int iRegIndex; 00144 00145 usRegInputBuf[eData_MBInCounter]++; 00146 usRegInputBuf[eData_MBOutCounter]++; 00147 00148 if (eMode == MB_REG_READ) 00149 { 00150 usRegInputBuf[eData_DI] = GetValueOnDipSwitch(); 00151 00152 if( ( usAddress >= REG_INPUT_START ) 00153 && ( usAddress + usNRegs <= REG_INPUT_START + REG_INPUT_NREGS ) ) 00154 { 00155 iRegIndex = ( int )( usAddress - usRegInputStart ); 00156 while( usNRegs > 0 ) 00157 { 00158 *pucRegBuffer++ = 00159 ( unsigned char )( usRegInputBuf[iRegIndex] >> 8 ); 00160 *pucRegBuffer++ = 00161 ( unsigned char )( usRegInputBuf[iRegIndex] & 0xFF ); 00162 iRegIndex++; 00163 usNRegs--; 00164 } 00165 } 00166 } 00167 00168 if (eMode == MB_REG_WRITE) 00169 { 00170 if( ( usAddress >= REG_INPUT_START ) 00171 && ( usAddress + usNRegs <= REG_INPUT_START + REG_INPUT_NREGS ) ) 00172 { 00173 iRegIndex = ( int )( usAddress - usRegInputStart ); 00174 while( usNRegs > 0 ) 00175 { 00176 usRegInputBuf[iRegIndex] = ((unsigned int) *pucRegBuffer << 8) | ((unsigned int) *(pucRegBuffer+1)); 00177 pucRegBuffer+=2; 00178 iRegIndex++; 00179 usNRegs--; 00180 } 00181 } 00182 } 00183 00184 led3=!led3; 00185 00186 return eStatus; 00187 } 00188 00189 00190 eMBErrorCode 00191 eMBRegCoilsCB( UCHAR * pucRegBuffer, USHORT usAddress, USHORT usNCoils, 00192 eMBRegisterMode eMode ) 00193 { 00194 return MB_ENOREG; 00195 } 00196 00197 eMBErrorCode 00198 eMBRegDiscreteCB( UCHAR * pucRegBuffer, USHORT usAddress, USHORT usNDiscrete ) 00199 { 00200 return MB_ENOREG; 00201 }
Generated on Thu Jul 14 2022 06:06:31 by
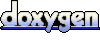